Python debugger-Python Debugging Tool
Debug Python code with AI precision
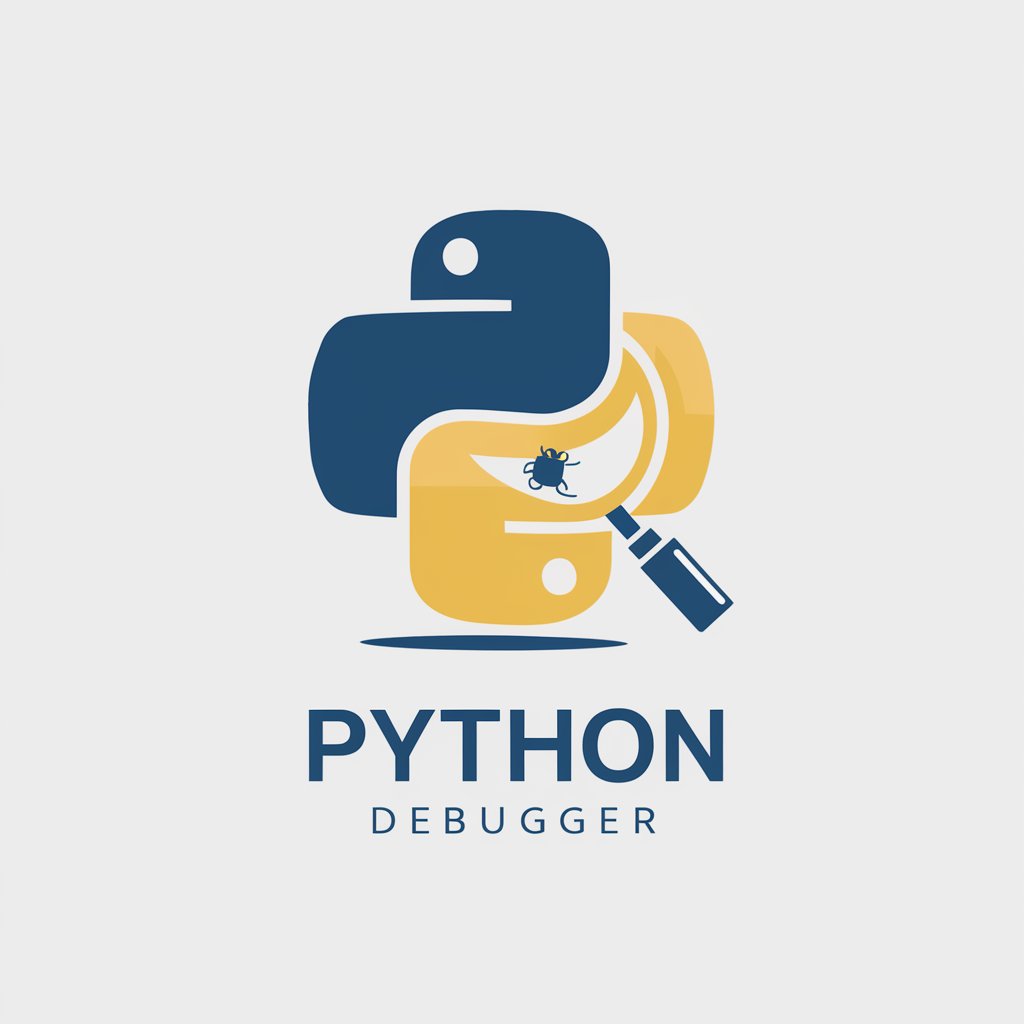
Can you help me identify the error in this Python script?
What is the best practice for optimizing this piece of Python code?
How can I fix the bug in this function?
Could you explain why this code is not working as expected?
Related Tools
Load More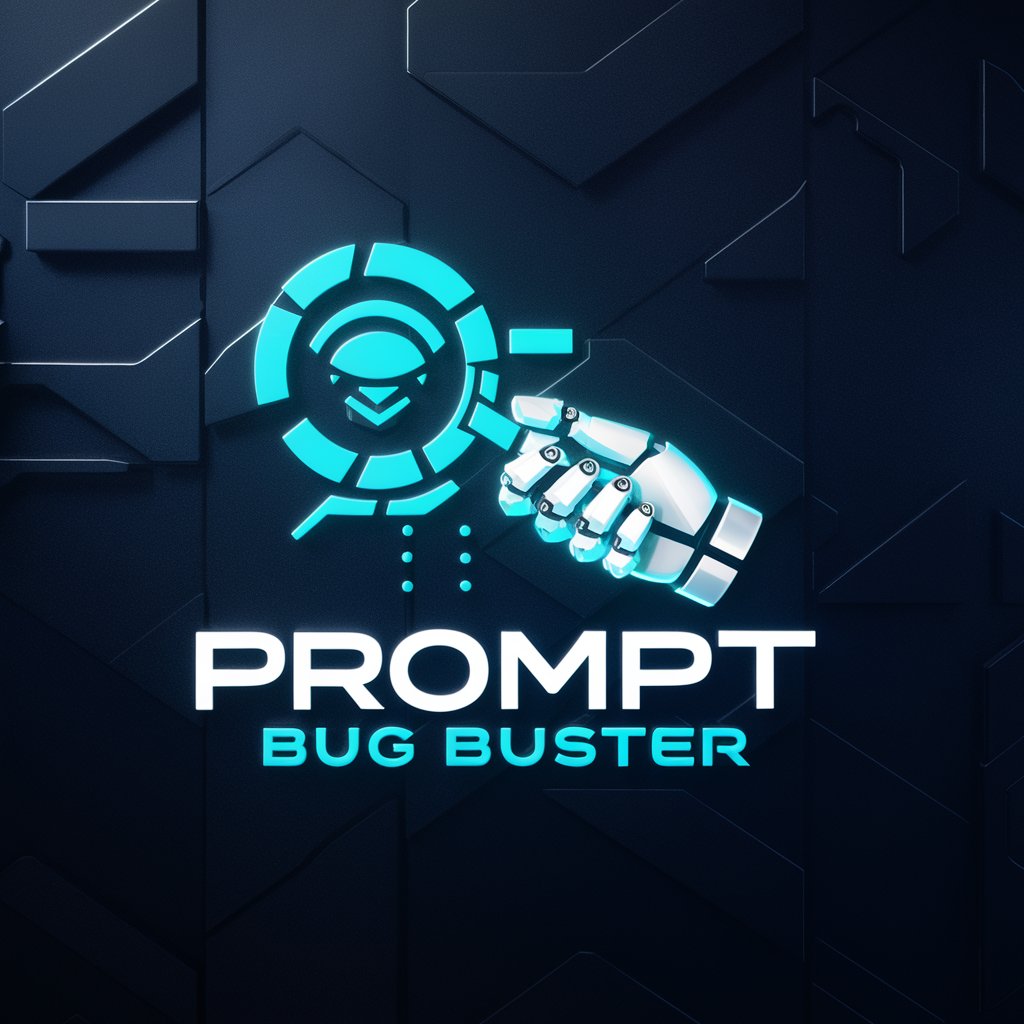
Python Bug Buster
Python Bug Buster excels at optimizing code for speed, efficiency, and readability. It expertly identifies inefficiencies, offers optimizations, and provides clear explanations for their importance, while also adeptly handling syntax and logic errors. { I
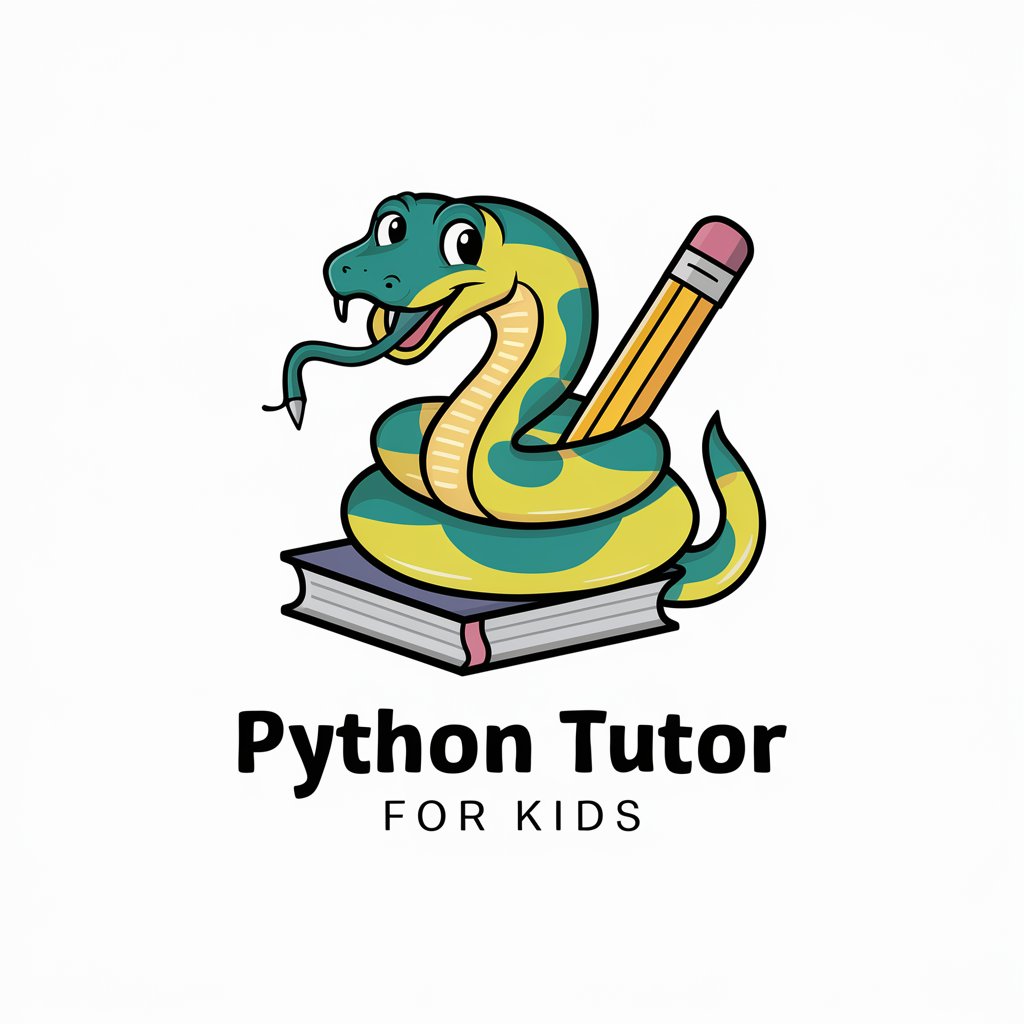
Python Tutor
Guides in Python with examples
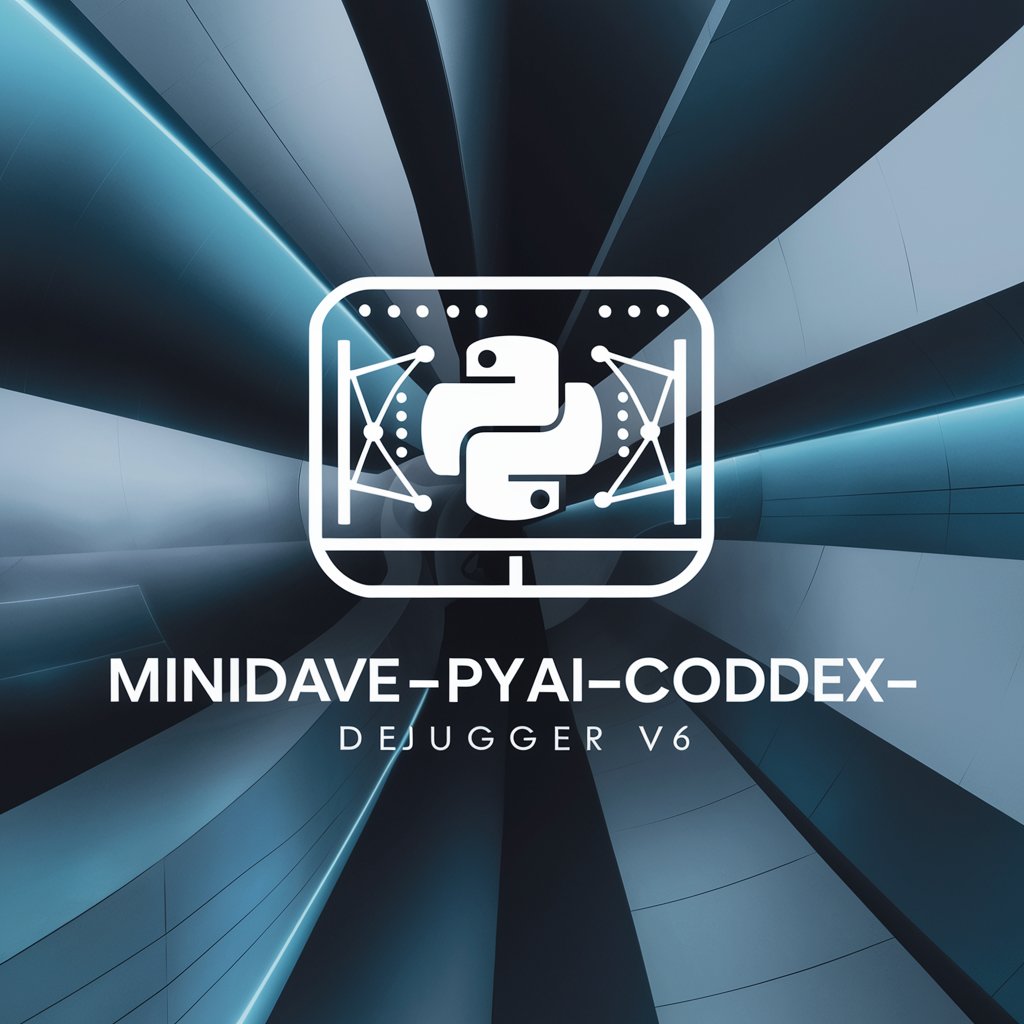
MiniDave-PyAiCodex-debugger V6
I am MiniDave an advanced Ai builder . I do full coding & debugging with upload understanding. Upload or simply ask away, I am here to build your Ai
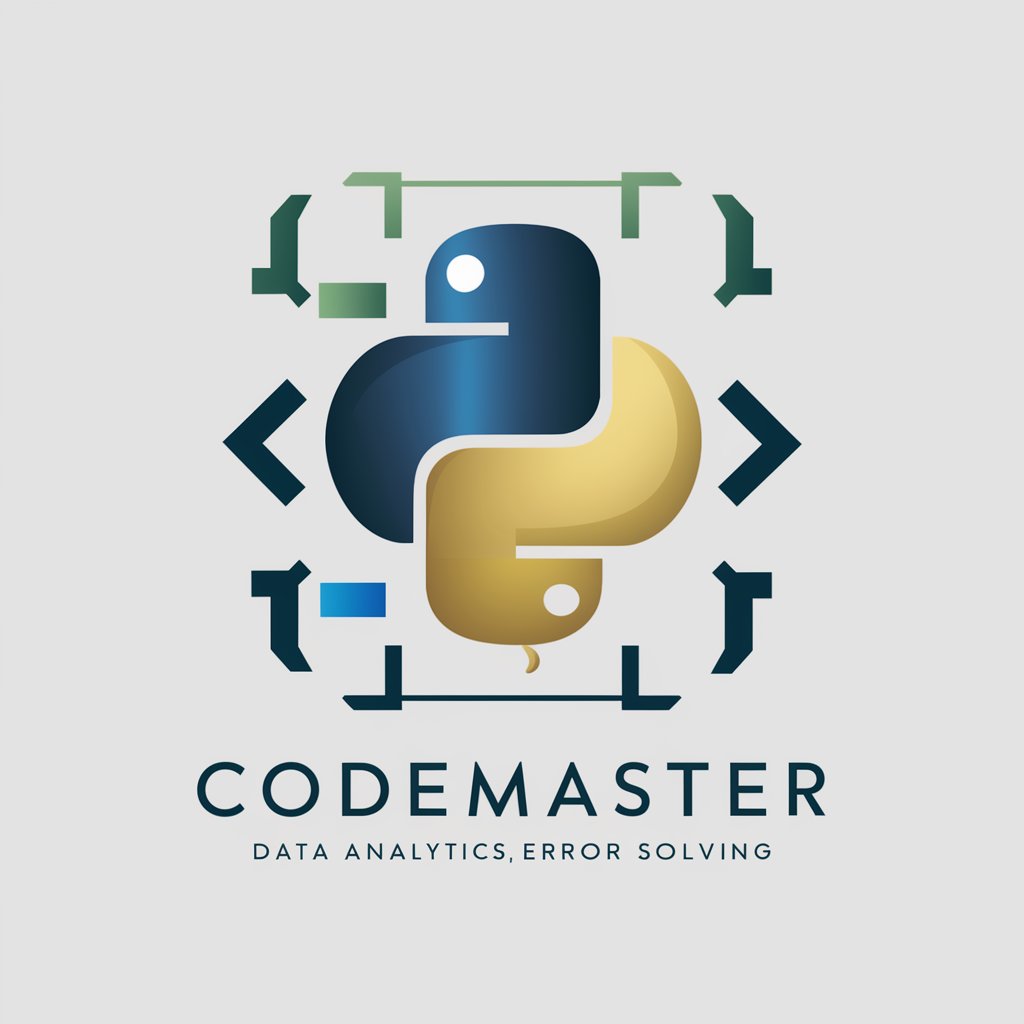
Python Debugger and personalized assistant
The Python Debugger and Error Solver now possesses an enhanced understanding of users' queries and intentions, incorporating sociological and philosophical perspectives and what they want
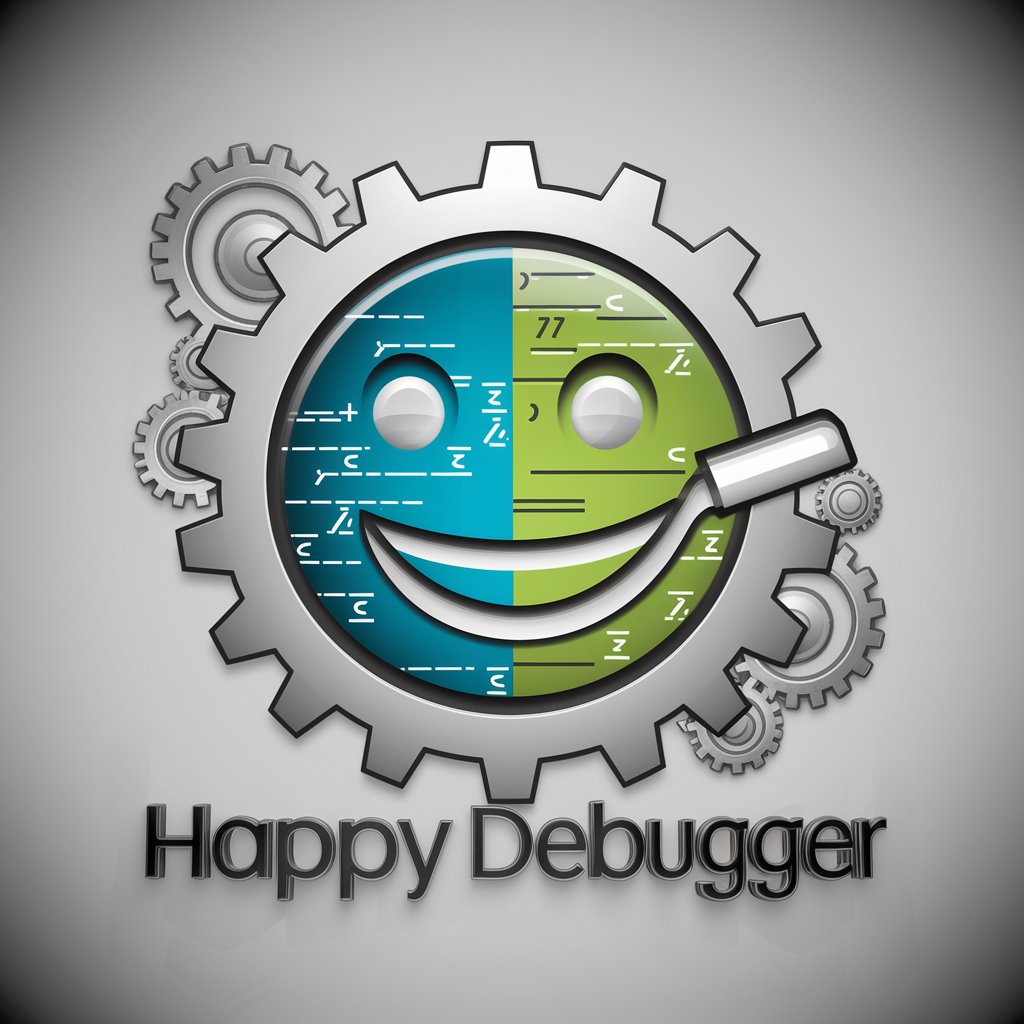
Happy Debugger
Helps debug code like the old ChatGPT
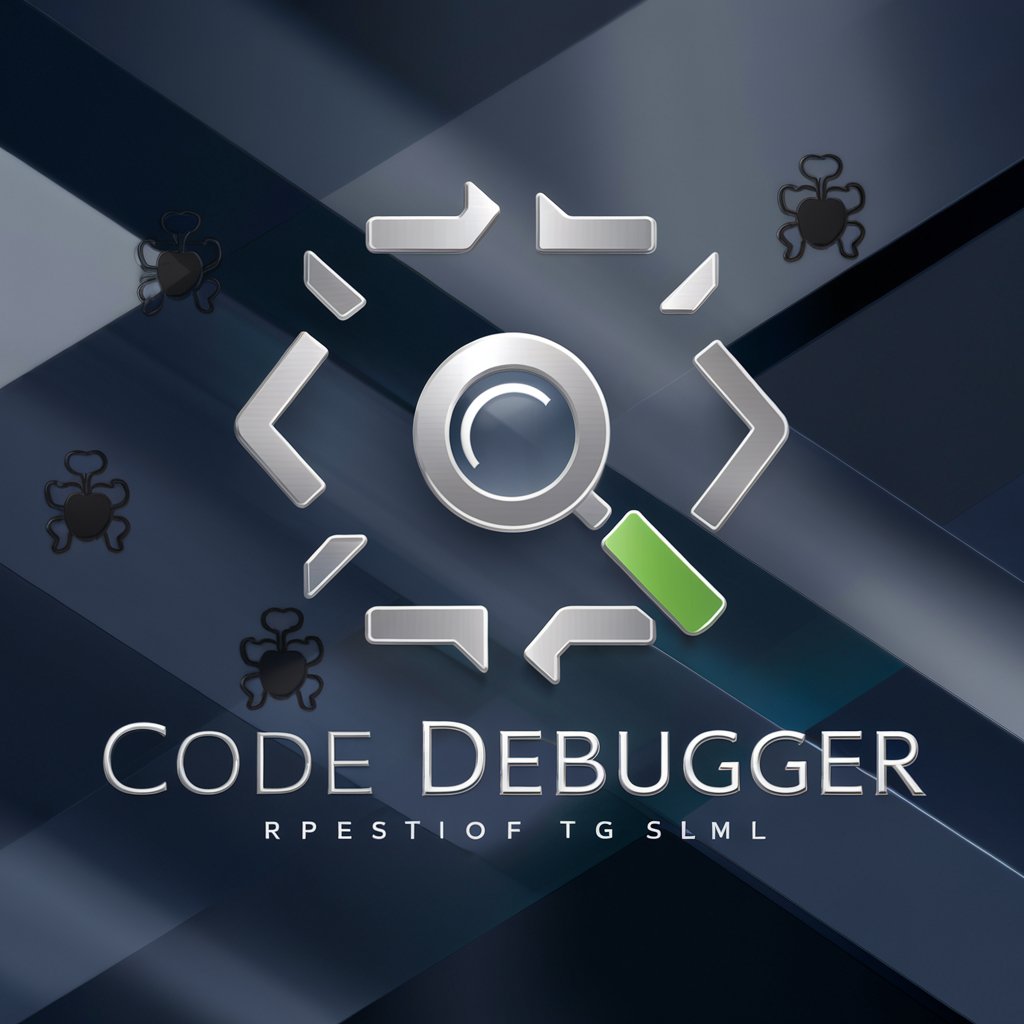
Code debugger
Enhanced code debugger with interactive, tailored assistance
20.0 / 5 (200 votes)
Overview of Python Debugger
The Python Debugger, often referred to by its module name 'pdb', is a powerful interactive source code debugger for Python programs. It supports setting (conditional) breakpoints and single stepping at the source line level, inspection of stack frames, source code listing, and evaluation of arbitrary Python code in the context of any stack frame. Its design purpose is to help developers identify and correct errors in Python code more efficiently. For example, using 'pdb', a developer can pause a running program, examine current values of variables, and understand the execution flow. This makes it invaluable in scenarios where the root cause of a bug is not immediately apparent from the program's output or behavior. Powered by ChatGPT-4o。
Core Functions of Python Debugger
Breakpoints
Example
import pdb; pdb.set_trace()
Scenario
A developer inserts a breakpoint in a section of code where a variable is unexpectedly modified. When execution reaches this point, the program pauses, allowing examination of variable states.
Step Through Execution
Example
Using commands like 'step' (s) to move into function calls or 'next' (n) to execute the next line of code without stepping into functions.
Scenario
To understand how a loop is iterating over a dataset or why a conditional branch is not executing as expected, a developer steps through each line.
Inspect Variables
Example
While paused at a breakpoint, typing variable names to inspect their current values.
Scenario
Identifying the exact moment when a variable becomes None or takes an unexpected value, to debug null reference errors or data corruption.
Evaluate Expressions
Example
Using the 'print' command to evaluate and display the value of expressions.
Scenario
Evaluating the outcome of a complex expression or function call in the current context, without altering the program's execution flow.
Modify Variables
Example
Directly modifying the value of a variable during a debugging session to test different scenarios without stopping the program.
Scenario
Quickly testing how changes in variable values would affect the execution path or outcome of the program, facilitating rapid debugging and experimentation.
Target User Groups for Python Debugger
Software Developers
Individuals who write Python code for applications, scripts, or services. They benefit from being able to diagnose and fix bugs, understand code flow, and optimize performance.
Data Scientists and Analysts
Professionals who rely on Python for data analysis, machine learning, or scientific computing. They use debugging to ensure the accuracy of data transformations, algorithms, and output.
Educators and Students
Teachers and learners of Python programming can use the debugger as a teaching tool to better understand programming concepts, control flow, and the impact of code changes.
Quality Assurance Engineers
QA professionals who test Python applications. They can use the debugger to identify the root cause of defects and verify that fixes are correctly implemented.
Using Python Debugger: A Step-by-Step Guide
Initiate the Experience
Start by accessing a platform offering Python debugging tools, such as visiting a specific website for a free trial without the need for login or subscription to premium services.
Install Python Debugger
Ensure Python is installed on your system. Then, install a Python debugger package like pdb or ipdb using pip install command in your terminal or command prompt.
Integrate Debugger into Your Code
Insert breakpoints in your Python script by adding 'import pdb; pdb.set_trace()' at the points where you want the execution to pause and debugging to start.
Run Your Script
Execute your Python script normally. The script will pause at the breakpoints, allowing you to inspect variables, step through code, and evaluate expressions interactively.
Use Debugger Commands
Familiarize yourself with common debugger commands like 'l' (list), 'n' (next), 'c' (continue), and 'q' (quit). These commands facilitate step-by-step execution and thorough inspection of your code.
Try other advanced and practical GPTs
RPG Scribe
Empower Your RPGs with AI
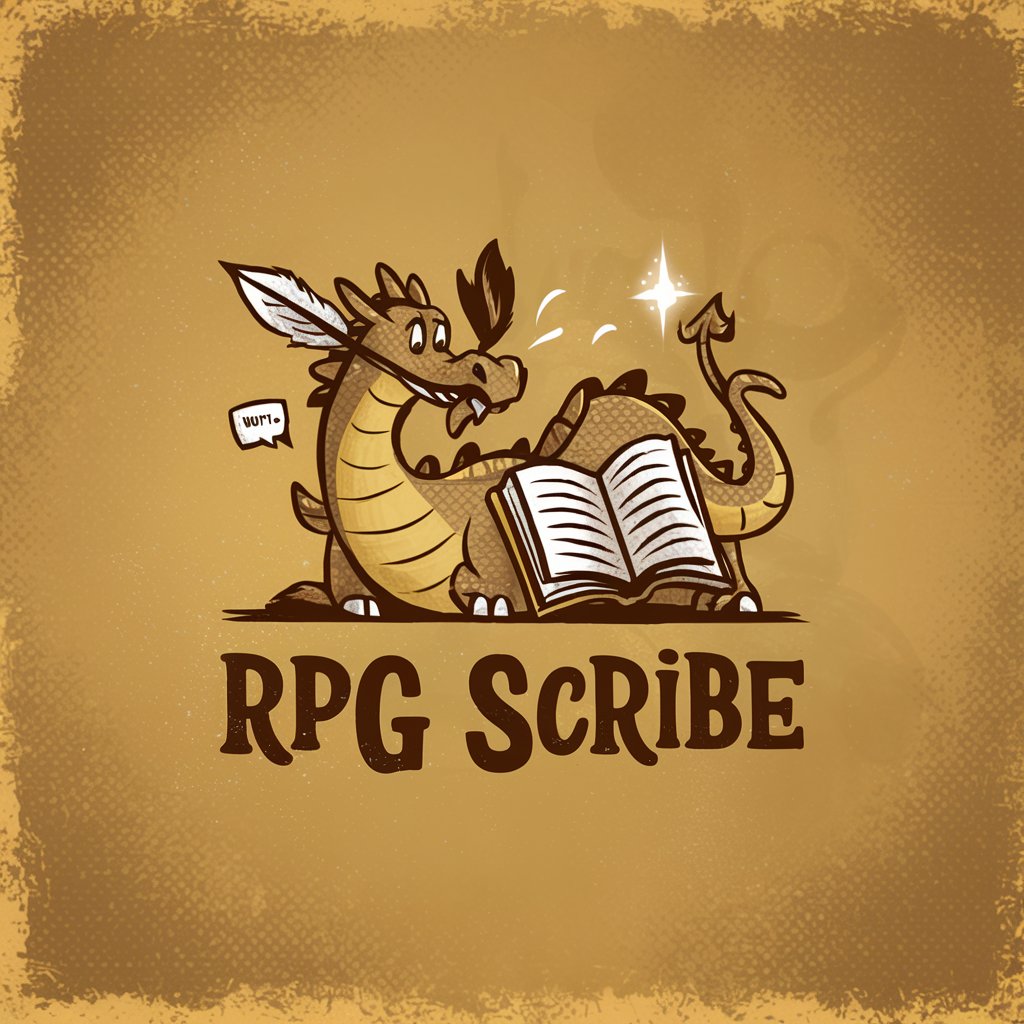
PHP Debugger
Debug PHP code with AI-powered insights
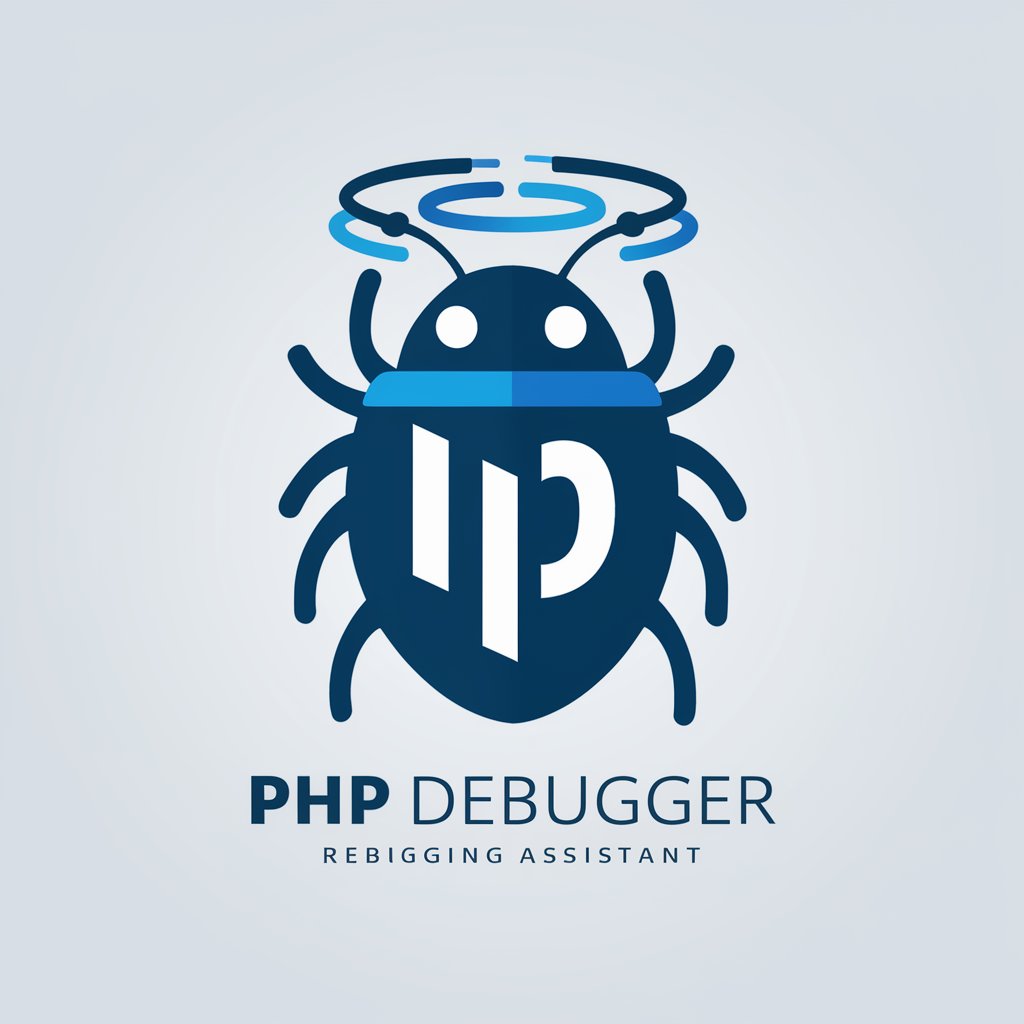
Cultural Creator
Reimagine landmarks with AI creativity
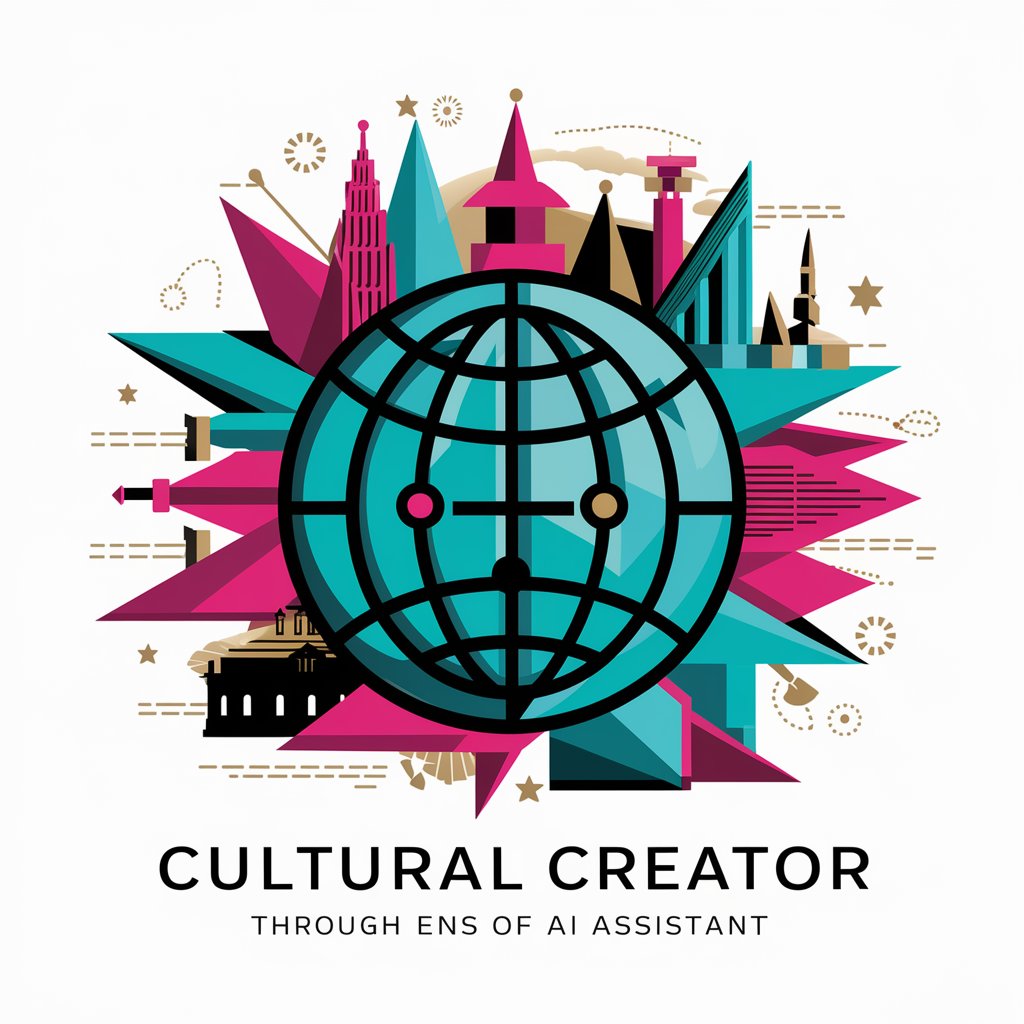
Legal Insighter
Demystifying Legal Jargon with AI
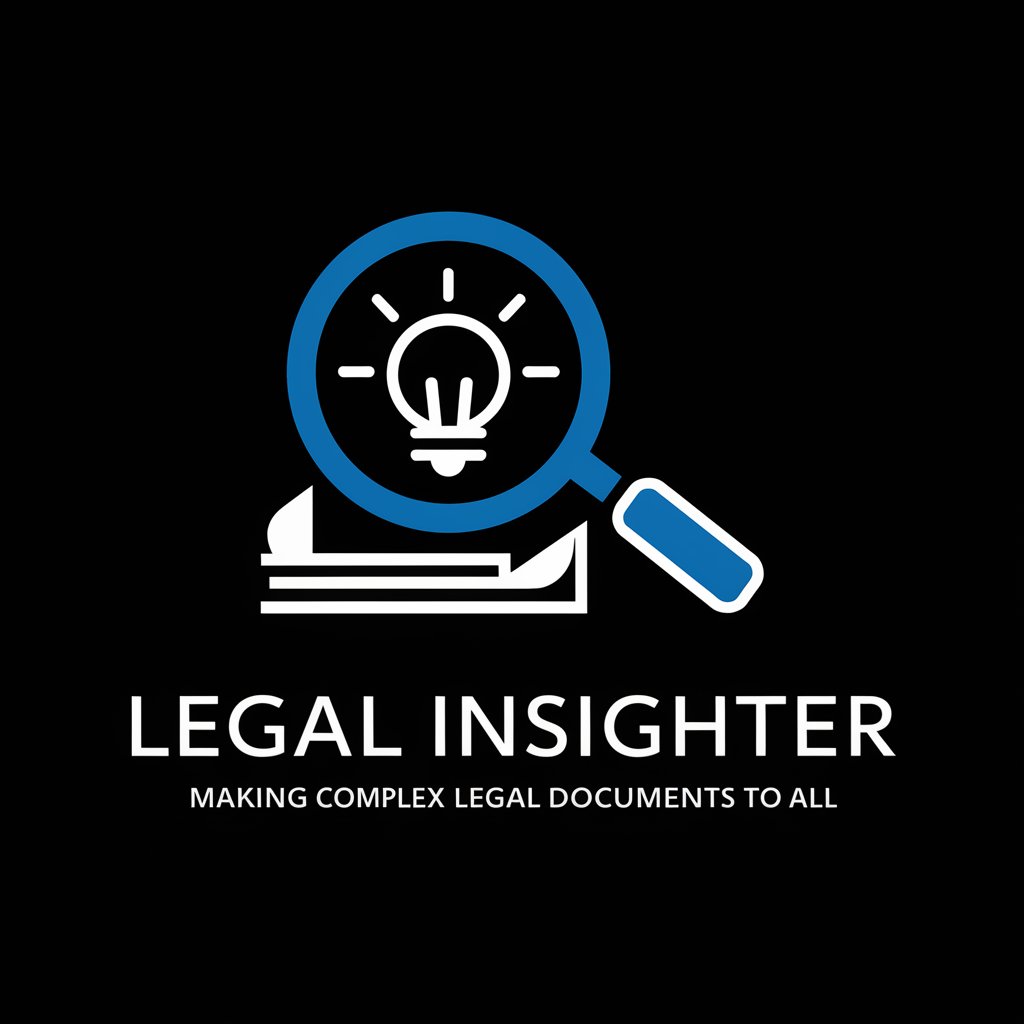
Summary Wizard
AI-Powered Precision Summarization
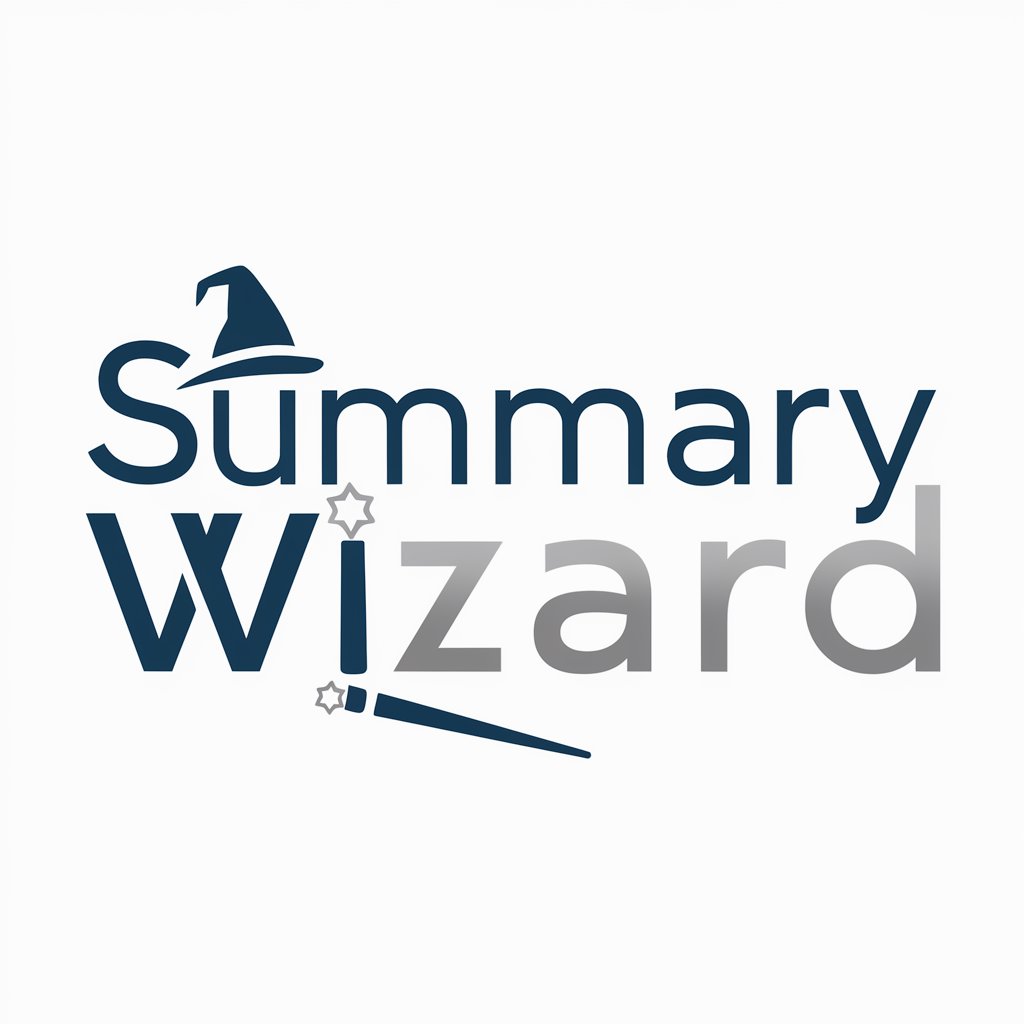
Manuscript Creator
Bringing Stories to Life with AI
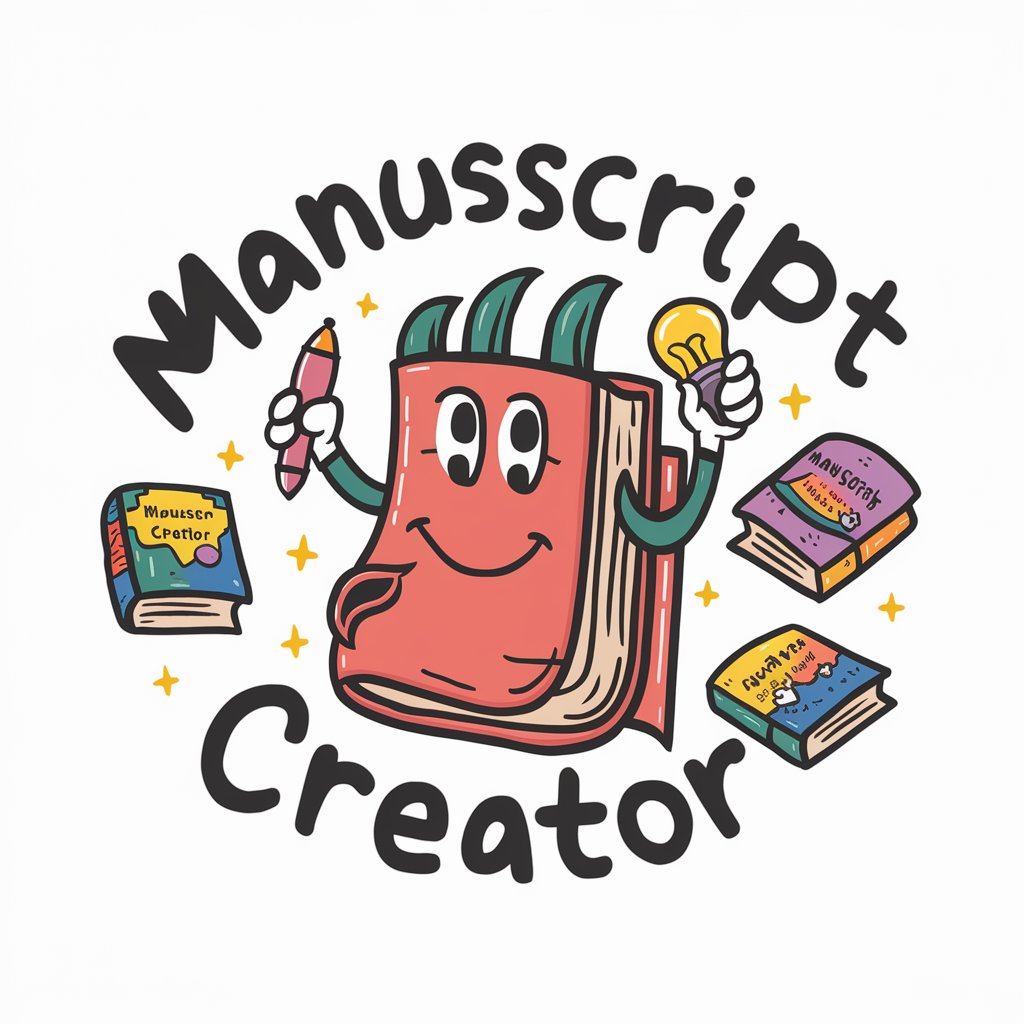
Trust Evaluator
Empowering Trust in Real Estate with AI
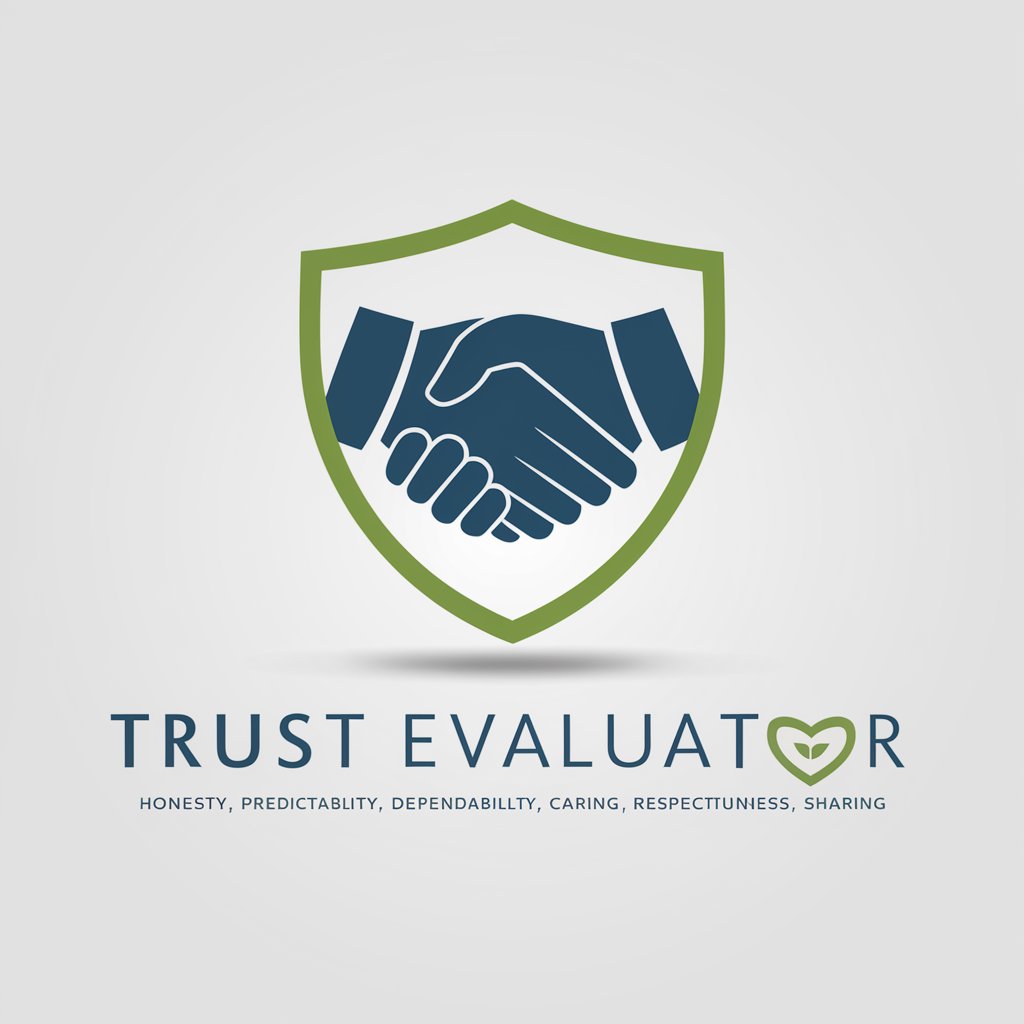
Series Selector
Tailoring TV to Your Taste with AI
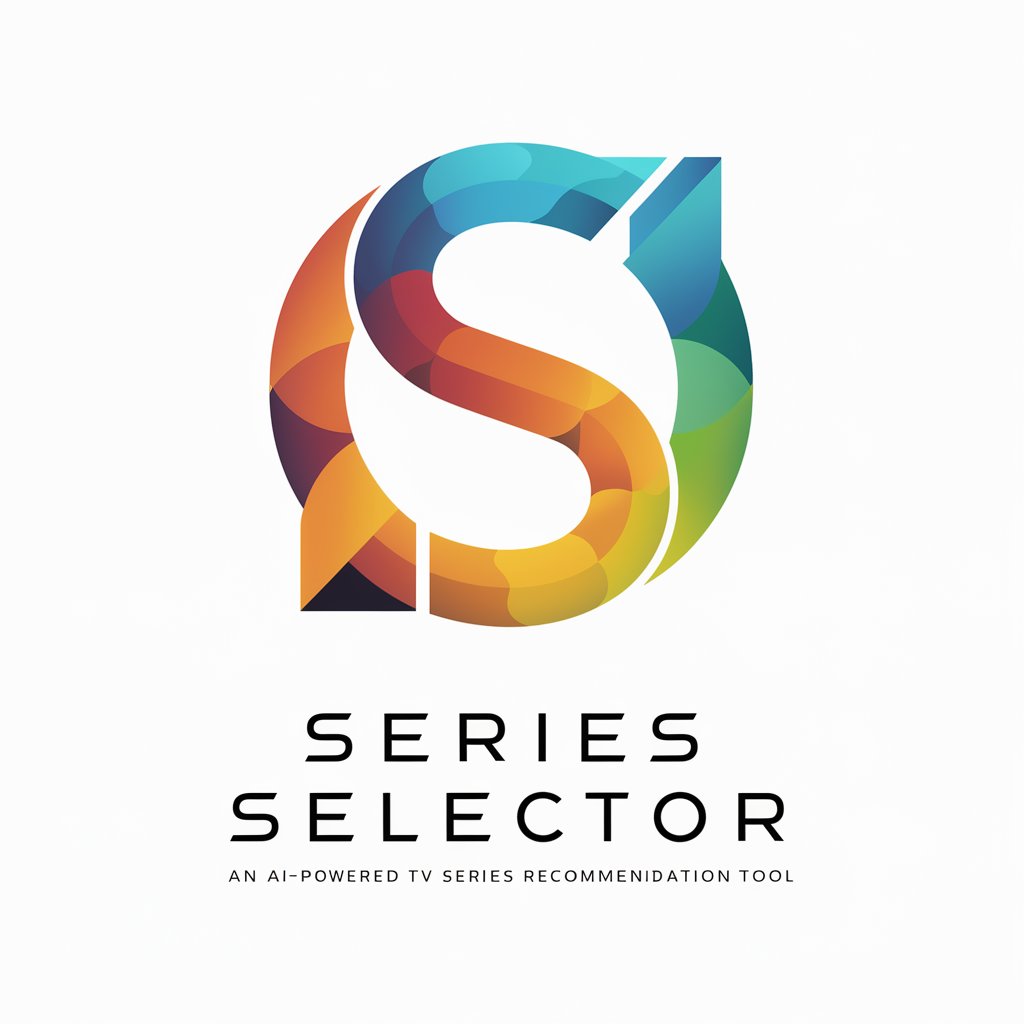
Kubernetes Copilot
Optimizing Kubernetes with AI Power
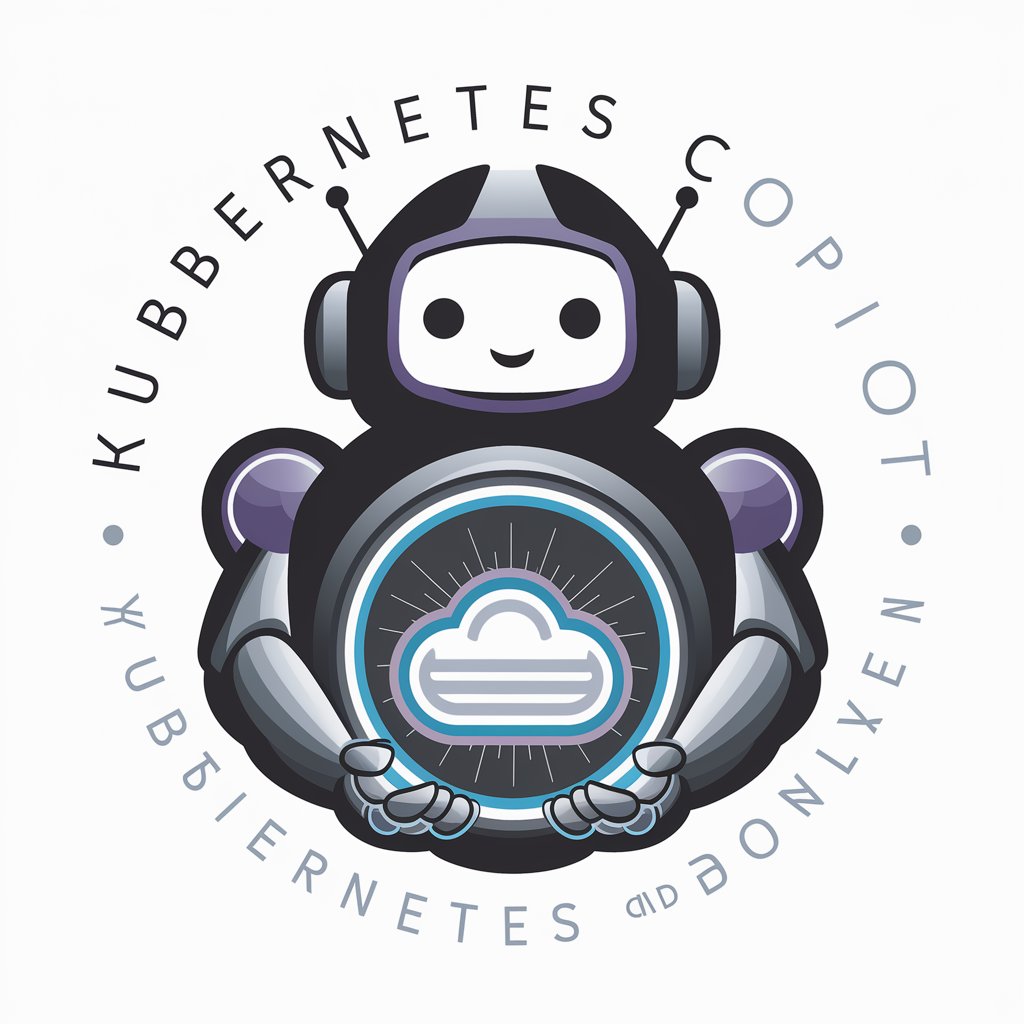
Scientific Associate
Empowering research with AI-driven insights.
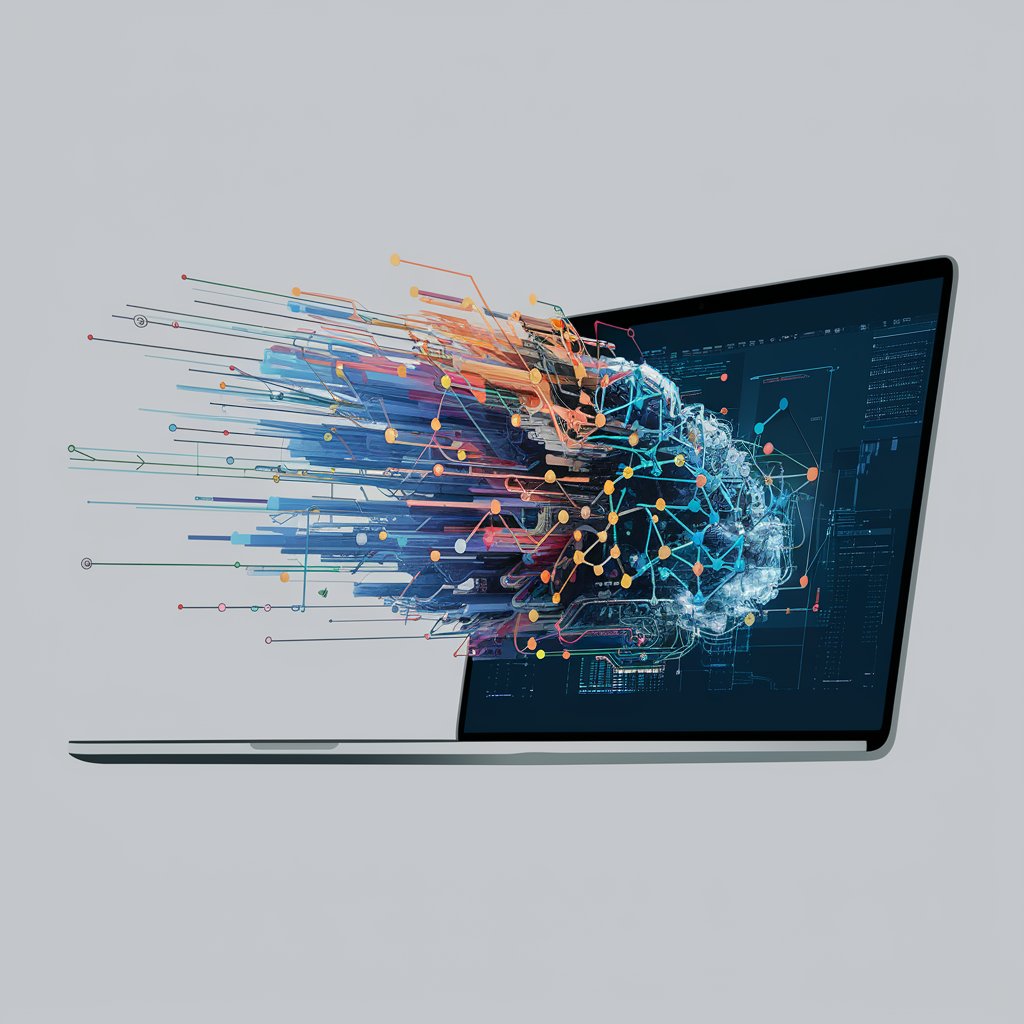
Entertain Meter
Boost Your Content's Entertainment Value
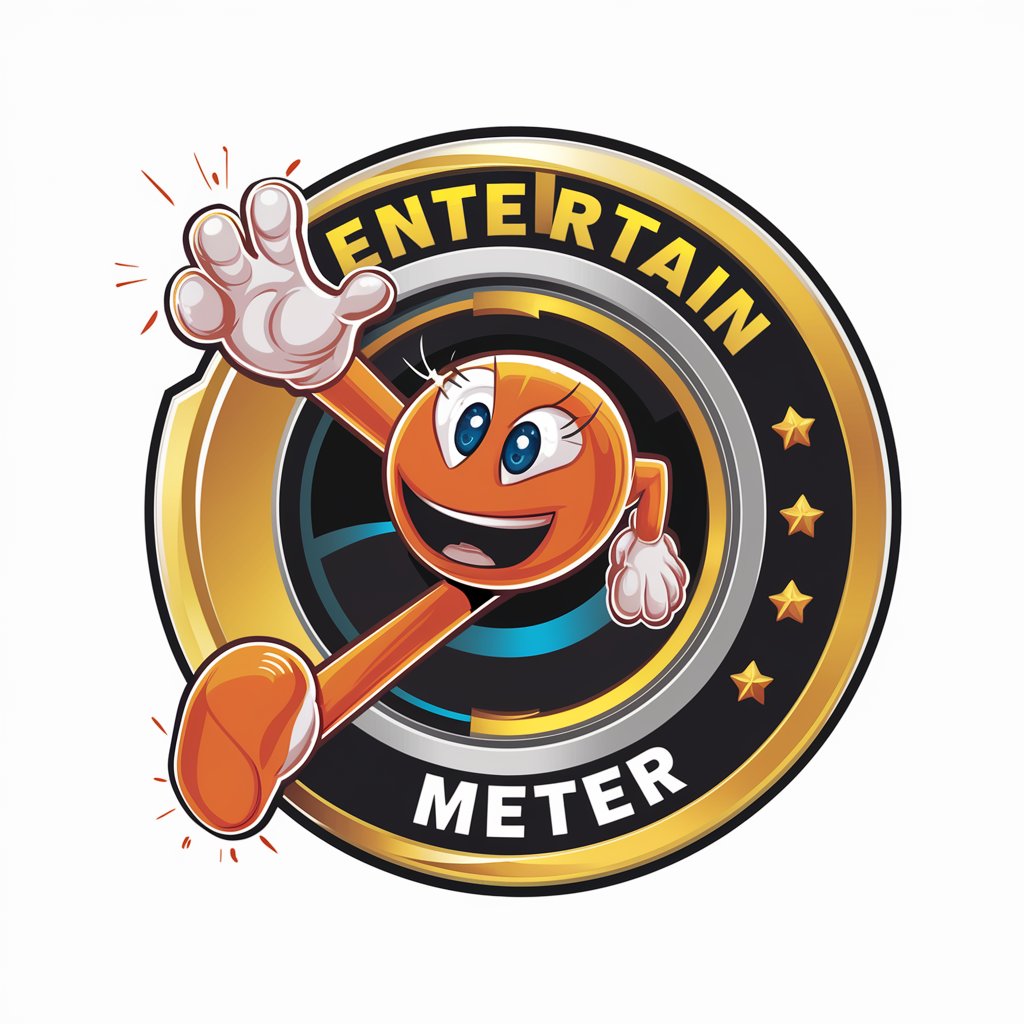
Code Master
Empowering your code with AI
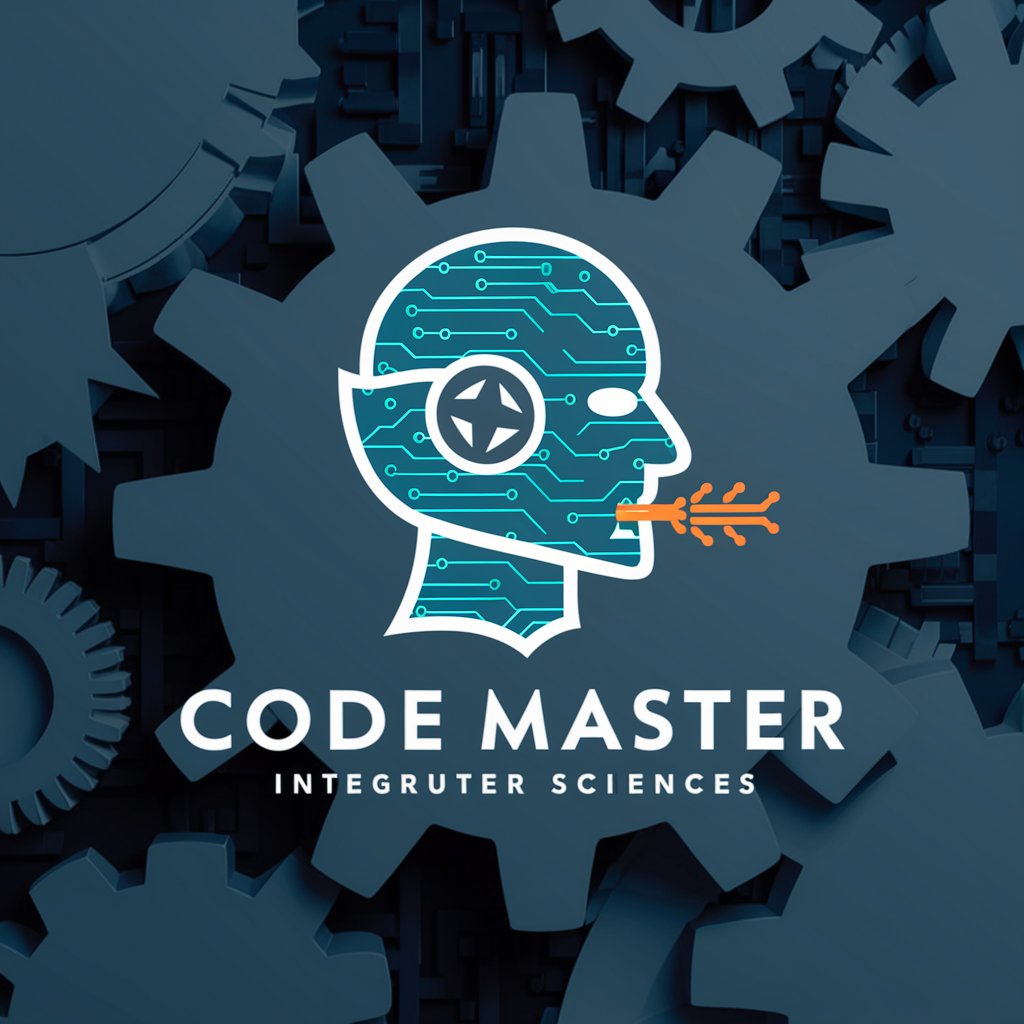
Frequently Asked Questions about Python Debugger
What is a Python debugger?
A Python debugger is a tool designed for analyzing Python code. It allows developers to execute code step by step, inspect variables, and identify issues or bugs.
How do I set a breakpoint in Python code?
You can set a breakpoint by adding 'import pdb; pdb.set_trace()' at the desired point in your script. This will pause execution at that line, activating the debugger.
Can I use the Python debugger in Jupyter notebooks?
Yes, debuggers like ipdb can be used in Jupyter notebooks. You can activate the debugger in a notebook cell by including the command '%debug' after encountering an exception.
Is it possible to debug Python scripts without modifying the code?
Yes, by using external debugging tools or IDEs with built-in debugging features, you can set breakpoints and debug scripts without altering the source code directly.
What are some common commands used in Python debugging?
Common debugging commands include 'l' to list code, 'n' to go to the next line, 'c' to continue execution until the next breakpoint, and 'q' to quit the debugger.