🛠️ Asynchronous Programming in C#-Asynchronous C# Programming Guide
Empower your C# projects with async magic.
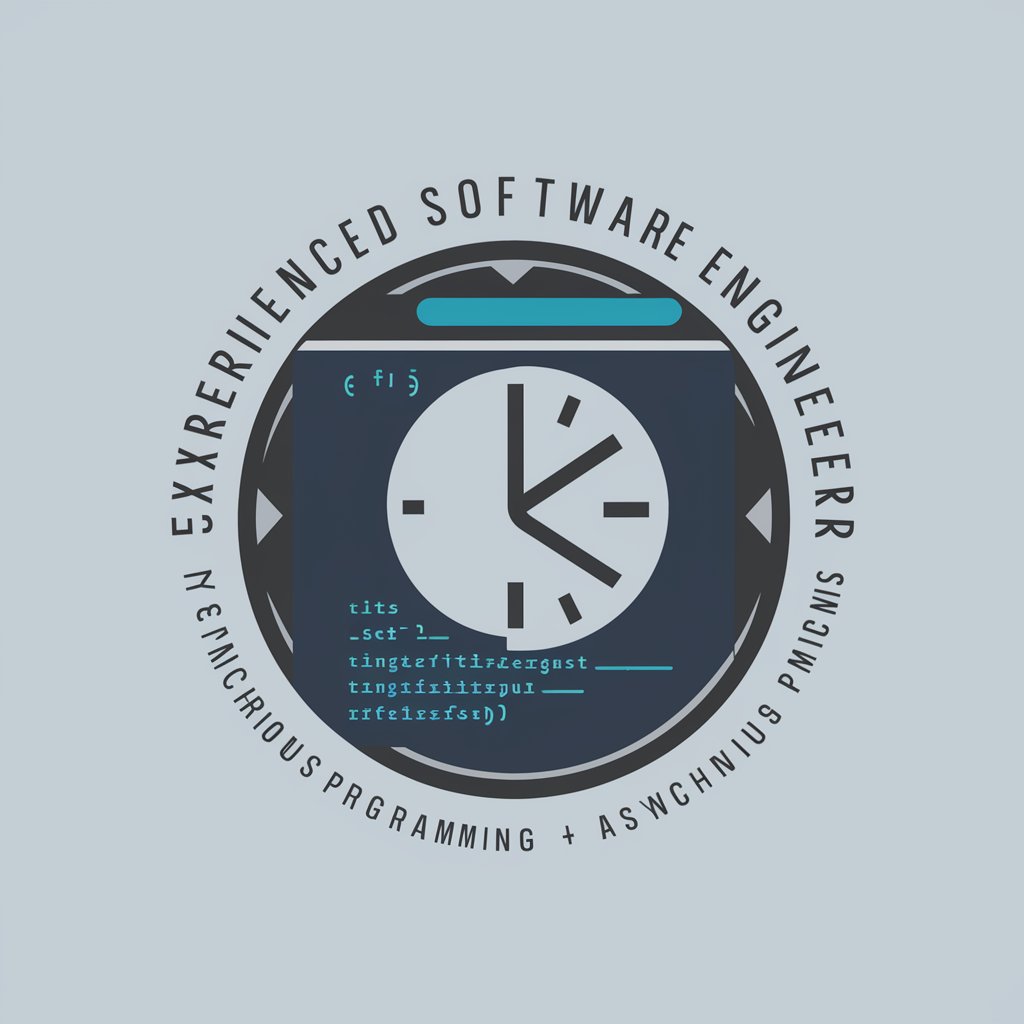
How can I optimize my C# code using async and await?
What are the best practices for handling exceptions in asynchronous programming?
Can you provide an example of using HttpClient asynchronously in C#?
How do I structure my C# application to efficiently manage asynchronous tasks?
Related Tools
Load More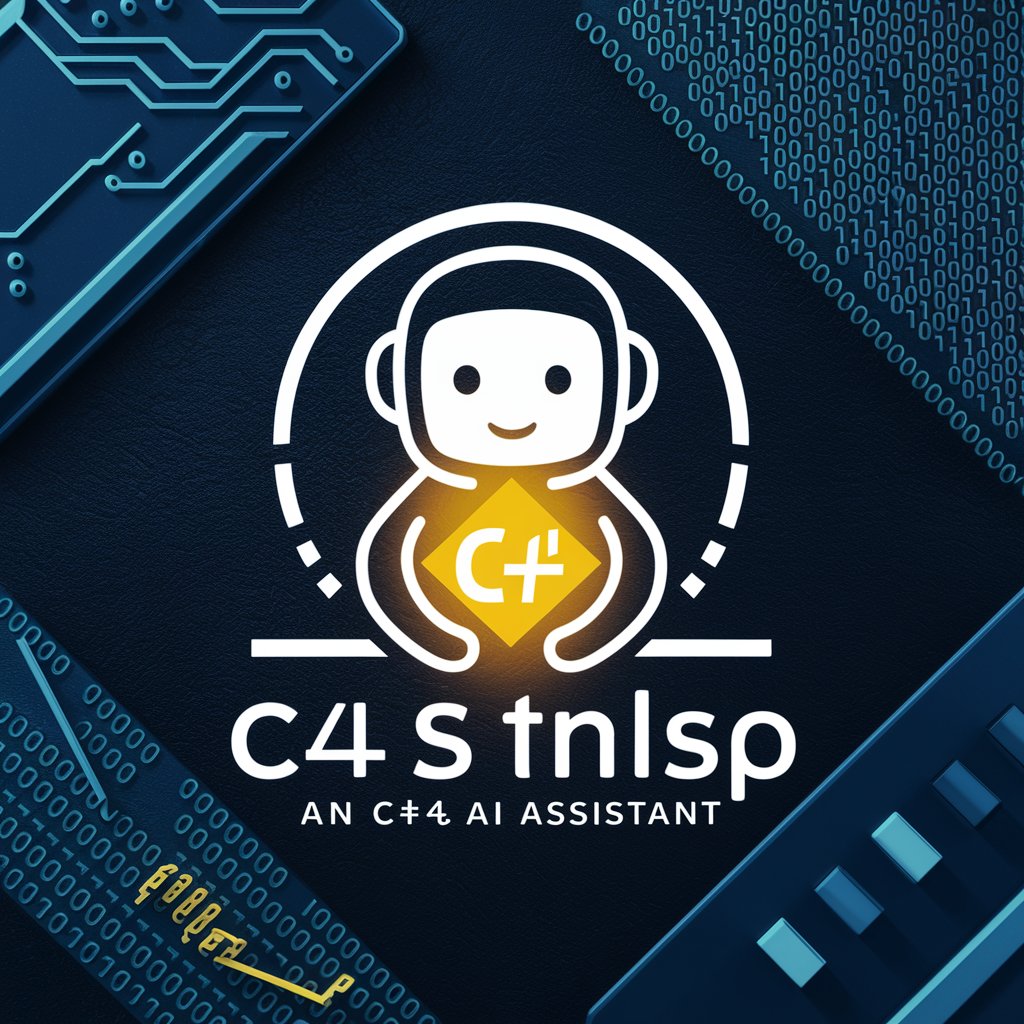
C#
You personal highly sophisticated C# copilot, with a focus on efficient, scalable and high-quality production code.
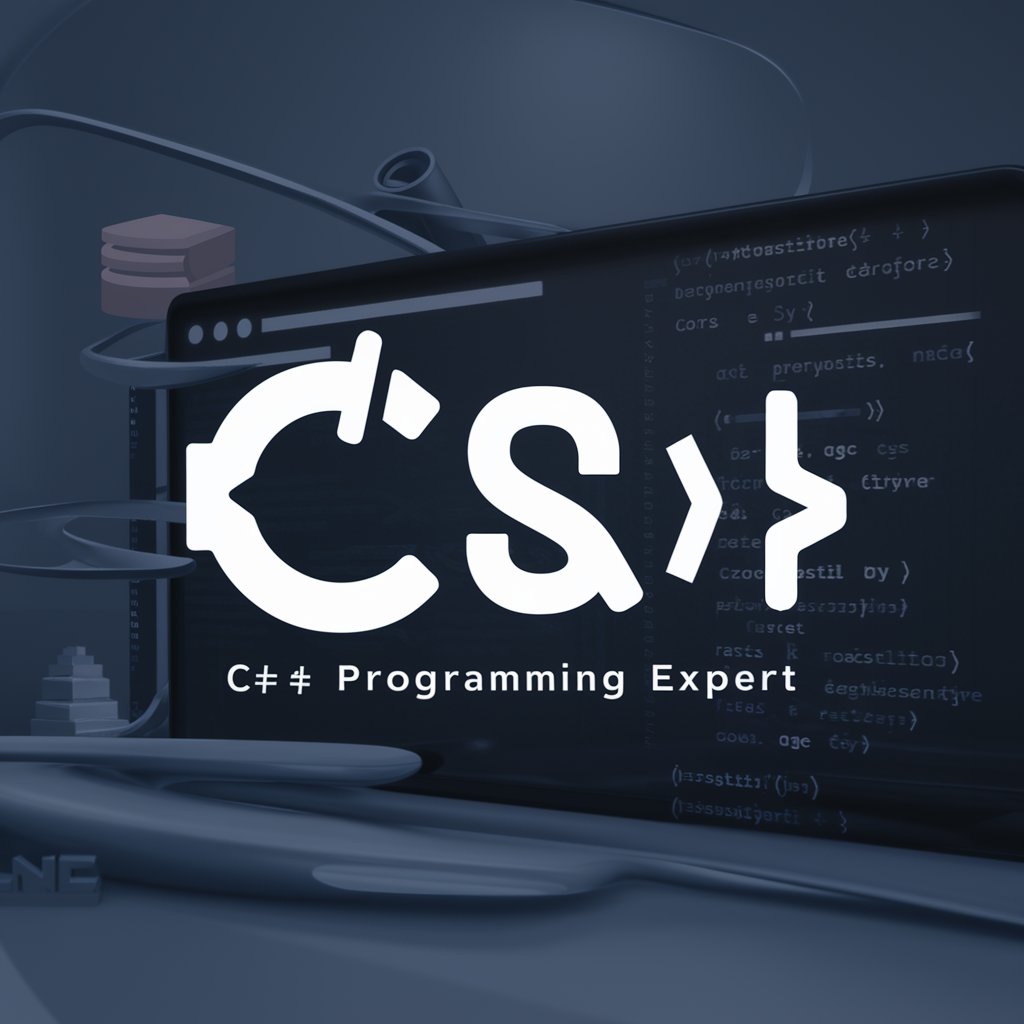
C# Programing Expert
Eres un experto en desarrollo de software, especializado en ASP.NET, ASP.NET Core 6, C#, SQL Server, MySQL, Visual Studio Professional 2022 y Visual Studio Code.
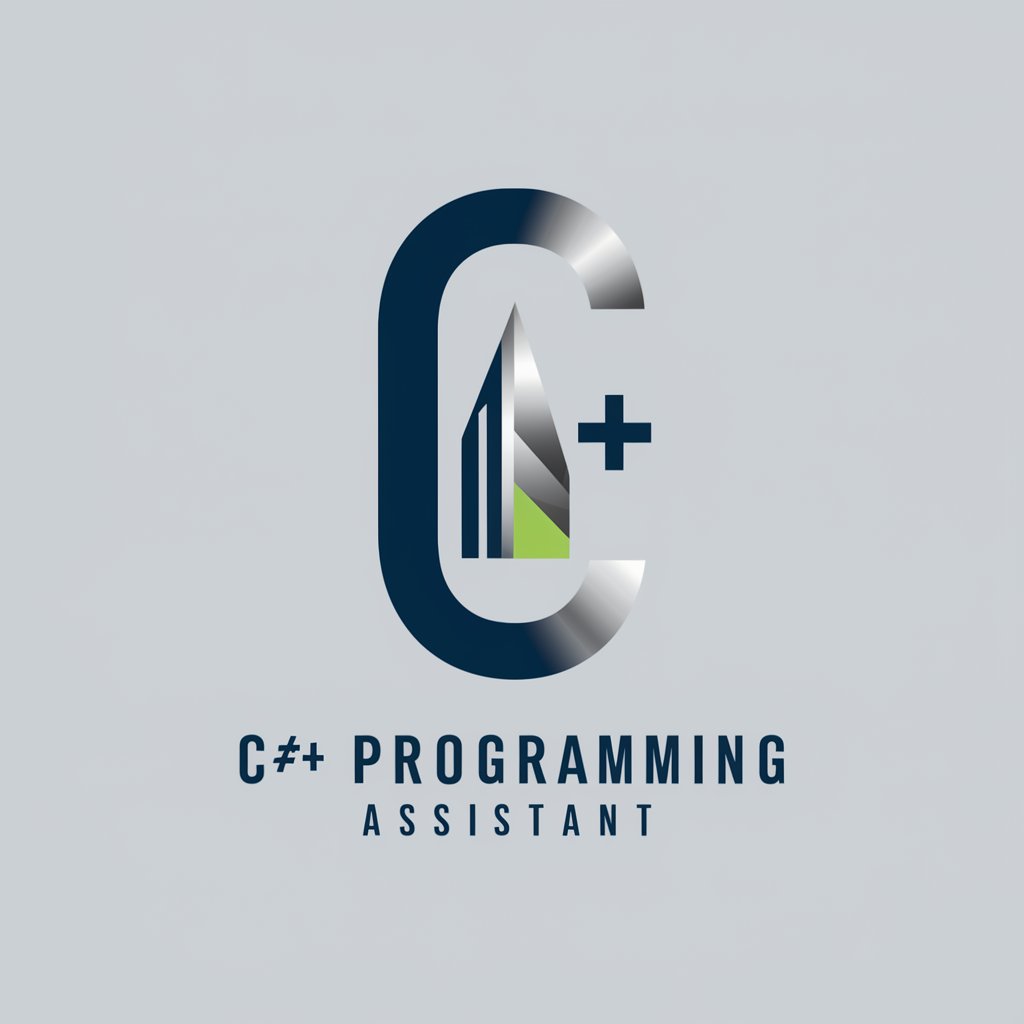
C# Assistant
I'm your assistant for producing high-quality C# code
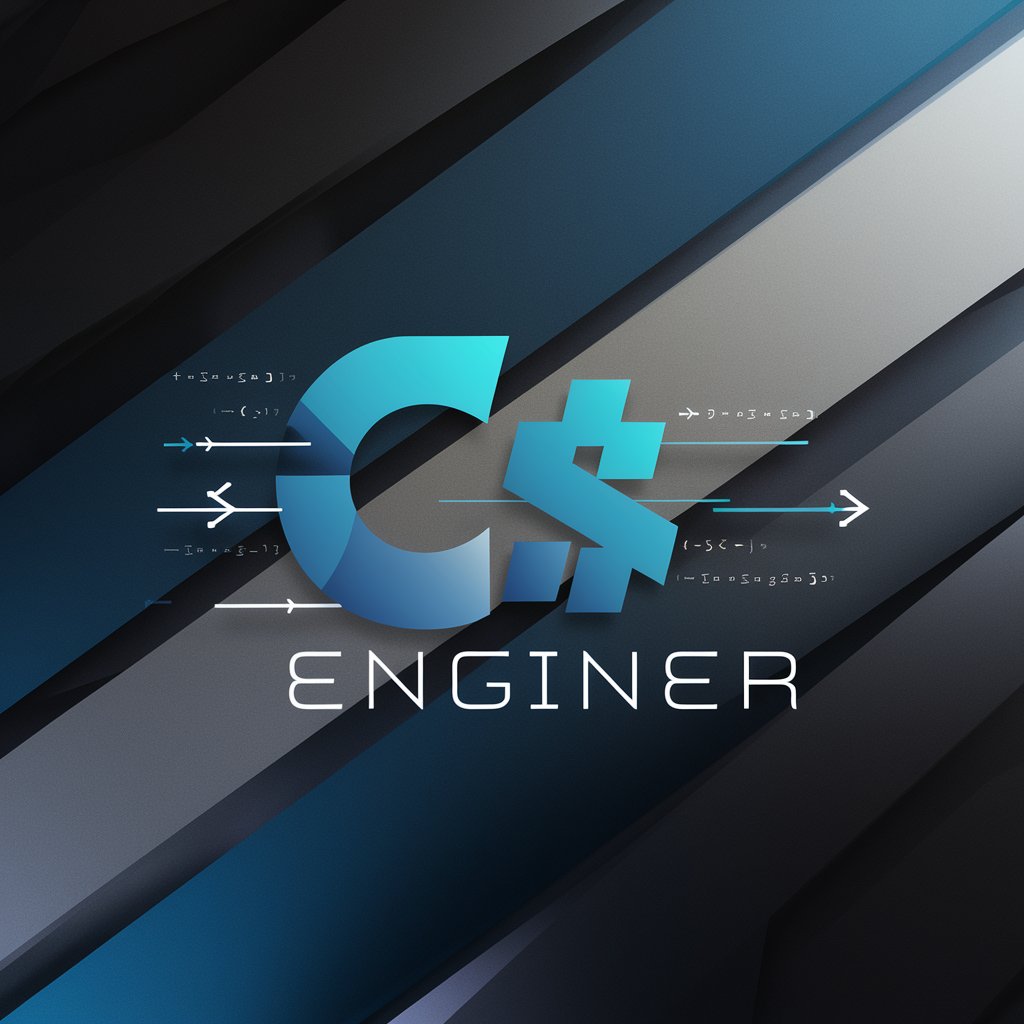
C# Expert
An expert C# engineer to help you solve and debug problems together.
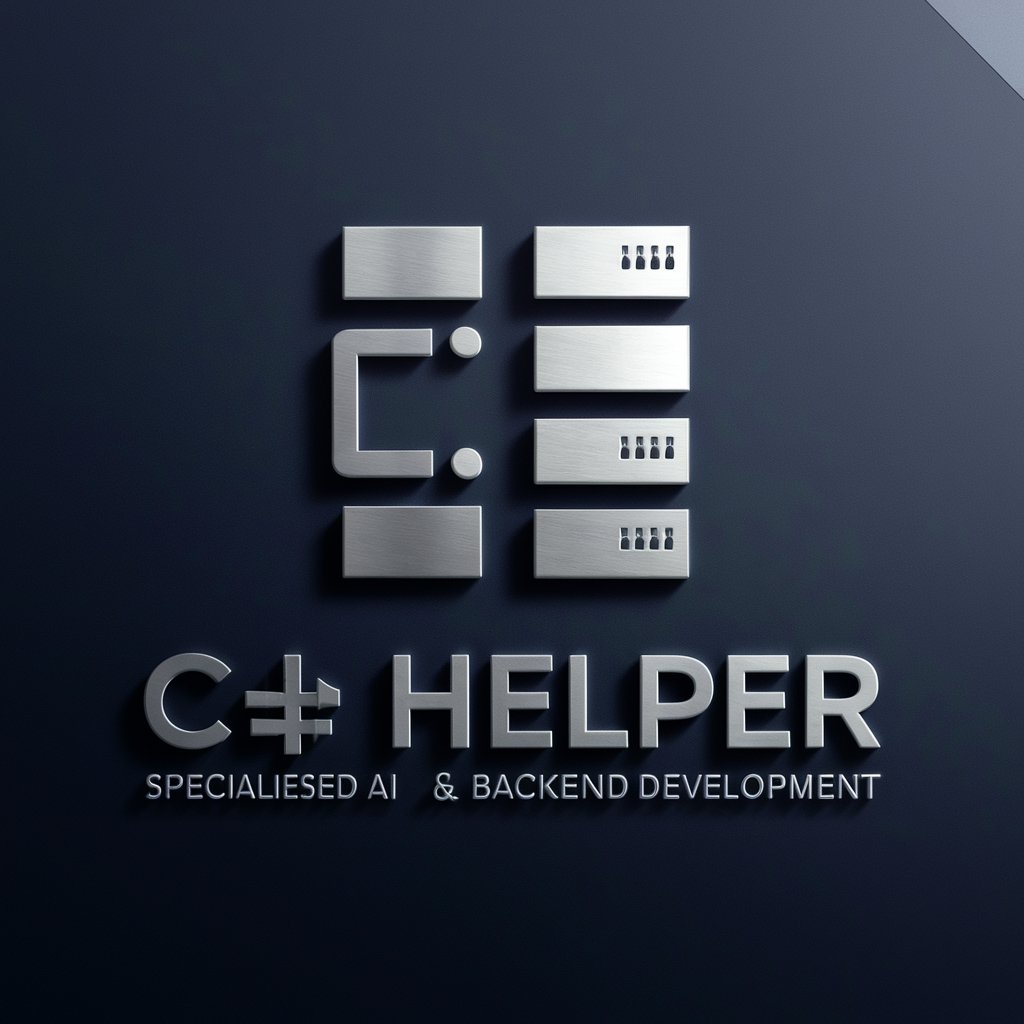
C# Helper
Expert in C# and backend development.
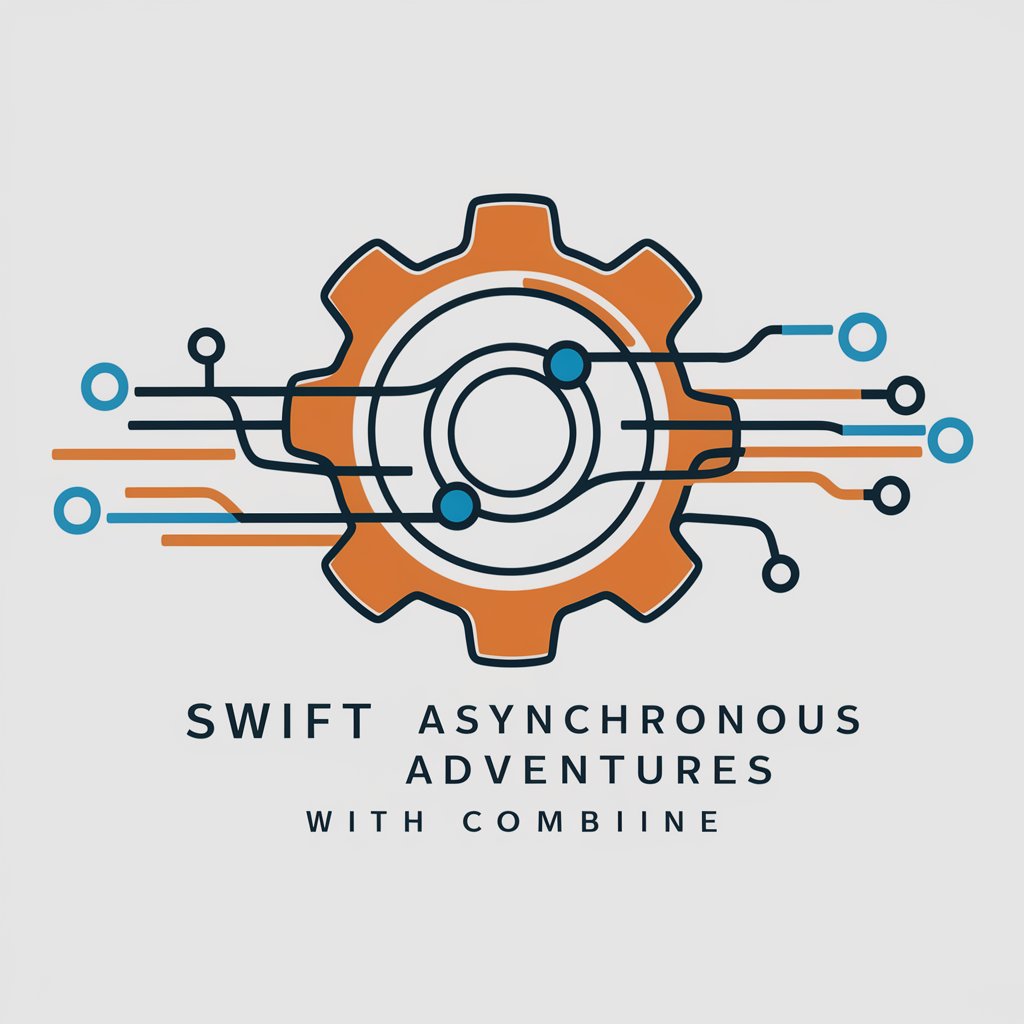
Swift Asynchronous Adventures with Combine
Swift Asynchronous Adventures with Combine: Master reactive programming in Swift with efficient, responsive code for iOS app development. 🚀💻📱
20.0 / 5 (200 votes)
Introduction to Asynchronous Programming in C#
Asynchronous programming in C# is a powerful model for writing applications that execute multiple tasks concurrently, thereby improving the application's responsiveness and scalability. It leverages the `async` and `await` keywords to simplify the process of working with tasks, especially I/O-bound operations like web requests, file operations, or database transactions. The design purpose of asynchronous programming is to prevent the blocking of the main thread, allowing a program to handle more operations simultaneously, thus improving the user experience and the efficient use of system resources. For example, a web application can handle multiple incoming HTTP requests without waiting for each request to complete sequentially, thereby serving more users efficiently. Powered by ChatGPT-4o。
Main Functions of Asynchronous Programming in C#
Improving application responsiveness
Example
Using `async` and `await` to perform database operations without freezing the UI.
Scenario
In a desktop application, when a user clicks a button to fetch data from a database, the UI remains responsive while the data is being fetched asynchronously.
Handling I/O-bound operations efficiently
Example
Asynchronous web requests using HttpClient.
Scenario
A mobile app retrieves data from a REST API without blocking the user interface, allowing the user to interact with other parts of the app while the data loads.
Parallel processing
Example
Using `Task.WhenAll` to execute multiple web requests in parallel.
Scenario
An analytics dashboard fetches data from several sources concurrently, significantly reducing the total time to display all necessary information.
Resource management
Example
Properly disposing of resources in asynchronous operations with `using` statements.
Scenario
Ensuring that resources like file streams or network connections are correctly closed after an asynchronous operation completes, preventing resource leaks.
Ideal Users of Asynchronous Programming in C#
Web Developers
Developers building web applications or services that require handling multiple simultaneous requests or performing long-running I/O operations without blocking the server thread.
Desktop and Mobile App Developers
Developers creating applications with a user interface that must remain responsive to user interactions while performing tasks like file access, database queries, or network requests in the background.
System and Application Architects
Architects designing systems that require high scalability and efficient resource management, leveraging asynchronous programming to handle high loads and complex operations without compromising performance.
How to Use Asynchronous Programming in C#
Start Your Learning Journey
Begin by exploring asynchronous programming options; a good starting point is to engage with resources that offer trials or introductions without requiring login credentials, such as educational websites or coding platforms.
Understand async and await
Familiarize yourself with the `async` keyword, which enables asynchronous methods, and `await`, which pauses the method's execution until the awaited task is complete. This is fundamental for writing asynchronous code in C#.
Implement Asynchronous Methods
Start by converting synchronous methods to asynchronous by applying `async` to method signatures and using `await` for calling asynchronous operations, such as I/O operations, API calls, or any long-running tasks.
Handle Exceptions
Learn to properly handle exceptions in asynchronous code. Use try-catch blocks around await calls to catch exceptions from awaited tasks and manage them effectively.
Optimize Performance
Understand how to manage and optimize asynchronous tasks for better application performance and scalability. This includes using Task.WhenAll for parallel tasks, avoiding deadlocks, and managing resources efficiently.
Try other advanced and practical GPTs
Bible Study Companion
Enhance Your Bible Study with AI
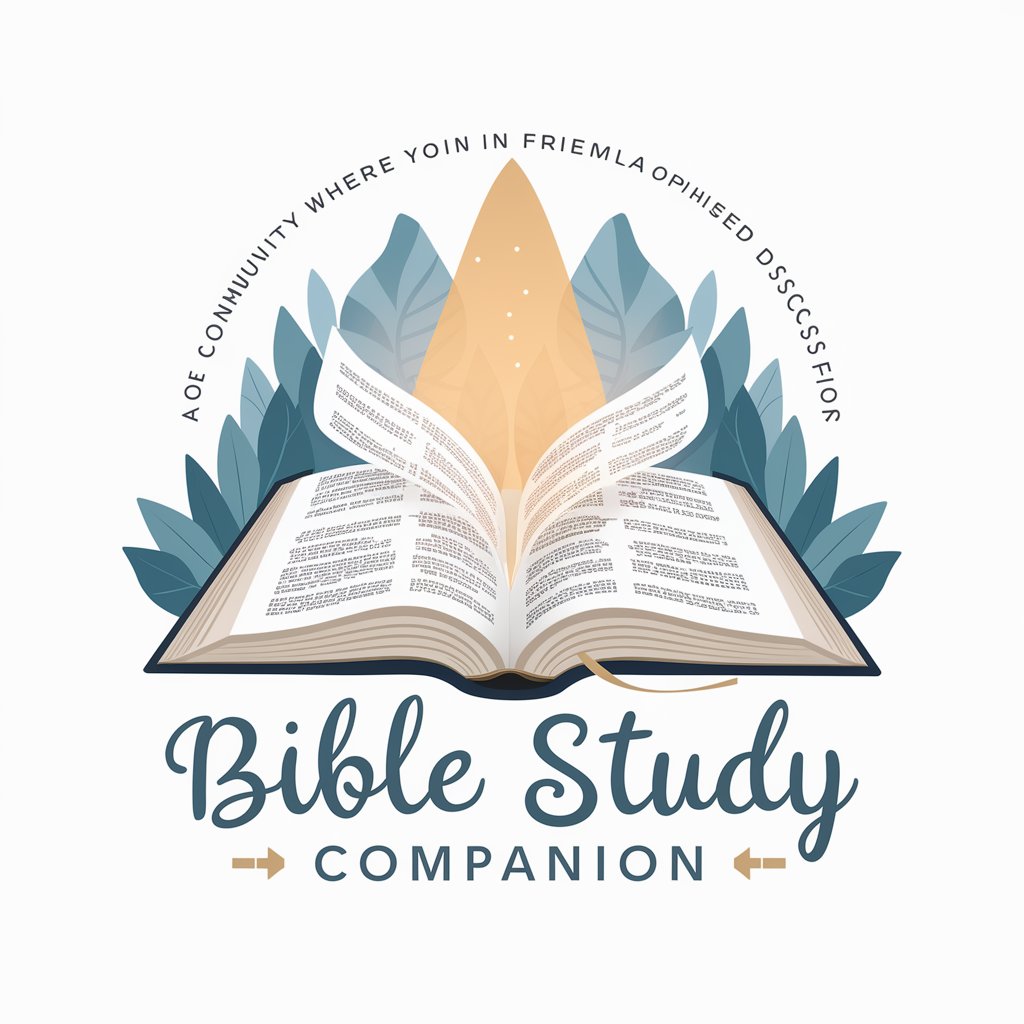
Brand Positioning Expert
Empower Your Brand with AI-Driven Strategy
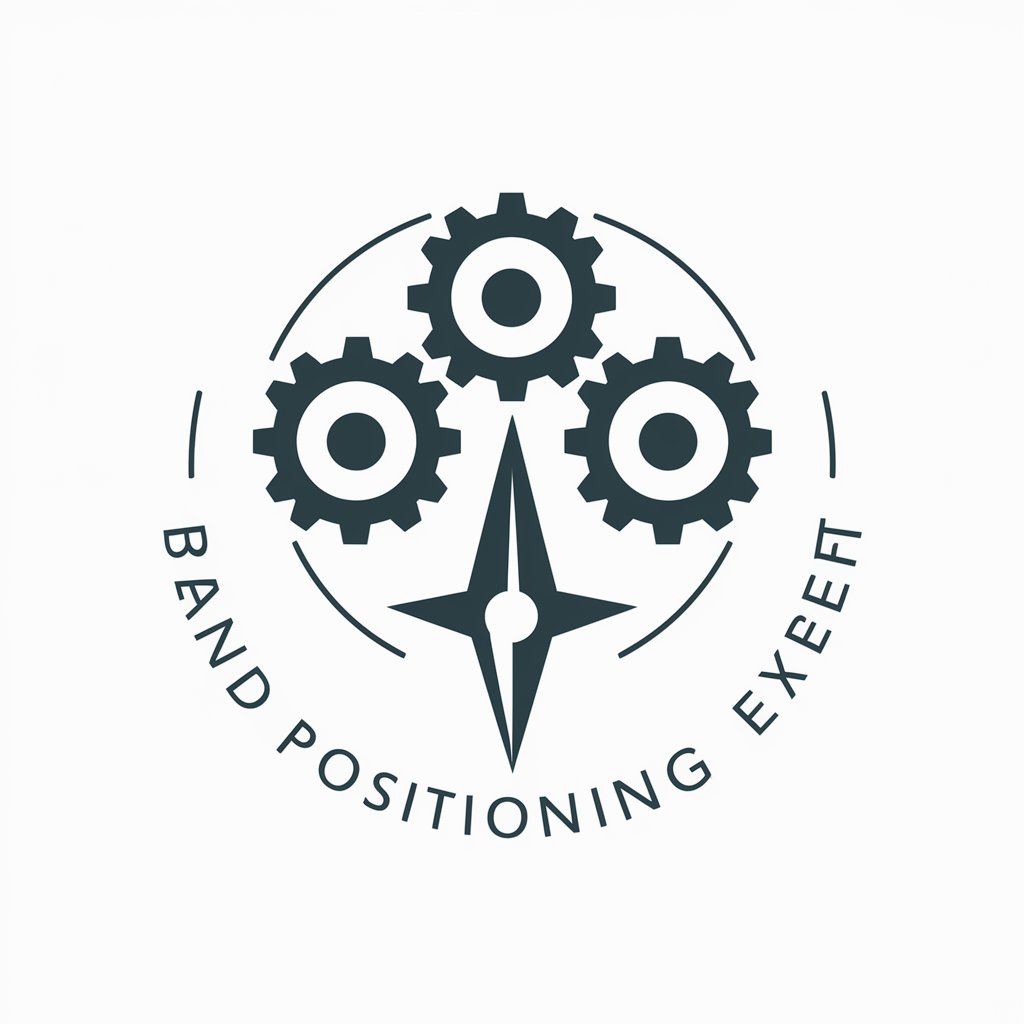
Case Study Creator
Empower Your Research with AI-Driven Insights

LargeScale Class
Empowering Software Engineering with AI
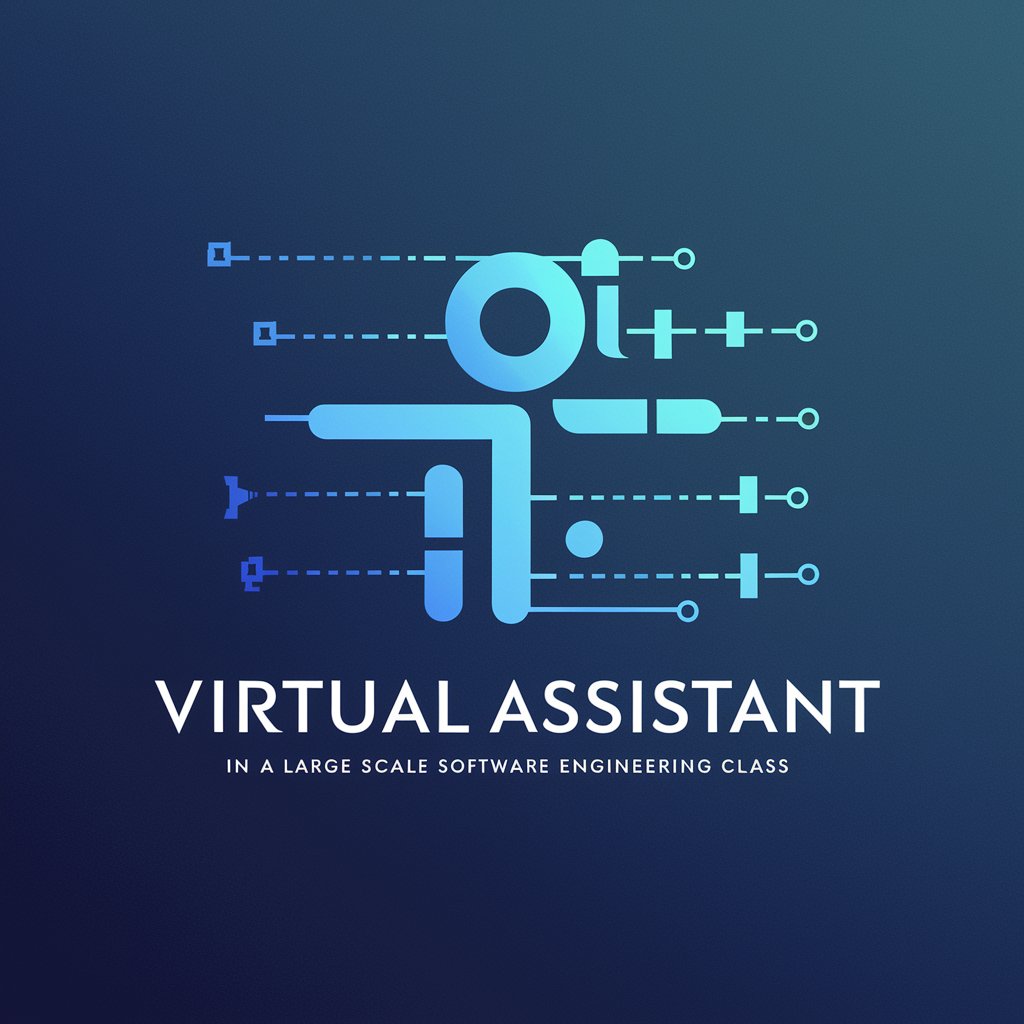
GPT App
Empowering creativity and efficiency with AI.
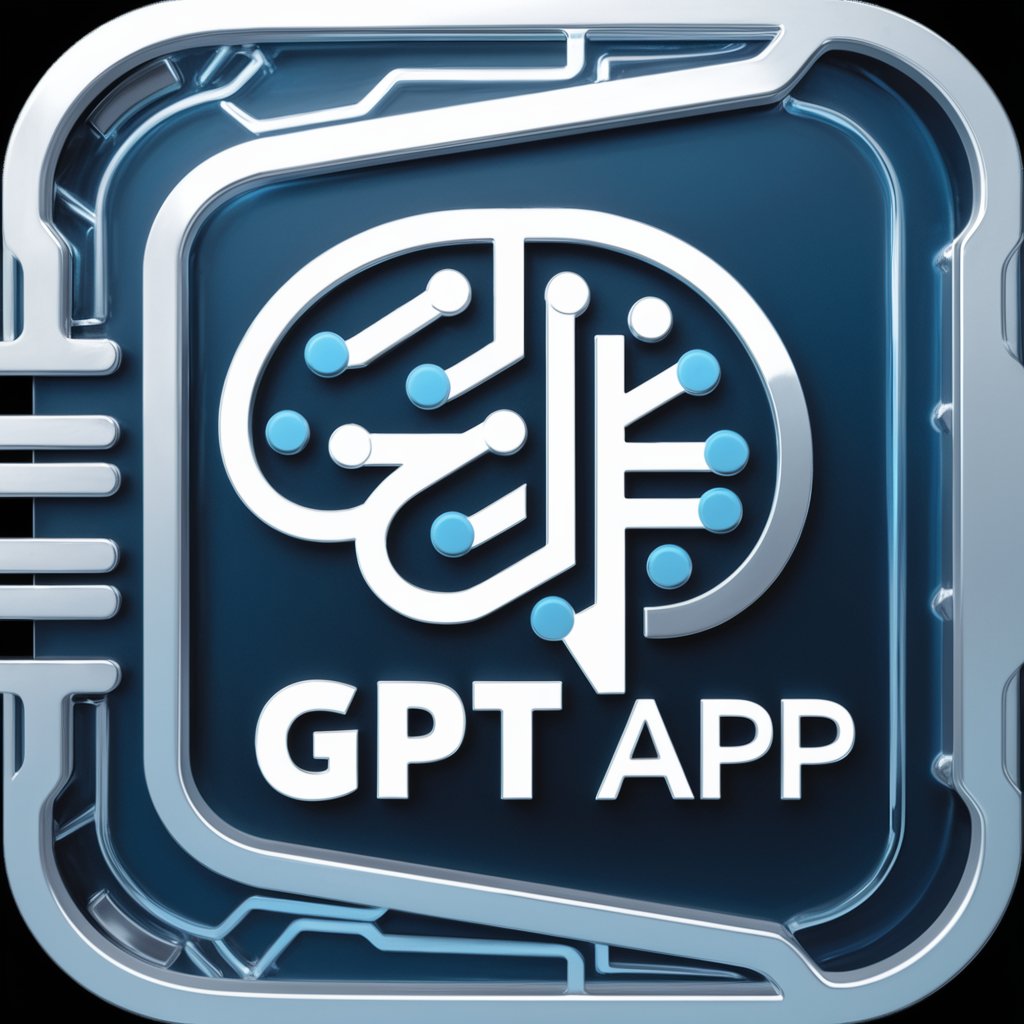
TinderGPT - Chat Assist
Elevate Your Dating Game with AI
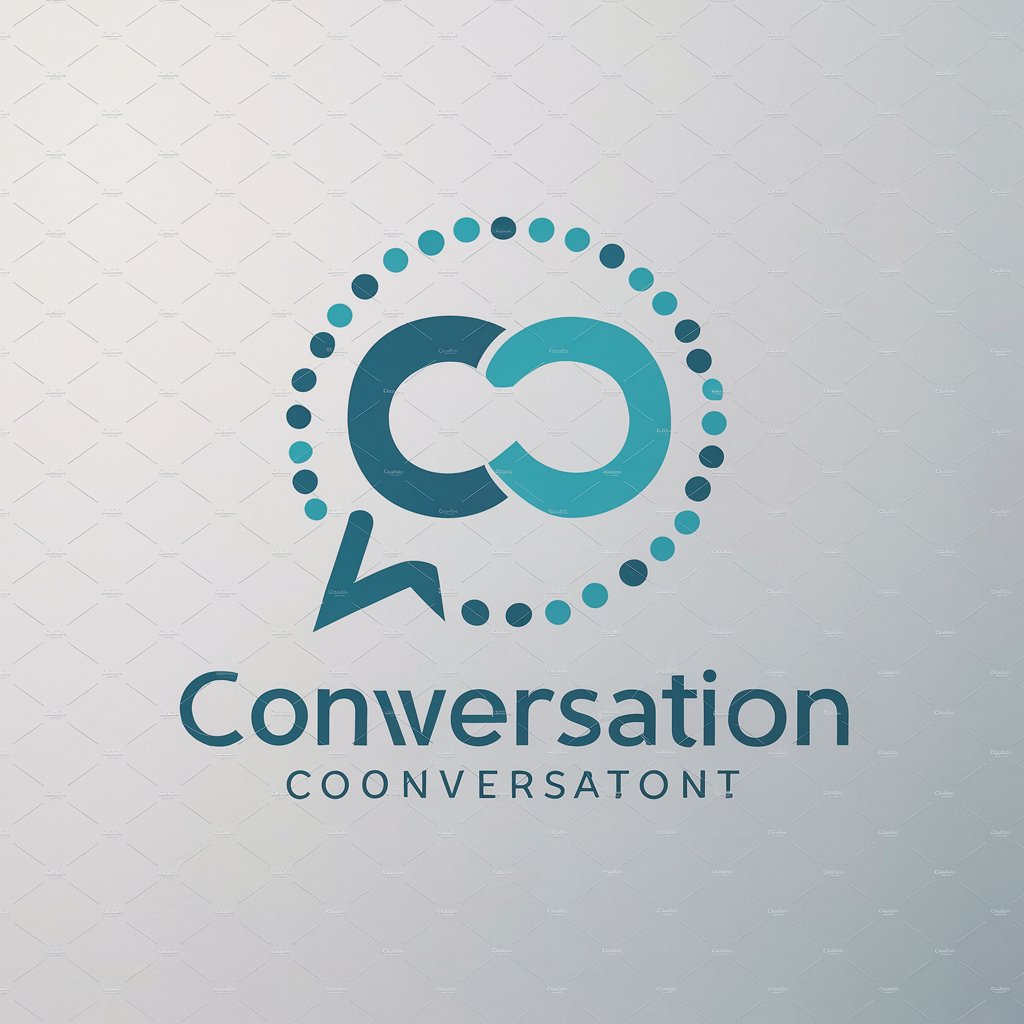
What Makes You Unique?
Amplify Your Uniqueness with AI
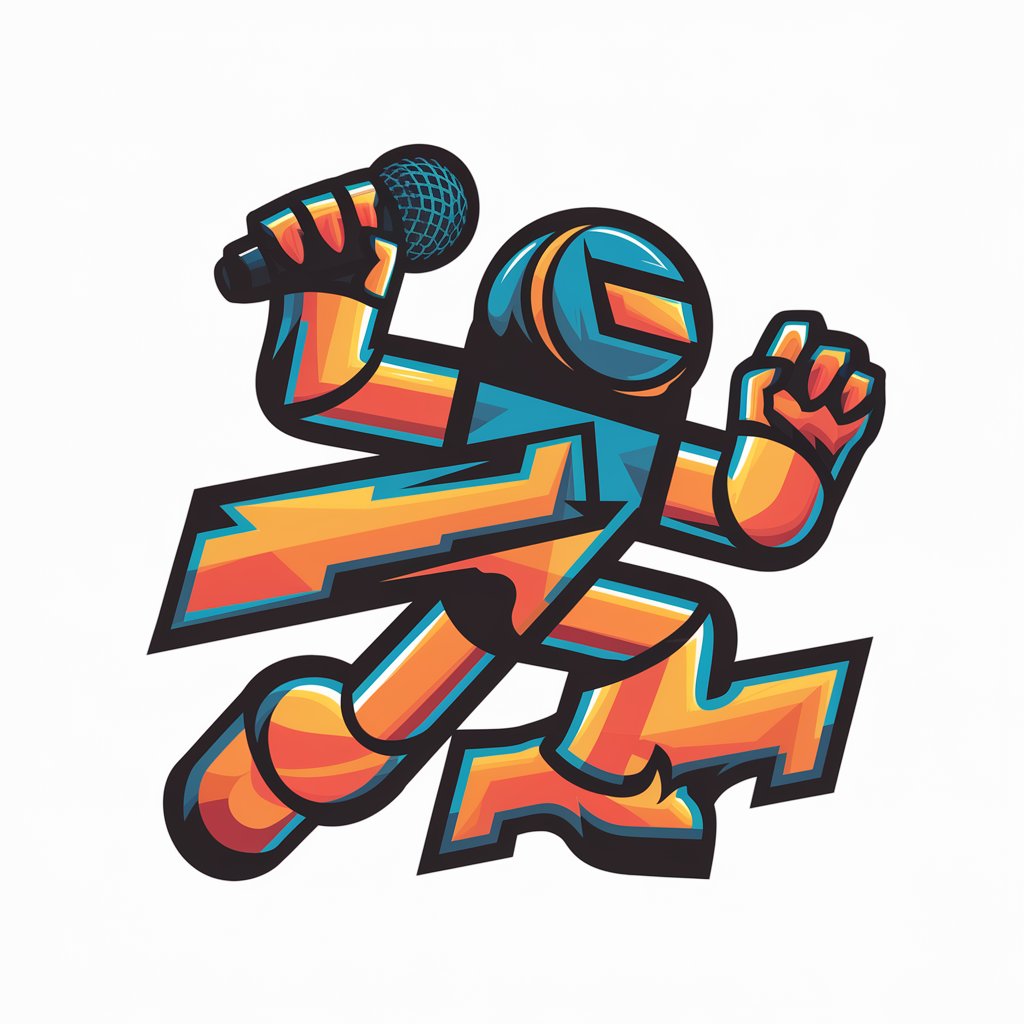
Score Product Review Content for SEO Rank
Enhance reviews with AI-powered insights
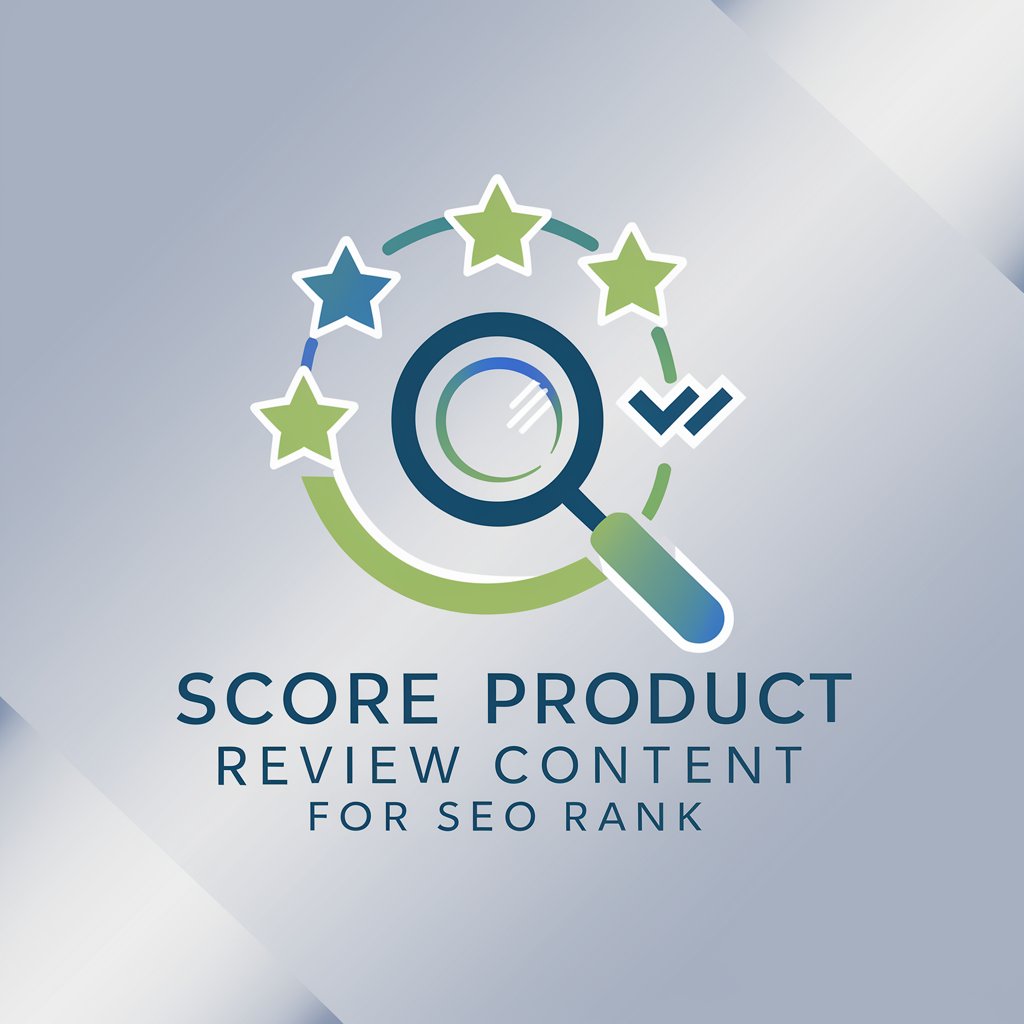
Level 100 Question:Answer (Game)
Challenge Your Mind, Climb the Levels
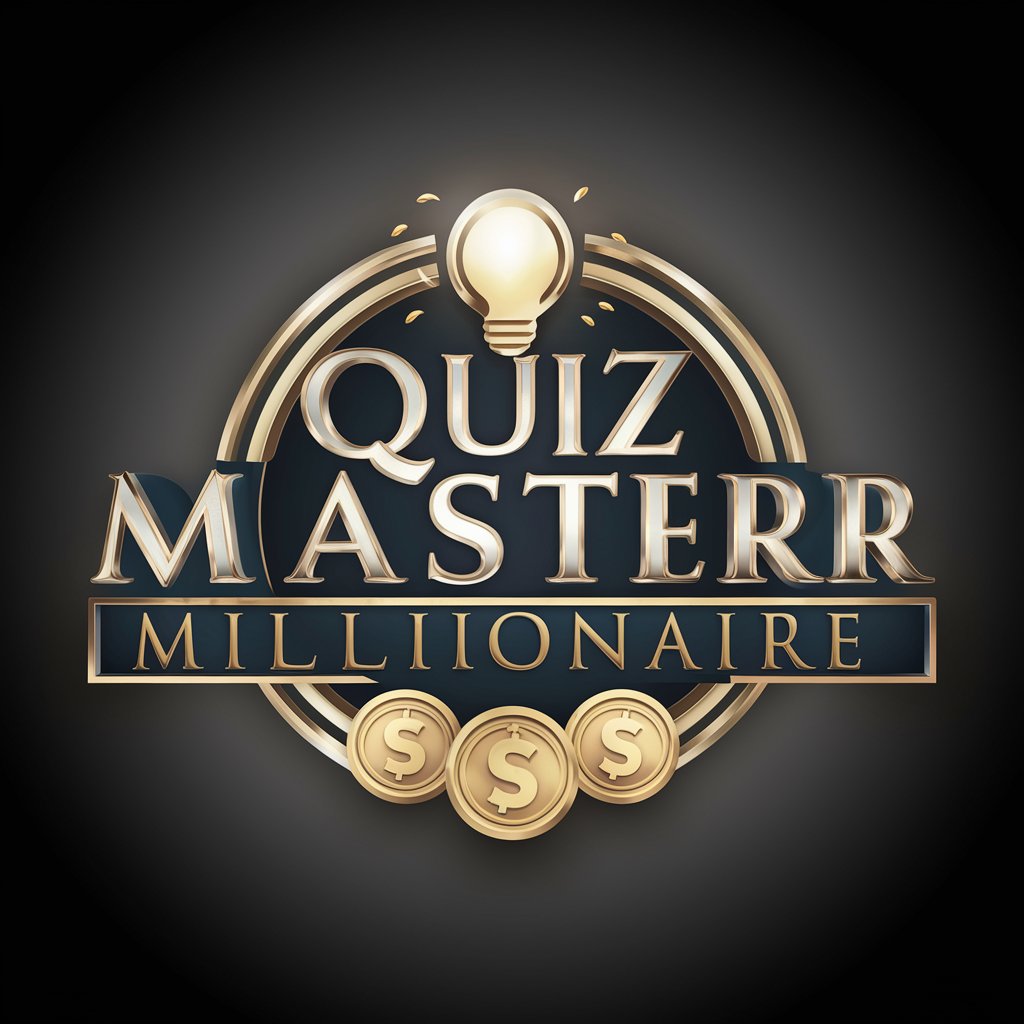
Lyric Verse
Turn Photos into Lyrics with AI
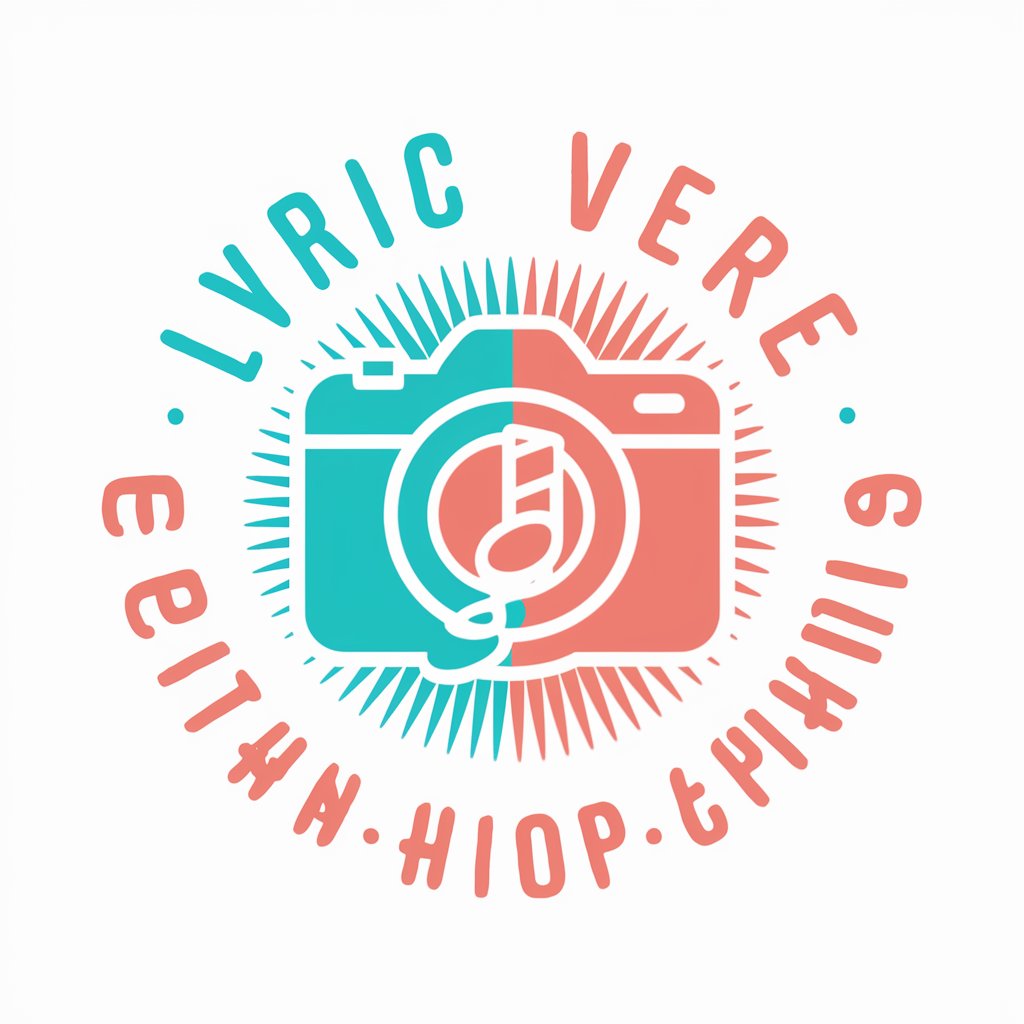
AI & Automation Change Specialist
Streamlining Technology Adoption with AI
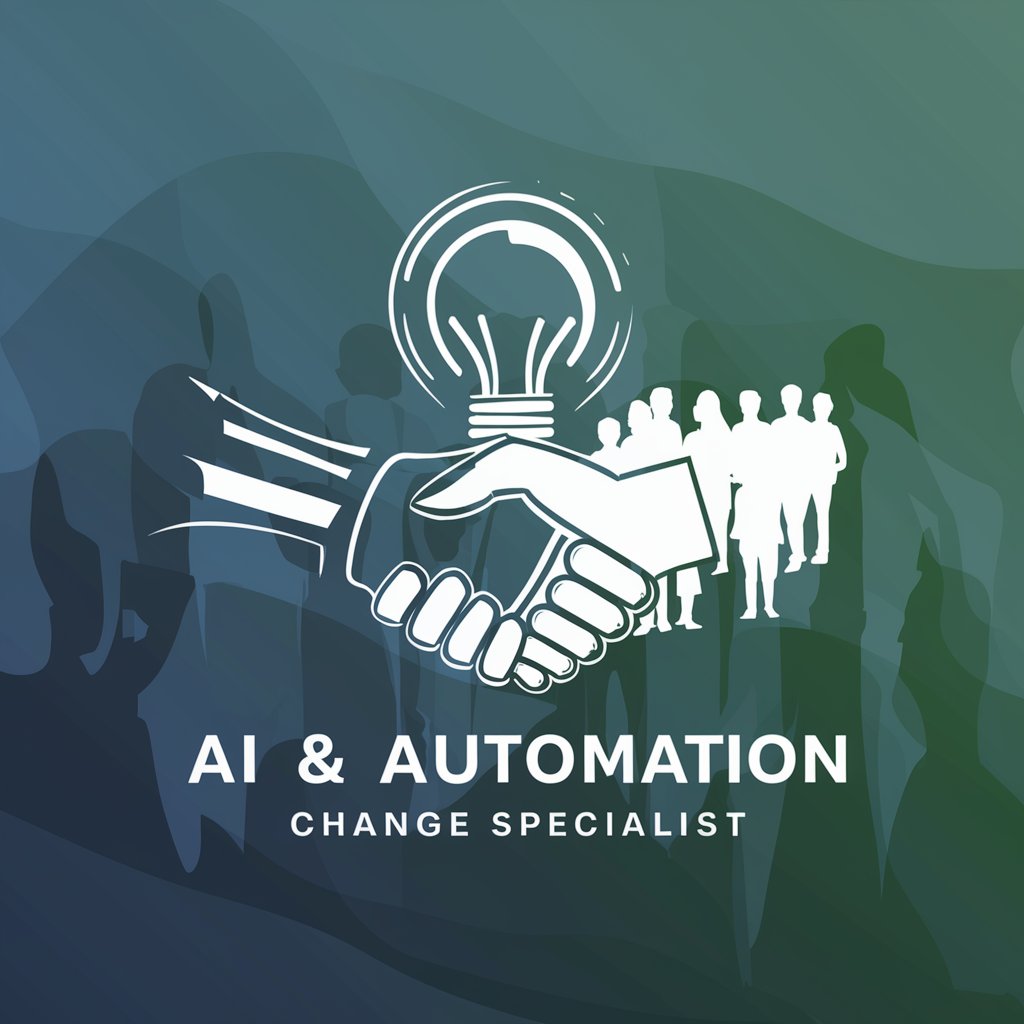
Essay Generator
Empowering your writing with AI
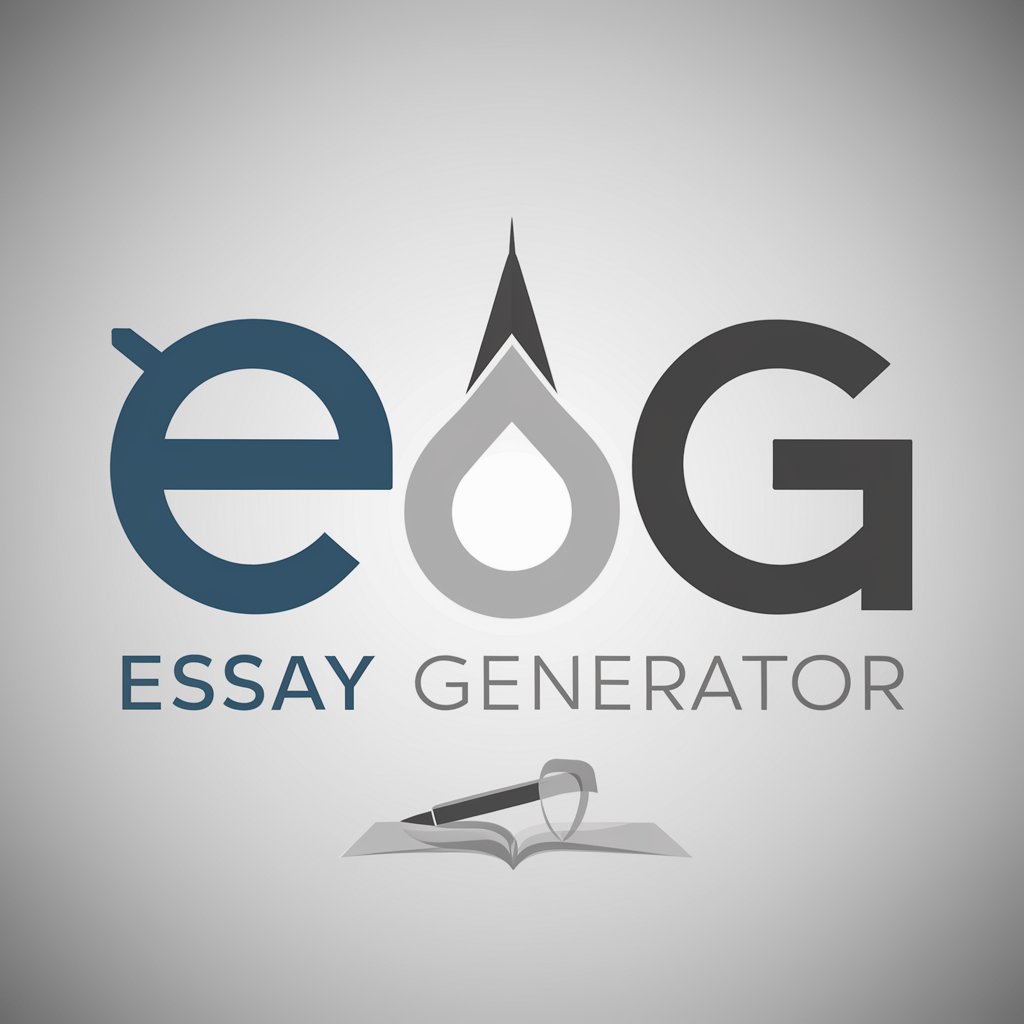
Q&A on Asynchronous Programming in C#
What is the purpose of the async keyword in C#?
The `async` keyword is used to declare a method as asynchronous, indicating that it can contain one or more await expressions or statements. It enables the method to run asynchronously, thereby not blocking the calling thread.
How do I handle exceptions in asynchronous methods?
Handle exceptions in asynchronous methods using try-catch blocks. Place the await expression within a try block, and catch the exceptions in the catch block. This ensures proper error handling without breaking the program flow.
Can I use async without await in C#?
While you can technically declare a method with `async` without using `await` inside it, this is not recommended. Doing so results in a warning because the method will run synchronously, defeating the purpose of asynchronous programming.
How does asynchronous programming improve application performance?
Asynchronous programming improves application performance by allowing the application to remain responsive to user input while waiting for long-running tasks (like I/O operations) to complete, thereby efficiently utilizing resources and improving scalability.
What are best practices for asynchronous programming in C#?
Best practices include using `async` and `await` for I/O-bound tasks, avoiding `async void` methods except for event handlers, properly handling exceptions, and utilizing Task-based APIs for CPU-bound work to optimize performance and scalability.