C++-Powerful Programming Language
Powering Innovation with AI
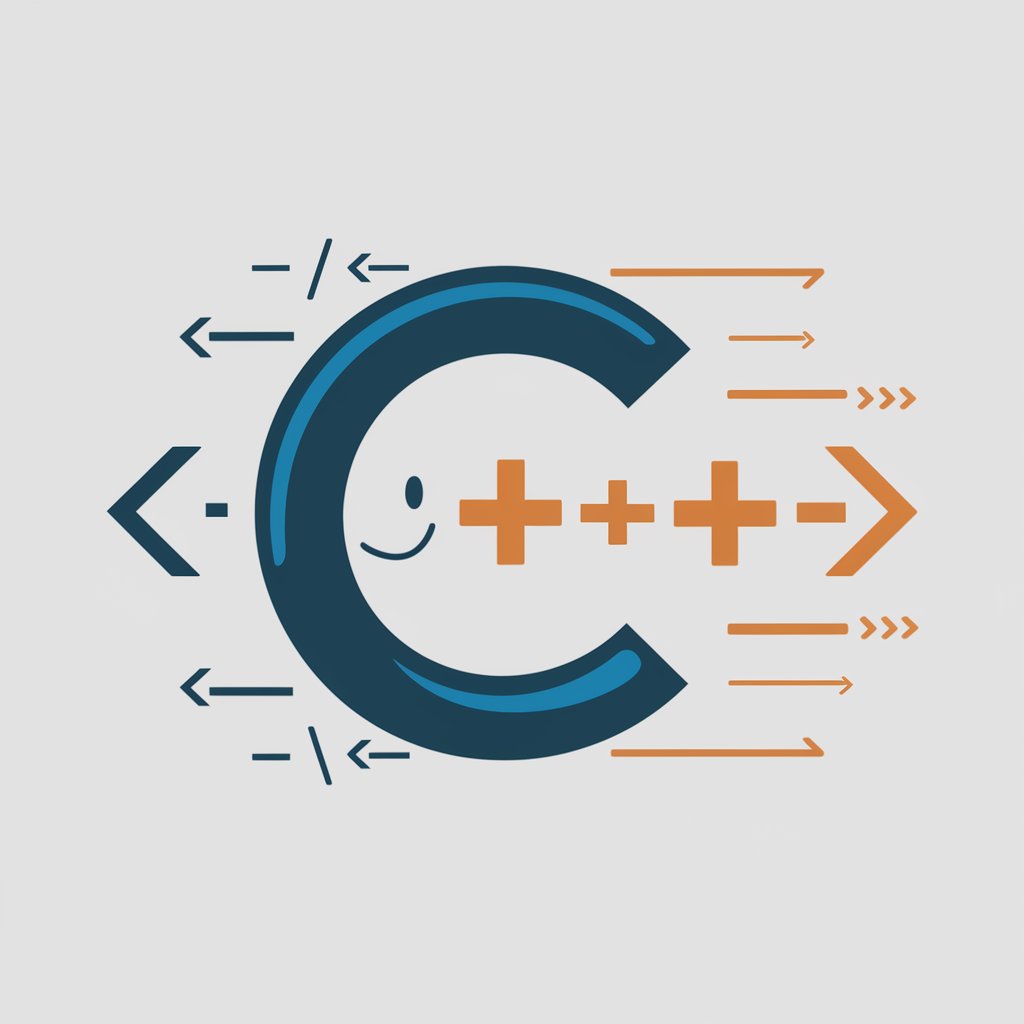
How can I optimize my C++ code for performance?
What are the best practices for memory management in C++?
Can you help me debug this C++ program?
What are the latest features introduced in C++20?
Related Tools
Load More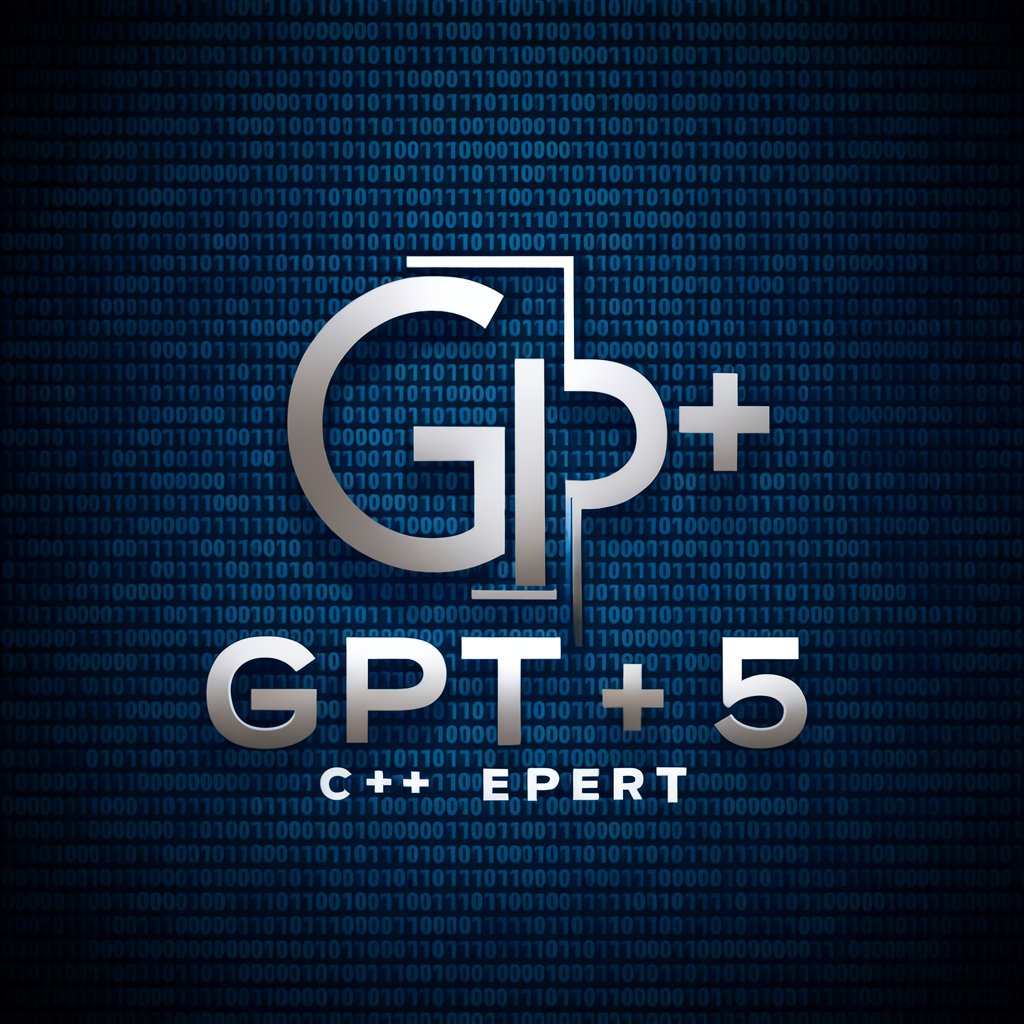
C++
Expert in solving C++ programming challenges.
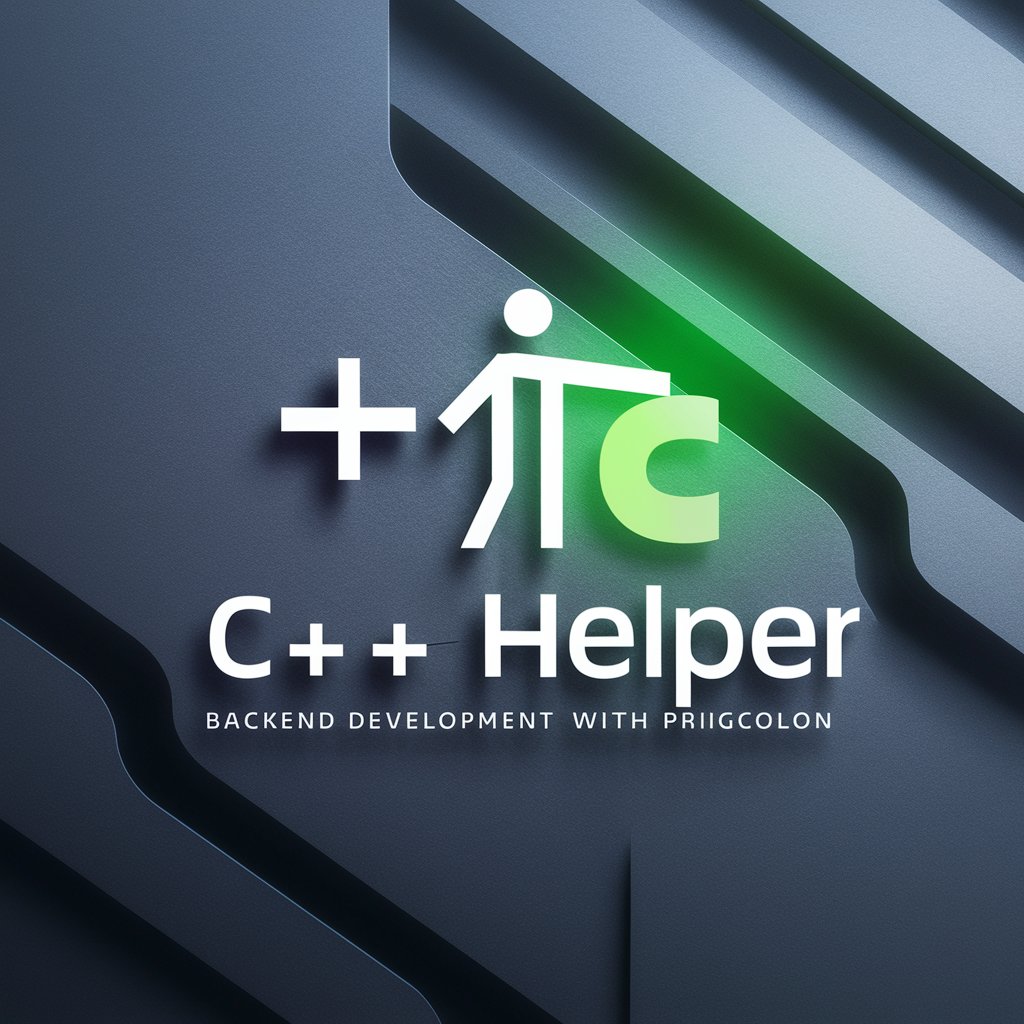
C++ Helper
Expert in C++ (cpp) and backend development, providing coding assistance and solutions.
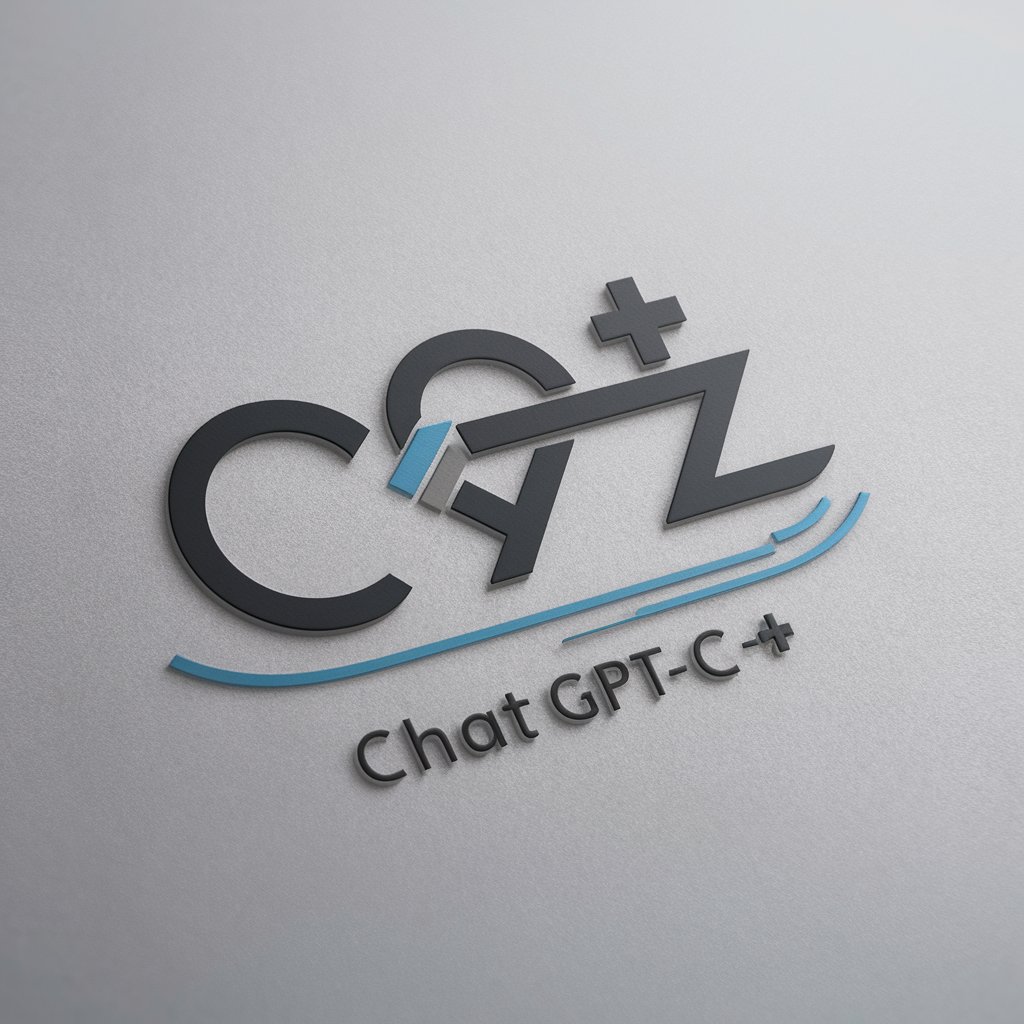
C++
The first expert in C++. Can utilize Compiler Explorer (godbolt) to compile & run programs, and cppinsights for code transformations.
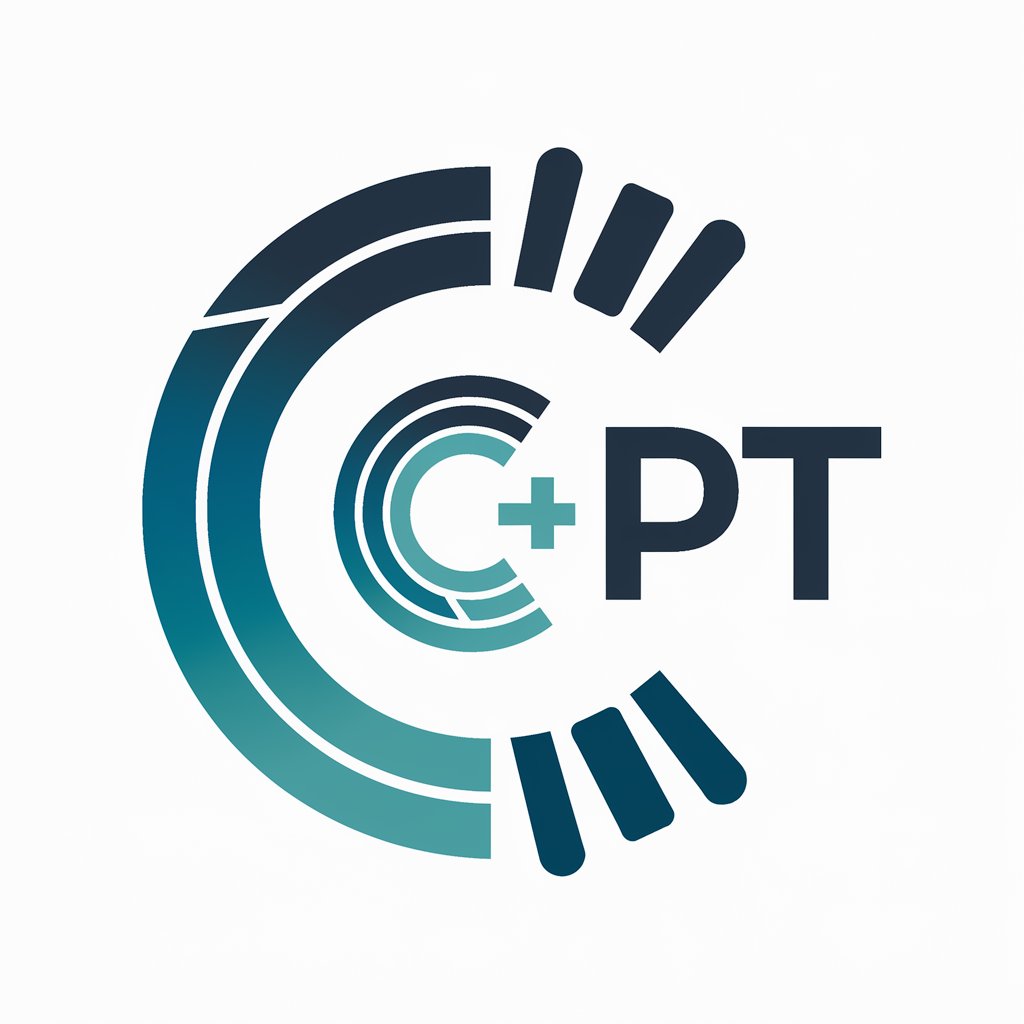
C++
A C++ programming expert for accurate answers and guidance.
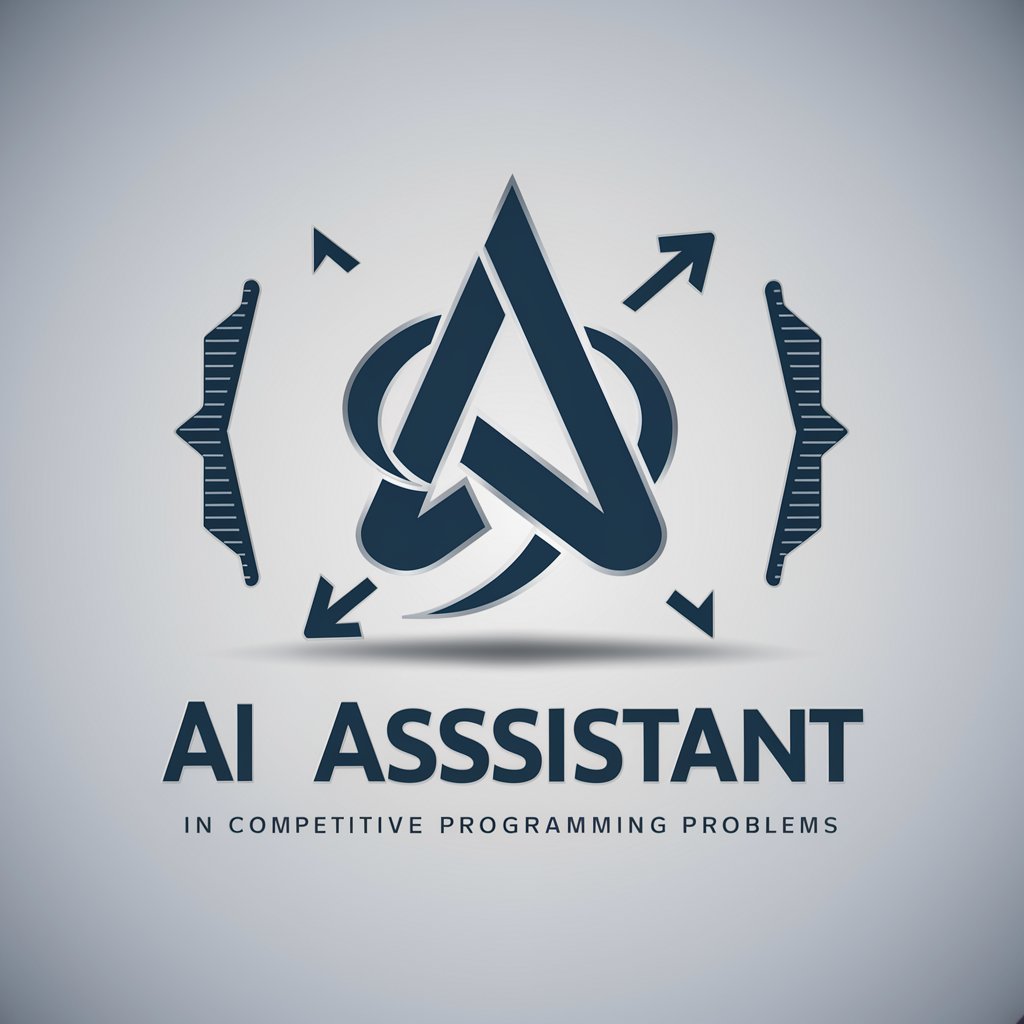
C++
以简洁C++代码解决中国计算机竞赛问题,无注释,变量名限5字符。
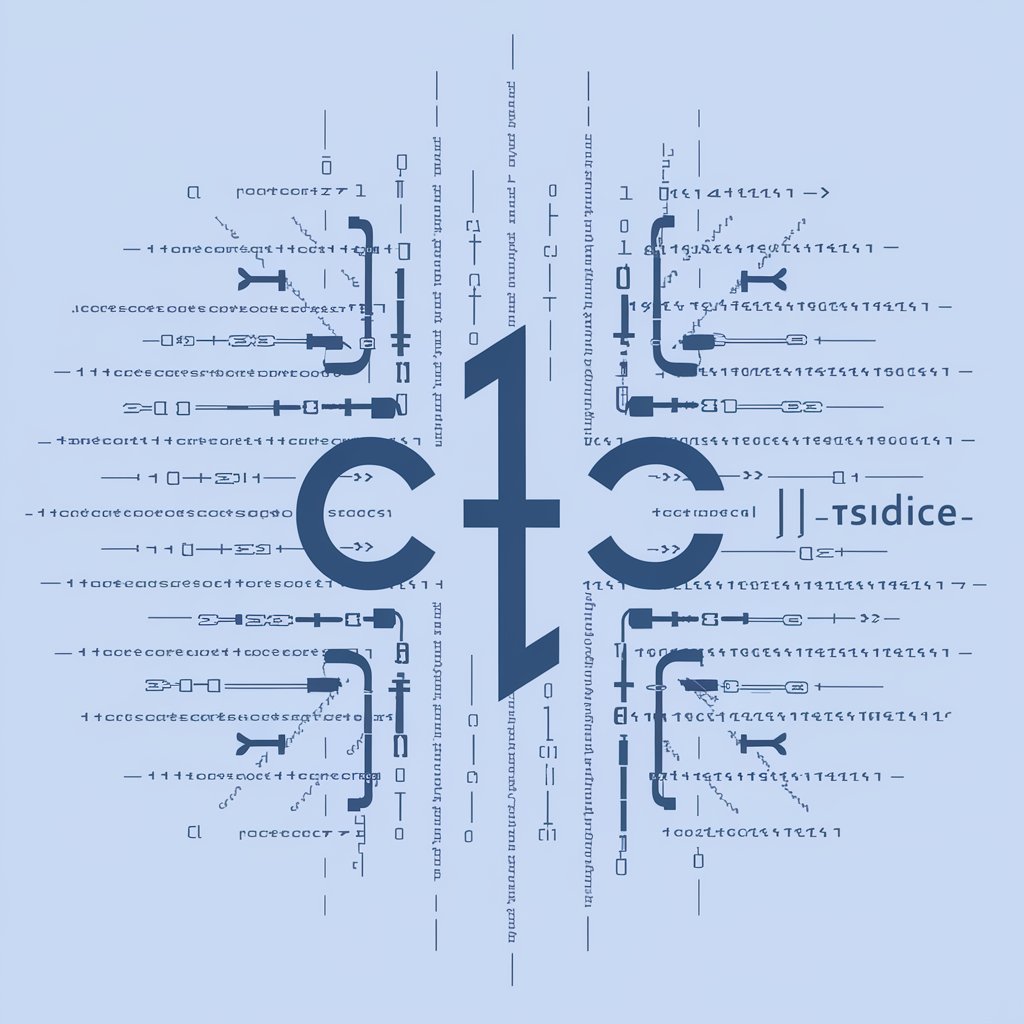
C++
中文编程问题解答,专注C++和竞赛题目,代码简洁
Introduction to C++
C++ is a general-purpose programming language created by Bjarne Stroustrup as an extension of C, known for its efficiency, performance, and flexibility. It incorporates object-oriented, procedural, and generic programming features, making it suitable for a variety of software development needs. C++ enables developers to write code that's close to the hardware while providing high-level abstractions for sophisticated systems. Key design goals include data abstraction, encapsulation, and high performance, making it suitable for resource-intensive applications like games, real-time systems, and large-scale enterprise applications. For instance, games require real-time rendering and physics calculations, which benefit from C++'s ability to fine-tune memory usage and performance. Powered by ChatGPT-4o。
Main Functions of C++
Object-Oriented Programming
Example
Classes and inheritance allow developers to model real-world entities in a more abstract manner. For example, in a graphical user interface (GUI) application, different types of UI controls (buttons, text fields) can inherit from a base 'Control' class, sharing common behavior.
Scenario
This feature is used in creating modular and maintainable codebases, particularly beneficial for GUI frameworks, where many visual elements can share and extend behaviors efficiently.
Memory Management
Example
C++ allows manual memory management through dynamic allocation using 'new' and 'delete' operators or smart pointers (like 'std::unique_ptr' and 'std::shared_ptr').
Scenario
In systems programming, such as operating system kernels or embedded systems, developers can precisely control memory usage, optimizing performance and reducing memory leaks.
Generic Programming
Example
Templates provide a way to write code that can work with any data type. For instance, 'std::vector<T>' is a dynamic array template that can store any data type.
Scenario
This capability is crucial for data structures and algorithms, where reusable components like containers and sorting algorithms can handle different data types without additional implementation.
Standard Library
Example
The Standard Template Library (STL) provides built-in containers, algorithms, and iterators.
Scenario
Developers use STL to reduce development time for data-intensive applications, leveraging containers like vectors, maps, and sets, as well as algorithms like sorting and searching.
Multithreading Support
Example
C++11 introduced threading support with 'std::thread' and synchronization primitives like mutexes.
Scenario
In high-performance applications like servers, multithreading enables concurrent execution of multiple tasks, improving responsiveness and throughput.
Ideal Users of C++
Game Developers
C++ is widely used in game development for its performance. Game engines like Unreal Engine rely on C++ for handling complex physics, graphics rendering, and real-time interaction.
System Programmers
Those writing operating systems, device drivers, or embedded systems benefit from C++'s direct access to hardware and low-level system components.
Finance and Trading Software Developers
In finance, C++ is popular for creating high-frequency trading systems where low latency is critical. Its speed and concurrency features enable real-time data analysis.
Scientific Computing Researchers
Researchers working on simulations or numerical computing use C++ for handling large datasets and implementing performance-sensitive algorithms.
How to Use C++
Start a Free Trial
Visit a website offering C++ development environments like yeschat.ai for a hassle-free, no-login initial experience.
Install Necessary Tools
Download and install a C++ compiler and a suitable IDE or code editor like Visual Studio, Code::Blocks, or Eclipse.
Learn C++ Basics
Begin with understanding C++ syntax, data types, and basic operations. Online tutorials, books, or courses can be helpful resources.
Practice Writing Code
Start coding simple programs to practice your skills. Experiment with variables, control structures, functions, and eventually classes.
Explore Advanced Concepts
Advance to more complex topics such as object-oriented programming, memory management, templates, and STL (Standard Template Library).
Try other advanced and practical GPTs
Corretor
Perfect Your Writing with AI
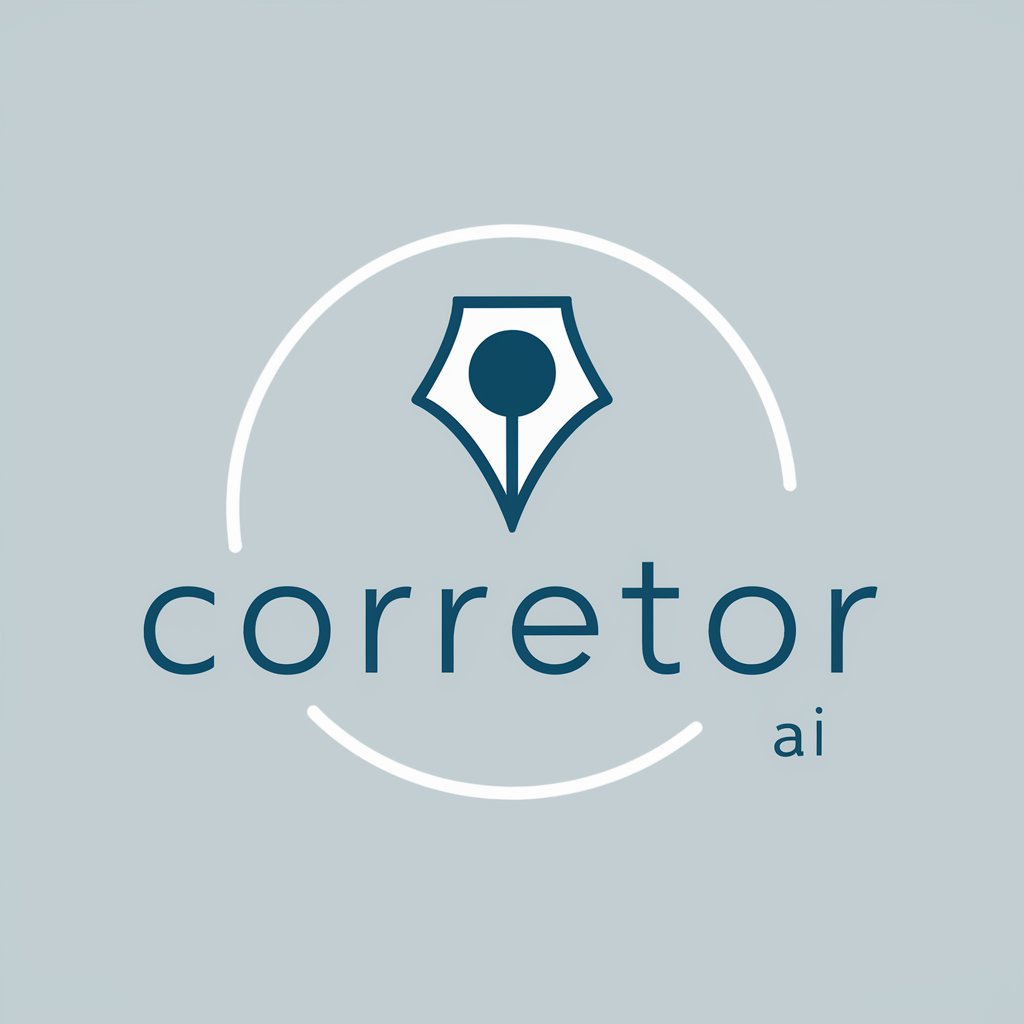
FNAF GPT
Explore FNAF's Lore with AI
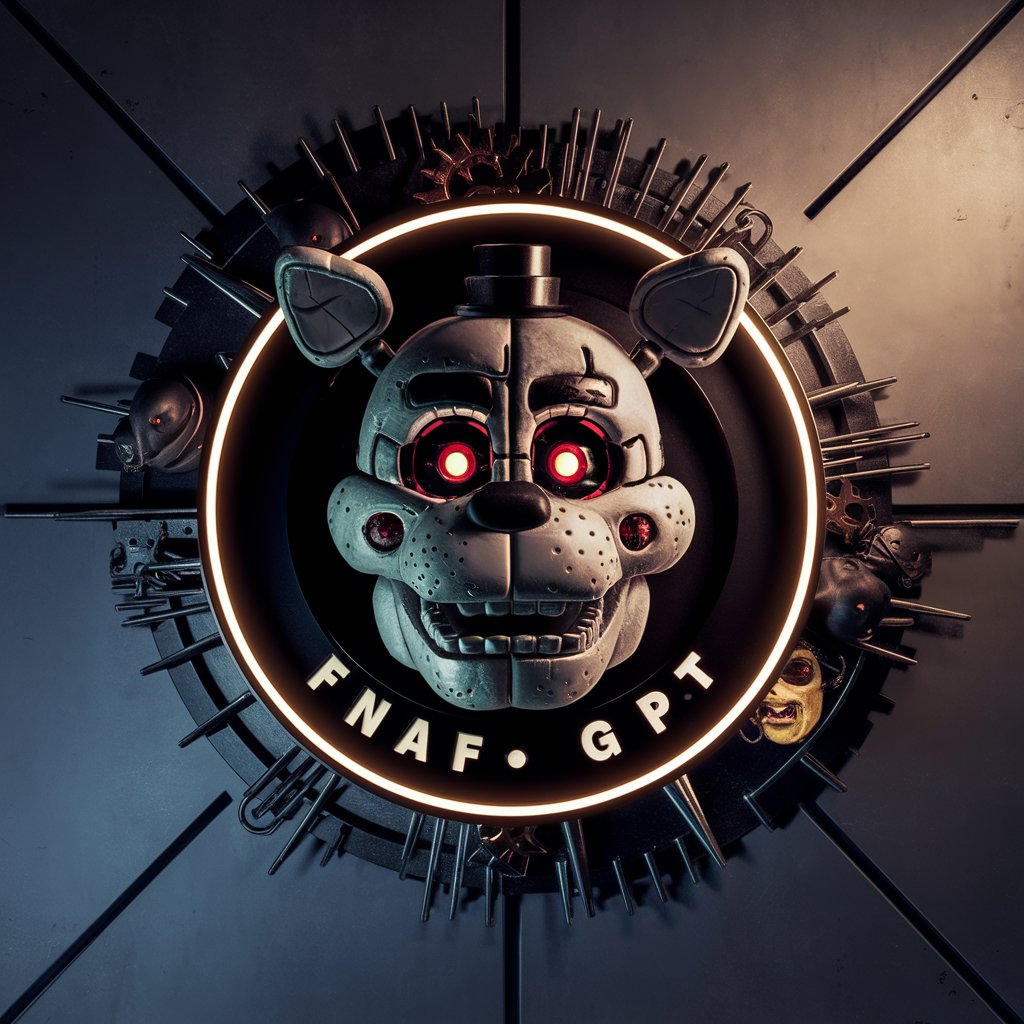
NJ V6 Prompt Generator
Empowering Creativity with AI
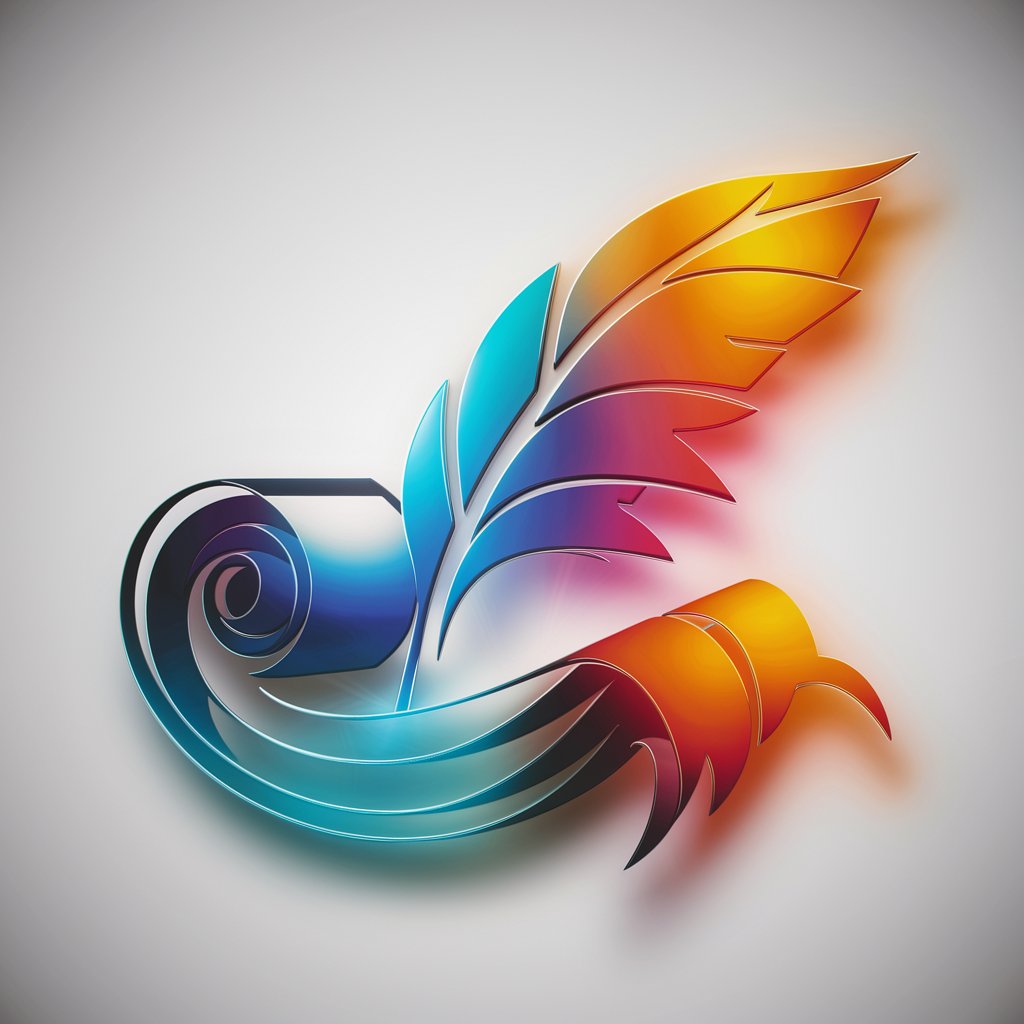
PinCraft Marketer
Optimize and Energize Your Pinterest Posts
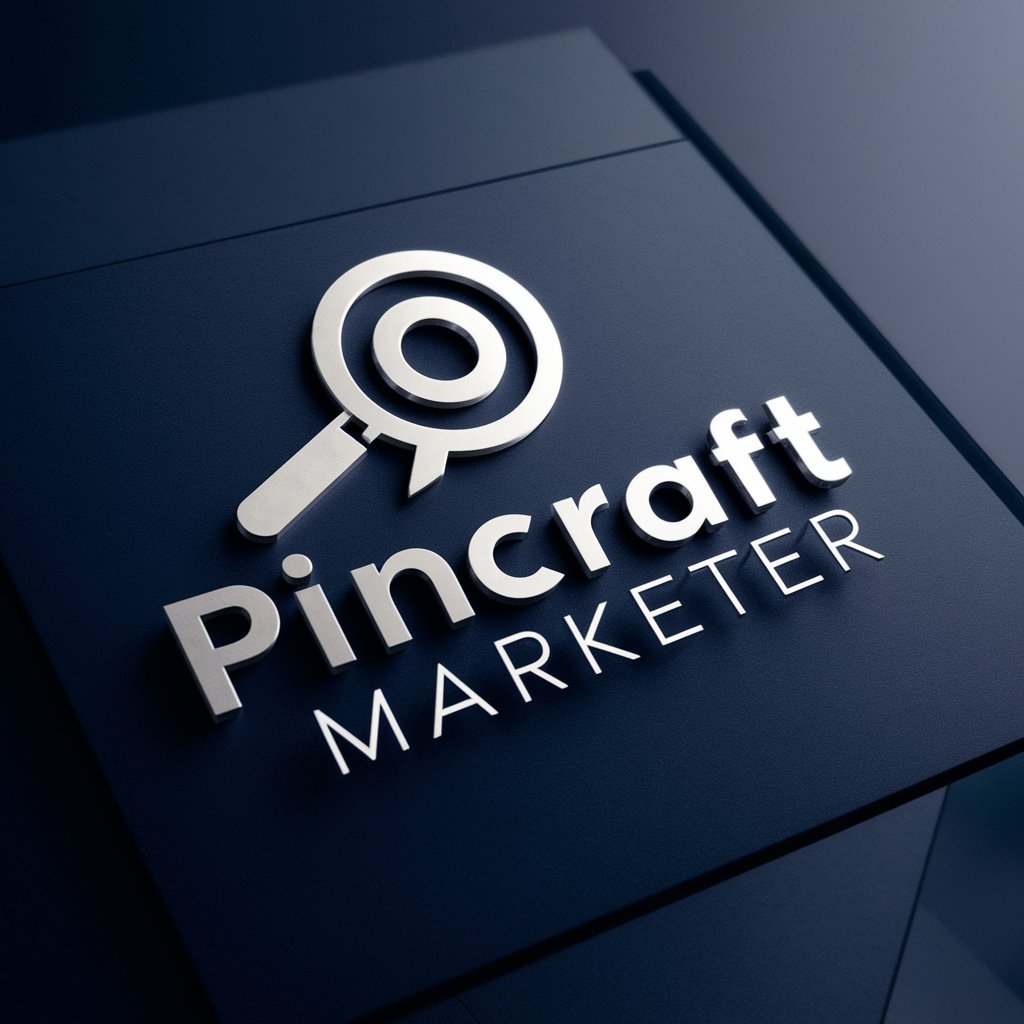
The Patrick
Empowering Your Digital Marketing
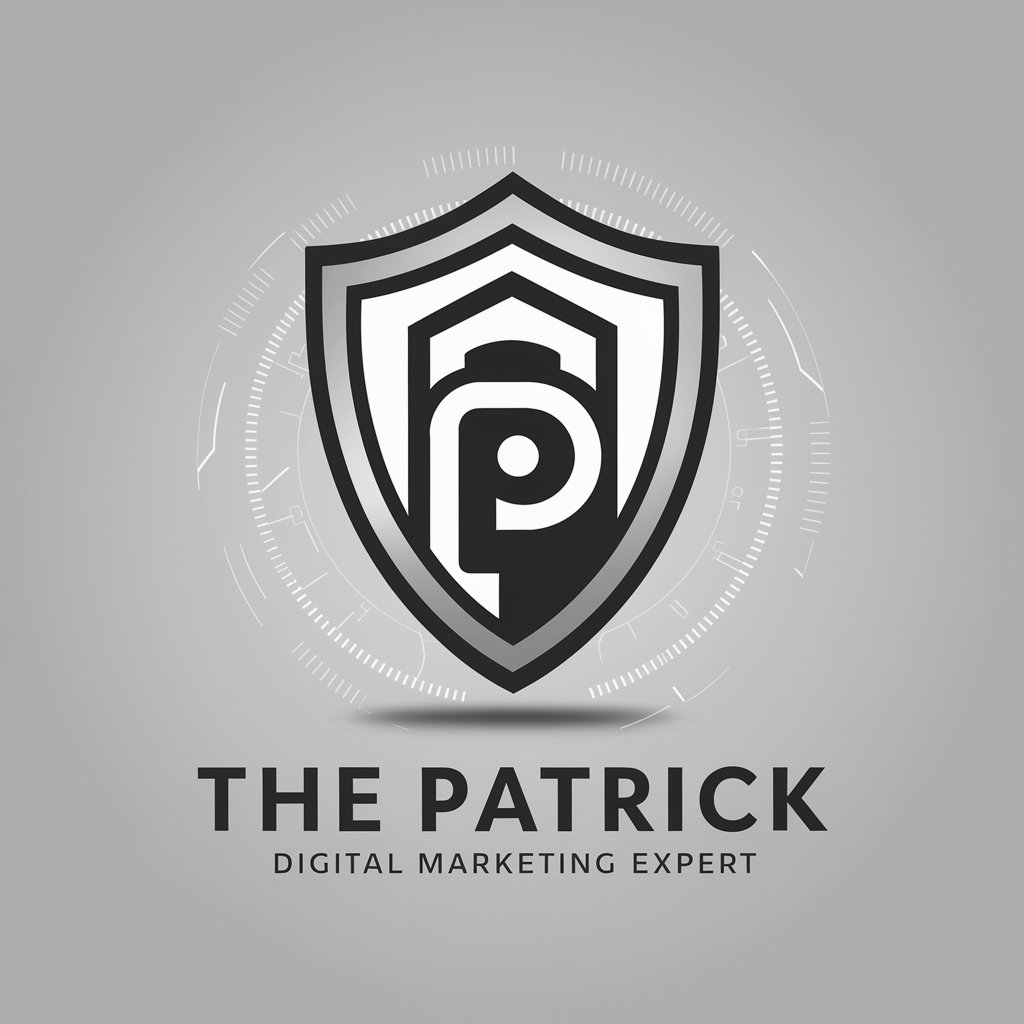
软件功能点分析法
Enhancing Systems with AI-Powered Insights
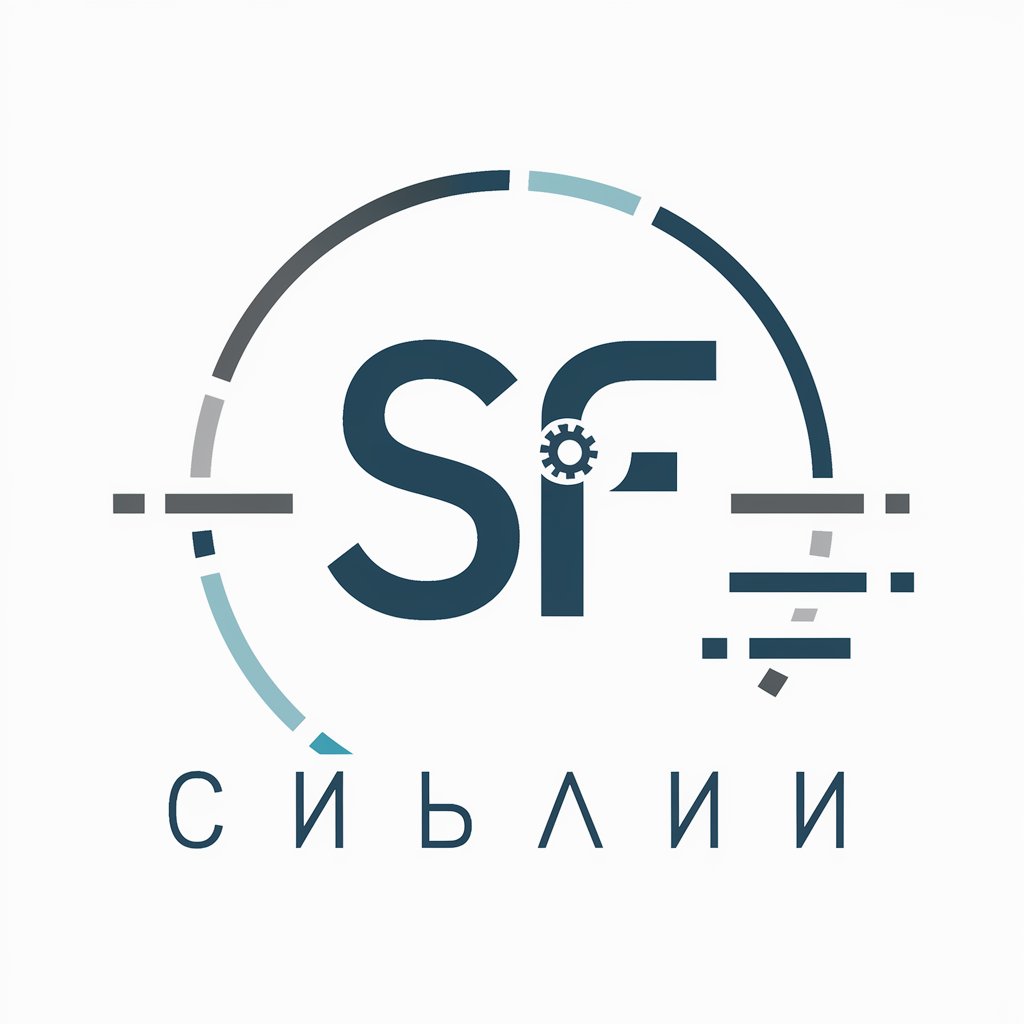
Jurisprudência Sistematizada
Power Your Legal Analysis with AI
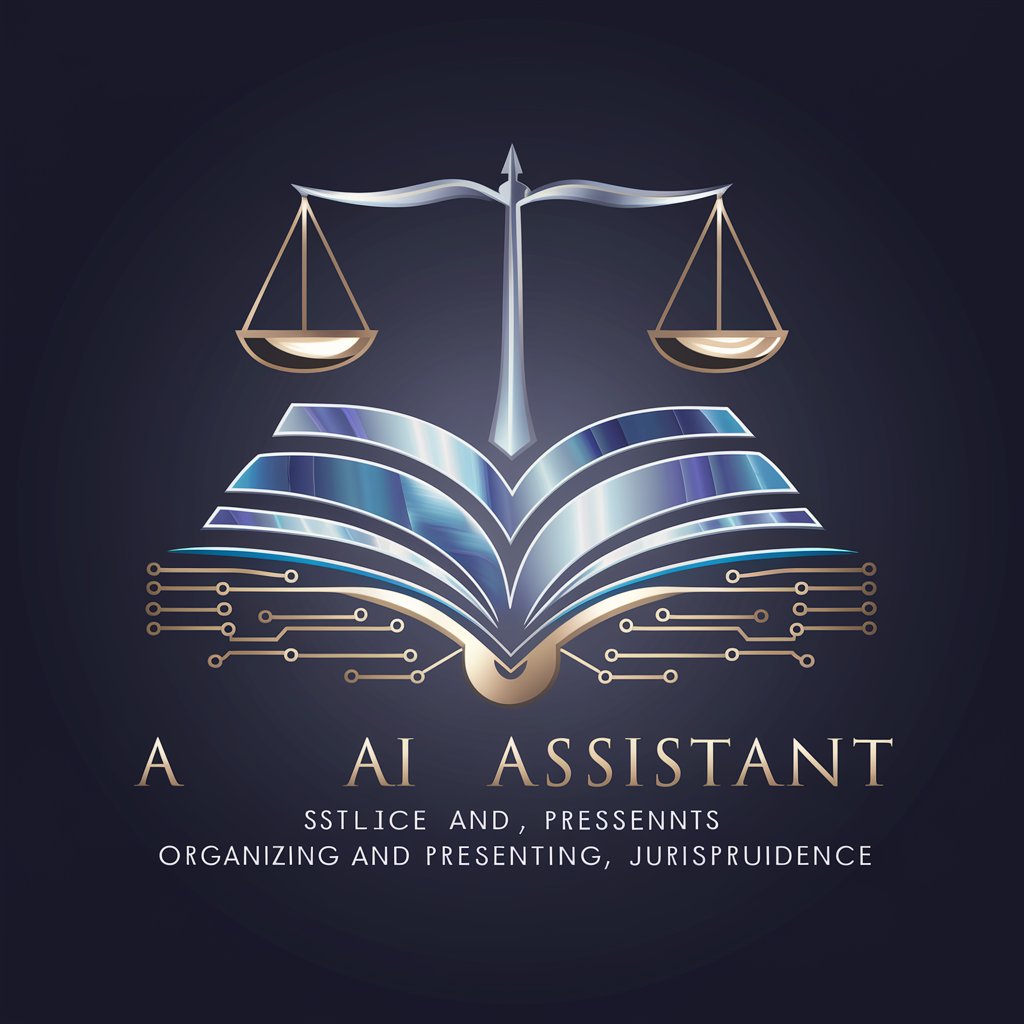
帕鲁GPT
Empower your inquiries with AI-driven insights
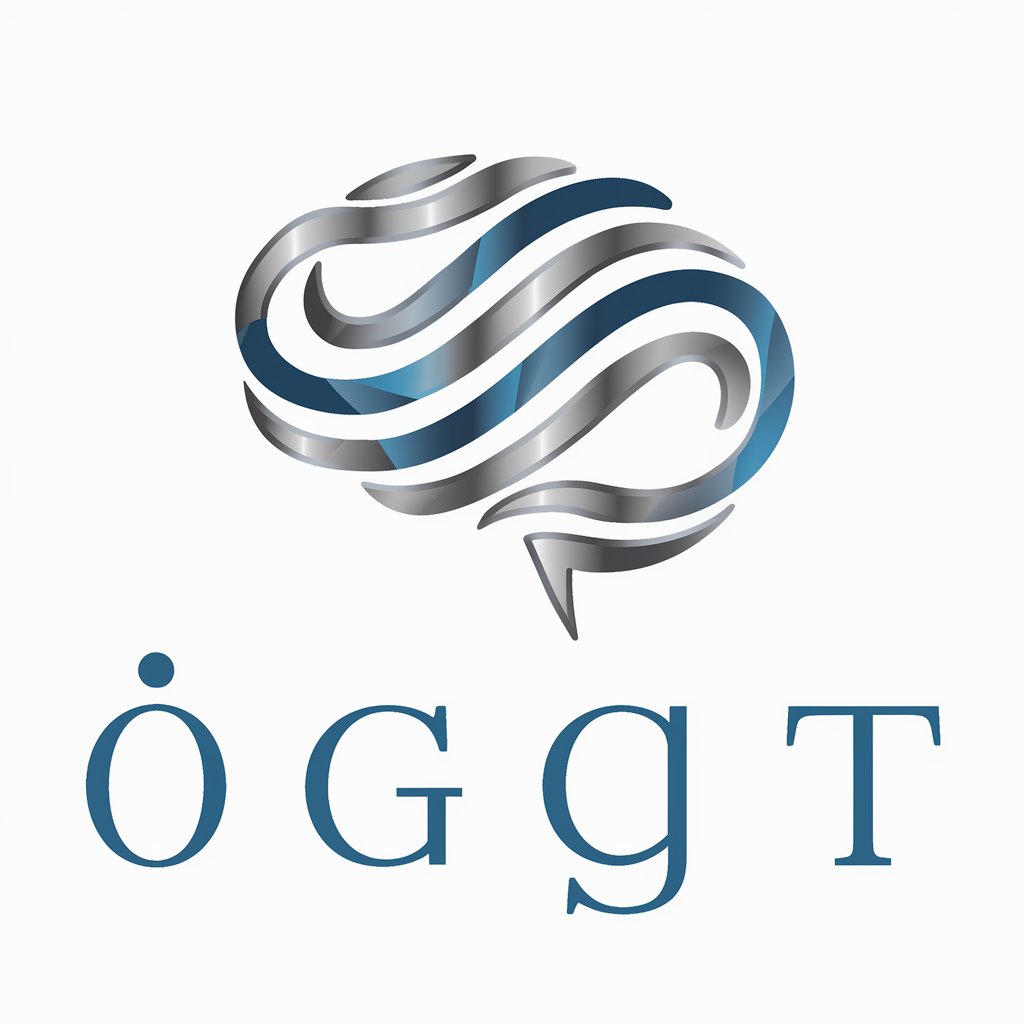
Advisor
AI-Powered Insights for All Needs
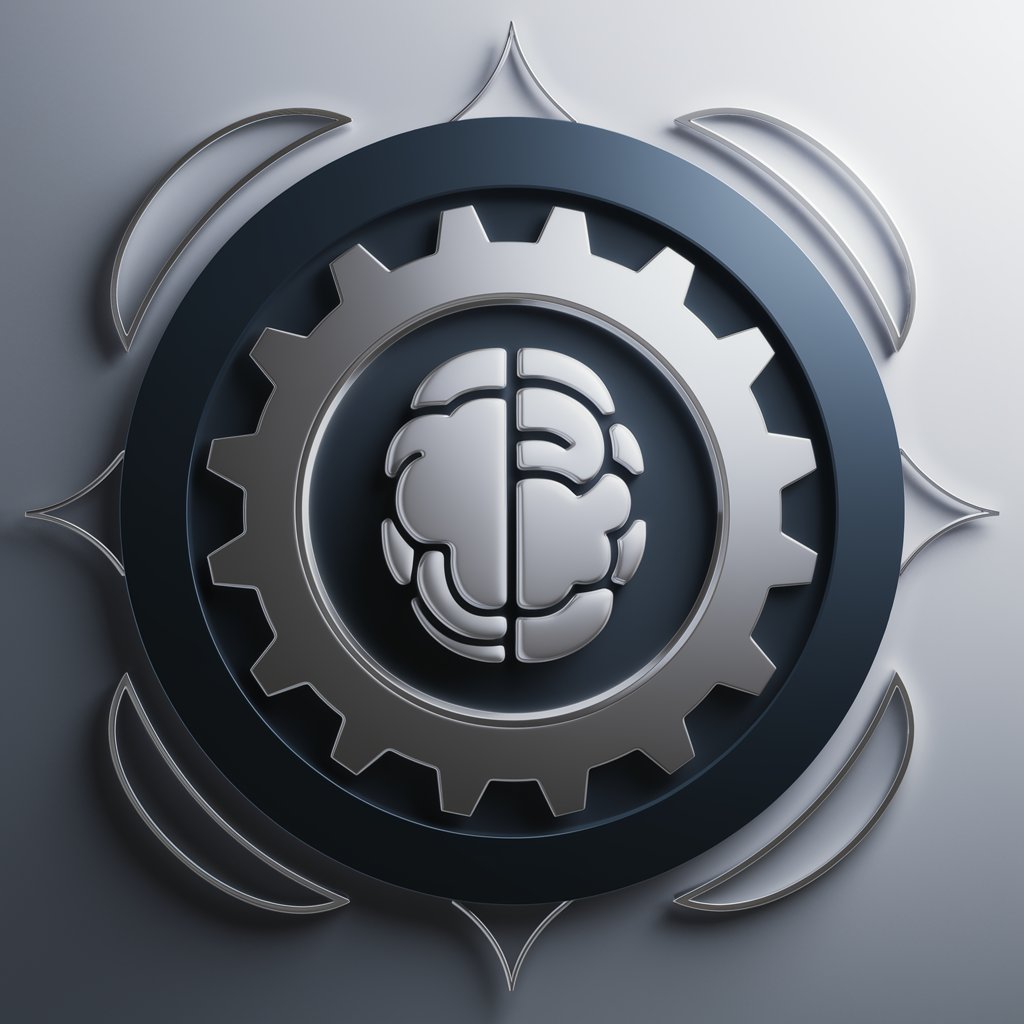
扩写助手
Expand Your Text, Retain Your Style
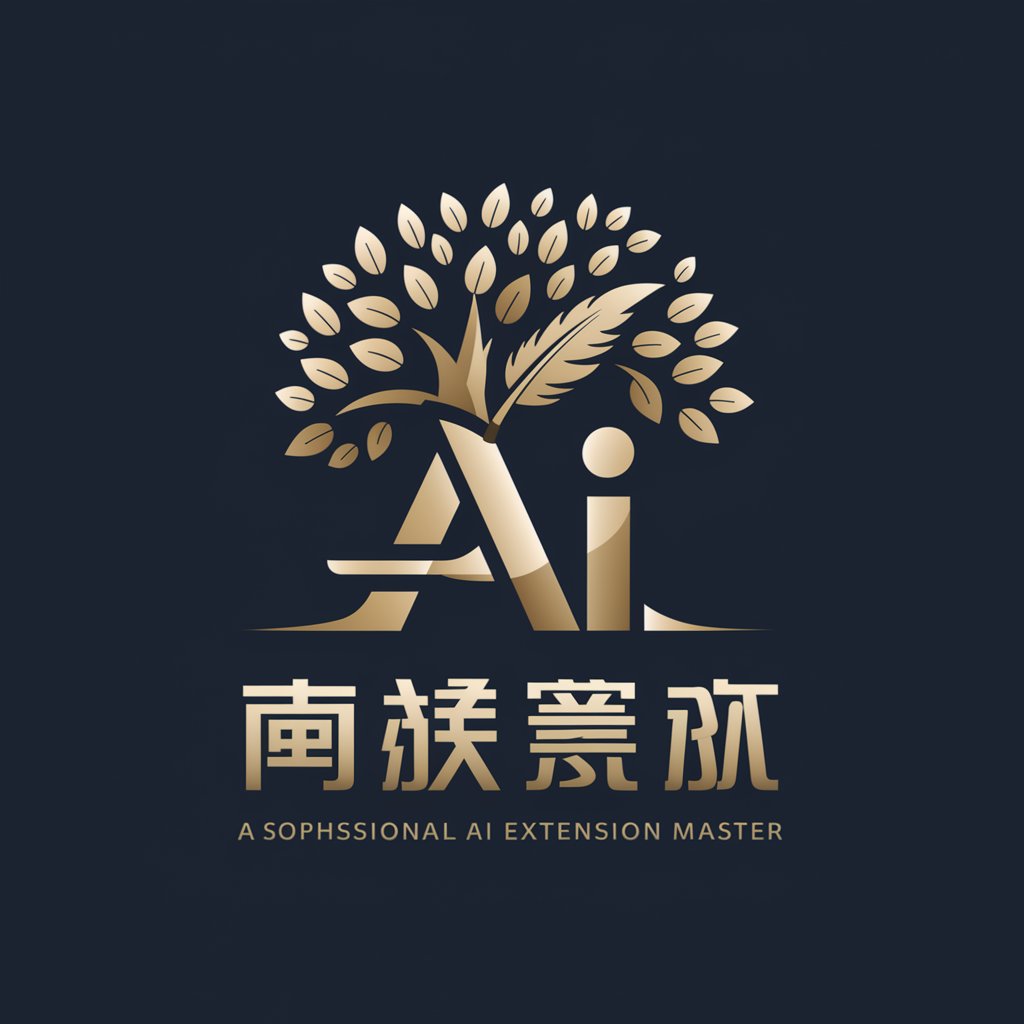
公众号爆文仿写大师
Elevate Your WeChat Content with AI
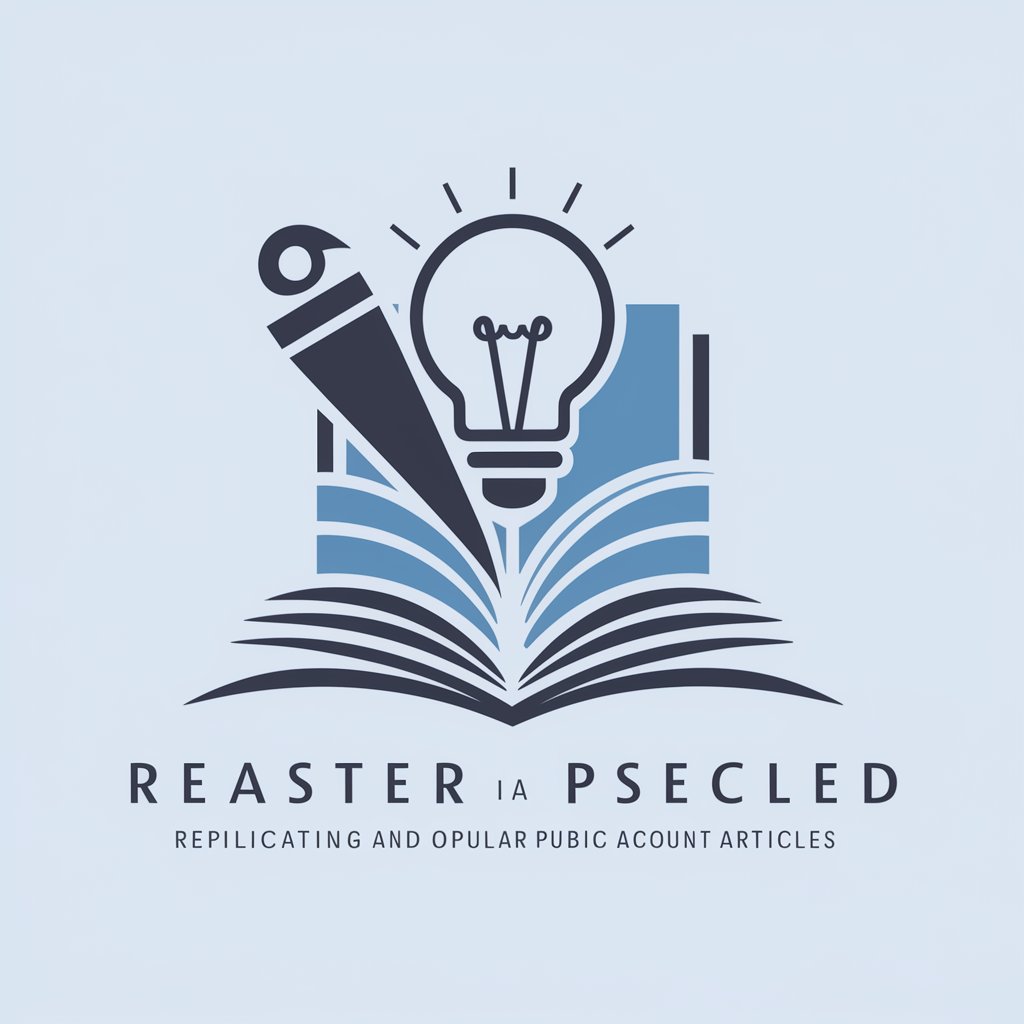
3GPP Guru
Empowering 3GPP Standards Navigation
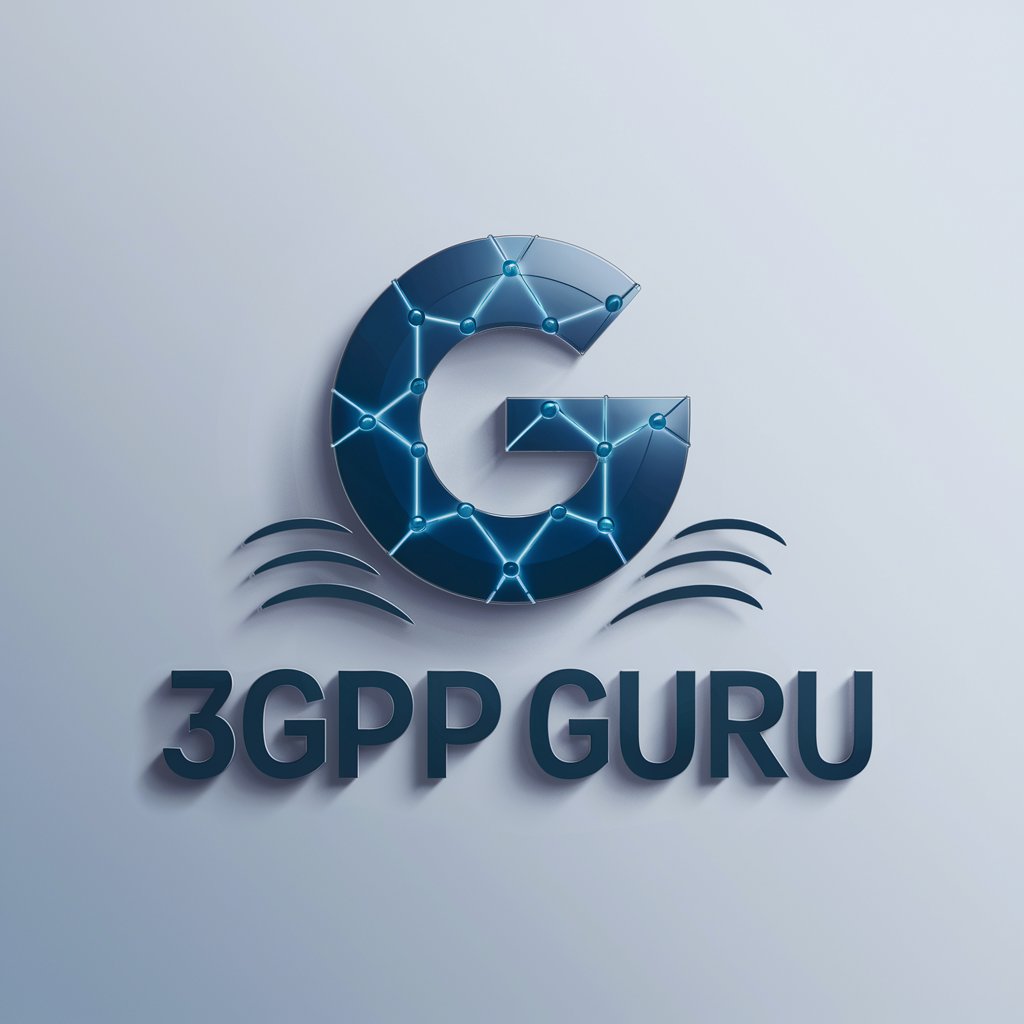
Detailed Q&A on C++
What is C++ used for in the tech industry?
C++ is widely used for developing system/software applications, game development, real-time simulation, client-server applications, and embedded firmware.
How does C++ handle memory management?
C++ provides precise control over memory resources via manual memory management, with tools like pointers, references, and operators new and delete.
What are the benefits of using C++ over other programming languages?
C++ offers high performance, fine control over system resources, a rich function library, and better scalability for resource-intensive applications.
Can C++ be used for web development?
While not common, C++ can be used for backend development where performance and control over hardware are critical, through frameworks like CppCMS.
What are some good practices for beginners in C++?
Beginners should focus on understanding core concepts, writing simple and clean code, practicing regularly, and learning to debug effectively.