C-C programming: compiler, code, run
Empower your code with AI assistance
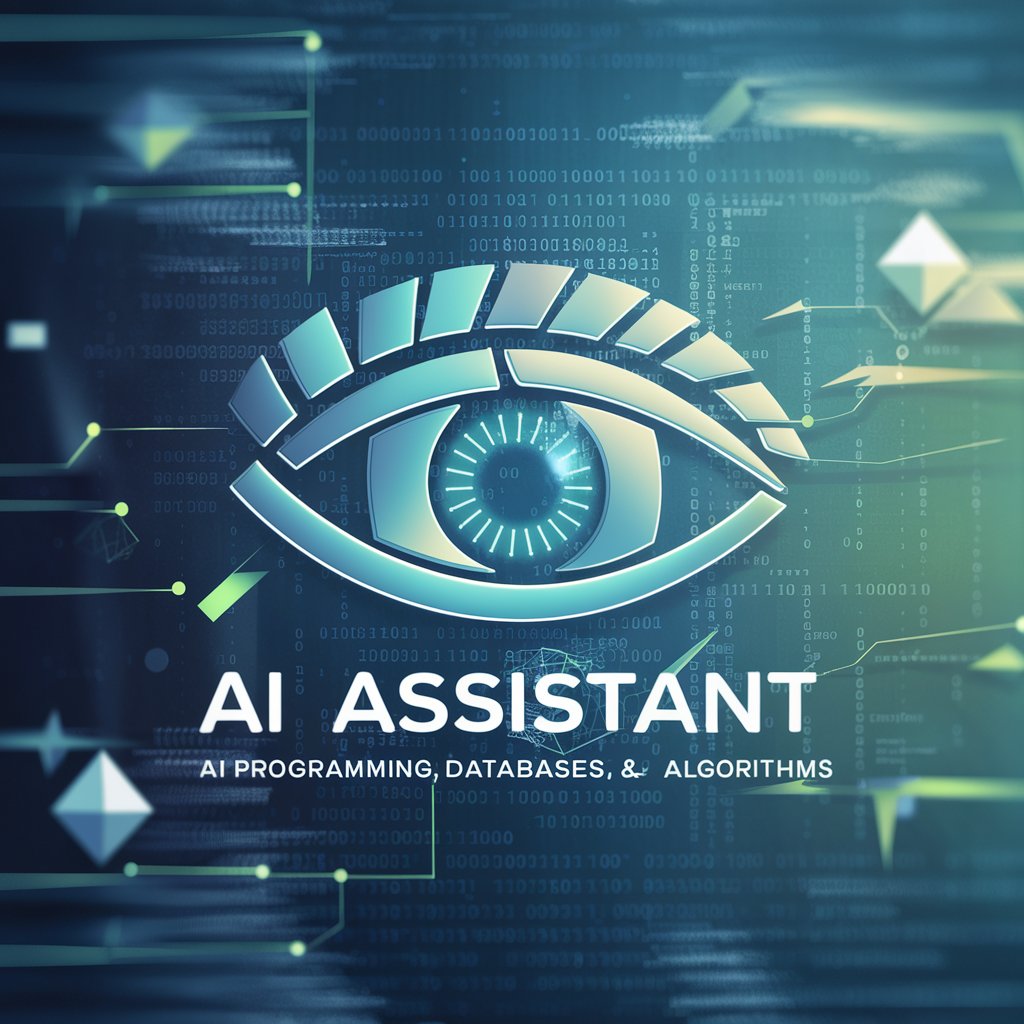
Write a C program that implements...
How can I optimize my C code for...
Create a database structure in C that...
Explain how to implement an algorithm for...
Related Tools
Load More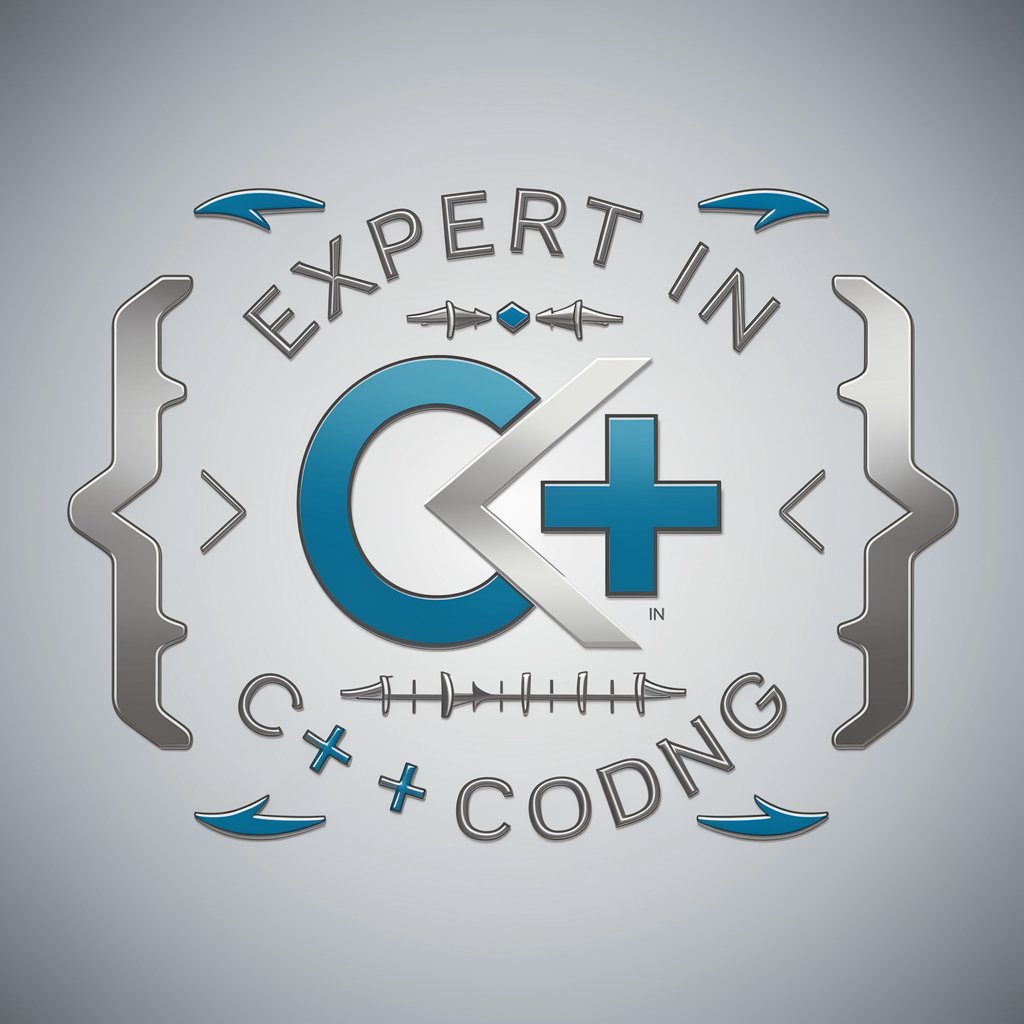
Expert in C/C++ Coding
C/C++ coding expert offering guidance and best practices.

C 语言助手
以中文帮助初学者分析和理解C语言问题。
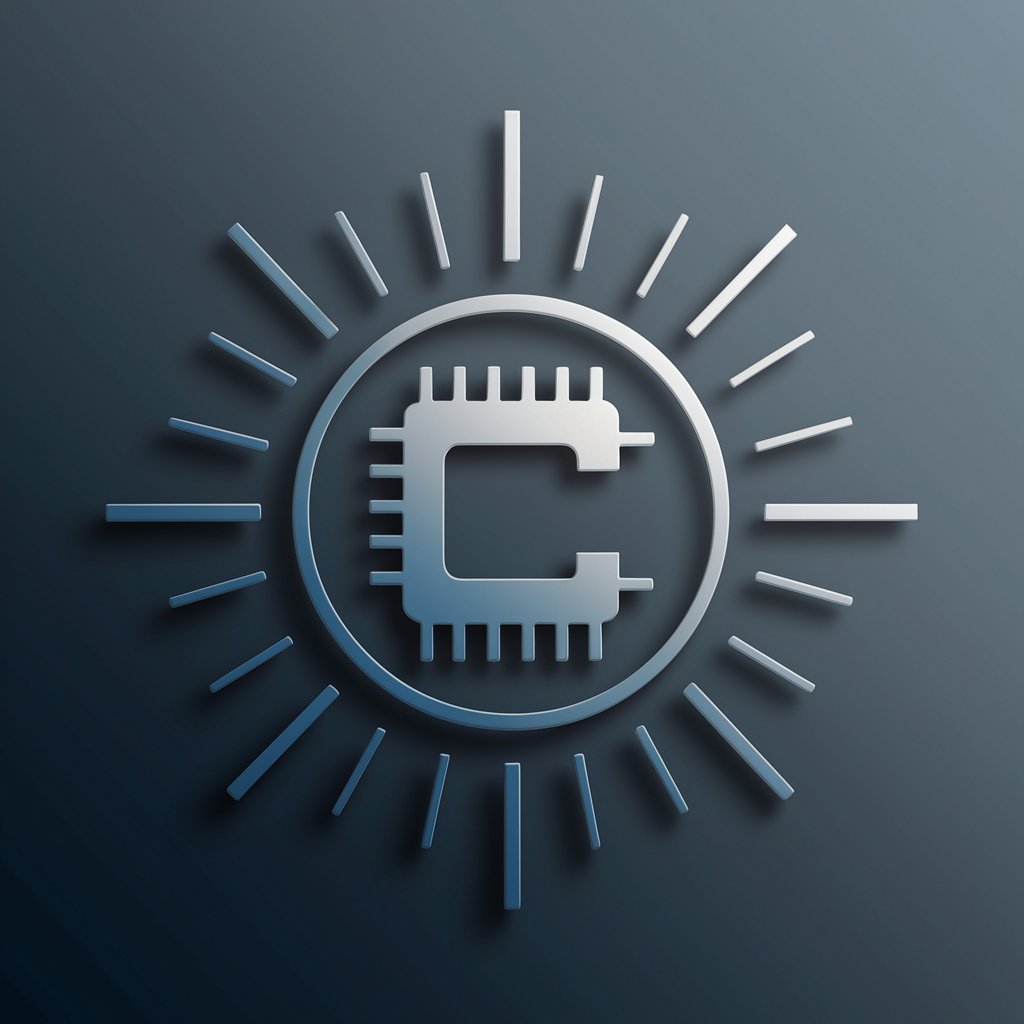
C HELPER
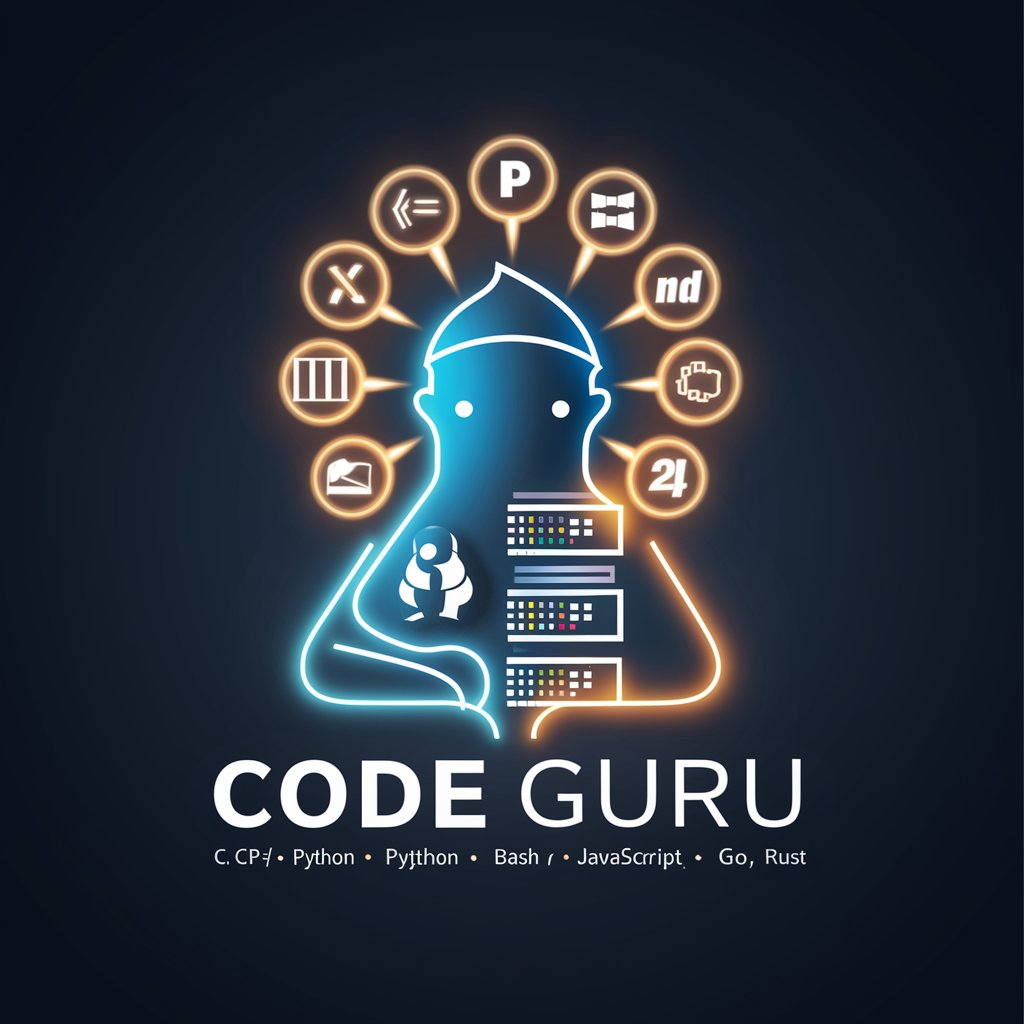
Code Guru
Expert in Linux & Windows development, skilled in multiple programming languages.
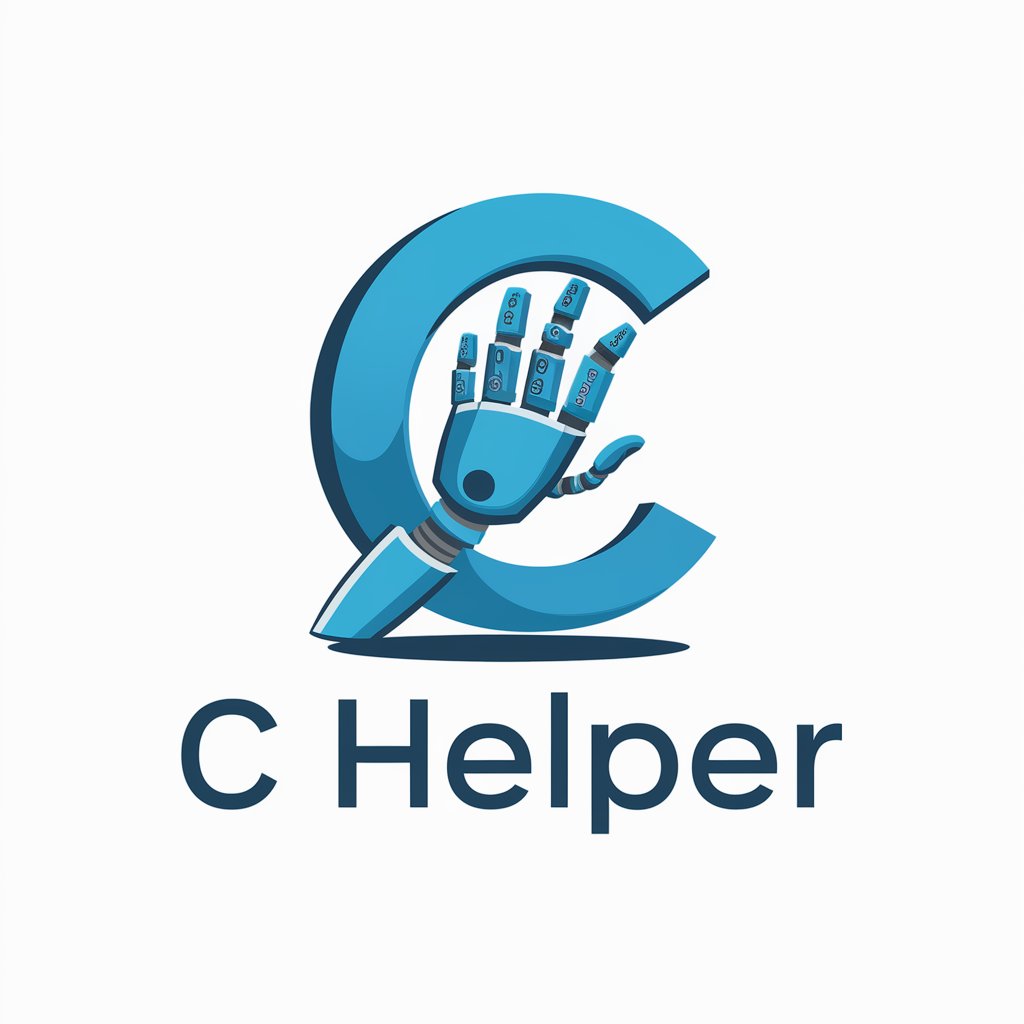
C Helper
An assistant for learning and coding in C language.
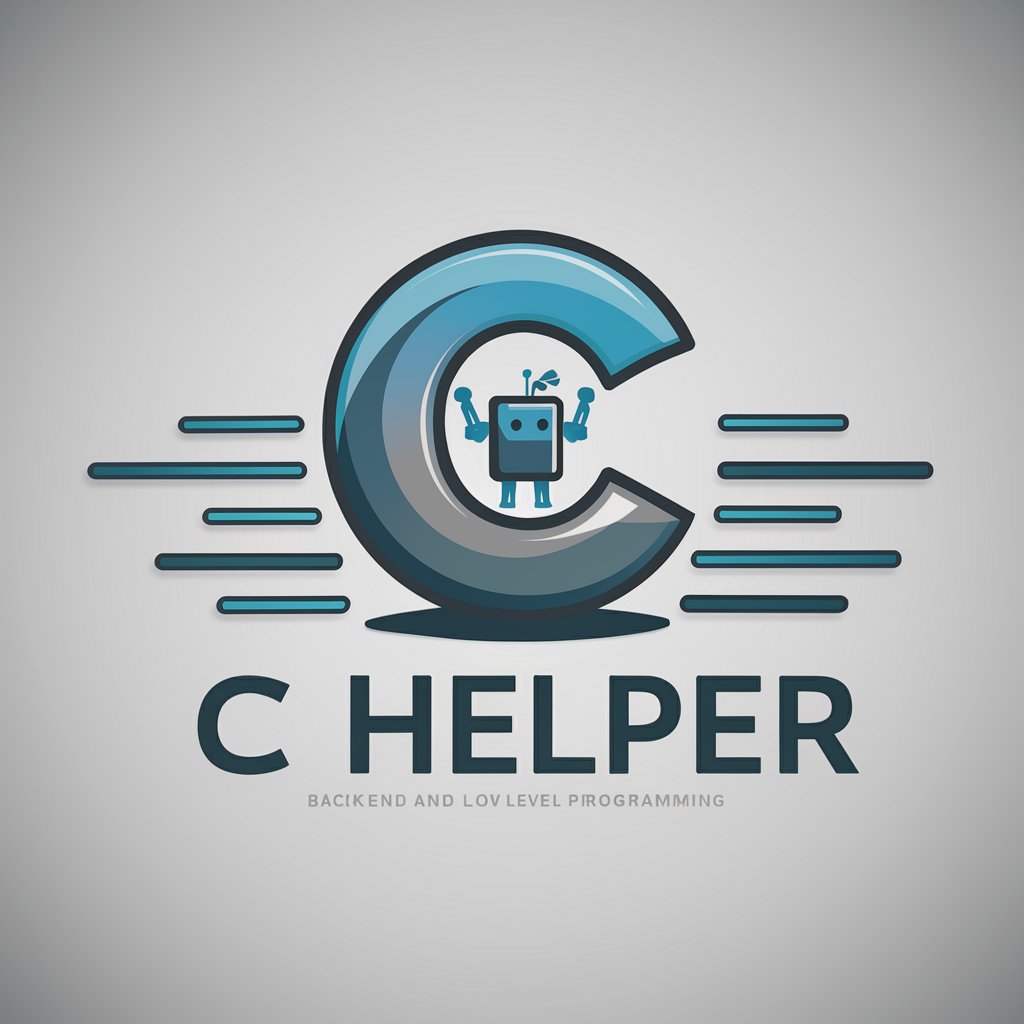
C Helper
Expert in C coding and development
Introduction to the C Programming Language
C is a general-purpose programming language developed in the early 1970s by Dennis Ritchie at Bell Labs. It was designed to provide low-level access to memory while maintaining high-level abstractions that make it suitable for developing operating systems, system software, and various applications. C's syntax is relatively simple, making it easy to learn, while its powerful features like pointers, structures, and direct memory manipulation make it extremely versatile. The language's portability allows C programs to be compiled on different platforms with minimal changes, and its influence extends to numerous modern languages like C++, Java, and Python. Powered by ChatGPT-4o。
Main Features of C and Their Use Cases
Pointers
Example
int *ptr = &variable;
Scenario
Pointers in C are variables that store the address of another variable. They allow direct memory manipulation and can be used to dynamically allocate memory, handle arrays efficiently, and facilitate complex data structures like linked lists and trees.
Structures
Example
struct Person { char name[50]; int age; };
Scenario
Structures (structs) allow grouping different data types into a single unit. They are useful for modeling real-world entities with varied attributes, such as a 'Person' with a name and age, or a 'Point' with x and y coordinates.
Standard Library Functions
Example
printf("Hello, World!");
Scenario
The C standard library provides functions for input/output, string manipulation, mathematical computations, and more. For example, 'printf' is widely used for formatted output, 'fopen' for file handling, and 'memcpy' for memory operations.
Conditional Compilation
Example
#ifdef DEBUG printf("Debug mode"); #endif
Scenario
Conditional compilation enables the inclusion or exclusion of code based on certain conditions. This is useful for building different versions of software for testing, debugging, or specific environments by using preprocessor directives like '#ifdef'.
Ideal Users of the C Programming Language
System Programmers
System programmers require the ability to interact closely with hardware. C offers low-level capabilities like pointers and bit manipulation, making it ideal for developing operating systems, embedded software, device drivers, and firmware.
Game Developers
Game developers value performance and efficiency. C enables them to write high-performance graphics engines and physics calculations that can maximize hardware potential and deliver smooth gaming experiences.
Scientific Computing Professionals
Professionals in scientific computing or research often require precise control over memory and performance for computational models or data processing algorithms. C allows them to handle complex numerical calculations efficiently.
General Application Developers
Developers building desktop, network, and server applications can use C to create efficient, cross-platform software. The portability and standardization of C make it suitable for developing software that needs to run on multiple operating systems.
Using C: Step-by-Step Guide
Visit yeschat.ai for a free trial without login, also no need for ChatGPT Plus.
YesChat.AI offers a free trial for using C programming language without requiring any login or ChatGPT Plus subscription.
Install a C compiler on your system
Choose and install a C compiler suitable for your operating system, such as GCC for Unix-like systems or MinGW for Windows.
Write your C code
Use a text editor or an integrated development environment (IDE) to write your C code. Be sure to save your code with the .c extension.
Compile your code
Open a command prompt or terminal, navigate to the directory containing your C code, and use the appropriate compiler command to compile it.
Run your program
After successful compilation, execute your program either from the command line or within your IDE to see the results.
Try other advanced and practical GPTs
哄哄模拟器
AI-powered simulator for calming conflicts.

MJプロンプトメーカー
Unlock Your Creativity with AI-Powered Prompts
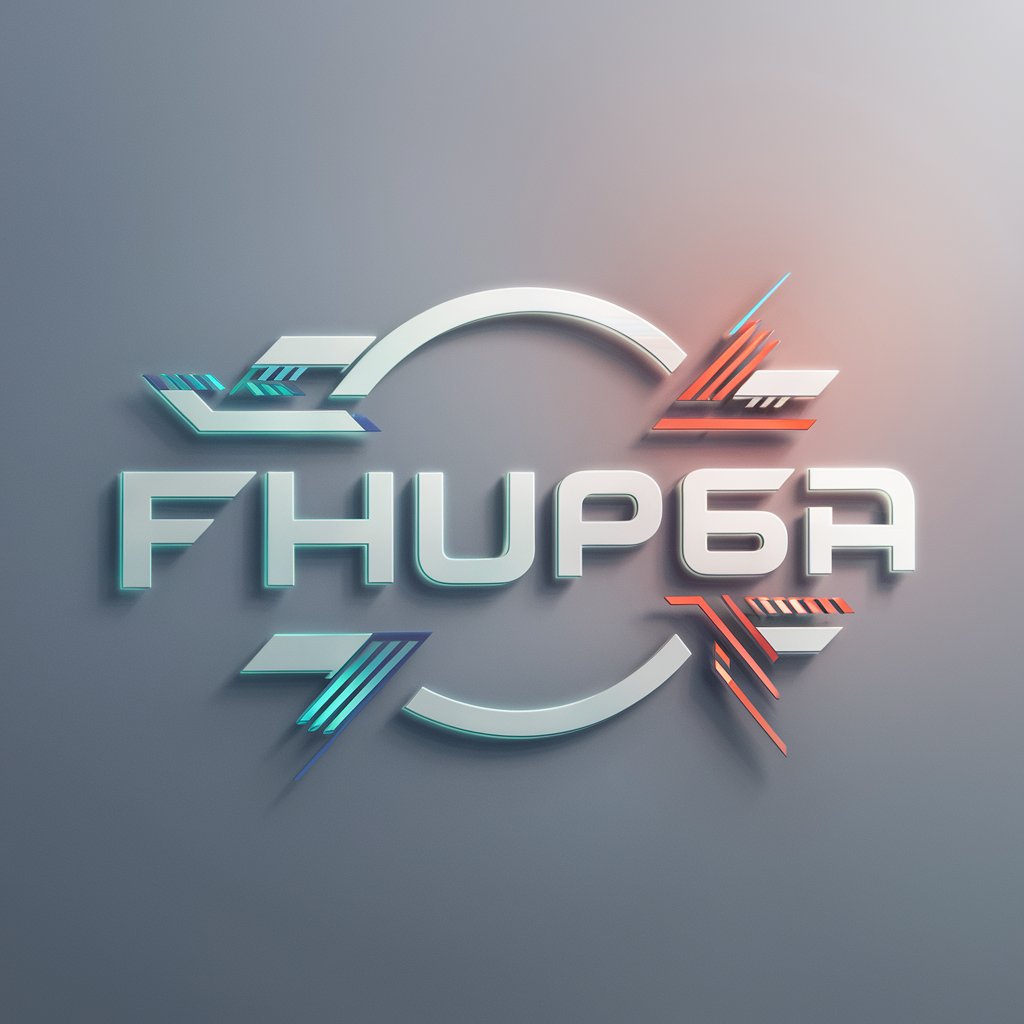
Java
AI-Powered Java Programming Assistant.
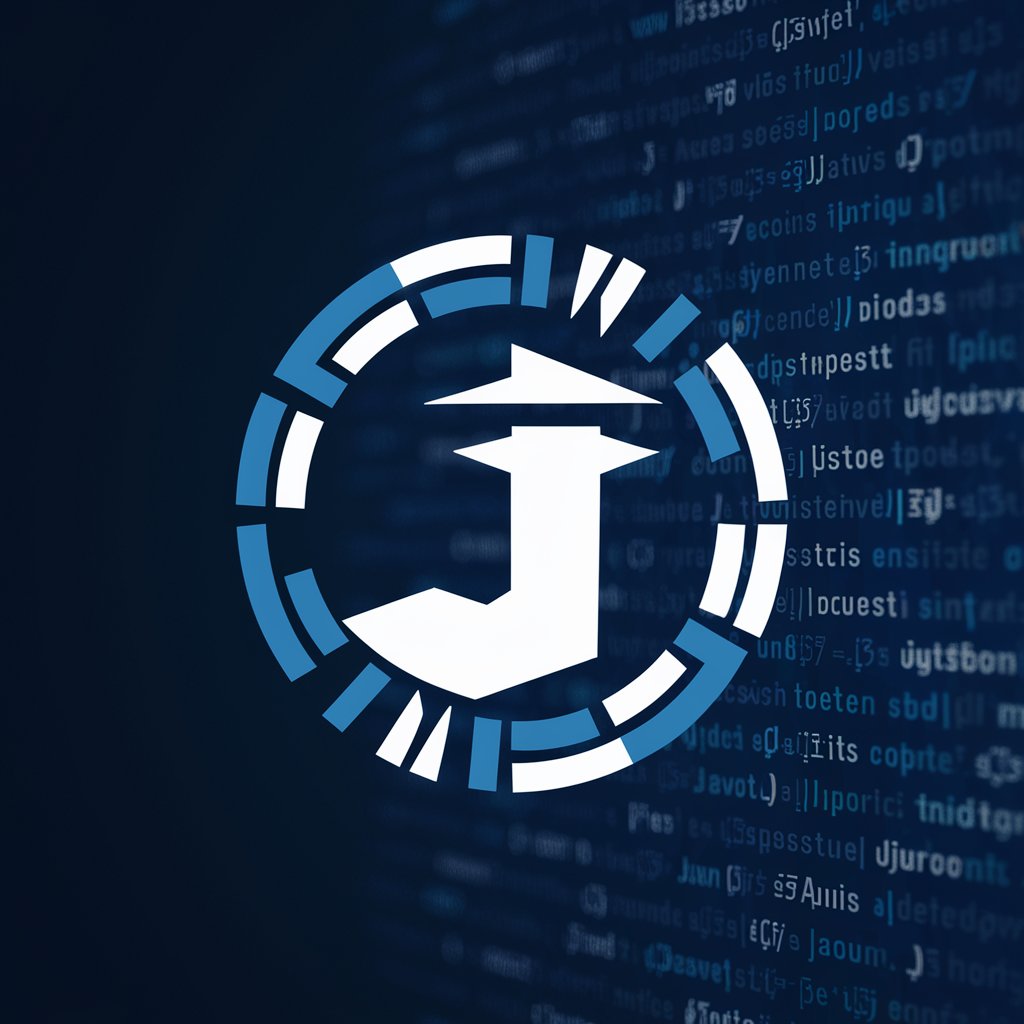
Rewrite Maker
Craft Clarity with AI
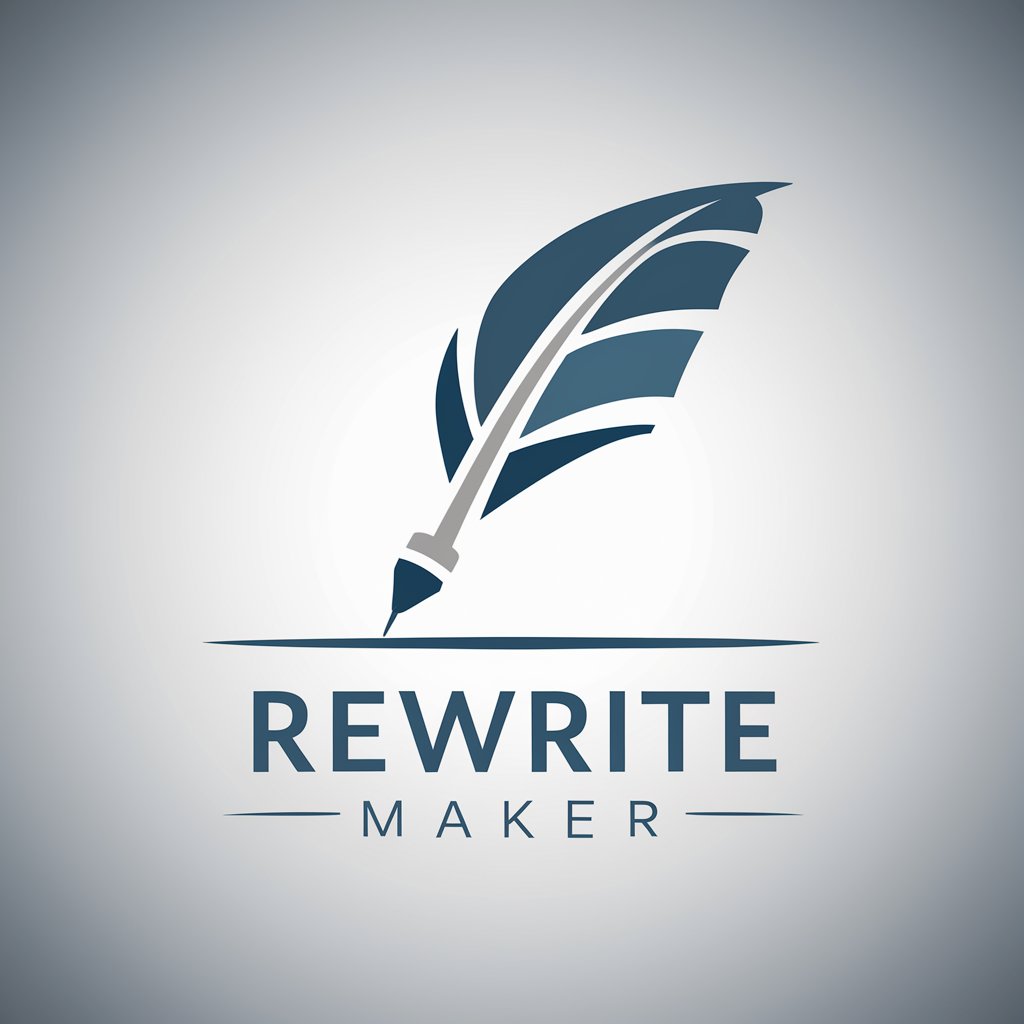
Generative Pretrained Transformer 5
Empowering Language with AI Precision
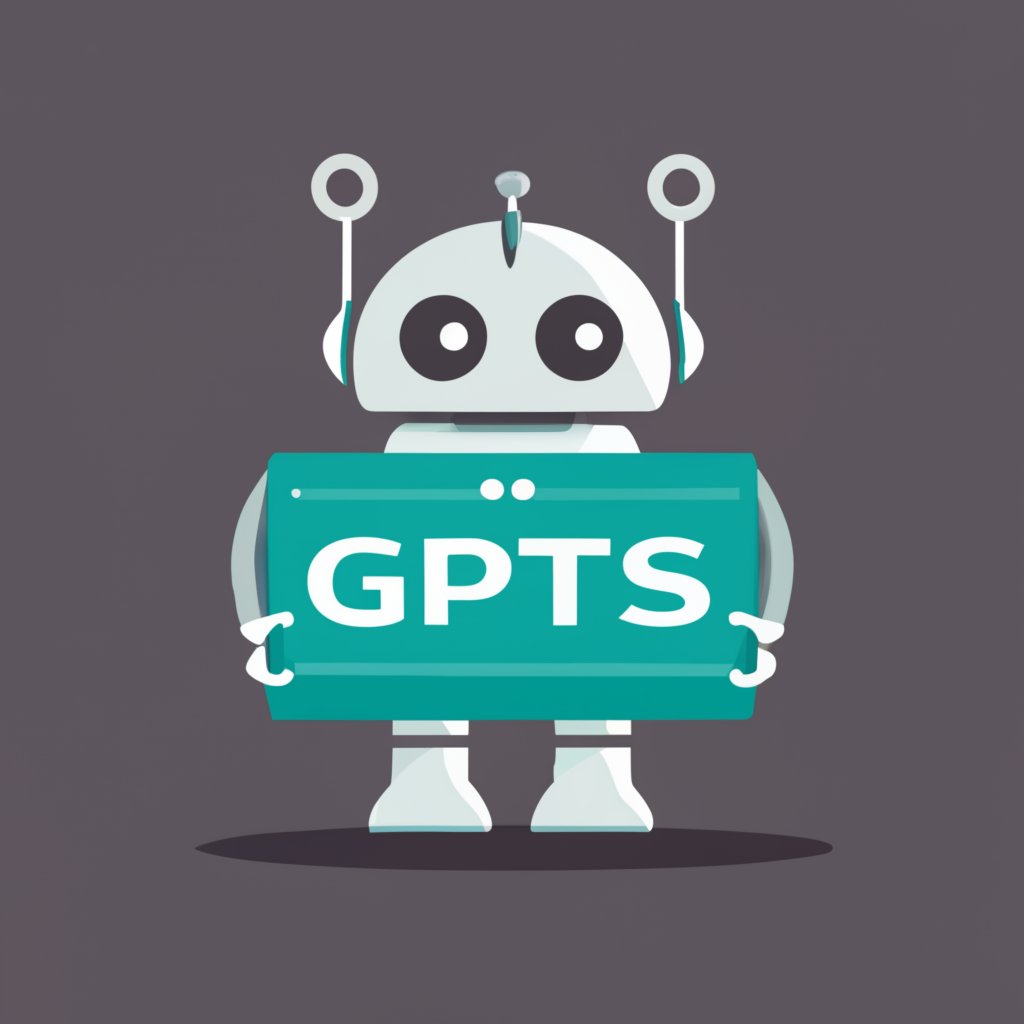
web
Unlock Comprehensive Insights with AI
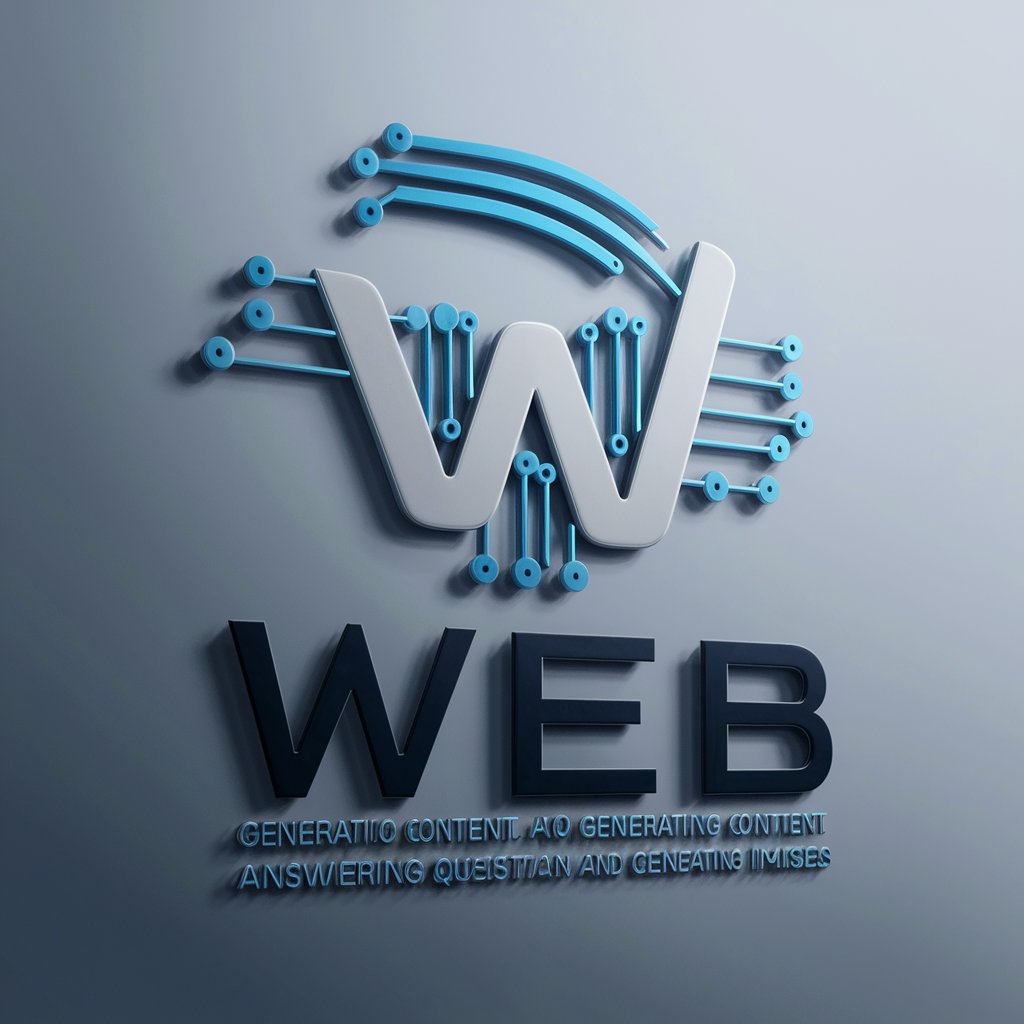
C HELPER
Enhance your C coding with AI.
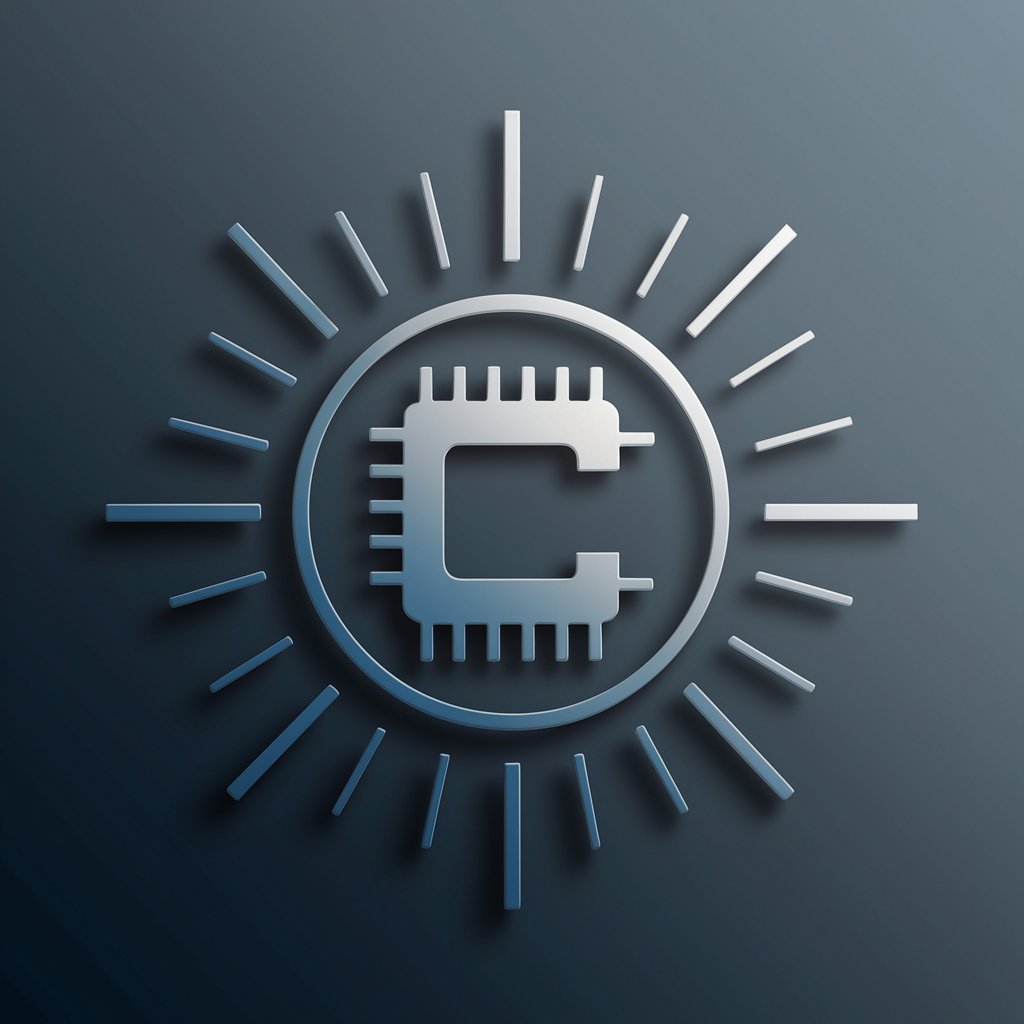
Cartoon
Bring Your Photos to Animated Life
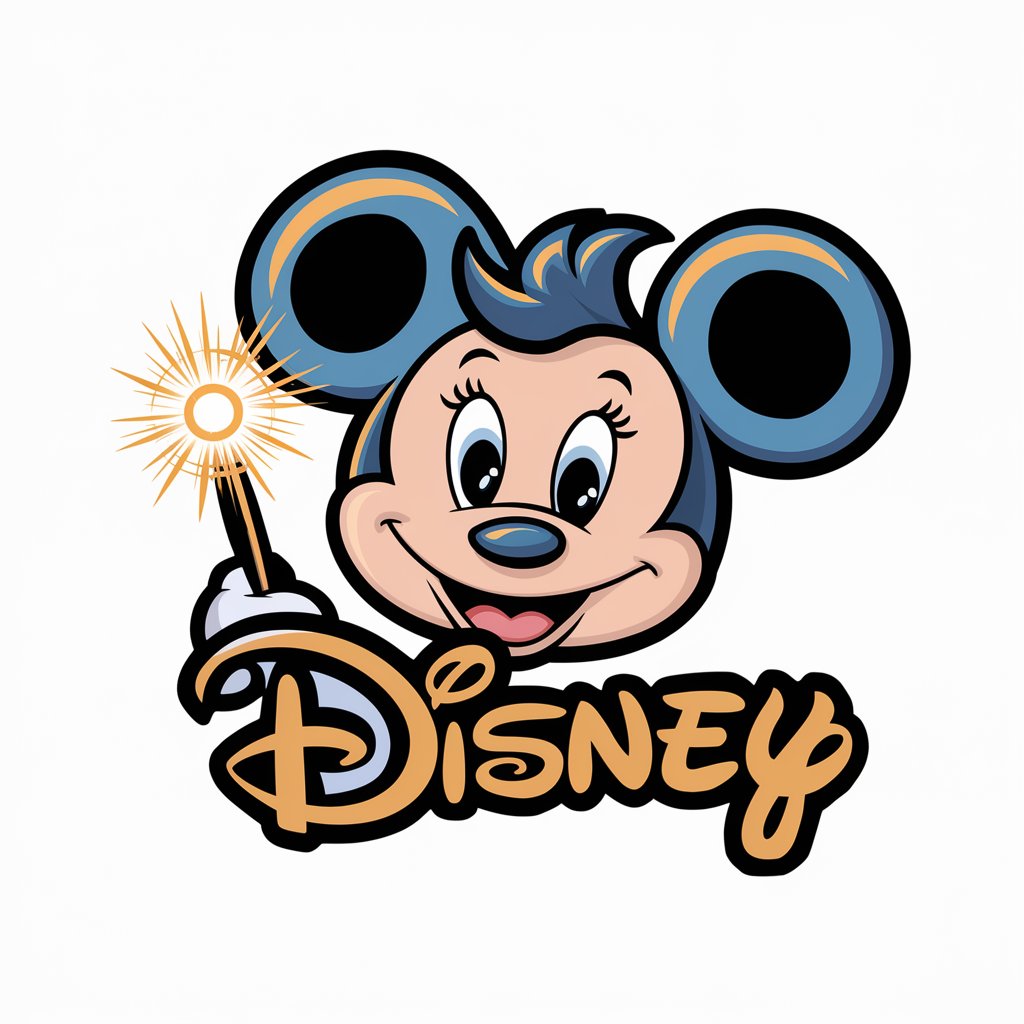
GoDaddy
Empowering Your Online Presence with AI
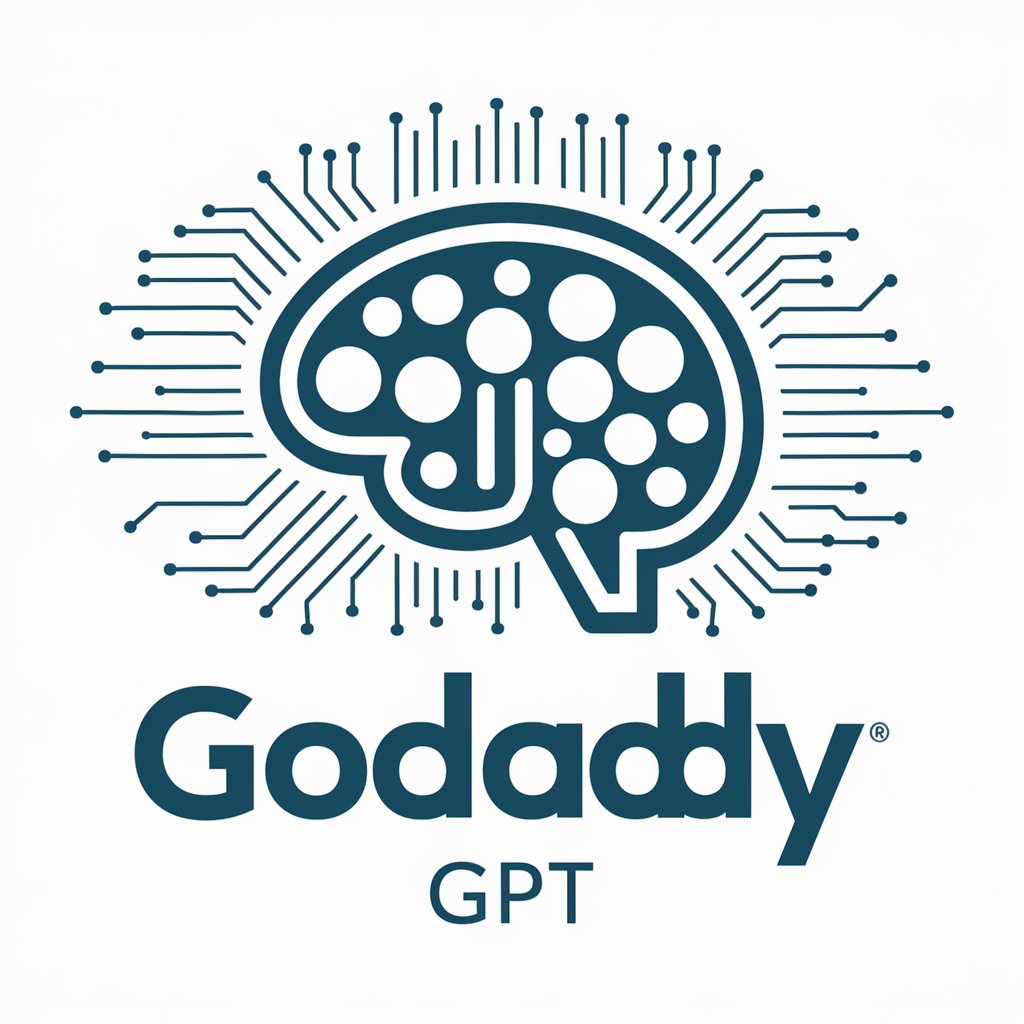
商务英语翻译
Empowering seamless bilingual communication with AI.
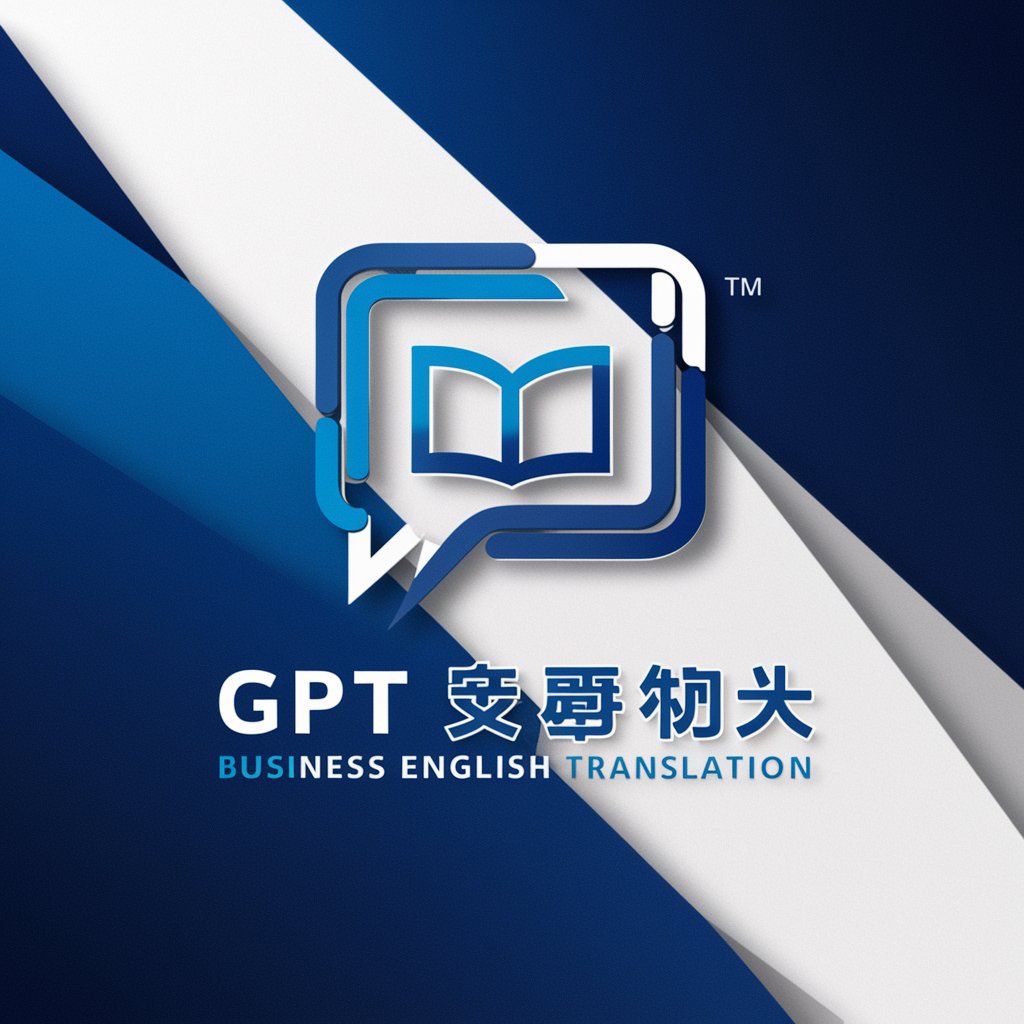
卡通形象生成器
Transform images into cartoons with AI.
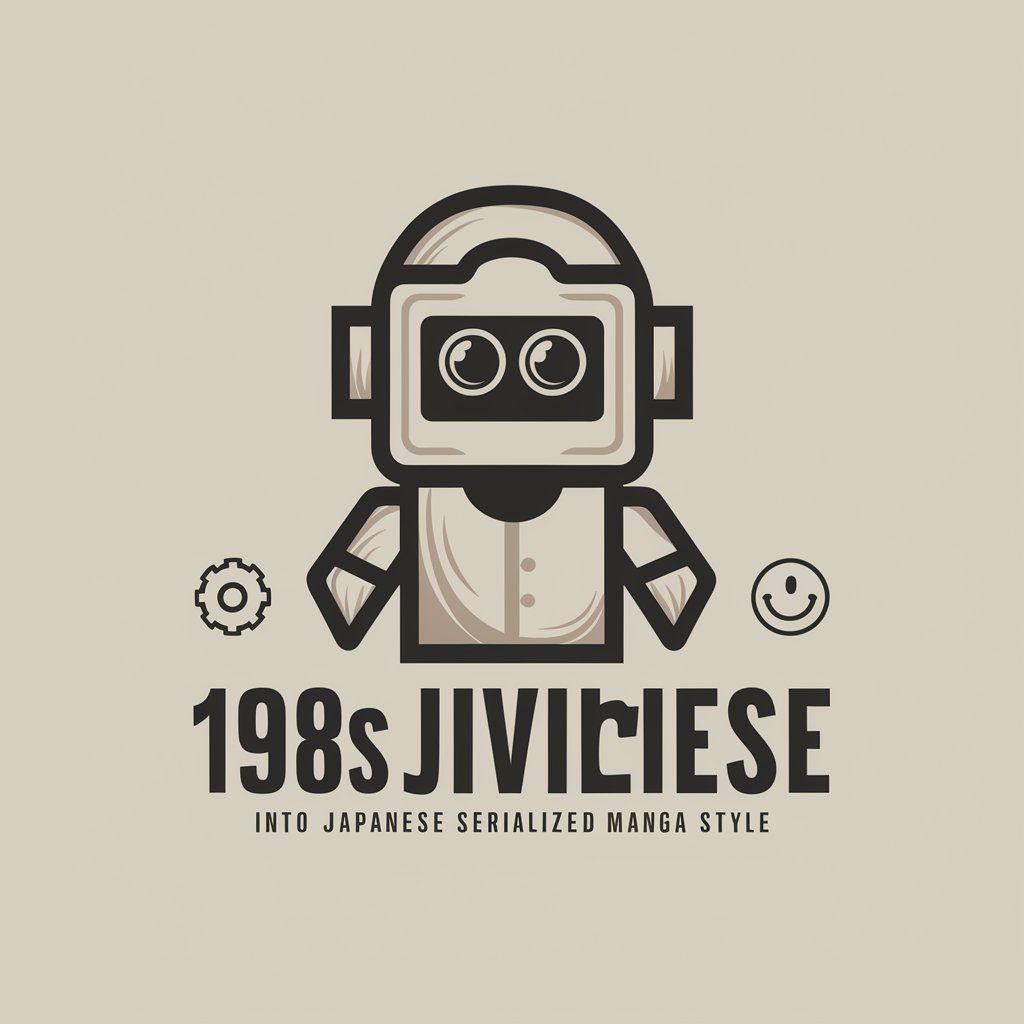
Social Media
Empower your social media with AI.
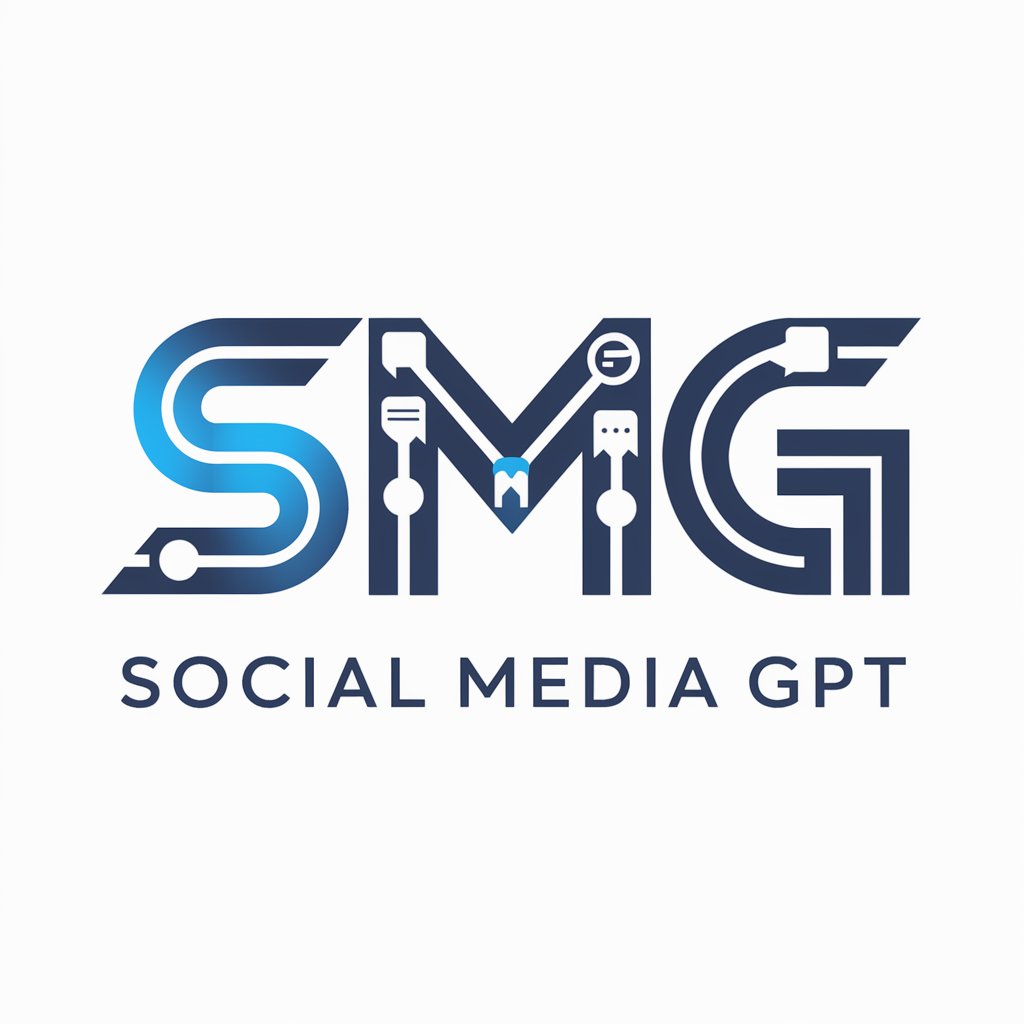
C Programming Q&A
What is C programming language?
C is a general-purpose programming language initially developed by Dennis Ritchie in the early 1970s. It is widely used for system programming, embedded systems, and application development.
What are the main features of C?
C is known for its simplicity, efficiency, portability, and flexibility. It provides low-level access to memory, a rich set of operators, and a versatile standard library.
How do I declare variables in C?
In C, variables are declared by specifying the data type followed by the variable name. For example, 'int x;' declares an integer variable named 'x'.
What is the difference between 'printf' and 'scanf' in C?
'printf' is used to print formatted output to the screen, while 'scanf' is used to read formatted input from the keyboard.
How do I allocate memory dynamically in C?
Memory can be allocated dynamically in C using functions such as 'malloc', 'calloc', and 'realloc'. Remember to free dynamically allocated memory using the 'free' function when it is no longer needed to avoid memory leaks.