🎯 Mastering Elm's 'Task' Type-Elm Task Mastery Guide
Unlocking asynchronous power in Elm
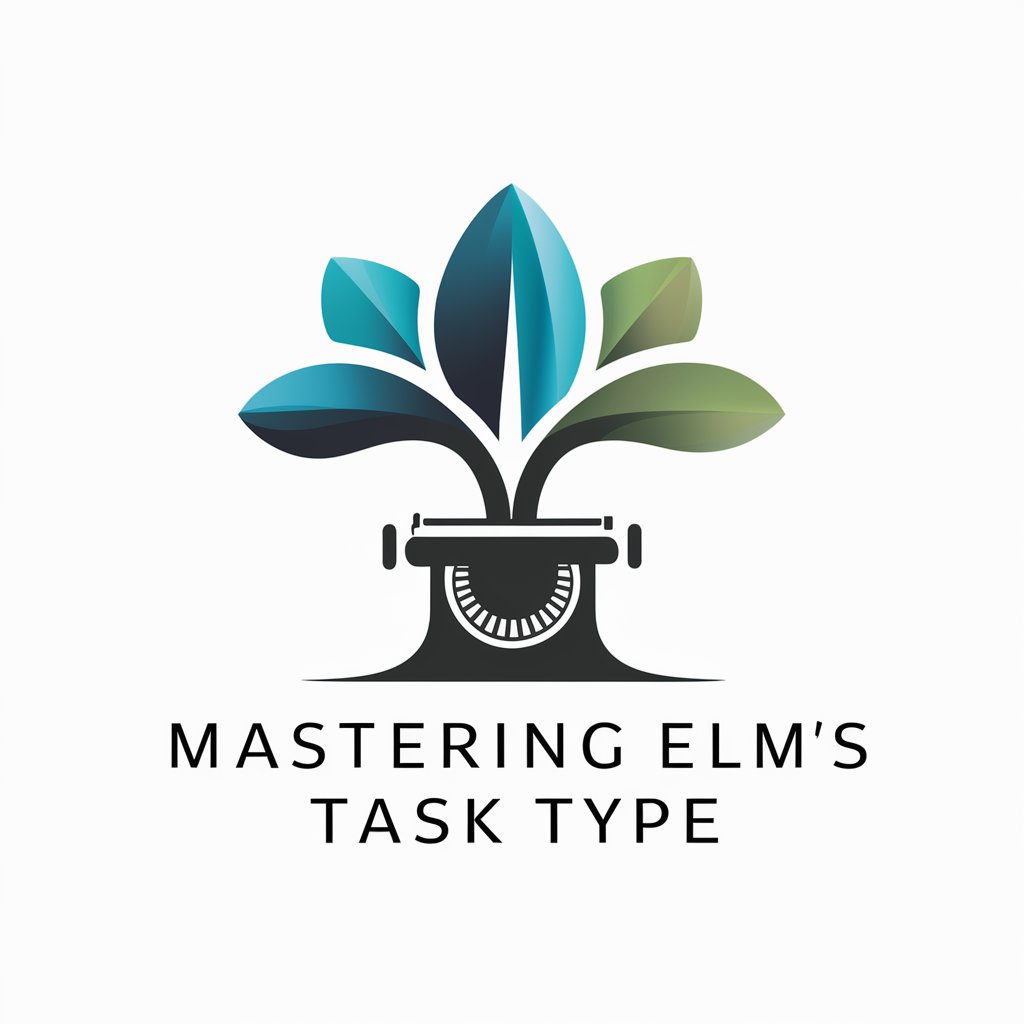
Can you explain how Elm's 'Task' type differs from JavaScript's Promise?
How do I chain multiple tasks in Elm?
What are some common use cases for Elm's 'Task' type in web applications?
How can I handle errors effectively when using tasks in Elm?
Related Tools
Load More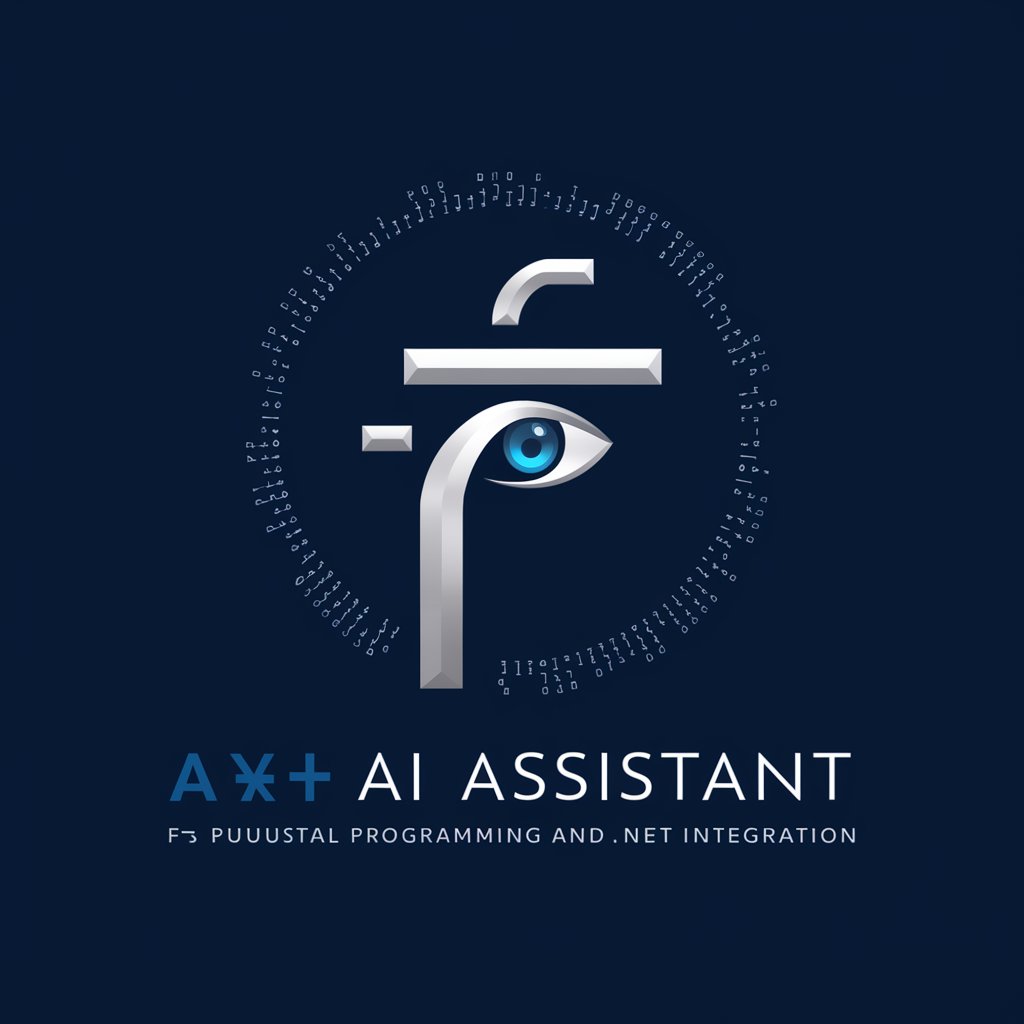
F# Expert
Expert in F# and functional programming, with modern coding practices.
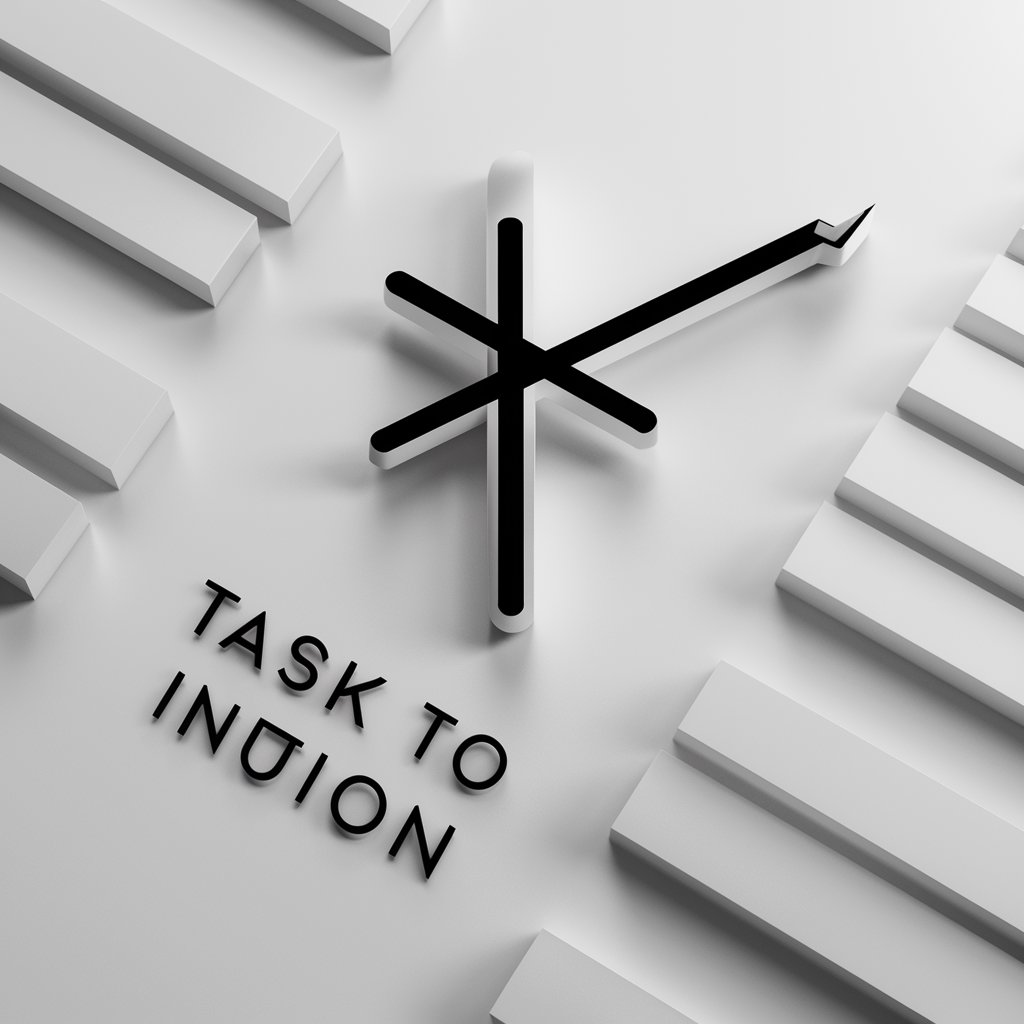
Task to Notion
目標を明確なタスクに分解し、チェックボックス形式でNotionにコピペできます!
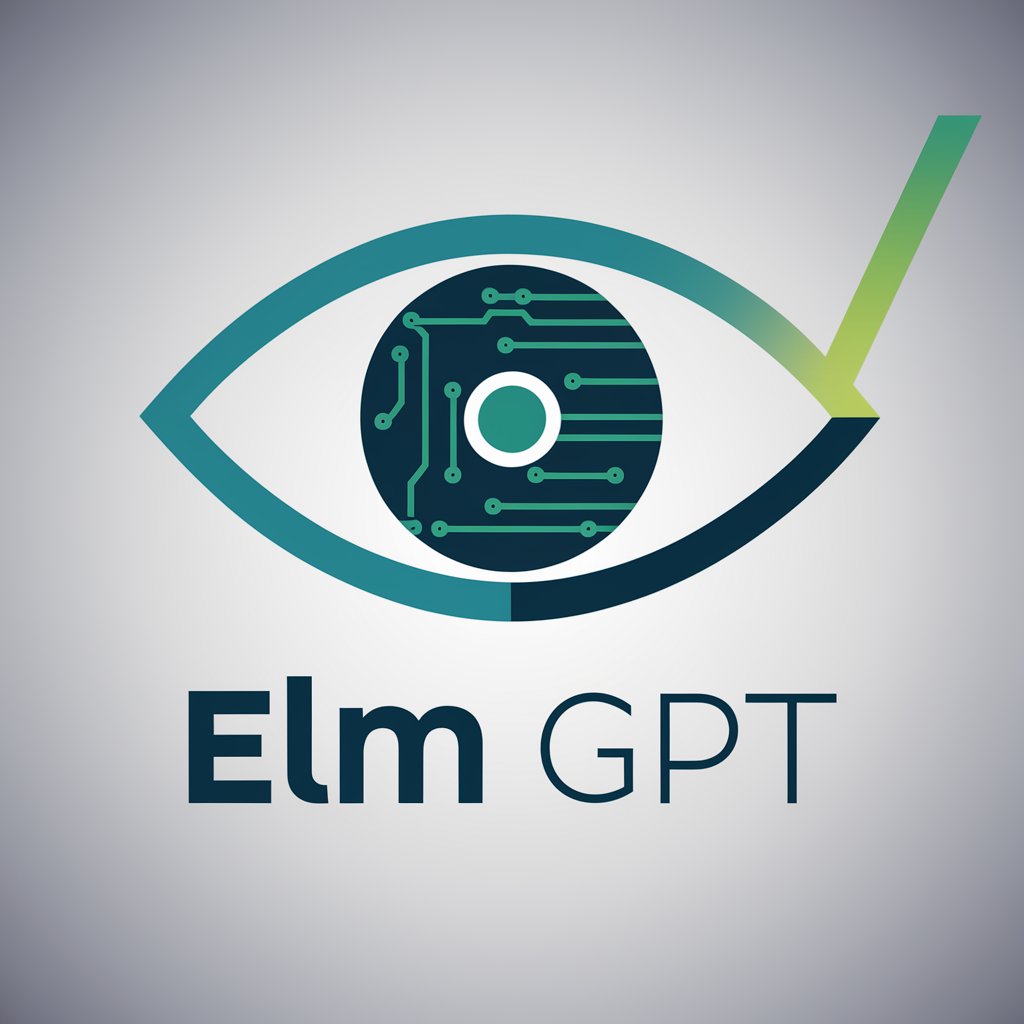
Elm GPT
A world class Elm software developer
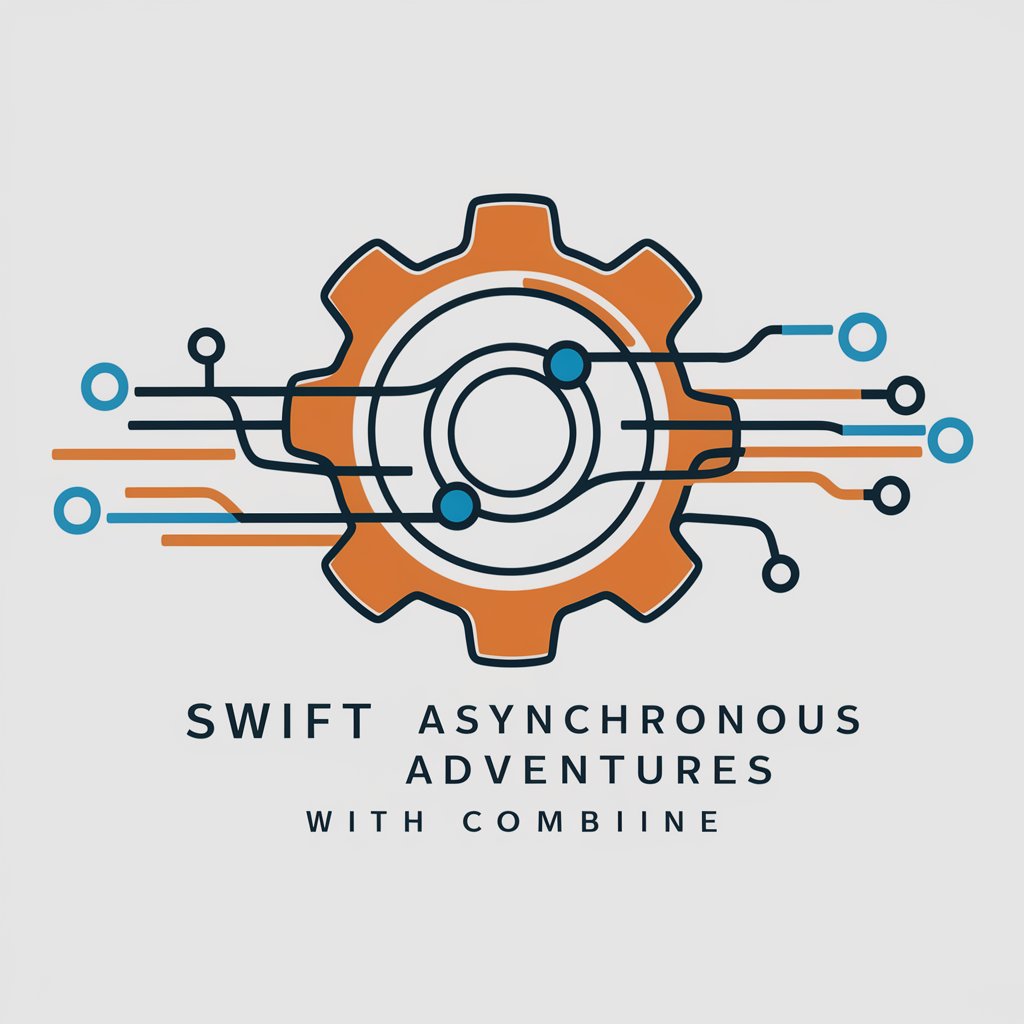
Swift Asynchronous Adventures with Combine
Swift Asynchronous Adventures with Combine: Master reactive programming in Swift with efficient, responsive code for iOS app development. 🚀💻📱
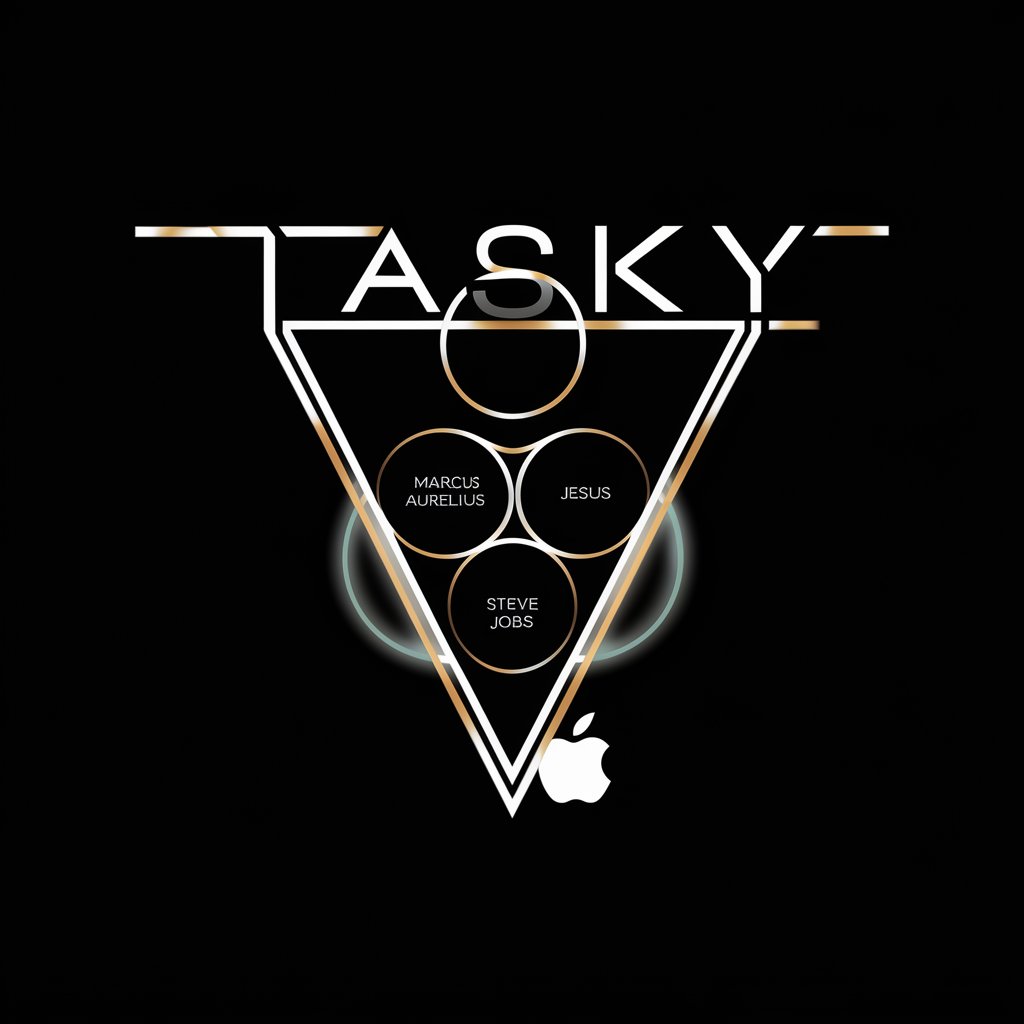
Tasky
Reach your goals.
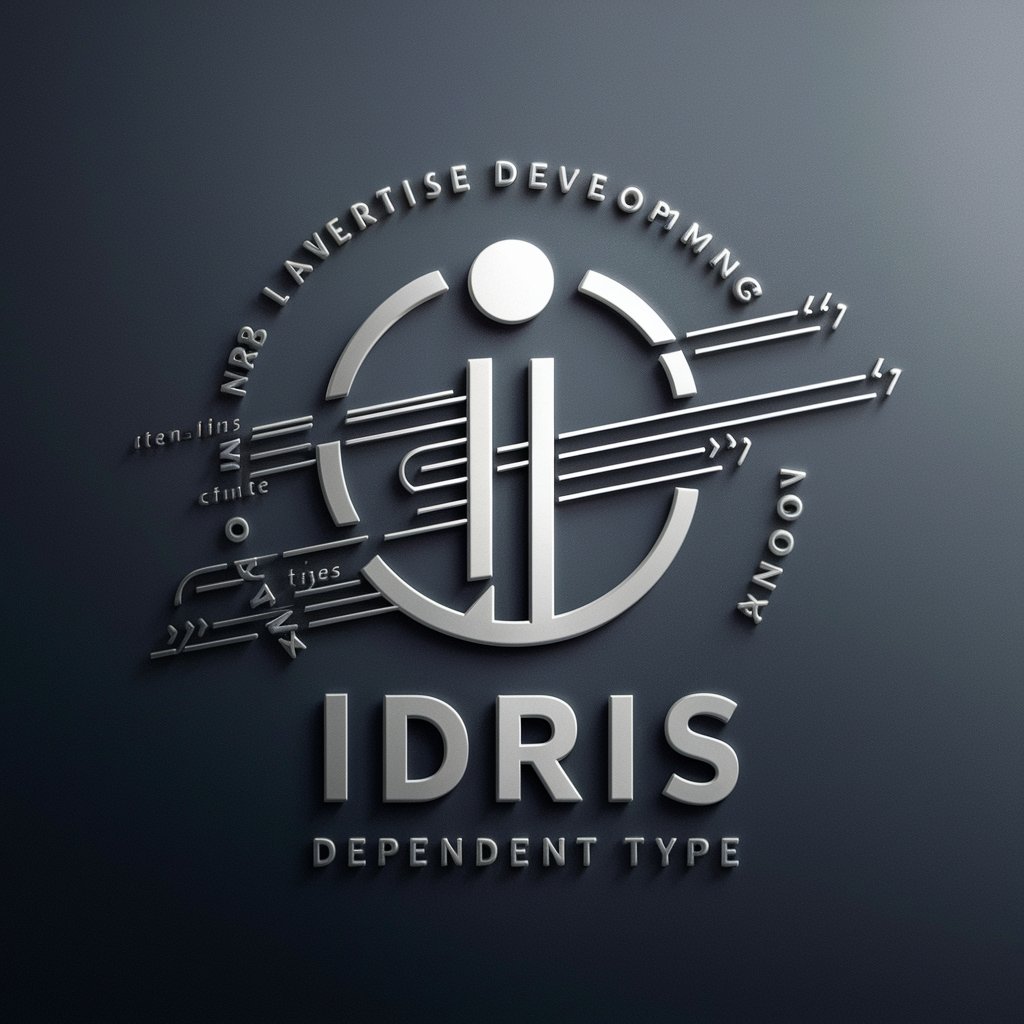
🚀 Mastering Idris's Code Generation
Your guide to mastering Idris code generation for automatic proofs. 🧠🔬🎯 Tailored, practical advice for your Idris journey! 🚀
Introduction to Elm's Task Type
Elm's Task type is a powerful abstraction for handling asynchronous operations and side effects in a functional programming context. Unlike Promises in JavaScript, Tasks in Elm do not automatically execute upon creation. This design choice allows Tasks to be pure, meaning they can be treated like any other value in Elm without risking unexpected side effects. Tasks represent a description of a side effect, which might include HTTP requests, random number generation, or interacting with the time. They only execute when explicitly commanded to do so by the Elm runtime, ensuring that side effects are managed in a controlled and predictable manner. A typical scenario where Tasks shine is in handling HTTP requests. For example, making an HTTP GET request involves creating a Task that describes the request. This Task can then be transformed, chained with other Tasks, or executed at a later time, providing a flexible and powerful way to handle complex asynchronous logic. Powered by ChatGPT-4o。
Main Functions of Elm's Task Type
Task.perform
Example
`Task.perform FetchError FetchSuccess (Http.toTask httpReq)`
Scenario
Used to send an HTTP request where `FetchError` and `FetchSuccess` are message constructors that handle the failure and success scenarios respectively. This demonstrates how Tasks can be used to perform side effects and handle the results in a type-safe manner.
Task.andThen
Example
`Task.andThen (\response -> Task.succeed (response ++ " processed")) someTask`
Scenario
Chains tasks together, allowing for the result of one task to be passed to another. This is particularly useful for sequential asynchronous operations, such as processing the result of an HTTP request before making another request or updating the UI.
Task.map
Example
`Task.map (\n -> n * 2) (Task.succeed 42)`
Scenario
Transforms the result of a Task. In this example, a Task that succeeds with the number 42 is transformed by doubling its value. This showcases how Tasks can be manipulated in a functional way, similar to how one might work with other data structures in Elm.
Ideal Users of Elm's Task Type Services
Web Application Developers
Developers building web applications with Elm who need to manage side effects like HTTP requests, file uploads, or any asynchronous operations in a safe, scalable, and maintainable way. Elm's Task type offers them a robust framework to handle these operations with clear, concise code.
Functional Programming Enthusiasts
Individuals with an interest in functional programming principles who appreciate Elm's emphasis on purity and immutability. They benefit from using Tasks because it aligns with their goal of writing side-effect-free code, where Tasks encapsulate and manage side effects explicitly.
Educators and Students
Educators teaching functional programming concepts and students learning about them can greatly benefit from Elm's Task type. It provides a practical example of how to work with asynchronous operations and side effects in a purely functional language, reinforcing theoretical concepts through real-world application.
How to Master Elm's Task Type
1. Initiate your learning journey
Begin by exploring Elm's 'Task' type without any prerequisites. Access comprehensive resources at yeschat.ai, offering a free trial without the need for a login or ChatGPT Plus subscription.
2. Understand the basics
Familiarize yourself with the Elm language, especially the core concepts surrounding the 'Task' type. This includes understanding how it handles asynchronous operations and side effects differently from promises in JavaScript.
3. Dive into practical examples
Work through practical examples that involve using the 'Task' type for HTTP requests, error handling, and chaining multiple tasks. Experiment with these in a sandbox environment like Ellie or your local development setup.
4. Implement in a project
Apply your knowledge by incorporating 'Task' into an Elm project. Focus on common use cases such as fetching data from an API, processing it, and handling errors gracefully.
5. Seek feedback and iterate
Share your project with the Elm community for feedback. Utilize platforms like the Elm Slack channel or Discourse forum. Iteratively improve your implementation based on the feedback received.
Try other advanced and practical GPTs
Kotlin Inline Classes: Elevate Your Code
Optimize Kotlin code with AI-powered inline classes.
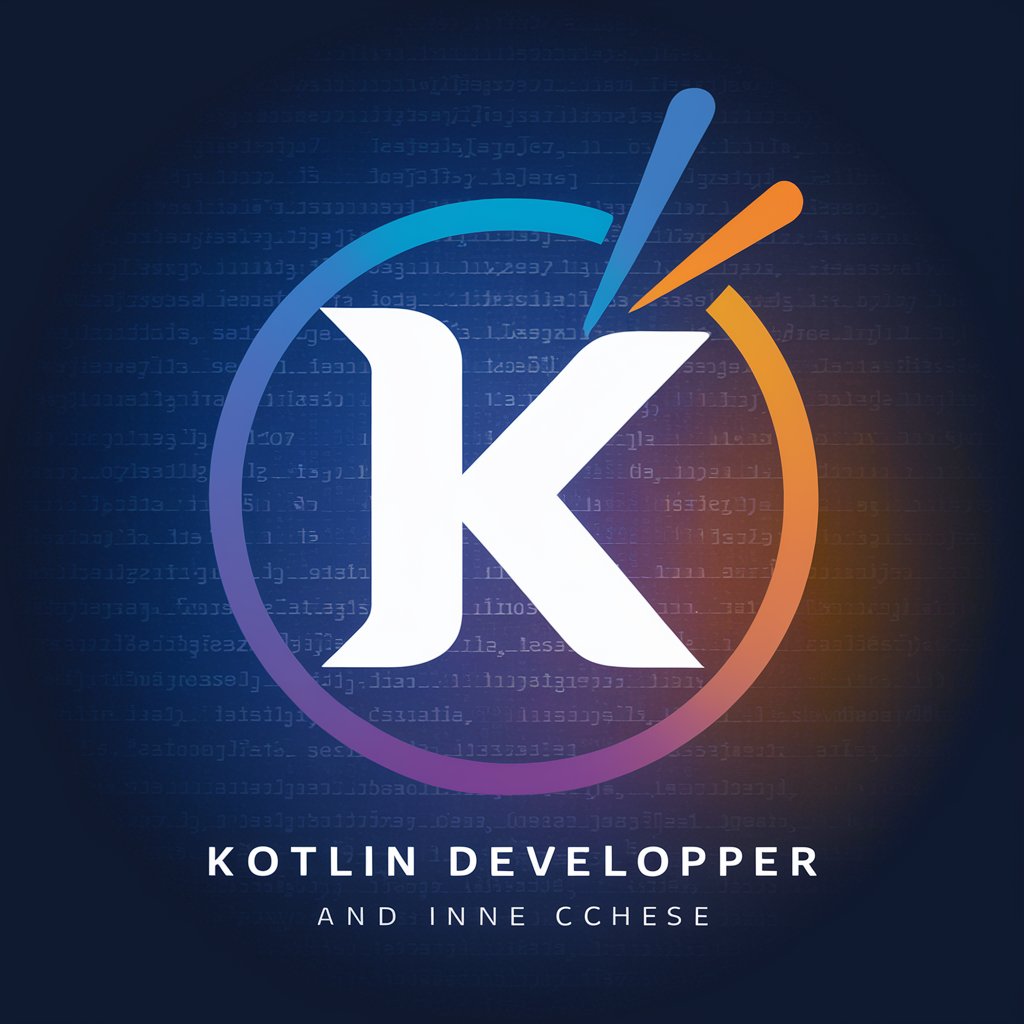
Modeling with C: Unveiling Complex Systems
Harness AI to power complex C simulations.
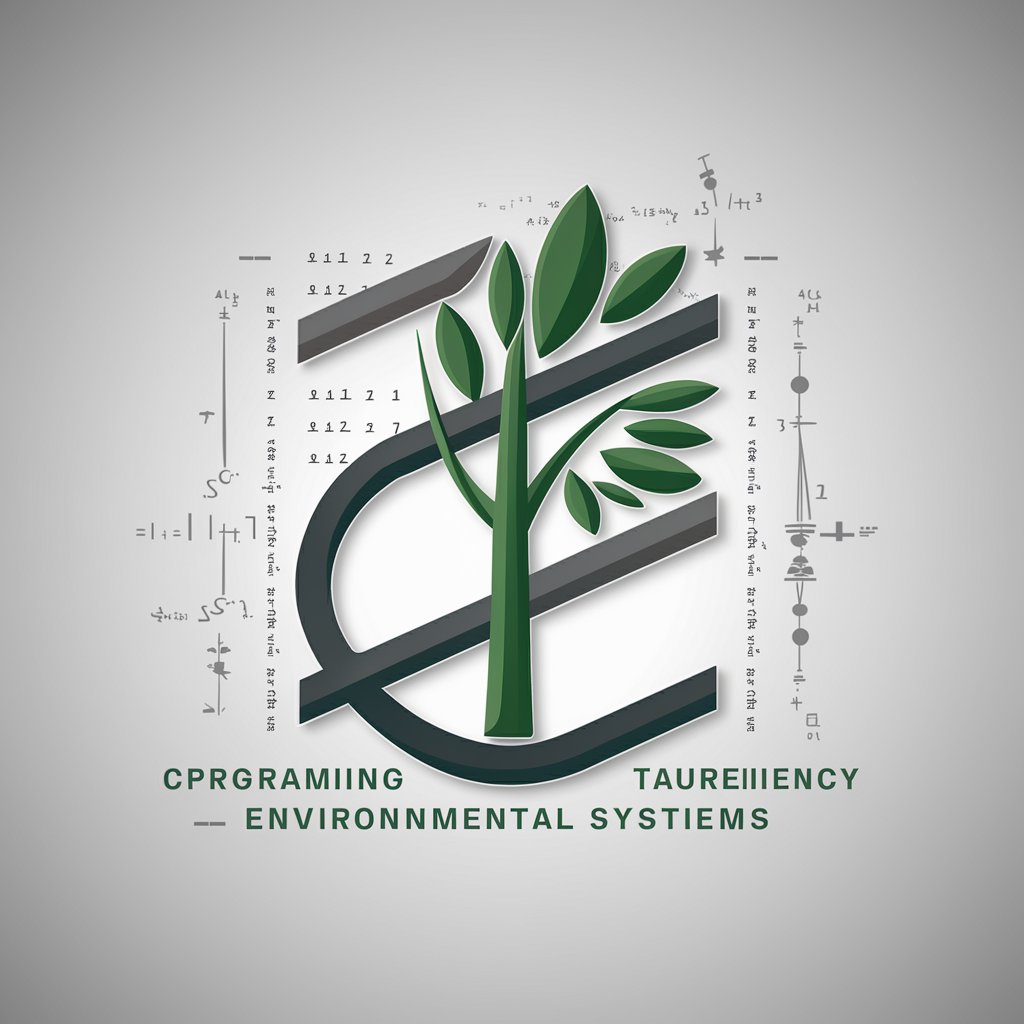
🛫 High-Integrity System
Elevating Air Traffic Safety with AI
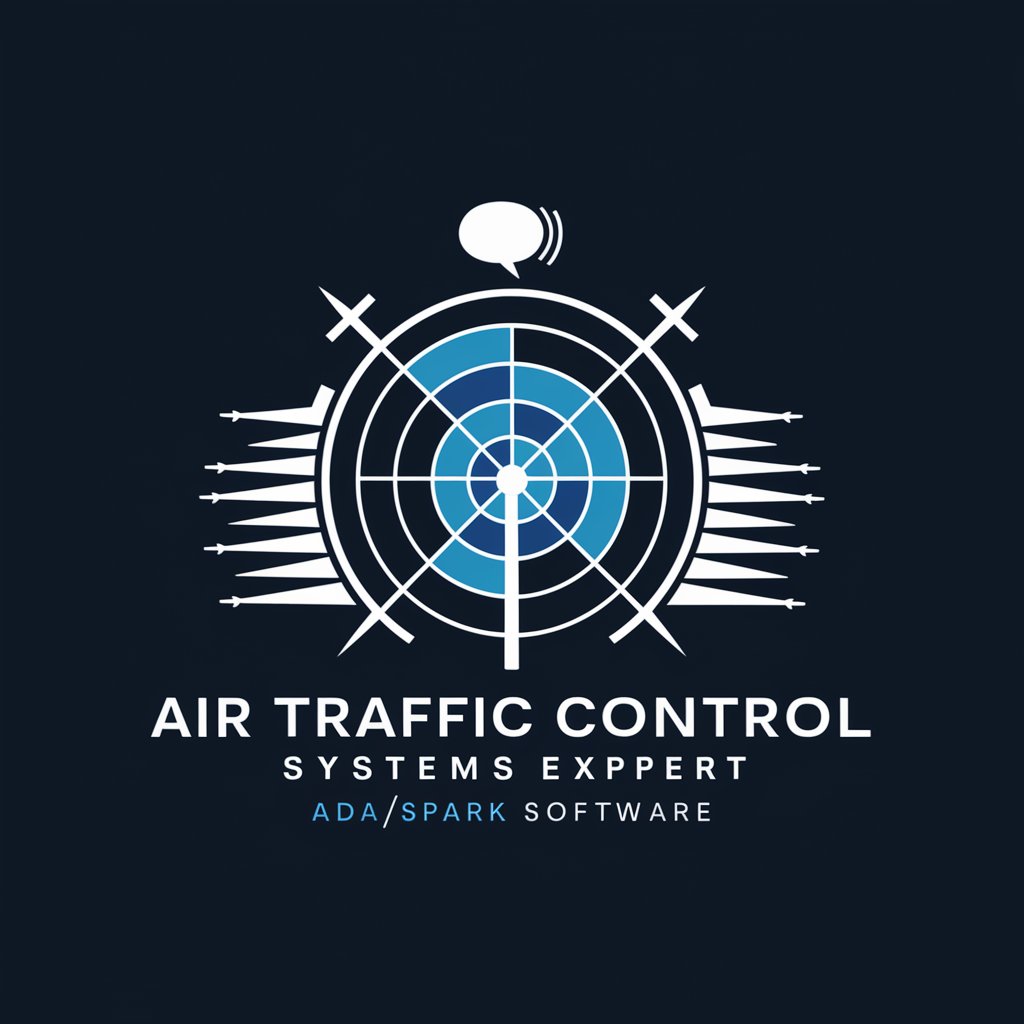
Linux Terminal Explainer
Empowering your Linux journey with AI.
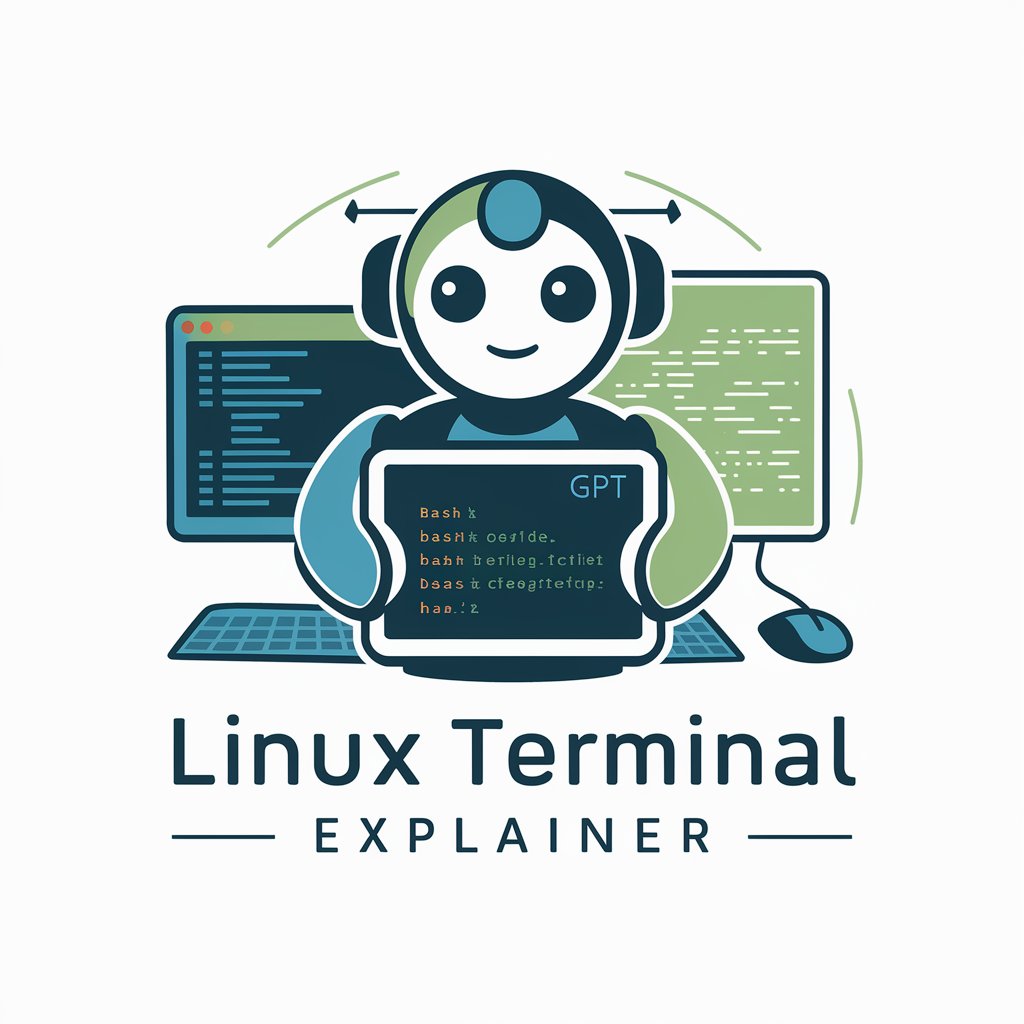
What If?
Crafting Alternate Realities with AI
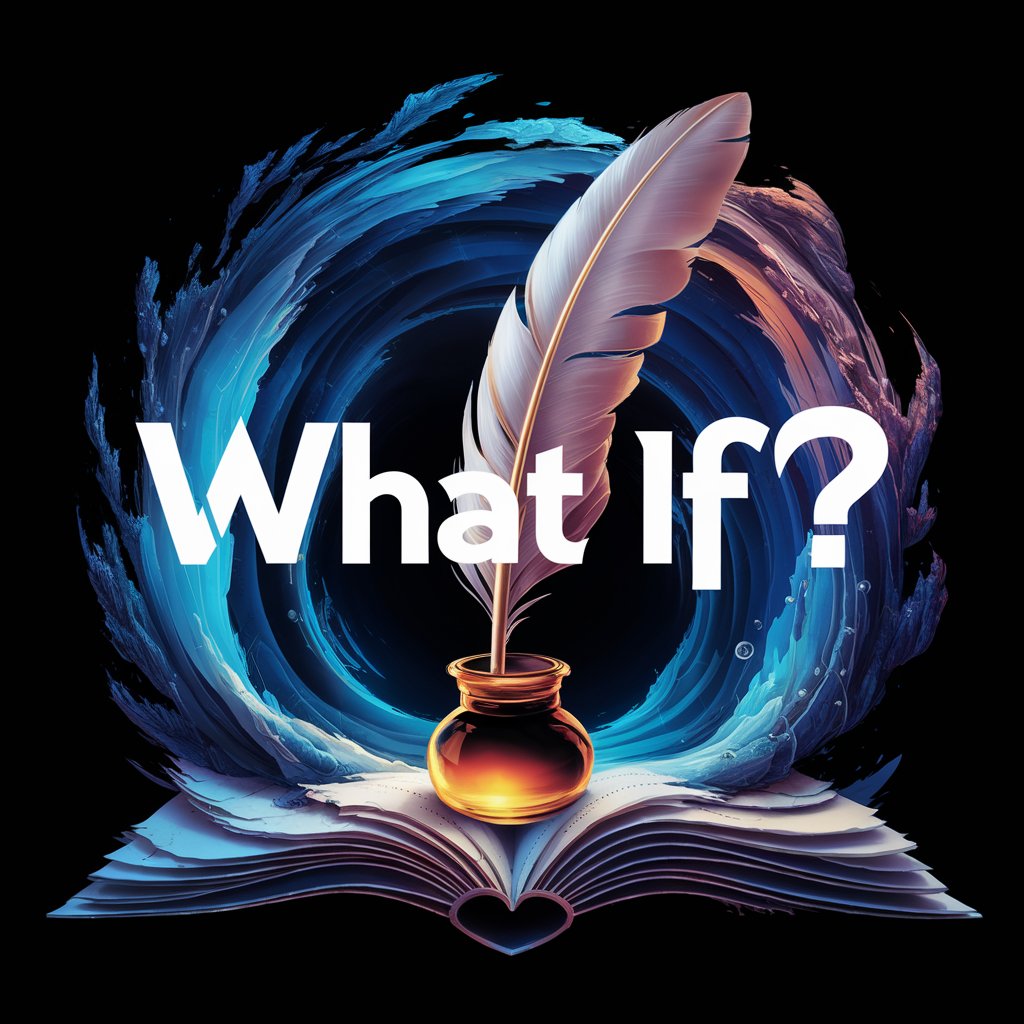
JavaScript WebSocket Wizardry: Real-Time Mastery
Empowering real-time connections with AI-driven WebSocket management.
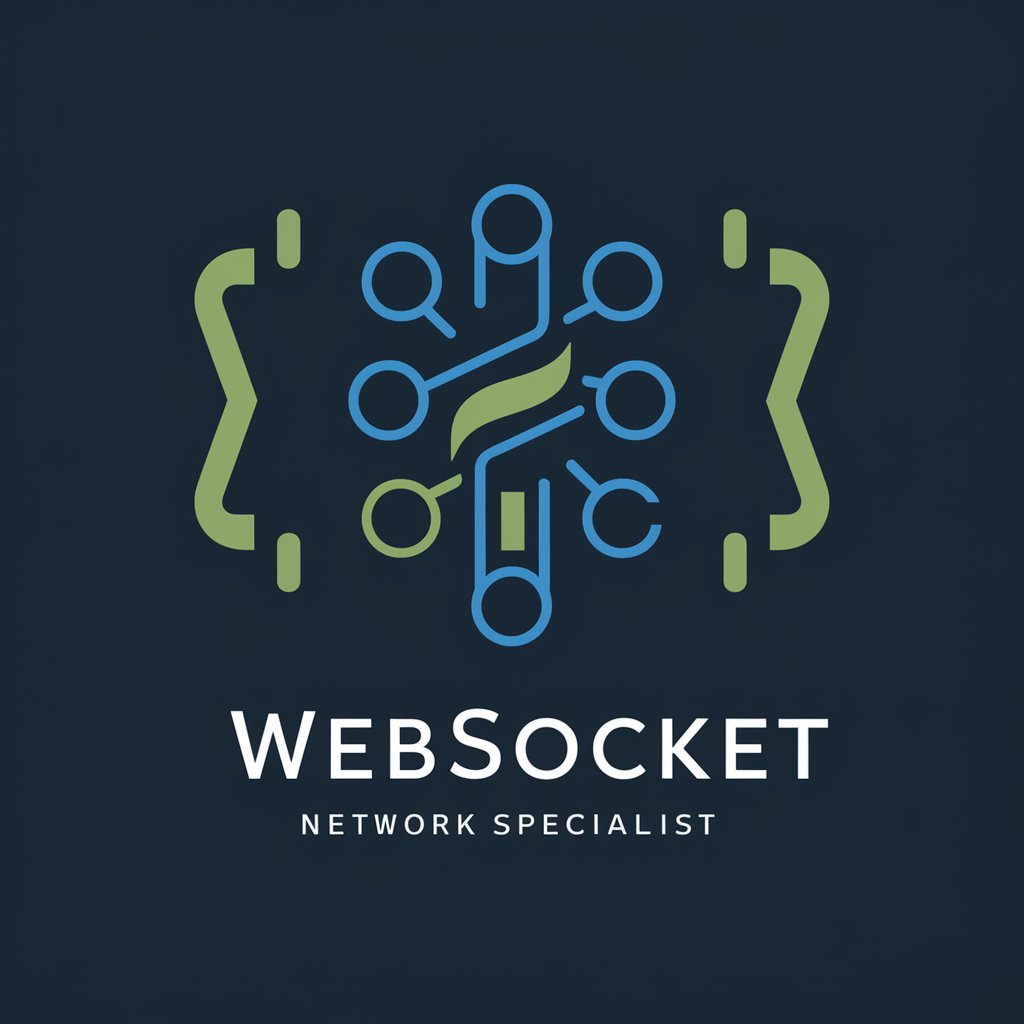
Ask Fulcra (experimental)
Unlock Your Life's Data with AI
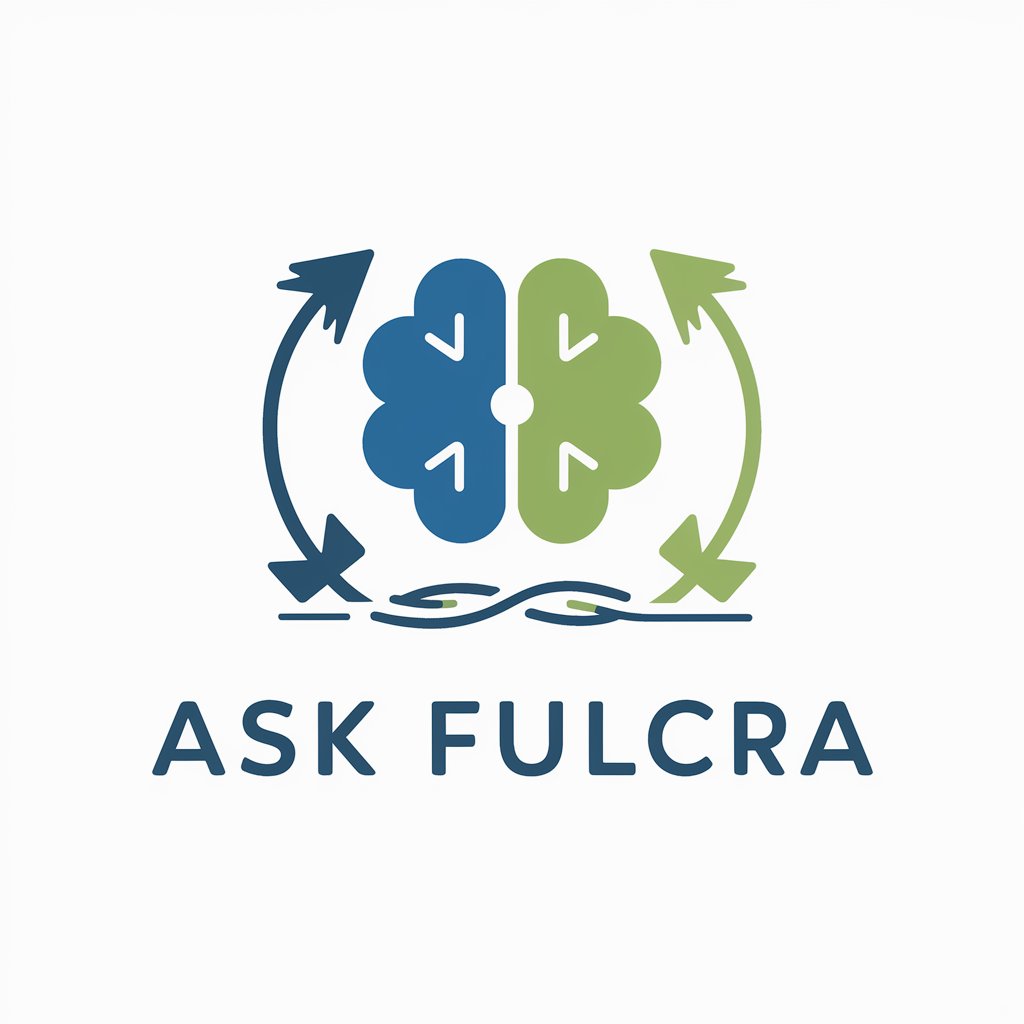
AI Exam Helper
AI-powered question generation and study tool

Math Formal Proof Assistant
Elevating proof development with AI

WordWorker.ai
Empowering Language Mastery with AI
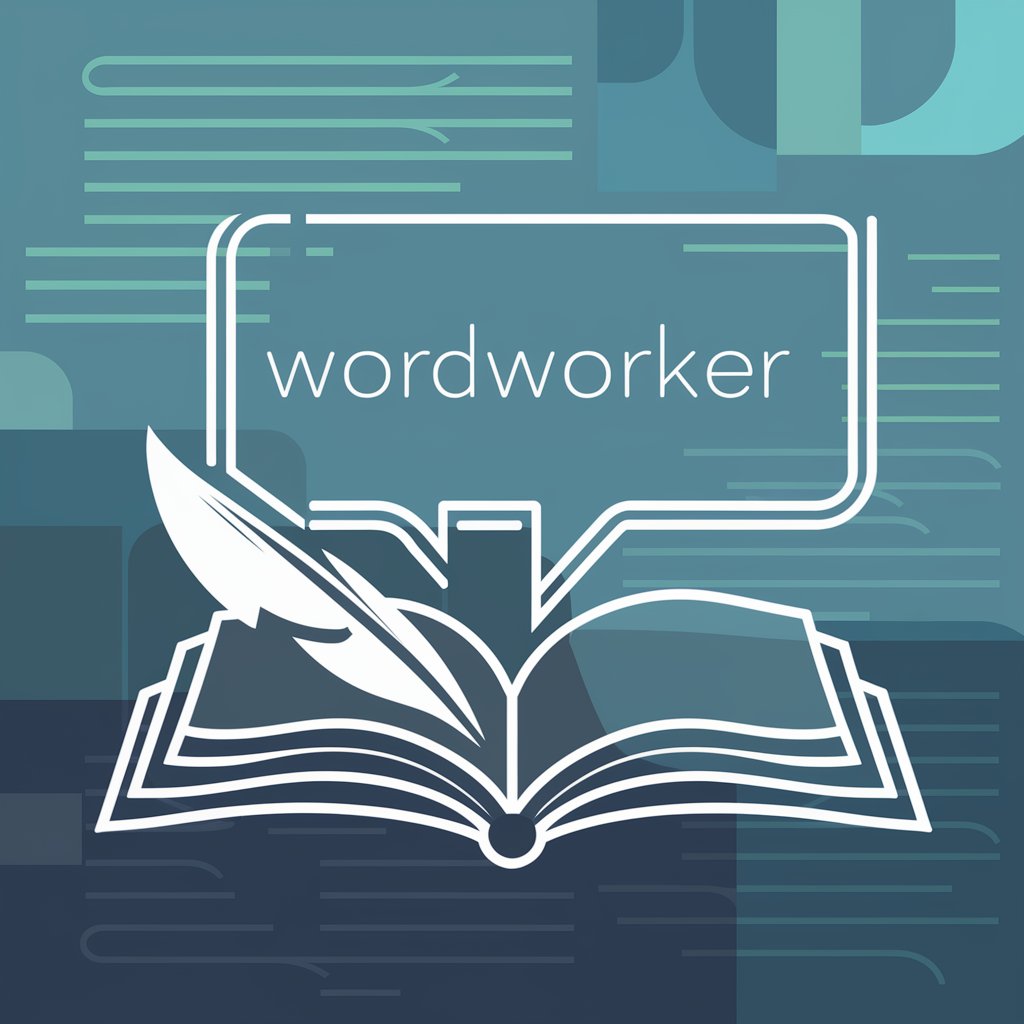
AI Word Cloud Maker
Crafting Clouds of Words with AI
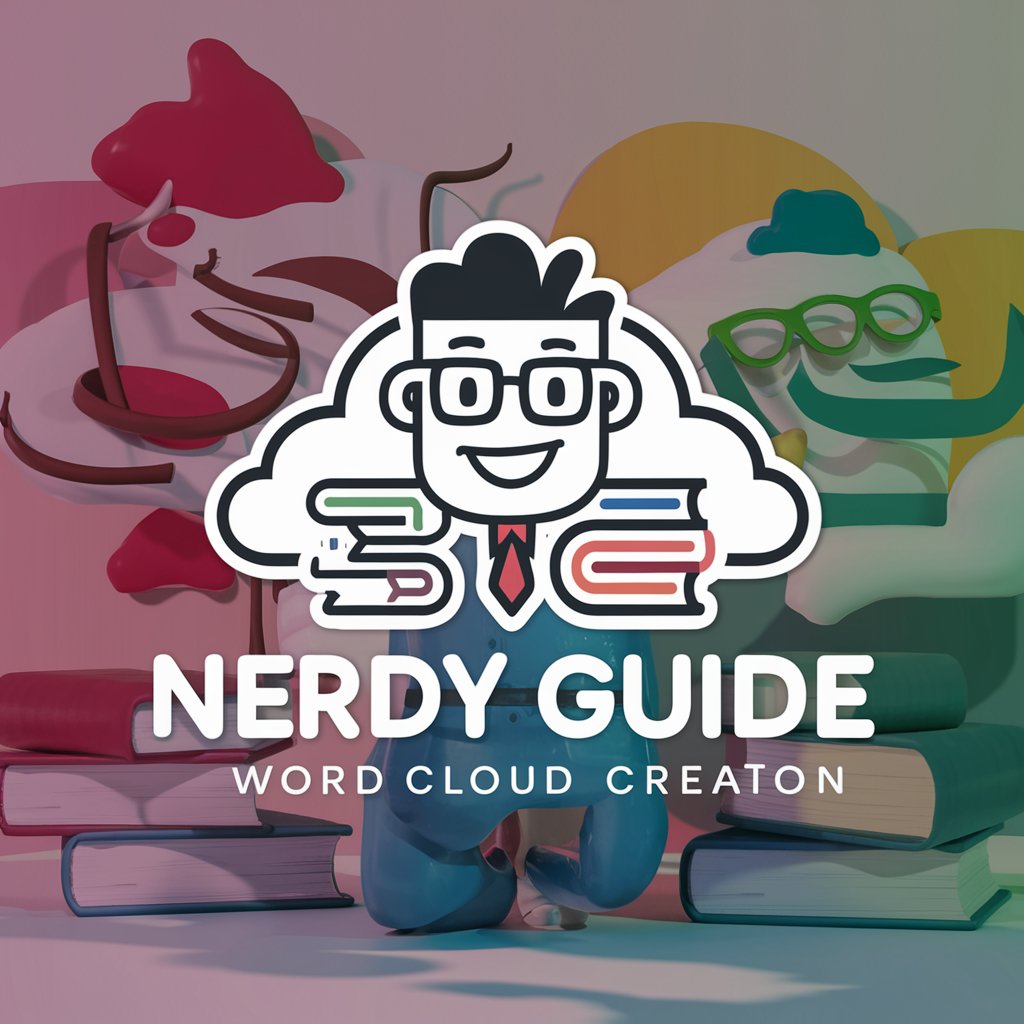
Pixel Prowess
Empowering Creativity with AI
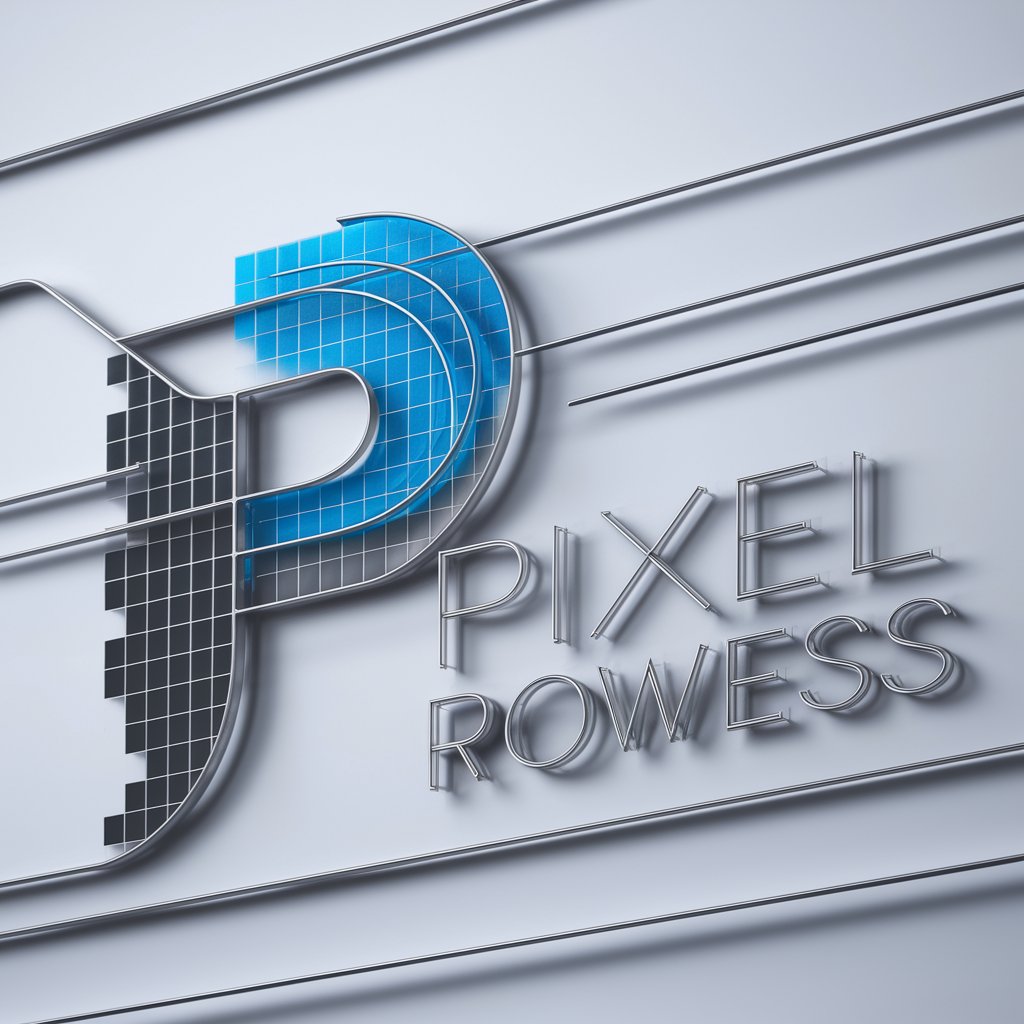
FAQs on Mastering Elm's Task Type
What is Elm's 'Task' type?
Elm's 'Task' type represents asynchronous operations that may fail, such as HTTP requests. Unlike JavaScript's Promises, Tasks defer execution until explicitly invoked, allowing for more controlled and predictable side effects management.
How do I chain multiple tasks in Elm?
To chain multiple tasks, use the `Task.andThen` function. This allows you to sequence tasks where the output of one task is the input to the next, enabling sophisticated asynchronous workflows with error handling.
Can 'Task' manage all types of side effects?
Yes, 'Task' is designed to manage a wide range of side effects, from HTTP requests to time-based operations. It provides a unified interface for handling asynchronous actions in a safe, Elm-like manner.
How do I handle errors in tasks?
Use `Task.onError` to handle errors within tasks. This function allows you to specify how to handle errors, either by recovering with a default value or chaining to another task for error recovery.
What are the best practices for debugging tasks?
For debugging, insert `Debug.log` statements before and after task execution points to inspect the task's state. Additionally, use Elm's strong type system to ensure your tasks are set up correctly, reducing runtime errors.