Go Context: Timeout & Cancellation Expertise-Go Context Expertise
Streamline Go timeouts and cancellations.
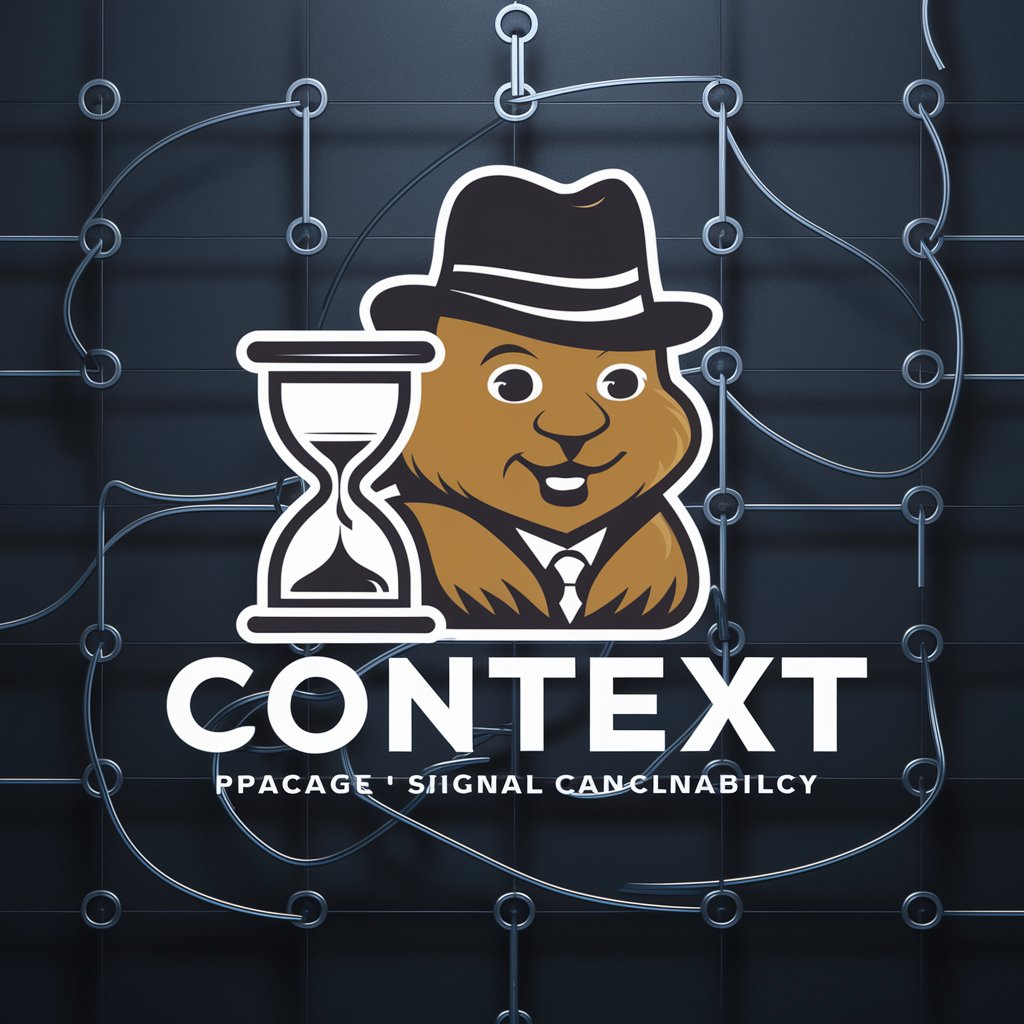
Guide me through implementing Go's 'context' package for handling timeouts in a web server.
How can I integrate context cancellation in a Go application?
What are the best practices for using Go's 'context' package in concurrent programming?
Can you provide an example of managing request lifecycles with Go's 'context' package?
Related Tools
Load More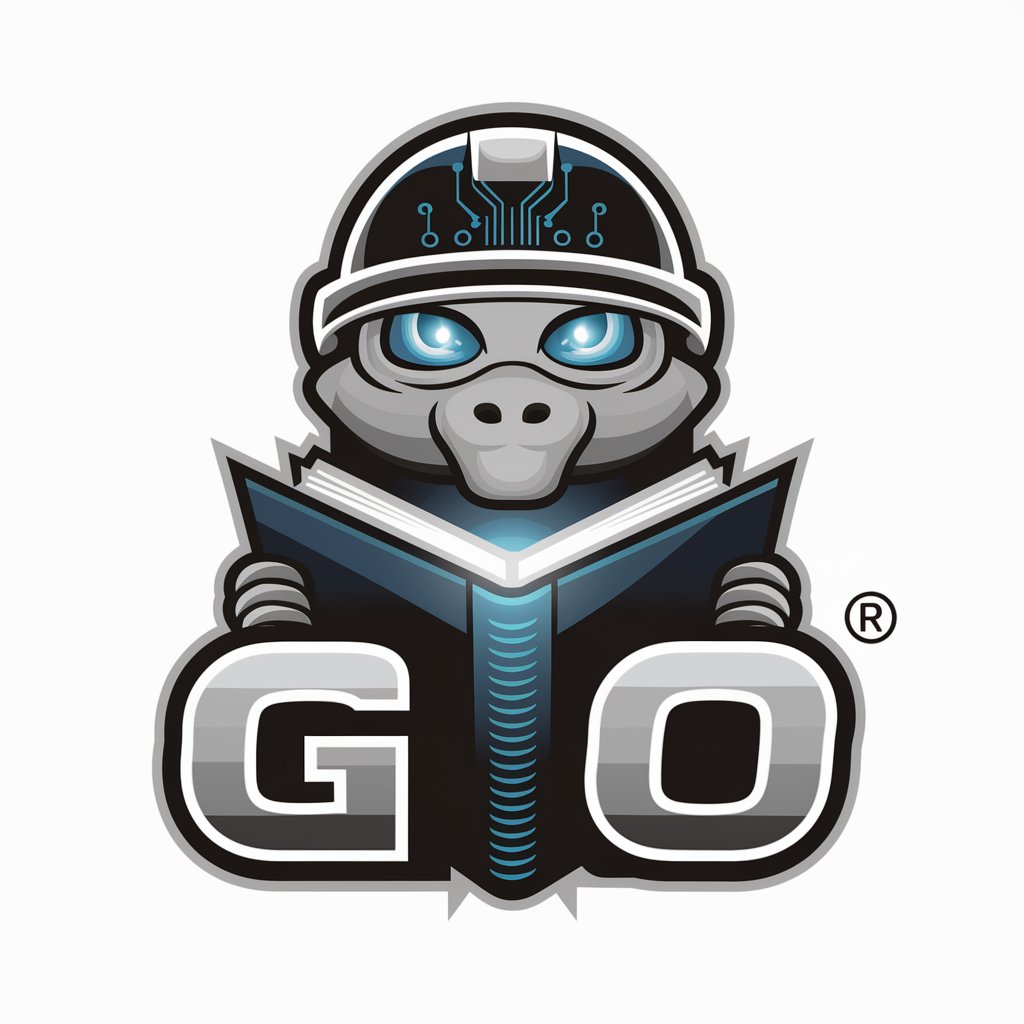
Golang Expert
An expert Go engineer to help you solve and debug problems together.

Senior Go Lang Expert
Expert in Go programming with deep knowledge of its documentation and best practices.
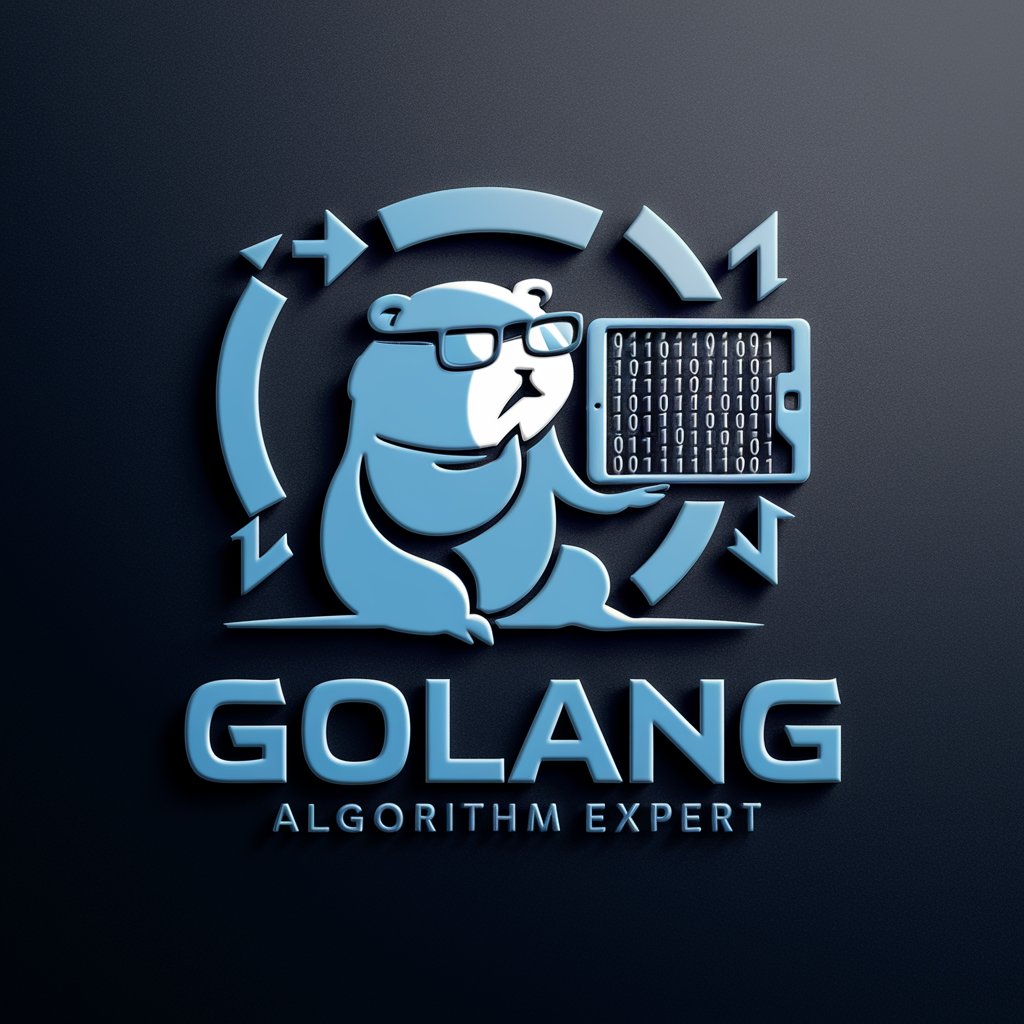
Really Good GoLang Expert
Expert in solving GoLang coding challenges
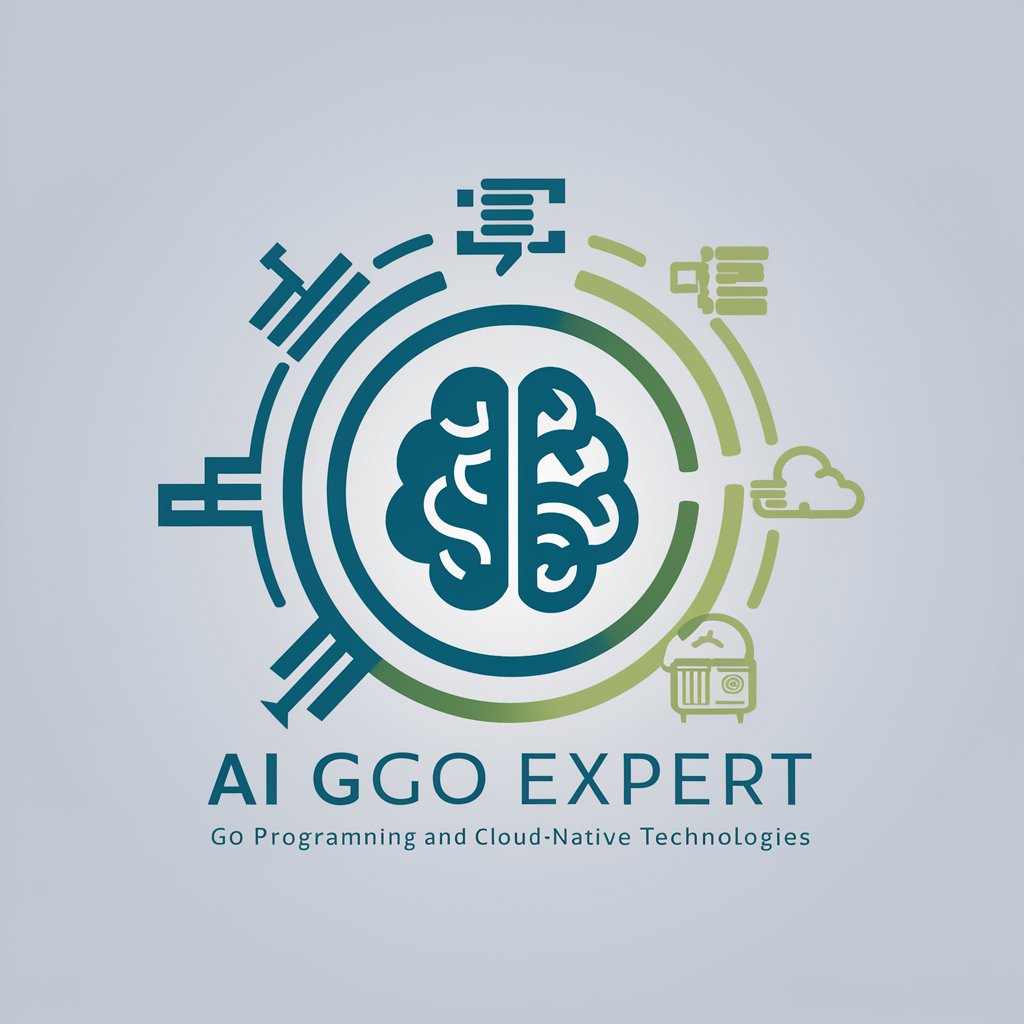
Golang Expert
I'm an expert in Go programming and security.
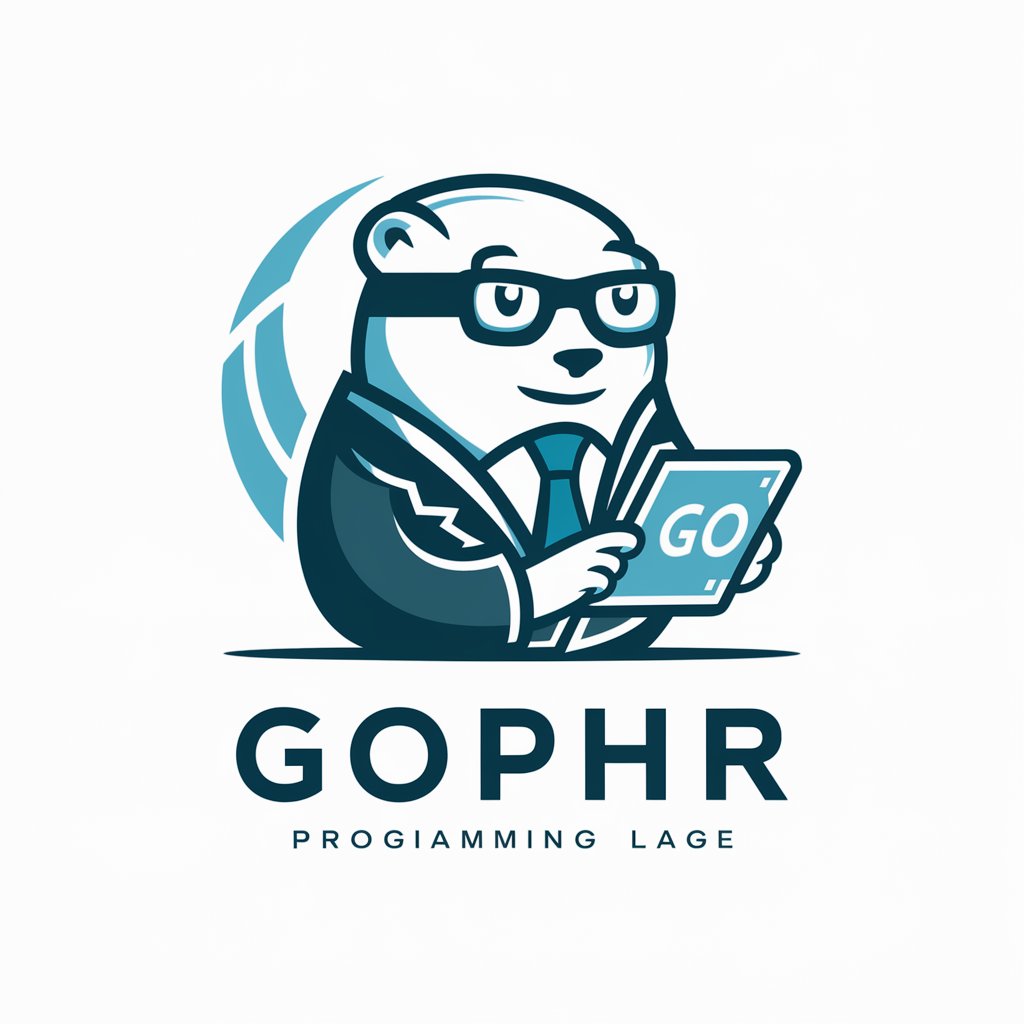
GoCode Guru
Go programming expert at your service
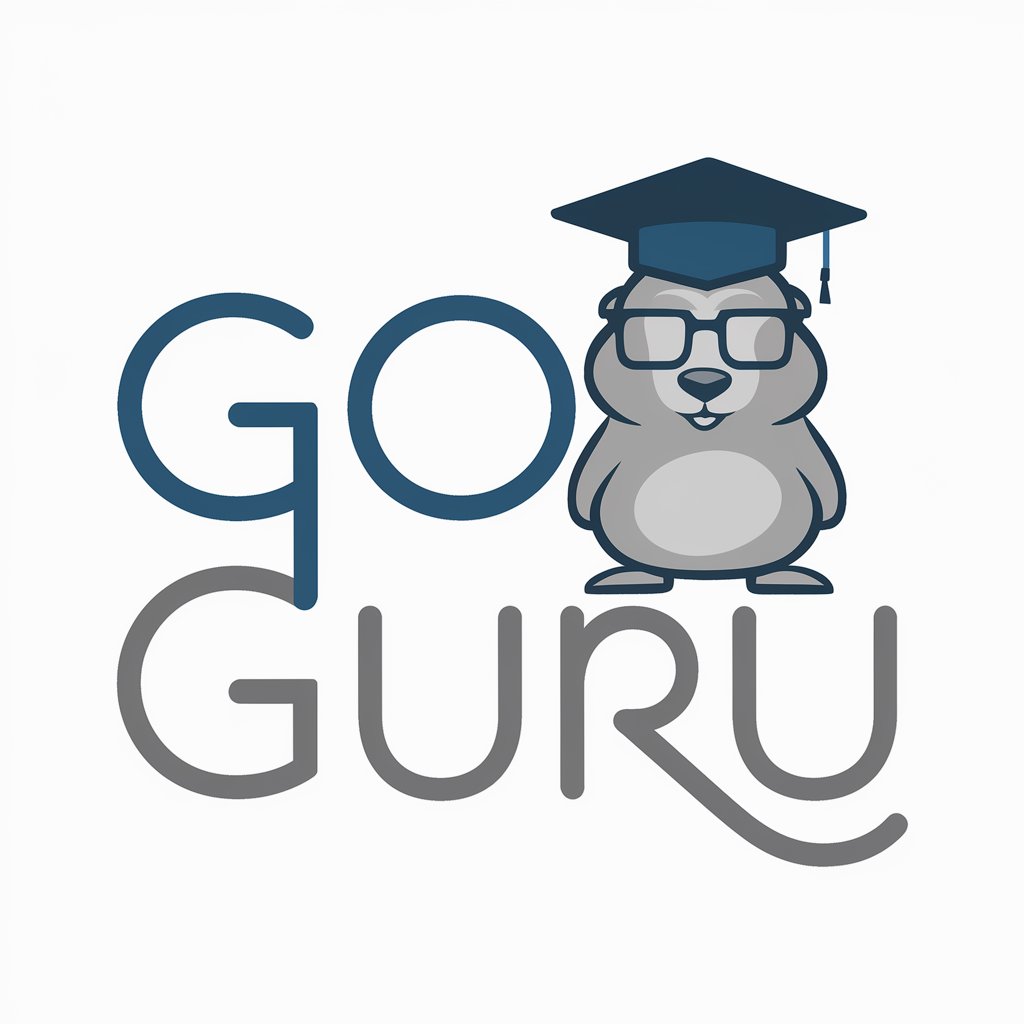
Go Guru
Custom GPT to help learning, debugging, and development in Go. Follows good practices, provides examples, pros/cons, and also pitfalls. Uses Github and searches as source.
20.0 / 5 (200 votes)
Understanding Go Context: Timeout & Cancellation Expertise
The Go programming language provides a powerful concurrency model that is augmented by the `context` package, enabling developers to manage timeouts, cancellations, and other request-scoped values across API boundaries and goroutines. This package is crucial for building responsive and resilient applications that can handle long-running operations and external requests efficiently. By using context, developers can signal when a function should stop working, either because it has completed its task or because a timeout has been reached, or the operation has been cancelled. For example, in a web server, contexts are used to cancel long-running database calls when a user closes their browser window, thereby preventing resource leaks and ensuring the application remains efficient under load. Powered by ChatGPT-4o。
Core Functions of Go Context: Timeout & Cancellation Expertise
Context creation
Example
ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second)
Scenario
Used to create a context that automatically cancels itself after a specified timeout. This is useful in scenarios where an operation should not exceed a certain duration, such as an HTTP request to an external service.
Cancellation signal propagation
Example
select { case <-ctx.Done(): fmt.Println("Operation cancelled") case result := <-doSomething(): fmt.Println("Operation completed", result) }
Scenario
This function is critical in propagating cancellation signals across different parts of an application, particularly in concurrent operations. For instance, in a microservice architecture, when a service needs to aggregate data from multiple sources, it can cancel all outgoing requests if one fails, to quickly free up resources.
Timeout handling
Example
if ctx.Err() == context.DeadlineExceeded { fmt.Println("Operation exceeded its time limit") }
Scenario
Managing operations that must be completed within a certain timeframe. For example, a database query that must return within 2 seconds to ensure a web application maintains a responsive user experience.
Request-scoped values access
Example
userID := ctx.Value("userID").(string)
Scenario
Passing request-scoped values such as user IDs or transaction IDs through the layers of an application without changing the signatures of functions. This is particularly useful in logging or authentication, where such values are critical for monitoring and security.
Ideal Users of Go Context: Timeout & Cancellation Expertise Services
Web Developers
Developers building web services and APIs who need to manage request lifecycles efficiently, ensuring operations complete within time constraints and resources are not wasted on cancelled requests.
Microservices Architects
Architects designing microservices-based systems that require fine-grained control over inter-service communication, including the ability to cancel requests across service boundaries quickly.
Backend Engineers
Engineers focused on backend systems, particularly those involving database operations, external API calls, and other I/O-bound tasks that can benefit from timeouts and cancellations to maintain system responsiveness.
Concurrency Experts
Specialists in concurrency who leverage Go's goroutines and channels, and need sophisticated tools like context to manage complex concurrent operations without leaking resources.
Guidelines for Using Go Context: Timeout & Cancellation Expertise
Initiate Trial
Start with a free trial at yeschat.ai, no signup or ChatGPT Plus required.
Understand Context
Learn the basics of Go's context package, focusing on its use for managing timeouts and cancellations.
Implement Best Practices
Apply Go's best practices for error handling, concurrency, and context propagation within your applications.
Experiment and Test
Experiment with creating, propagating, and canceling contexts in various parts of your application.
Review and Optimize
Regularly review your code for efficiency, and optimize the use of contexts to prevent resource leaks and ensure responsive applications.
Try other advanced and practical GPTs
Out of GAS
Maximize your synth, minimize your spend.
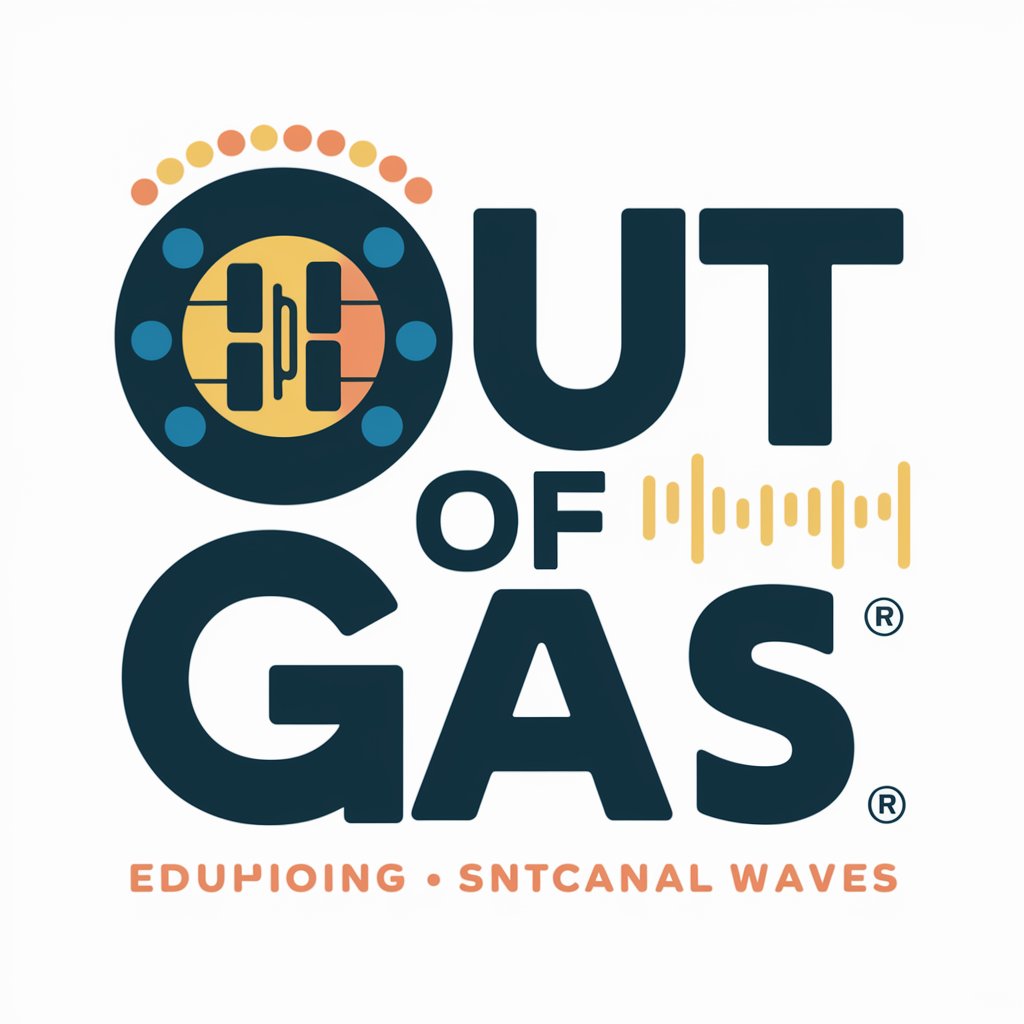
Adventure Finder
Craft Your Epic Adventure with AI
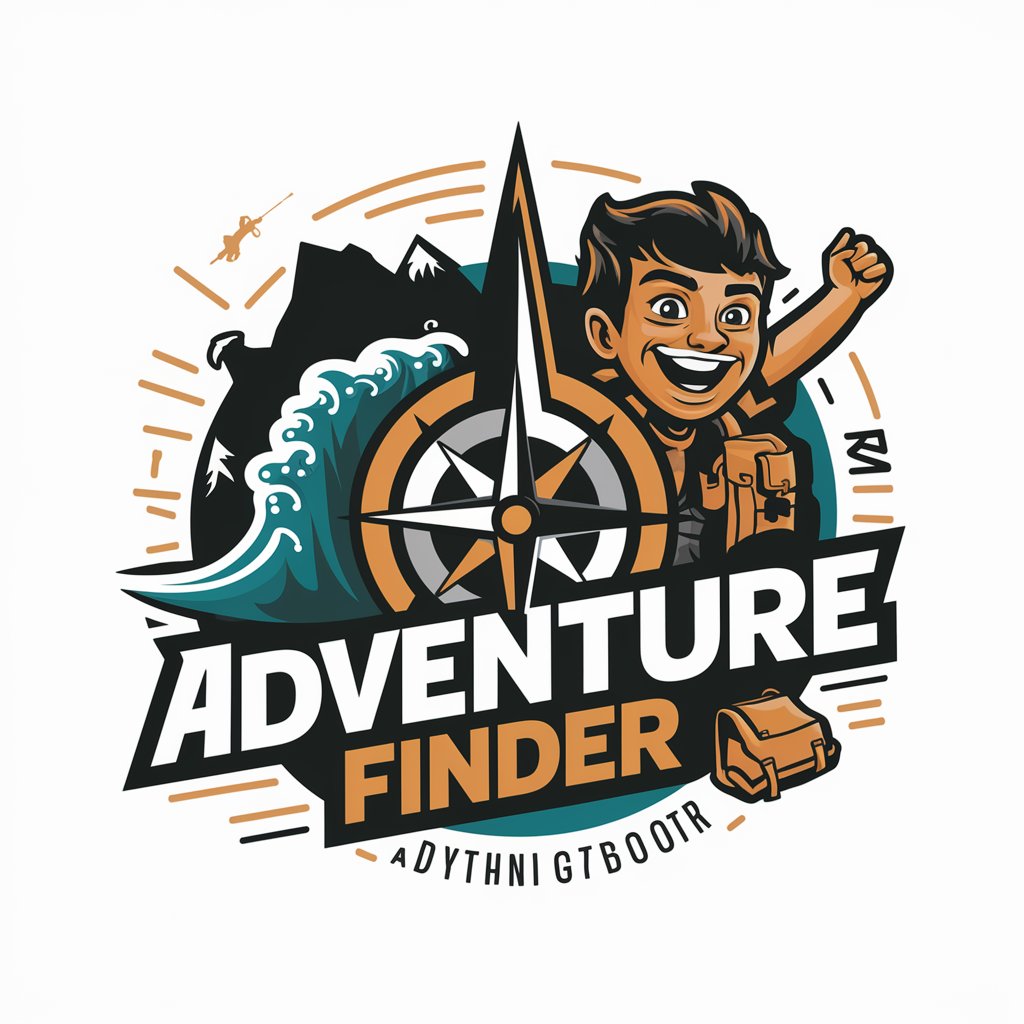
JavaScript and Cloud: Scaling the Cloud Frontier
Elevate Your Code to the Cloud
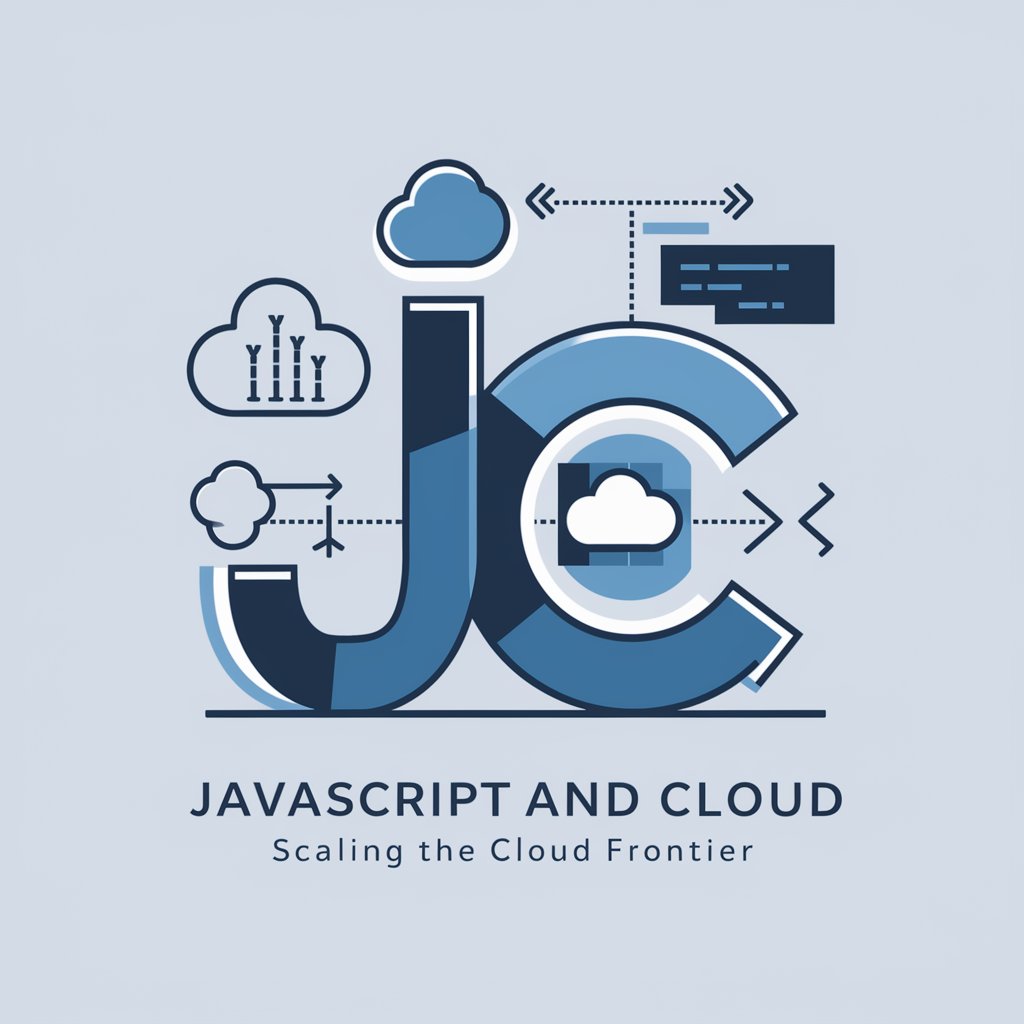
Python Cloud Deployment: Seamless & Secure
Deploy Python apps seamlessly with AI-powered cloud support.
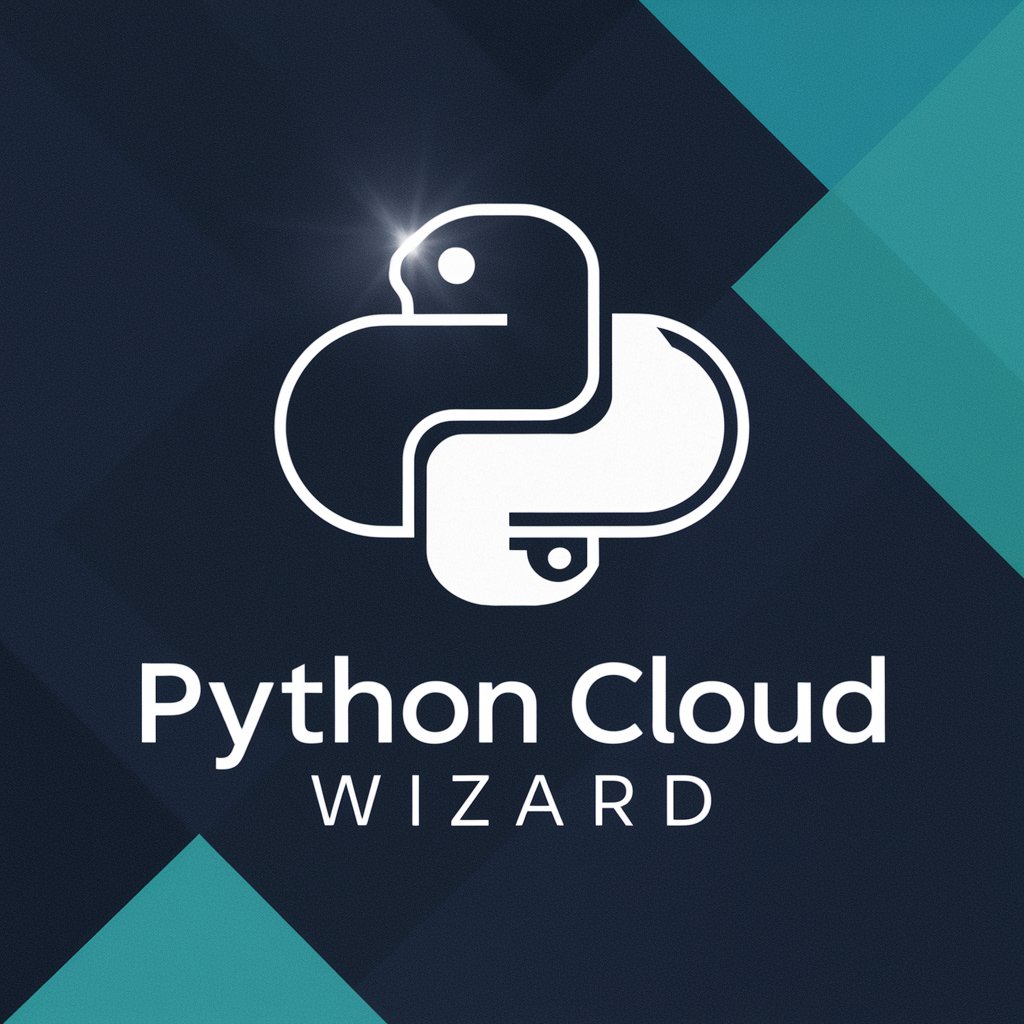
Protocol Pro
Streamlining Instruction with AI
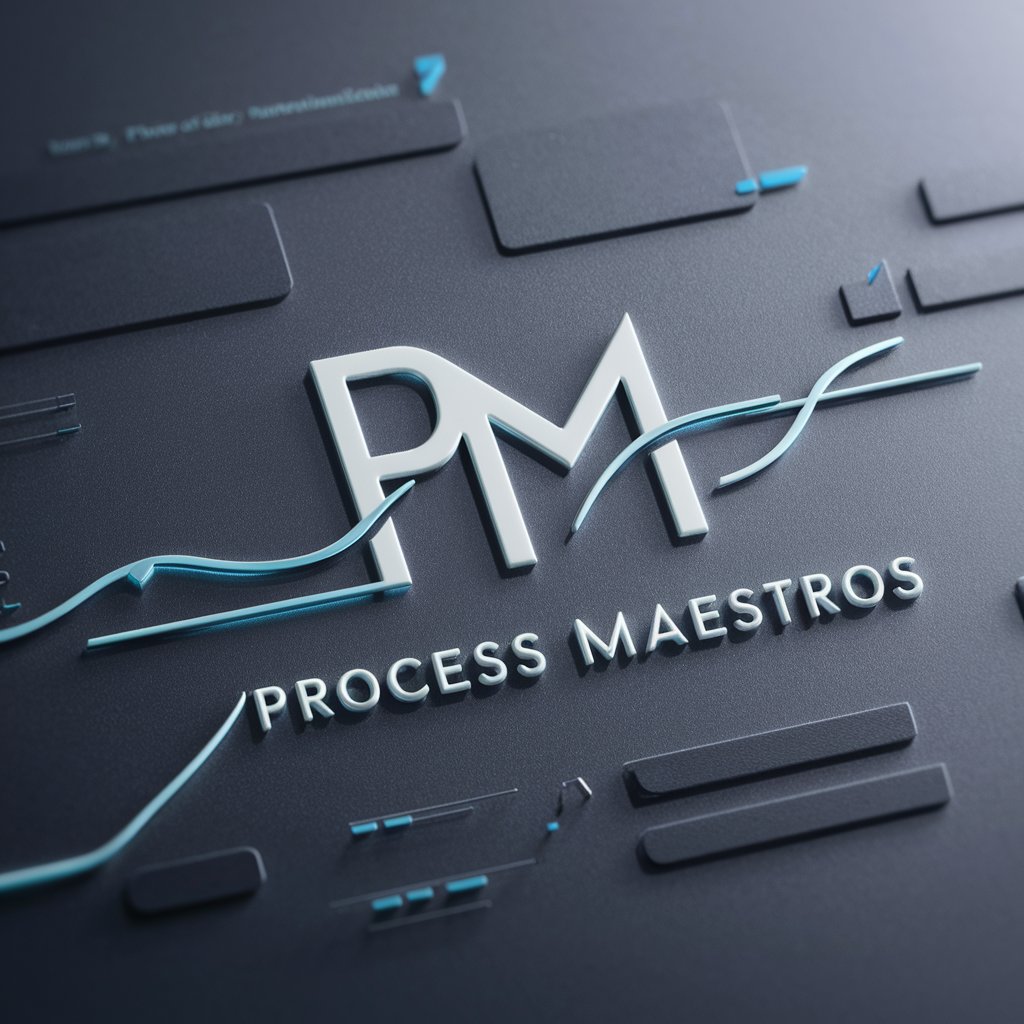
Book Scout
Unleashing Literature with AI
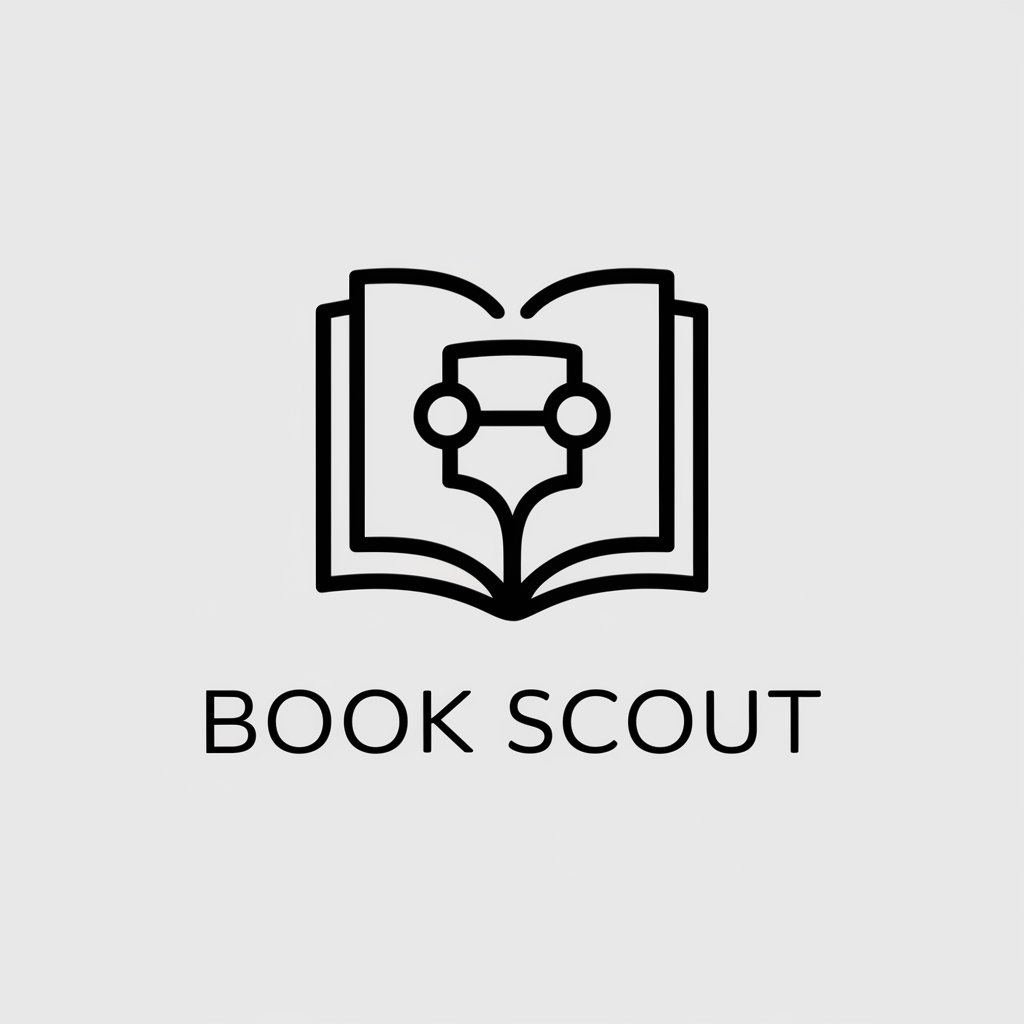
Culinary Creator Vege Friendly
Crafting Vegetarian Delights with AI
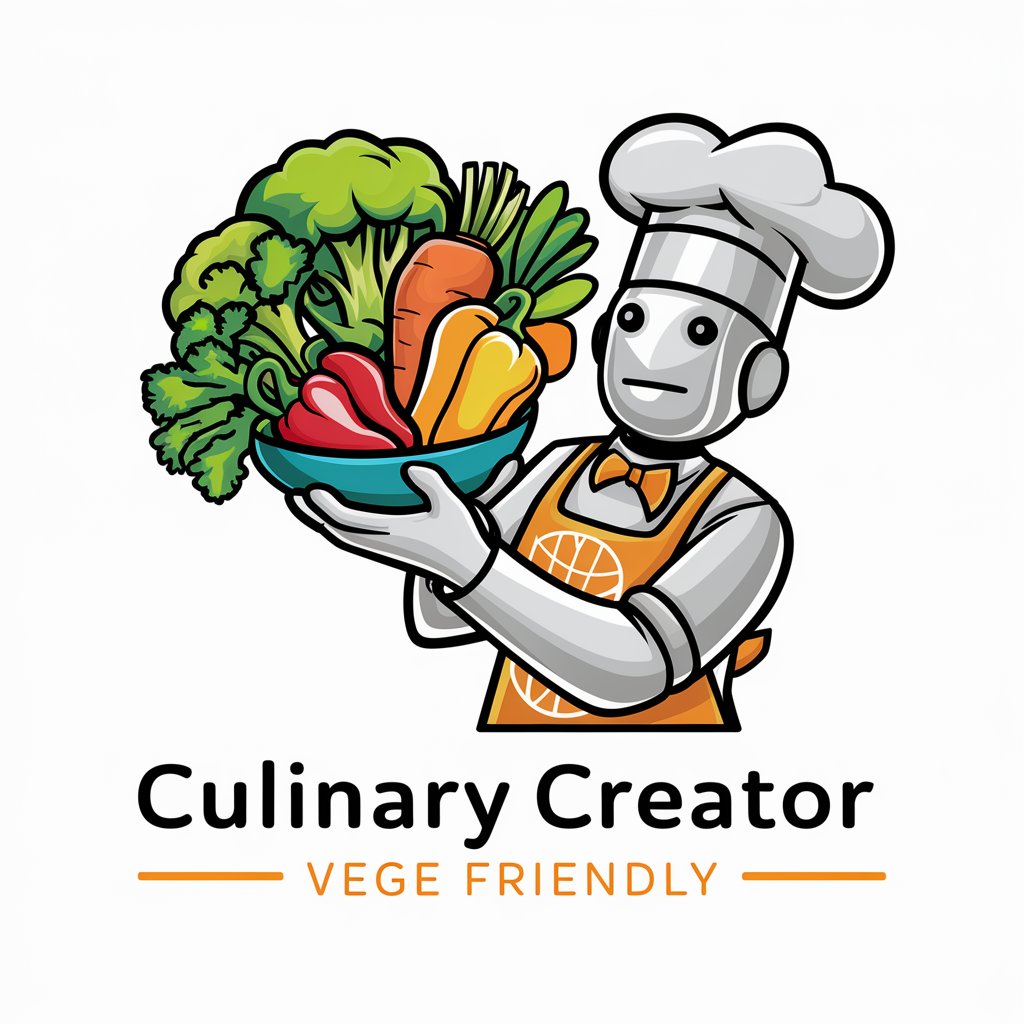
Anxiety Ease Wizard - AI
Transform anxiety into calmness with AI-powered empathy.
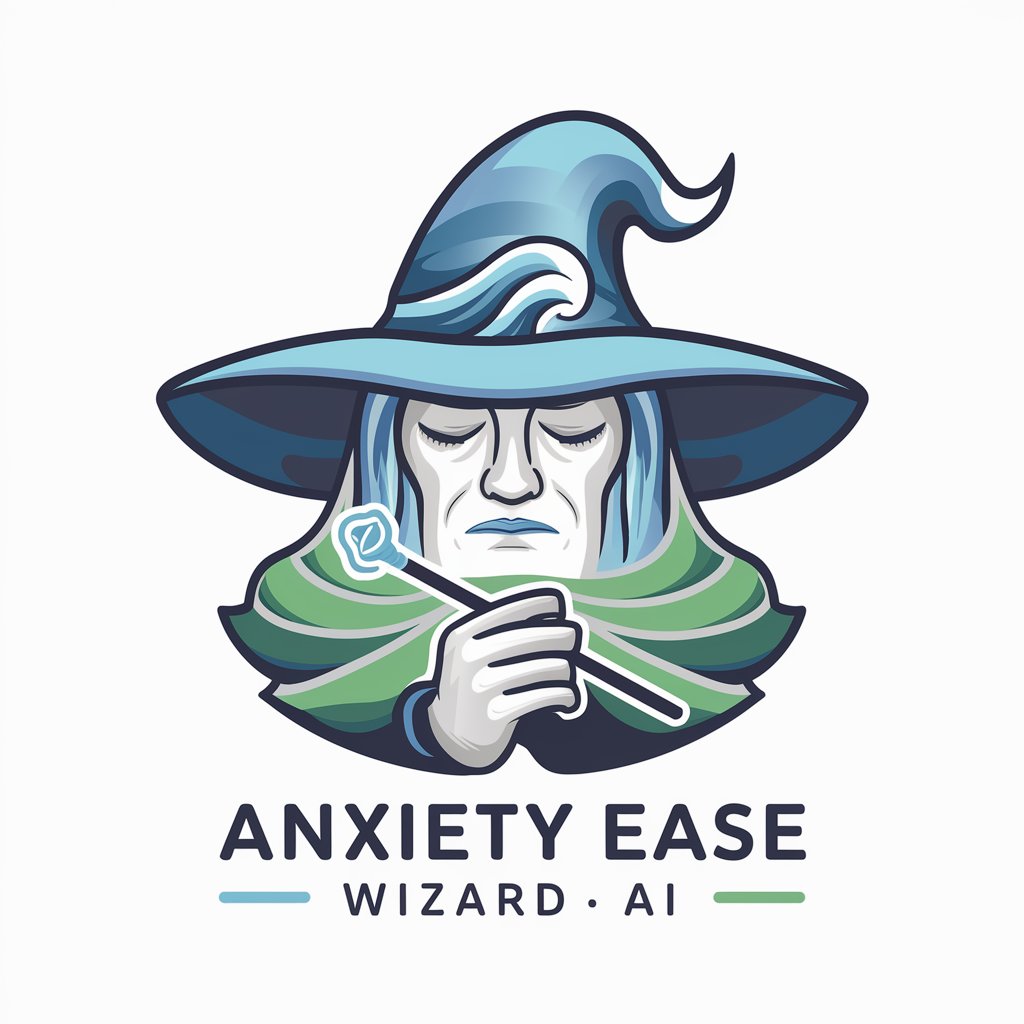
Party Talk Creator
Crafting Authentic In-game Conversations
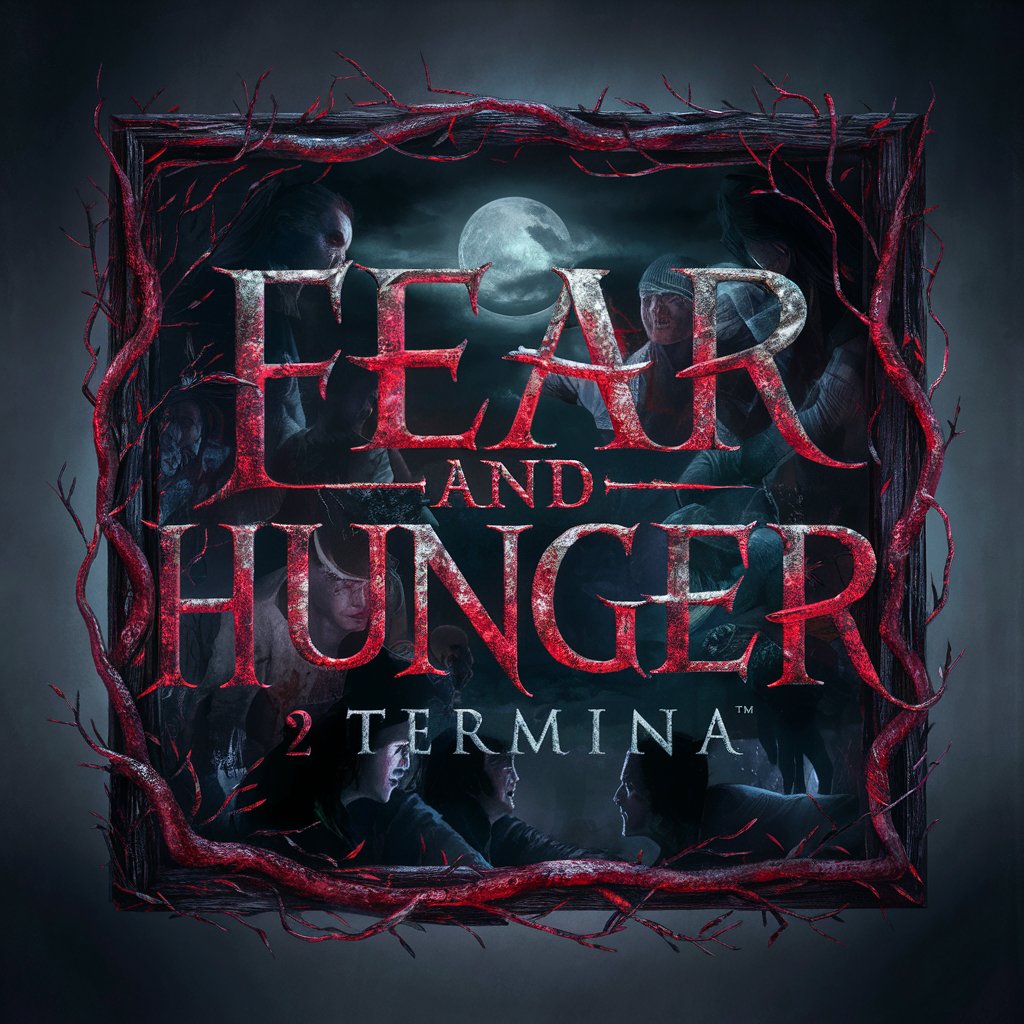
The Symbiosis of AI and Human Intuition
Empowering Decisions with AI-Driven Intuition
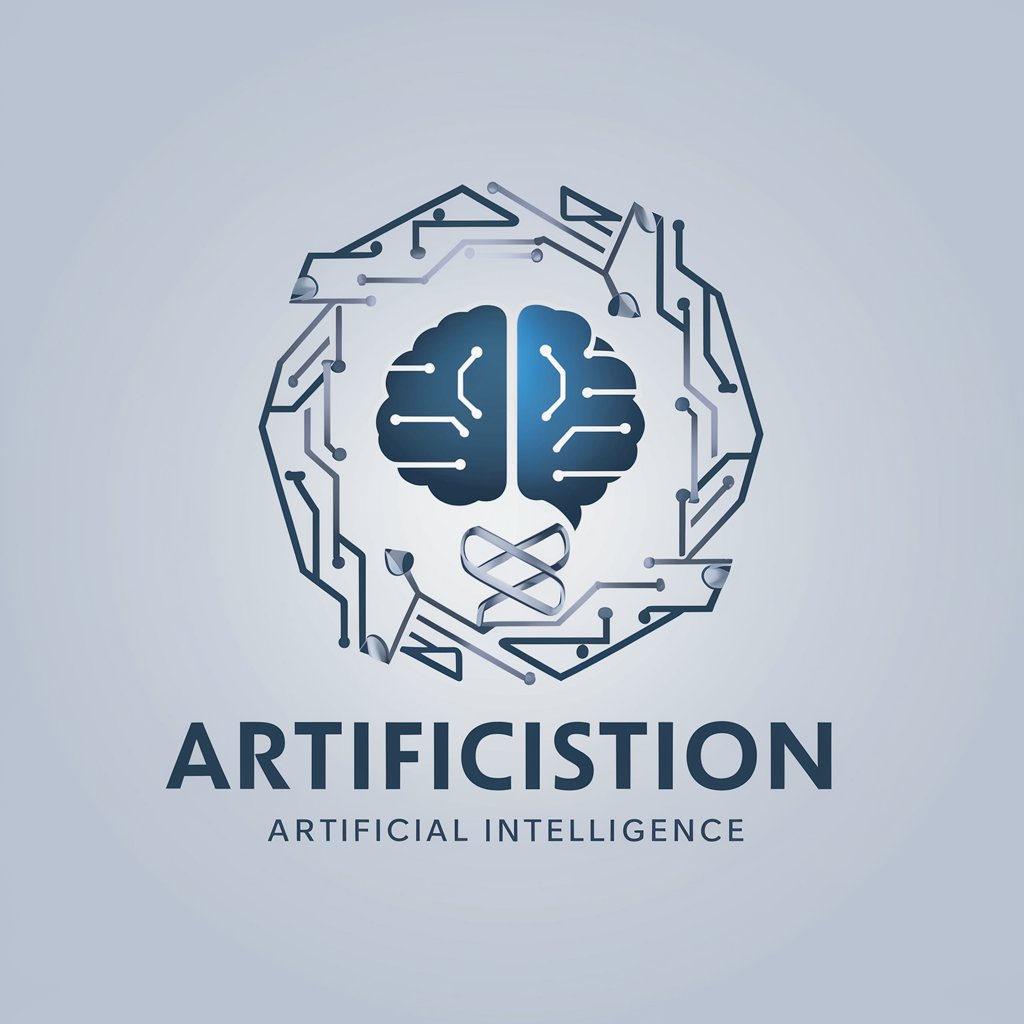
Middle Ground
Discover Unseen Connections, Powered by AI
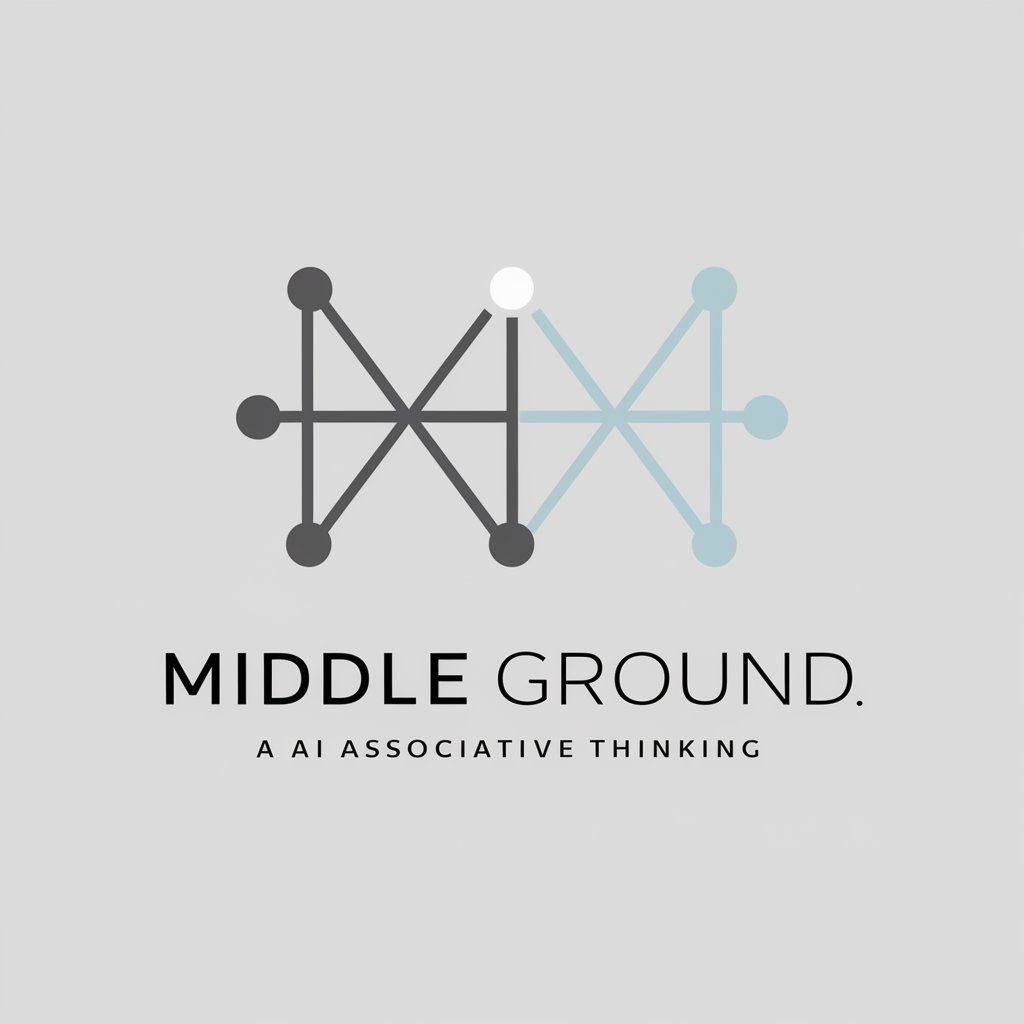
👨💻 C++ Compilation with g++
Effortless C++ compilation with AI insights.
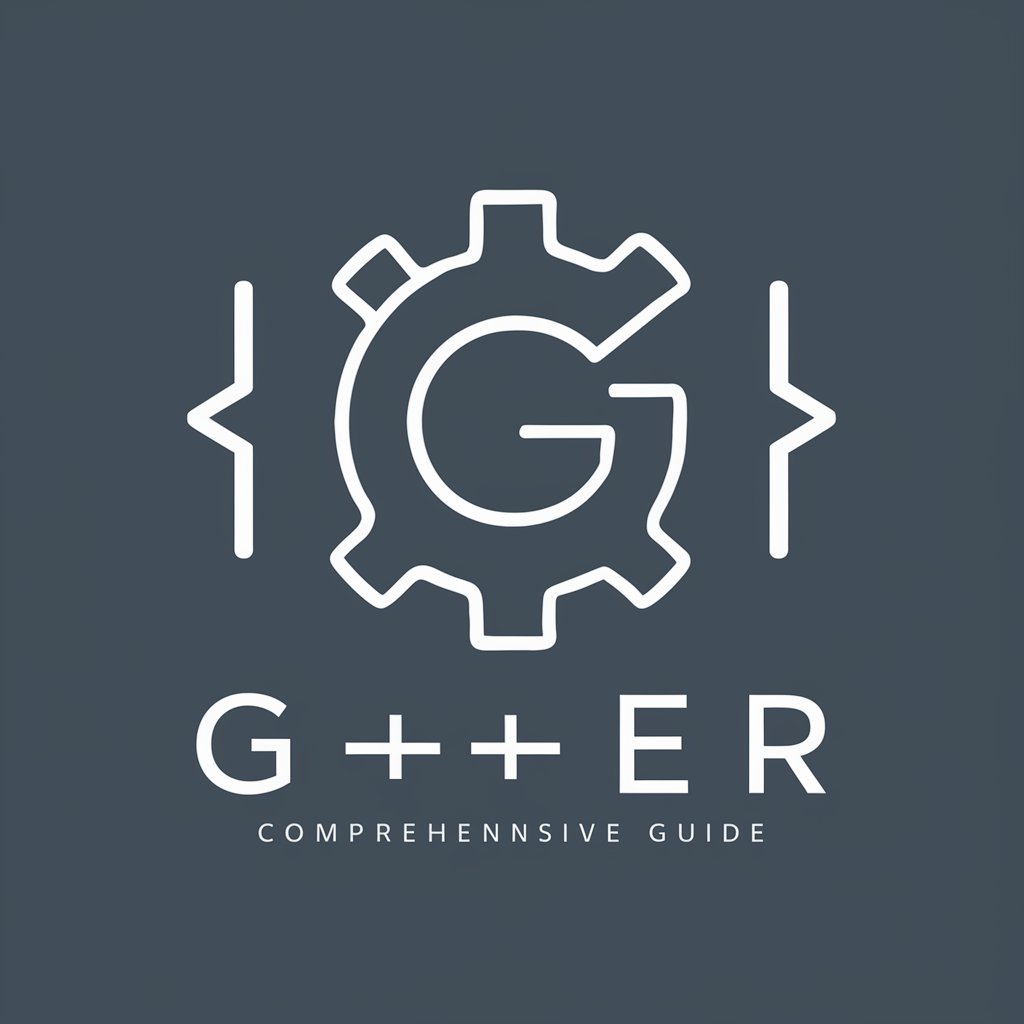
FAQs about Go Context: Timeout & Cancellation Expertise
What is the primary use of Go's context package?
The primary use is to manage request lifecycles, particularly handling timeouts and cancellations in a controlled and consistent manner across concurrent operations.
How can one handle resource leaks using context?
By using context to manage timeouts and cancellations, you can ensure that goroutines and other resources are properly released when they are no longer needed, thereby preventing leaks.
What are some common pitfalls when using context in Go?
Common pitfalls include passing contexts too broadly, creating contexts without cancellation, and not propagating contexts through your application's call stack.
How do I set a timeout for a request using context?
You can set a timeout by using the context.WithTimeout function, which creates a new context that automatically cancels itself after the specified duration.
Can context be used for non-HTTP requests?
Yes, the context package is designed to be used broadly across all types of operations, not just HTTP requests, including database calls, file I/O, and other long-running processes.