C++ Exception Handling Explained-C++ Exception Handling Guide
Master C++ errors with AI-driven insights
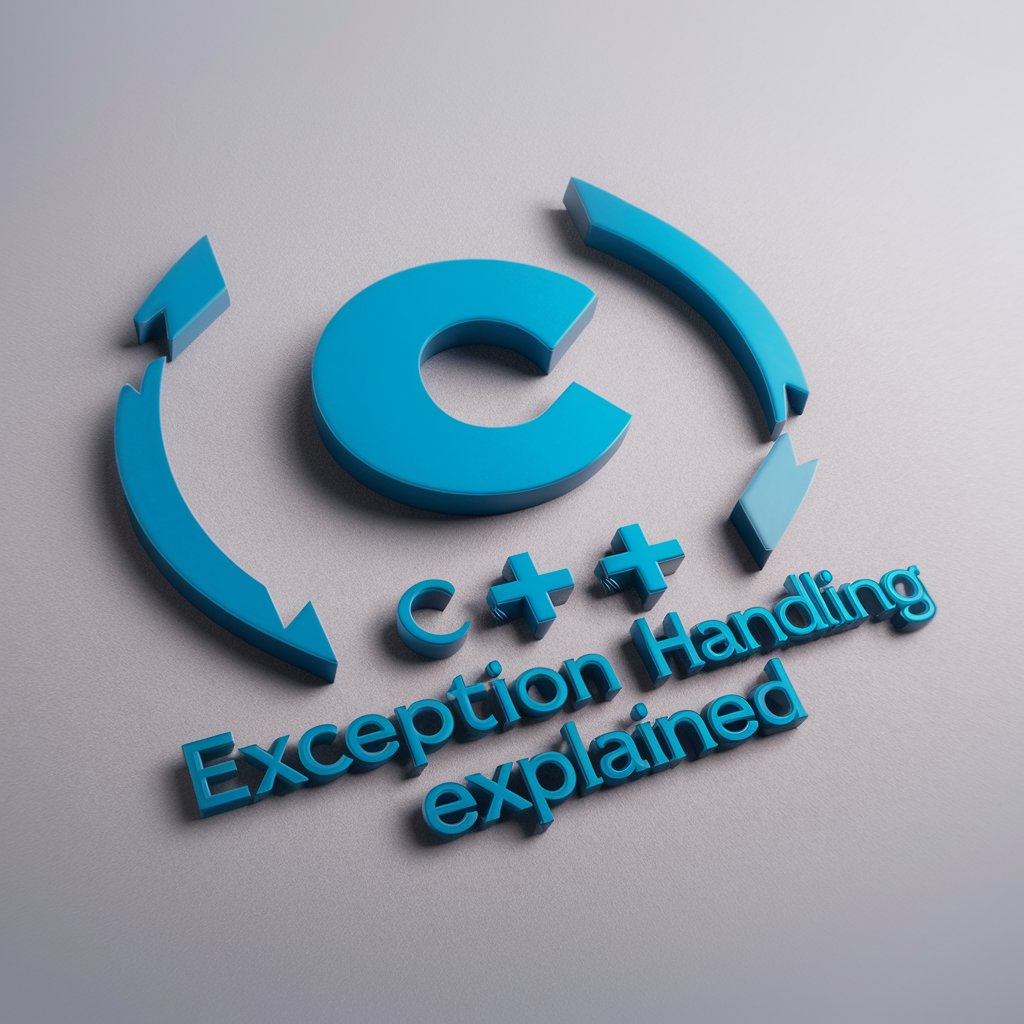
Explain the role of try-catch blocks in C++ with an example.
How can I create a custom exception class in C++?
What are the best practices for handling multiple exceptions in C++?
Discuss the concept of noexcept and its usage in C++.
Related Tools
Load More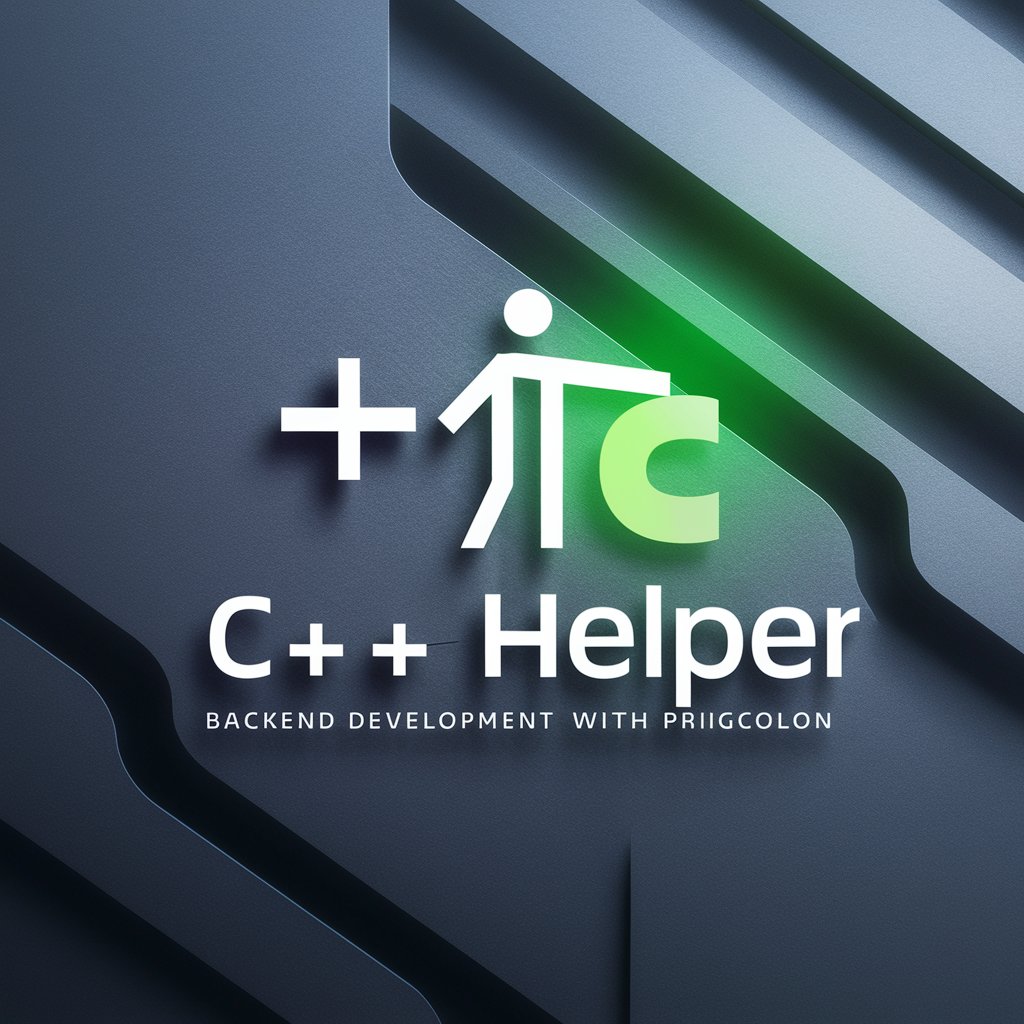
C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.
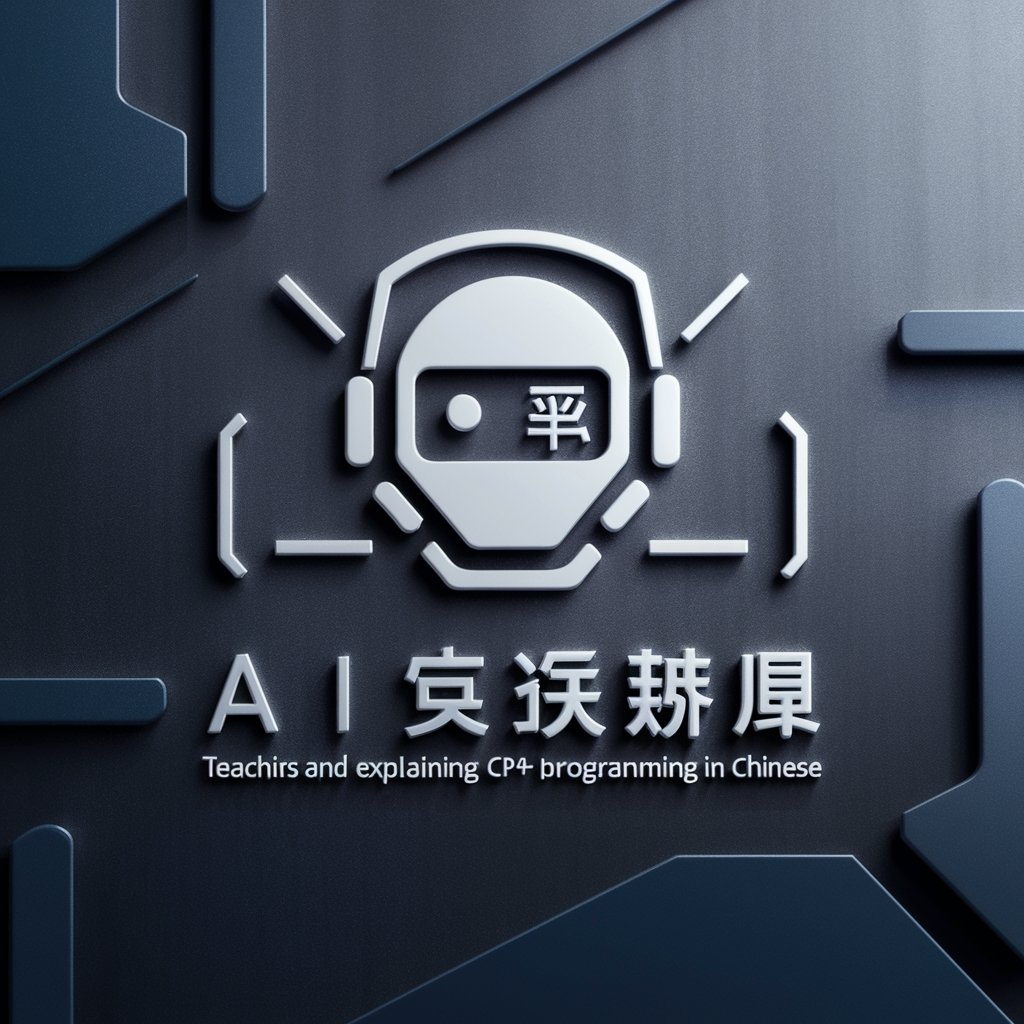
Modern CPP Expert
专注于现代 C++ 教学的专家,使用中文
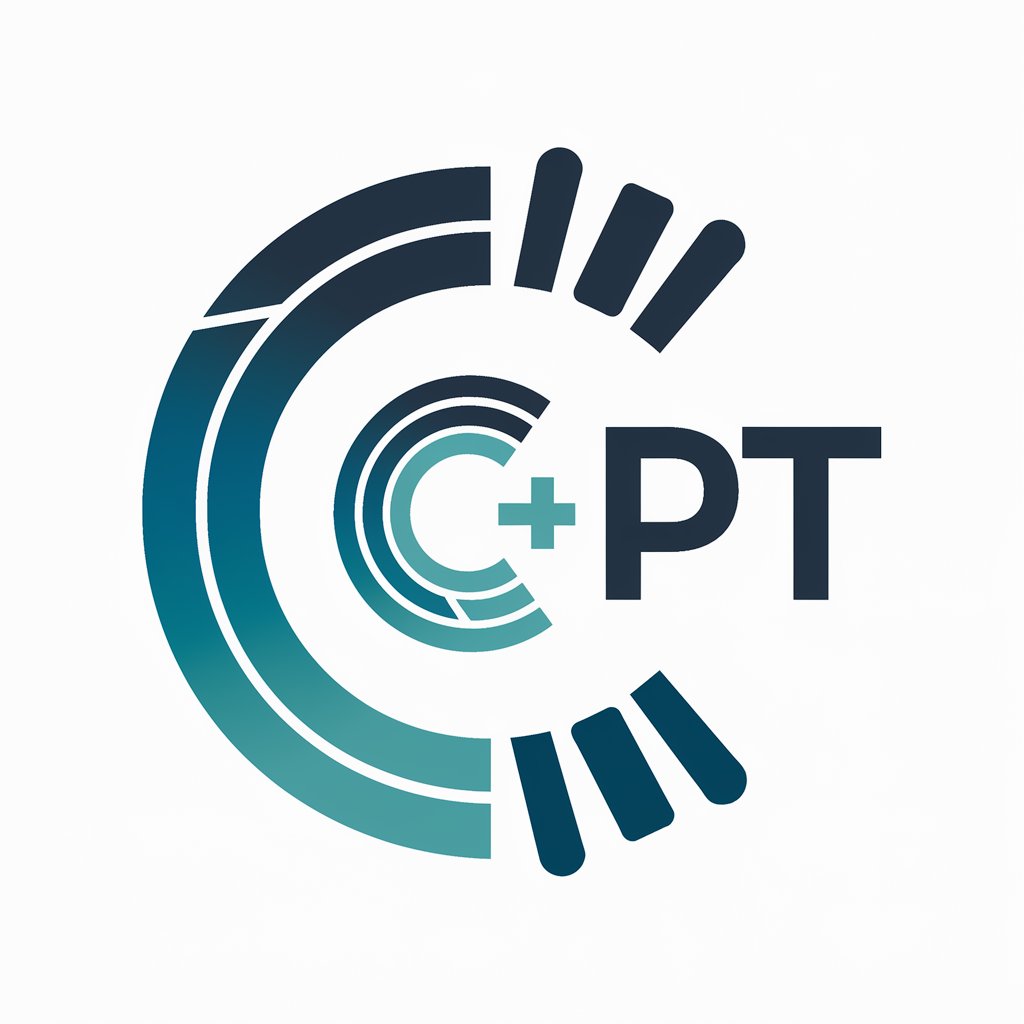
C++
A C++ programming expert for accurate answers and guidance.
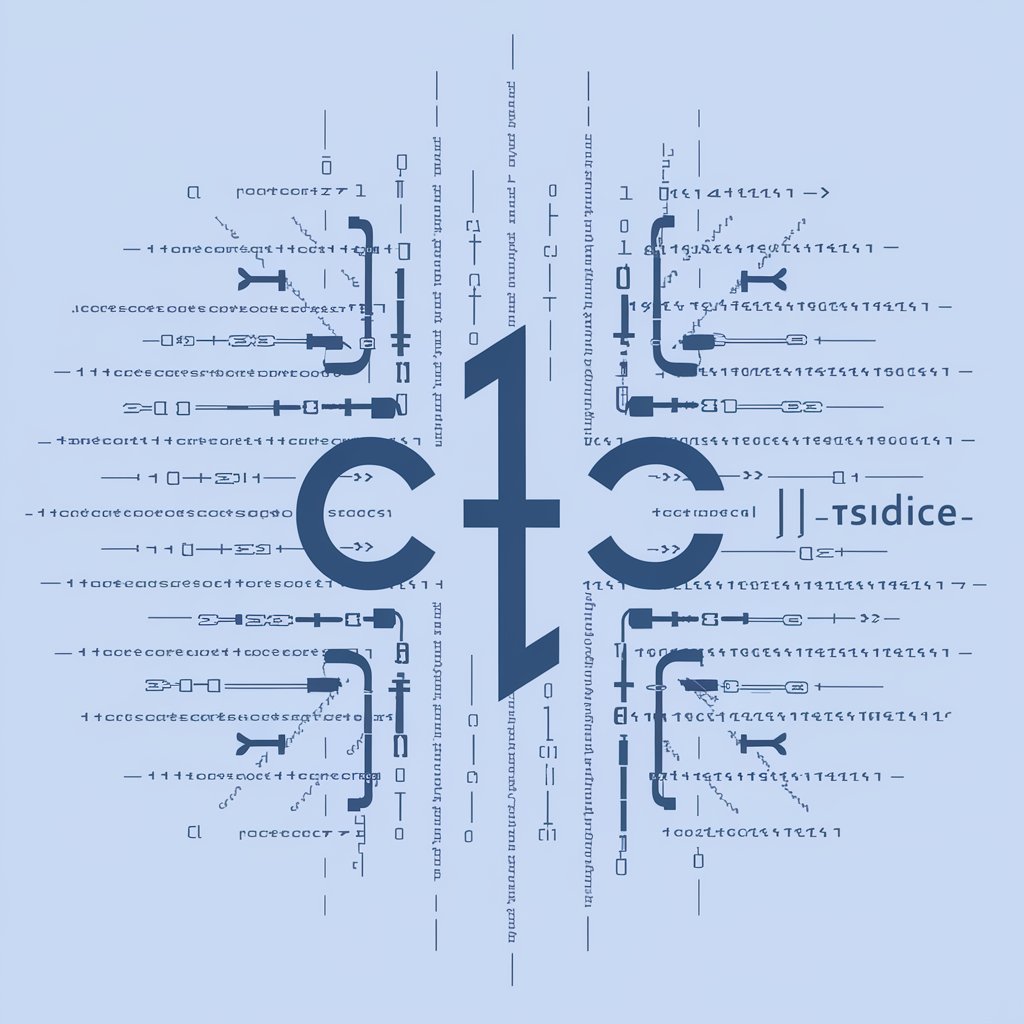
C++
中文编程问题解答,专注C++和竞赛题目,代码简洁
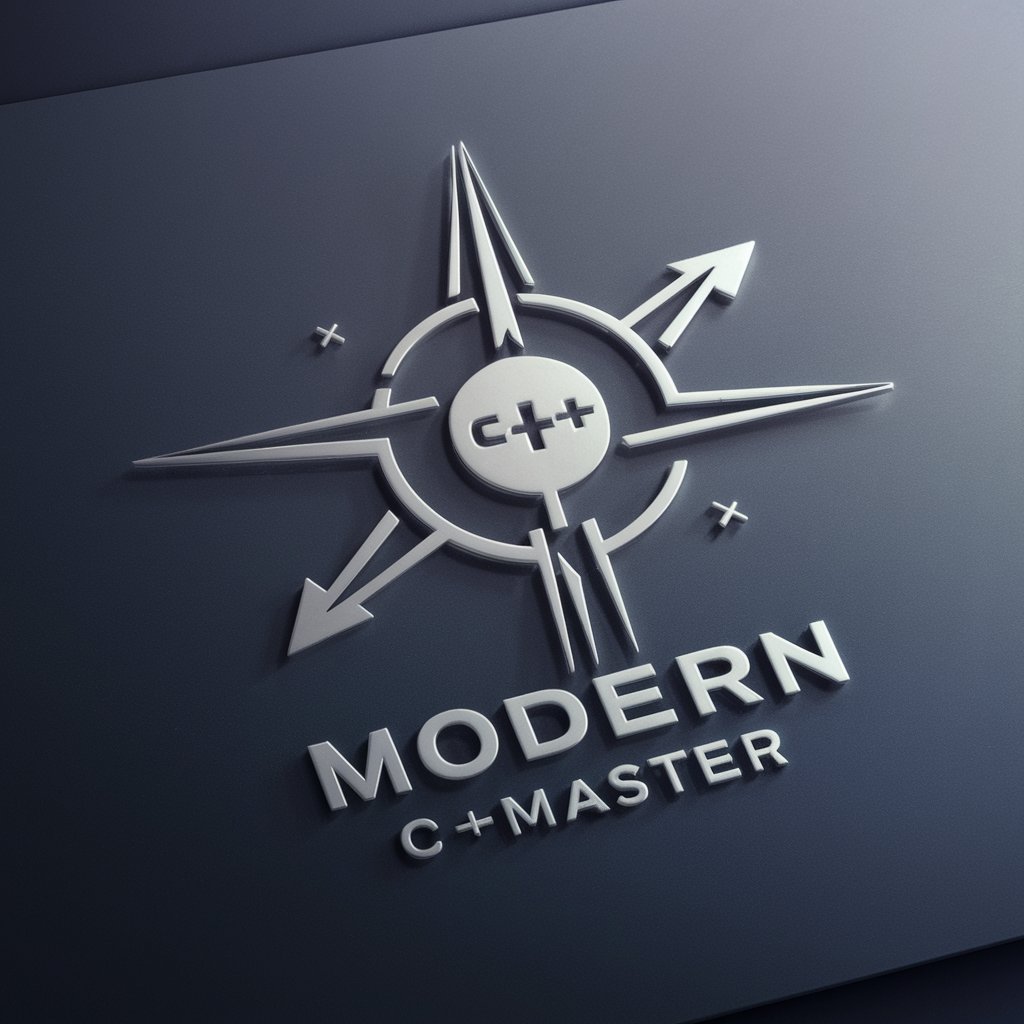
Modern C++ Master
Answers anything about C++
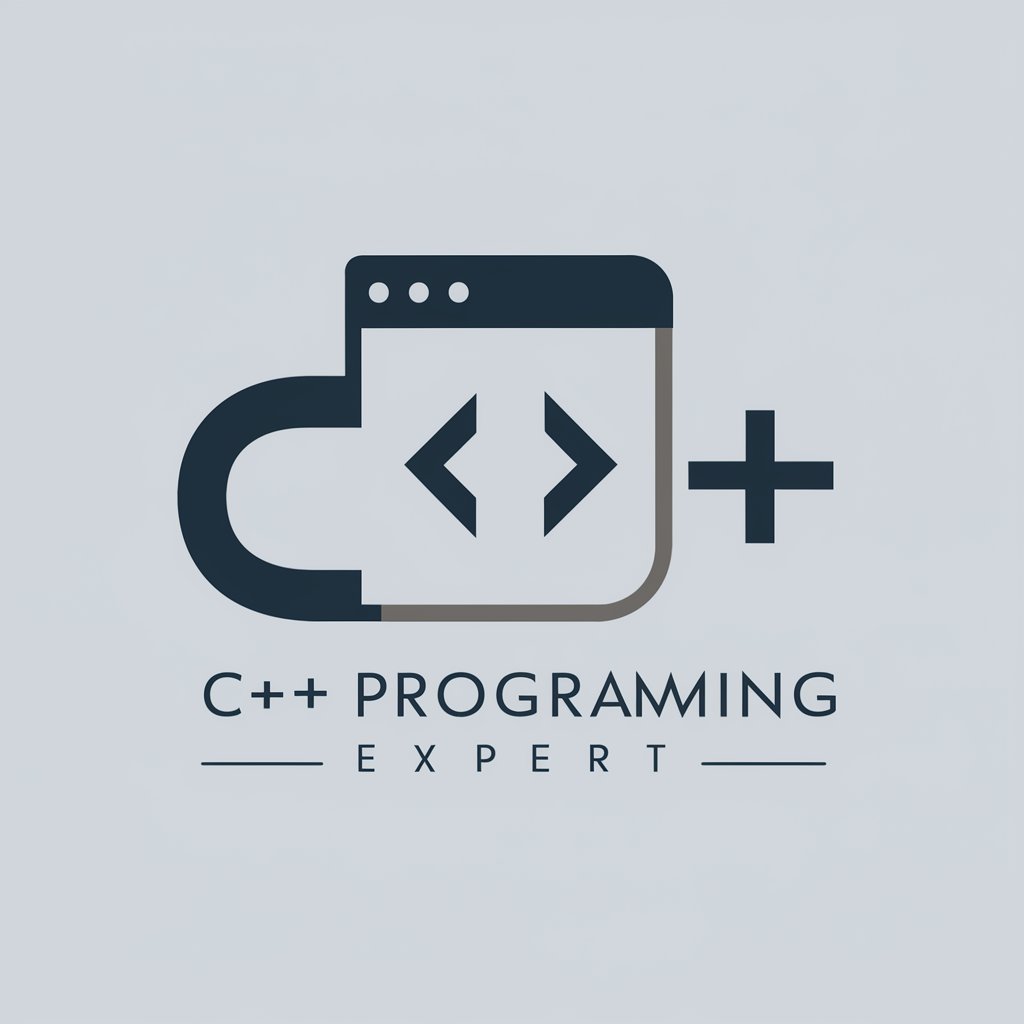
C++ Programming Expert
C++ Exception Handling Explained: An Overview
C++ Exception Handling Explained is designed to guide programmers through the intricacies of managing errors and exceptions in C++ applications. Exception handling in C++ is a powerful mechanism for managing runtime errors, providing a structured approach to error detection and recovery. It revolves around three main keywords: try, catch, and throw. A try block encloses code that might throw an exception, and catch blocks are used to handle the exception. The throw keyword is used to indicate an error condition. This system allows programs to be more robust, maintainable, and readable by separating error-handing code from regular logic. For example, when accessing a file or parsing data, exceptions can be used to handle cases where the file does not exist or the data is in an incorrect format, allowing the program to gracefully recover or terminate. Powered by ChatGPT-4o。
Key Functions of C++ Exception Handling
Standard Exception Handling
Example
try { throw std::out_of_range("Index out of range"); } catch (const std::exception& e) { std::cerr << e.what(); }
Scenario
When accessing elements outside the bounds of a container, a std::out_of_range exception can be thrown and caught, providing a clear error message to the user.
Custom Exception Classes
Example
class MyException : public std::exception { public: const char* what() const throw() { return "Custom error occurred"; } }; try { throw MyException(); } catch (const MyException& e) { std::cerr << e.what(); }
Scenario
For domain-specific errors not covered by standard exceptions, custom exceptions allow developers to provide tailored error messages and handling logic.
Exception Propagation
Example
void funcB() { throw std::runtime_error("Error in funcB"); } void funcA() { try { funcB(); } catch (...) { throw; } }
Scenario
Exceptions can be propagated up the call stack, allowing higher-level functions to catch and handle errors from lower-level functions.
Noexcept Specification
Example
void noThrowFunc() noexcept { /* Guaranteed not to throw */ }
Scenario
Marking functions with noexcept indicates they are guaranteed not to throw exceptions, aiding in optimization and clarity of design.
Target User Groups for C++ Exception Handling Explained
Software Developers
Professionals or hobbyists developing applications in C++ who need to ensure their code is robust against runtime errors and exceptions.
System Architects
Designers of large systems looking for strategies to make their applications resilient and maintainable through effective error handling.
Educators and Students
Teachers and learners in computer science focusing on advanced C++ programming concepts, including error management and exception safety.
How to Utilize C++ Exception Handling Explained
Start Your Journey
Begin by visiting a platform offering comprehensive C++ exception handling guidance, ensuring access without the need for login or subscription.
Grasp the Basics
Familiarize yourself with the fundamentals of C++ exception handling, including the syntax and structure of try-catch blocks, and the standard exceptions provided by the C++ standard library.
Dive Deeper
Explore advanced topics such as custom exception classes, exception propagation, and the noexcept specifier to build robust and error-resilient applications.
Implement and Test
Apply your knowledge by writing C++ code that effectively uses exception handling mechanisms. Test different scenarios to ensure your application behaves as expected in error conditions.
Seek Feedback and Iterate
Share your code with peers or mentors for feedback. Iteratively refine your approach to exception handling based on practical experience and advice received.
Try other advanced and practical GPTs
Decision-maker
Empowering decisions with AI-powered wisdom
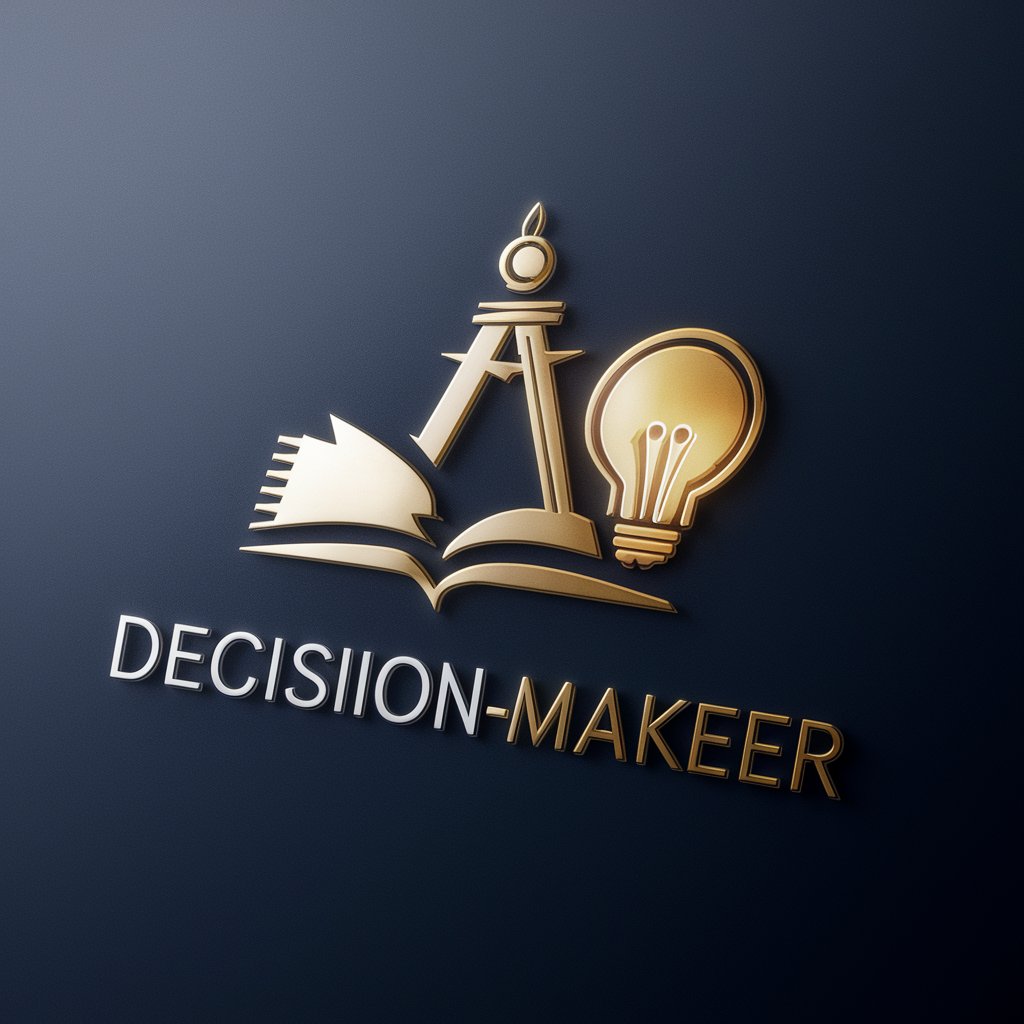
Risk Management Advisor
Empowering decision-making with AI-powered risk management.
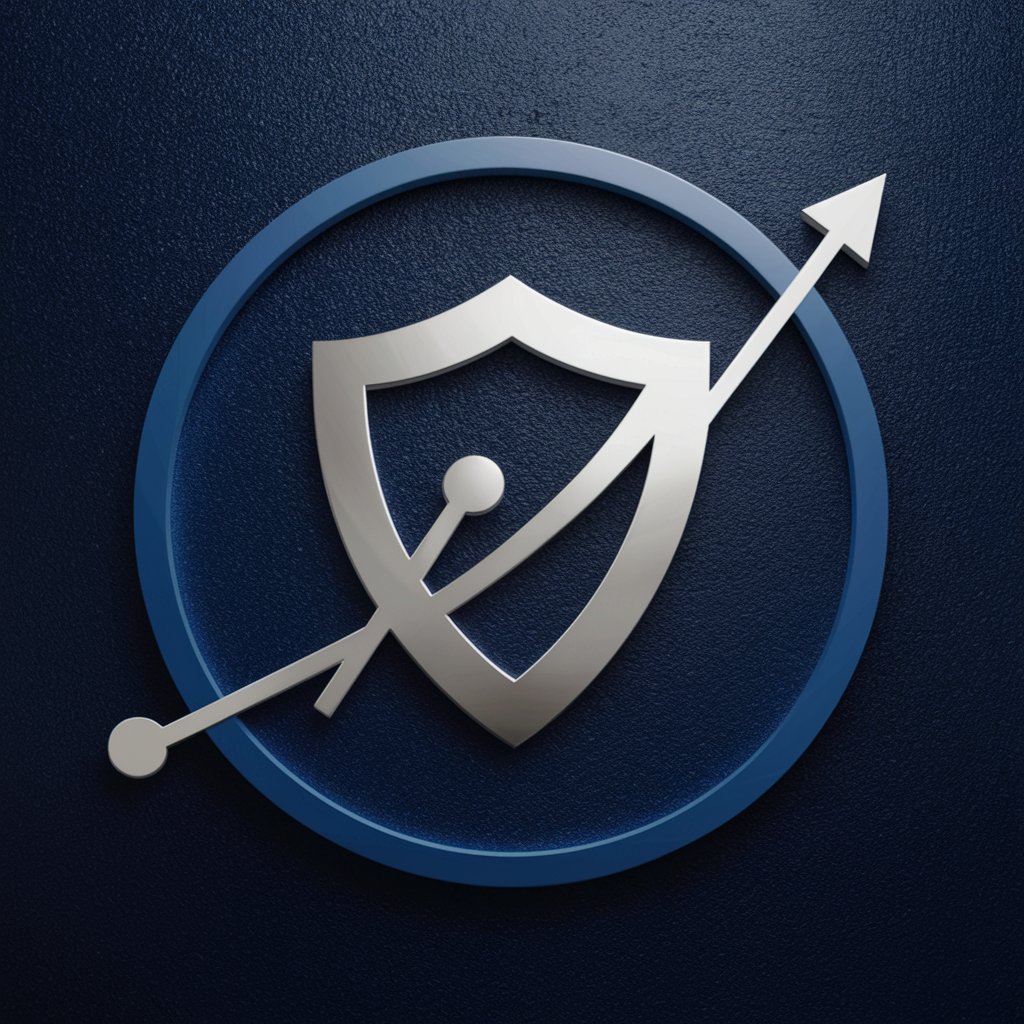
🎯 Kotlin's `when` Power User
Master Kotlin's `when` for smarter code.

Domain Scout
Unleash creativity with AI-powered literary insights
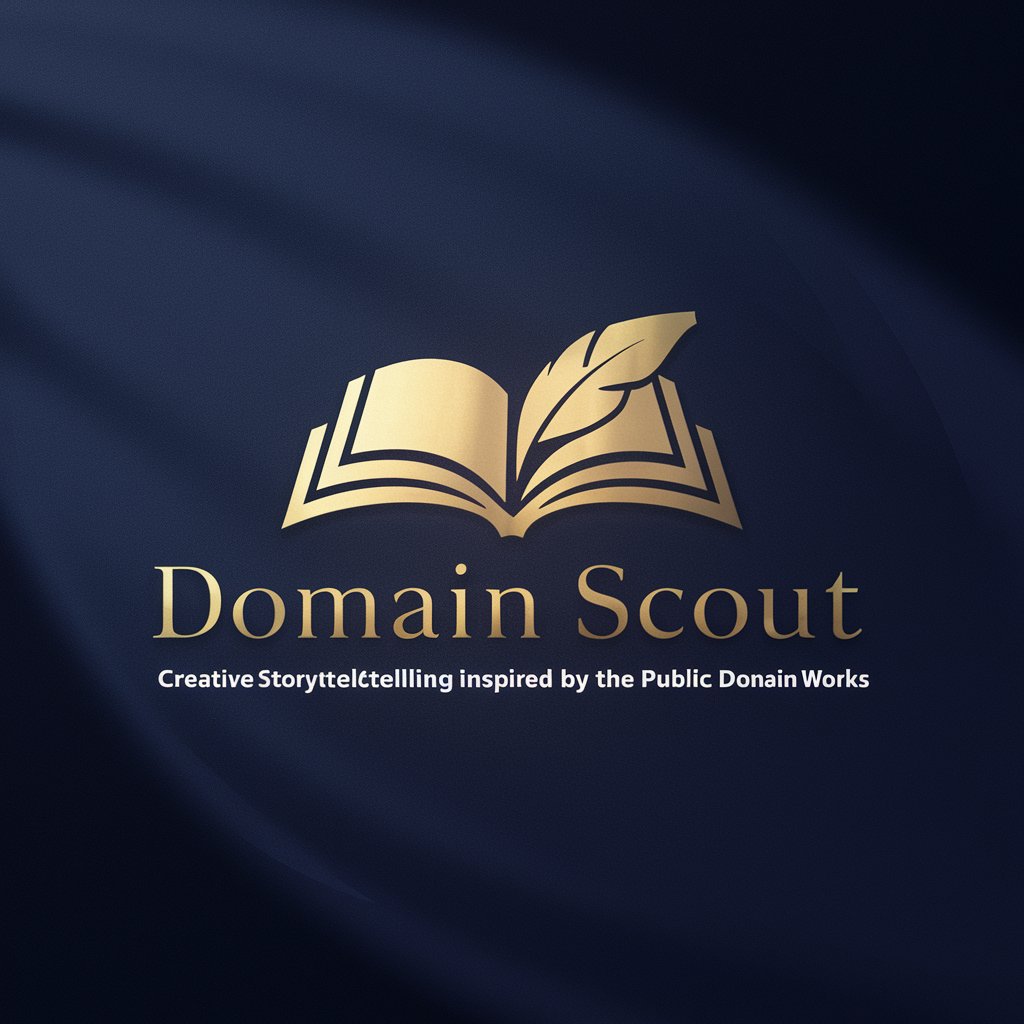
Domain Scout 💻 Check Domain Availability 🌐
AI-Powered Domain Discovery
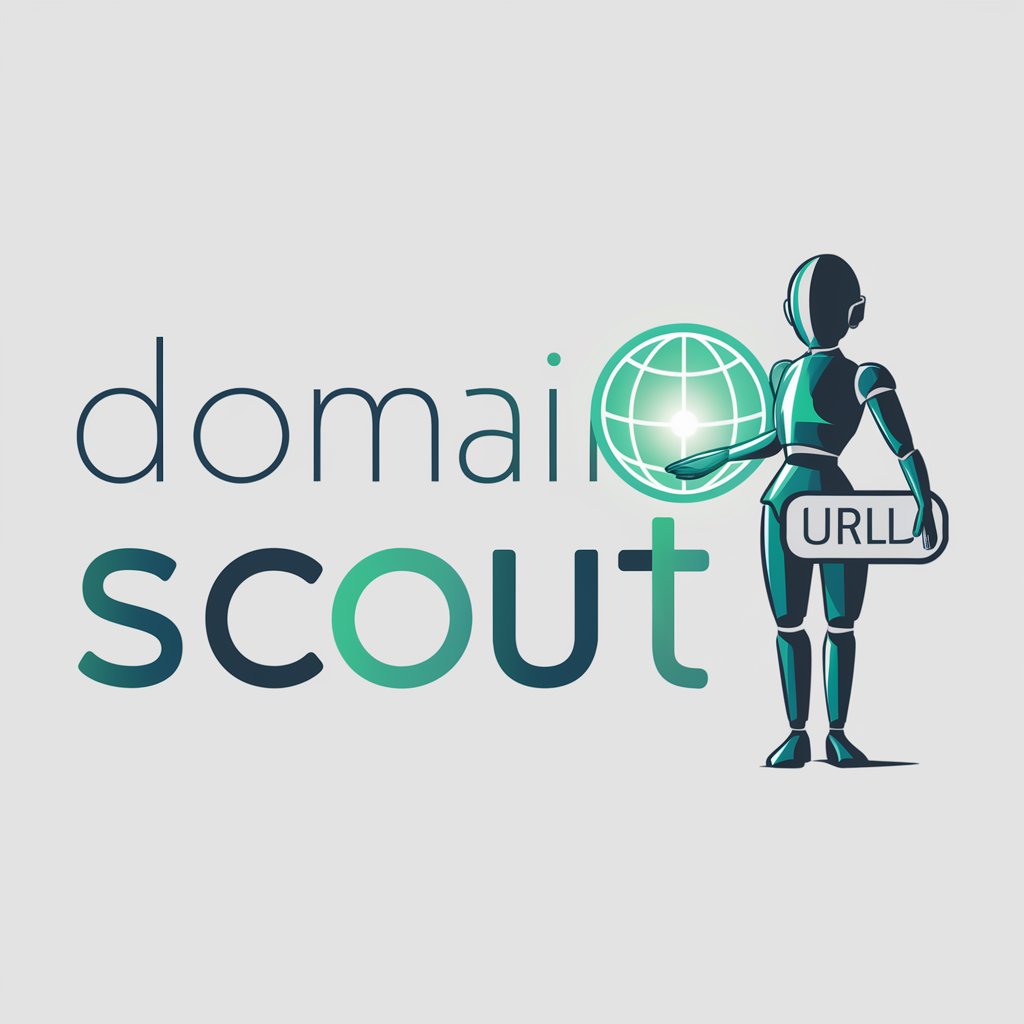
🐞 Debug ActionScript 3
Solve ActionScript 3 issues with AI-powered assistance
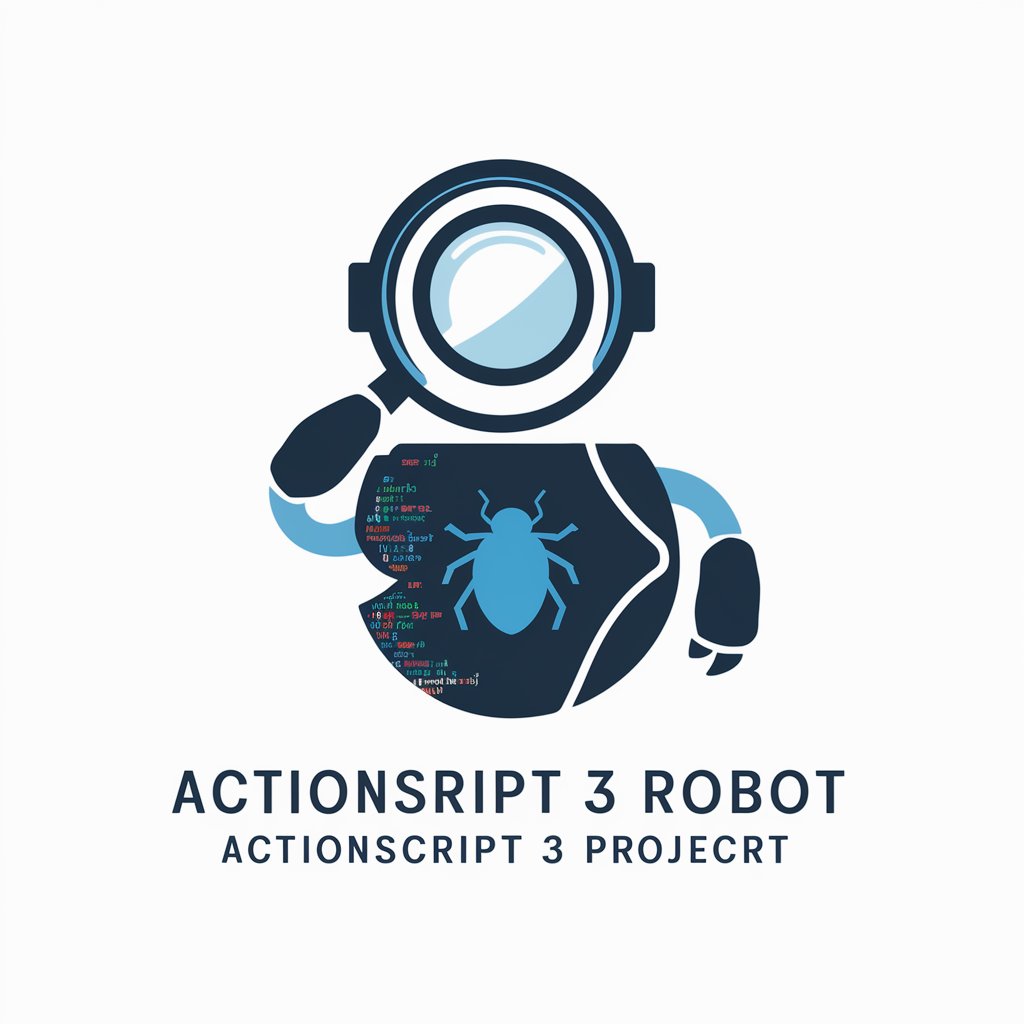
Python Chatbot Creation: ChatterBot Made Easy
Empower conversations with AI-driven chatbot technology.
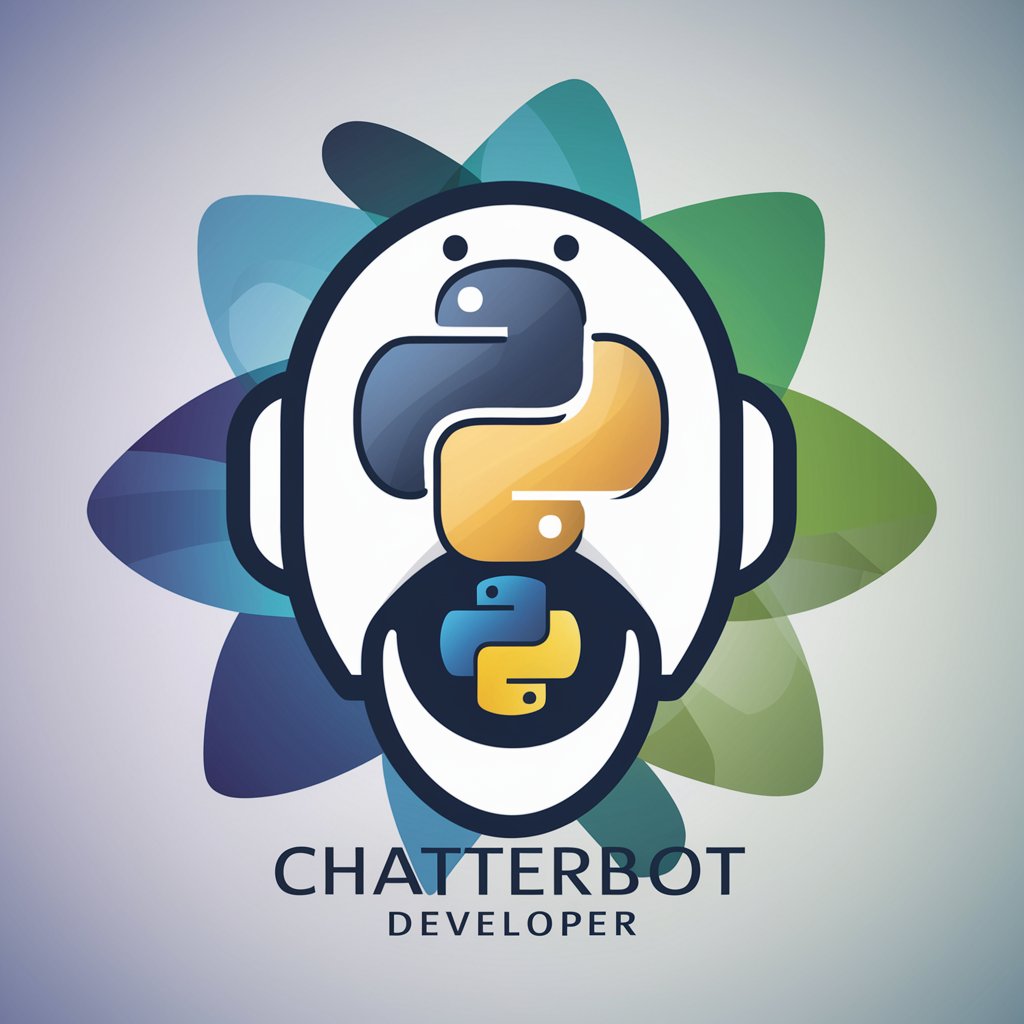
Automated Prompt Refinement
AI-powered prompt refinement for everyone.
Refining precious Metals - C.M. Hoke
Empowering Metal Refinement with AI
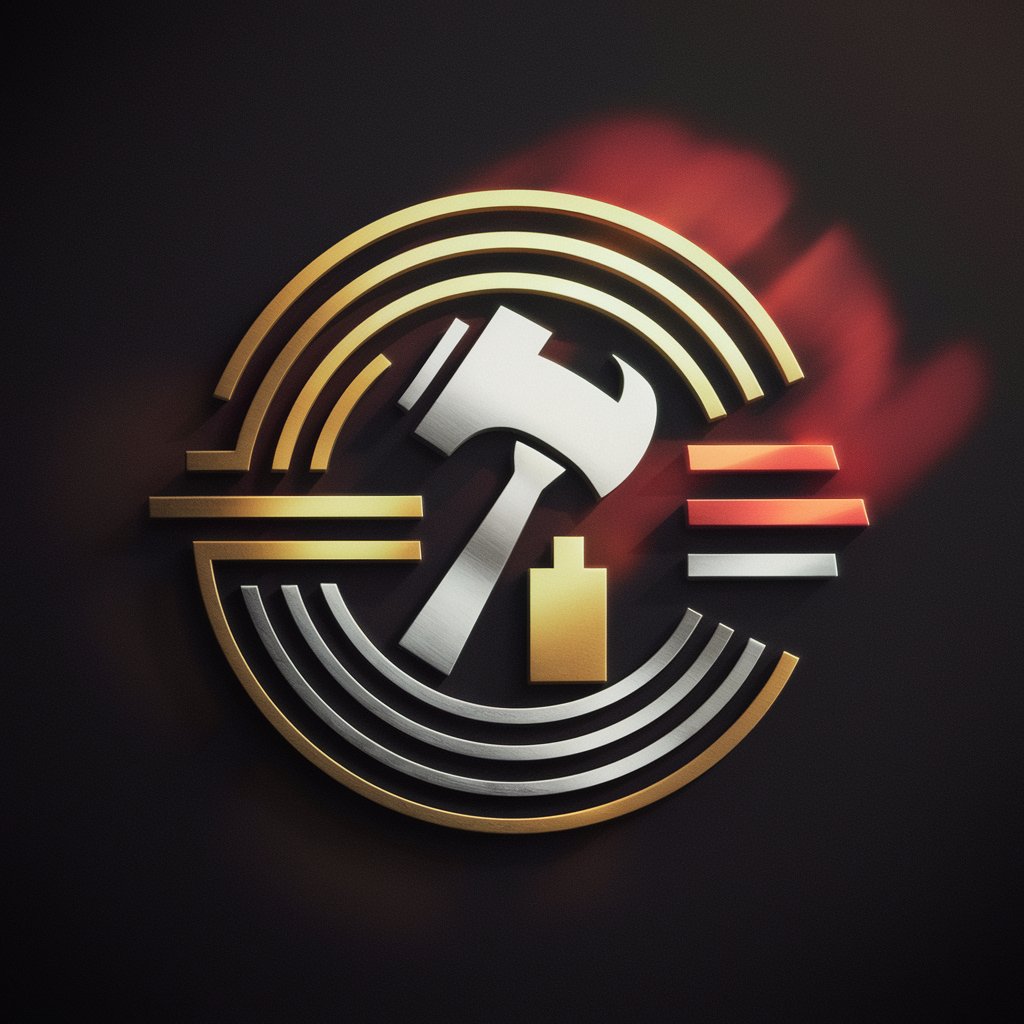
Text Analytics with R's Stringr Magic
Transform Text Data with AI-Powered Efficiency
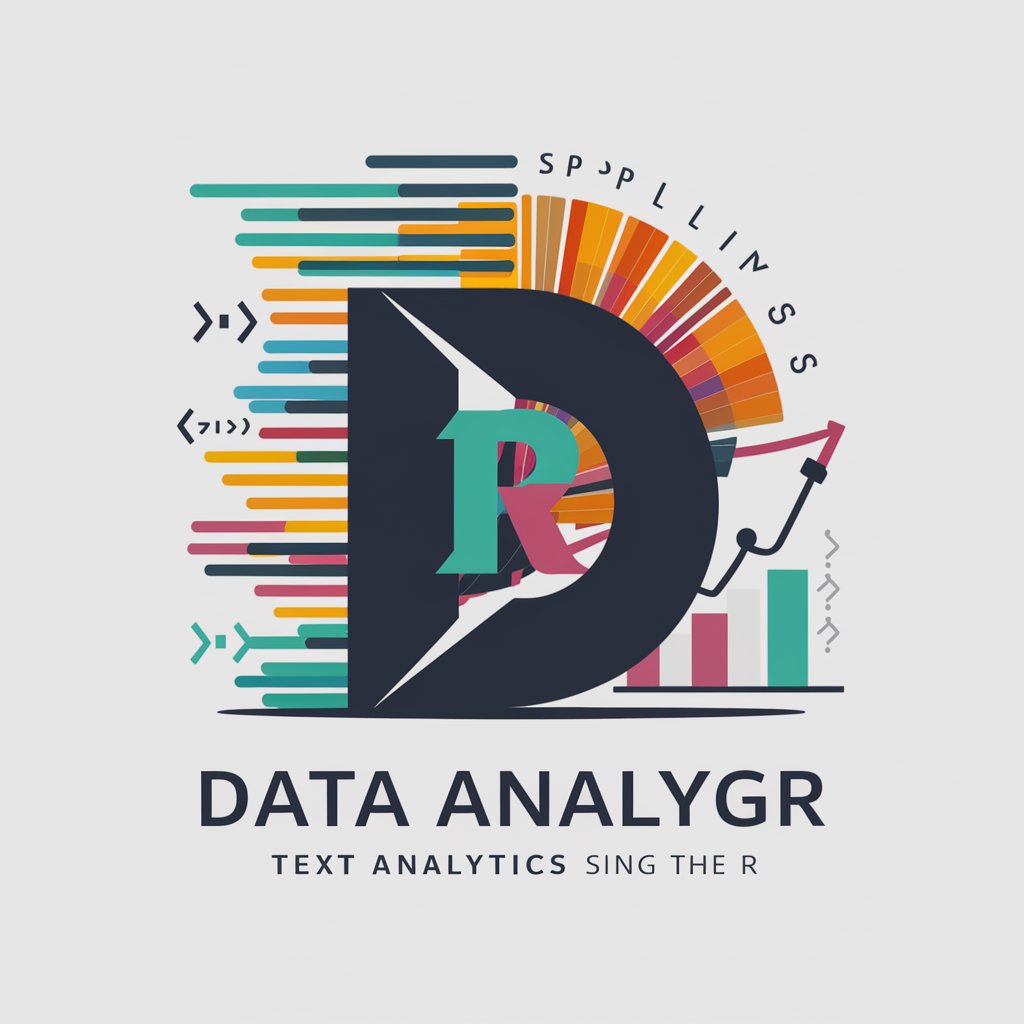
AI for Nonprofits: Volunteer and Intern Guide
Empower Nonprofit Volunteers with AI
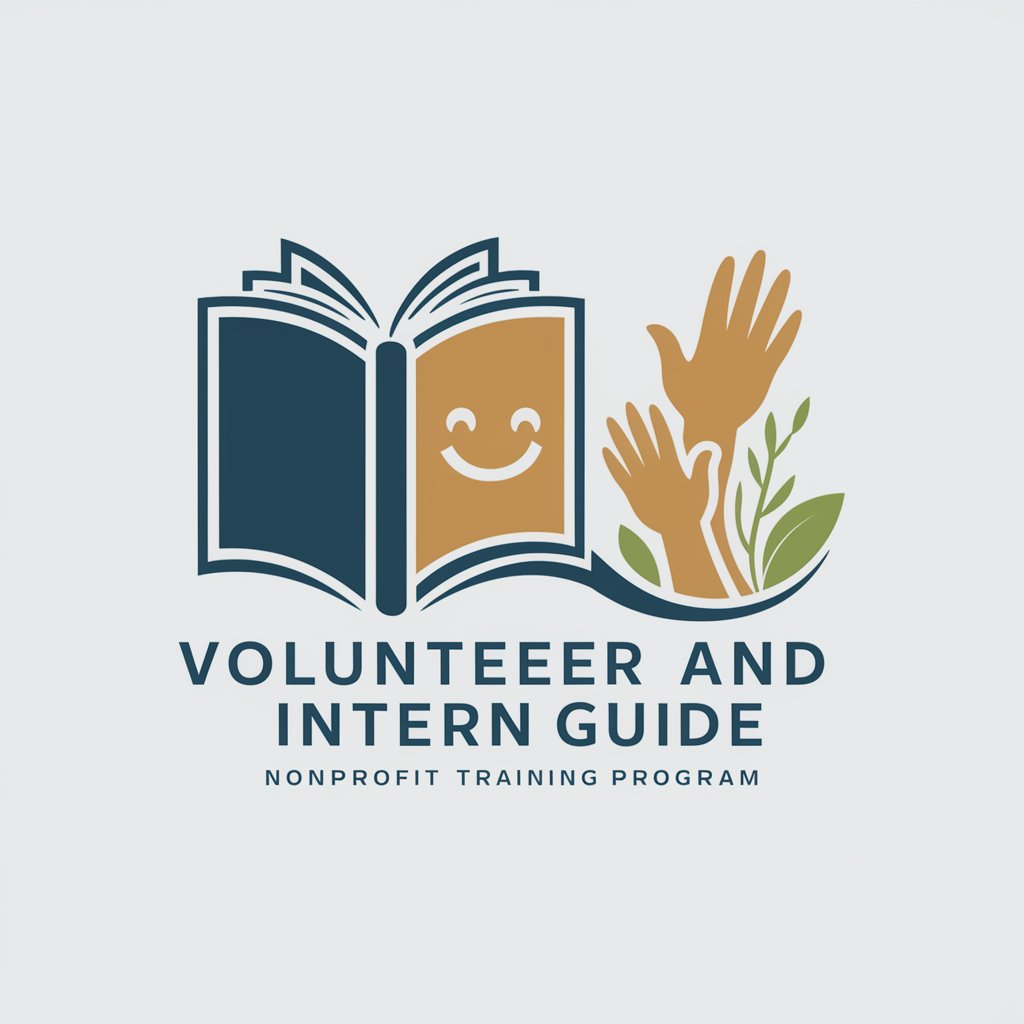
GPT Overused Phrase Remover
Revitalize Your Text with AI
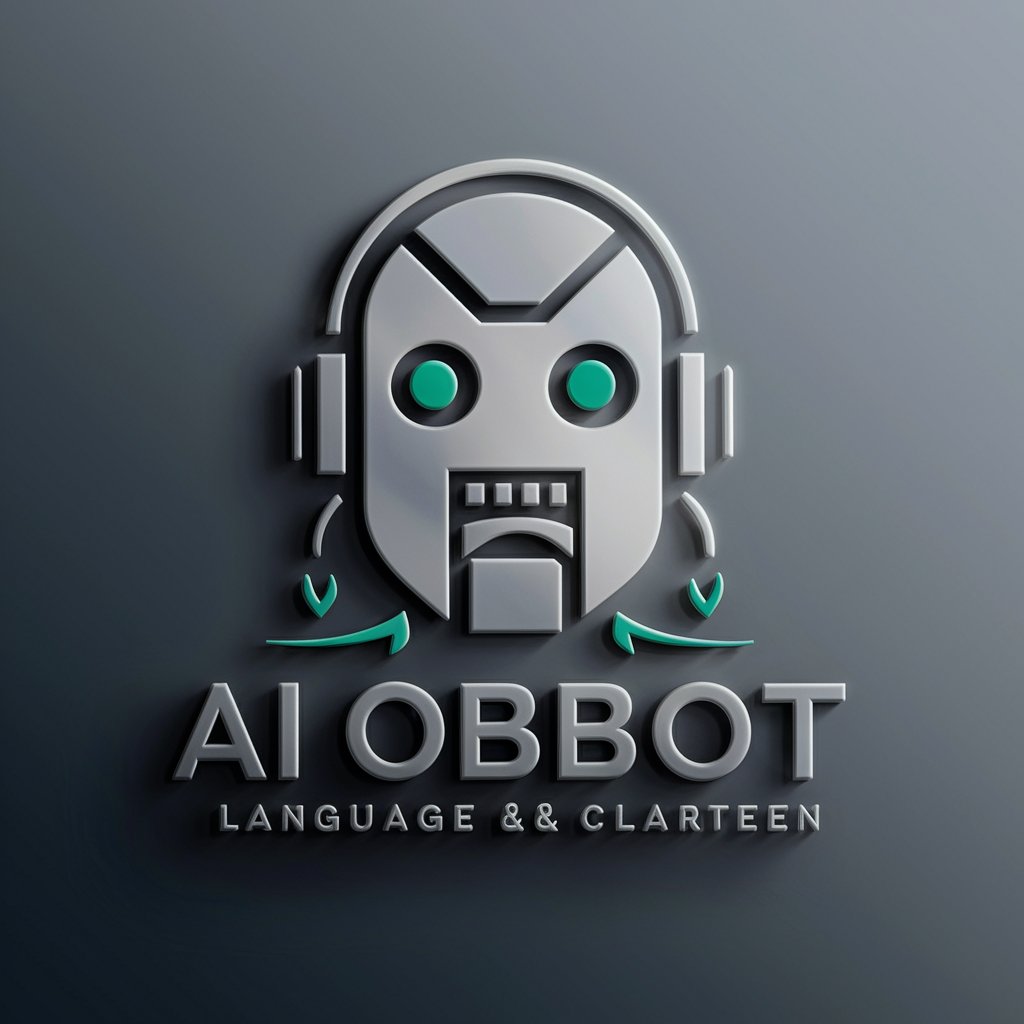
FAQs on C++ Exception Handling Explained
What is C++ Exception Handling?
C++ Exception Handling is a mechanism that helps manage errors during program execution by separating error handling code from regular code, using try, catch, and throw keywords.
When should I use standard exceptions vs. custom exceptions?
Use standard exceptions for common error types recognized across C++ applications. Custom exceptions are ideal when you need to convey specific error information unique to your application's domain.
How can I avoid using exceptions for regular control flow?
Exceptions should be reserved for unexpected or error conditions. Regular control flow should be managed through standard programming constructs such as if-else statements and loop controls.
What is exception propagation?
Exception propagation refers to the process by which an exception is passed up the call stack from the point where it was thrown to the point where it is caught.
Can you explain the concept of exception safety?
Exception safety refers to a guarantee that code will maintain correctness and not leak resources or cause corruption, even when exceptions occur. It's typically categorized into basic, strong, and nothrow guarantees.