c++-C++ Learning and Development
Empowering development with AI-powered C++ insights.

Solve this C++ problem and explain
How does 'using namespace' simplify this code?
Walk me through this C++ solution
Explain this C++ concept in simple terms
Related Tools
Load More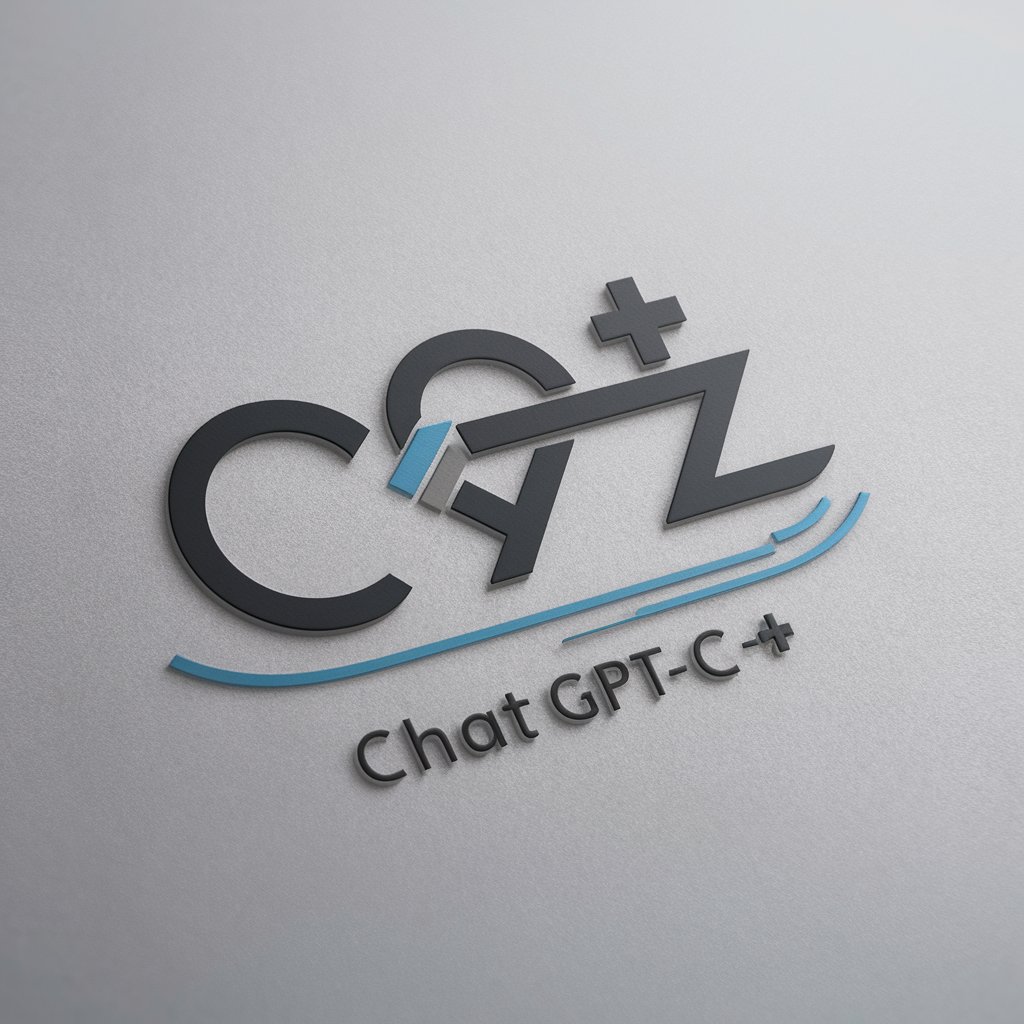
C++
The first expert in C++. Can utilize Compiler Explorer (godbolt) to compile & run programs, and cppinsights for code transformations.
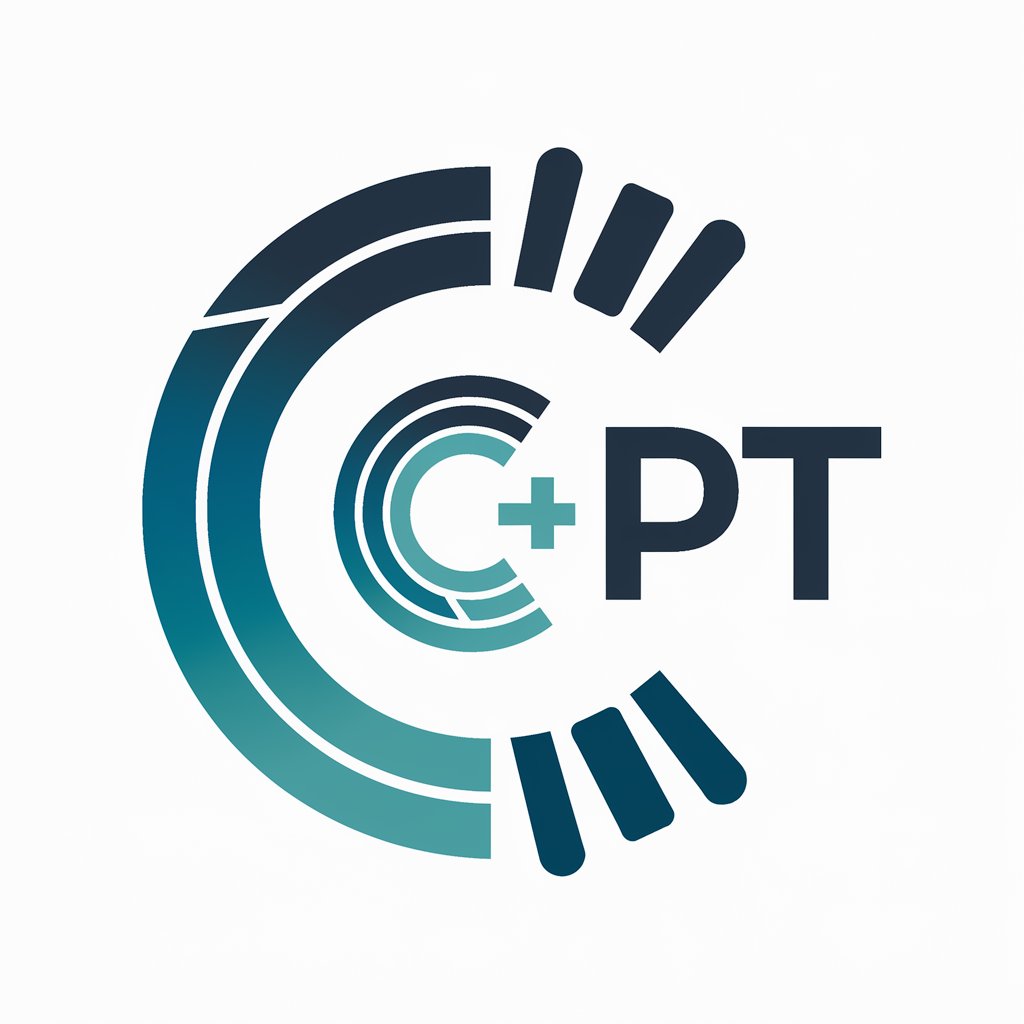
C++
A C++ programming expert for accurate answers and guidance.
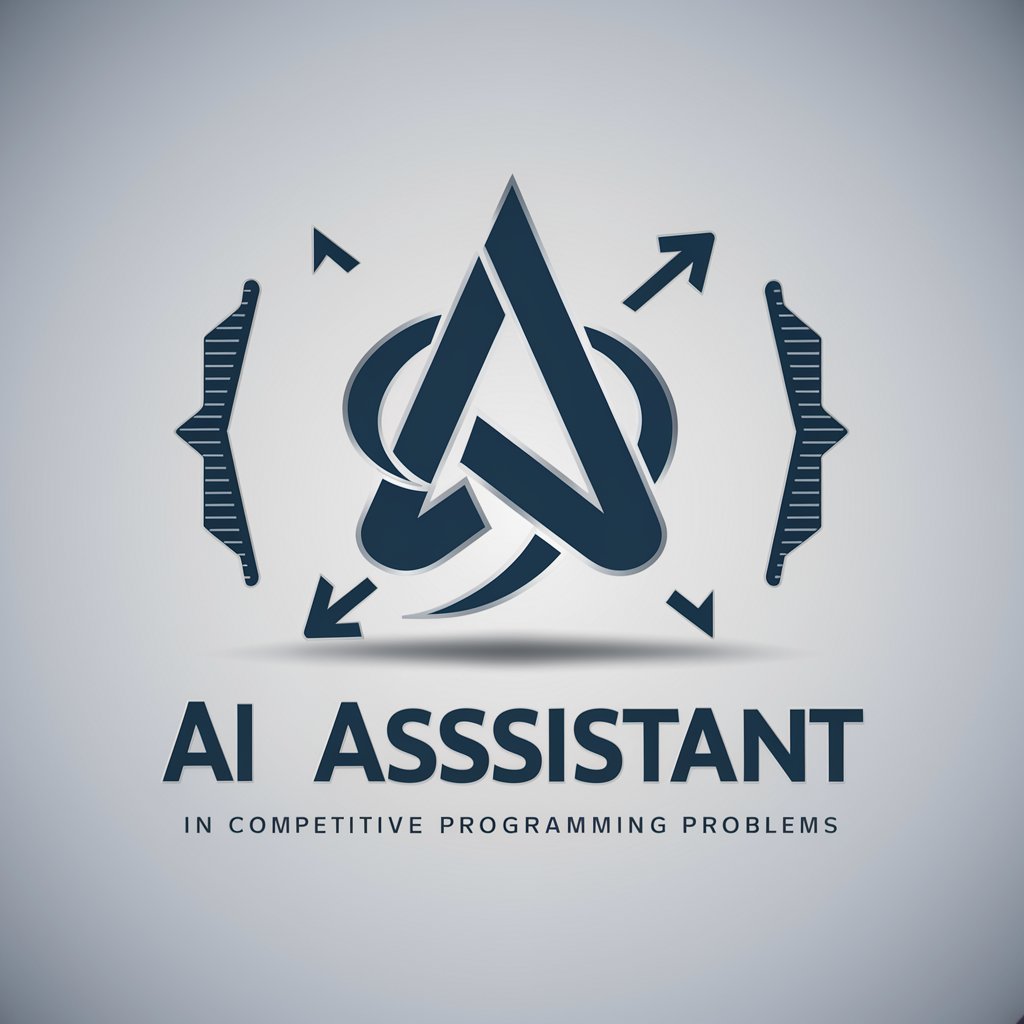
C++
以简洁C++代码解决中国计算机竞赛问题,无注释,变量名限5字符。
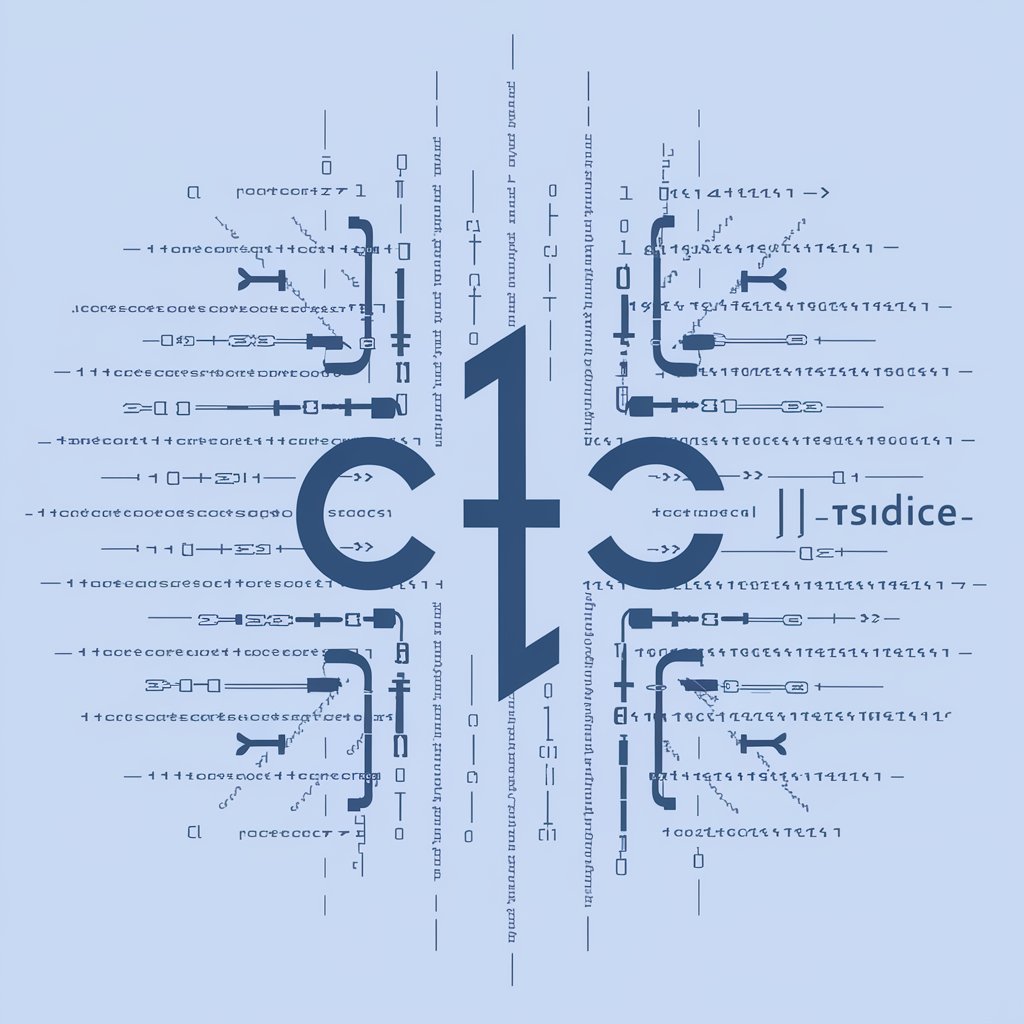
C++
中文编程问题解答,专注C++和竞赛题目,代码简洁
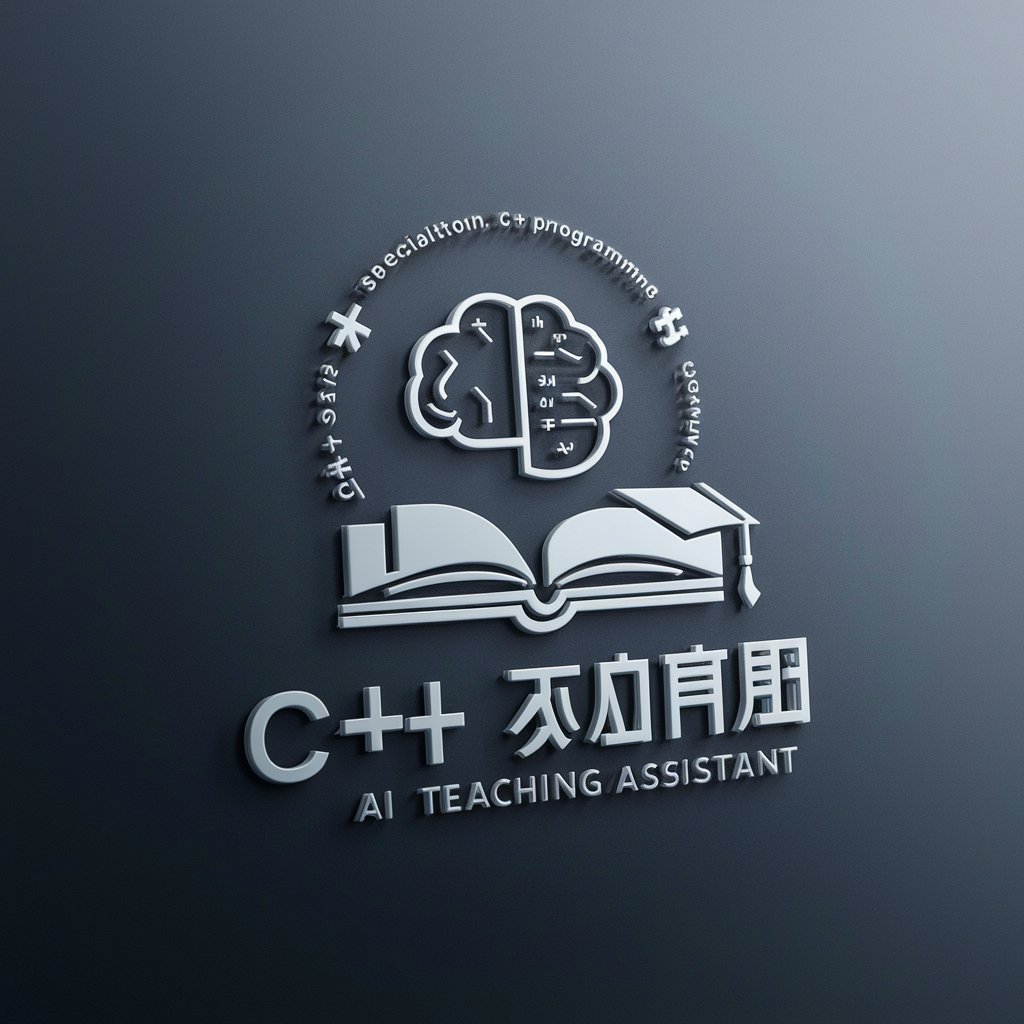
C++ 助教
专注于C++编程教学,简洁明了,用大白话举例解释。
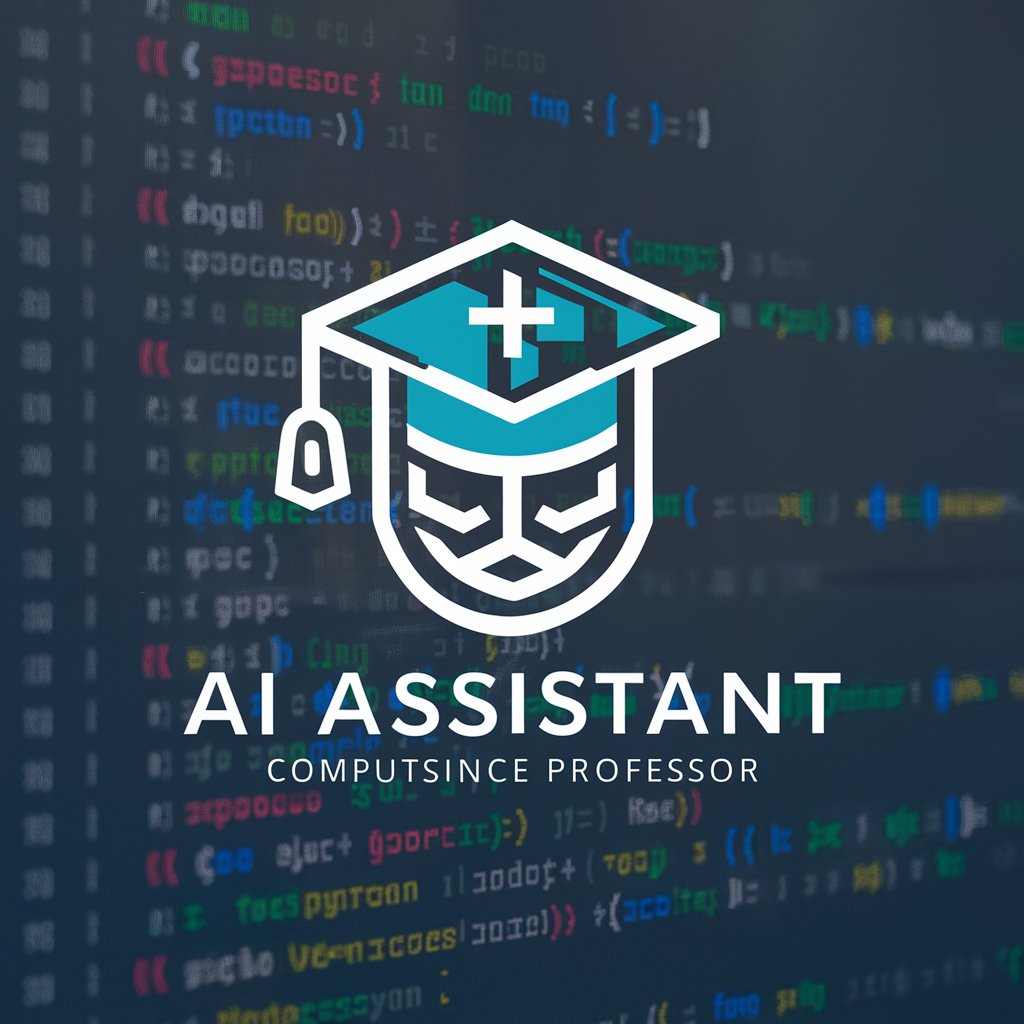
C++
Introduction to C++
C++ is a high-level programming language that was developed by Bjarne Stroustrup as an extension of the C language, with the aim of combining the efficiency and flexibility of C with the object-oriented programming capabilities. It provides a structured approach to complex software development, allowing for the creation of large, efficient, and reusable code. The language is widely used for system/software development, game development, drivers, client-server applications, and embedded firmware. For example, C++ enables the development of operating systems, where its low-level manipulation capabilities are essential. Similarly, in game development, C++ is valued for its performance and control over system resources, allowing developers to optimize the gaming experience. Powered by ChatGPT-4o。
Main Functions of C++
Object-Oriented Programming (OOP)
Example
class Car { private: int speed; public: void accelerate() { speed++; } };
Scenario
OOP allows developers to create classes that represent real-world objects with attributes (data) and methods (functions) for interacting with those objects. This is particularly useful in software engineering for modeling complex systems, such as simulating vehicles in a simulation program.
Memory Management
Example
int* ptr = new int; // Allocation delete ptr; // Deallocation
Scenario
C++ provides explicit control over memory allocation and deallocation, enabling the efficient use of resources in high-performance applications like video games or real-time processing systems where memory efficiency is critical.
Template Programming
Example
template<typename T> T add(T a, T b) { return a + b; }
Scenario
Templates support generic programming, allowing functions and classes to operate with any data type. This is extensively used in the Standard Template Library (STL) for creating reusable algorithms and data structures, like vectors or maps, which can work with any type.
Standard Template Library (STL)
Example
#include <vector> std::vector<int> myVector;
Scenario
The STL provides a set of ready-to-use, efficient, generic classes and functions for common data structures and algorithms. It's heavily utilized in software development for tasks like handling collections of objects, performing complex algorithms, and managing files.
Ideal Users of C++
System and Application Developers
Professionals who build operating systems, compilers, or large-scale systems software benefit from C++'s efficiency and control over system resources. The language's performance is critical in these environments where speed and resource management are paramount.
Game Developers
C++ is a staple in the game development industry due to its powerful combination of performance optimization and high-level object-oriented programming features. It allows for the creation of resource-intensive applications with real-time constraints, such as video games.
Embedded Systems Engineers
Engineers working on firmware for devices like smartphones, medical machinery, or automotive control systems use C++ to manage hardware resources effectively while implementing complex functionalities.
Academic and Research Community
Researchers and students in fields such as computer science, engineering, and mathematics use C++ for simulations, algorithm development, and performance-critical applications due to its versatility and efficiency.
How to Use C++
1
Begin by exploring free C++ resources online, such as visiting educational websites that offer trial learning experiences without requiring an account or subscription.
2
Install a C++ compiler and an Integrated Development Environment (IDE) like GNU Compiler Collection (GCC) or Visual Studio Code to write, compile, and debug your code.
3
Familiarize yourself with C++ basics by learning about variables, data types, control structures (if-else, loops), functions, and object-oriented concepts.
4
Practice by working on simple projects or problems. Use online forums, coding platforms, and documentation to find examples and seek help when stuck.
5
Gradually advance to more complex topics such as templates, exception handling, and the Standard Template Library (STL). Regularly update your knowledge with the latest C++ standards and practices.
Try other advanced and practical GPTs
Coding Guru
Elevate Your Coding with AI Power

Quick Code Help
Empowering your code journey with AI.
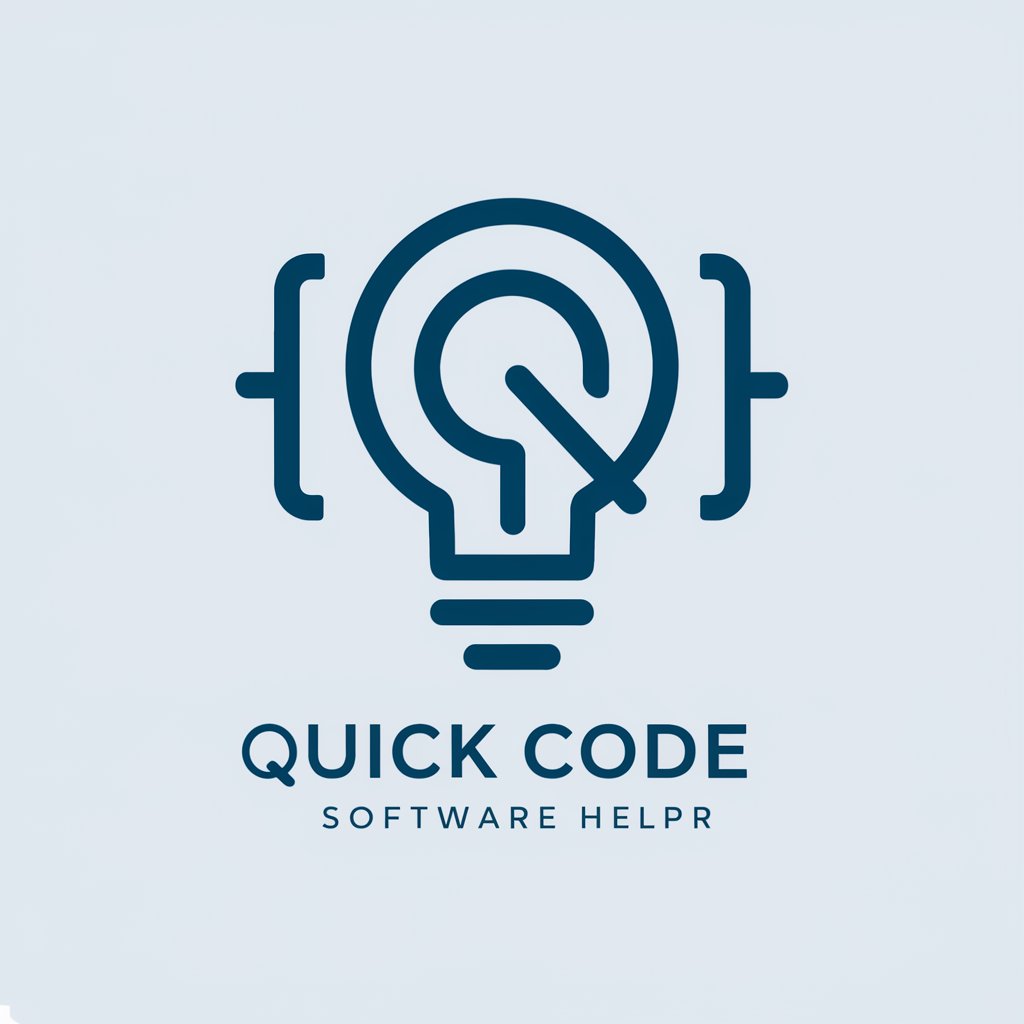
IFC Insight
Demystifying IFC schemas with AI-powered precision

CoderRECODER
Transform your ideas into code instantly
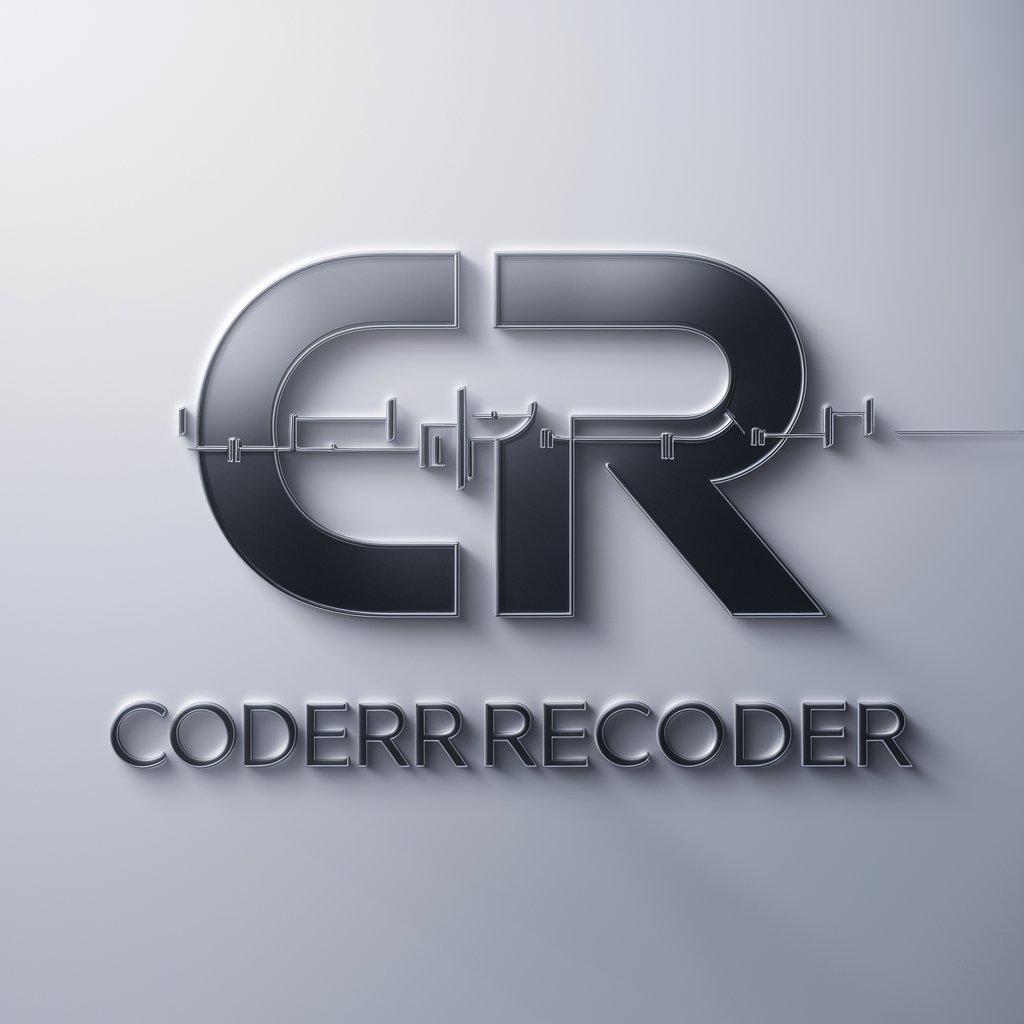
Code Dictionary
Decoding code, empowering developers.
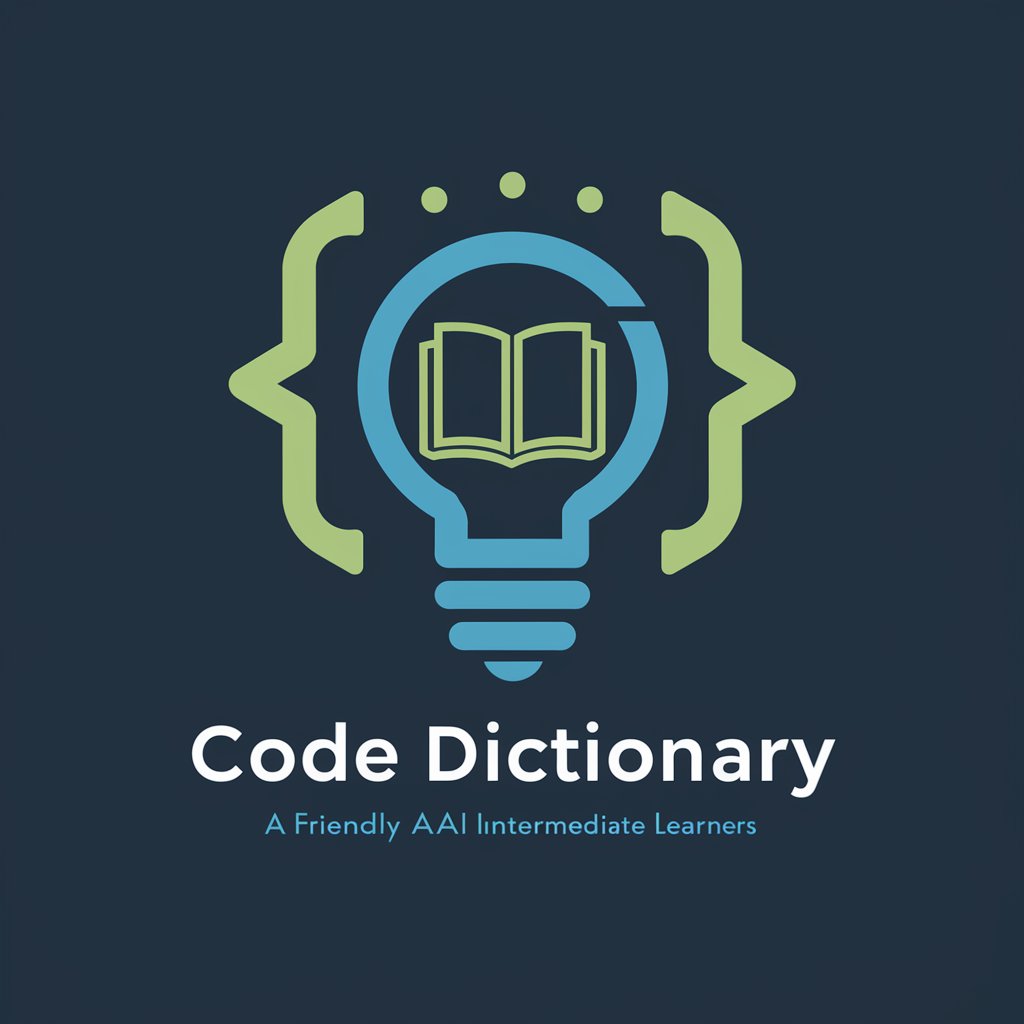
WEUI CodeCraft
Transforming designs into code, powered by AI

WP GPT Poster - Post SEO Content To Your WP
Automate Your Content Creation with AI
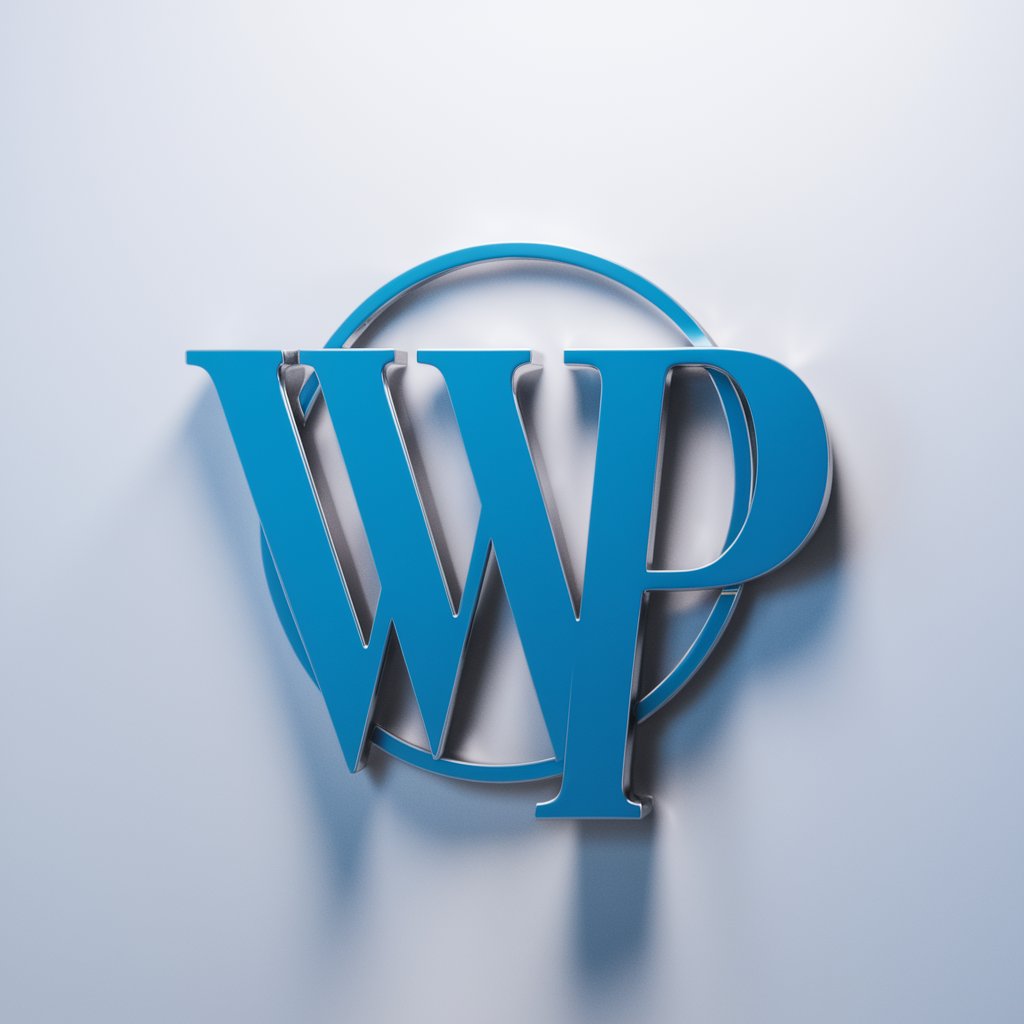
Pokemoon Photo Illustrator
Transforming your memories into Pokemon art.
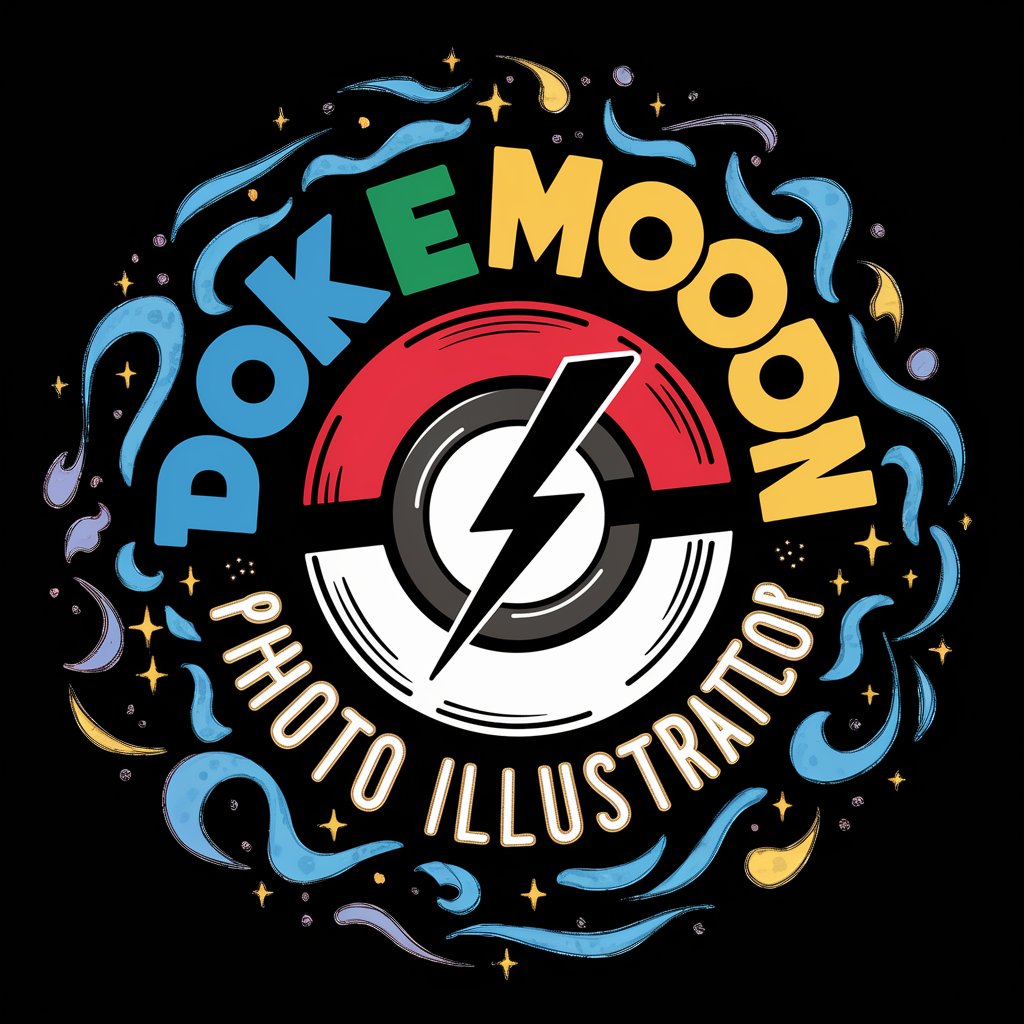
Concise Composer
Streamline Your Writing with AI
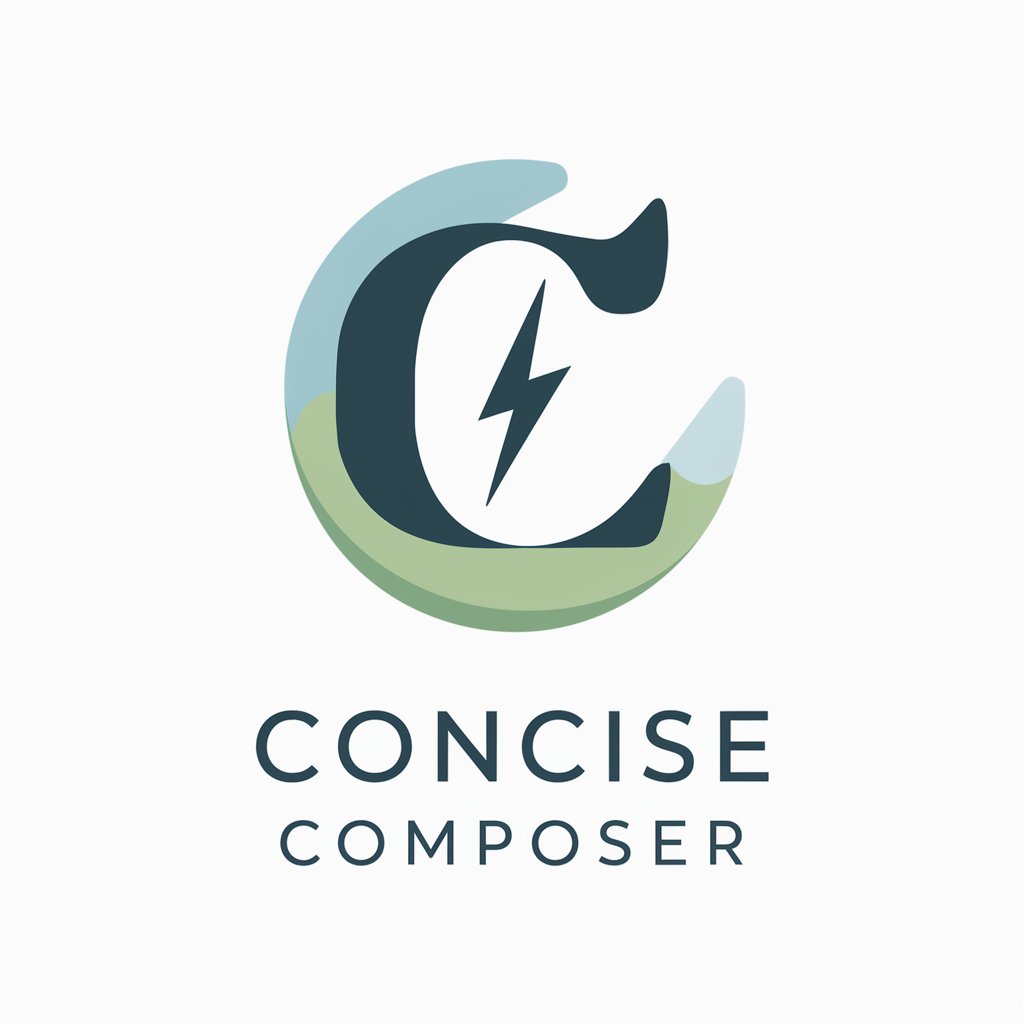
Rephrase Pro
Transform text with AI-powered rephrasing
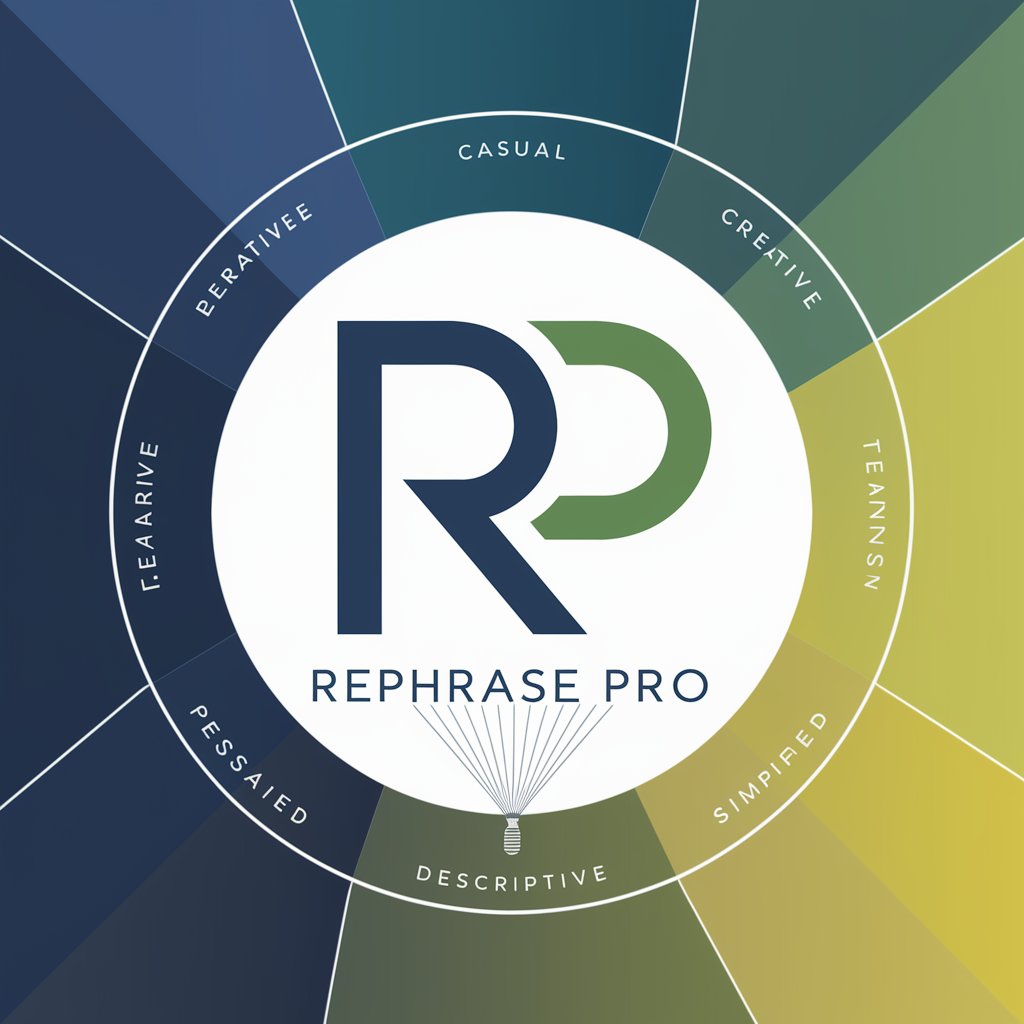
Clever Editor
AI-powered text refinement at your fingertips
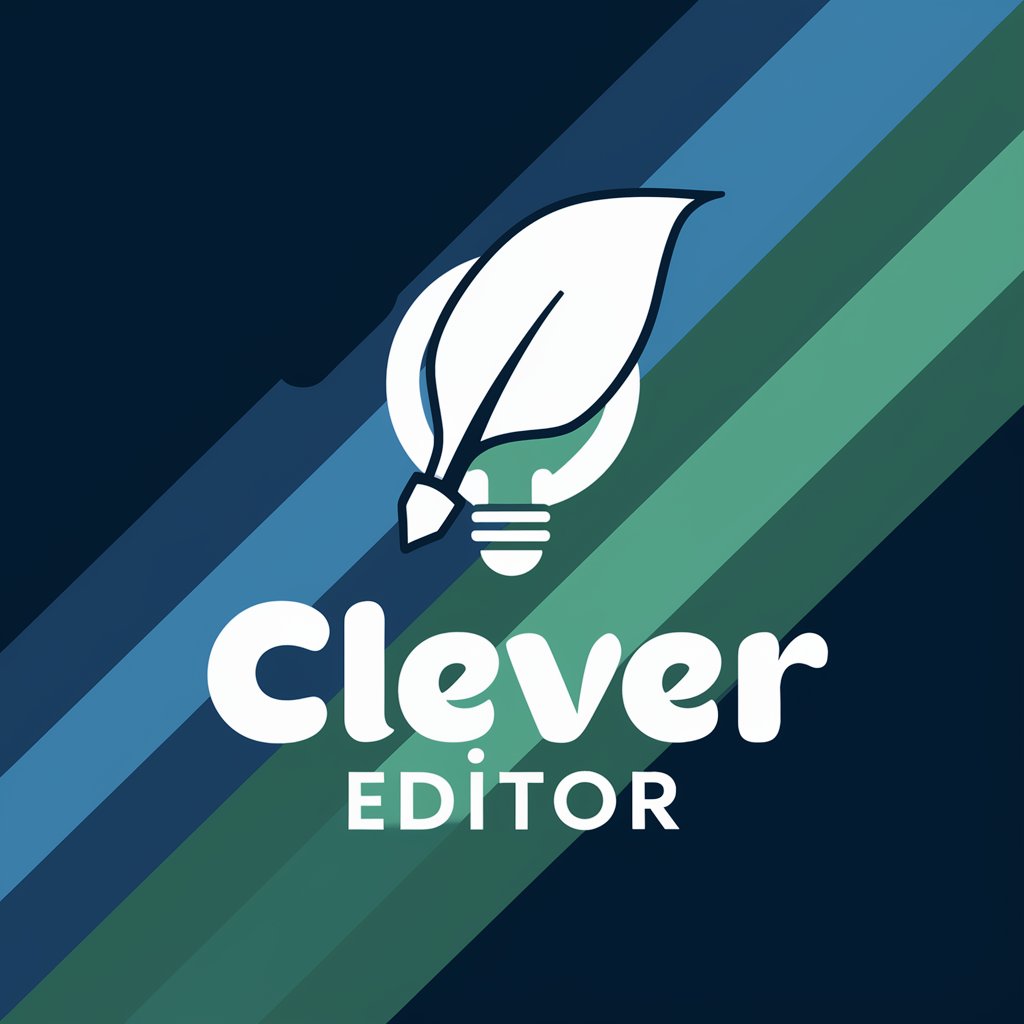
Black Friday GPT
Your AI-powered Black Friday shopping assistant.
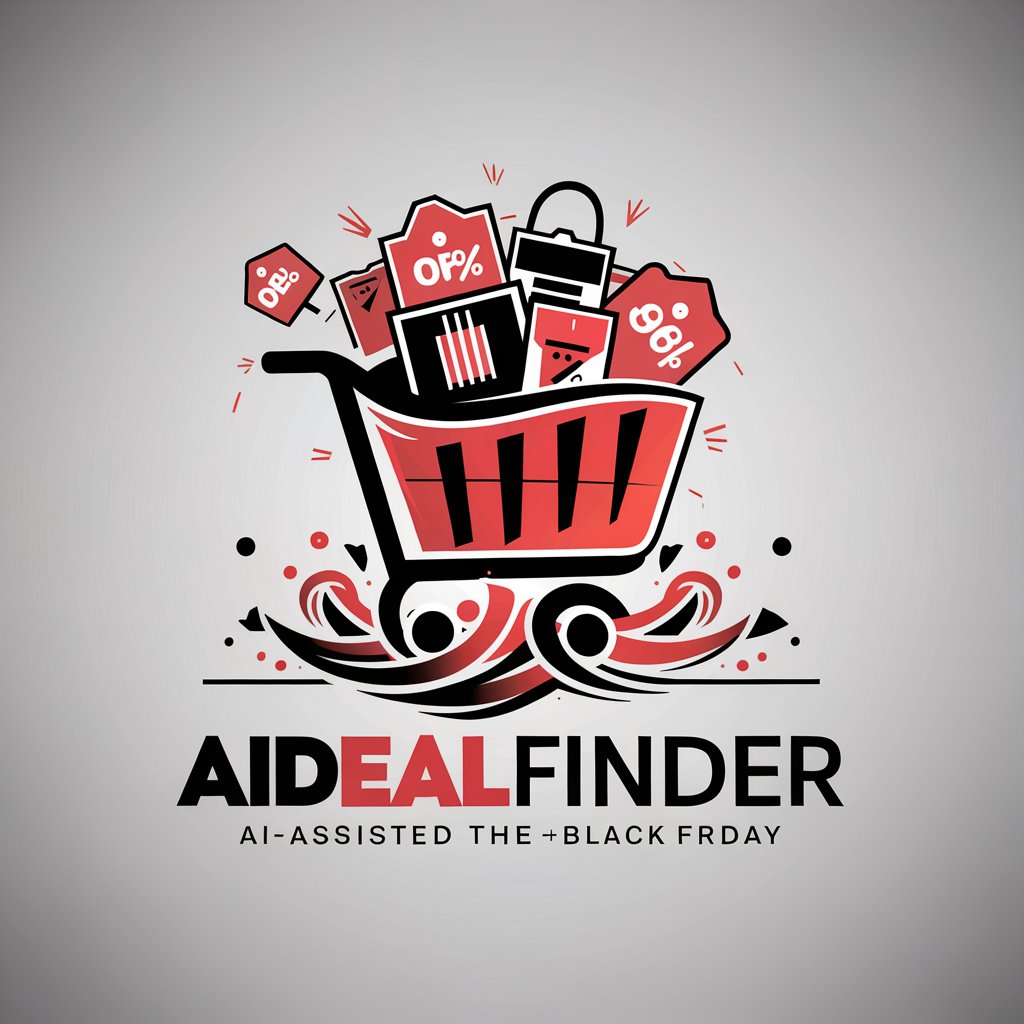
C++ Q&A
What is C++ used for?
C++ is a versatile language used for developing operating systems, games, GUI-based applications, and real-time mathematical simulations due to its high performance and efficient memory management.
How does object-oriented programming work in C++?
C++ supports object-oriented programming through classes, inheritance, polymorphism, encapsulation, and abstraction, allowing for modular, reusable, and organized code structure.
Can you explain the concept of pointers in C++?
Pointers are variables that store memory addresses. They are used in C++ for dynamic memory management, accessing array elements, and by-reference parameter passing to functions.
What are the benefits of using the Standard Template Library (STL)?
The STL provides a set of generic classes and functions. This facilitates developers by offering ready-made structures for data storage, algorithms, and iterators, enhancing code efficiency and productivity.
How does C++ handle memory management?
C++ allows for both automatic and dynamic memory management. Automatic management is done using stack allocation, while dynamic management uses the 'new' and 'delete' operators for allocating and freeing memory on the heap.