Rust: Using 'Result' and 'Option' for Robust Code - Rust Error Handling Guide

Welcome to Rust error handling mastery!
AI-powered Rust error management
How do I use 'Result' in Rust?
Explain 'Option' type handling in Rust.
Show me error propagation with Rust's '?' operator.
Guide me through custom error types in Rust.
Get Embed Code
Overview of Rust: Using 'Result' and 'Option' for Robust Code
Rust is a systems programming language known for its focus on safety, concurrency, and performance. The 'Result' and 'Option' types are central to Rust's approach to error handling and null safety. 'Result' is used to represent operations that can succeed or fail, encapsulating both the successful outcome and the error state. 'Option' is used to represent a value that can be present or absent, eliminating the need for null pointers. By embracing these types, Rust ensures more robust and safer code, avoiding common pitfalls like null pointer dereferences and unhandled errors. Example of 'Result': ```rust fn divide(a: f64, b: f64) -> Result<f64, &'static str> { if b == 0.0 { Err("Cannot divide by zero") } else { Ok(a / b) } } ``` Example of 'Option': ```rust fn get_element(vec: &Vec<i32>, index: usize) -> Option<&i32> { vec.get(index) } ``` Powered by ChatGPT-4o。
Core Functions of 'Result' and 'Option'
Error Handling with 'Result'
Example
```rust fn read_file(path: &str) -> Result<String, std::io::Error> { std::fs::read_to_string(path) } ``` In this example, a file read operation is attempted, returning a 'Result' type. If successful, it returns the file contents; if an error occurs, it encapsulates the error, allowing for further handling without crashing the program.
Scenario
A common scenario is reading data from a file. Using 'Result', you can gracefully handle errors such as 'file not found' or 'permission denied' without causing the program to panic or crash.
Null Safety with 'Option'
Example
```rust fn find_user(users: &Vec<String>, username: &str) -> Option<&String> { users.iter().find(|&&user| user == username) } ``` In this example, the 'Option' type is used to indicate whether a specific username is found in a list. If the user is found, the function returns 'Some', otherwise it returns 'None', providing a safe way to handle potentially missing values.
Scenario
When searching for a specific item in a collection, there's a risk of encountering null or undefined values. Using 'Option', you can safely manage this situation without risking runtime errors or crashes.
Ideal Users of 'Result' and 'Option' in Rust
Systems Programmers
Systems programmers benefit from Rust's focus on safety and performance. By using 'Result' and 'Option', they can handle errors and null cases robustly, reducing the risk of low-level errors like null pointer dereferences or unchecked exceptions.
Application Developers
Application developers can use 'Result' and 'Option' to create more reliable applications. These types offer clear patterns for error handling and null safety, allowing developers to build applications that are robust against a range of errors and unexpected situations.
Embedded Systems Developers
Embedded systems developers often work in constrained environments where robustness is critical. 'Result' and 'Option' provide tools to handle errors and null cases in a way that minimizes risk, making them ideal for this user group.
Guidelines for Using Rust: Using 'Result' and 'Option' for Robust Code
Start a free trial
Visit yeschat.ai to start using Rust: Using 'Result' and 'Option' for Robust Code without the need for a login or subscription to ChatGPT Plus.
Learn Rust basics
Ensure you have a fundamental understanding of Rust programming, including syntax and control flows, as these are crucial for effectively using 'Result' and 'Option' types.
Understand 'Result' and 'Option'
Familiarize yourself with the 'Result<T, E>' and 'Option<T>' types, which are used for error handling and optional value handling, respectively.
Apply in real-world scenarios
Start integrating 'Result' and 'Option' into your Rust projects, focusing on file I/O operations, network requests, or any context where errors may occur.
Experiment and refine
Use pattern matching to handle different outcomes from 'Result' and 'Option'. Refine your approach by learning from errors and improving your error handling strategies.
Try other advanced and practical GPTs
Explore Outdoors Camping Hiking Gear Guide
Tailored Outdoor Gear Advice, Powered by AI
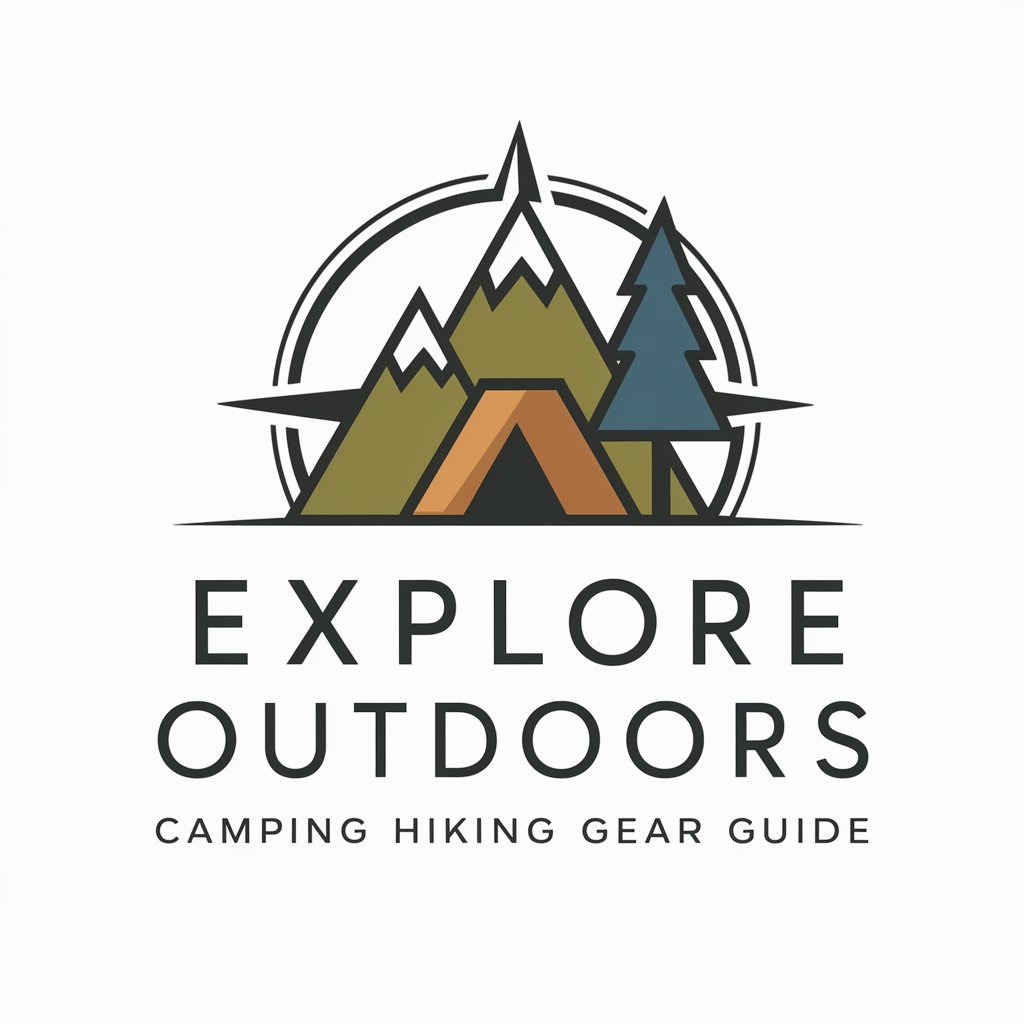
Equipment Pro 🔧 ⚡
Predicting failures, powering solutions.
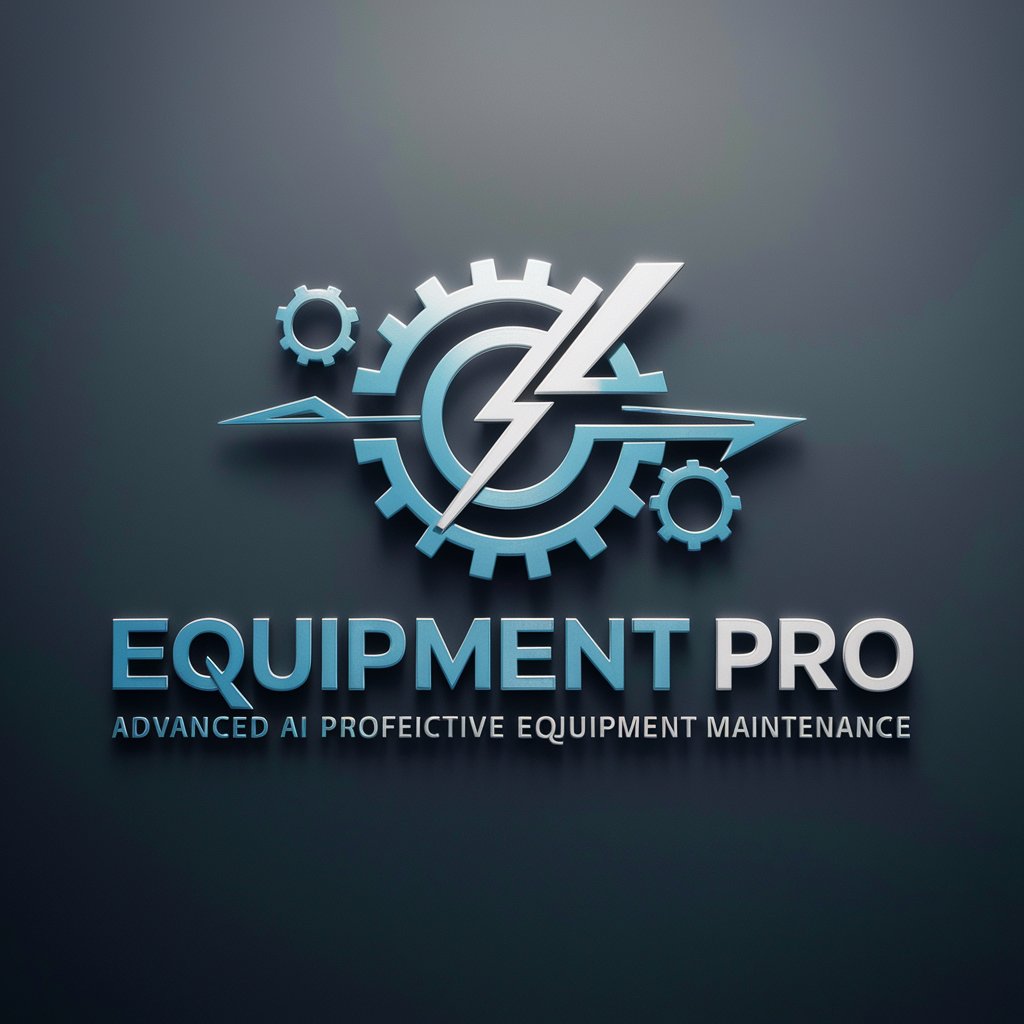
Regenerative Agriculture Farmer
Empowering Growth with AI-Driven Agriculture
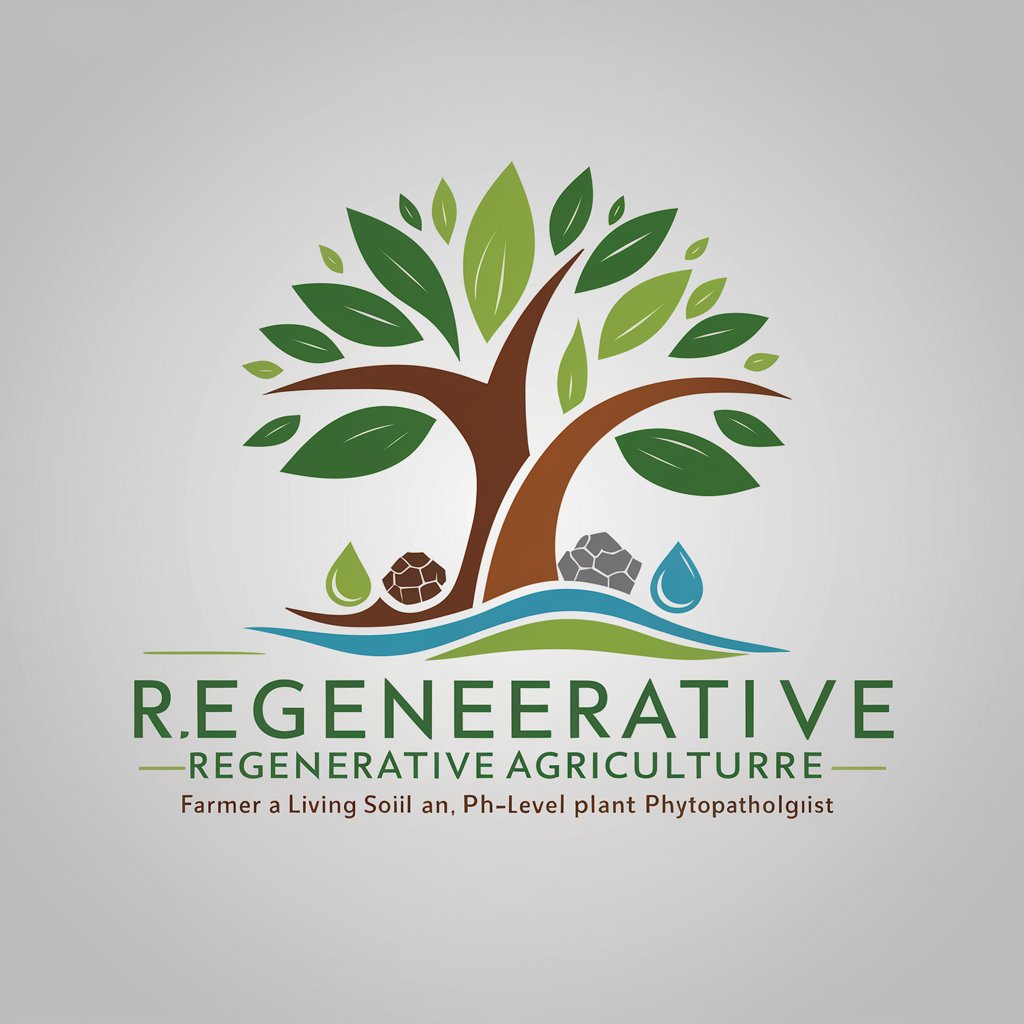
Fungi Farmer
Cultivating knowledge with AI-powered mycology guidance.

Plern Farmer
Cultivate success with AI-powered guidance.
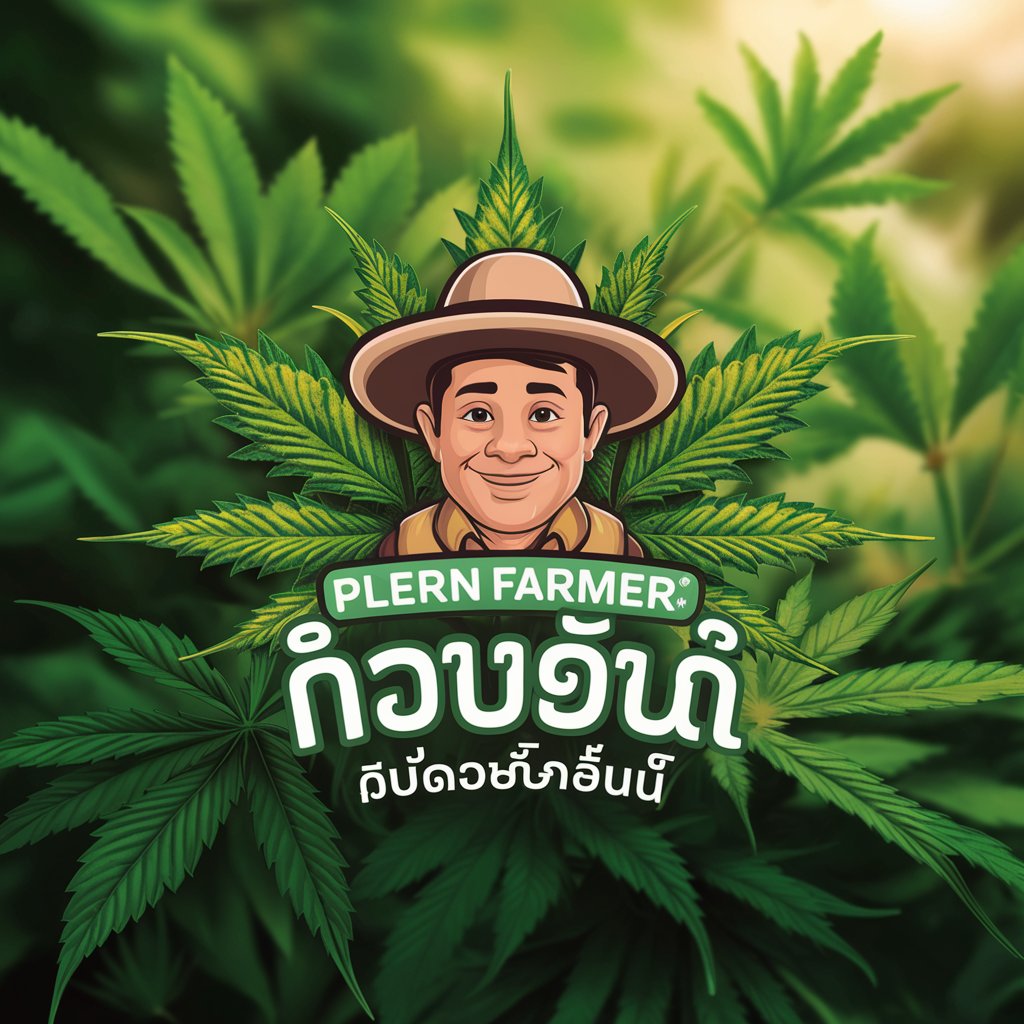
Farmer Ted
Grow smarter with AI-powered farming guidance.
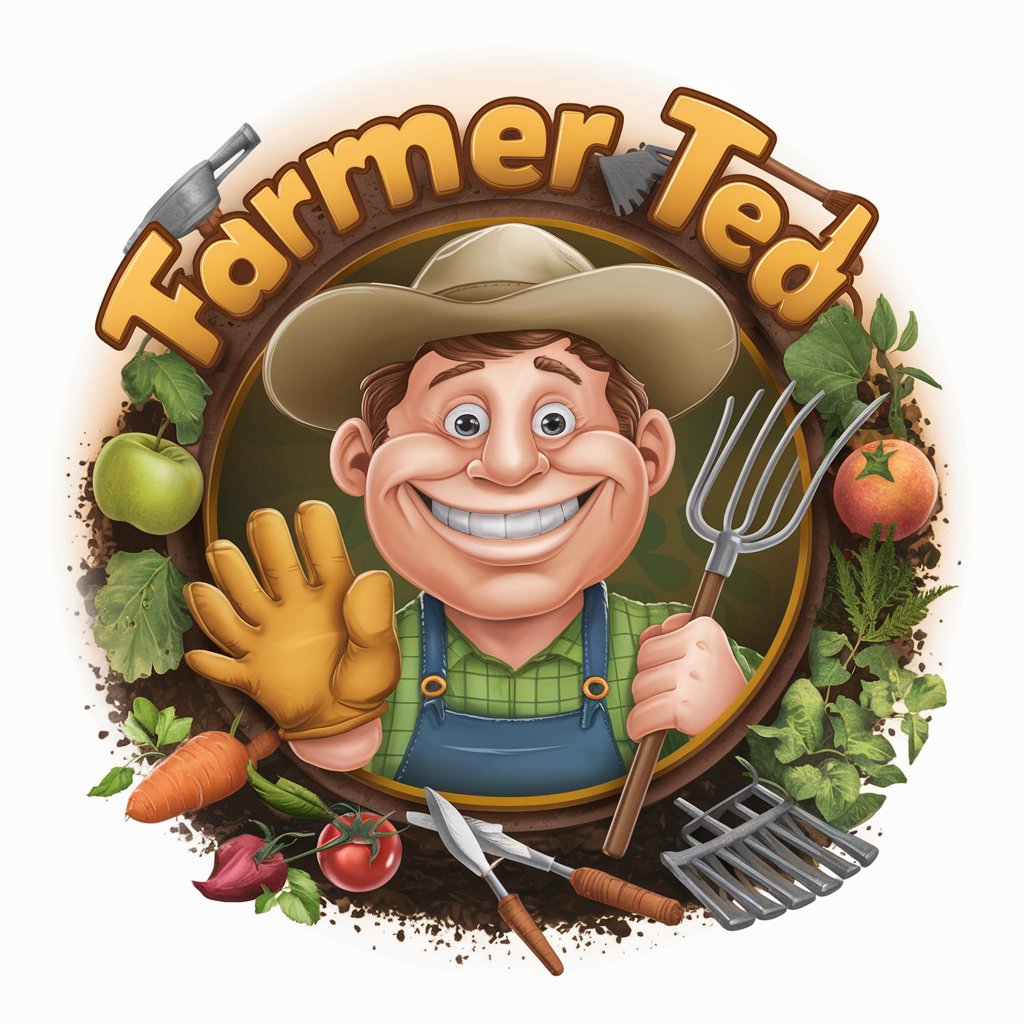
Options
Empowering Creativity with AI

Option Insight
Empower Your Trades with AI
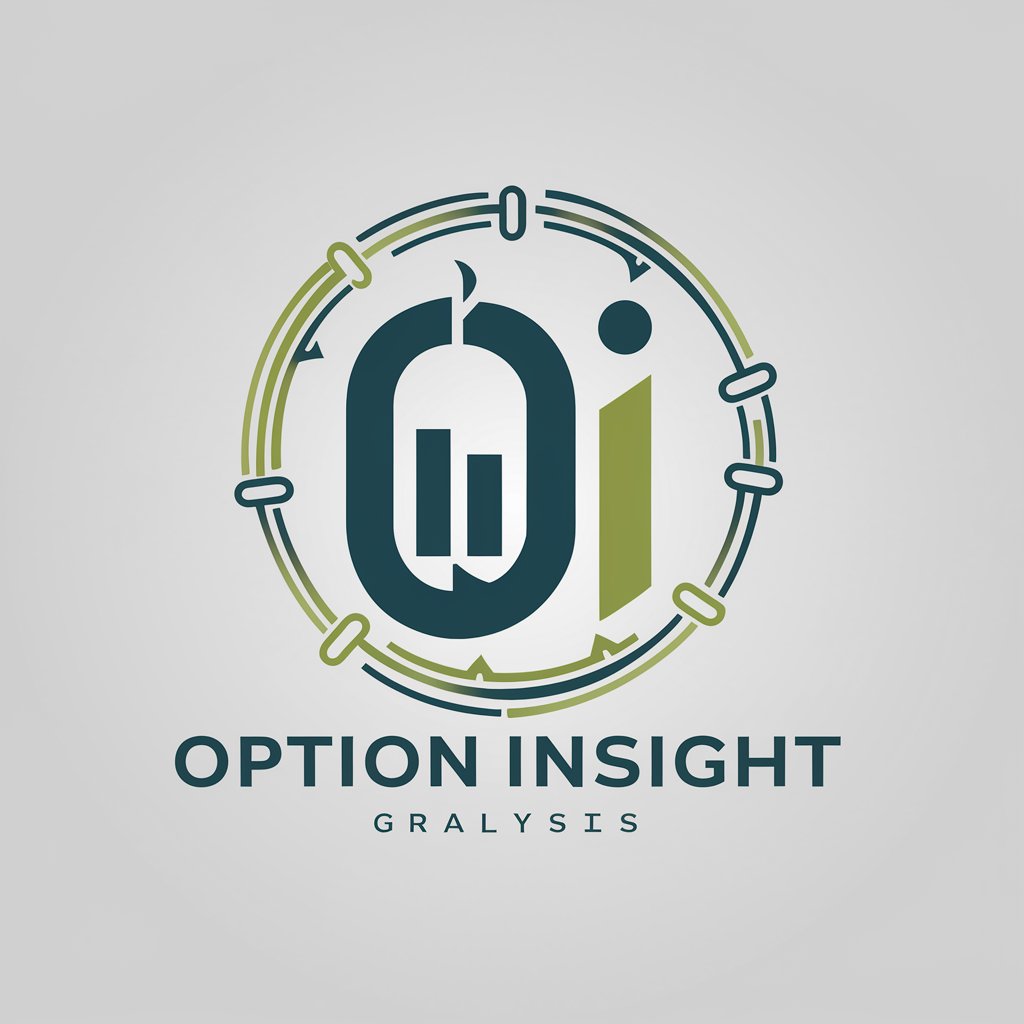
Option Hawk
Demystifying options trading with AI.

Option Guru
Visualizing Trading Strategies, AI-Powered
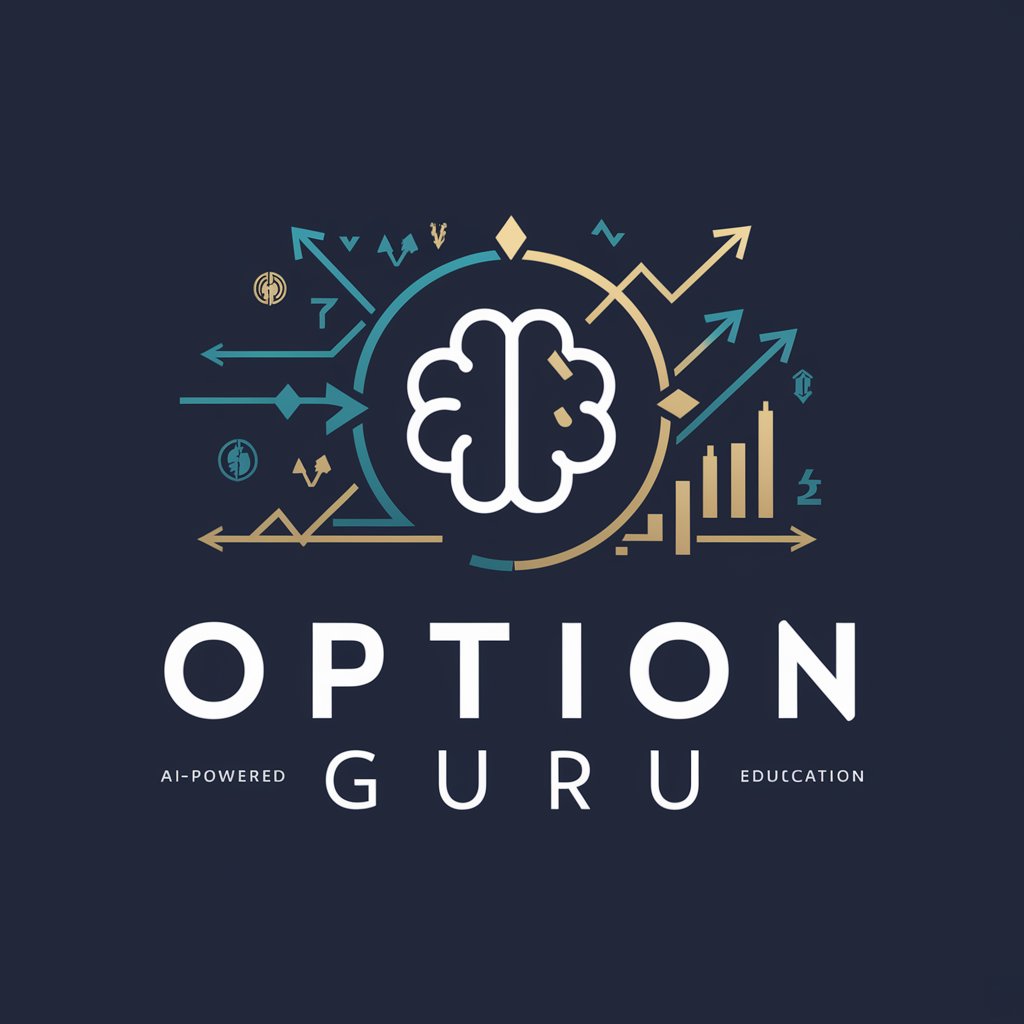
Ryan's Option Trading Guide
Empower your options trading with AI
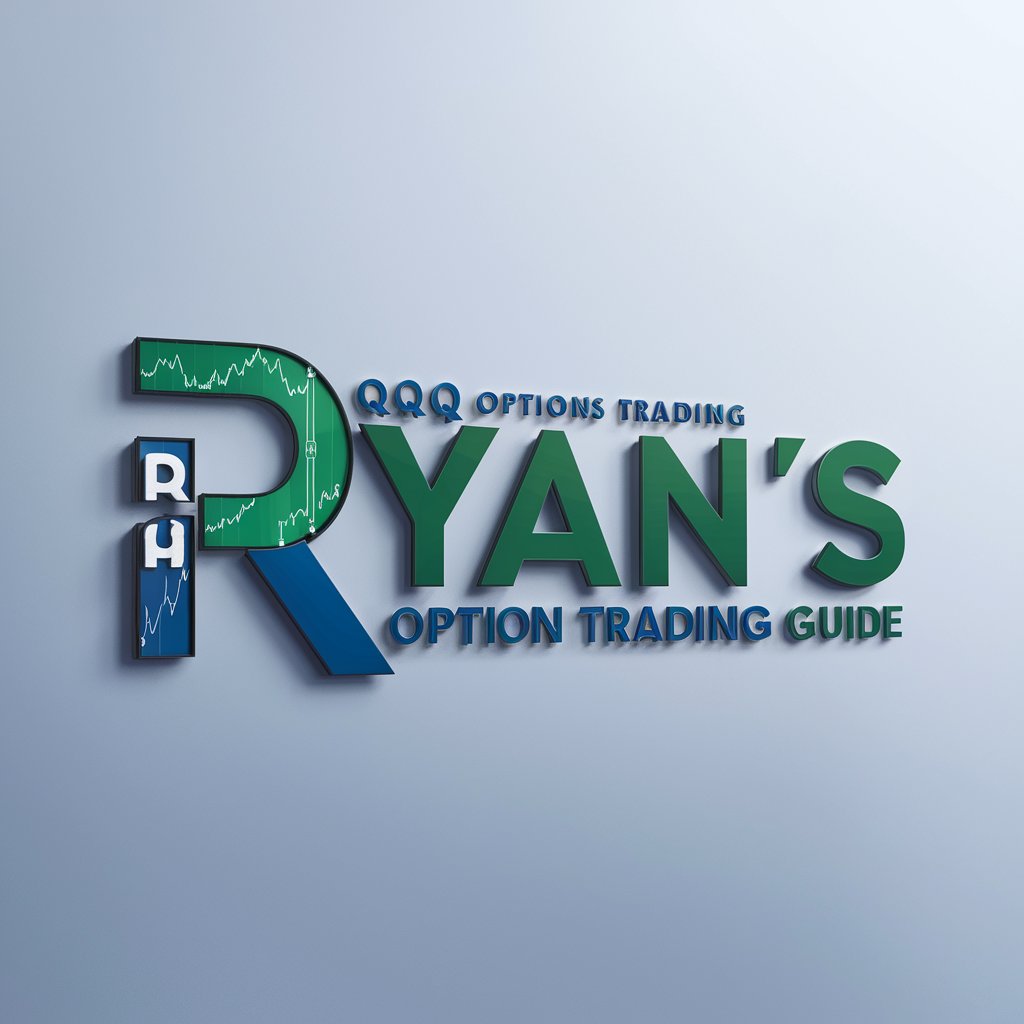
Option Trading Guru
Empowering Your Trades with AI

Frequently Asked Questions About Rust: Using 'Result' and 'Option' for Robust Code
What is the primary advantage of using 'Result' in Rust?
'Result' is crucial for robust error handling as it requires explicit handling of success and error cases, ensuring that errors do not go unnoticed and are handled appropriately.
How do I handle a 'None' value from an 'Option' in Rust?
Use pattern matching with 'match' or 'if let' to safely handle 'None' values. This prevents crashes from unwrapping 'None' and allows for alternative actions or default values.
Can 'Result' and 'Option' be converted between each other?
Yes, Rust provides methods like 'ok()', 'err()', and 'and_then()' to convert between 'Option' and 'Result', allowing for flexible error handling strategies.
What are some common patterns for propagating errors with 'Result'?
Common patterns include using the '?' operator for concise error propagation and creating custom error types to provide more detailed error information.
How can I improve error handling robustness using 'Result'?
Define custom error types, utilize logging for errors, and combine multiple error types with enums or other structures to handle complex scenarios comprehensively.