three.js - Powerful 3D Web Graphics

Hello, how can I assist you with three.js today?
Revolutionizing Visualization with AI-Powered 3D Graphics
How do I create a rotating cube in three.js?
Help me install and setup three.js
Create a cyberpunk city with random buildings
How to add lighting in a three.js project?
Get Embed Code
Introduction to Three.js
Three.js is a high-level JavaScript library that provides an easy-to-use API to create and display animated 3D graphics in a web browser. It acts as a wrapper around lower-level WebGL (Web Graphics Library) functionality, making it accessible without requiring in-depth knowledge of 3D mathematics or WebGL. The primary purpose of Three.js is to simplify the process of creating rich and interactive 3D experiences on the web. Typical applications include web-based games, data visualization, interactive animations, and virtual reality experiences. For example, you can easily create a rotating 3D cube with customized textures, or an interactive 3D model viewer for product showcases. Powered by ChatGPT-4o。
Key Functions of Three.js
3D Scene Creation
Example
const scene = new THREE.Scene();
Scenario
Used for setting up a 3D environment where objects, lights, and cameras are placed.
Mesh Generation
Example
const geometry = new THREE.BoxGeometry(); const material = new THREE.MeshBasicMaterial({color: 0x00ff00}); const cube = new THREE.Mesh(geometry, material);
Scenario
Allows the creation of 3D objects with various shapes and materials. Common in product visualizations and gaming.
Lighting
Example
const light = new THREE.PointLight(0xffffff, 1, 100); light.position.set(50, 50, 50); scene.add(light);
Scenario
Adds lights to the scene, impacting the way materials and surfaces appear. Essential for creating realistic environments and enhancing visual effects.
Animation
Example
function animate() { requestAnimationFrame(animate); cube.rotation.x += 0.01; renderer.render(scene, camera); }
Scenario
For creating dynamic scenes with moving or interactive elements, widely used in games and interactive educational content.
Camera Controls
Example
const camera = new THREE.PerspectiveCamera(75, window.innerWidth/window.innerHeight, 0.1, 1000);
Scenario
Defines the perspective from which the scene is viewed. Used in virtual tours, architectural visualizations, and interactive storytelling.
Ideal Users of Three.js
Web Developers
Web developers use Three.js to create interactive 3D web applications, enhancing user experience and engagement. It's particularly beneficial for those looking to add a visually rich interface to their website without deep knowledge of 3D graphics programming.
Game Developers
Game developers leverage Three.js for creating browser-based 3D games. The library provides a more accessible platform for indie developers or those aiming for simple but visually appealing web games.
Artists and Designers
Artists and designers use Three.js for digital art installations and interactive graphics. It offers a creative platform for experimenting with 3D designs and animations directly in the browser.
Educators and Researchers
Educators and researchers find Three.js useful for creating educational content and data visualizations. It helps in simplifying complex concepts through interactive 3D models, making learning more engaging.
Guidelines for Using Three.js
1
Visit yeschat.ai for a free trial without login, also no need for ChatGPT Plus.
2
Install three.js library via npm using 'npm install three' or include it directly in your HTML using a CDN link.
3
Set up your project environment by creating a new HTML file and include three.js via a script tag or import it in your JavaScript file.
4
Start by creating a basic scene, adding a camera, and rendering using WebGLRenderer. Experiment with different geometries, materials, and lights.
5
Explore advanced features like animations, controls, and loaders for complex projects. Utilize the Three.js documentation and community for support.
Try other advanced and practical GPTs
Chinese Grammar Wiki Tutor
AI-powered Mandarin Grammar Assistant
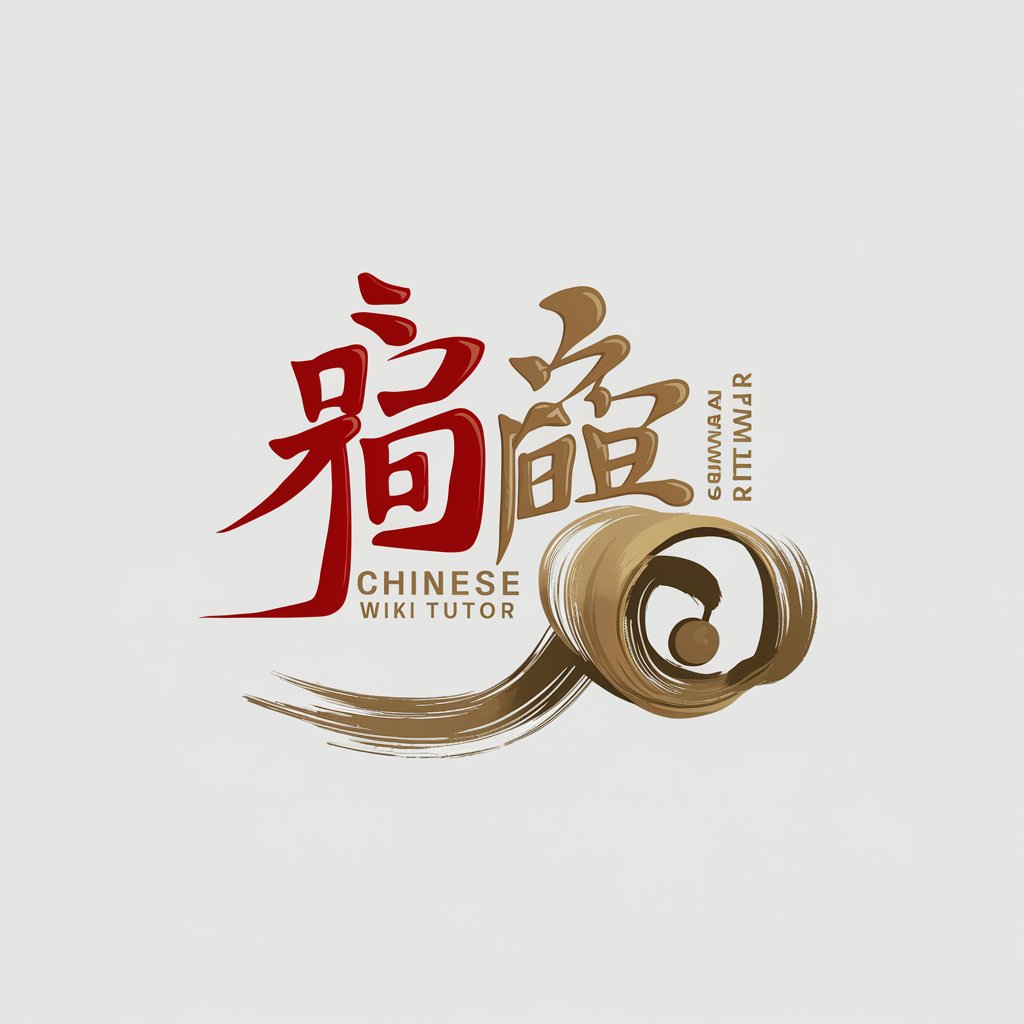
Real Estate Listing Writer [GPT-4.5 Unofficial]
AI-Powered Real Estate Listings Made Easy
![Real Estate Listing Writer [GPT-4.5 Unofficial]](https://files.oaiusercontent.com/file-srIYQF0ltrdNcHbDhKmD8LWg?se=2023-11-18T23%3A56%3A34Z&sp=r&sv=2021-08-06&sr=b&rscc=max-age%3D3599%2C%20immutable&rscd=attachment%3B%20filename%3DIMG_6318.jpg&sig=c1Pq5jtoXRnhgPr6aAZQ511%2BSojlOlSW4WzcLJH3Nm4%3D)
Kenya Constitution Explorer
Navigating Kenyan Law with AI
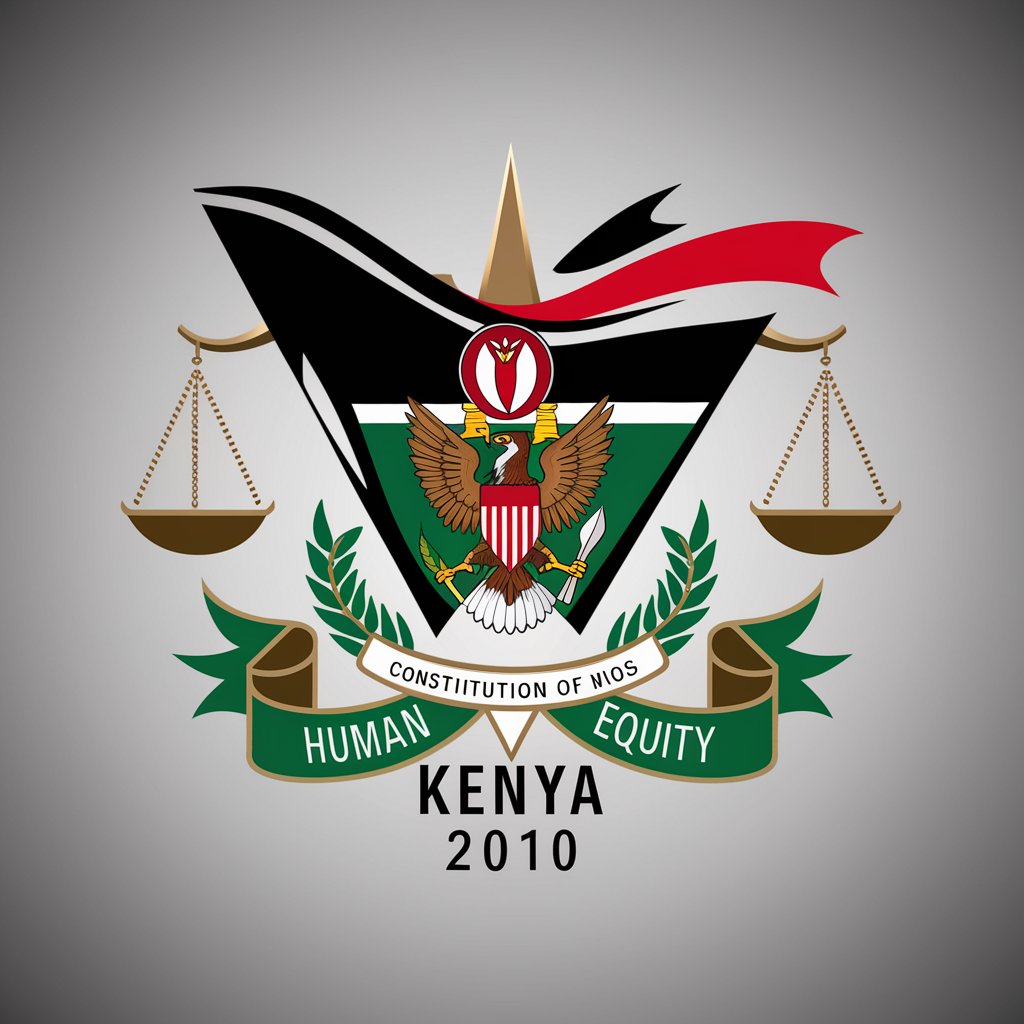
PDF Translator GPT
Effortless, AI-Powered Document Translation
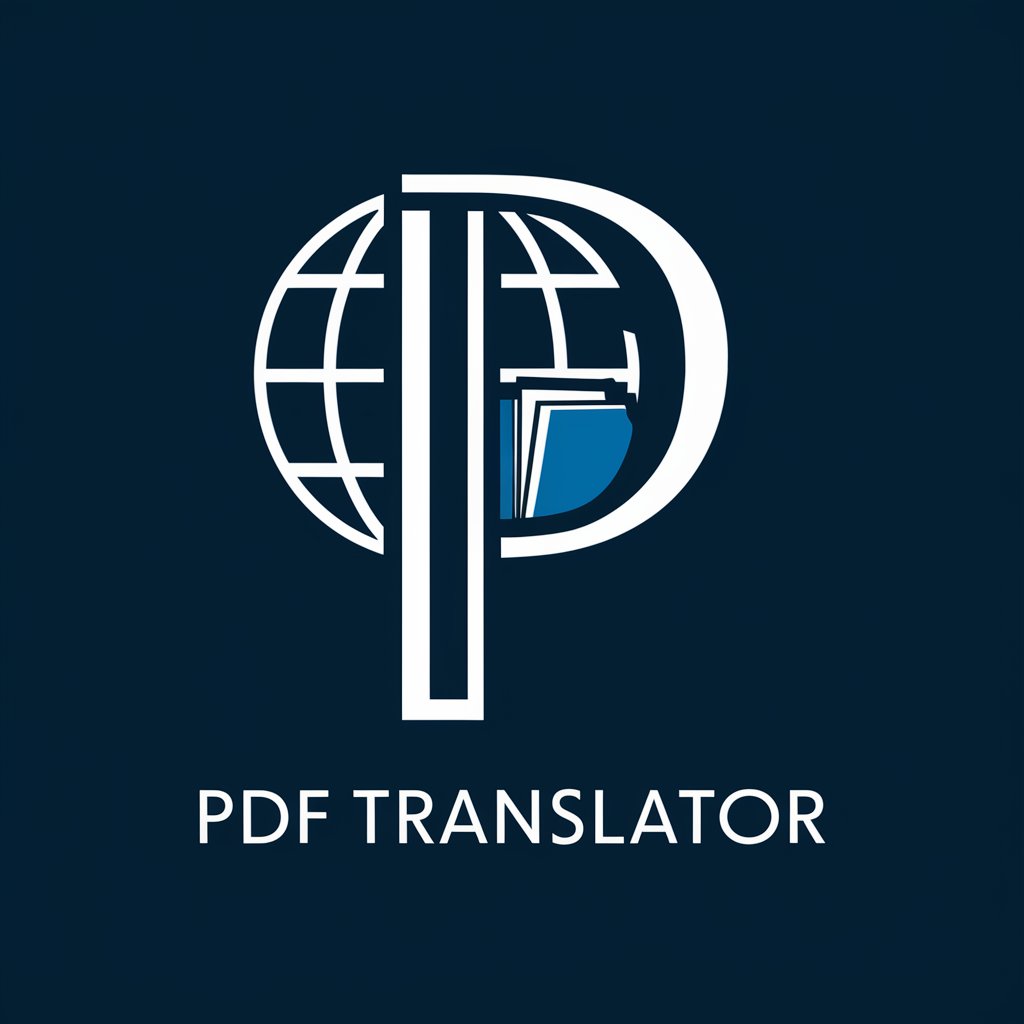
Learn Bubble.io
Master Bubble.io with AI-Powered Guidance
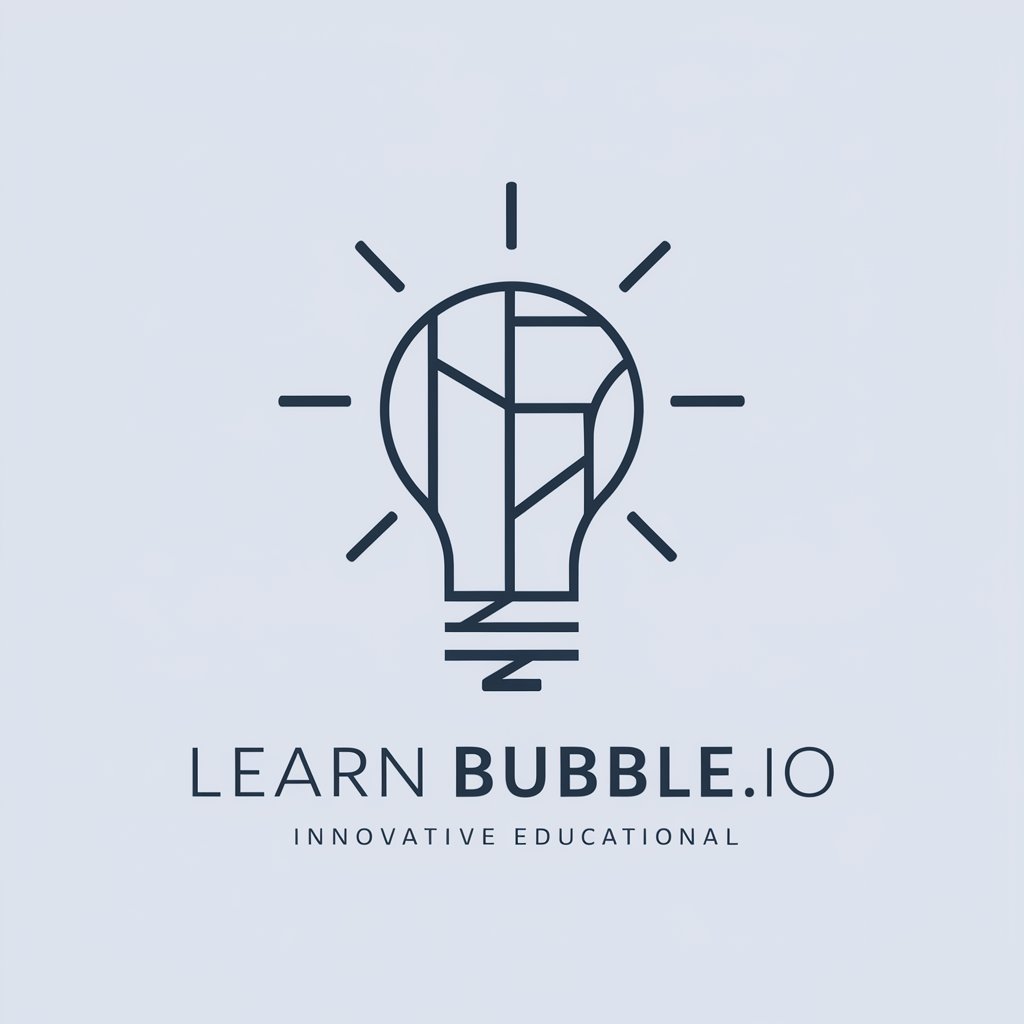
Anime Art Creator
Bringing Anime Visions to Life with AI
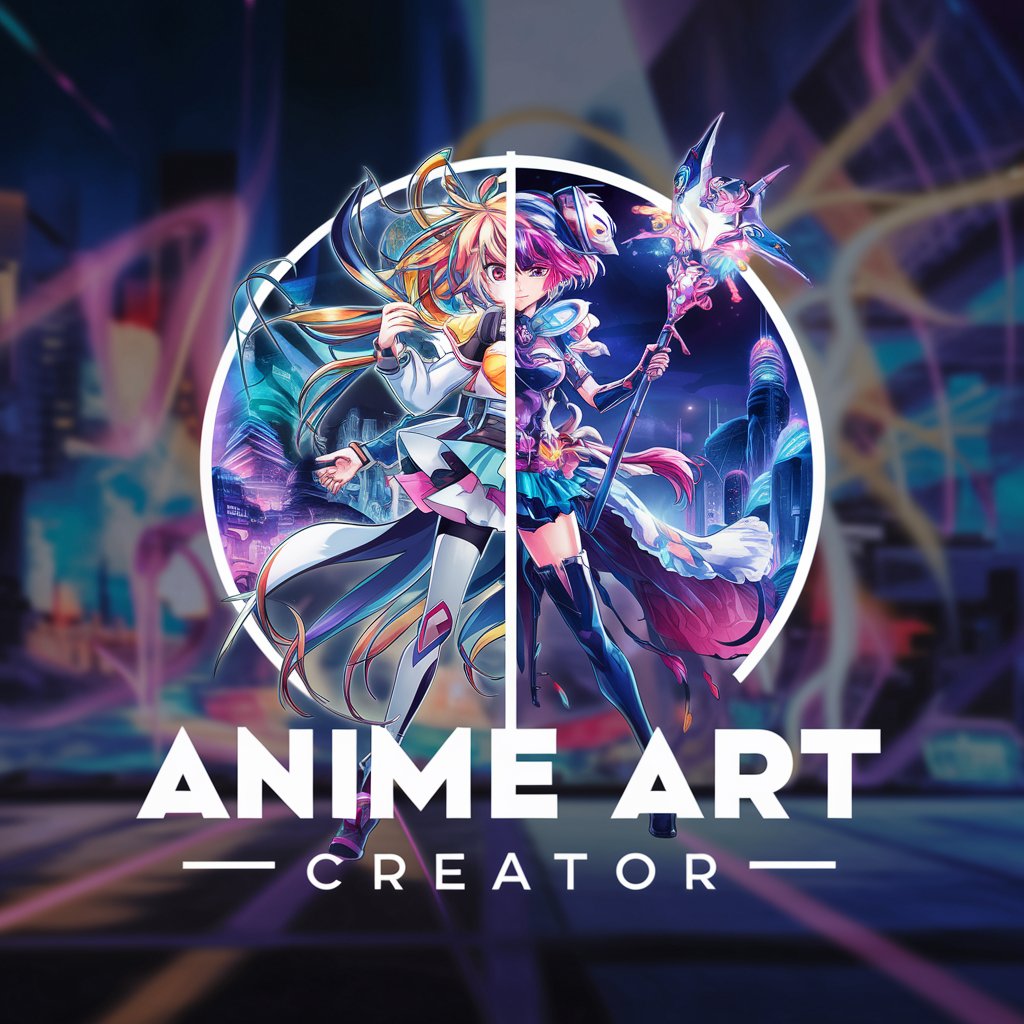
Financial Stress? - Get Tailored Advice
AI-powered Tailored Financial Guidance
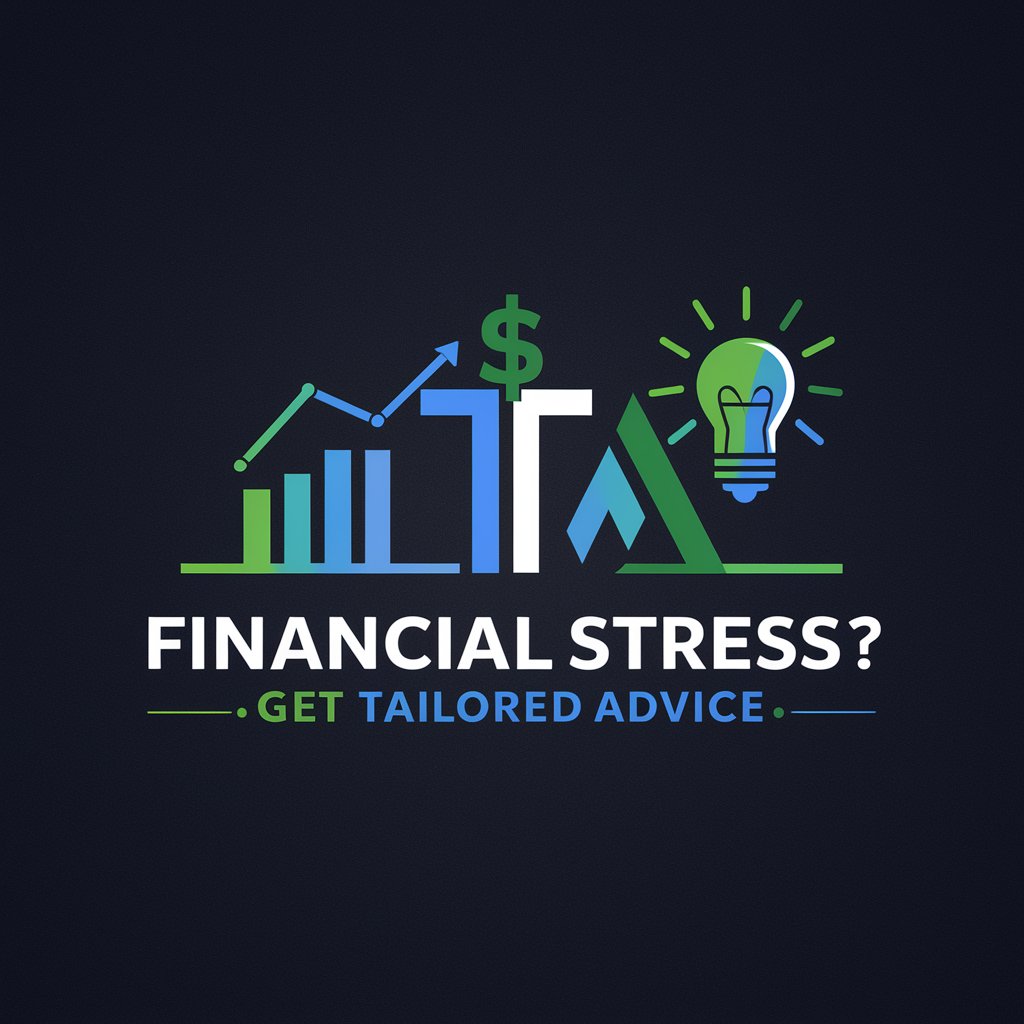
AstroJS Tips
Empowering Your AstroJS Journey with AI
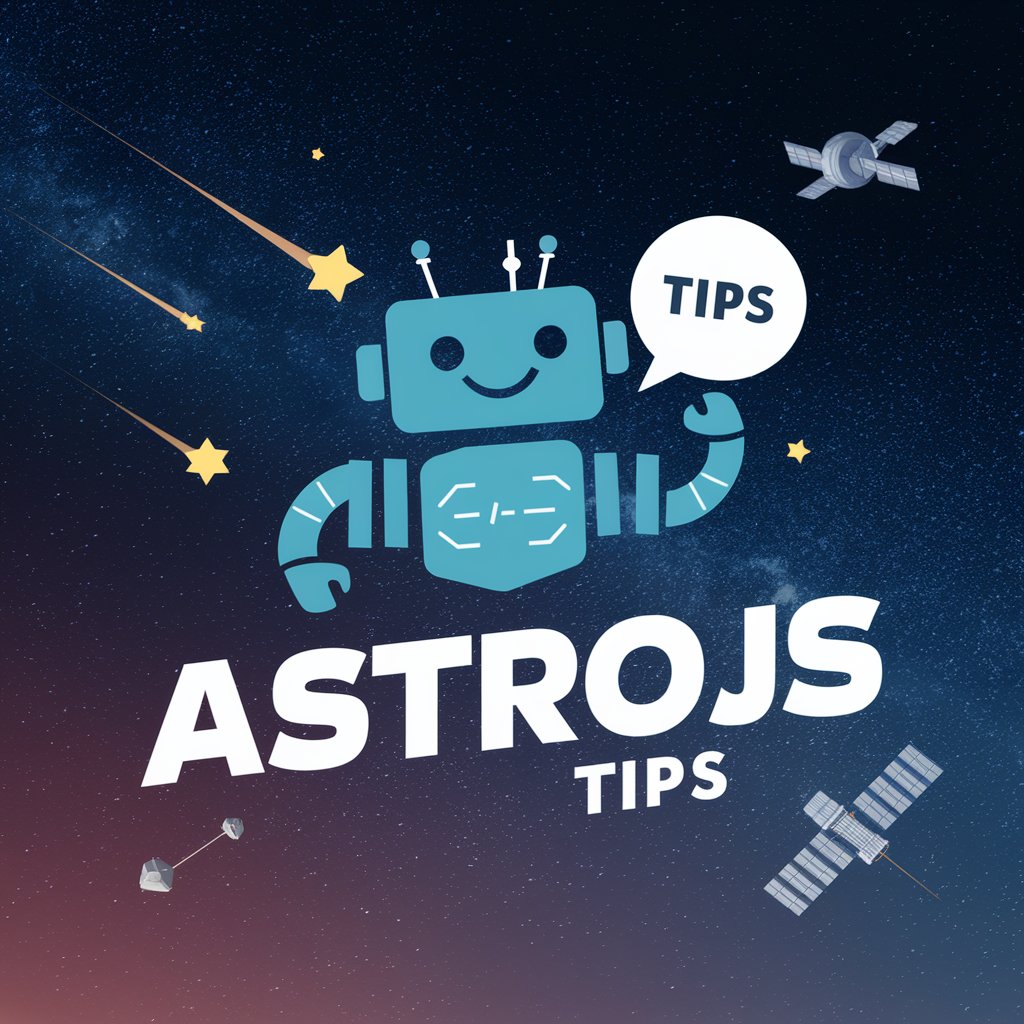
GeniePT Prompt Enhancer
Elevating Queries with AI Precision
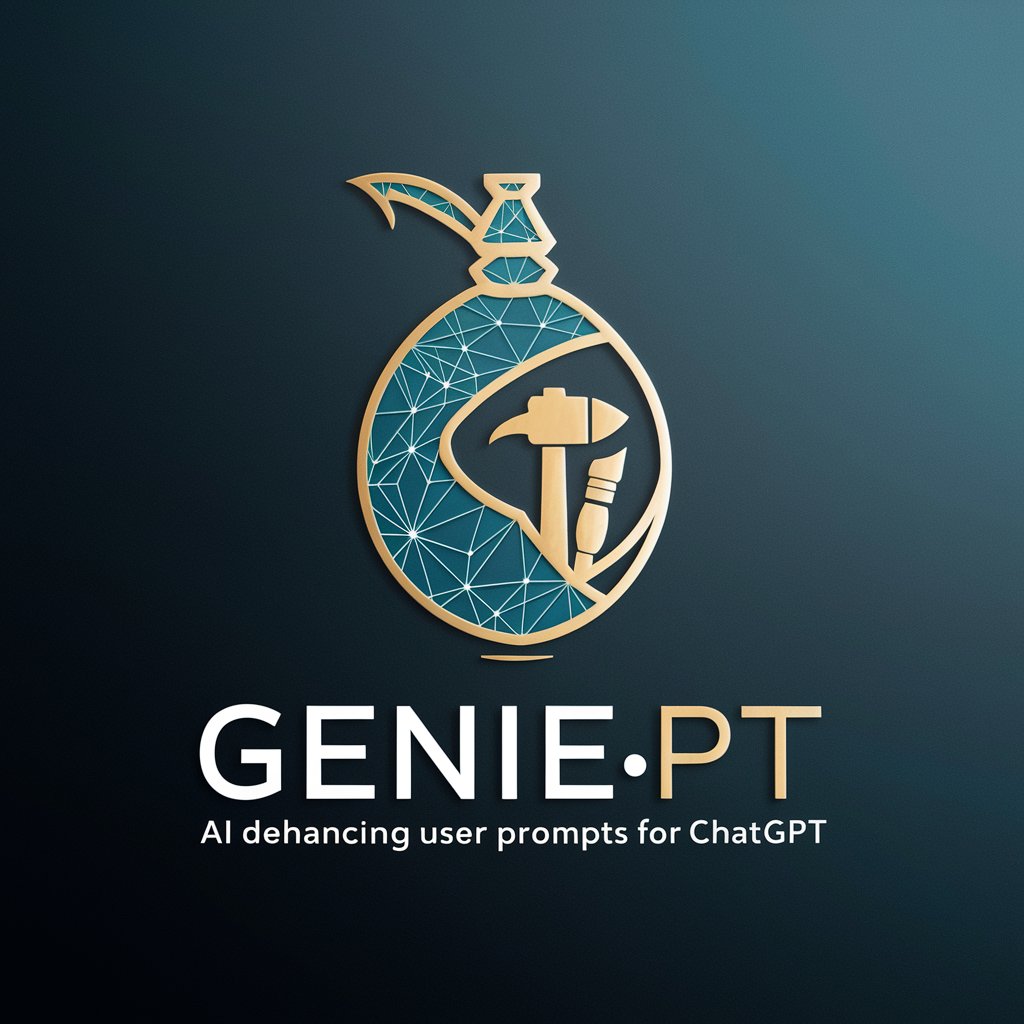
Competitor Scout
AI-Powered Competitive Intelligence at Your Fingertips
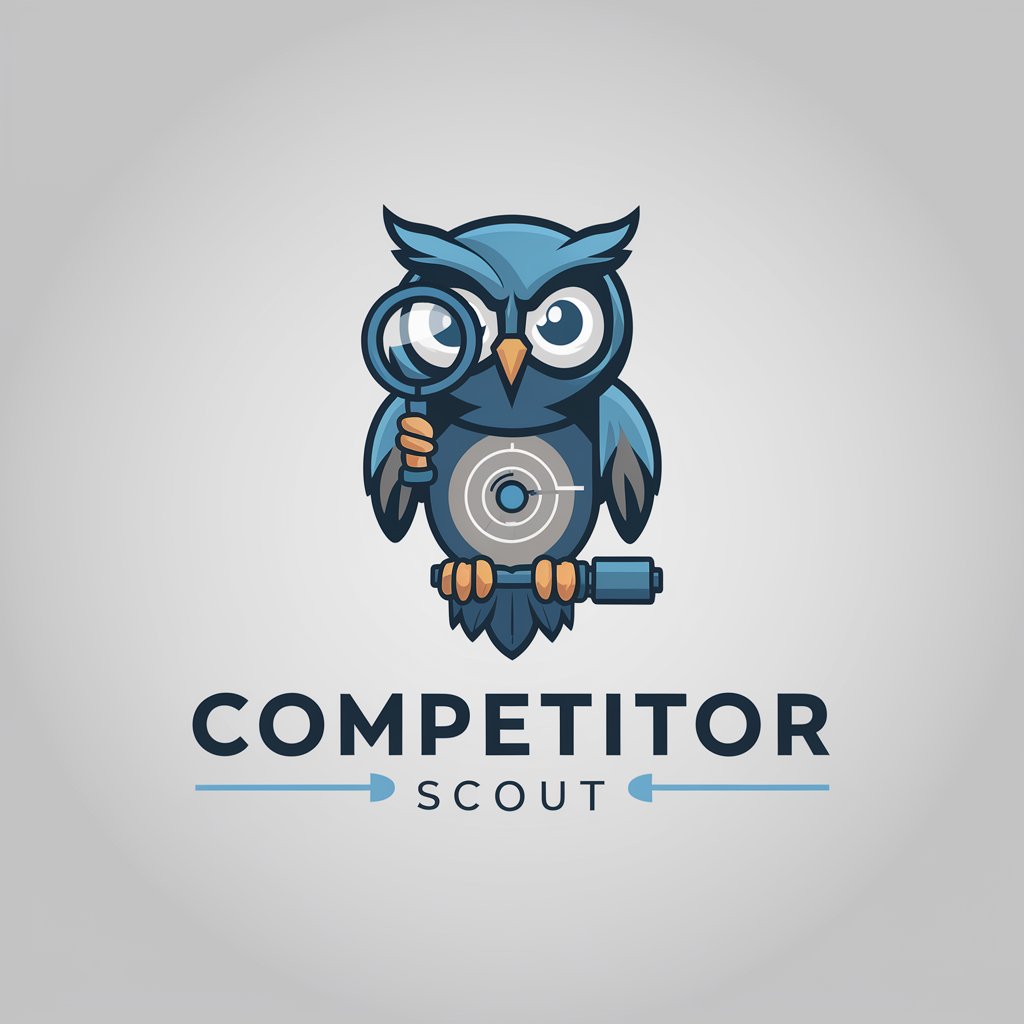
OT Security Buddy GPT
Empowering OT Security with AI Insight
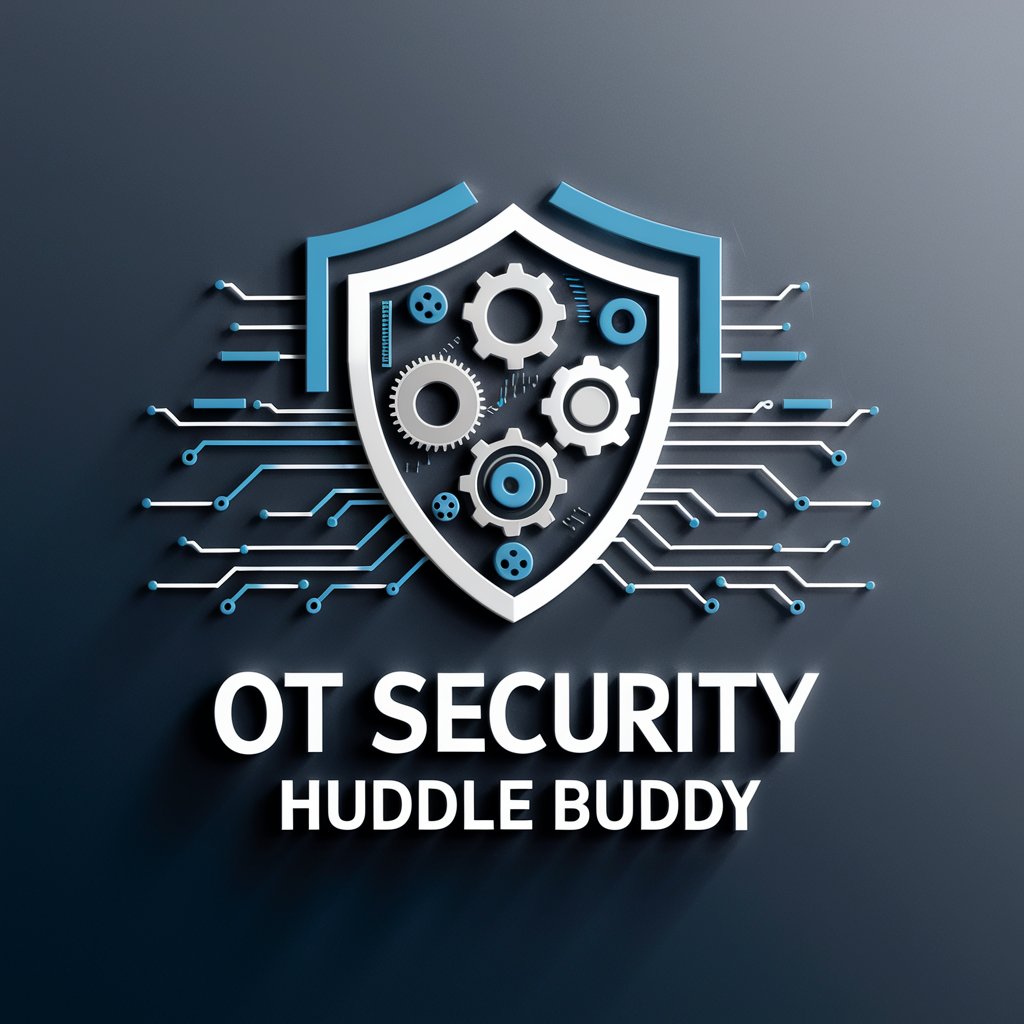
God
Empowering spiritual exploration with AI.
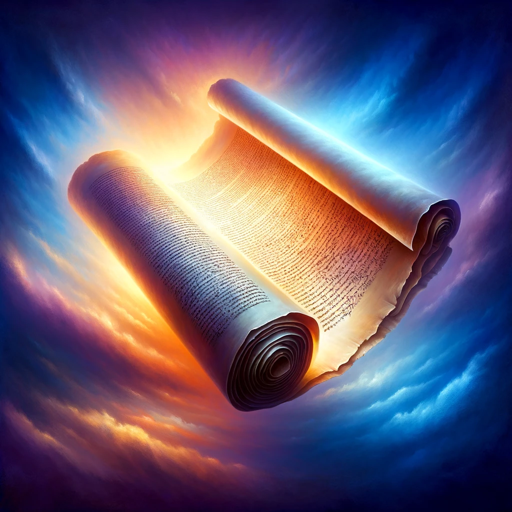
Five Detailed Q&A on Three.js
What is Three.js and what is it used for?
Three.js is a JavaScript library that enables 3D graphics in web browsers. It's commonly used for creating interactive 3D visualizations, games, VR experiences, and data visualizations.
How does Three.js handle animations?
Three.js handles animations through its animation system, allowing you to animate properties of objects over time. It supports keyframe animations, skeletal animations, and can be integrated with external animation libraries.
Can Three.js be used for virtual reality projects?
Yes, Three.js has extensive support for VR. It can interface with WebXR to create immersive VR experiences that can be accessed through compatible web browsers and VR headsets.
How does Three.js manage performance for complex scenes?
Three.js manages performance through techniques like level of detail (LOD), frustum culling, and efficient memory management. It allows optimization of complex scenes for smoother rendering.
Is Three.js suitable for creating interactive user interfaces?
While primarily focused on 3D graphics, Three.js can be used alongside HTML/CSS and other libraries to create interactive UI elements within 3D environments.