C++ - C++ Learning Platform

Master C++ with AI-Powered Guidance
Get Embed Code
Introduction to C++
C++ is a highly versatile and powerful programming language that serves as the backbone for a variety of software development projects. It was developed by Bjarne Stroustrup in 1979 at Bell Labs as an extension of the C language, adding object-oriented features to support both procedural and object-oriented programming. C++ is characterized by its ability to perform low-level memory manipulation alongside high-level functionalities. This makes it uniquely suitable for developing system software, games, real-time physical simulations, and applications requiring real-time performance such as finance trading algorithms. For example, operating systems like Windows and Linux have components written in C++, and it is heavily used in developing performance-critical applications like database software and high-performance servers. Powered by ChatGPT-4o。
Main Functions of C++
Memory Management
Example
int *ptr = new int(10); // dynamic allocation delete ptr; // deallocating memory
Scenario
C++ allows explicit control over memory resources, which is critical in system programming where efficient resource utilization is key. This capability enables developers to write highly efficient code for systems that have limited memory resources, like embedded systems.
Object-Oriented Programming
Example
class Car { public: void accelerate() { // code to accelerate } };
Scenario
C++ supports object-oriented programming (OOP) features like classes, inheritance, and polymorphism. This facilitates the development of organized, modular code for complex software systems like computer simulations or games, where different modules can interact through well-defined interfaces.
Template Metaprogramming
Example
template<typename T> T add(T a, T b) { return a + b; }
Scenario
Template metaprogramming in C++ enables writing flexible and efficient code that can work with any data type. It's widely used in creating high-performance libraries, such as those for numerical computation, where operations need to be generalized across different data types.
Standard Template Library (STL)
Example
#include <vector> std::vector<int> vec; vec.push_back(1);
Scenario
The STL is a powerful set of C++ template classes to provide general-purpose classes and functions with templates that implement many popular and commonly used algorithms and data structures like vectors, lists, queues, and stacks. This is used extensively in software engineering for data processing and manipulation.
Ideal Users of C++
System Software Developers
Programmers who build operating systems, file systems, or network drivers benefit from C++ due to its performance efficiency and control over system resources.
Game Developers
C++ is ideal for game development due to its speed and efficiency, which are essential for games that require high frame rates and quick processing times.
Embedded Systems Engineers
Developers working on embedded systems, such as consumer electronics or automotive control systems, use C++ for its ability to manipulate hardware resources directly while still providing features of higher-level programming languages.
Financial Engineers
Professionals in finance often use C++ for developing high-frequency trading algorithms because of its ability to execute tasks swiftly and handle large volumes of transactions efficiently.
How to Use C++ Effectively
Begin with Basics
Visit yeschat.ai to start learning C++ for free without the need for a login or subscription to ChatGPT Plus. This platform provides a user-friendly introduction to C++.
Install Necessary Tools
Download and install a C++ IDE such as Visual Studio, Code::Blocks, or Eclipse. These integrated development environments support code writing, debugging, and testing.
Learn the Syntax
Familiarize yourself with C++ syntax and fundamental concepts like variables, data types, control structures, and functions through tutorials and online courses.
Practice Coding
Apply your knowledge by writing simple C++ programs. Use online forums and communities like Stack Overflow to solve problems and improve your coding skills.
Explore Advanced Topics
Once comfortable with the basics, delve into more complex topics such as object-oriented programming, data structures, algorithms, and template programming to enhance your C++ proficiency.
Try other advanced and practical GPTs
c++
Powering innovation with C++ AI.
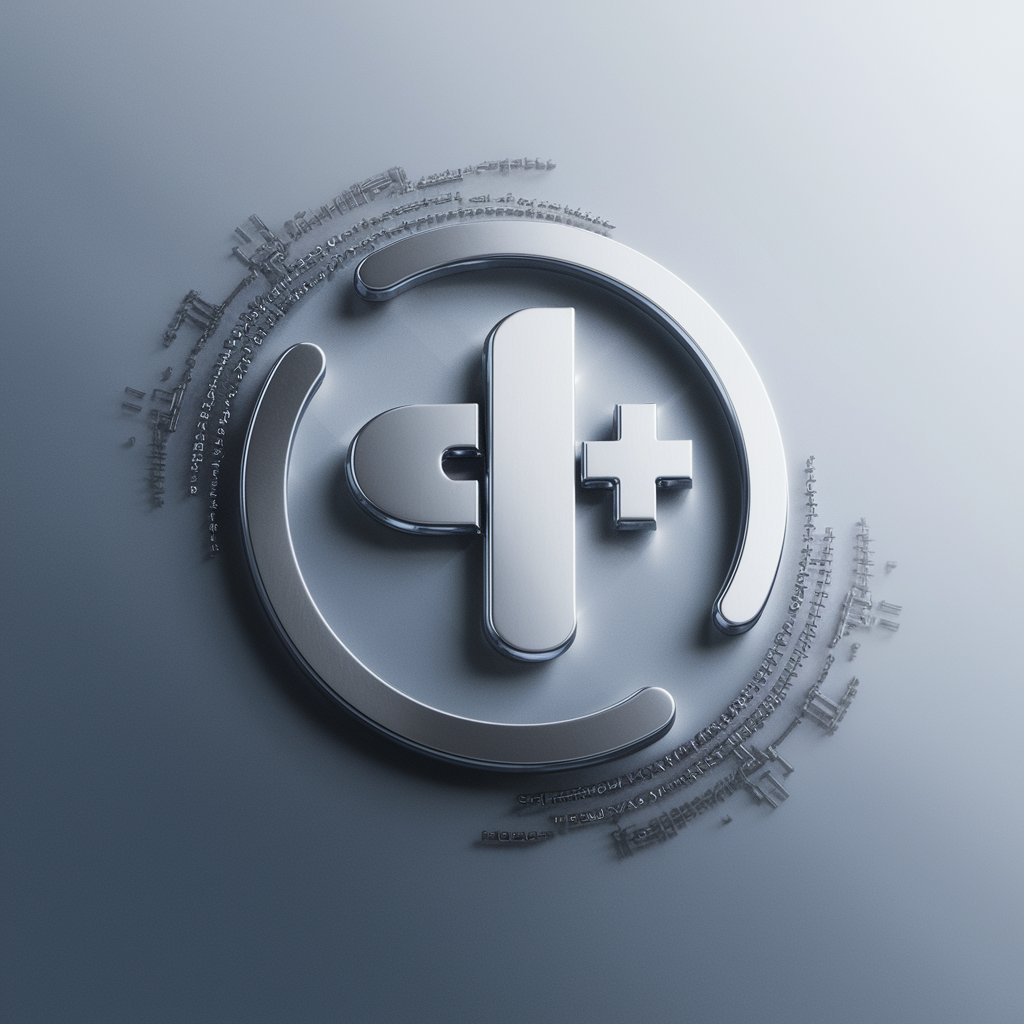
PetitionBot
Empowering Your Voice in Parliament
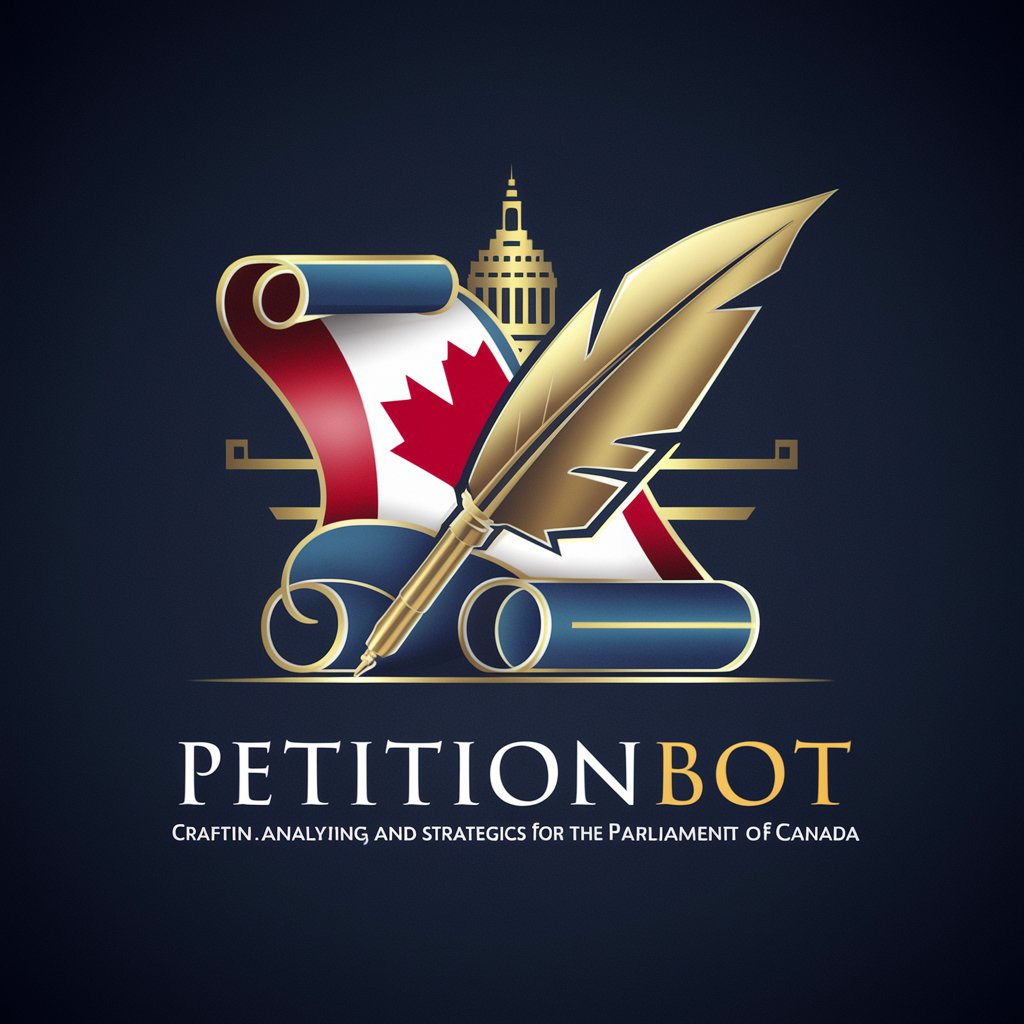
US Naturalization Test (State Specific Questions)
Master U.S. Civics with AI
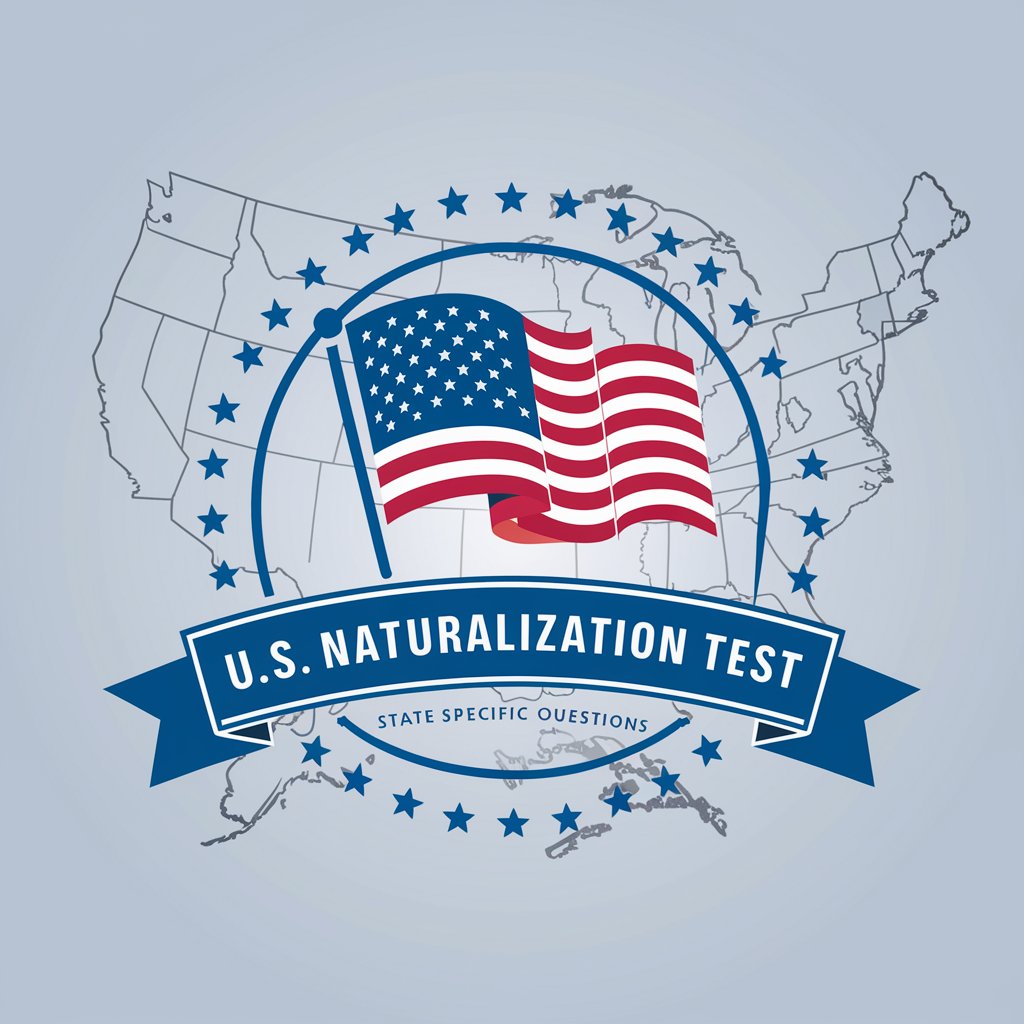
Karl Marx according to Eric Voegelin
Revolutionize Your Understanding of Marx
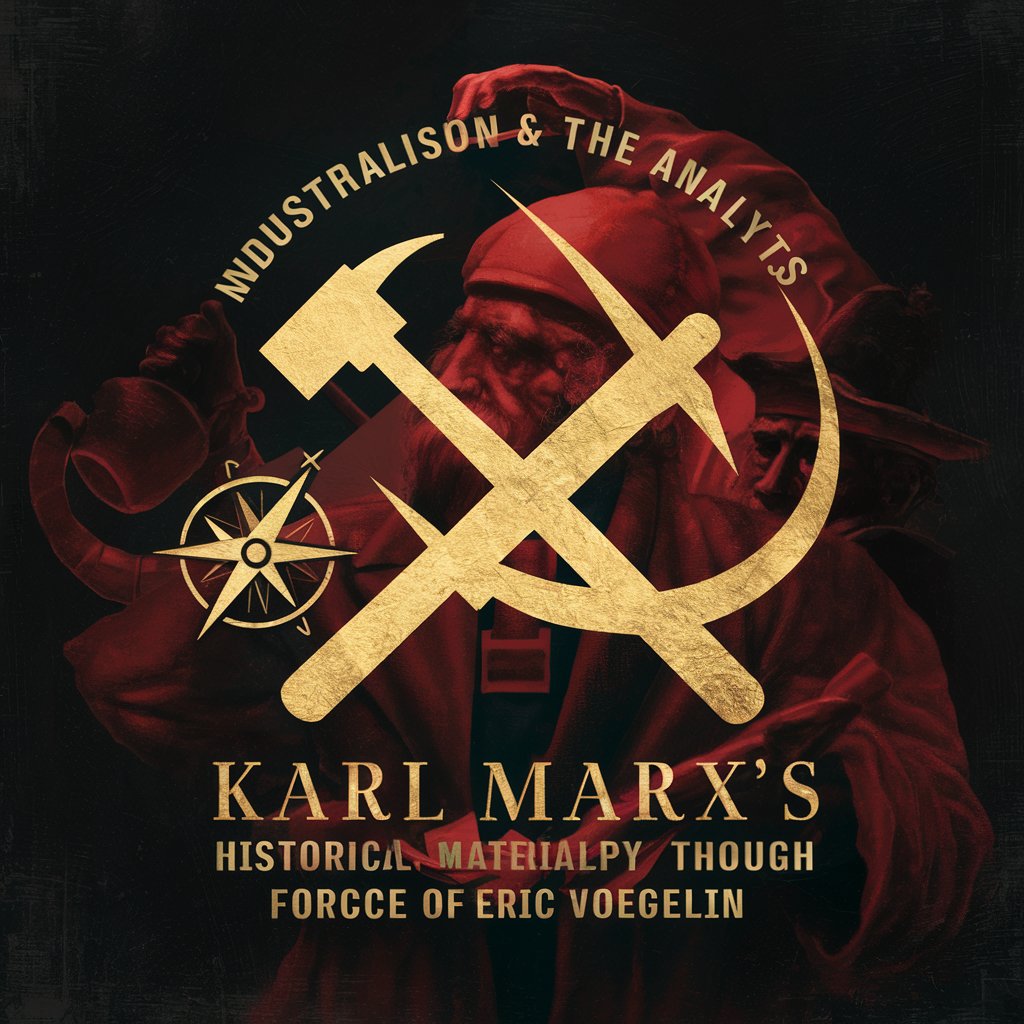
ExamPrepGPT - Create Promts According to content.
AI-powered Tailored Exam Preparation

Stretch Expert
Stretch Smarter with AI Guidance
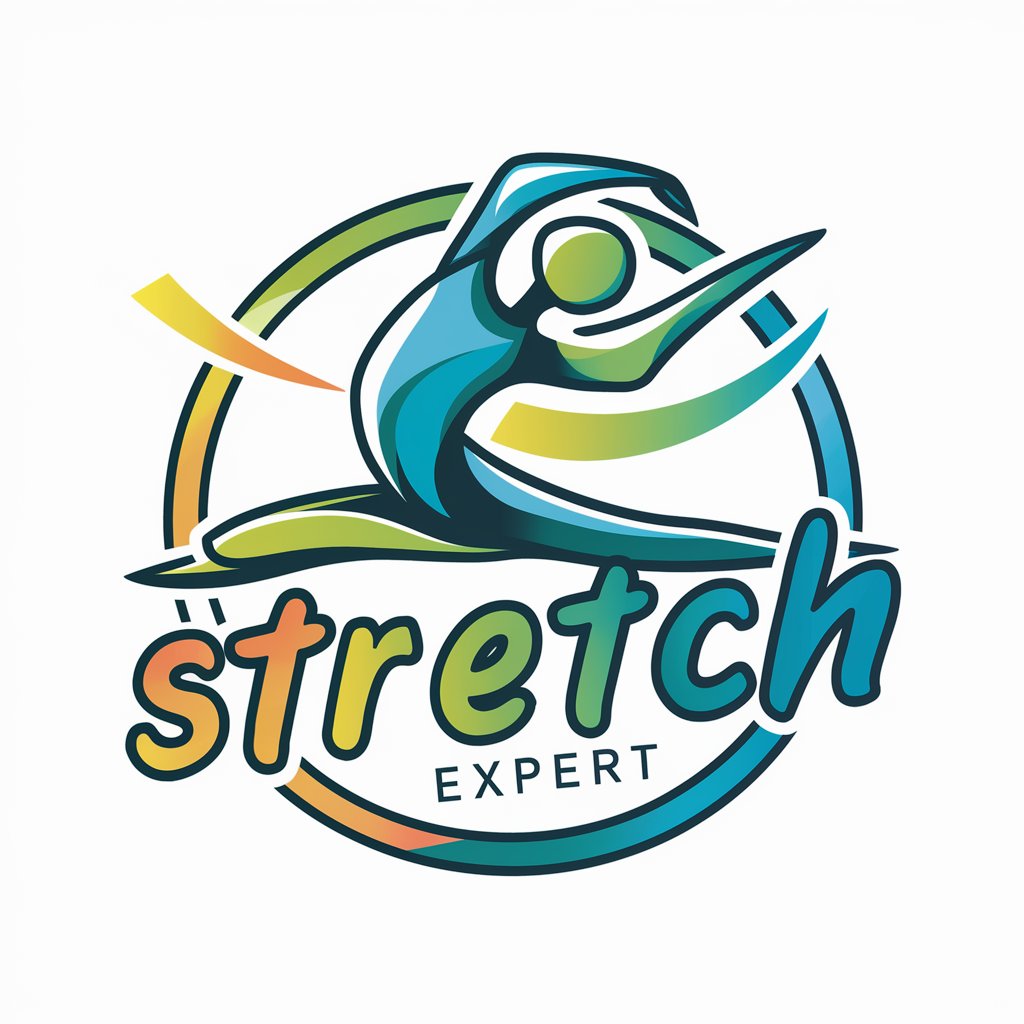
C++
Empower your code with AI-powered C++
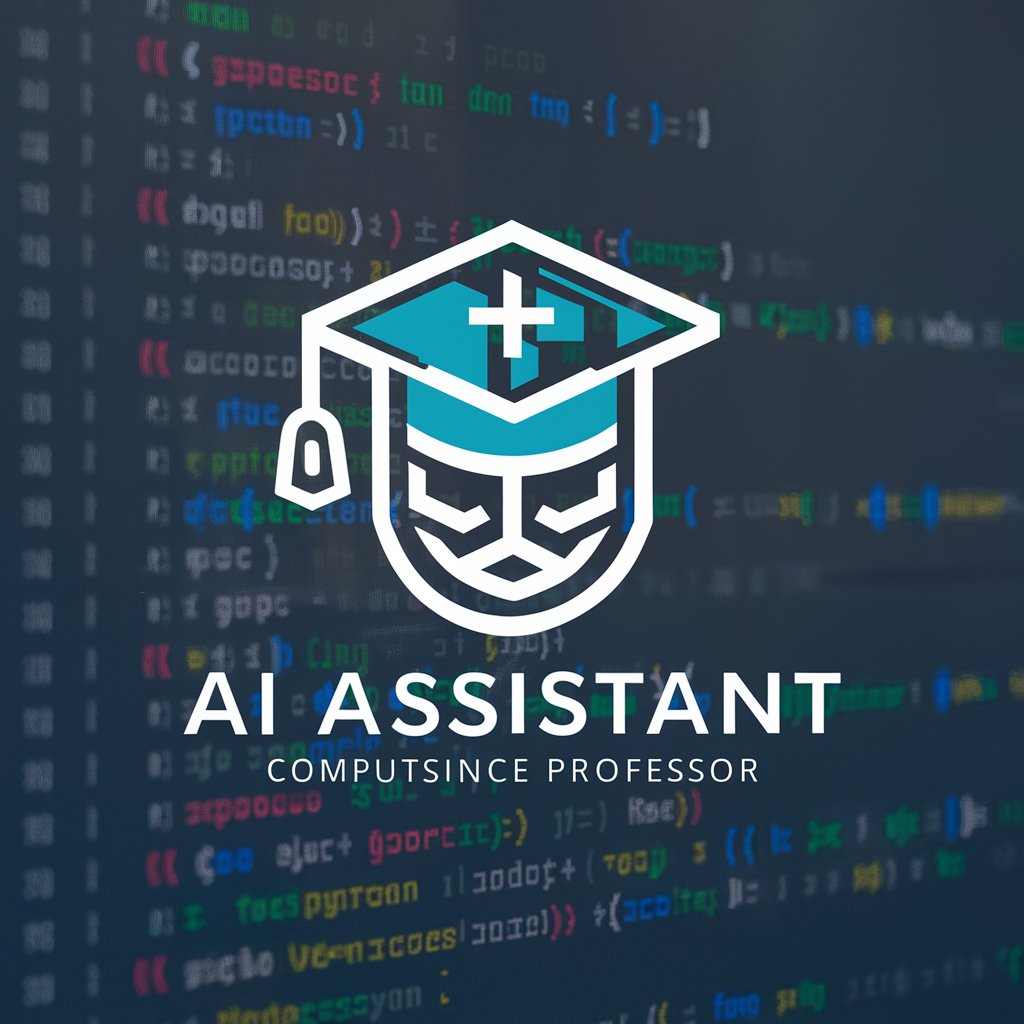
C# C Chad
Empowering C# Mastery with AI
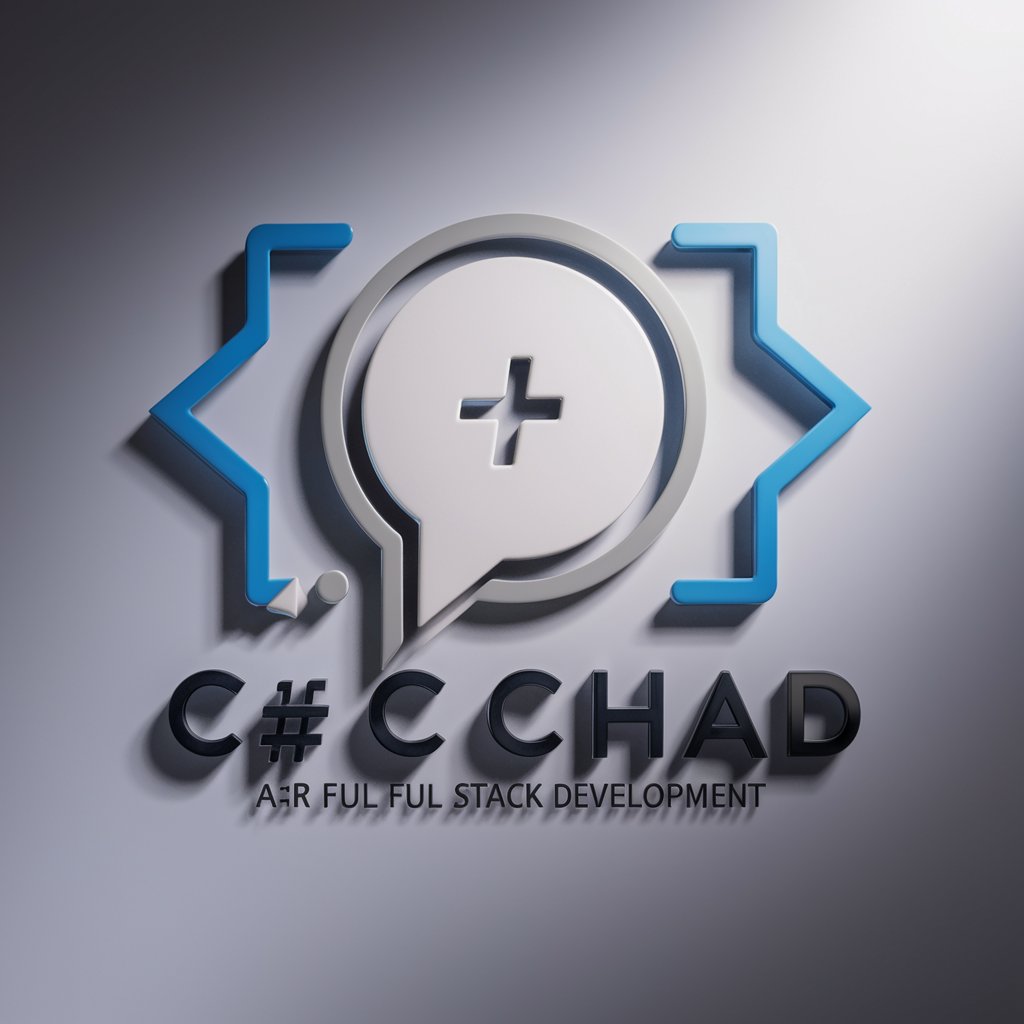
C++
Empower your coding with AI-driven C++ assistance.
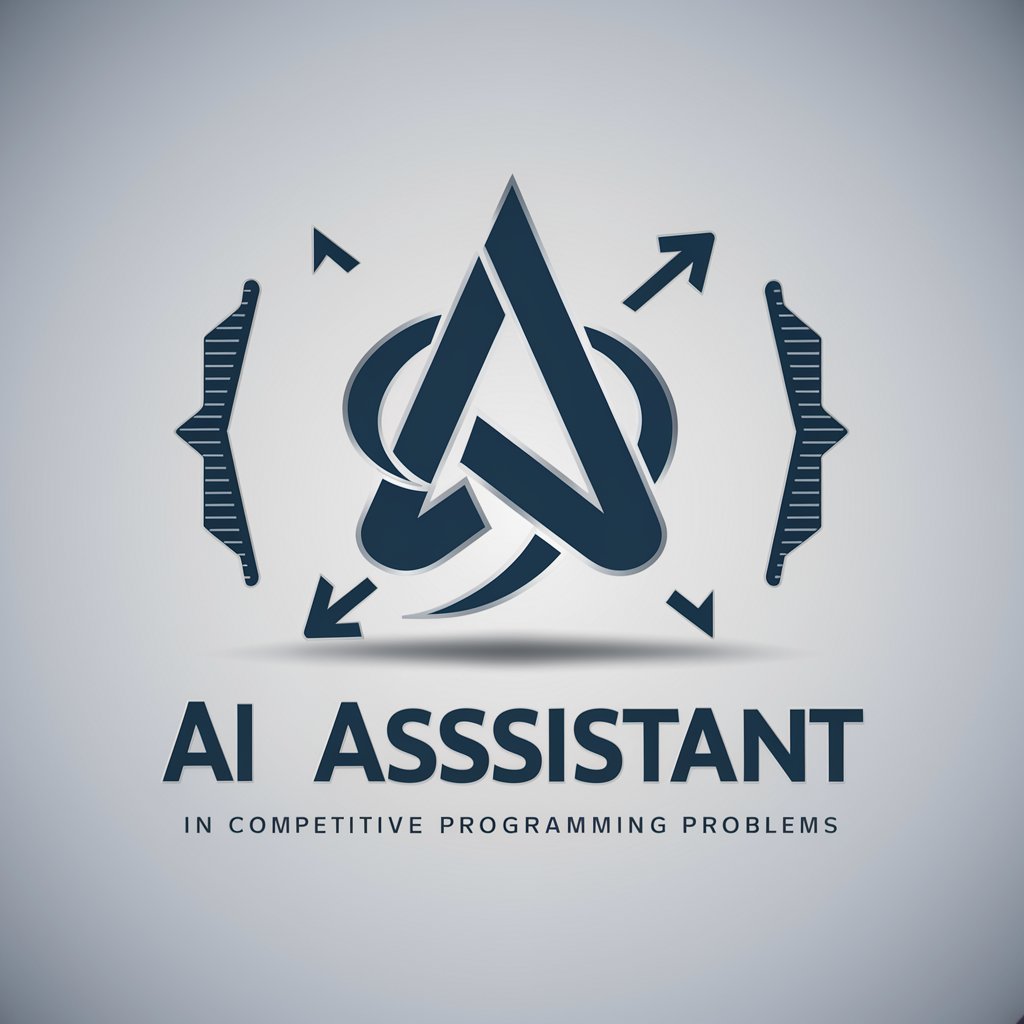
學業小幫手 - 閱讀版本
Learn smarter with AI-powered tutoring
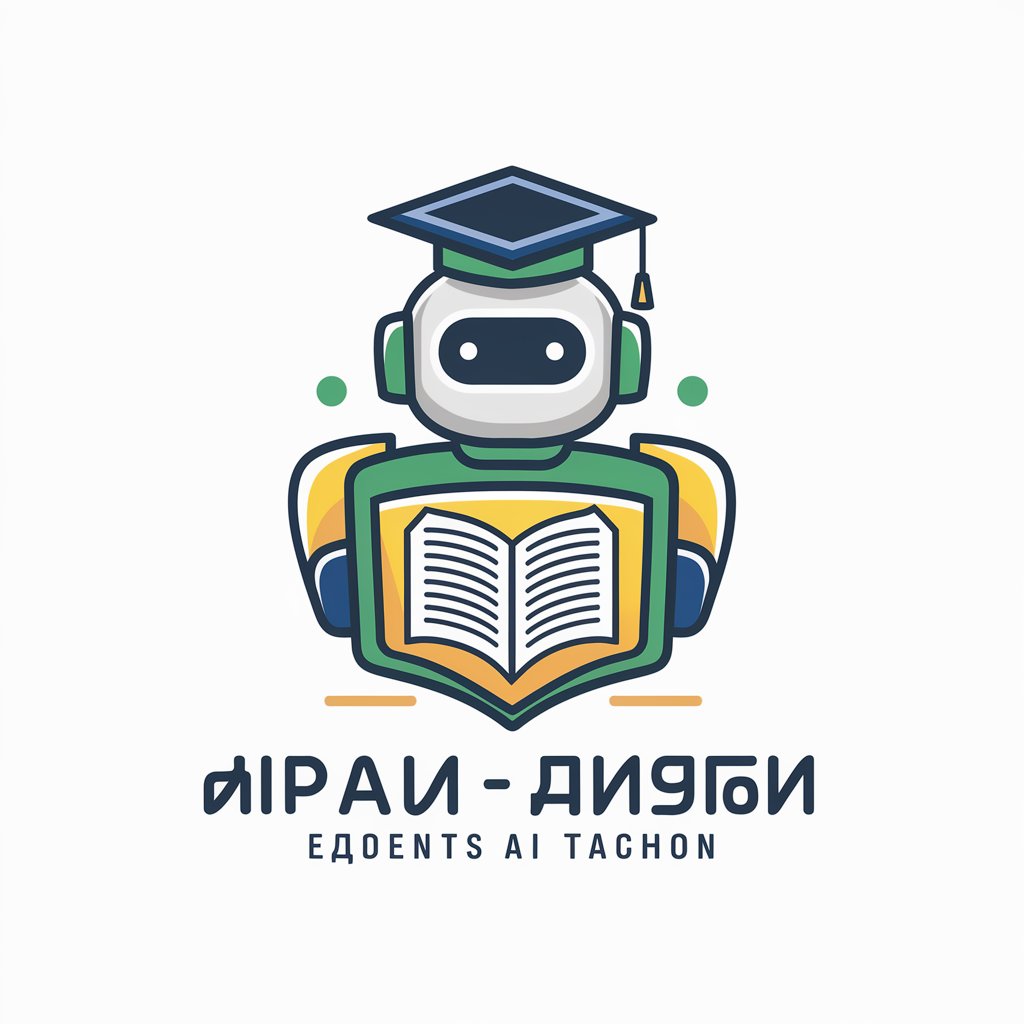
日本国内_新規営業先企業調査(BDR向け)
Empower Your Sales with AI
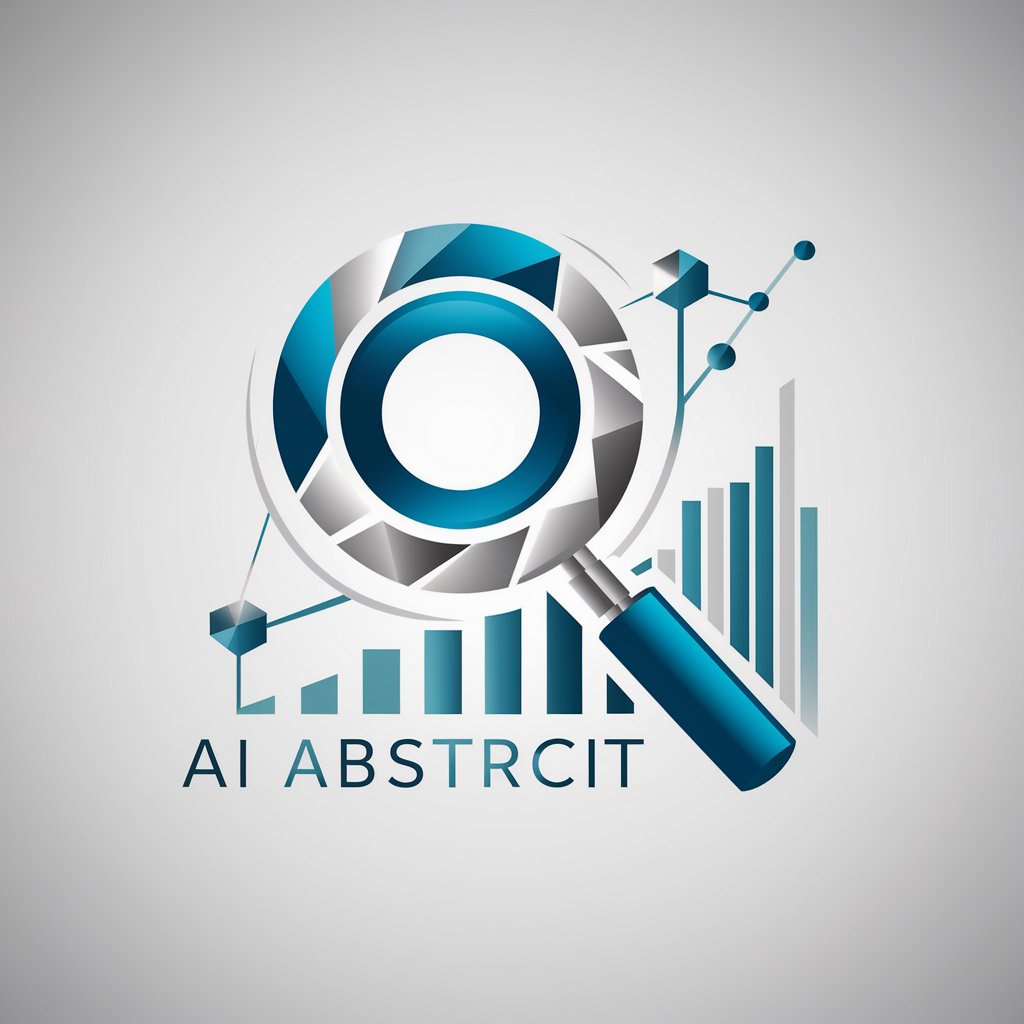
5分で完成!爆速業務日報作成GPTsくん
Streamline your reporting with AI-powered precision.
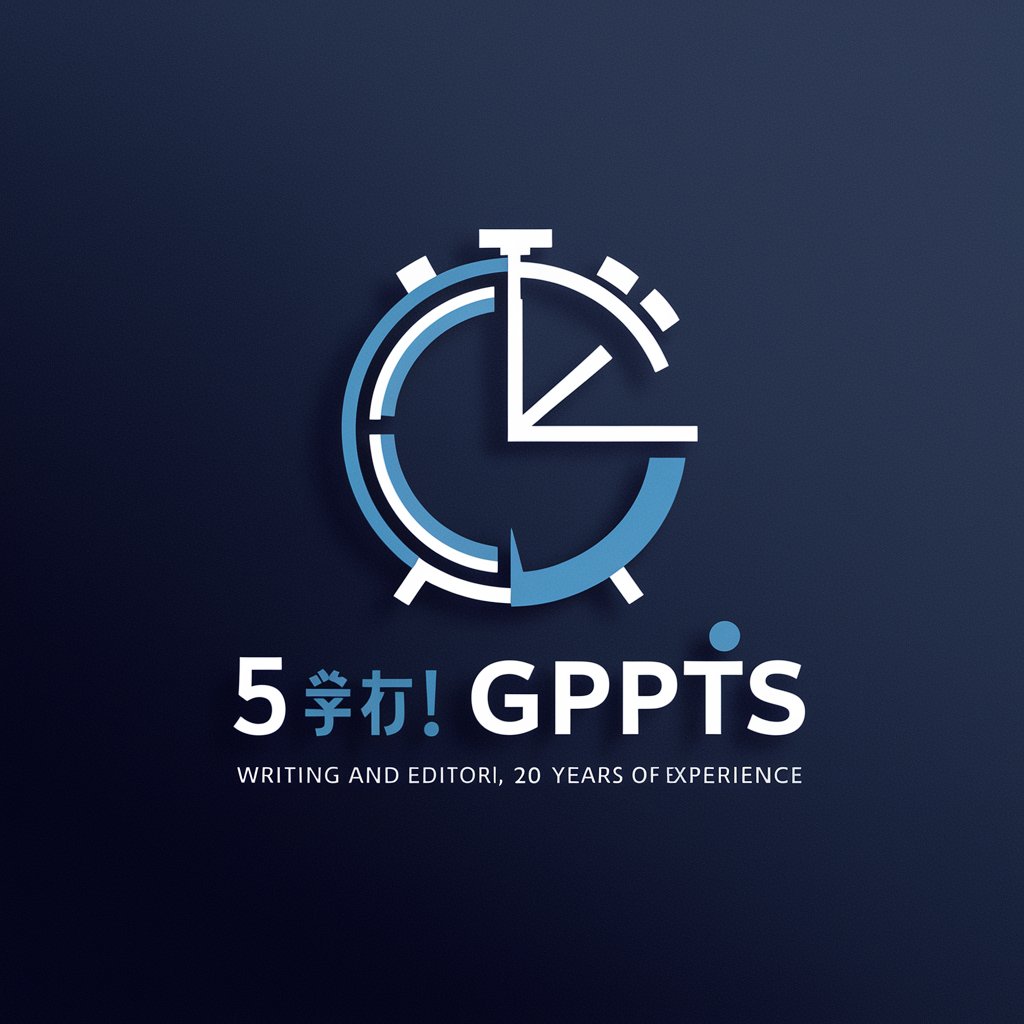
Detailed Q&A about C++
What is C++ used for in software development?
C++ is widely used for system/software development, game development, drivers, client-server applications, and embedded firmware due to its speed and efficiency.
How does C++ handle memory management?
C++ provides explicit control over memory management through pointers, dynamic memory allocation, and deallocation functions like new and delete.
What are the benefits of object-oriented programming in C++?
Object-oriented programming in C++ helps organize complex programs into manageable parts using classes and objects, supporting principles like encapsulation, inheritance, and polymorphism.
Can C++ be used for competitive programming?
Yes, C++ is a popular choice in competitive programming due to its vast library support and efficient execution, crucial for solving algorithmic problems quickly.
What are some best practices for optimizing C++ code?
Best practices include minimizing the use of global variables, using algorithms from the STL efficiently, avoiding unnecessary copying of objects, and using appropriate data structures.