Java Polymorphism Power: Overload & Override-Java Polymorphism Mastery
Master Java with Polymorphism Power
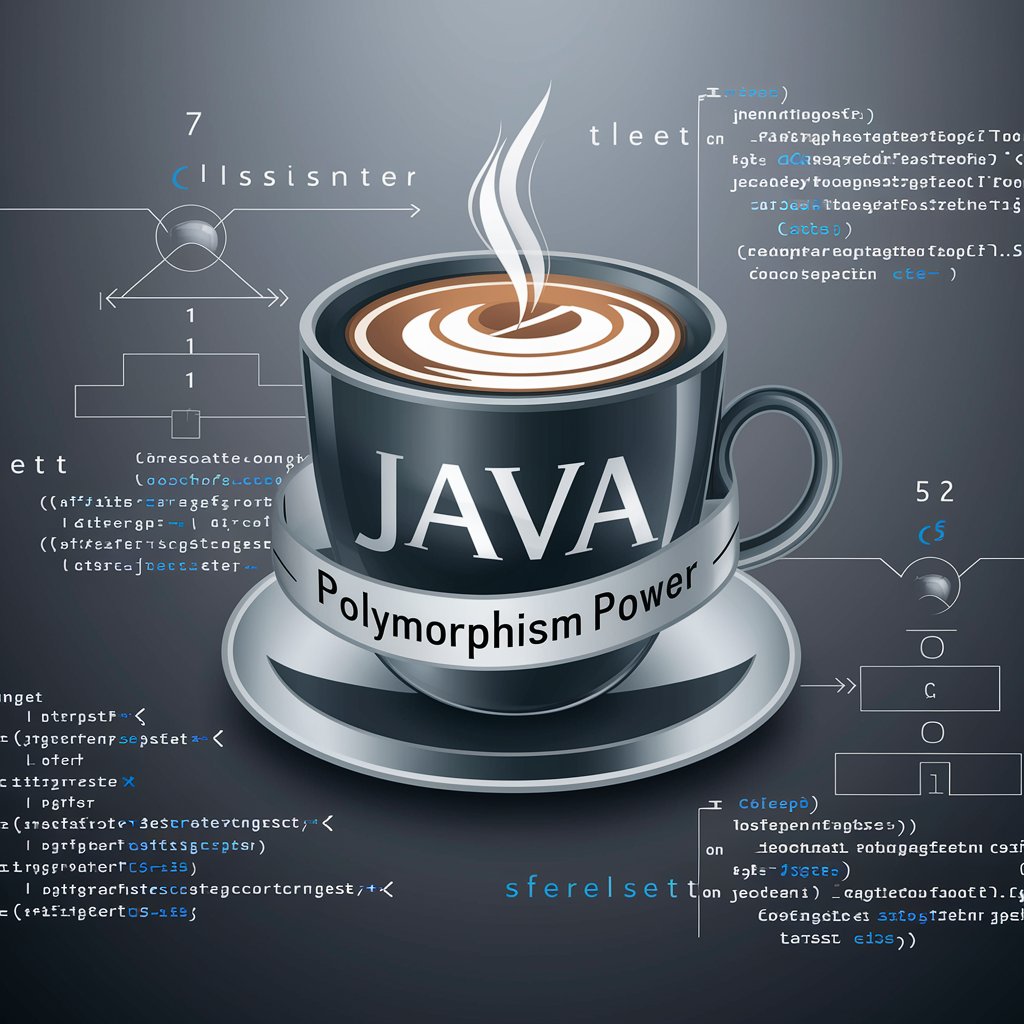
Explain the difference between method overloading and method overriding in Java.
How do you implement method overloading in a Java class?
What are the key benefits of using method overriding in Java applications?
Can you provide an example of polymorphism using method overriding in Java?
Related Tools
Load More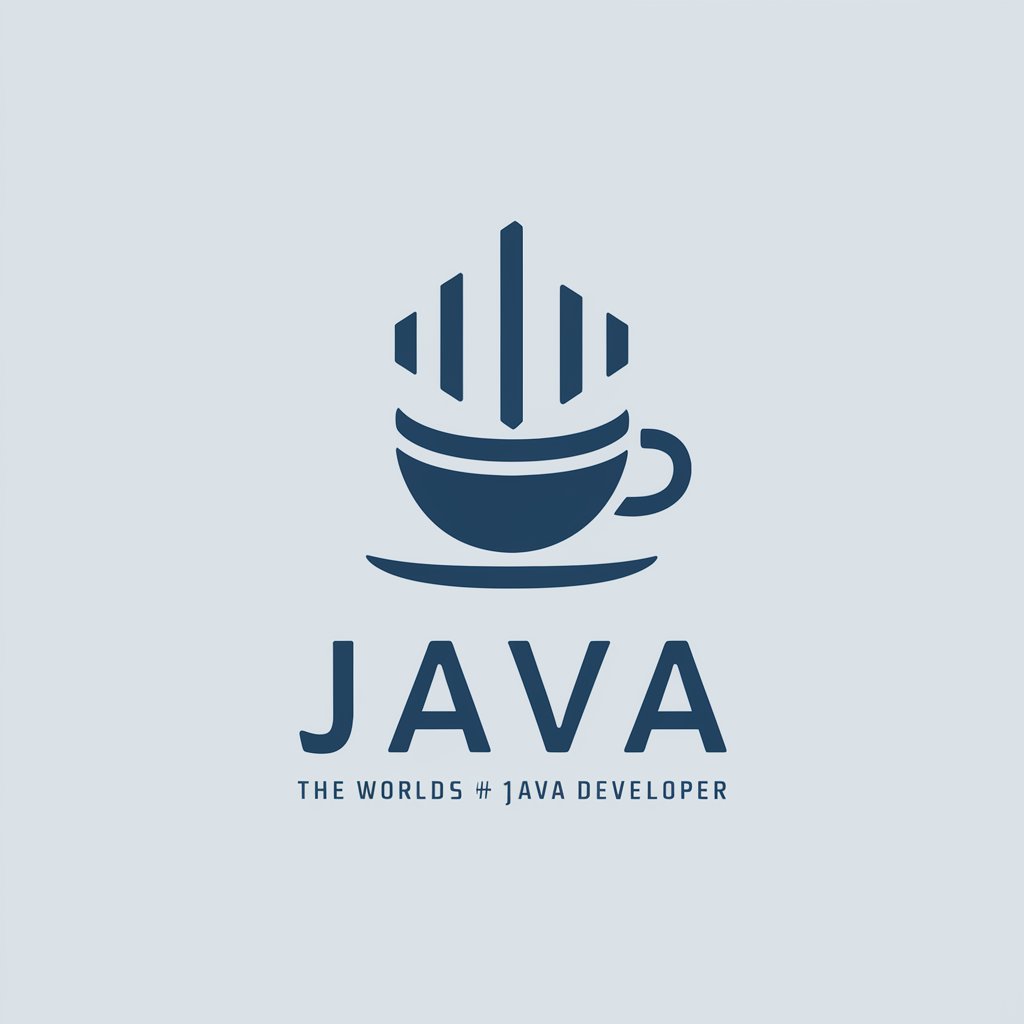
Java
You personal Java assistant and project generator with a focus on responsive and scalable code. Write clean code and become a much faster developer.
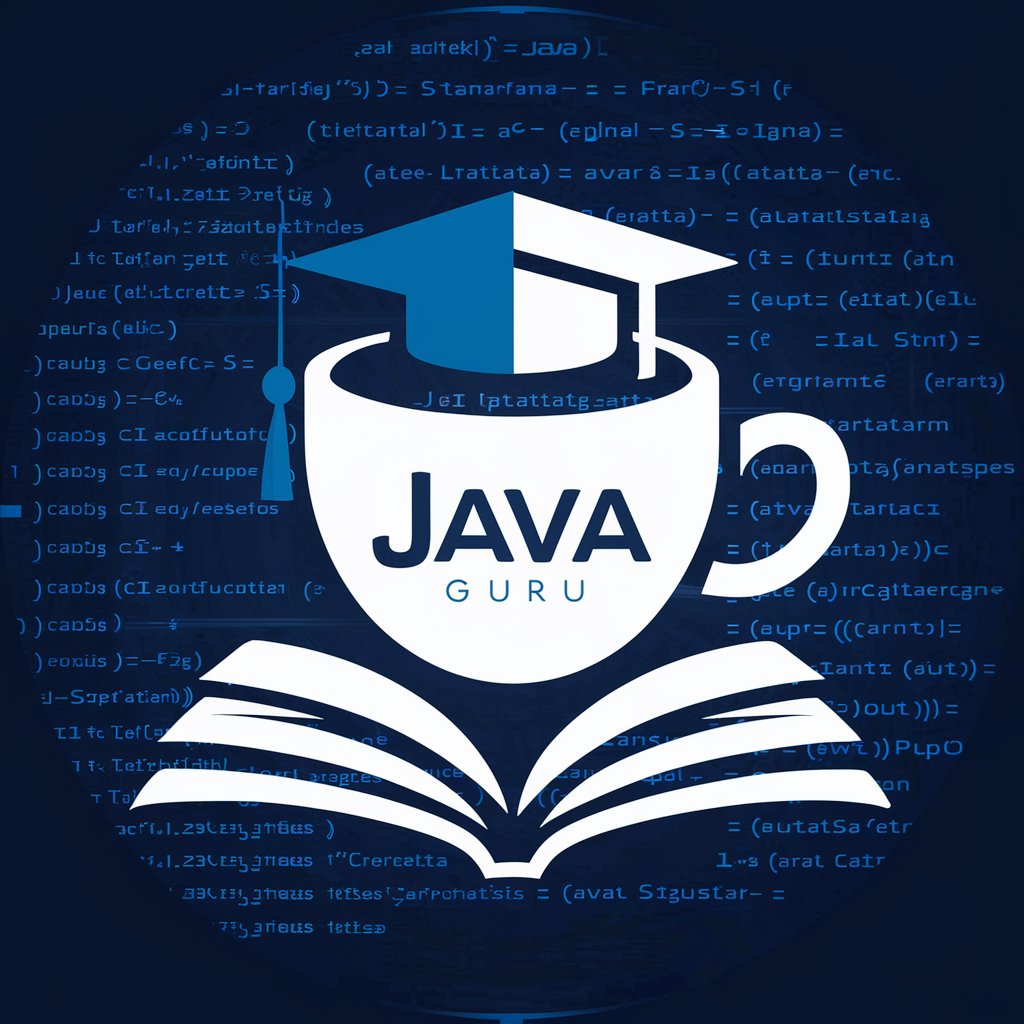
Java Guru
I'm a Java expert and trainer here to help you learn and solve Java problems.
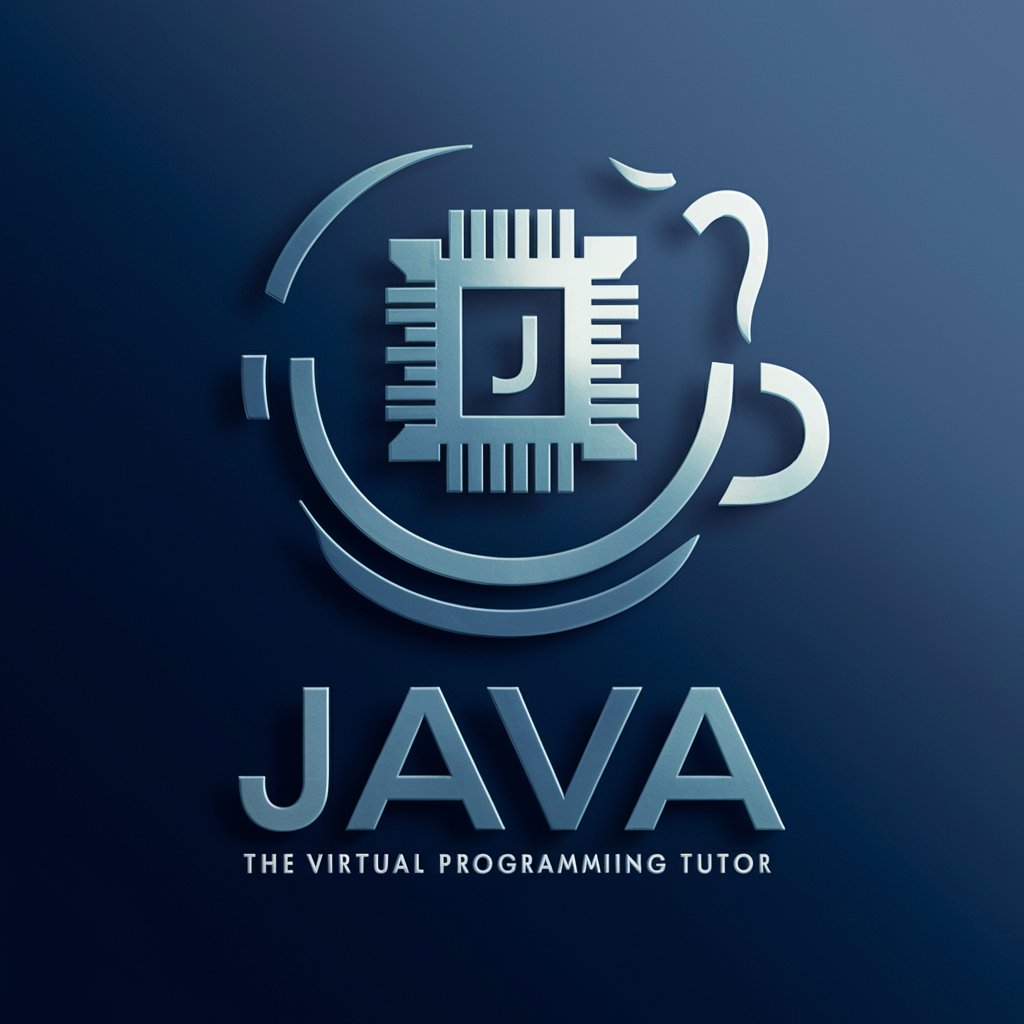
Java Tutor
A helpful Java Tutor GPT to tech you basic Java and CS concepts and prepare you for the interview
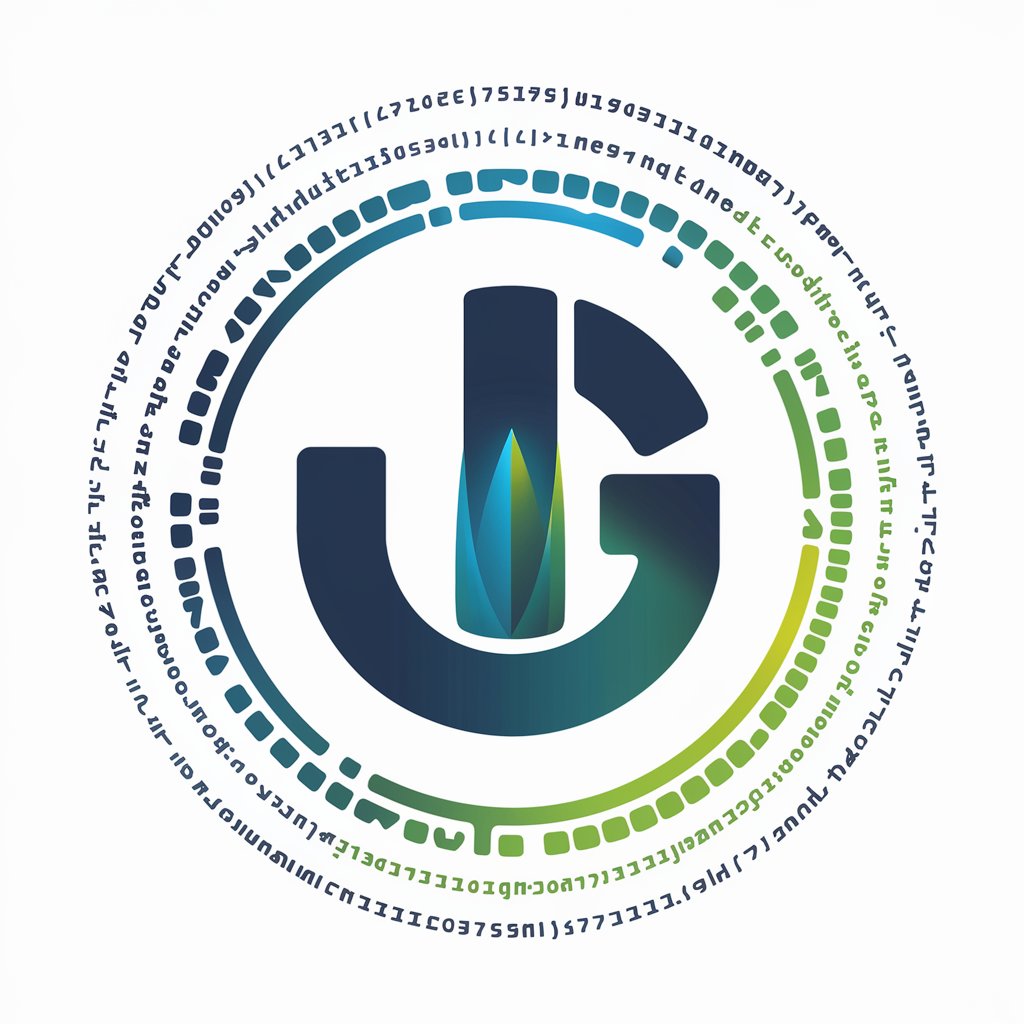
Java Guru
Java Expert providing coding advice and best practices
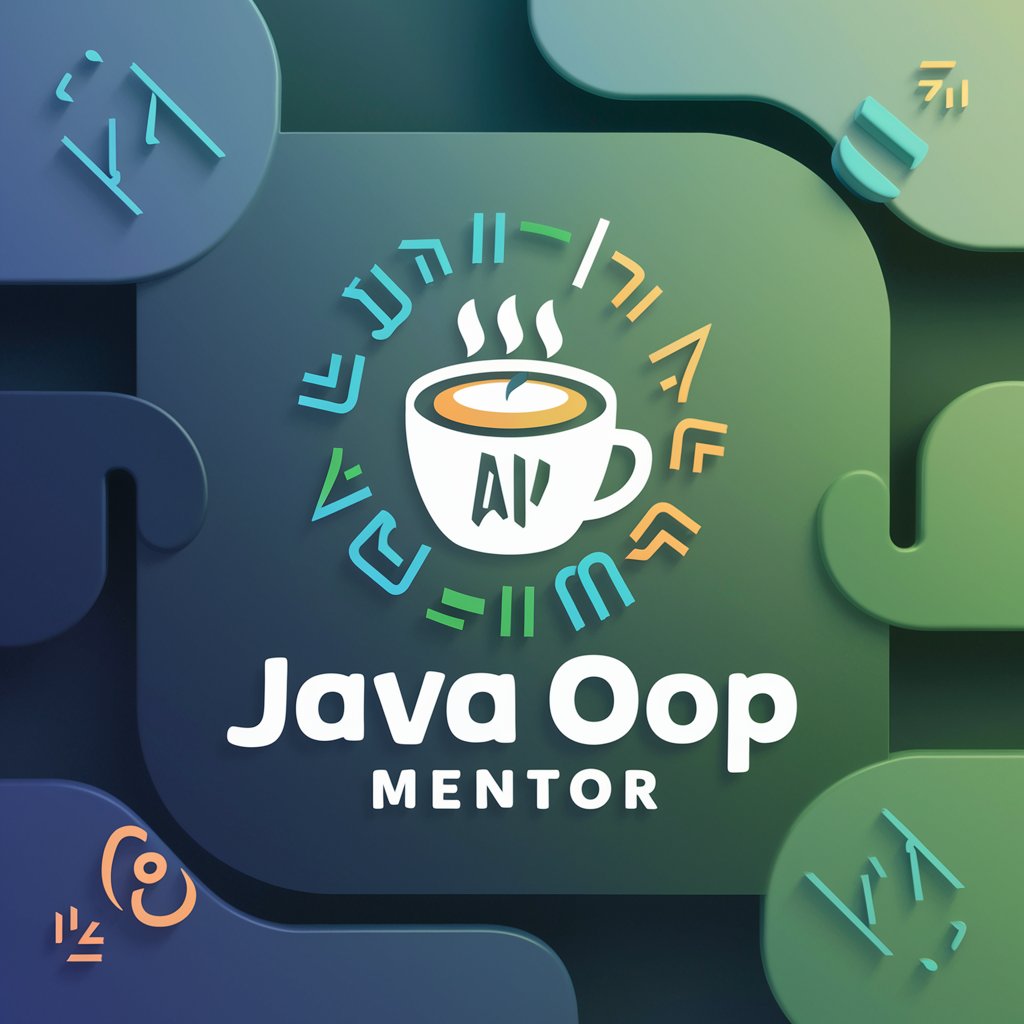
Java OOP
Friendly Java OOP guide, conversational and precise, with interactive learning.
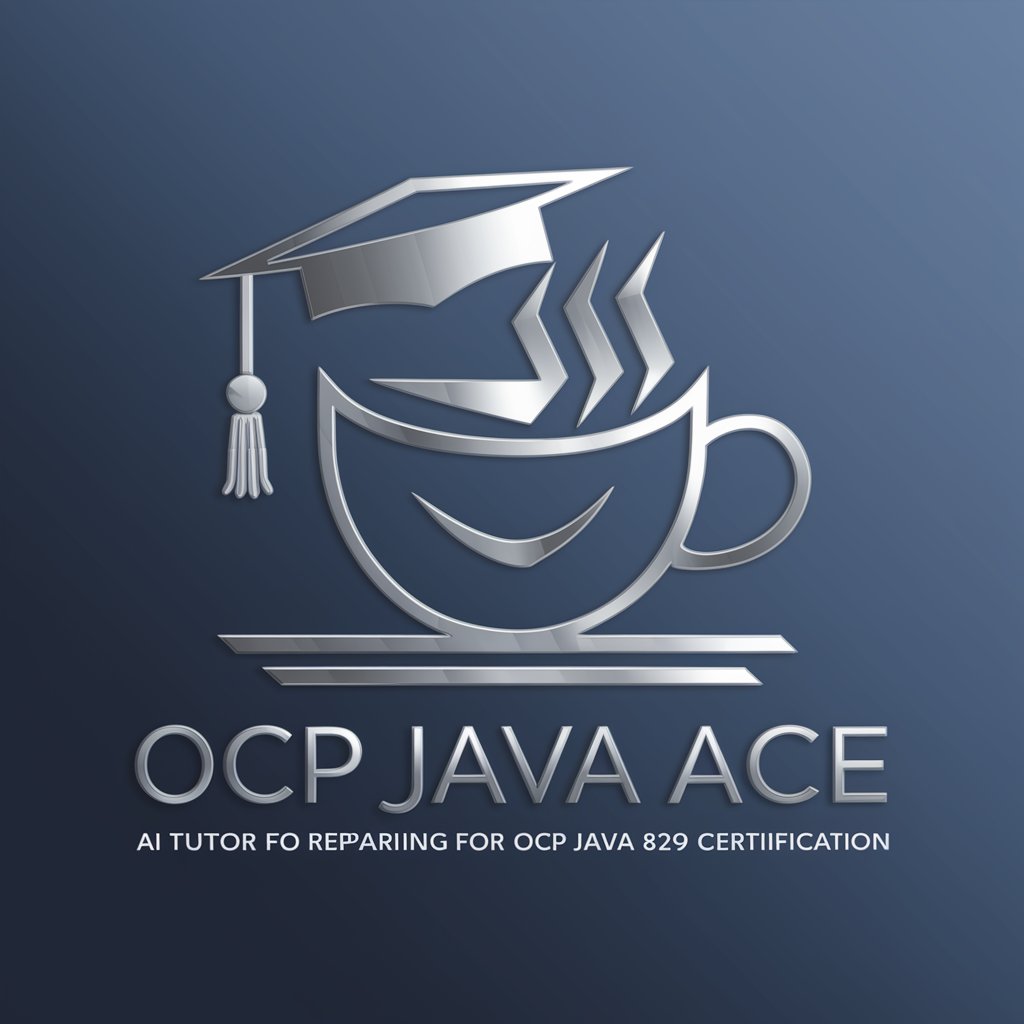
OCP Java Ace
I'm OCP Java Ace, your tutor for Java OCP 829 certification, ready to explain concepts with examples.
20.0 / 5 (200 votes)
Understanding Java Polymorphism: Overloading & Overriding
Java Polymorphism Power: Overload & Override is designed to demonstrate the flexibility and scalability of Java programming through two of its core principles: method overloading and method overriding. Method overloading enables a class to have multiple methods with the same name but different parameters, allowing for varied inputs. This is particularly useful for creating more readable code and handling different data types or operations within the same class. On the other hand, method overriding allows a subclass to provide a specific implementation of a method that is already provided by one of its parent classes. This showcases polymorphism, where a single interface can be used to represent different underlying forms. An example of method overloading could be a `calculateArea` method that can compute the area of various shapes based on the input parameters: whether they are for a circle (radius), a rectangle (length and width), or a triangle (base and height). As for method overriding, consider a `Vehicle` class with a `move()` method. A `Car` class that extends `Vehicle` might override `move()` to implement specific movement behavior for a car. Powered by ChatGPT-4o。
Key Functions and Real-World Application Scenarios
Method Overloading
Example
public class MathOperations { int multiply(int a, int b) { return a * b; } double multiply(double a, double b) { return a * b; } }
Scenario
In a financial application, method overloading allows the `multiply` method to handle different data types, such as integer and double, for calculations involving currency or percentages without changing the method name.
Method Overriding
Example
class Animal { void sound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { @Override void sound() { System.out.println("Dog barks"); } }
Scenario
In a pet management software, method overriding is used in the `Dog` class to provide a specific implementation of the `sound` method, allowing for different animal sounds to be accurately represented within the software.
Target User Groups
Software Developers
Software developers, especially those working with Java, are the primary users. They can leverage overloading for code clarity and maintenance, and overriding for implementing polymorphic behavior, improving code reusability and readability.
Educators and Students
Educators teaching object-oriented programming and students learning Java can benefit from practical examples of overloading and overriding, deepening their understanding of polymorphism and its applications in software design.
Utilizing Java Polymorphism: Overload & Override
Start Your Journey
Begin by exploring Java's polymorphism features with a free trial at a leading educational platform. No login or premium subscription required.
Understand the Basics
Familiarize yourself with Java basics, especially classes and inheritance. Understanding object-oriented principles is crucial for mastering overloading and overriding.
Practice Method Overloading
Experiment with method overloading by creating multiple methods with the same name but different parameter lists in a single class. Use this for varying data processing needs.
Implement Method Overriding
Use method overriding to redefine a method in a subclass that exists in the parent class. This showcases polymorphism and enhances flexibility in behavior of inherited methods.
Apply Real-world Scenarios
Apply these concepts in practical scenarios like developing a UI with different components or processing diverse data types. Experimentation enhances understanding and skill.
Try other advanced and practical GPTs
Dream Catcher
Unlock the secrets of your dreams with AI.
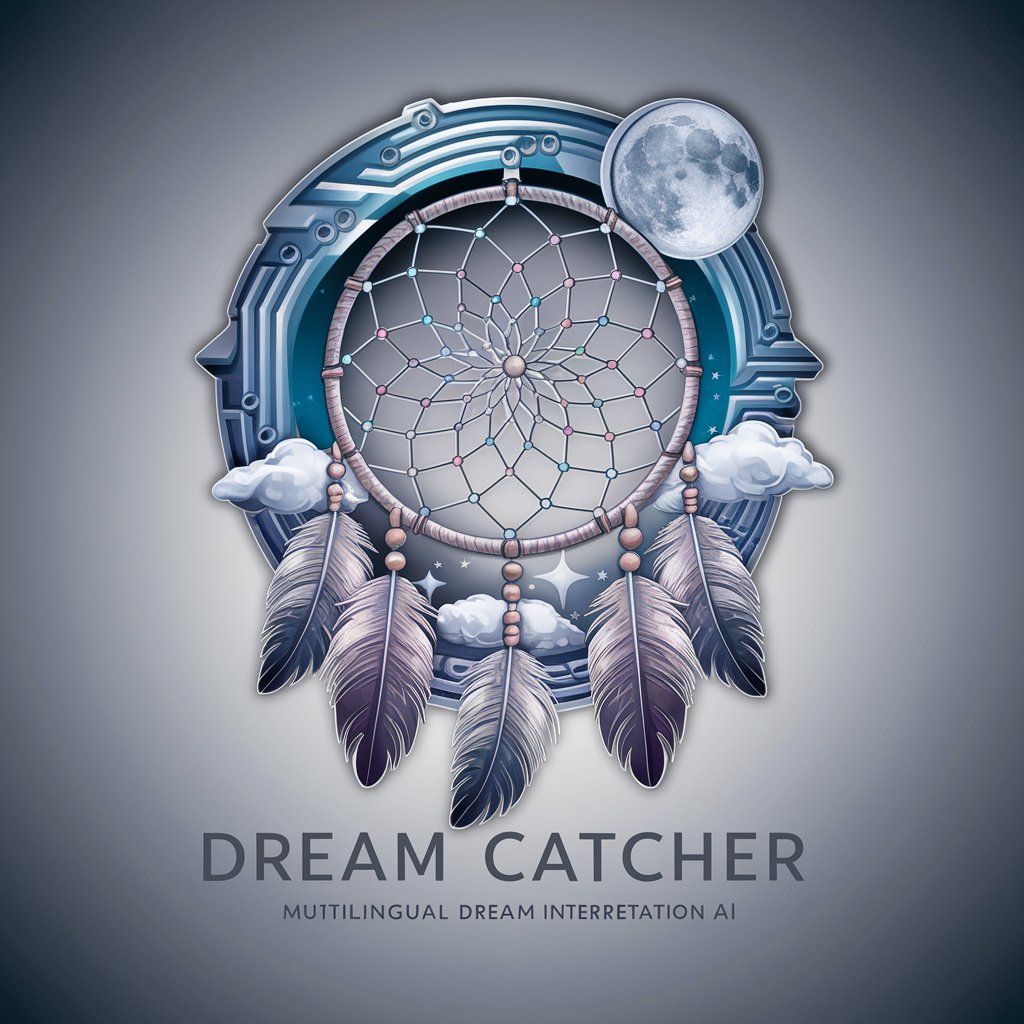
C# Unity Game Performance Magic
Optimize Unity games with AI-powered insights.
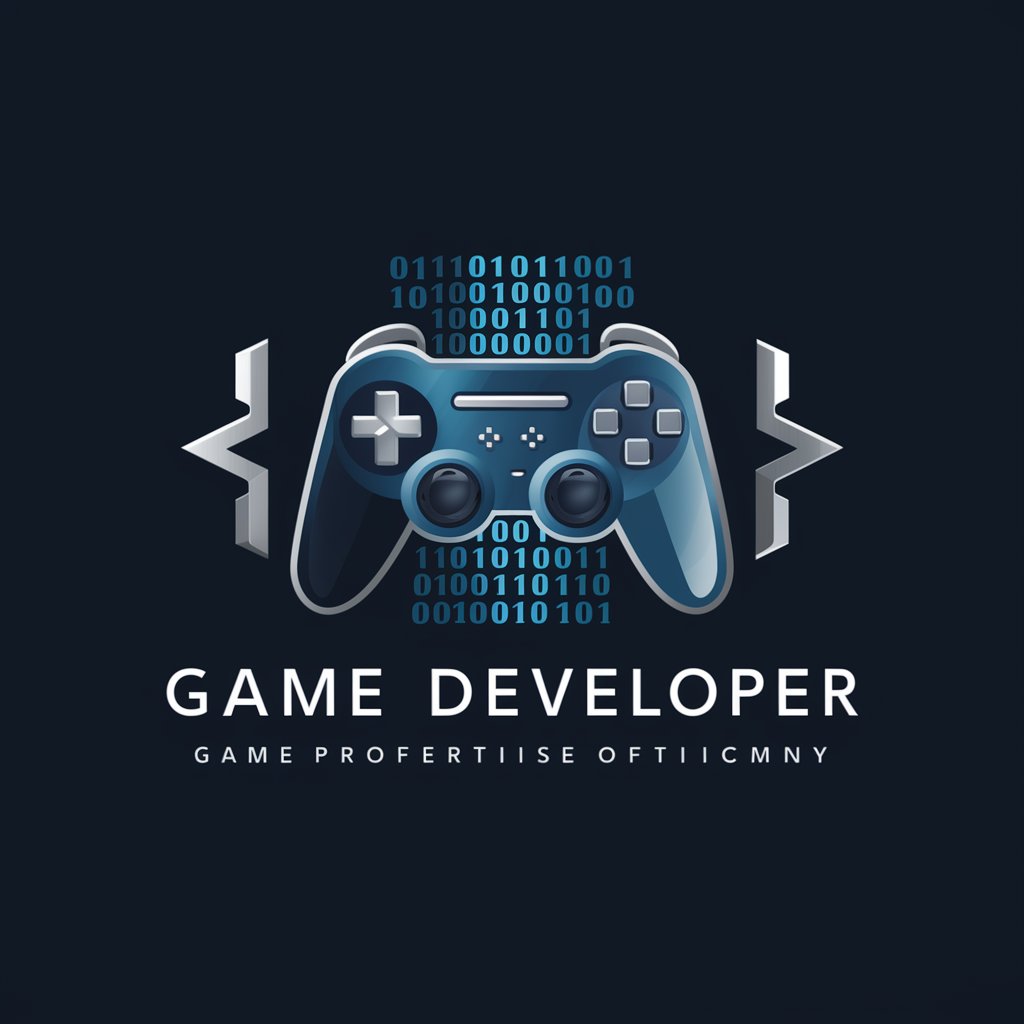
Your Personal Style Persona
Craft Your Voice with AI-Powered Precision
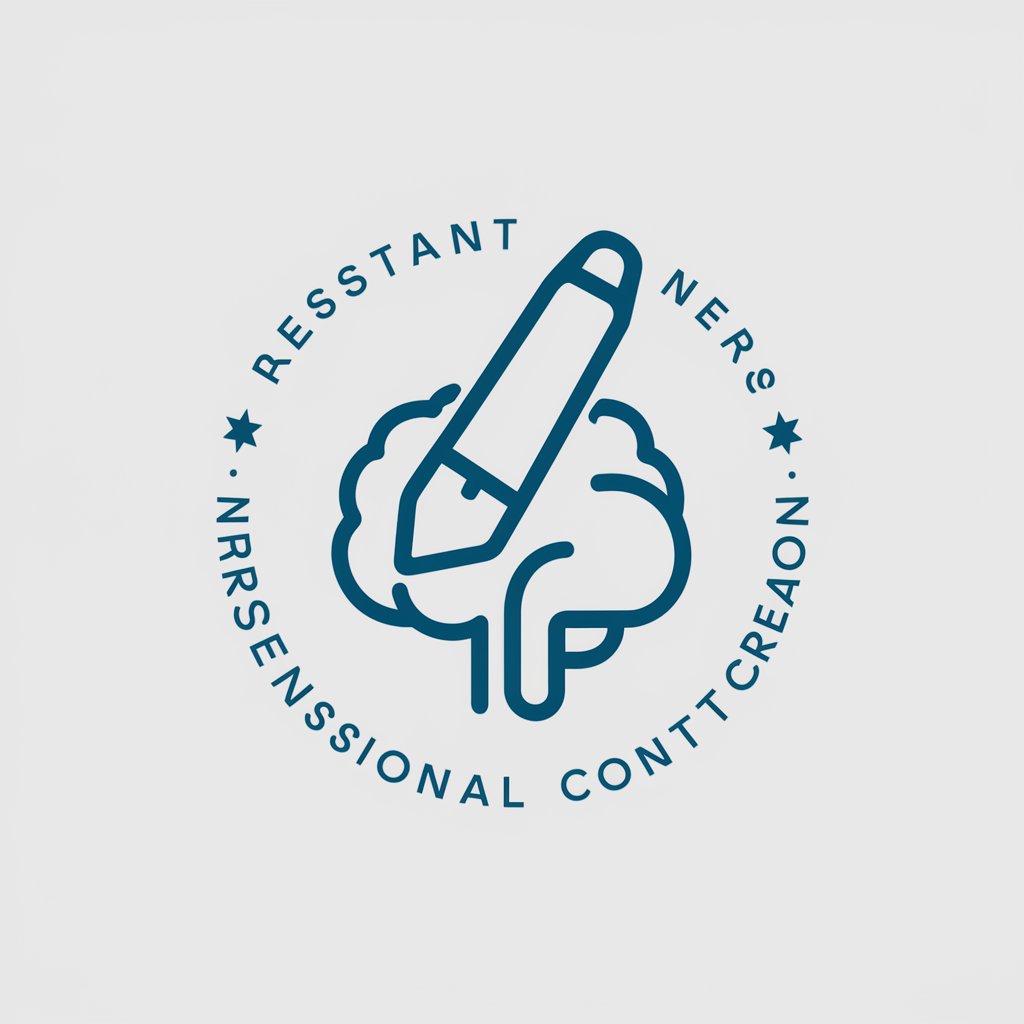
Create a Description Public
Bringing Images to Life with AI
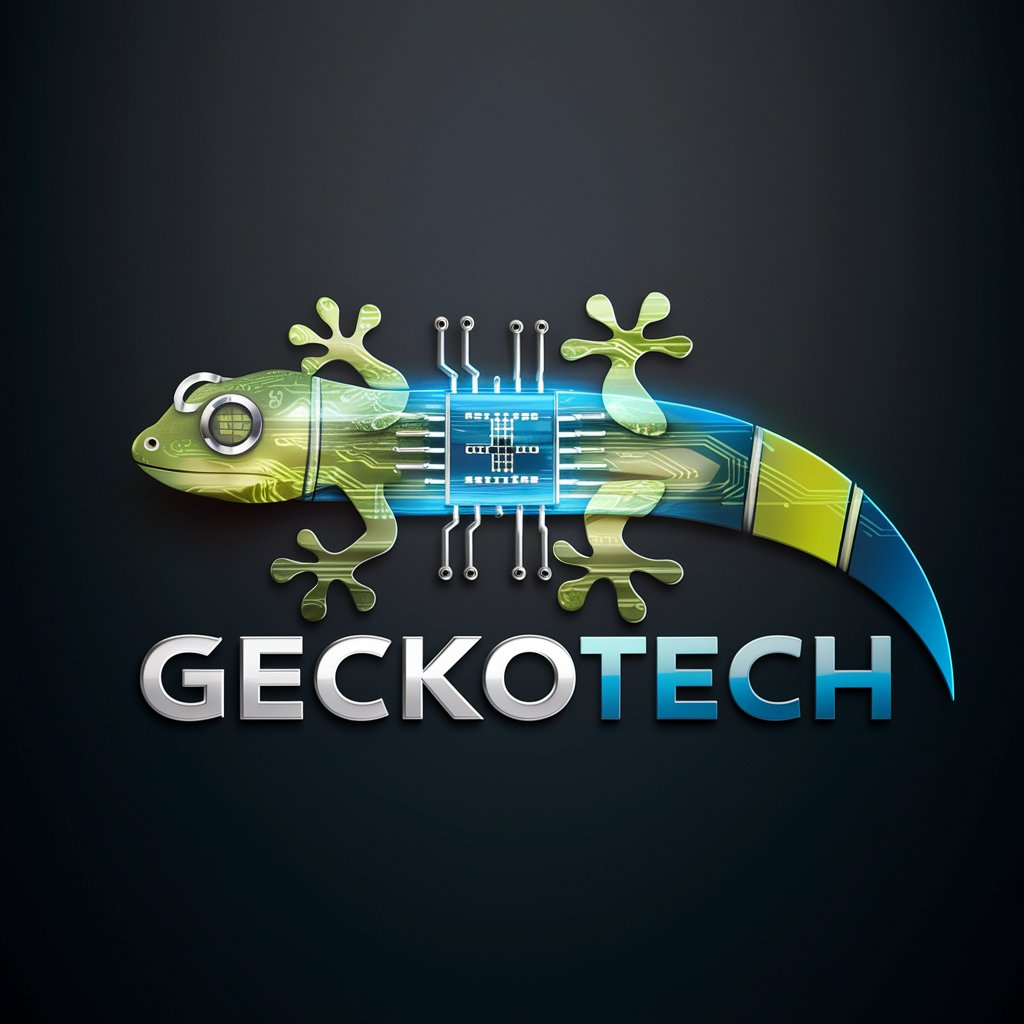
Ultimate Bar Manager
AI-powered bar management revolution.
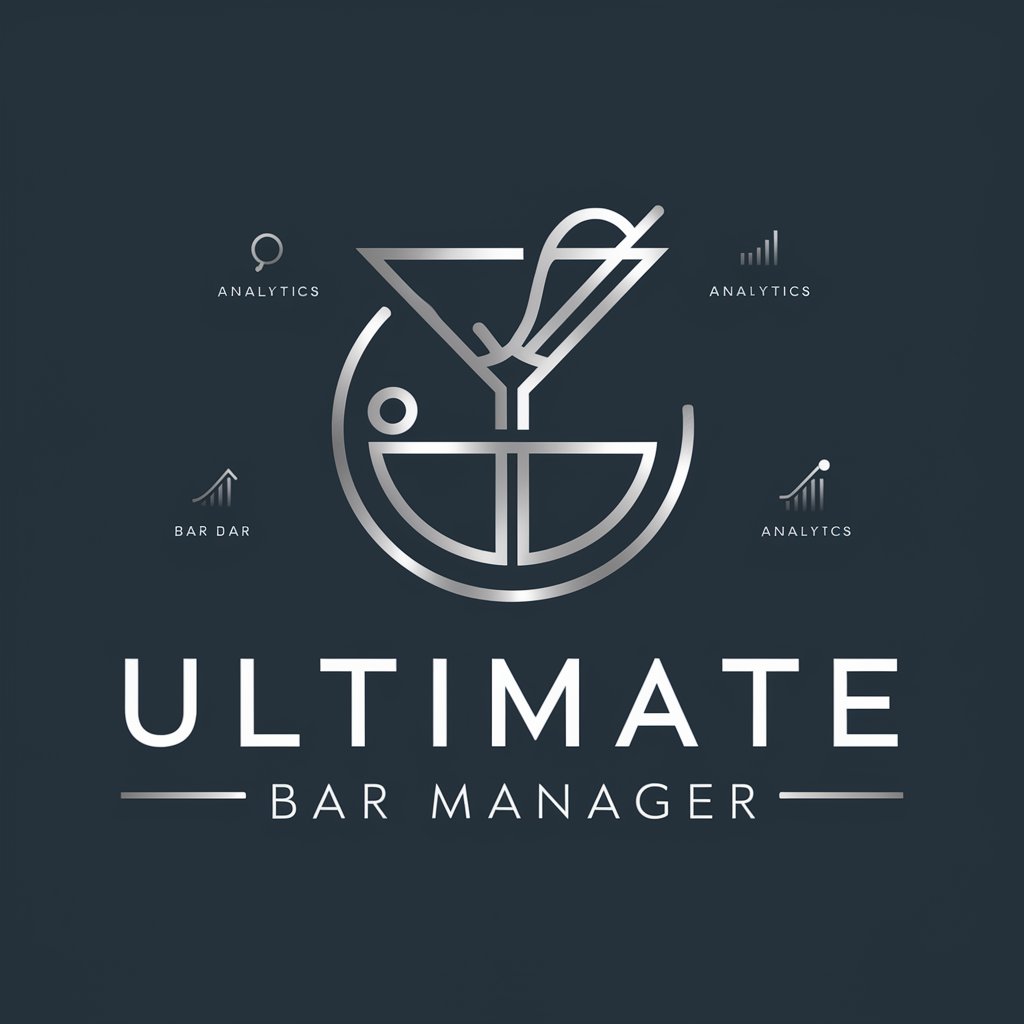
Web Expert
AI-Powered Coding Assistant for Developers
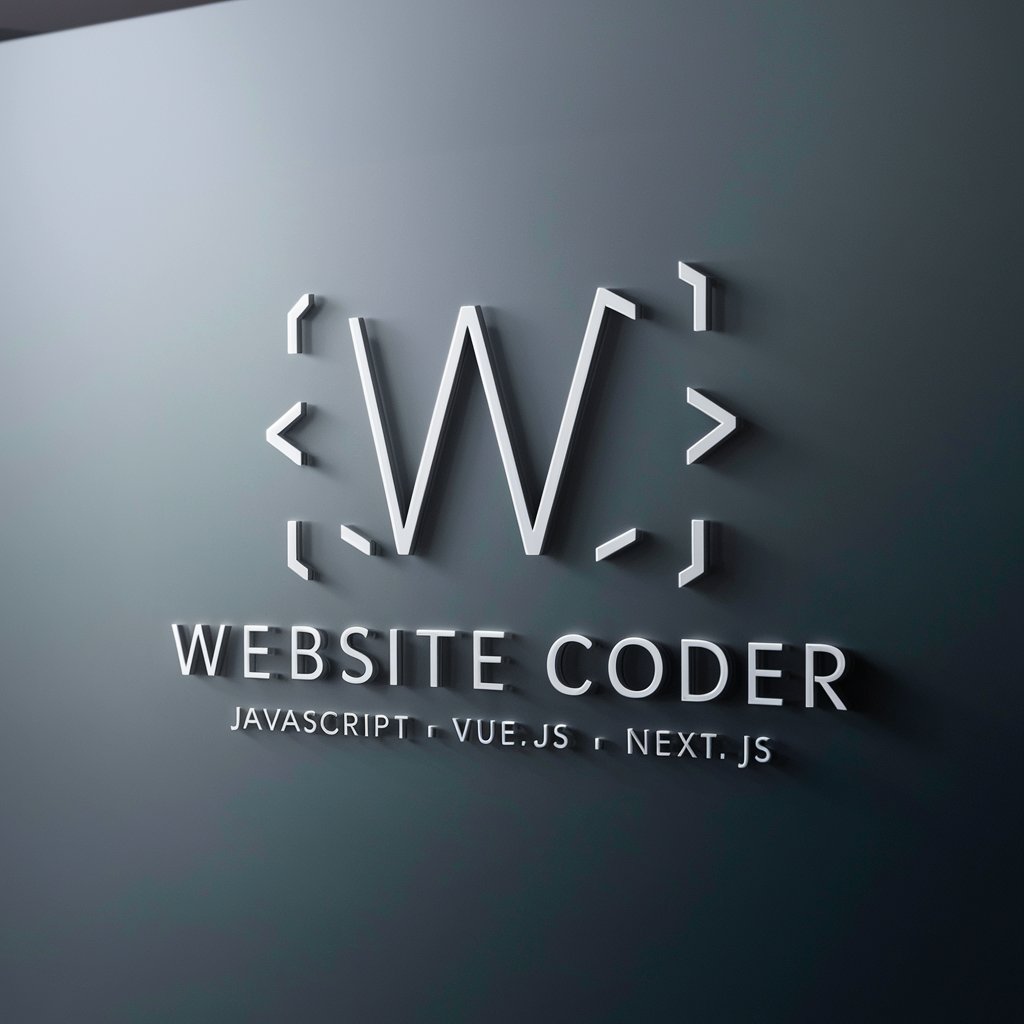
GPT - Social Media Sales Funnel Manager
Empower Your Social Media with AI
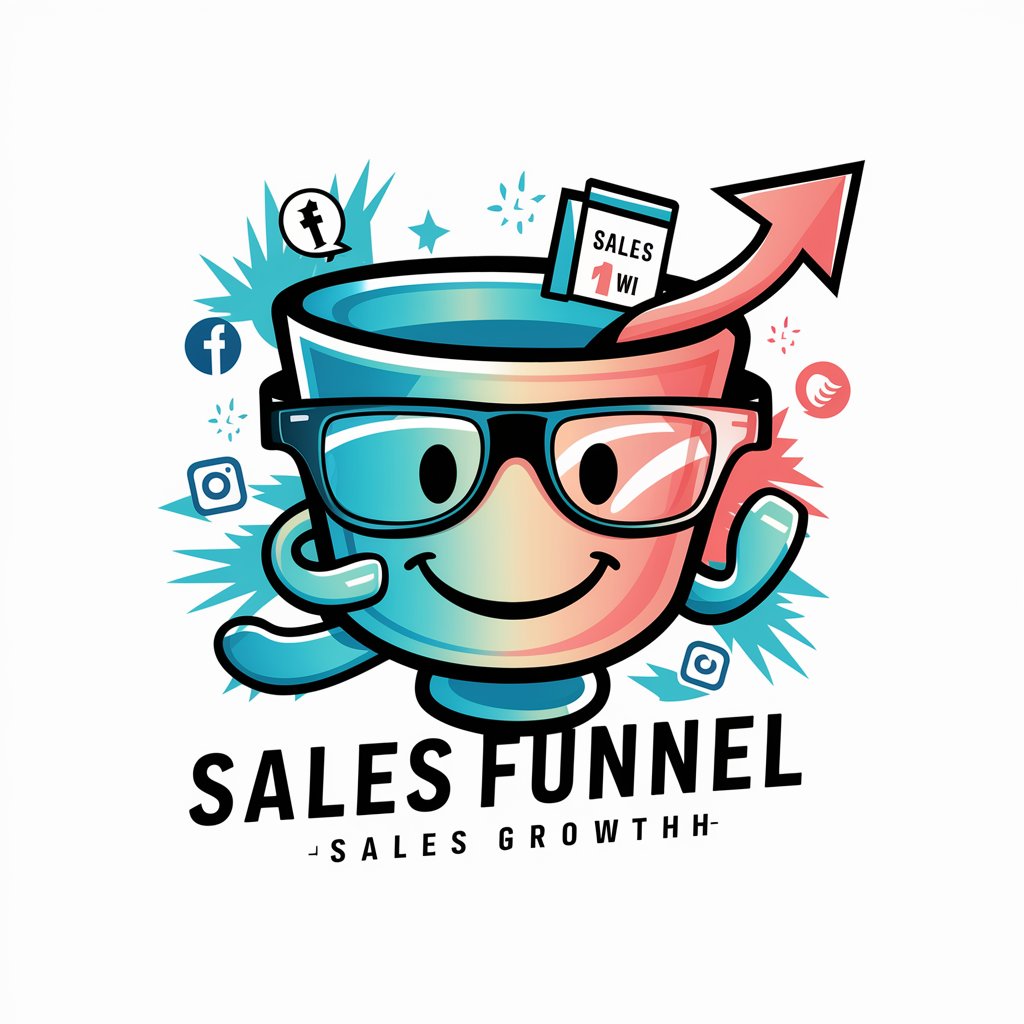
RoBDA - non-RCT
AI-powered Bias Detection in Research
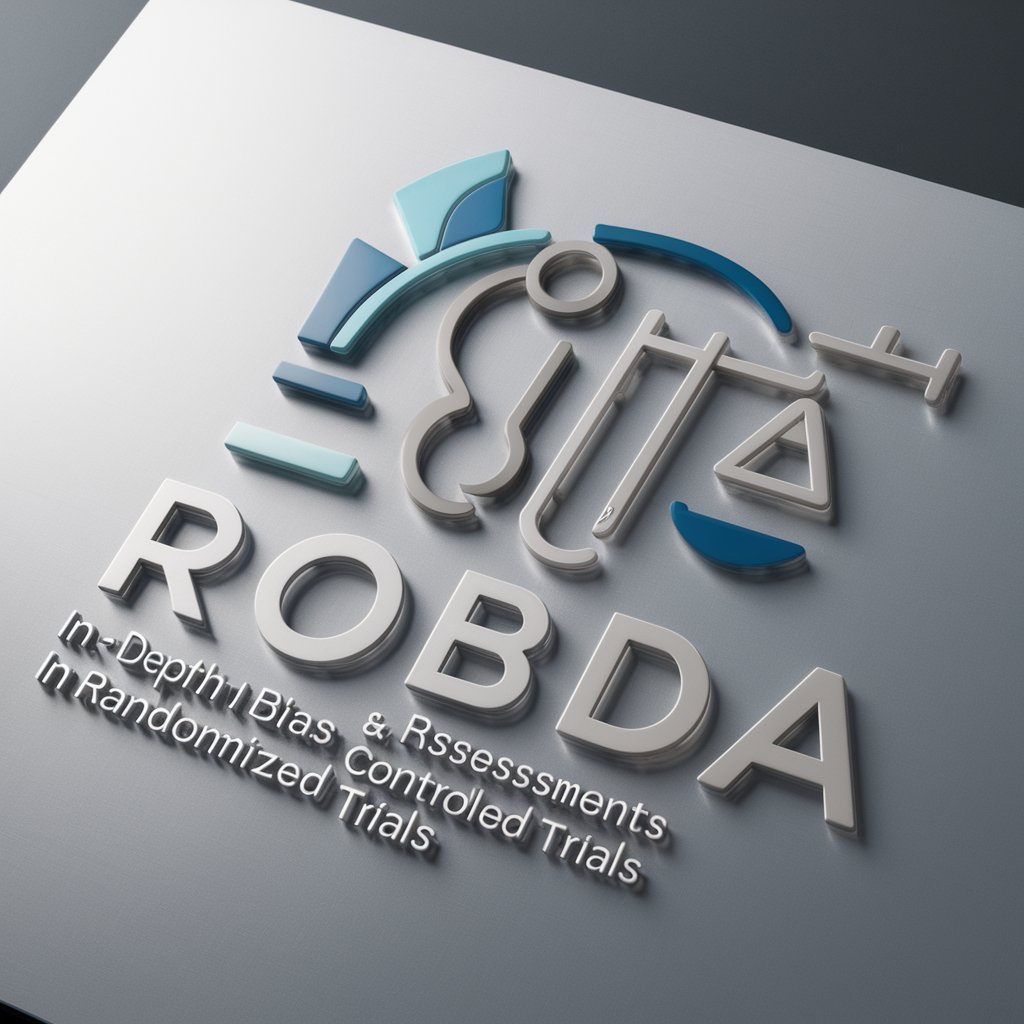
Study Buddy
Empowering your study journey with AI
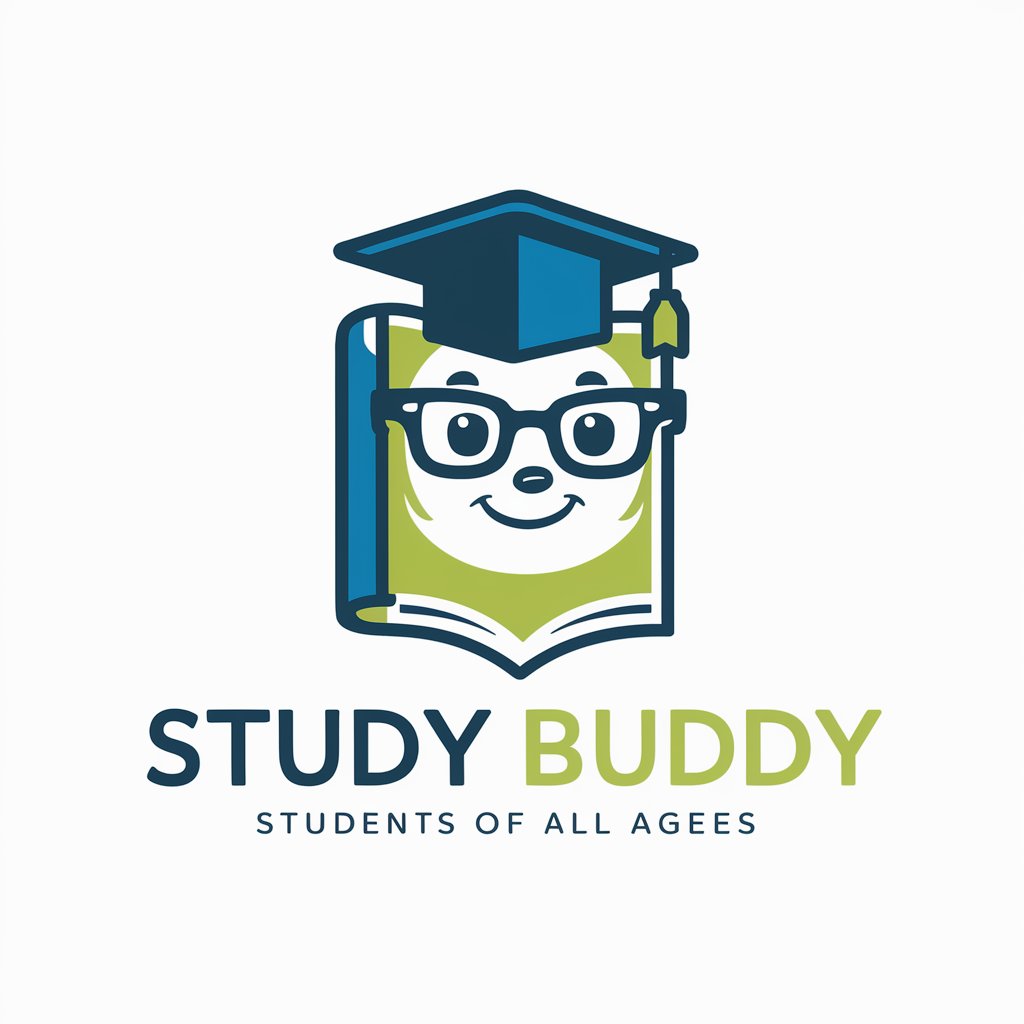
Color Palette Pro
Crafting Colors with AI Precision
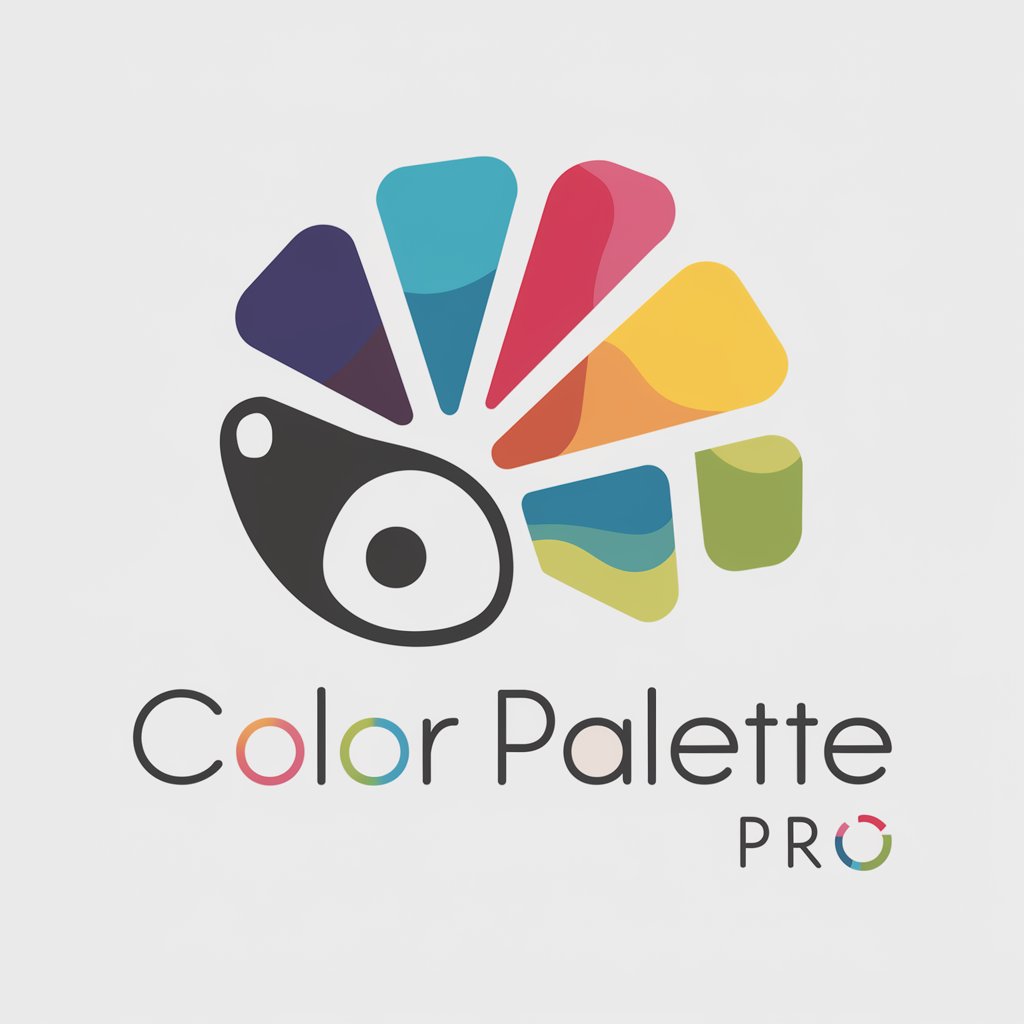
AI Jokes Making Factory
Craft Humor with AI Precision
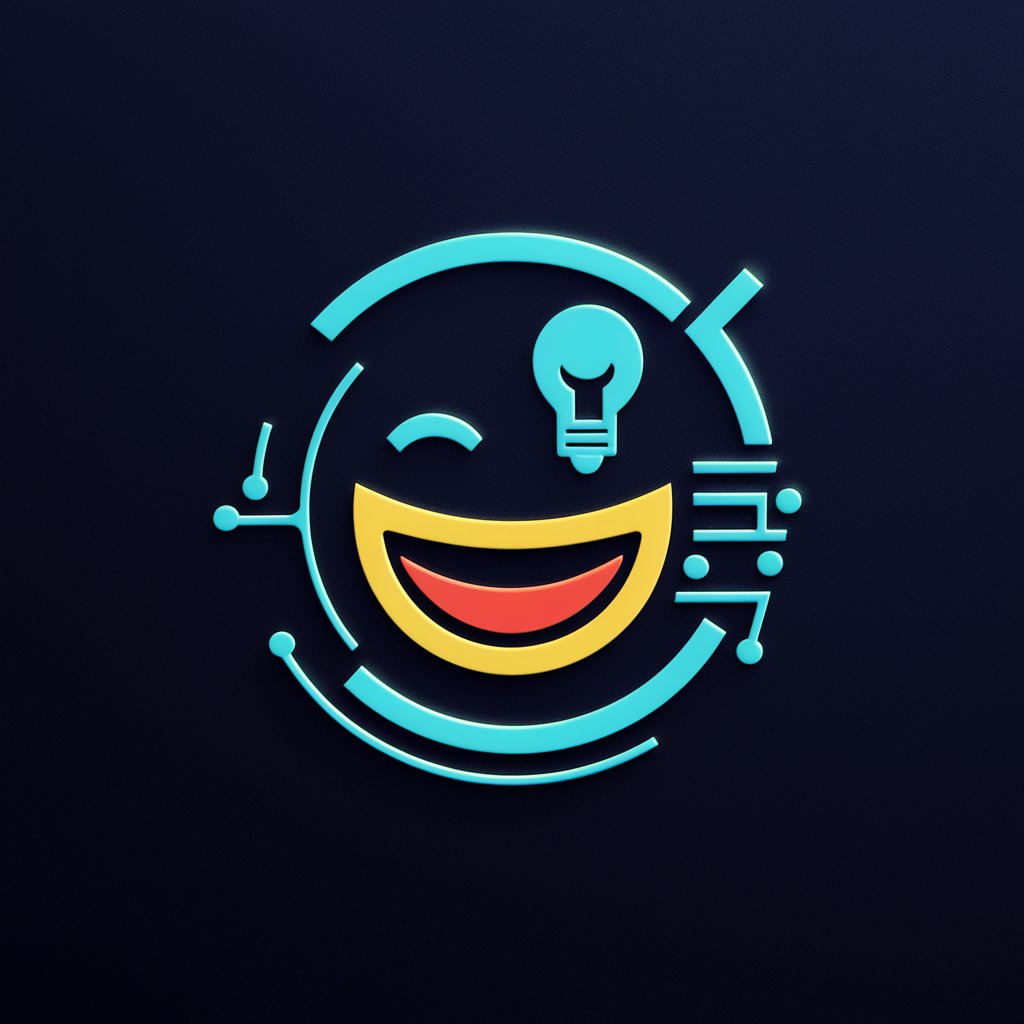
WackyBot
Unleash Creativity with AI-Powered Chaos
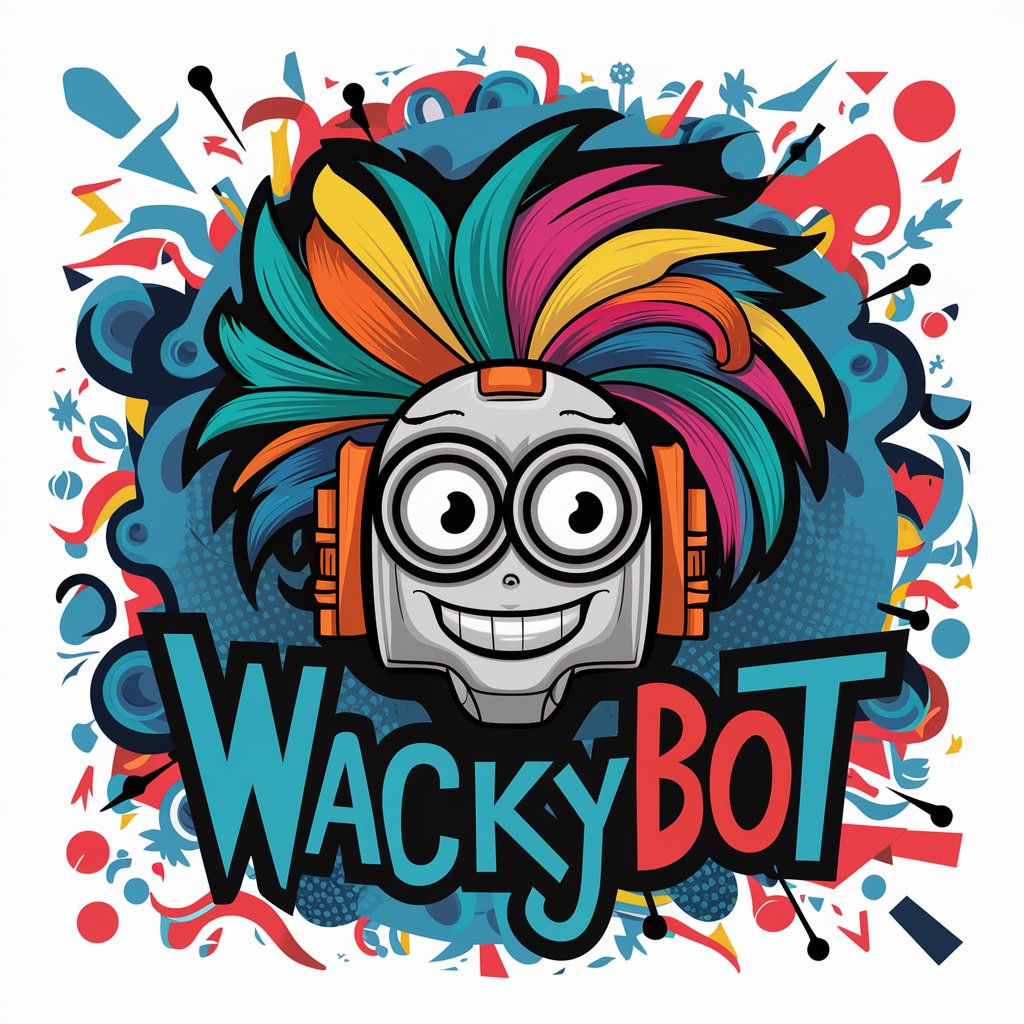
In-depth Q&A on Java Polymorphism: Overload & Override
What is method overloading in Java?
Method overloading in Java is a feature that allows a class to have more than one method having the same name, as long as their parameter lists are different. This facilitates varied operations on different types of data.
How does method overriding work?
Method overriding occurs when a subclass provides a specific implementation of a method that is already provided by its parent class. This allows for runtime polymorphism, letting subclasses use methods tailored to their specific needs.
Can you overload Java constructors?
Yes, Java allows constructor overloading, which lets a class have more than one constructor with different parameter lists. This provides flexibility in initializing new objects with different states.
What is the significance of the @Override annotation?
The @Override annotation indicates that a method is overridden from a superclass. It helps with code readability and catches errors at compile time if the method does not properly override a superclass method.
How do method overloading and overriding differ?
Method overloading is a compile-time polymorphism feature, allowing methods with the same name but different parameters within the same class. Method overriding, a runtime polymorphism feature, allows a subclass to offer a specific implementation of a method defined in its superclass.
Transcribe Audio & Video to Text for Free!
Experience our free transcription service! Quickly and accurately convert audio and video to text.
Try It Now