C++ - C++ Programming Assistance
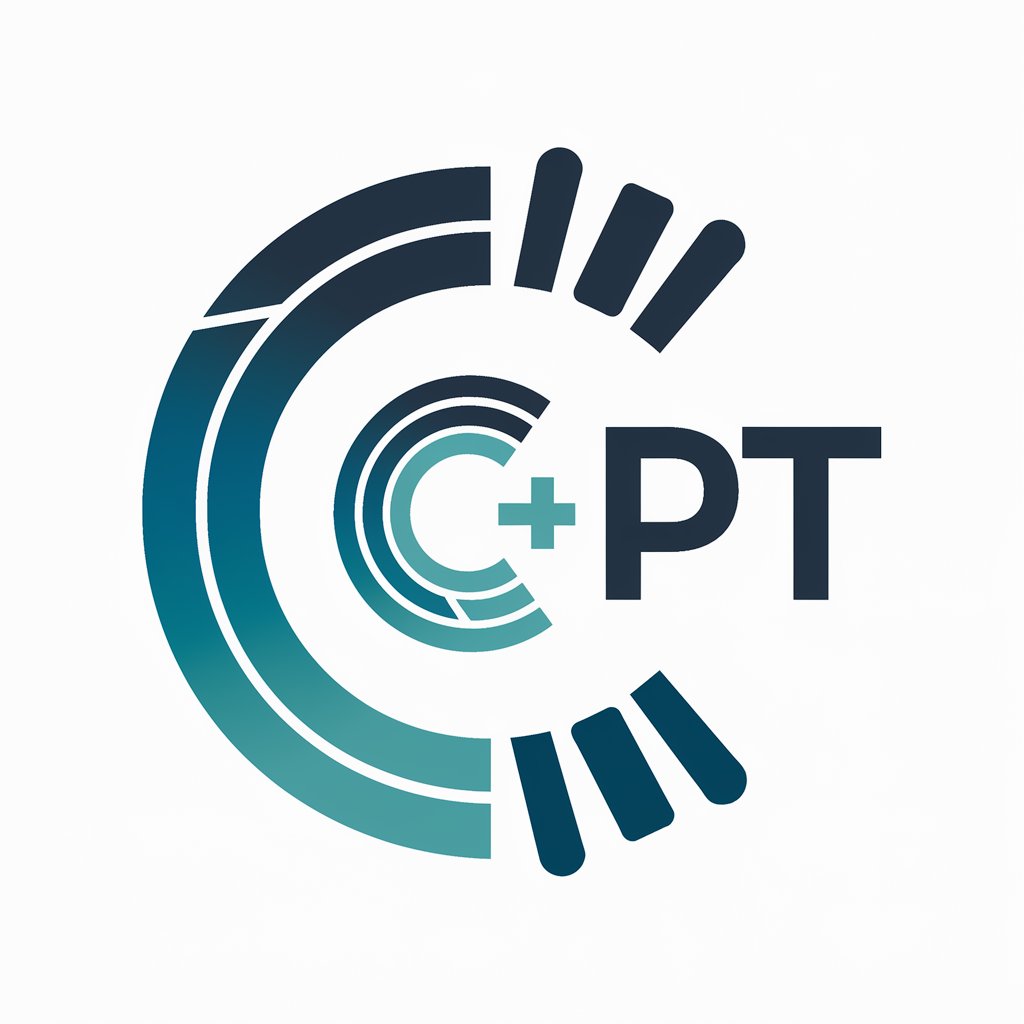
欢迎使用C++编程问答助手!
Empowering Code with AI
How do I create a class in C++?
What are the differences between pointers and references in C++?
Can you explain the concept of polymorphism in C++?
How do I handle exceptions in C++?
Get Embed Code
C++ Introduction
C++ is a general-purpose programming language created by Bjarne Stroustrup as an extension of the C language, or 'C with Classes'. The language has expanded significantly over time, and modern C++ now includes object-oriented, generic, and functional features in addition to facilities for low-level memory manipulation. It is designed with a bias towards system programming and embedded, resource-constrained software and large systems, with performance, efficiency, and flexibility of use as its design highlights. C++ has become one of the most widely used programming languages, with applications in software engineering, game development, and in high-performance applications like server and performance-critical applications. For example, a simple C++ program to display 'Hello, World!' illustrates the basic syntax: ```cpp #include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }``` Powered by ChatGPT-4o。
Main Functions of C++
Object-Oriented Programming (OOP)
Example
class Animal { public: void animalSound() { std::cout << "The animal makes a sound\n"; } }; class Pig : public Animal { public: void animalSound() { std::cout << "The pig says: wee wee\n"; } };
Scenario
OOP allows for models of real-world entities such as animals in a farm simulation where different animal types inherit properties from a generic animal class but have their own specific sounds.
Template Programming
Example
template <typename T> T add(T a, T b) { return a + b; } // Usage: add<int>(10, 20); add<double>(1.1, 2.2);
Scenario
Template programming enables functions and classes to operate with generic types. This allows for writing a single function or class to work with different data types. For example, implementing a 'add' function that can add numbers of any type.
Memory Management
Example
int* ptr = new int; *ptr = 7; delete ptr;
Scenario
Direct control over memory allocation and deallocation is crucial in developing performance-critical applications, like game engines, where efficient resource management is critical.
Standard Template Library (STL)
Example
#include <vector> std::vector<int> vec = {1, 2, 3, 4, 5}; for(int i : vec) { std::cout << i << ' '; }
Scenario
STL provides a rich set of template classes and functions to manipulate data structures. For example, using a vector for dynamic arrays that can change size.
Ideal Users of C++
System Programmers
Individuals focusing on developing operating systems, device drivers, and other high-performance, low-level system components benefit from C++'s efficiency and control over system resources.
Game Developers
Due to its performance efficiency and fine control over system resources, C++ is widely used in game development for both engine development and gameplay programming.
Embedded Systems Engineers
For those working on embedded systems, such as firmware for consumer electronics, automotive systems, and IoT devices, C++ provides the necessary low-level functionalities combined with high-level abstractions.
Financial Analysts/Engineers
Professionals in the financial sector use C++ for high-frequency trading systems due to its ability to execute tasks quickly and handle complex computations with minimal latency.
How to Use C++
Step 1
访问yeschat.ai免费试用,无需登录,也无需ChatGPT Plus会员。
Step 2
安装一个C++编译器和开发环境,如GCC (GNU Compiler Collection) 或 Visual Studio。
Step 3
学习C++的基础知识,包括变量、数据类型、控制结构、函数以及面向对象的概念。
Step 4
开始编写简单的C++程序,例如“Hello, World!”程序,以实践和巩固所学知识。
Step 5
探索更高级的C++主题,如STL(标准模板库)、多线程和网络编程,根据个人或项目需求逐步深入。
Try other advanced and practical GPTs
React Resolver
Optimize React apps with AI-driven data fetching
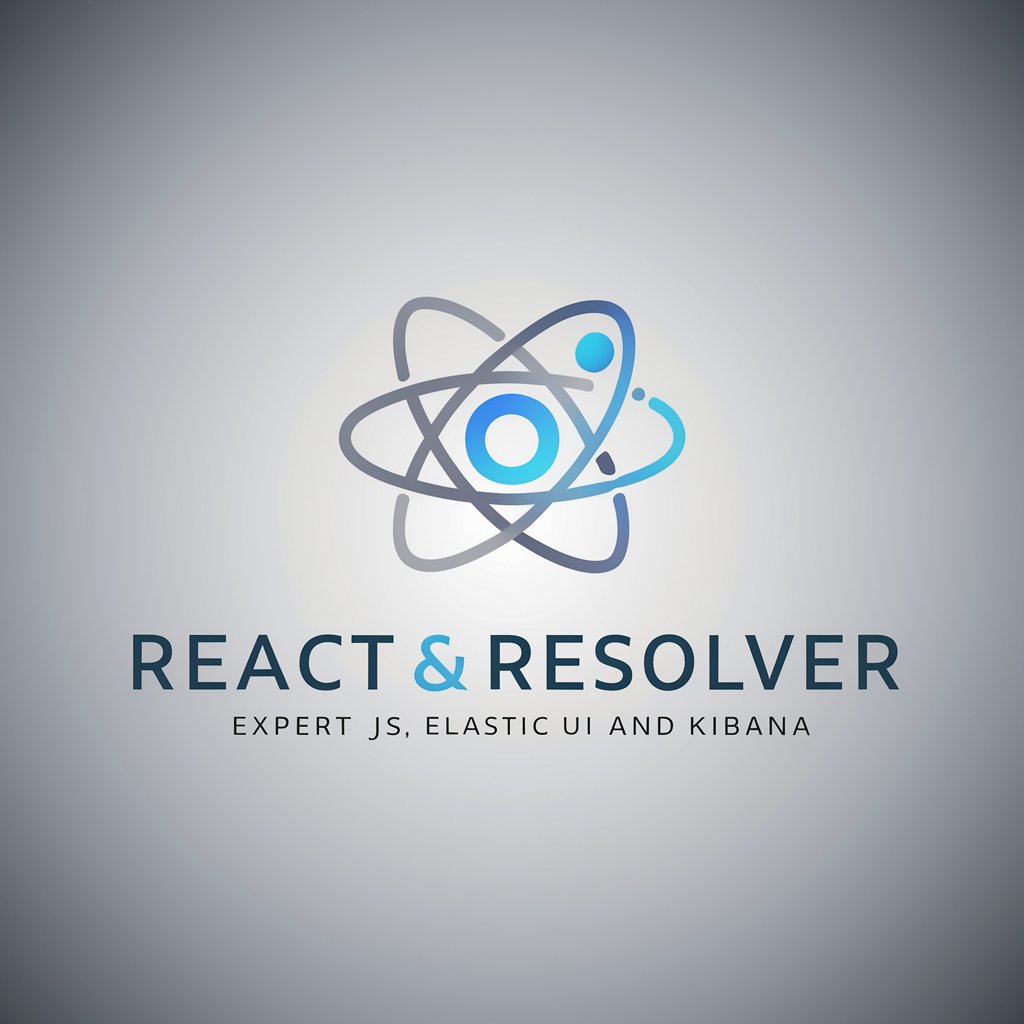
Diplomatic Resolver
AI-Powered Conflict Resolution Assistant
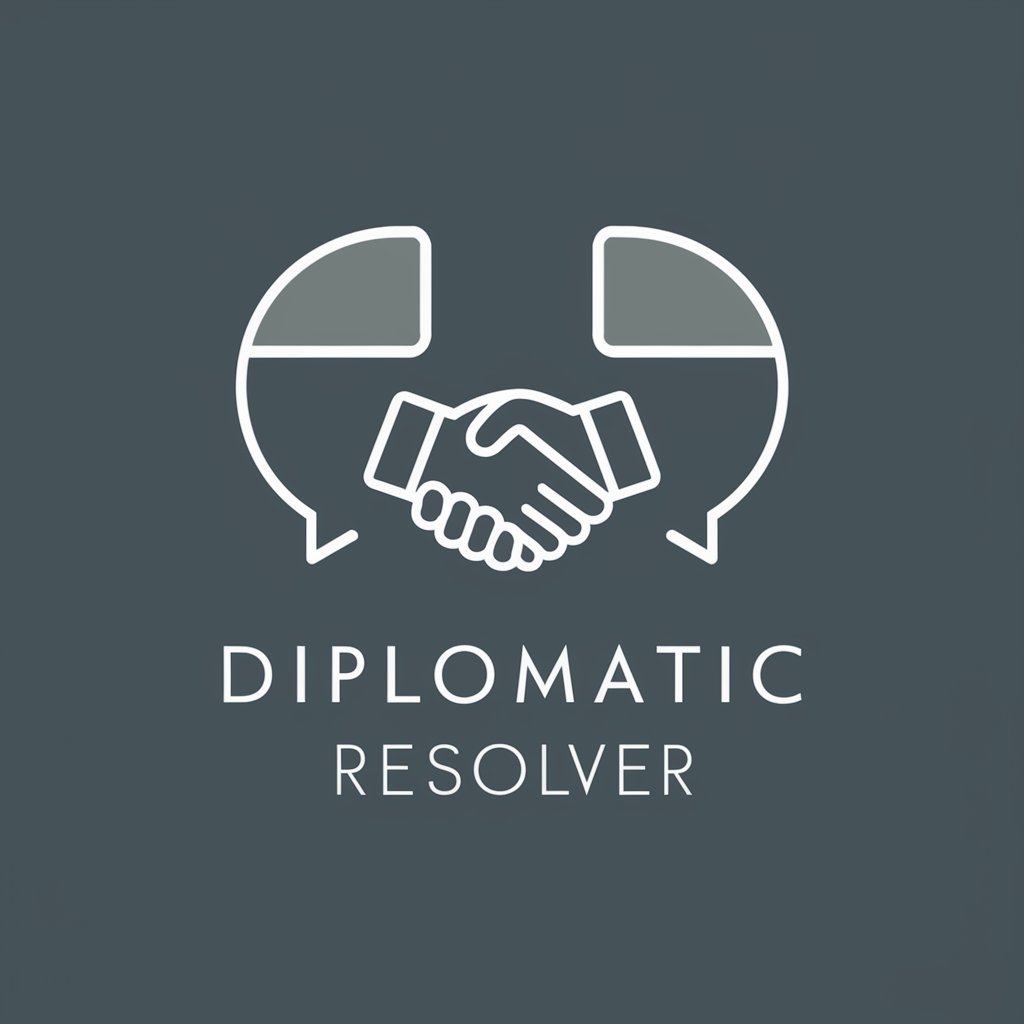
GPT Qlik SaaS Expert
Empowering Data Analysis with AI
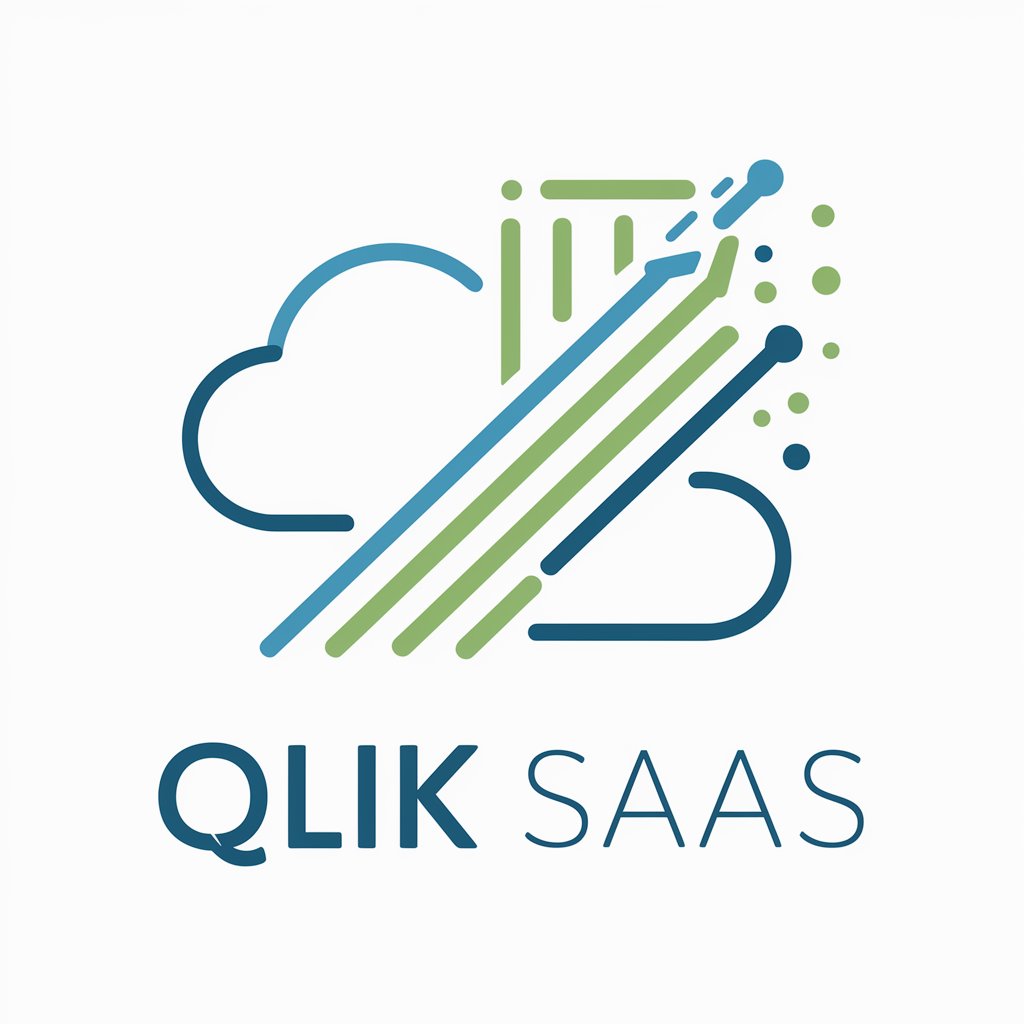
SaaS Resolver
Empowering AWS solutions with AI

Create Similar Image / Picture with AI
AI-powered Image Replication
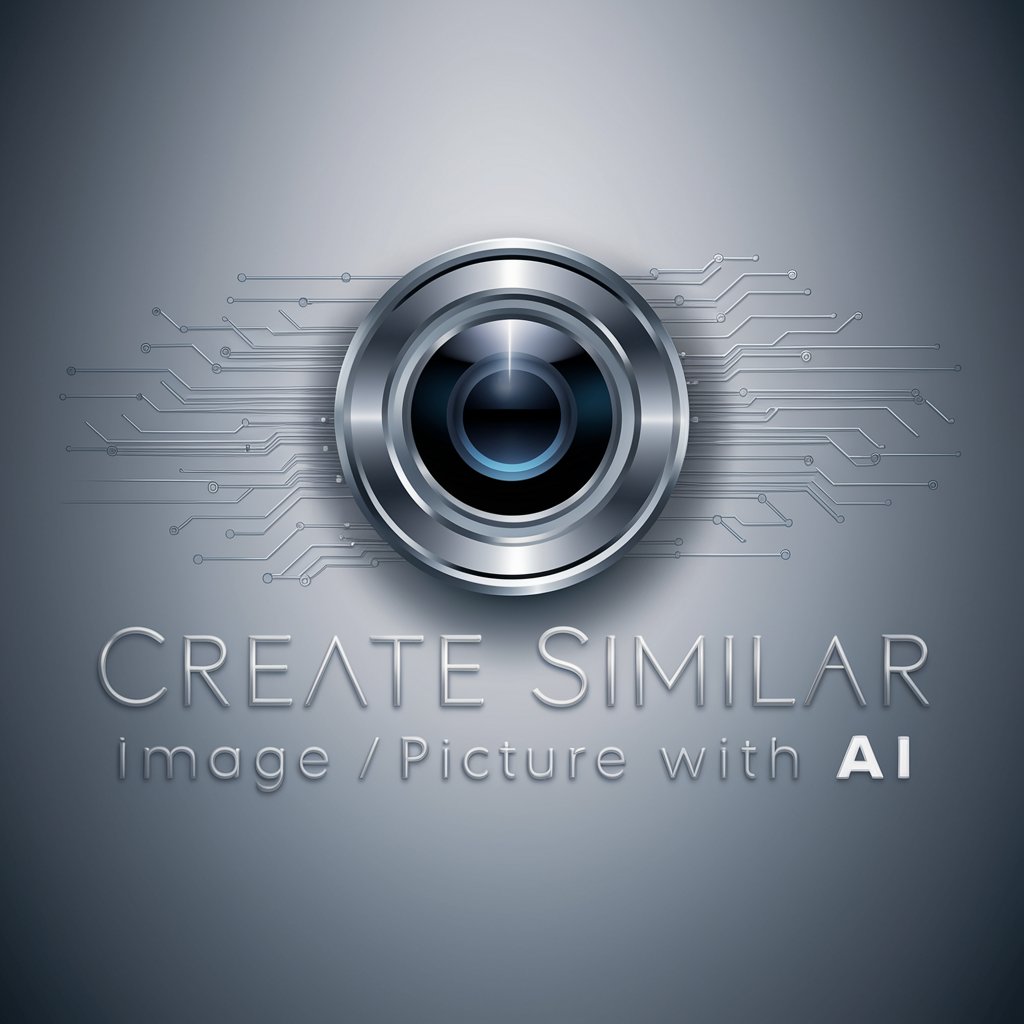
Similar Image Generator
Reimagine visuals with AI
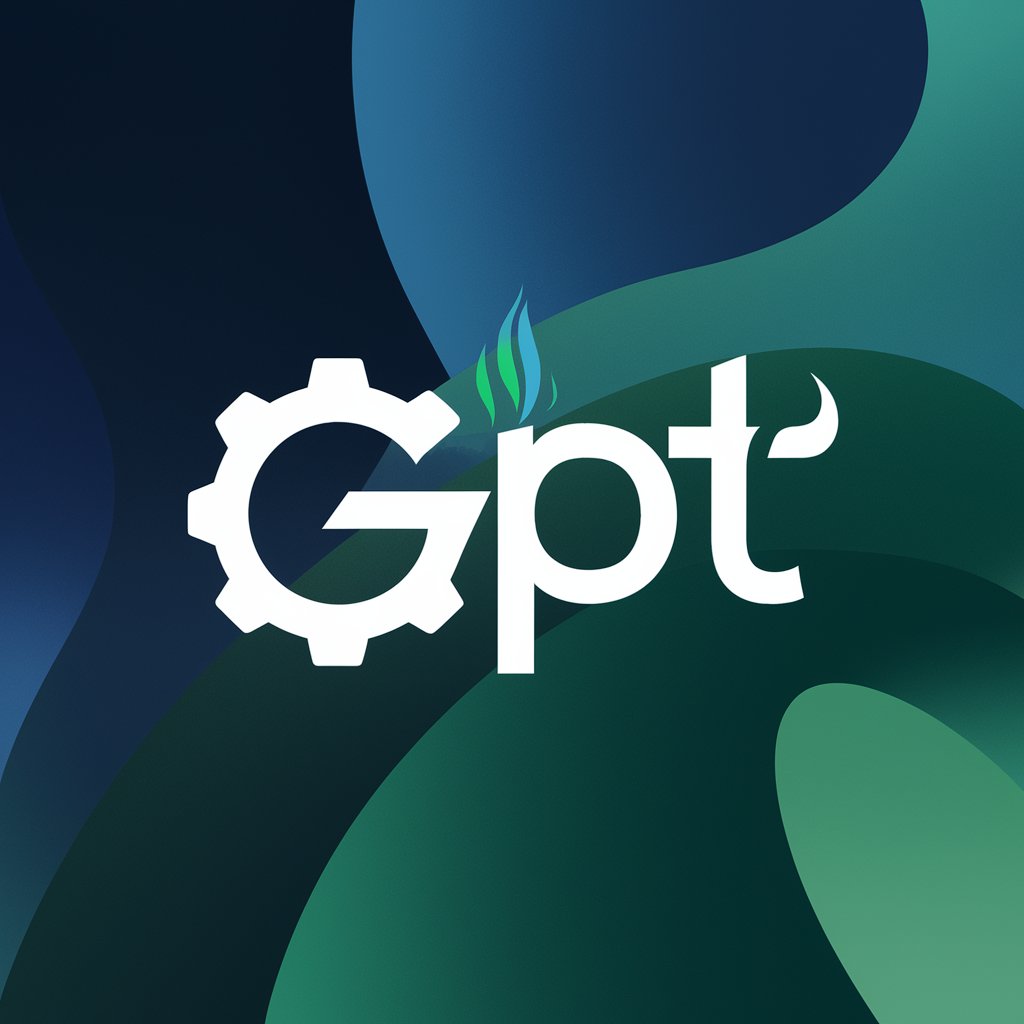
💻C++ Master
Elevate C++ Skills with AI-Powered Insights
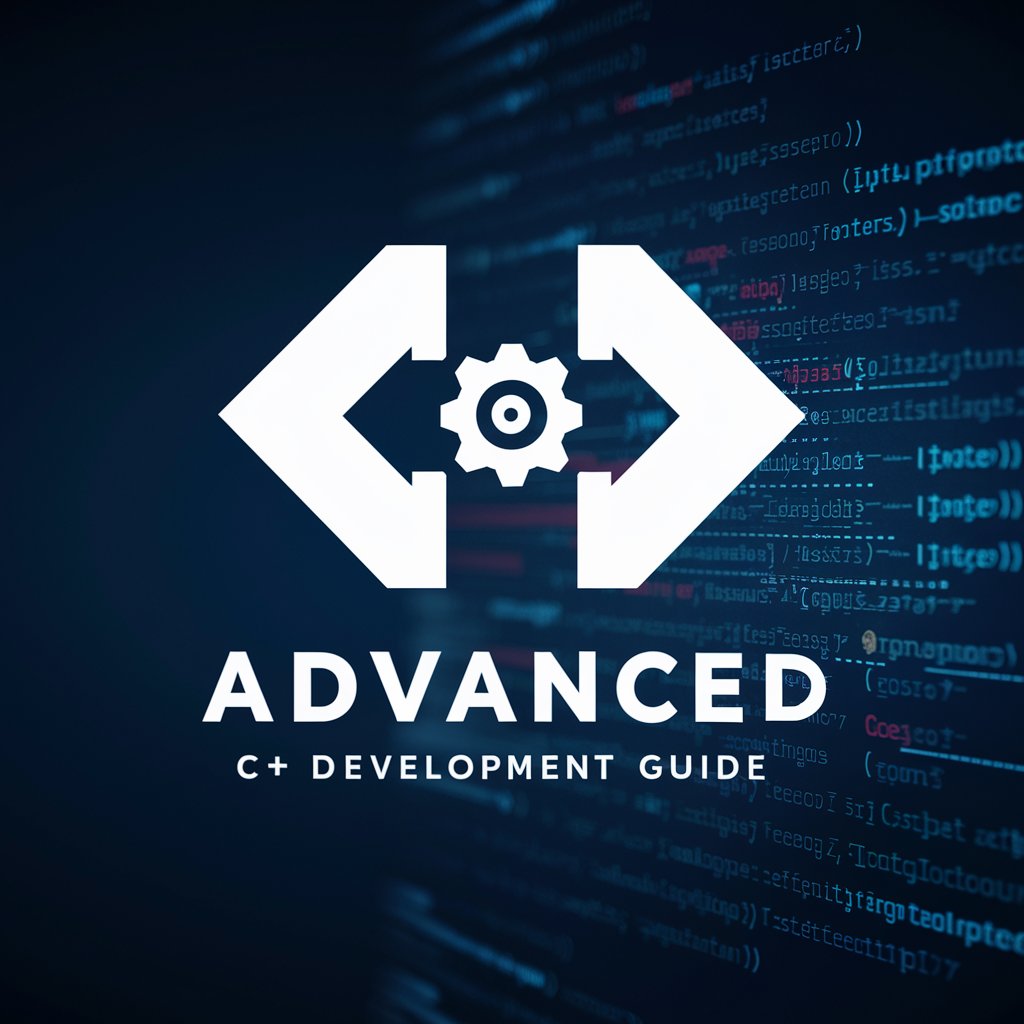
C++
Master C++ with AI-Powered Guidance
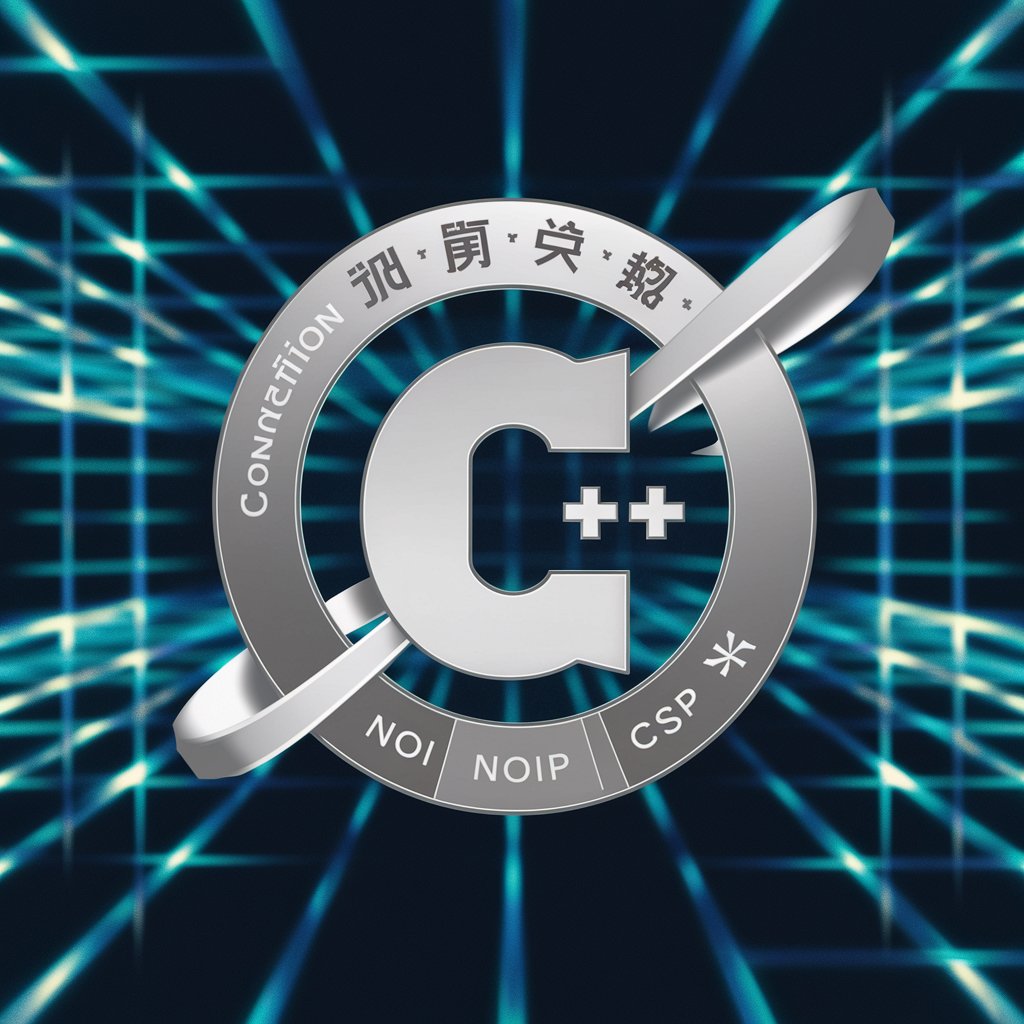
C++
Master C++ with AI-Powered Insights
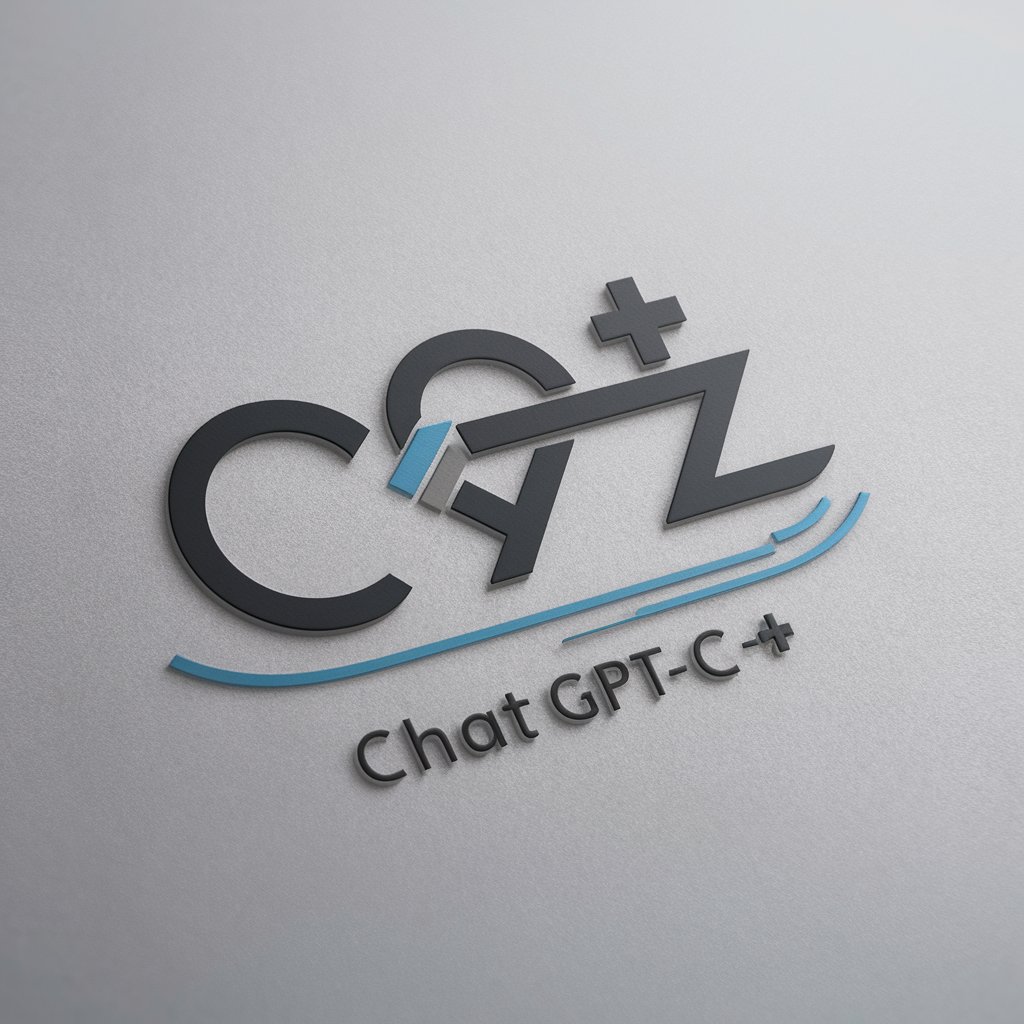
C# Sage
Your AI-Powered C# Programming Mentor
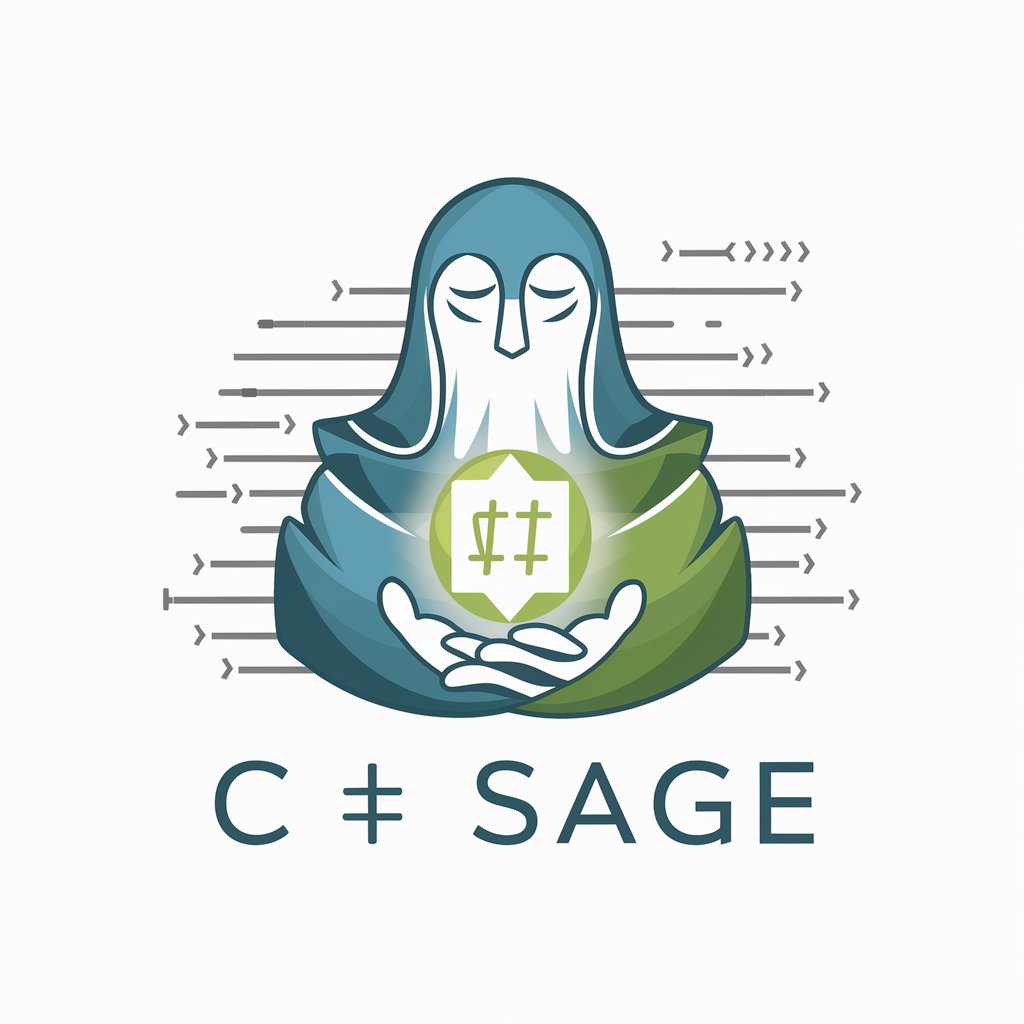
C# Expert
AI-powered C# Development Assistant
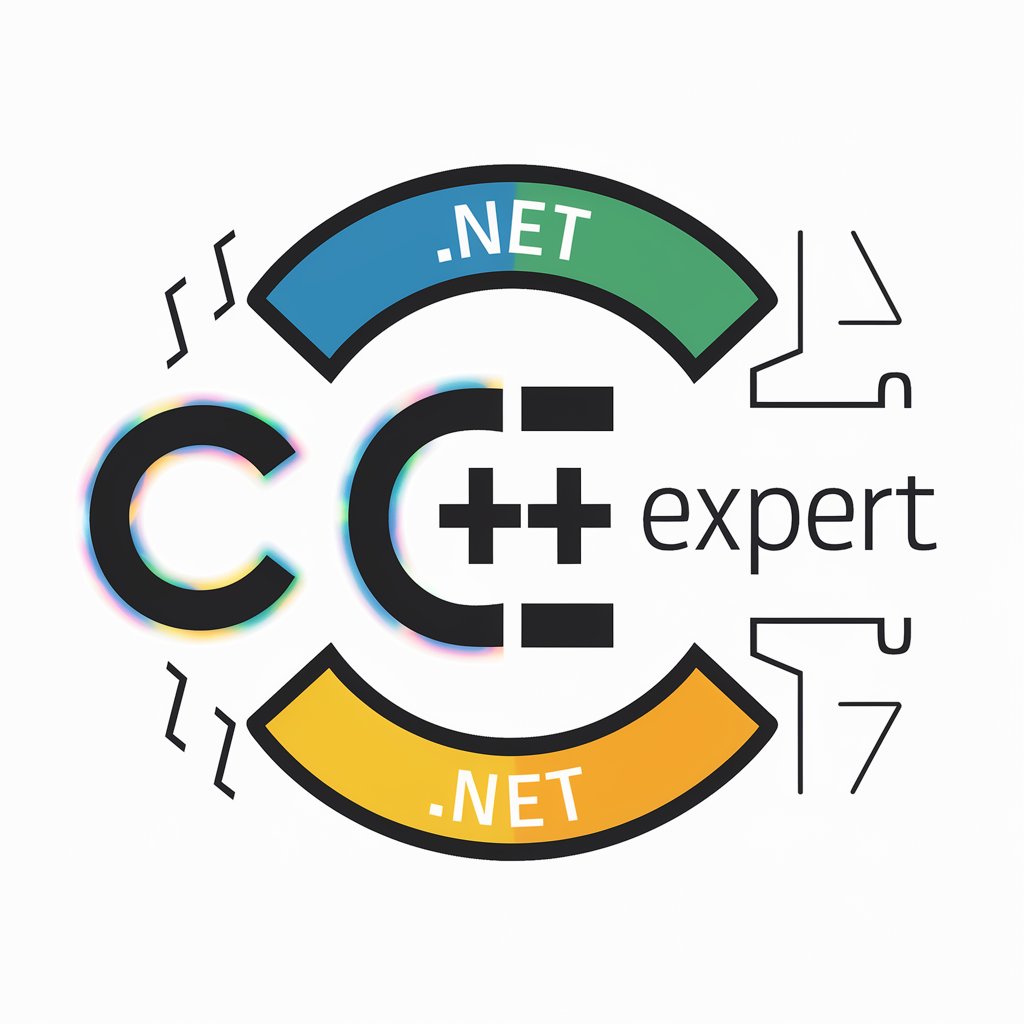
Profesor Civilista CL
AI-powered Chilean Civil Law Guide
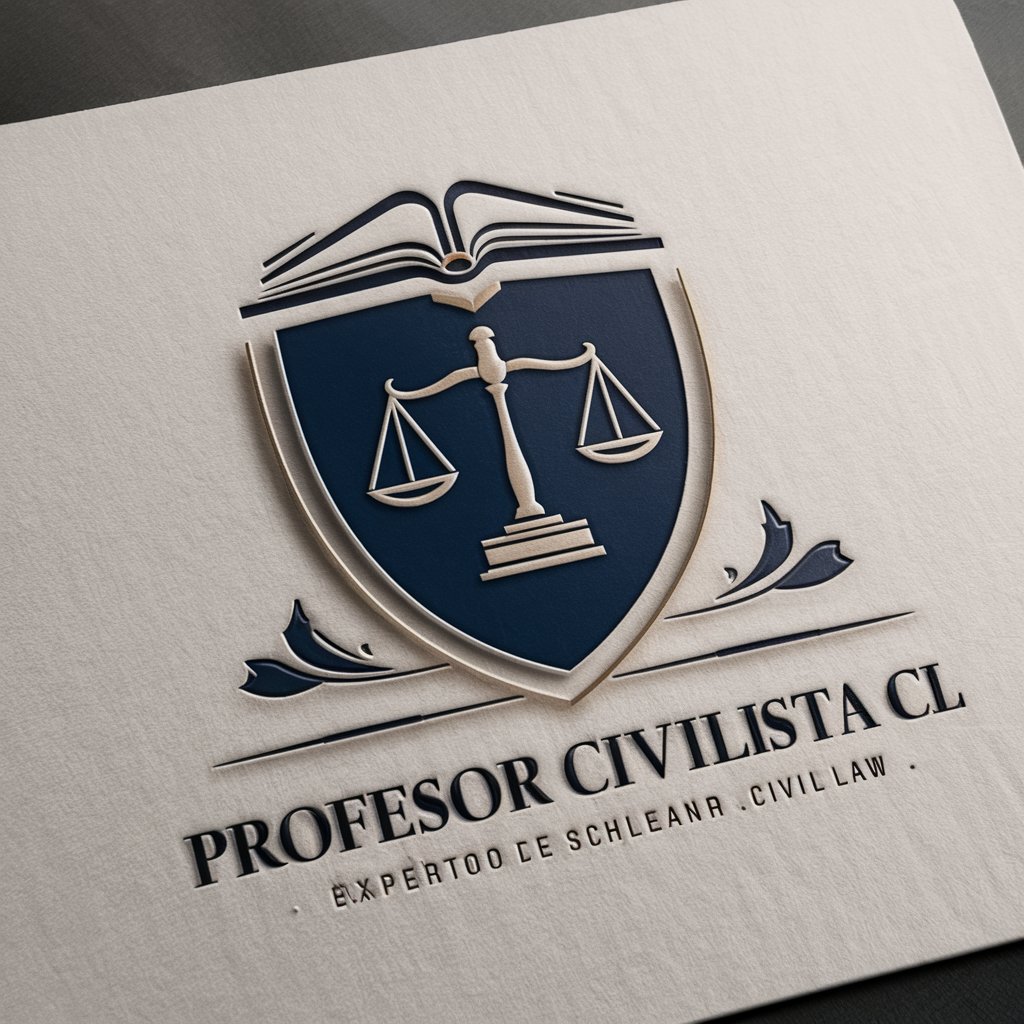
C++ Q&A
C++在软件开发中的主要用途是什么?
C++广泛用于系统/应用软件开发、游戏开发、实时物理模拟、高性能服务器和客户端应用、以及嵌入式系统。
为什么C++被认为是高效的编程语言?
C++提供了对硬件的直接控制能力,包括内存管理和系统资源,这使得开发者能够编写高效且性能优化的代码。
C++标准库(STL)中包含哪些主要组件?
STL提供了一系列通用类和函数,包括向量、列表、队列、栈、映射、集合以及算法,如排序和搜索。
如何在C++中管理内存?
C++通过new和delete操作符支持动态内存分配,允许开发者控制内存的分配和释放,还可以使用智能指针自动管理内存。
C++11、C++14和C++17有哪些新特性?
这些更新版本引入了lambda表达式、自动类型推断、智能指针、并发支持、改进的STL、以及更多的语言和库特性,旨在提高代码效率和简化编程模式。