Typescript - TypeScript for improved coding

AI-powered coding assistant for TypeScript
⚙️ Transform this javascript into typescript
✨ Create types and interfaces for this code
🪲 Find any bug or improvement in my code
💡 Teach me a useful skill or trick in Python
Get Embed Code
A Detailed Introduction to TypeScript
TypeScript is a statically typed superset of JavaScript, designed to enhance the development process by adding type safety and other advanced features that JavaScript lacks. TypeScript transpiles to plain JavaScript, meaning it runs on any environment that supports JavaScript, including browsers and Node.js. It introduces a type system that helps developers catch potential errors early in the development process, improving the quality of code, enhancing scalability, and supporting robust tooling. TypeScript was developed by Microsoft to address large-scale application development issues often encountered when using JavaScript, especially in terms of maintainability and code consistency. An example scenario where TypeScript shines is in enterprise-scale applications. In large codebases, keeping track of function signatures and ensuring that data types are correct can be cumbersome and error-prone in JavaScript. TypeScript addresses this by allowing developers to explicitly define types, helping prevent runtime errors and providing better tooling support like auto-completion and refactoring. Powered by ChatGPT-4o。
Key Functions and Features of TypeScript
Static Typing
Example
In TypeScript, you can define types explicitly: ```ts function add(a: number, b: number): number { return a + b; } ```
Scenario
A team building a large-scale eCommerce platform uses TypeScript to define strict data models for product inventories, user data, and orders. Static typing ensures that data passed between components adheres to these models, reducing runtime errors and increasing confidence in the system.
Type Inference
Example
TypeScript can infer the type based on the value: ```ts let count = 42; // inferred as number ```
Scenario
In a startup environment where speed is essential but maintainability is still important, developers benefit from TypeScript's ability to infer types from values, allowing them to write less boilerplate code while still gaining the advantages of type checking.
Interfaces and Type Aliases
Example
You can define custom types using interfaces or type aliases: ```ts interface User { name: string; age: number; } ```
Scenario
In a software project handling customer data, using interfaces ensures that objects passed around follow the same structure. For example, an API that returns a `User` object can ensure that the name and age properties are always correctly typed across the entire codebase.
Generics
Example
Generics allow for reusable components that can handle different types: ```ts function identity<T>(arg: T): T { return arg; } ```
Scenario
In a utility library where functions must work with different types (e.g., fetching data from multiple APIs), generics enable developers to write flexible, reusable functions while maintaining type safety.
Modules and Namespaces
Example
TypeScript supports ES6 modules for better code organization: ```ts import { User } from './models/User'; ```
Scenario
In a microservices architecture, each service could have its own TypeScript module that encapsulates related functionality. This modular approach enhances maintainability, especially when multiple teams are working on different parts of a system.
Decorators
Example
Decorators add metadata to classes and methods: ```ts function Log(target: any, key: string) { console.log(`${key} was called`); } class Example { @Log method() {} } ```
Scenario
In a backend system built using TypeScript with a framework like NestJS, decorators are used to define routes, middleware, or other metadata on class methods, streamlining code structure and readability.
Ideal Users and Beneficiaries of TypeScript
Enterprise Developers
Enterprise-level development teams benefit greatly from TypeScript due to its scalability, type safety, and support for complex codebases. In large projects where many developers work on different parts of the system, TypeScript helps ensure code consistency, reduces bugs, and makes refactoring safer.
JavaScript Developers Moving to Typed Systems
JavaScript developers looking for better tools to manage larger projects or those who want to reduce runtime errors would find TypeScript highly beneficial. It introduces typing and other modern programming features while remaining close to the JavaScript ecosystem, making the transition smooth.
Full-Stack Developers
Full-stack developers who work across both front-end and back-end can use TypeScript to maintain consistent types throughout the entire stack, whether it's a React frontend or a Node.js backend. This type consistency reduces errors when passing data between layers.
Open Source and Framework Developers
Developers building frameworks, libraries, or open-source projects benefit from TypeScript’s clear typing, making it easier for others to use and contribute to their projects. By providing type definitions, these projects ensure that consumers of the library have better tooling support and fewer integration issues.
Teams Adopting Agile Methodologies
Teams working in agile environments, where speed and quality need to be balanced, can leverage TypeScript’s type checking and error detection to quickly iterate on code without sacrificing stability. TypeScript helps prevent regressions as the codebase evolves, even with frequent changes.
How to Use TypeScript
1. Visit yeschat.ai
For a free trial without login and no need for ChatGPT Plus, start at yeschat.ai, where you can quickly test out TypeScript functionality in various chat environments.
2. Install Node.js and npm
Before using TypeScript, make sure you have Node.js installed, which includes npm (Node Package Manager). This allows you to install TypeScript and other dependencies easily.
3. Install TypeScript globally
Use npm to install TypeScript globally on your machine by running the command `npm install -g typescript`. This will give you access to the TypeScript compiler (`tsc`).
4. Create and compile a TypeScript file
Write your first TypeScript file (`.ts`) and compile it into JavaScript using the command `tsc yourfile.ts`. This will output a `.js` file that the browser or Node.js can run.
5. Configure tsconfig.json
To streamline compilation, set up a `tsconfig.json` file in your project. This file defines TypeScript settings, like target JavaScript version, module system, and other compiler options.
Try other advanced and practical GPTs
Django Copilot
AI-powered tool for Django development

Amelia
Unlock Environmental Insights with AI
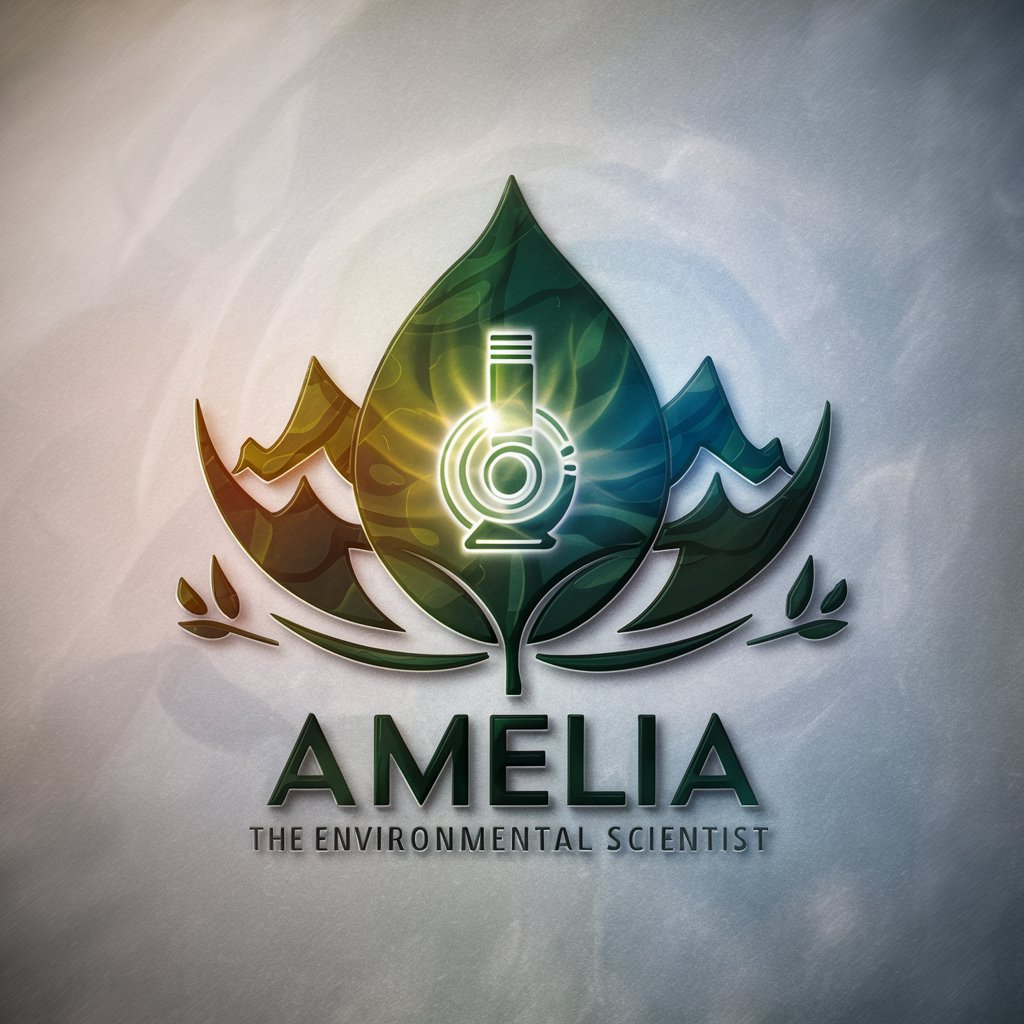
2012 Texas Accessibility Standards
Empowering Access for Everyone
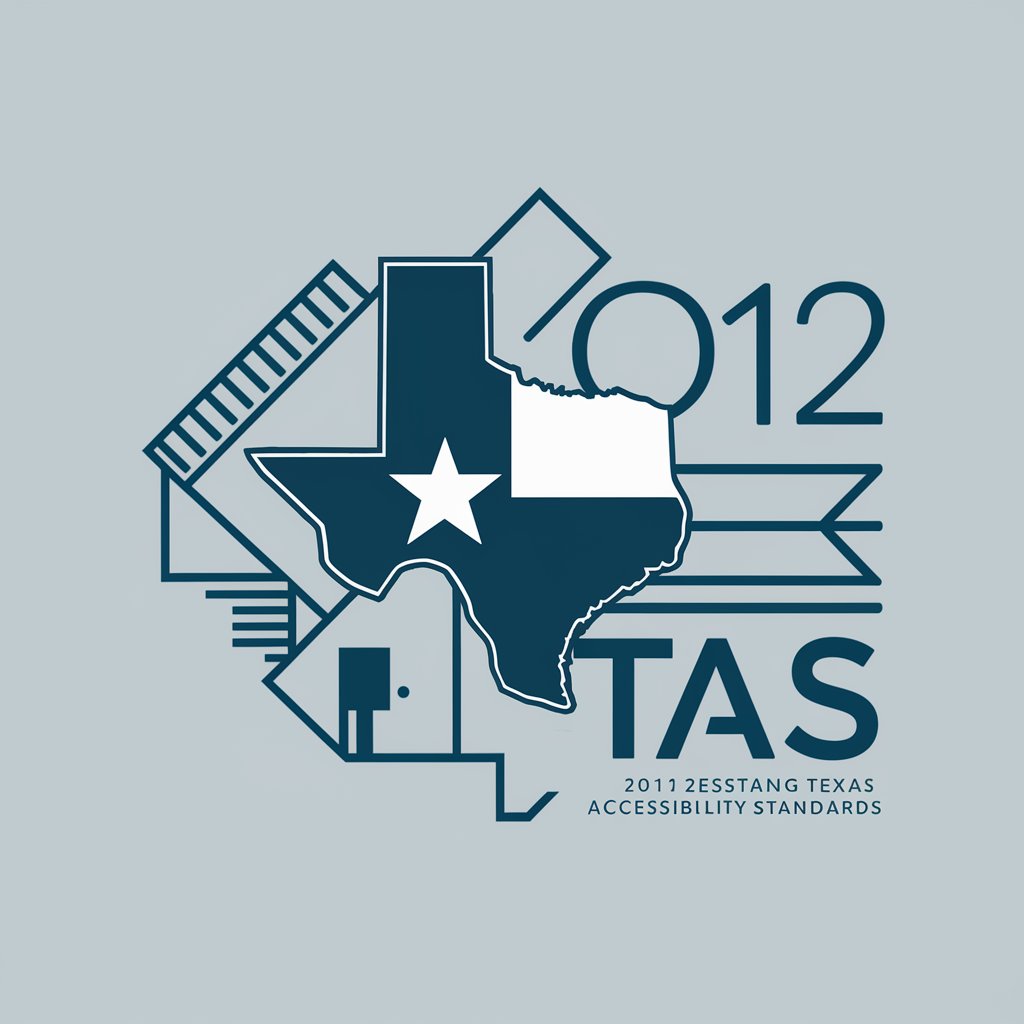
Health Standards Expert
Empowering Healthcare with AI-driven Standards
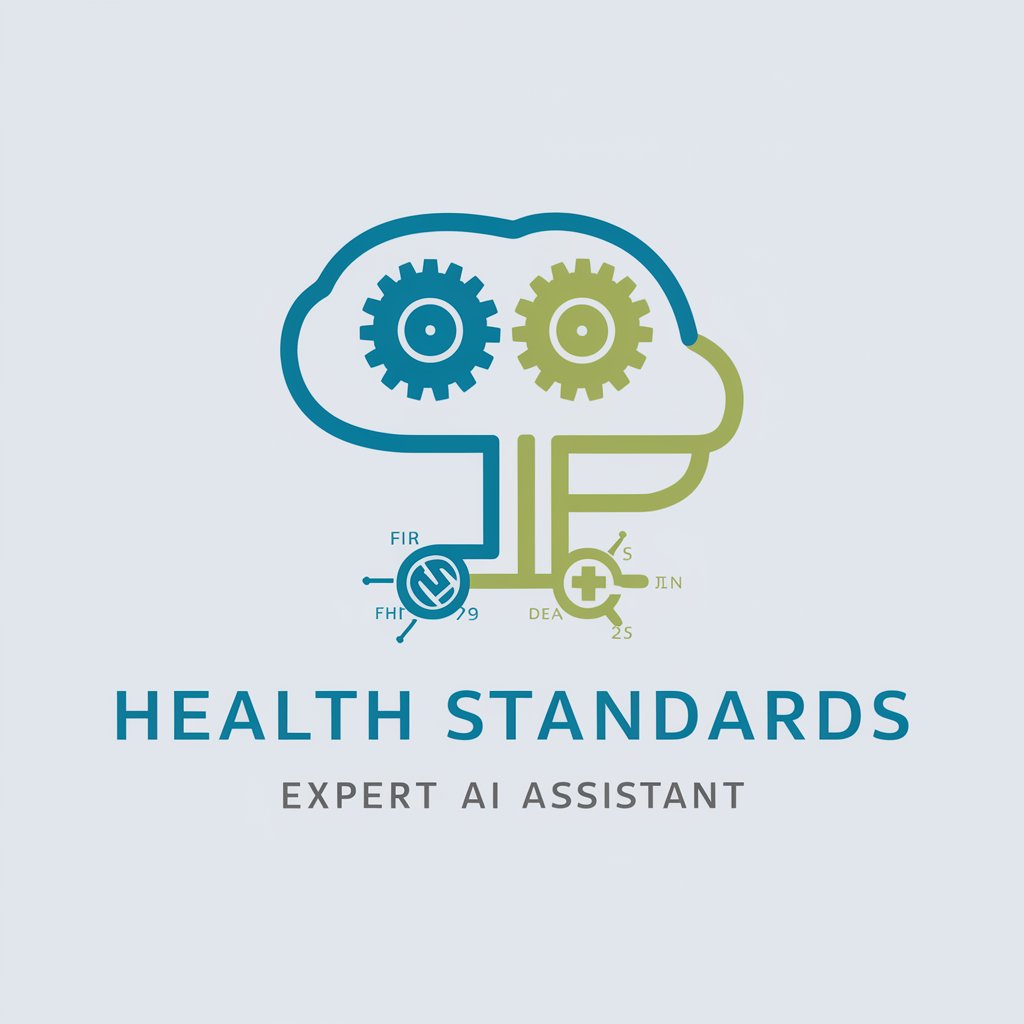
Standards Architect
Navigate Standards with AI Power
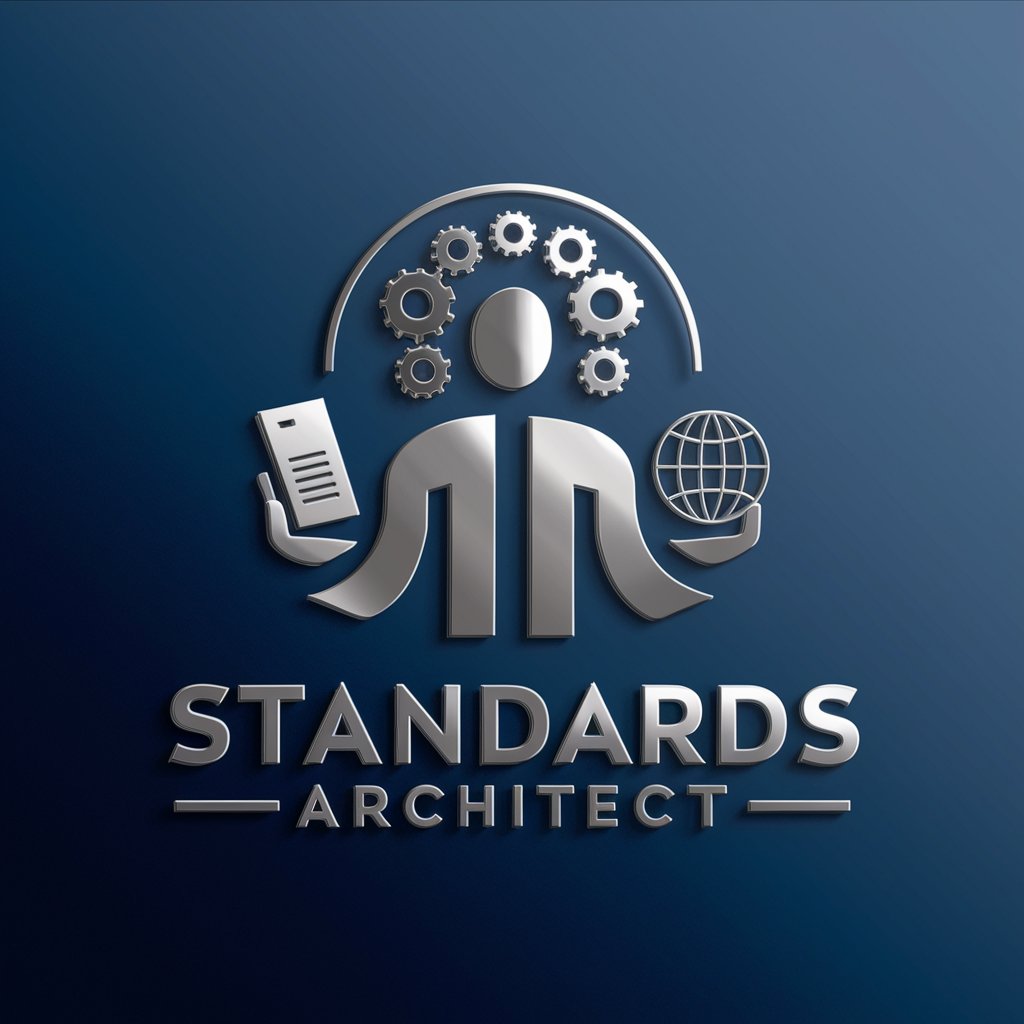
DNVGL Standards Assistant
AI-Powered Precision for Standards
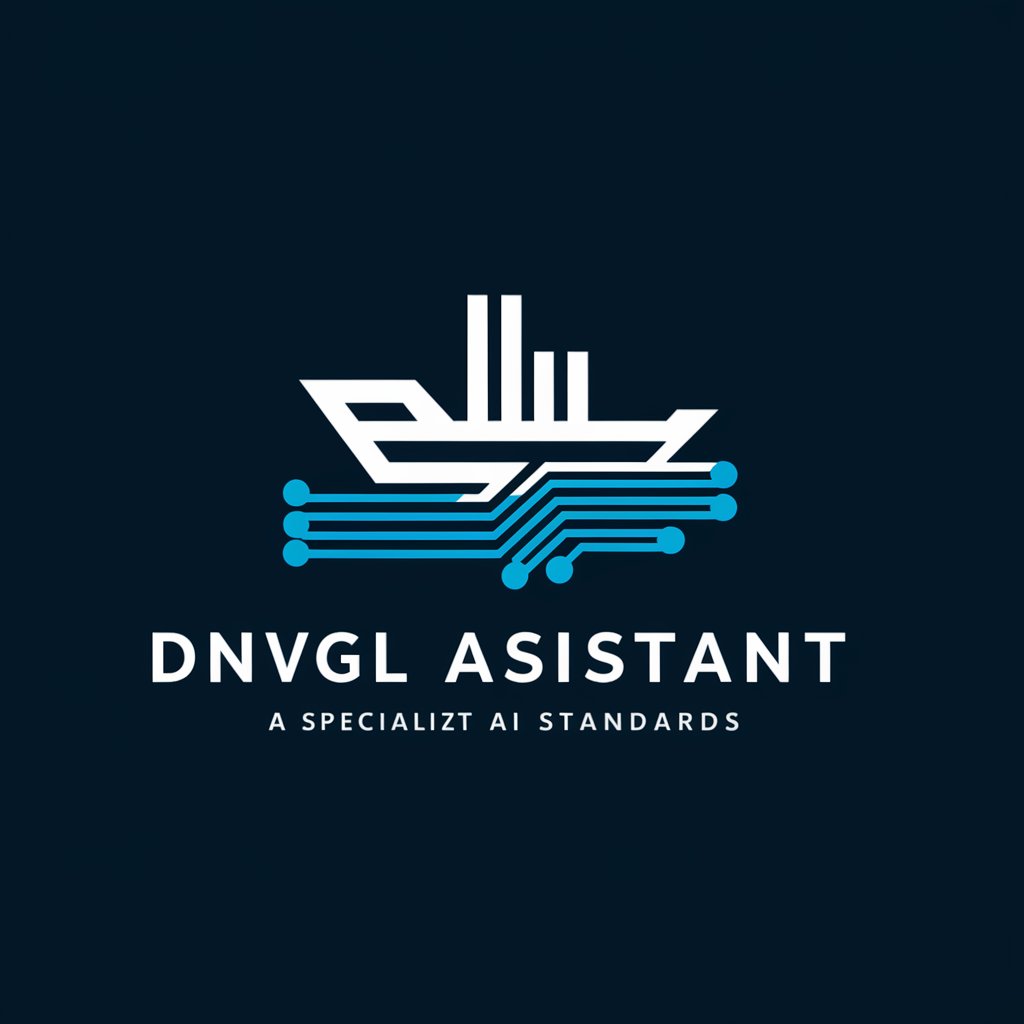
Web Search Copilot
AI-powered research at your fingertips.
Unit Test Engineer
Simplify Testing with AI-Powered Insights
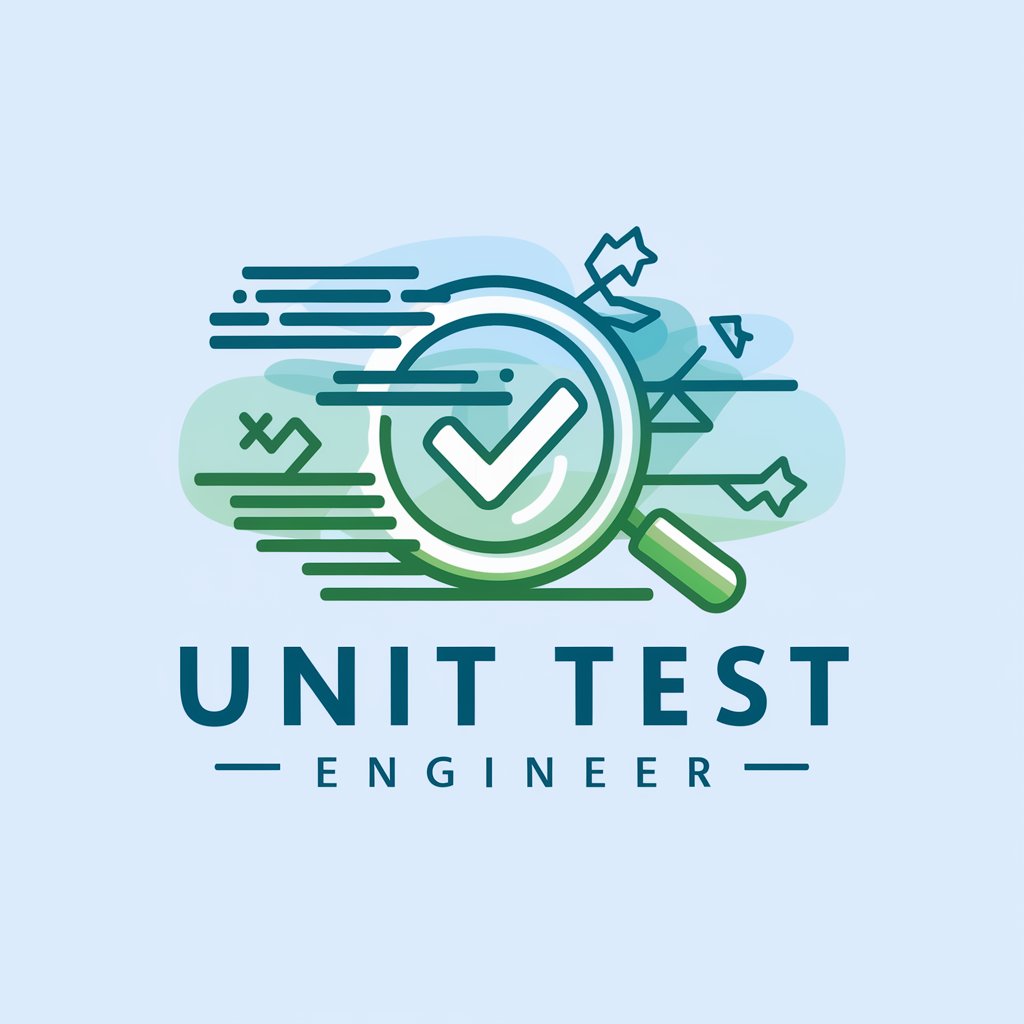
Homeschooling Helper
Empowering Education with AI
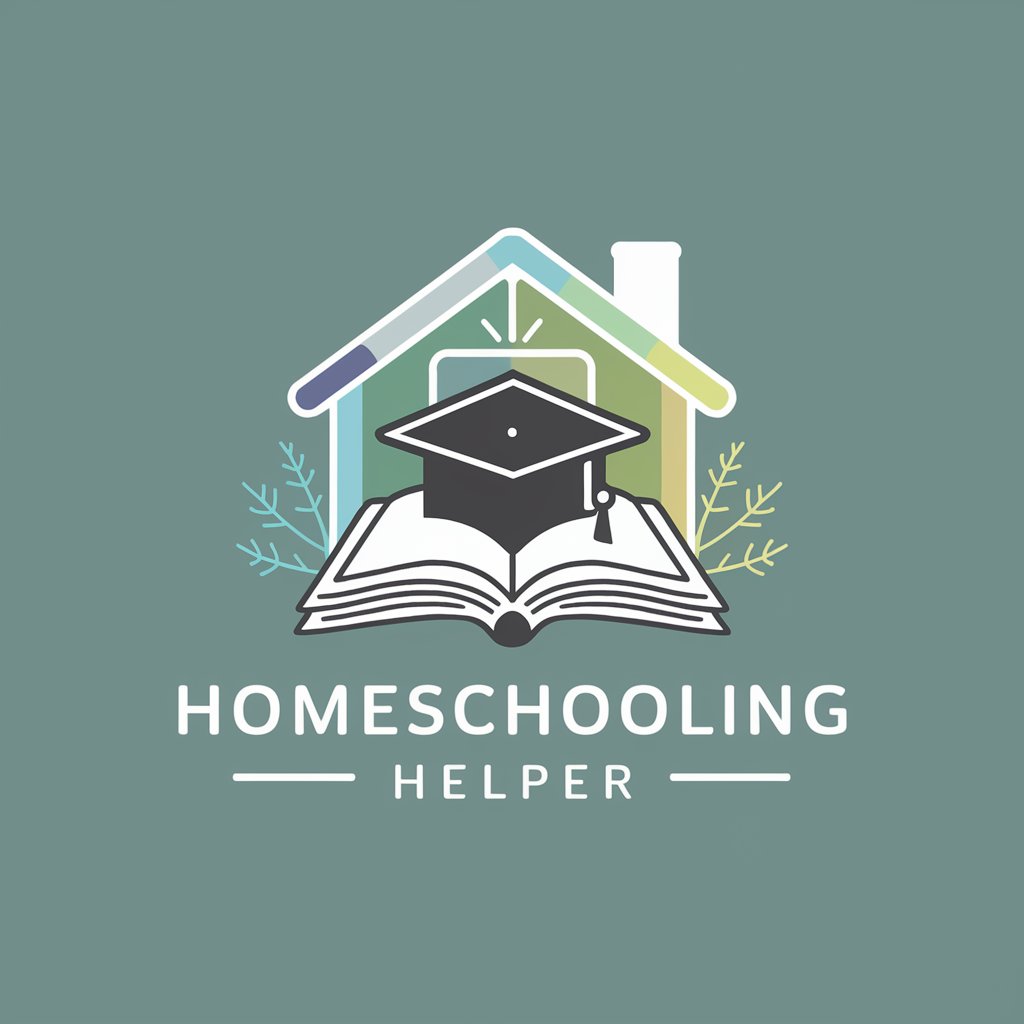
Windows and Doors
Elevate Your Design with AI-Powered Insights
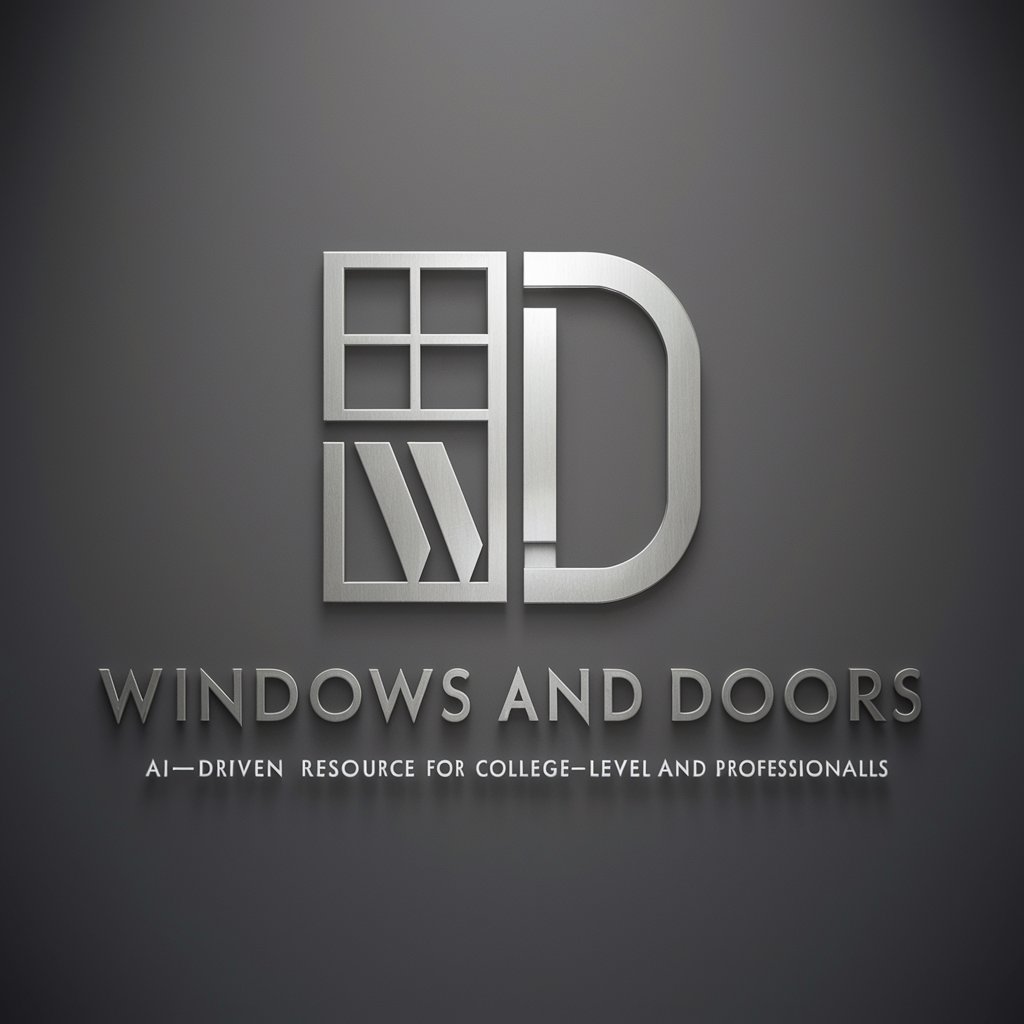
DOORS 👁️
Enhancing productivity with AI
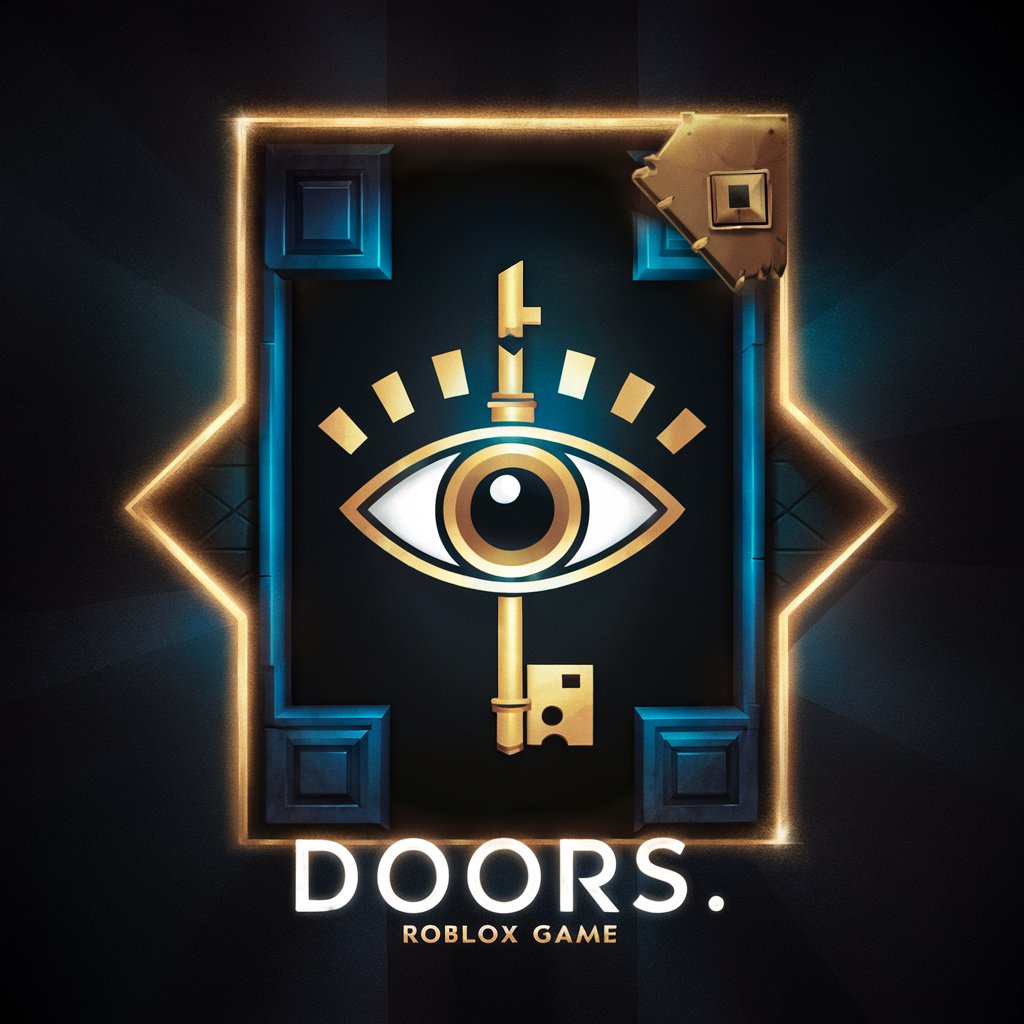
Medical Diagnosis Analysis
AI-Powered Medical Diagnosis and Insights

Common TypeScript Questions & Answers
What is TypeScript and how does it differ from JavaScript?
TypeScript is a typed superset of JavaScript that adds static type definitions. While JavaScript is dynamically typed, TypeScript helps prevent errors by enabling developers to define data types explicitly, which improves code reliability and readability.
Can I use existing JavaScript libraries in TypeScript?
Yes, you can. TypeScript is compatible with JavaScript libraries. You can use declaration files (`.d.ts`) to integrate type definitions for those libraries, either by writing them yourself or using community-provided types from DefinitelyTyped.
How does TypeScript improve the development experience?
TypeScript provides features like type-checking, autocompletion, and advanced refactoring tools, which reduce bugs and increase productivity. It also supports modern ECMAScript features and compiles them into compatible JavaScript for different environments.
What are some common use cases for TypeScript?
TypeScript is widely used in large-scale applications, React/Angular development, Node.js back-end services, and any project requiring long-term maintenance where type safety improves reliability.
How do I enable strict type checking in TypeScript?
In your `tsconfig.json` file, set `"strict": true`. This turns on strict type-checking options such as `noImplicitAny`, `strictNullChecks`, and `strictFunctionTypes`, ensuring more rigorous error detection.