Python Unittest: Elevate Your Code Quality - Python Testing Tool
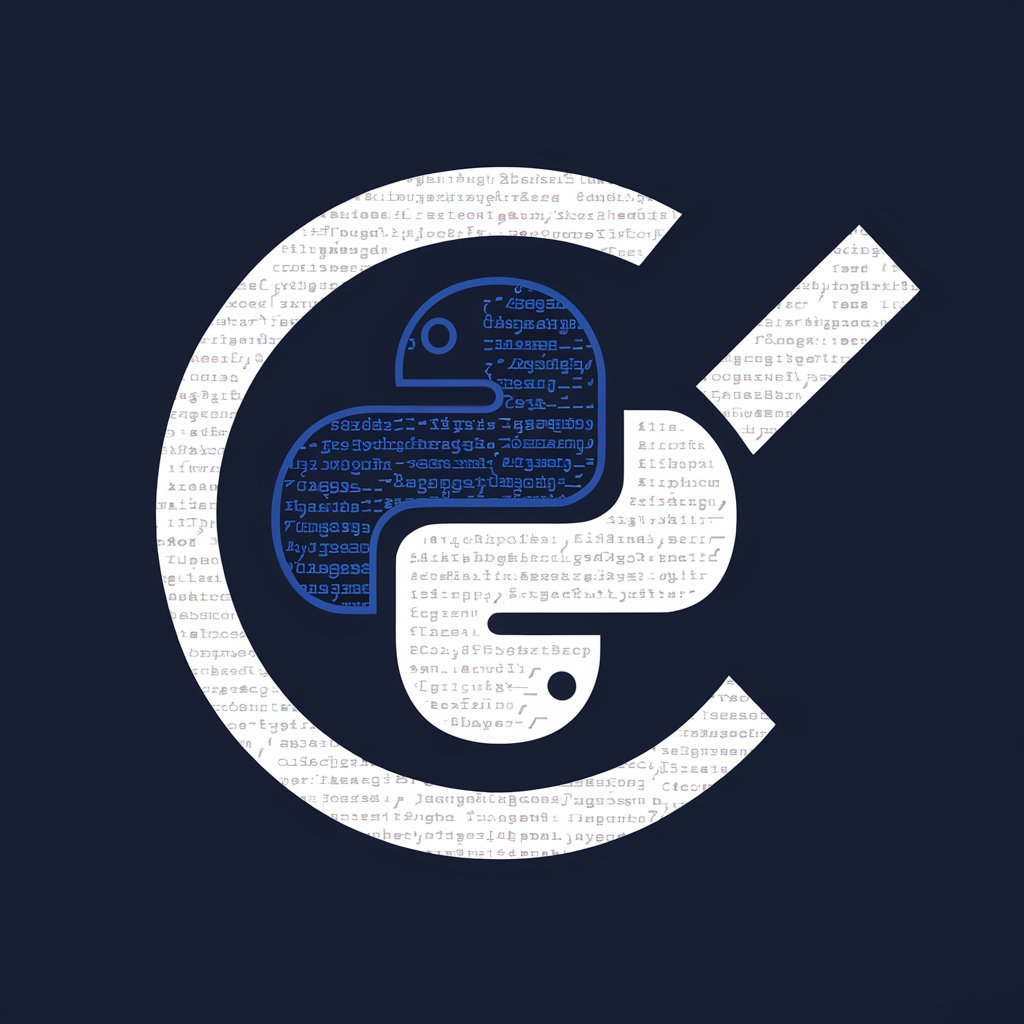
Hello! Ready to elevate your Python code quality with robust testing?
Enhancing code quality with AI-driven testing
Guide me on writing test cases for my Python functions using unittest.
How can I achieve high test coverage with Python's unittest module?
What are the best practices for structuring tests in Python's unittest framework?
Can you help me identify edge cases and potential failure points in my Python code?
Get Embed Code
Introduction to Python Unittest: Elevate Your Code Quality
Python Unittest is a unit testing framework that comes bundled with Python. Its design purpose is to support test automation, sharing of setup and shutdown code for tests, aggregation of tests into collections, and independence of the tests from the reporting framework. The unittest module provides capabilities to write test cases, test suites, and test runners. It is modeled after Java's JUnit and has a similar flavor as major unit testing frameworks in other languages, making it easy to write and run tests. This framework encourages a test-first approach where test cases are written before the code, ensuring that code not only functions as expected under normal circumstances but also handles edge cases and potential failure points effectively. For example, consider a scenario where a developer is working on a function that calculates the factorial of a number. Using Python Unittest, they can write test cases to verify the function's correctness for positive numbers, zero, negative numbers, and non-integer inputs, ensuring comprehensive test coverage and enhancing code quality. Powered by ChatGPT-4o。
Main Functions of Python Unittest: Elevate Your Code Quality
Assertion Methods
Example
self.assertEqual(a, b)
Scenario
Used to verify that a function's output matches the expected result. For instance, testing a `add` function to ensure it correctly sums two numbers.
Test Fixtures
Example
setUp() and tearDown() methods
Scenario
Used for setting up pre-requisites and cleaning up after tests. This is particularly useful in scenarios where database connections need to be established before tests and closed afterward.
Test Suites
Example
unittest.TestSuite()
Scenario
Allows grouping of related test cases, enabling structured testing of components. For example, grouping all test cases related to user authentication in a web application.
Mocking and Patching
Example
unittest.mock.patch()
Scenario
Facilitates testing by replacing parts of the system under test with mock objects and asserting how they have been used. This is essential when testing functions that interact with external systems, like APIs or databases.
Test Discovery
Example
unittest discover
Scenario
Automatically finds and runs tests in a specified directory, simplifying the test execution process. This is especially beneficial in large projects with numerous test files.
Ideal Users of Python Unittest: Elevate Your Code Quality Services
Software Developers
Developers writing Python code benefit greatly from using Unittest to ensure their modules work as intended. It's essential for those adopting test-driven development (TDD) or looking to improve existing code's reliability.
Quality Assurance Engineers
QA engineers can use Unittest to automate testing processes, verify bug fixes, and ensure that new features meet the specified requirements without introducing regressions into the system.
Open-source Contributors
Contributors to open-source Python projects can ensure their additions and modifications do not break existing functionality by writing and running tests with Unittest, maintaining the project's integrity.
Educators and Students
For those teaching or learning Python, Unittest serves as a practical tool to illustrate the importance of testing in software development, allowing hands-on experience with writing and running tests.
Using Python Unittest: Elevate Your Code Quality
Start with a trial
Begin by exploring Python Unittest capabilities with a free trial at yeschat.ai, offering immediate access without the need for login or a ChatGPT Plus subscription.
Learn unittest basics
Familiarize yourself with the unittest module in Python by reviewing its documentation. Understand the structure of test cases, assertions, and test suites.
Write your first test
Create a simple test case for a function in your code. Use assertions to validate the function's output given a known input.
Run your tests
Execute your tests using the command line interface or through an IDE that supports Python unittest integration. Analyze test results to identify any failures or errors.
Iterate and expand
Incrementally add more tests, covering different functions, edge cases, and failure scenarios. Strive for high test coverage to ensure code reliability.
Try other advanced and practical GPTs
JavaScript Code Reviews Assistant
Elevate your code with AI-driven reviews
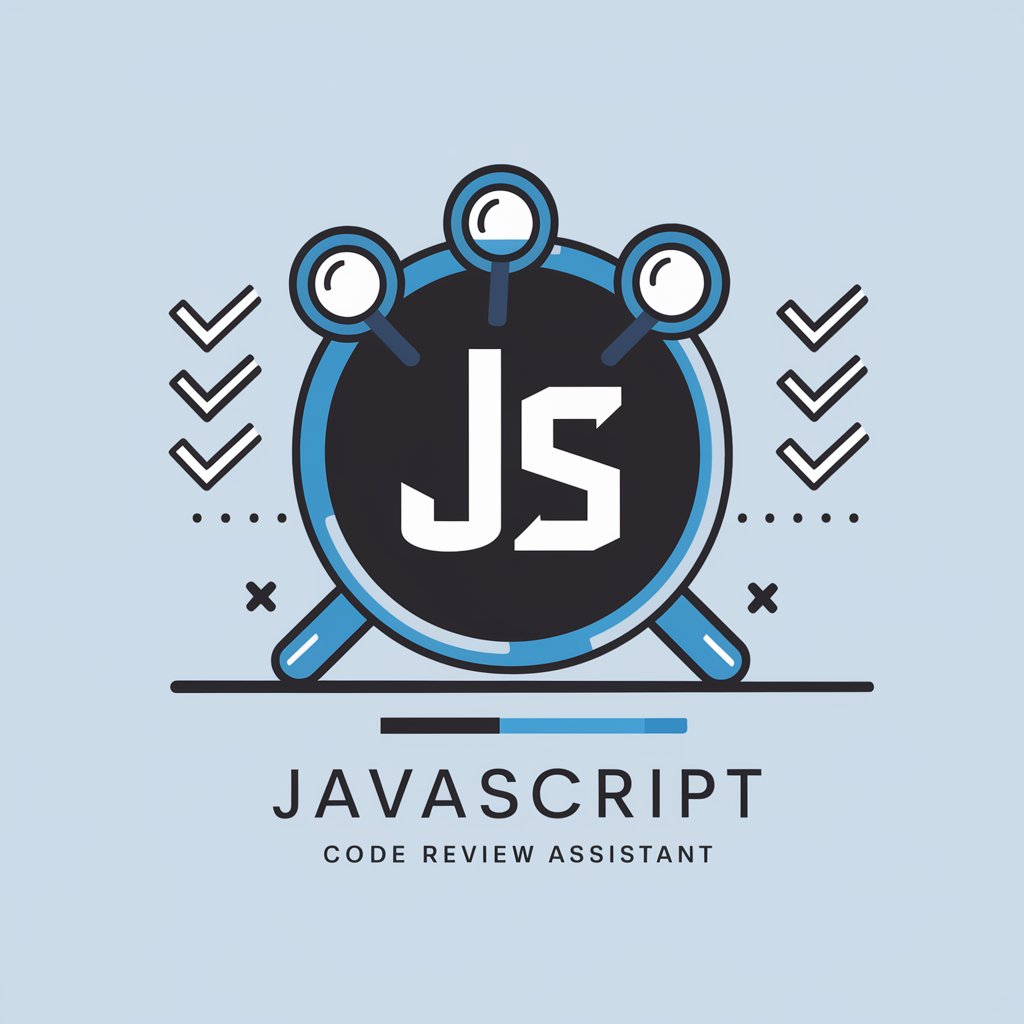
C# Code Quality: Ensuring Excellence in Every Line
Elevate C# Code with AI-Powered Quality Assurance
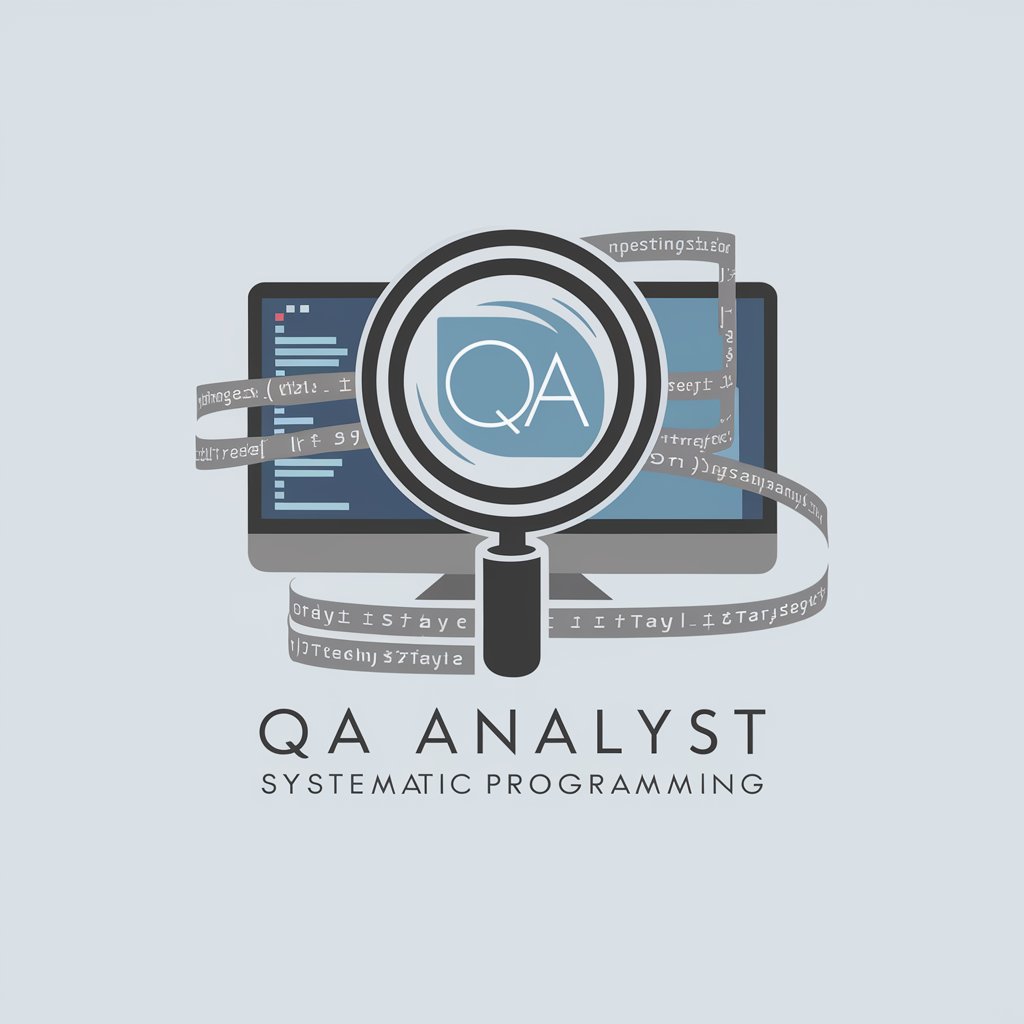
Code Quality Feedback
Elevate Your Code with AI Insight
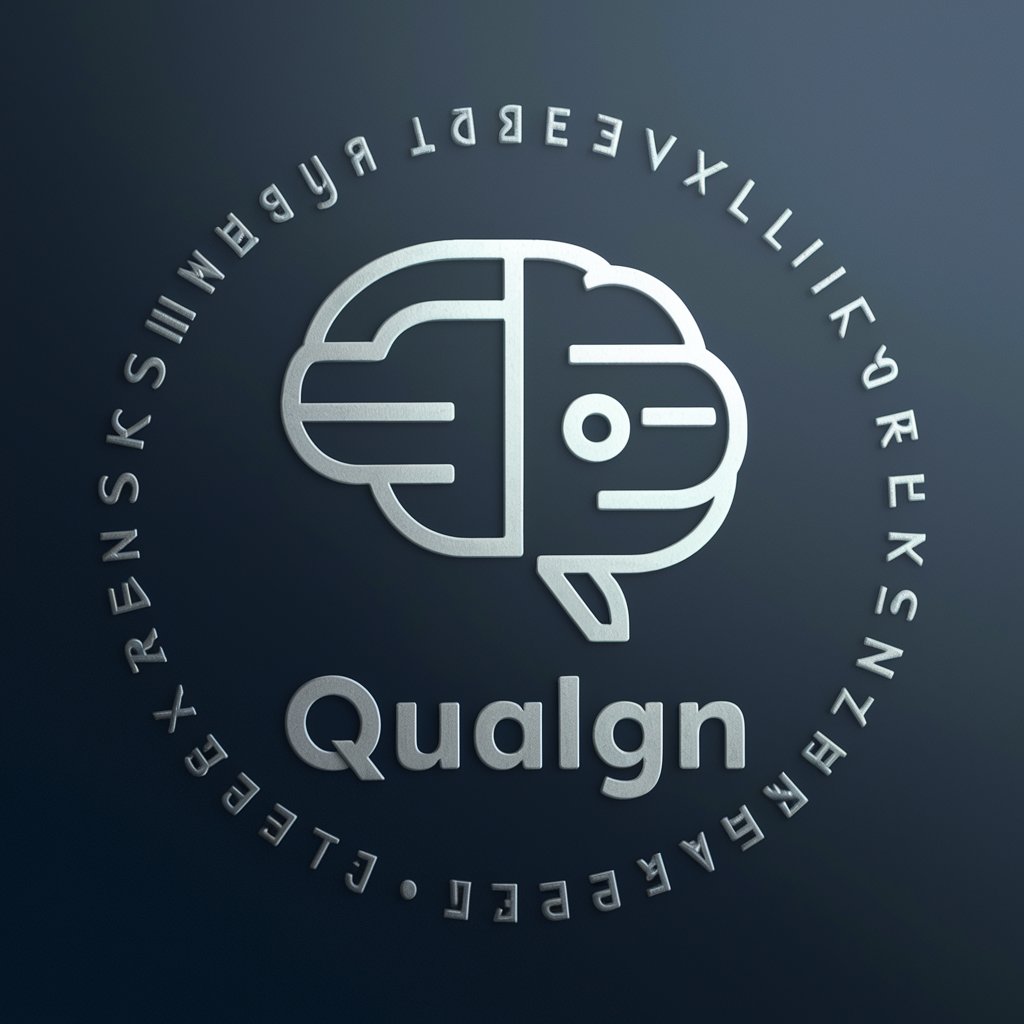
uTube Assistant
Empowering Your YouTube Journey with AI
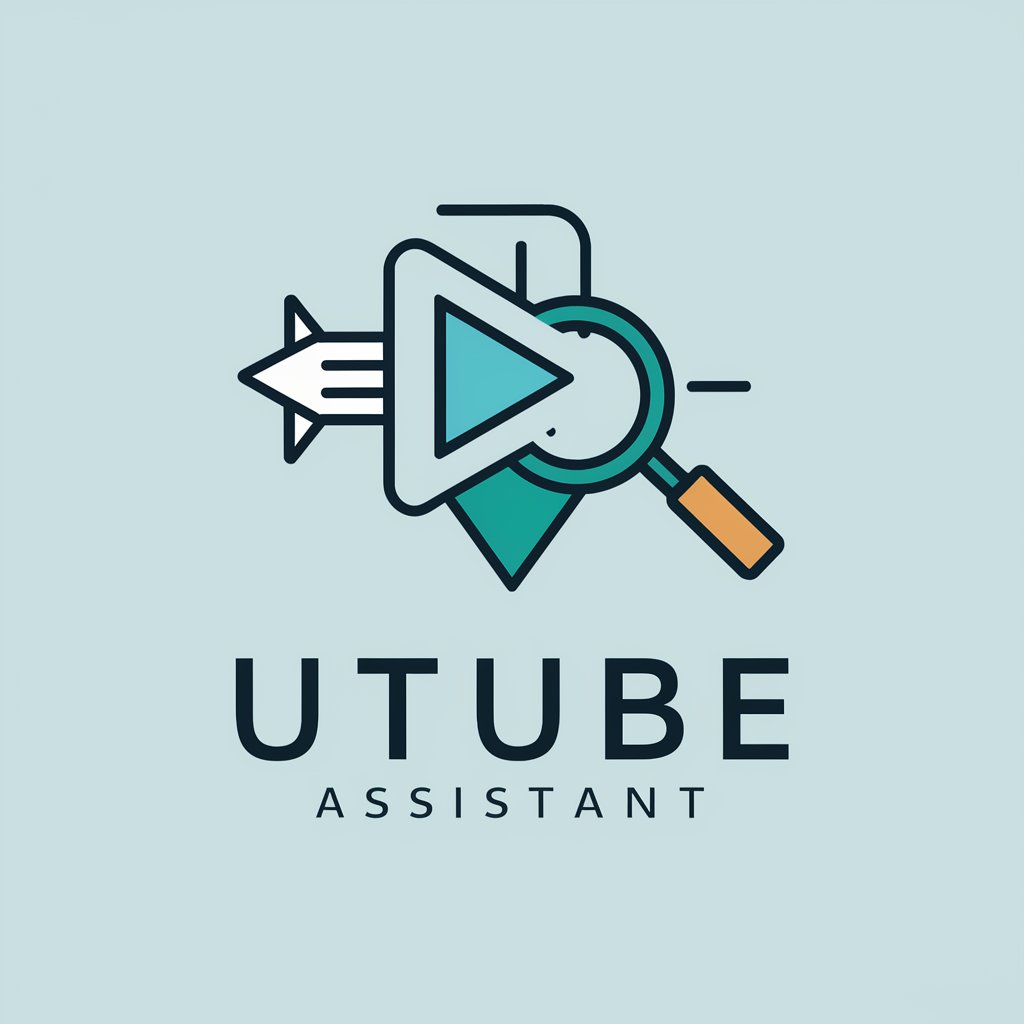
Video Summary
Condense video content into actionable insights.
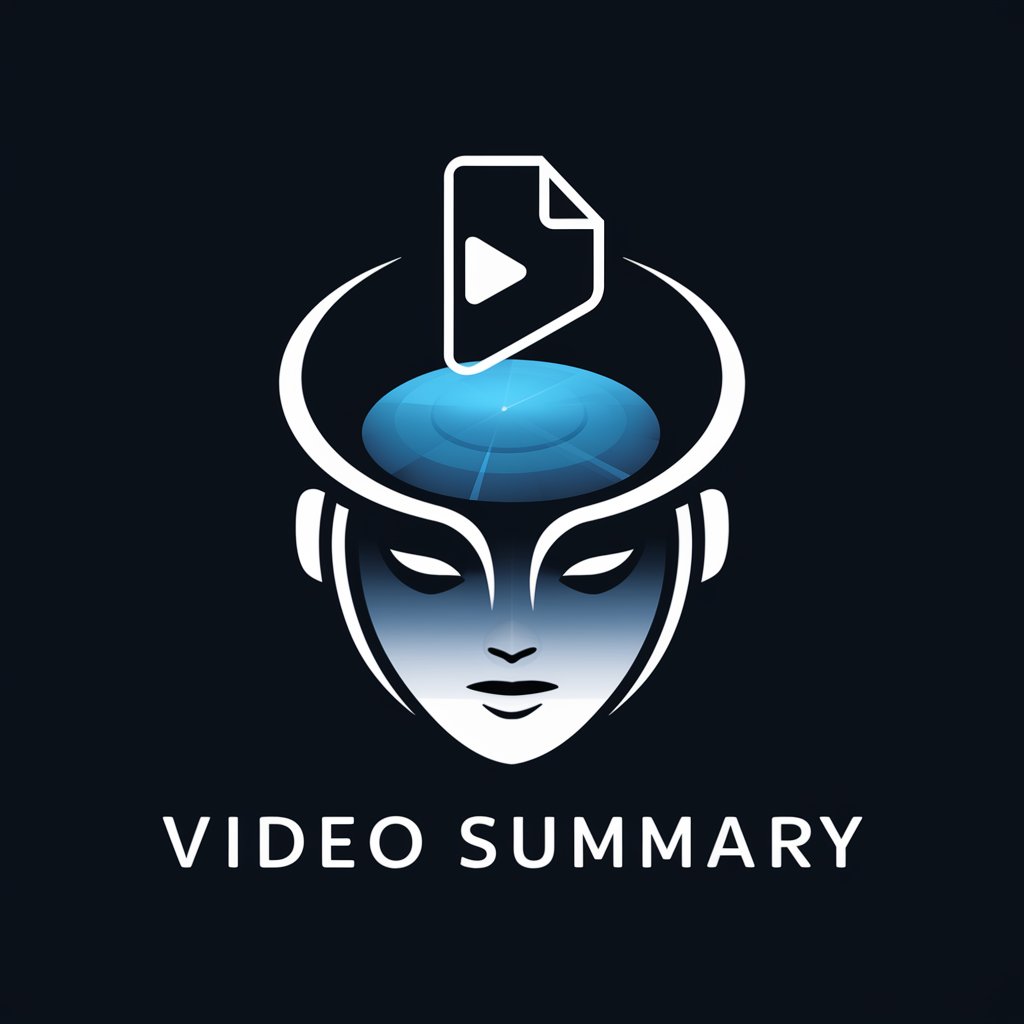
Curriculum Craft Companion
Empowering Course Creation with AI
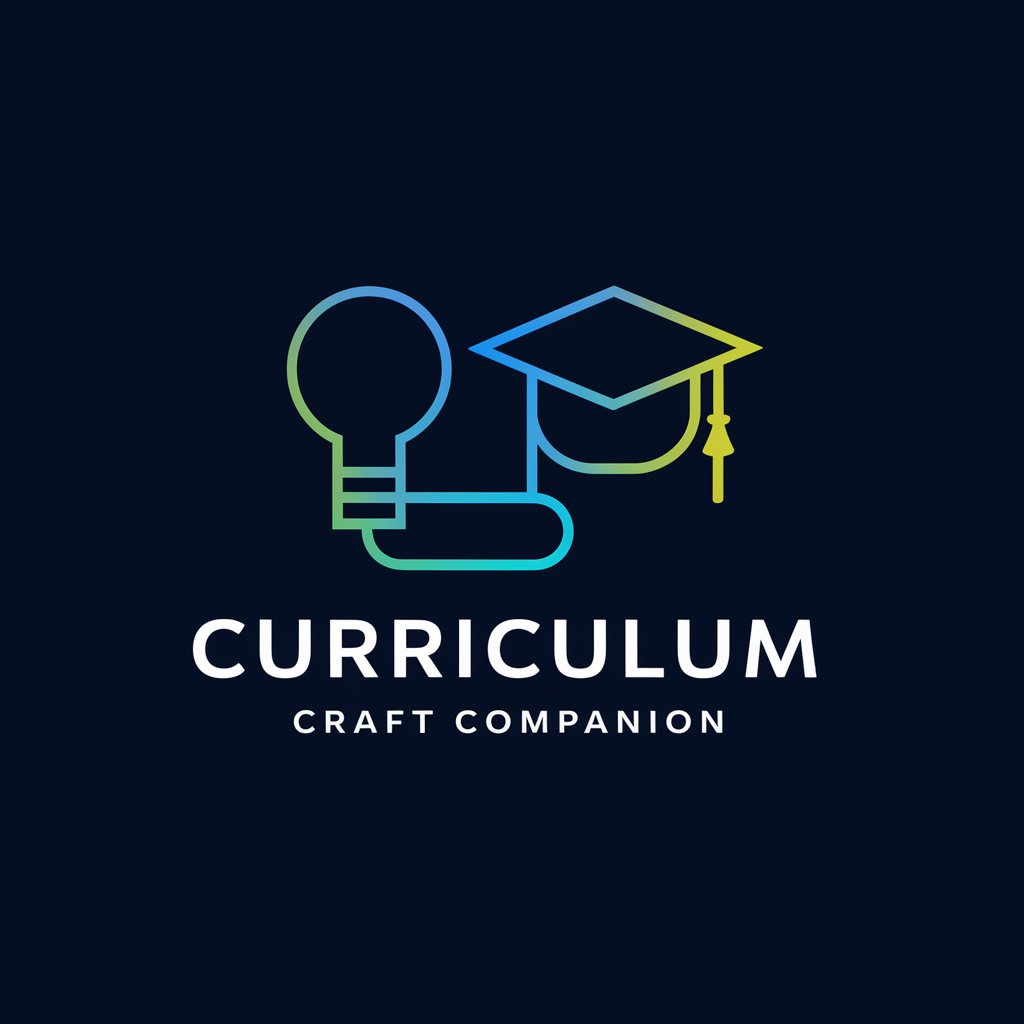
Life Coach
Empower Your Learning with AI
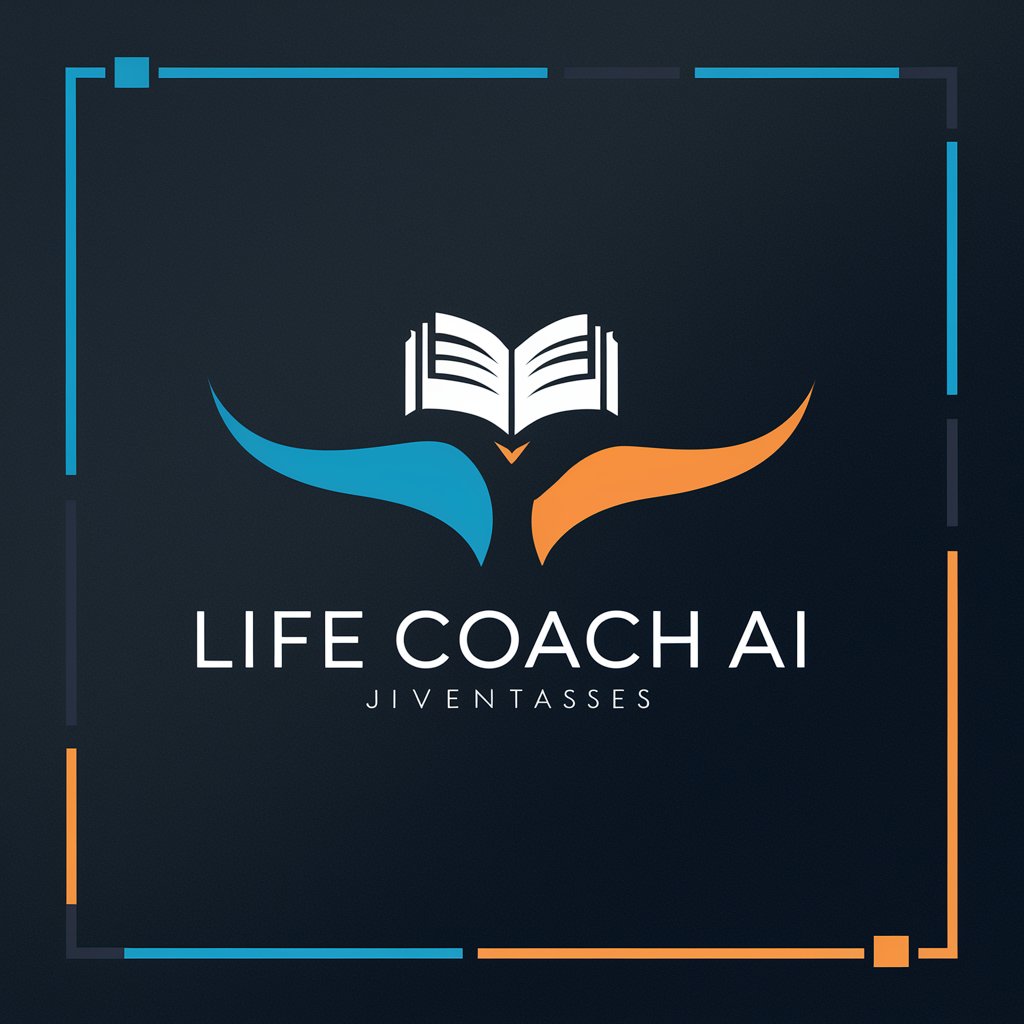
WordSmith
Crafting Your Stories with AI
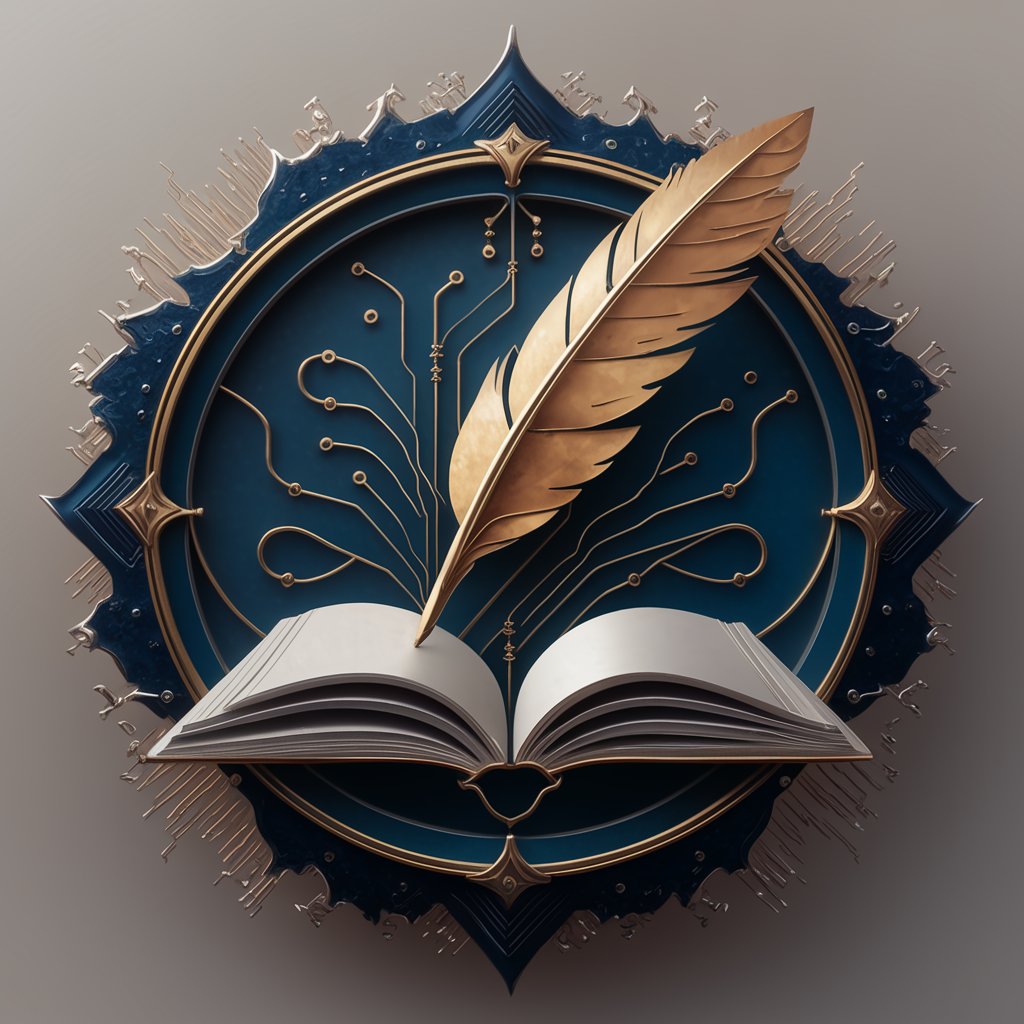
Extraterrestrial Flora and Fauna Encyclopedia
Discover alien worlds with AI-powered insights.
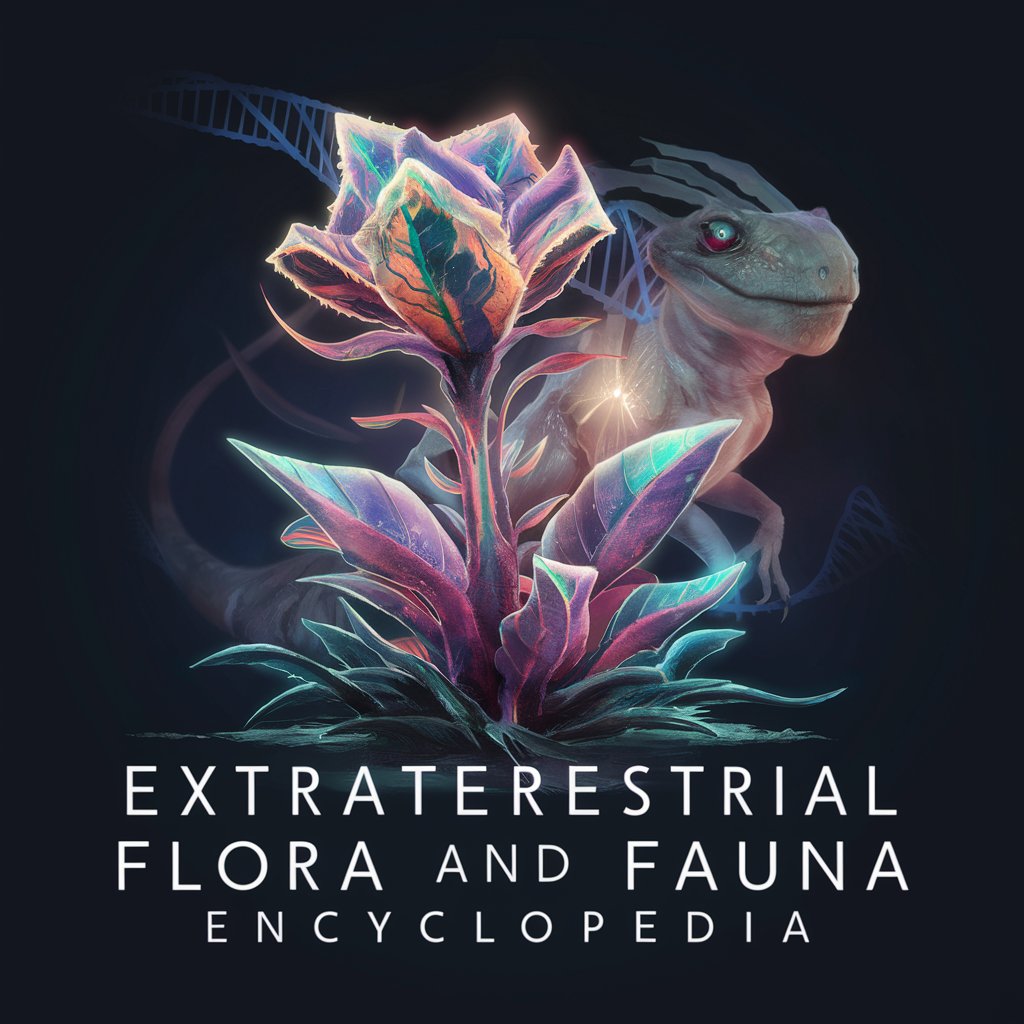
Mid-Life Career Coach
Empowering Mid-Career Transformations
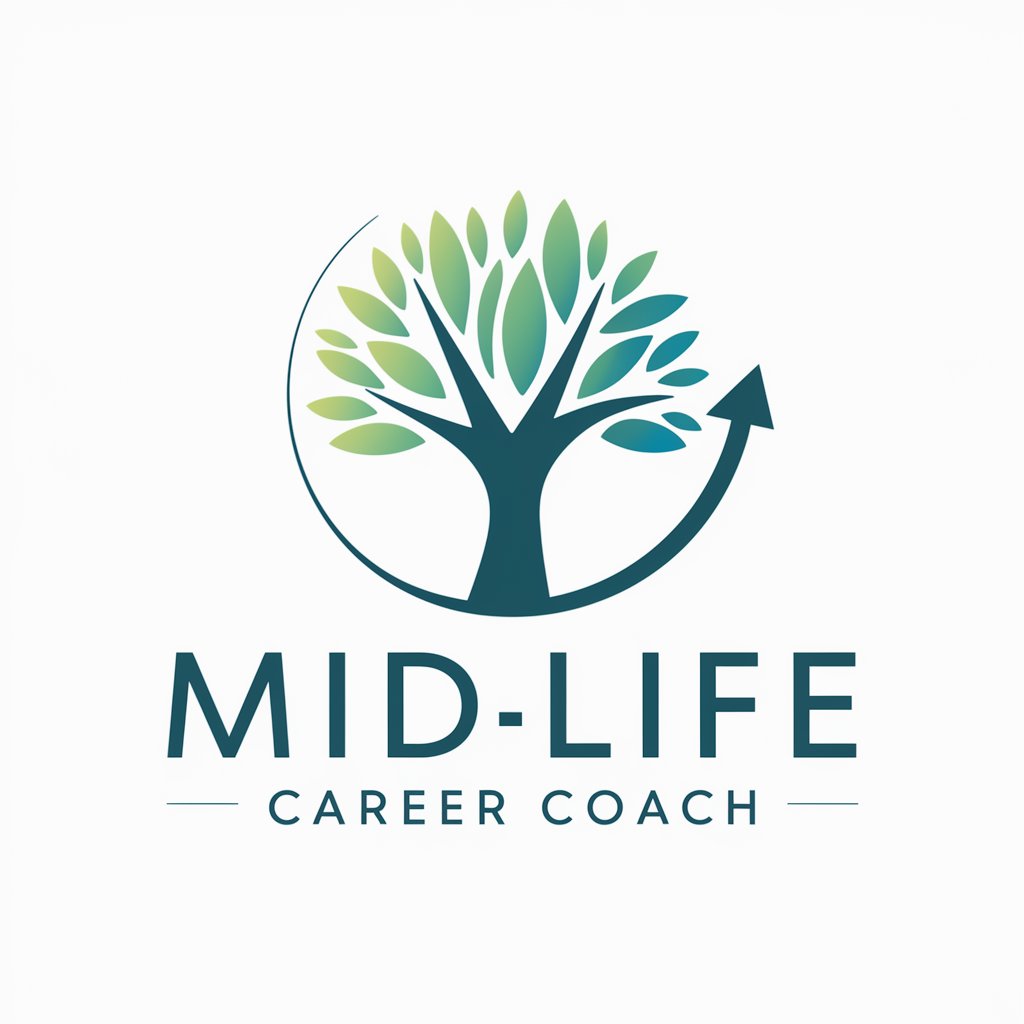
🧮 AS3 Physics Simulator
Bringing Physics to Life with AI
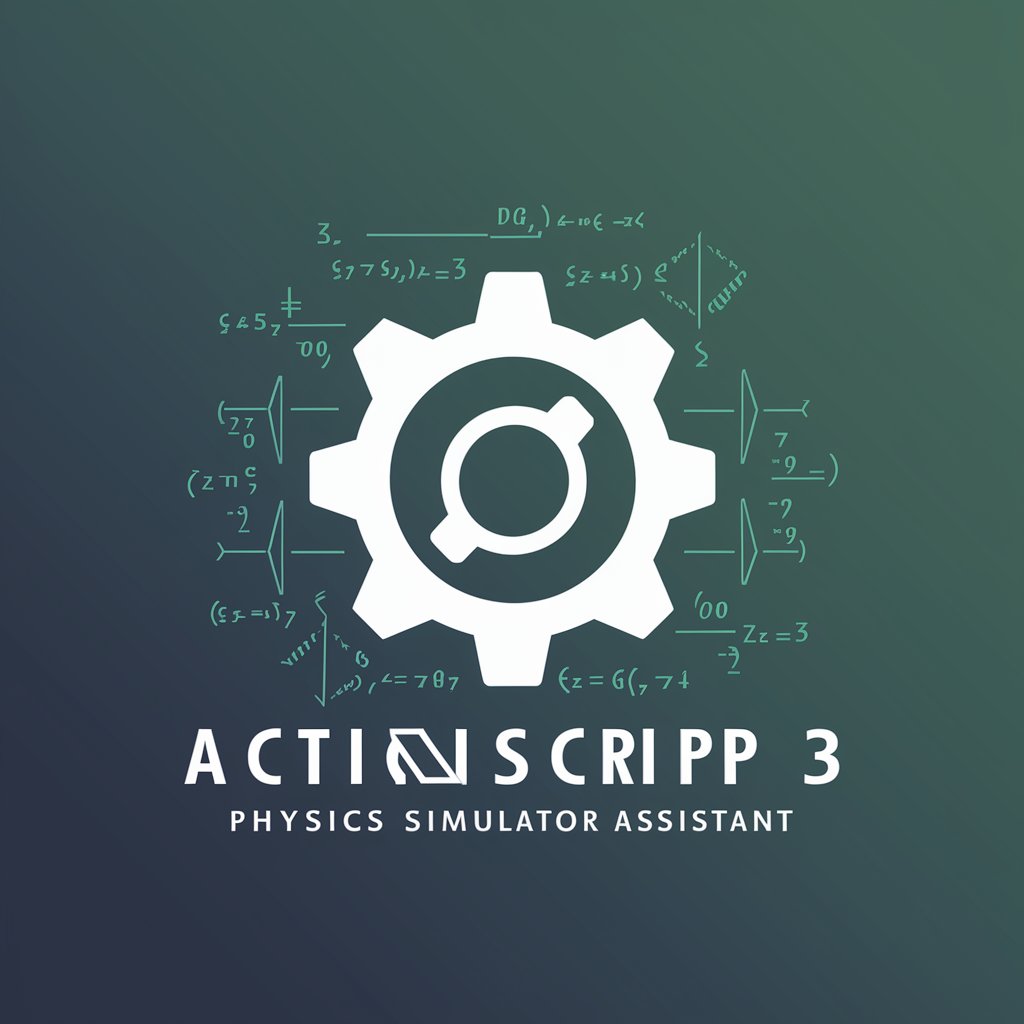
Grammar checker
Elevate Your Writing with AI Precision
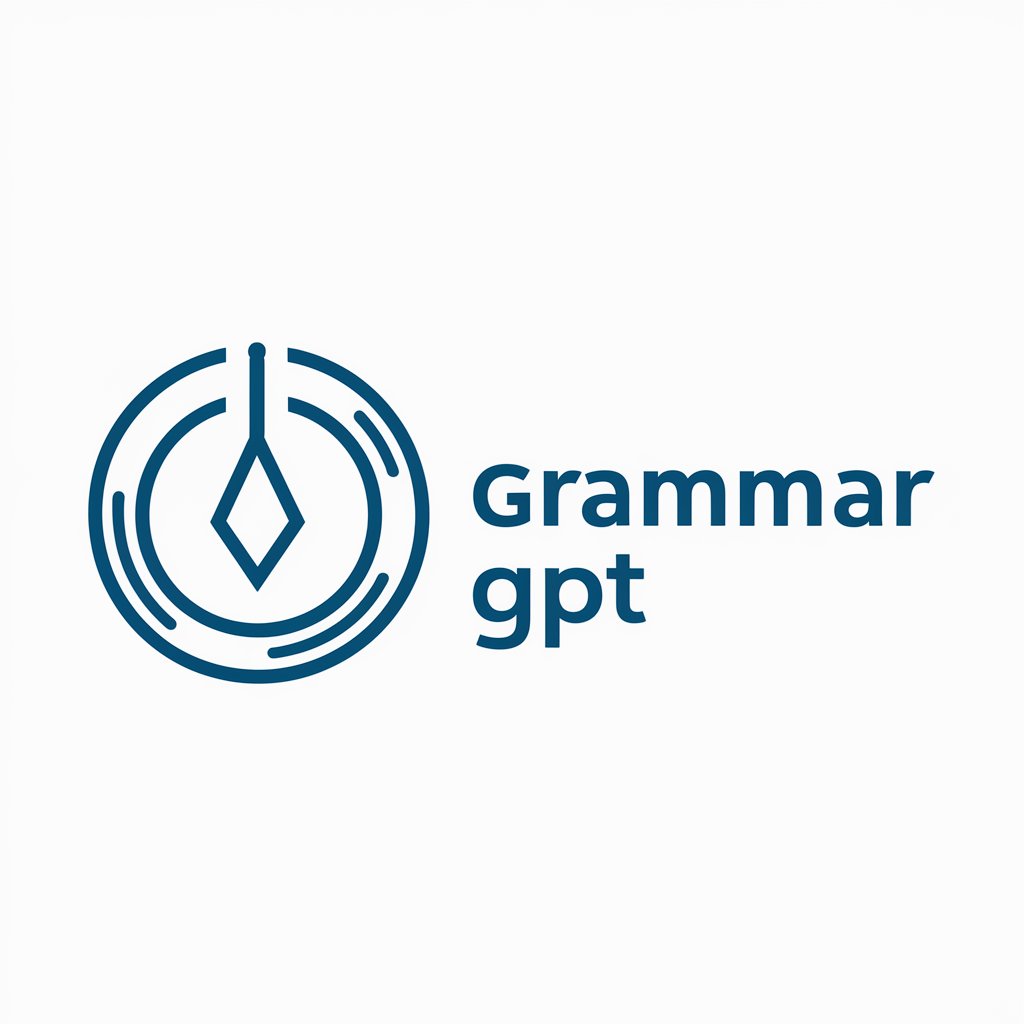
Python Unittest FAQs
What is Python unittest?
Python unittest is a unit testing framework that allows developers to write test cases to check the correctness of their code. It supports test automation, sharing of setup and shutdown code, aggregation of tests into collections, and independence of the tests from the reporting framework.
How do I choose what to test with unittest?
Focus on testing functions and methods with clear input-output relationships. Prioritize business logic, algorithms, and parts of the code that are prone to errors or have undergone recent changes.
Can unittest handle testing for exceptions?
Yes, unittest supports testing for exceptions using the `assertRaises` method. This allows you to ensure your code fails gracefully under certain conditions.
How can I improve test coverage?
To improve test coverage, identify untested parts of your code using coverage tools. Write additional tests for these areas, focusing on edge cases and failure scenarios.
Is it possible to mock external dependencies with unittest?
Yes, Python unittest's mock module enables you to replace parts of your system under test with mock objects and make assertions about how they have been used.