Django Rest API - Django Rest API for easy API development
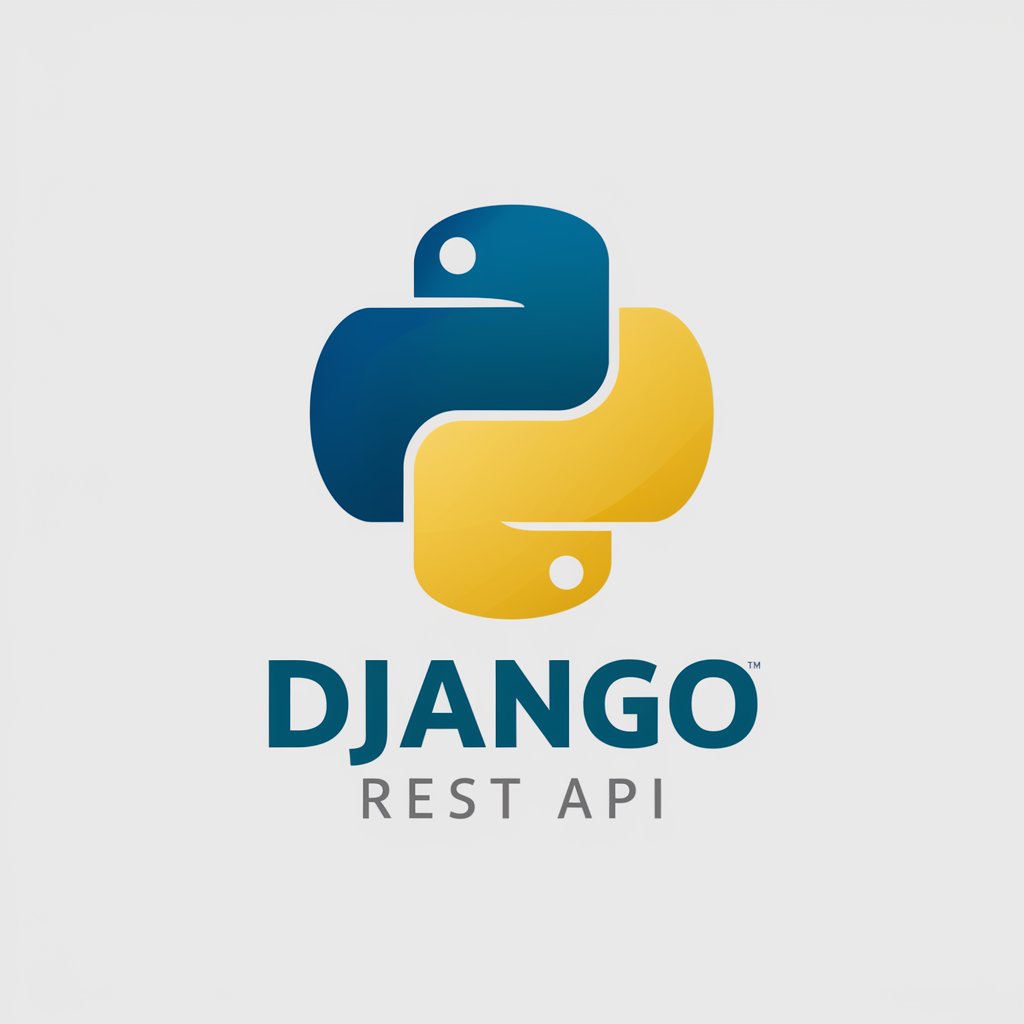
Hello! I'm here to assist with all your Python and Django needs.
Empower Your Apps with AI-Driven APIs
Explain how to set up a Django project from scratch...
What are the best practices for writing Django views...
How can I optimize database queries in Django...
Describe the process of creating RESTful APIs using Django...
Get Embed Code
Introduction to Django Rest API
Django Rest Framework (DRF) is a powerful toolkit for building Web APIs in Python using the Django framework. Its primary purpose is to simplify the process of creating RESTful APIs by providing a set of flexible, reusable components. DRF is built on top of Django and follows its design patterns, making it seamlessly integrate with Django projects. It offers serialization, authentication, and other features out of the box, allowing developers to focus on building API endpoints rather than boilerplate code. DRF follows the principles of DRY (Don't Repeat Yourself) and encourages best practices such as separation of concerns and modularity. Let's illustrate these aspects with an example: Suppose you want to create a simple API for managing a list of books. Using DRF, you can define a Book model, create serializers to convert between Python objects and JSON representations, and define API views to handle CRUD operations. With just a few lines of code, you can have a fully functional API endpoint for managing books, complete with pagination, filtering, and authentication. Powered by ChatGPT-4o。
Main Functions of Django Rest API
Serialization
Example
class BookSerializer(serializers.ModelSerializer): class Meta: model = Book fields = ['id', 'title', 'author', 'publication_date']
Scenario
Serialization is a key function of DRF that allows you to convert complex data types, such as Django model instances, into native Python data types suitable for rendering as JSON responses. In the example provided, a BookSerializer class is defined to serialize Book model instances into JSON format, including specific fields.
Views and Viewsets
Example
class BookViewSet(viewsets.ModelViewSet): queryset = Book.objects.all() serializer_class = BookSerializer
Scenario
DRF provides powerful class-based views and viewsets that handle common CRUD operations out of the box. In the example, a BookViewSet class is defined, which inherits from ModelViewSet to automatically provide CRUD functionality for the Book model. This eliminates the need to write repetitive view code for each CRUD operation.
Authentication and Permissions
Example
class IsOwnerOrReadOnly(permissions.BasePermission): def has_object_permission(self, request, view, obj): if request.method in permissions.SAFE_METHODS: return True return obj.owner == request.user
Scenario
DRF offers robust authentication and permission systems to secure your APIs. Custom permissions can be defined to control access to specific resources based on user roles or other criteria. In the example, a custom permission class IsOwnerOrReadOnly is created to allow read-only access to objects for non-owners while restricting write access to the owner.
Ideal Users of Django Rest API
Web Developers
Web developers who are building web applications or services that require RESTful APIs can greatly benefit from using Django Rest Framework. DRF provides a comprehensive set of tools and conventions for rapidly developing APIs, reducing development time and effort. It is particularly suitable for developers familiar with Django, as it leverages Django's ORM, authentication system, and other components.
Mobile App Developers
Mobile app developers who need to create backend APIs to support their mobile applications can find DRF invaluable. DRF simplifies the process of building RESTful APIs, allowing developers to focus on designing efficient communication between the mobile app and the server. With DRF, mobile app developers can quickly prototype, test, and deploy APIs to support their apps on various platforms.
API Service Providers
Companies or individuals offering API-based services or platforms can leverage Django Rest Framework to build robust, scalable APIs for their clients or users. DRF's flexibility, extensibility, and performance make it a popular choice for building APIs that serve diverse client needs. Whether it's a public API for third-party developers or an internal API for enterprise applications, DRF provides the tools necessary to create reliable APIs.
Using Django Rest API
Visit yeschat.ai for a free trial without login, also no need for ChatGPT Plus.
Navigate to yeschat.ai to access Django Rest API without any login or subscription requirements.
Install Django and Django Rest Framework
Ensure Django and Django Rest Framework are installed in your Python environment using pip.
Create a Django project and app
Initiate a new Django project and app using the django-admin command.
Define models and serializers
Design your data models and create serializers to convert them to JSON format.
Implement views and URLs
Write views to handle HTTP requests and define corresponding URLs in your Django app's urls.py file.
Try other advanced and practical GPTs
Mental Health Therapist
Streamlining Therapy Documentation with AI
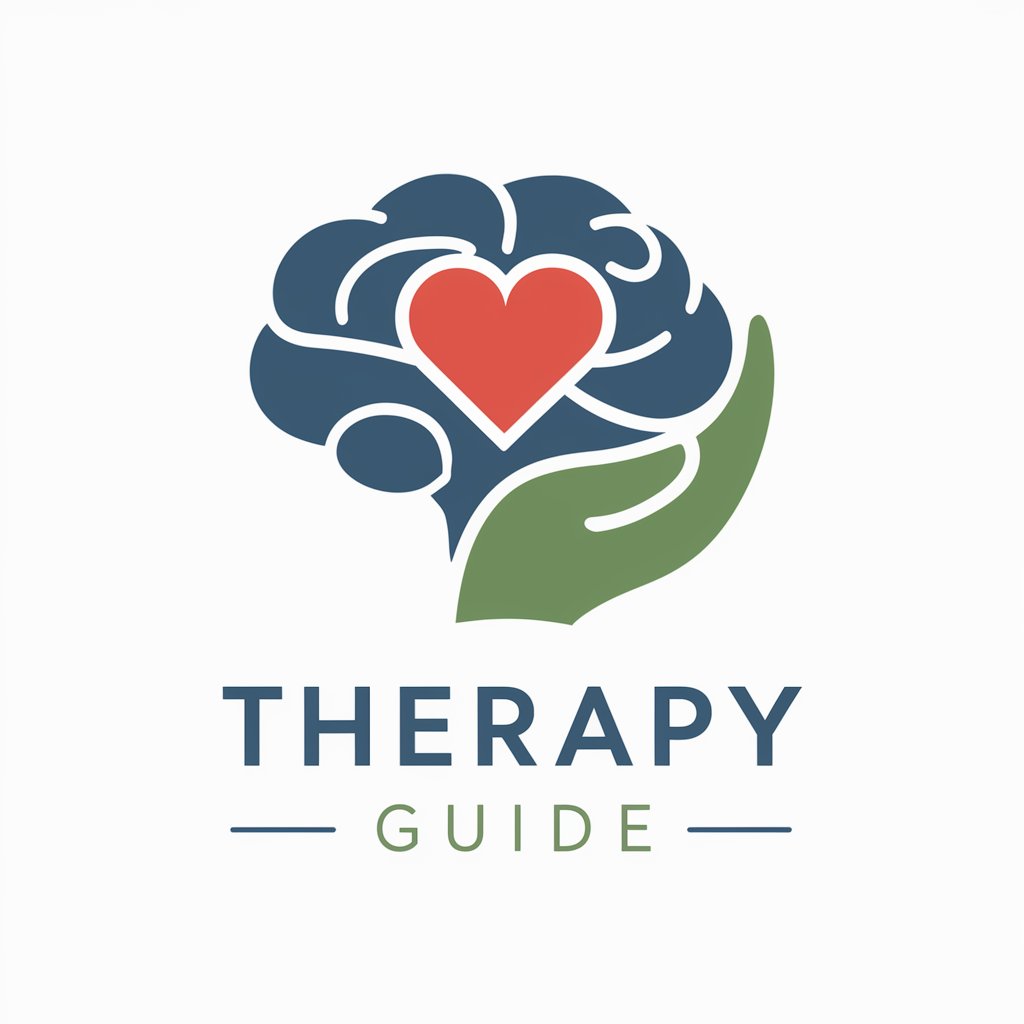
Economic Educator
Unlock Economics with AI
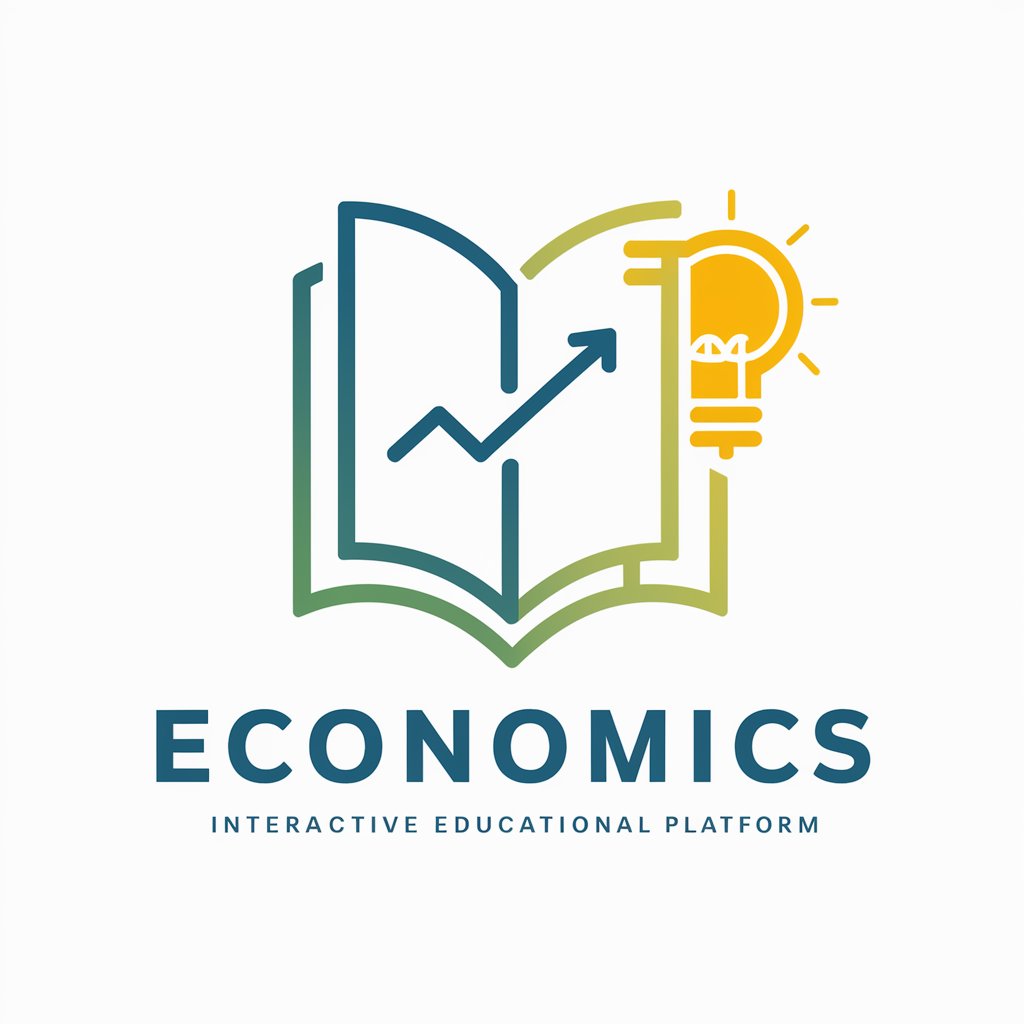
Direct Response
Unlock knowledge with AI precision
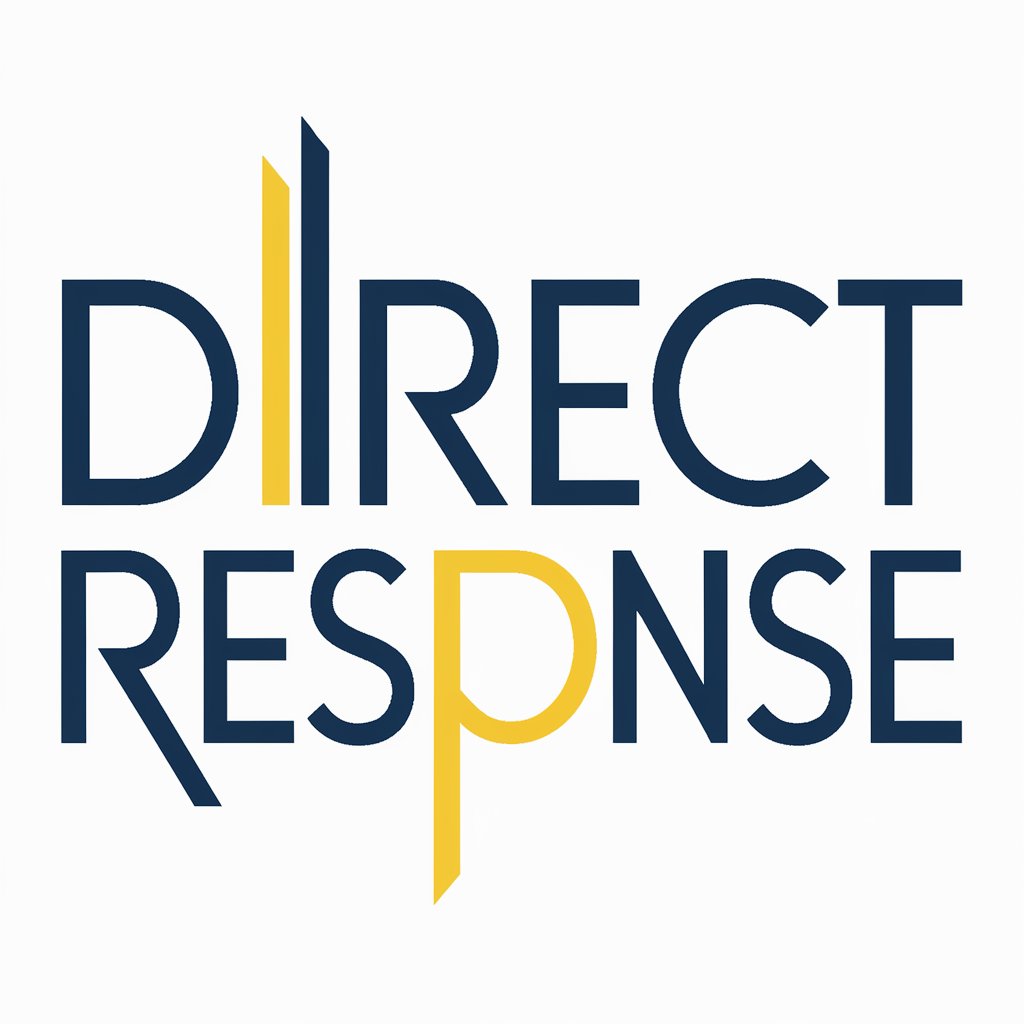
Crossfit Nutrition Coach
Empower Your Fitness with AI-Driven Nutrition
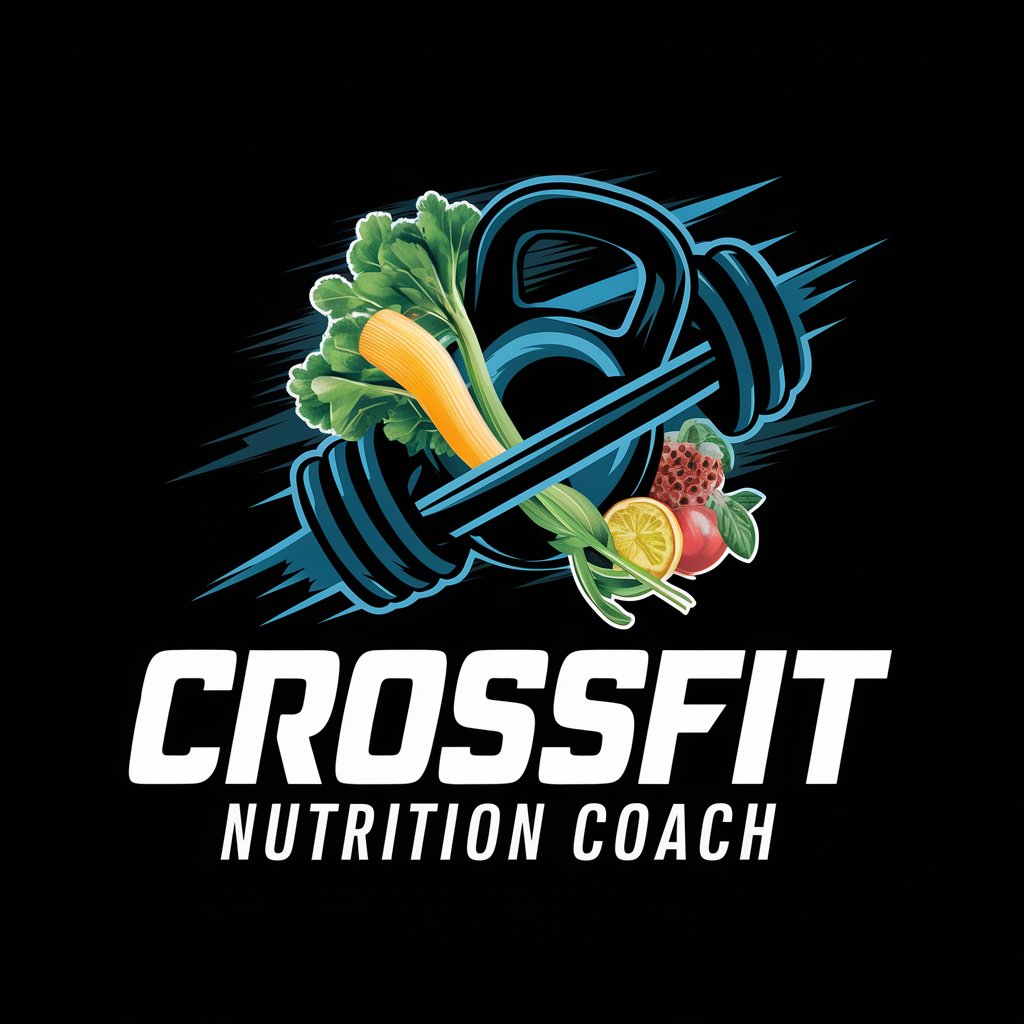
Expand Text
Elevate Your Text with AI
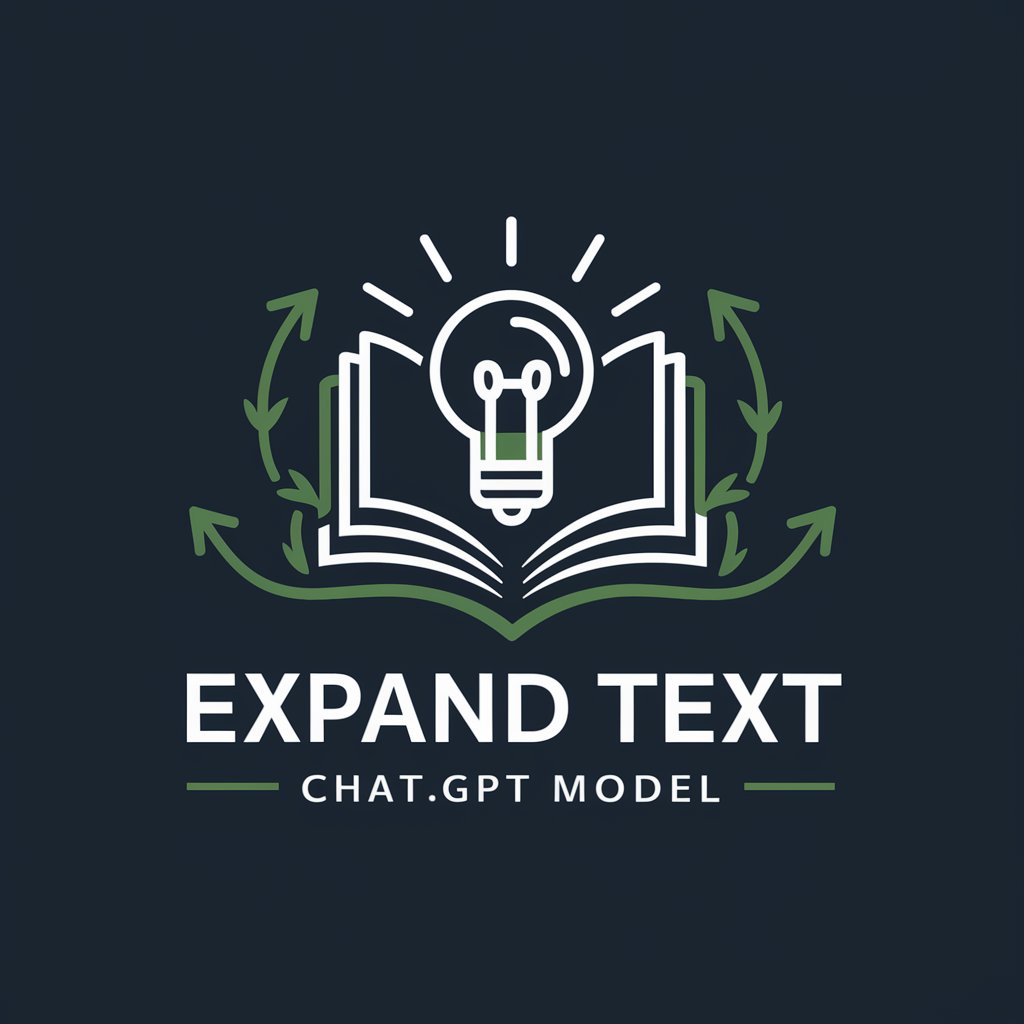
Meeting Summary and Action Item Specialist
Efficient Meeting Summaries with AI-Power
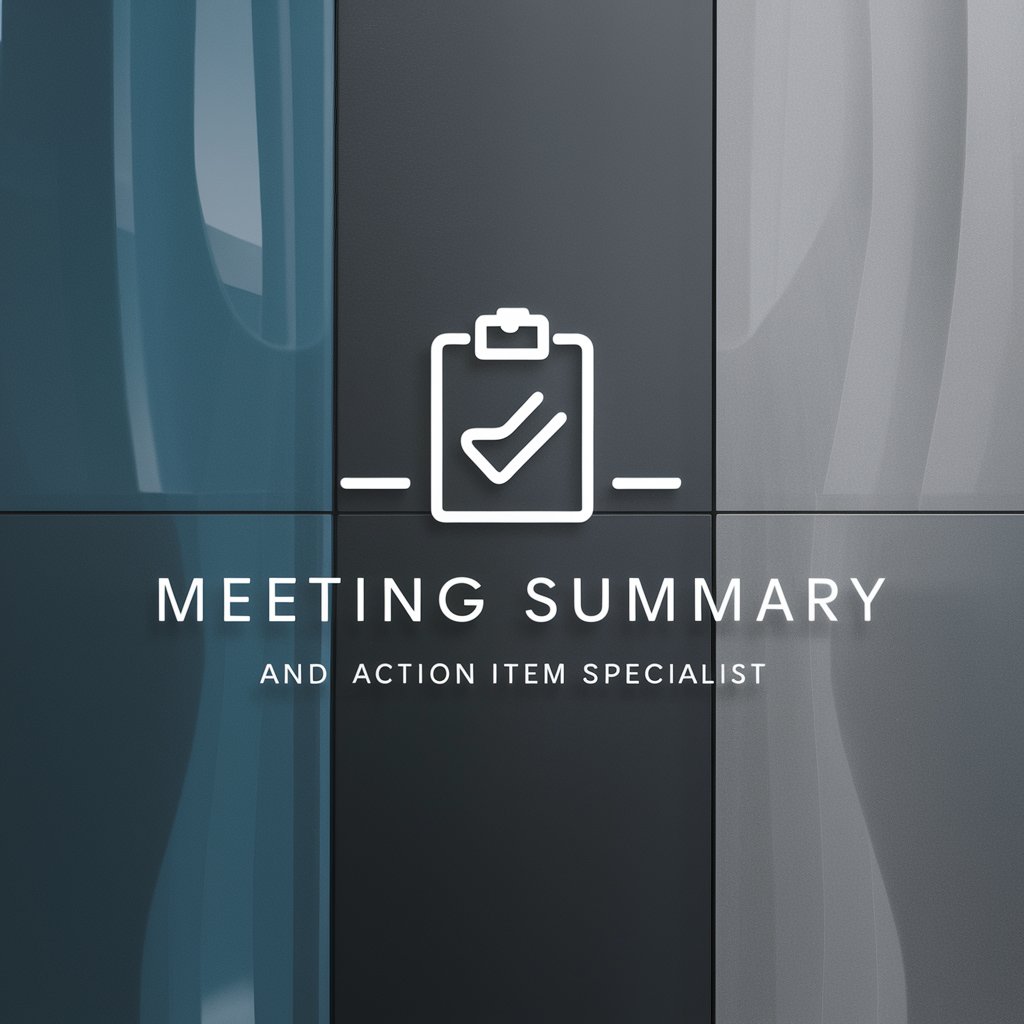
Your Writing Assistant, Simplified
Refine Your Writing with AI Precision
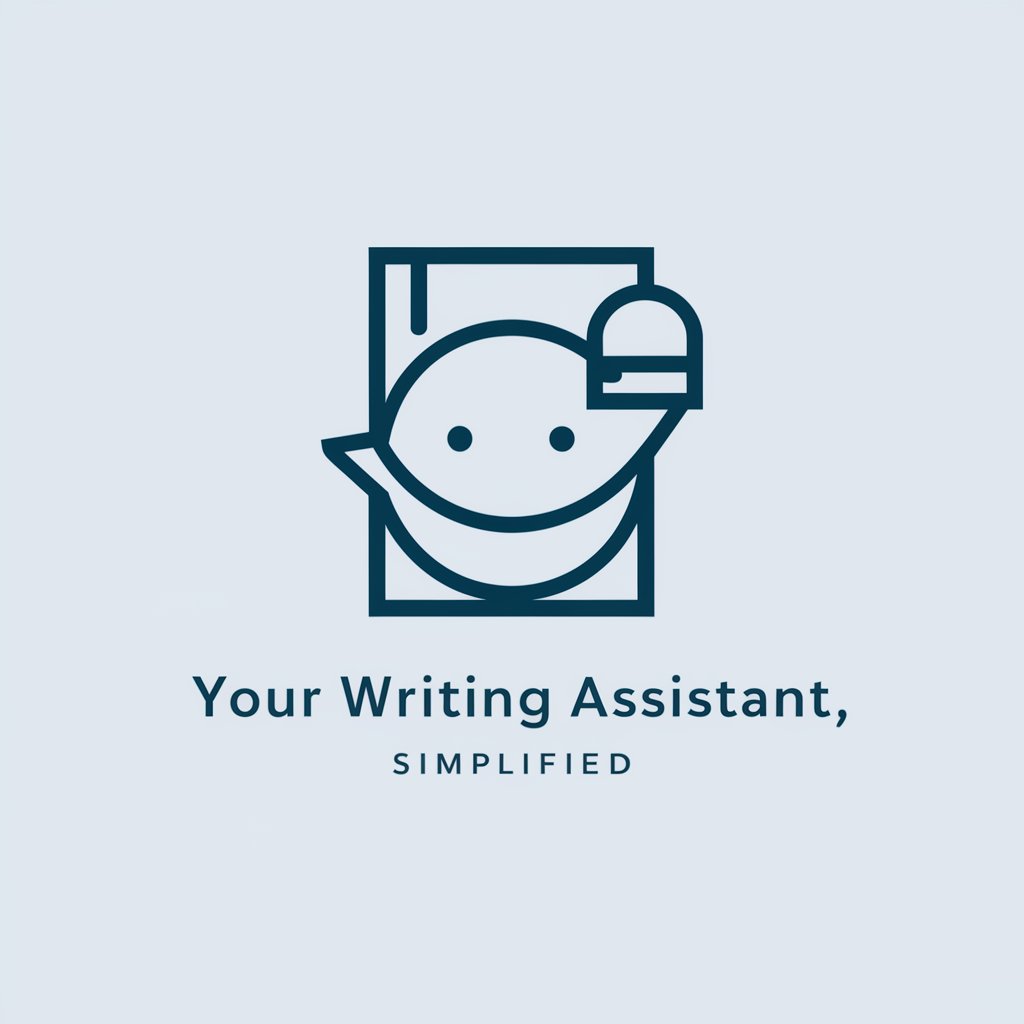
UML state diagram generator
Visualize System Behavior with AI-Powered UML State Diagram Generator
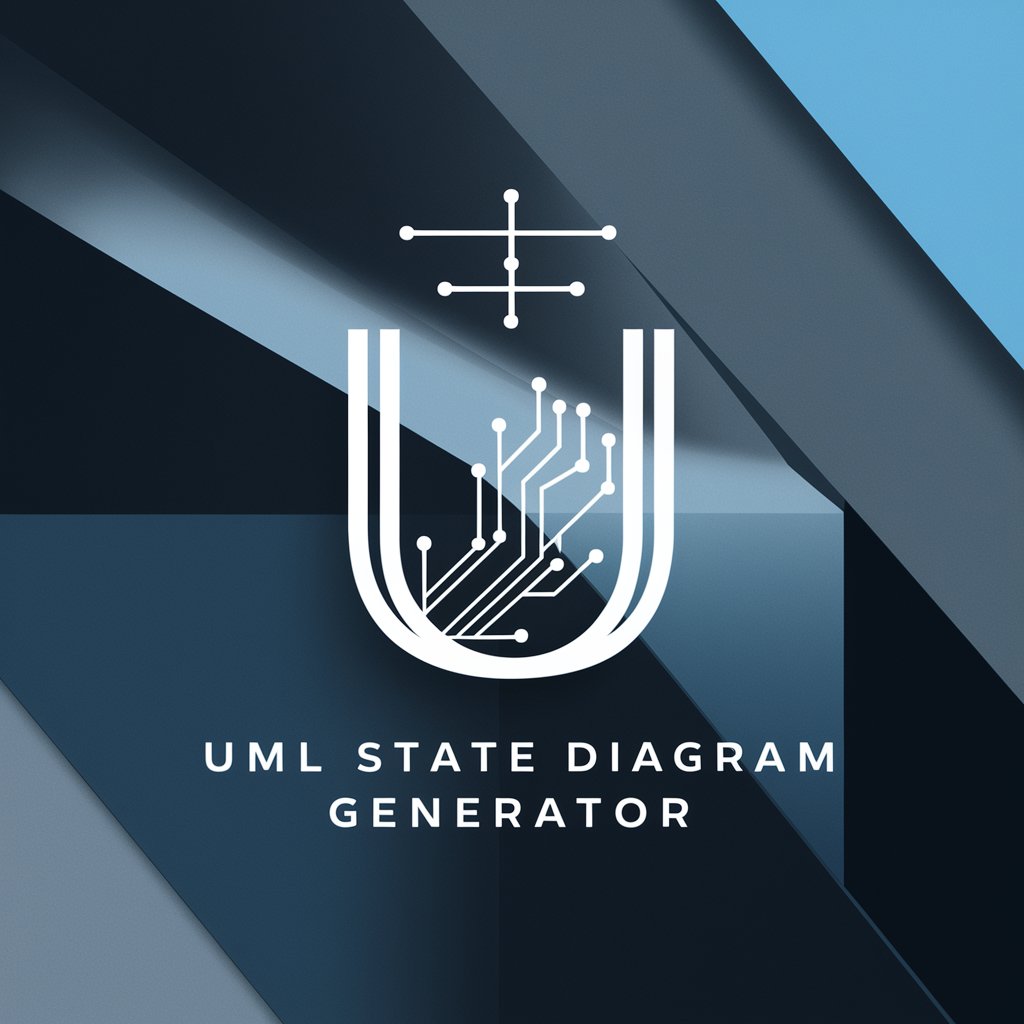
JUCE Coder
Unlock AI-driven coding efficiency.
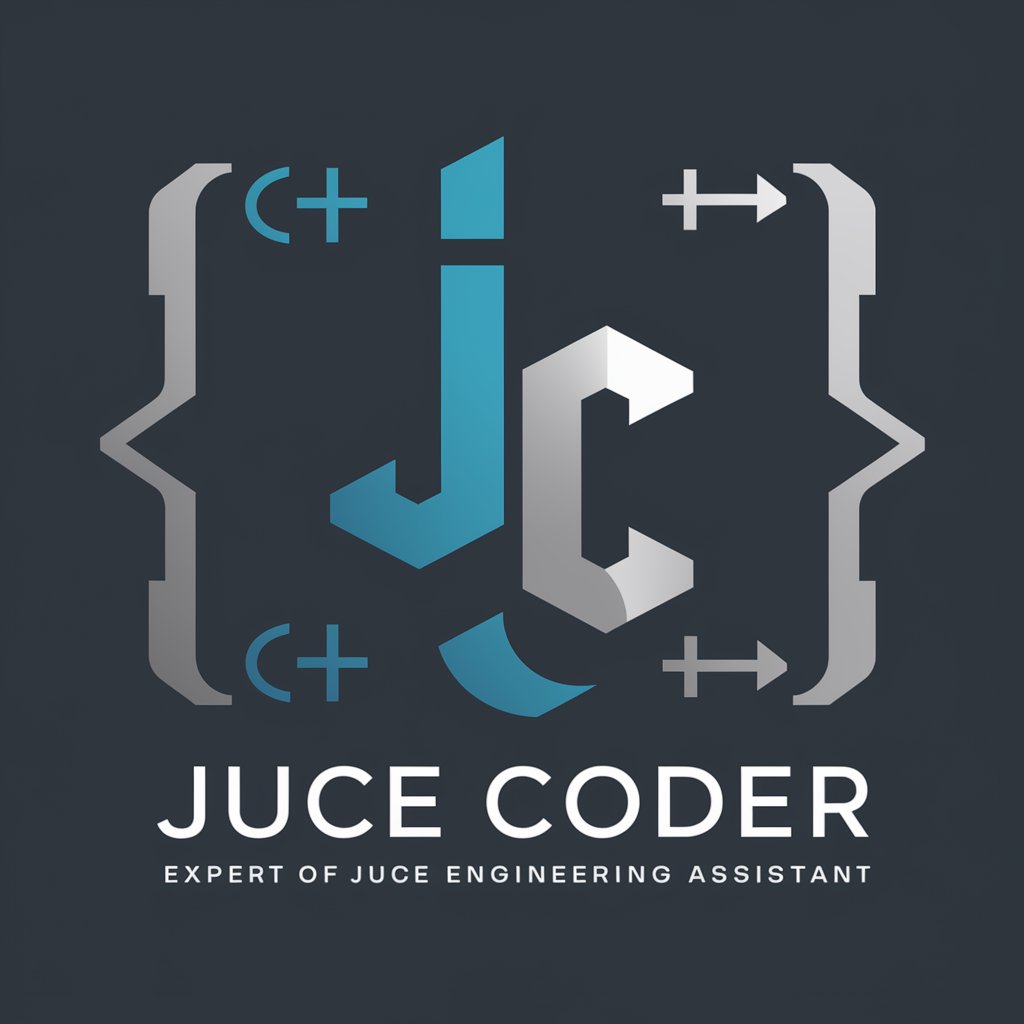
Search Internet
Unlock the power of AI-driven internet search.
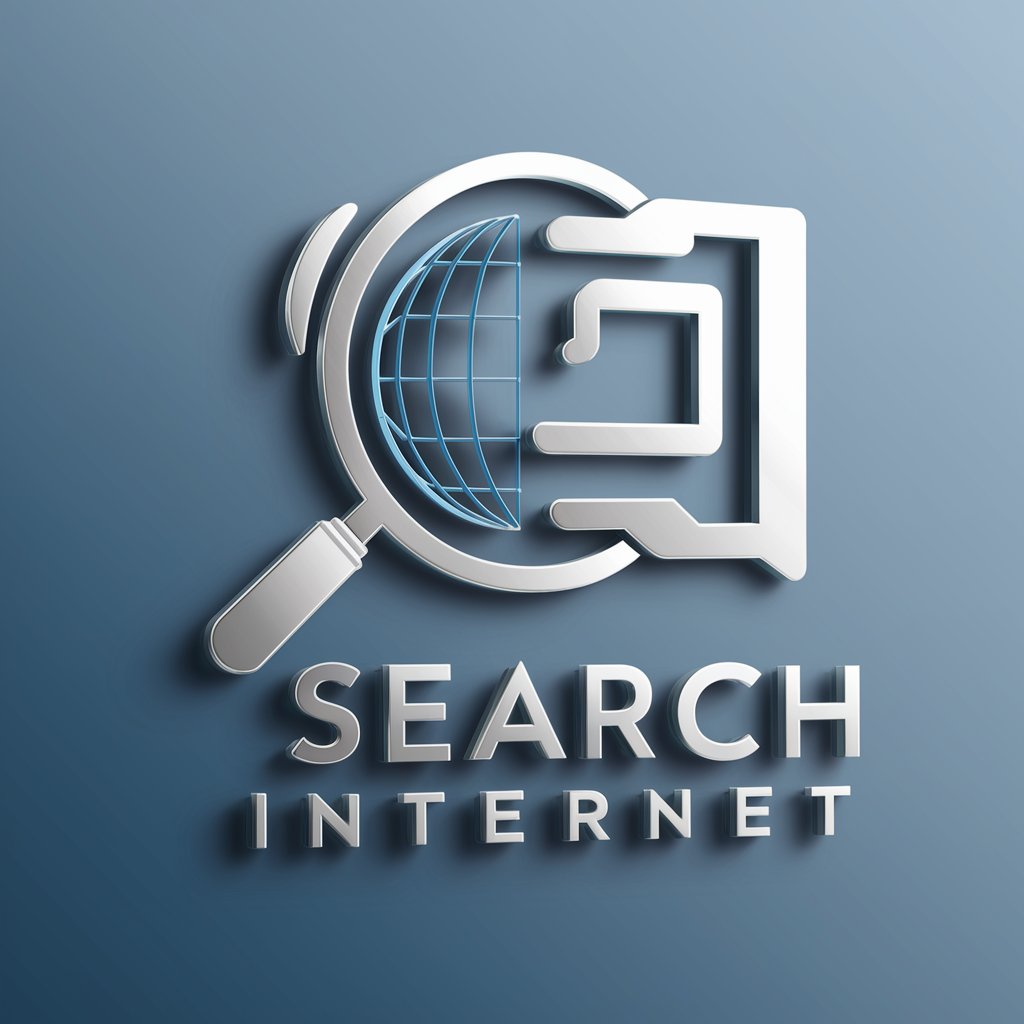
Trial
Empowering communication through AI translation
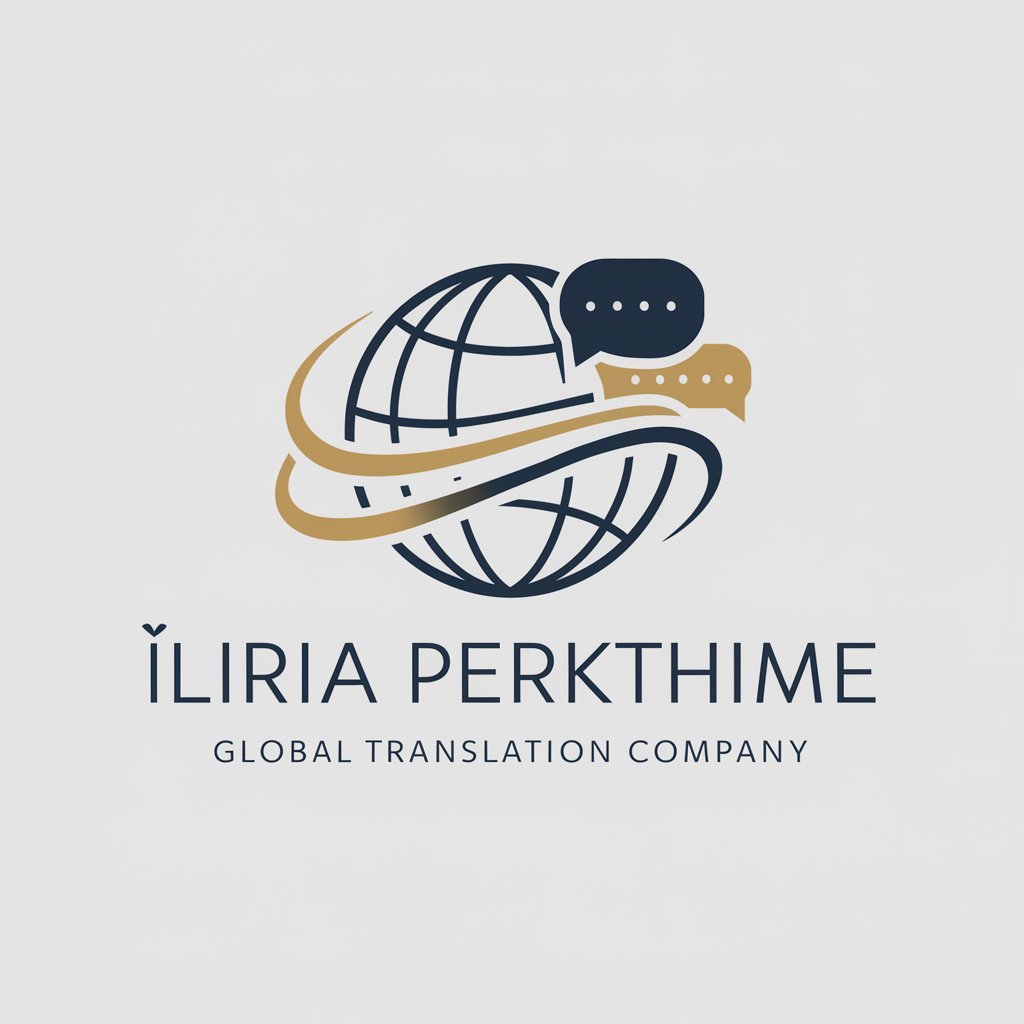
Translations ( trial )
Empower your communication with AI translation.
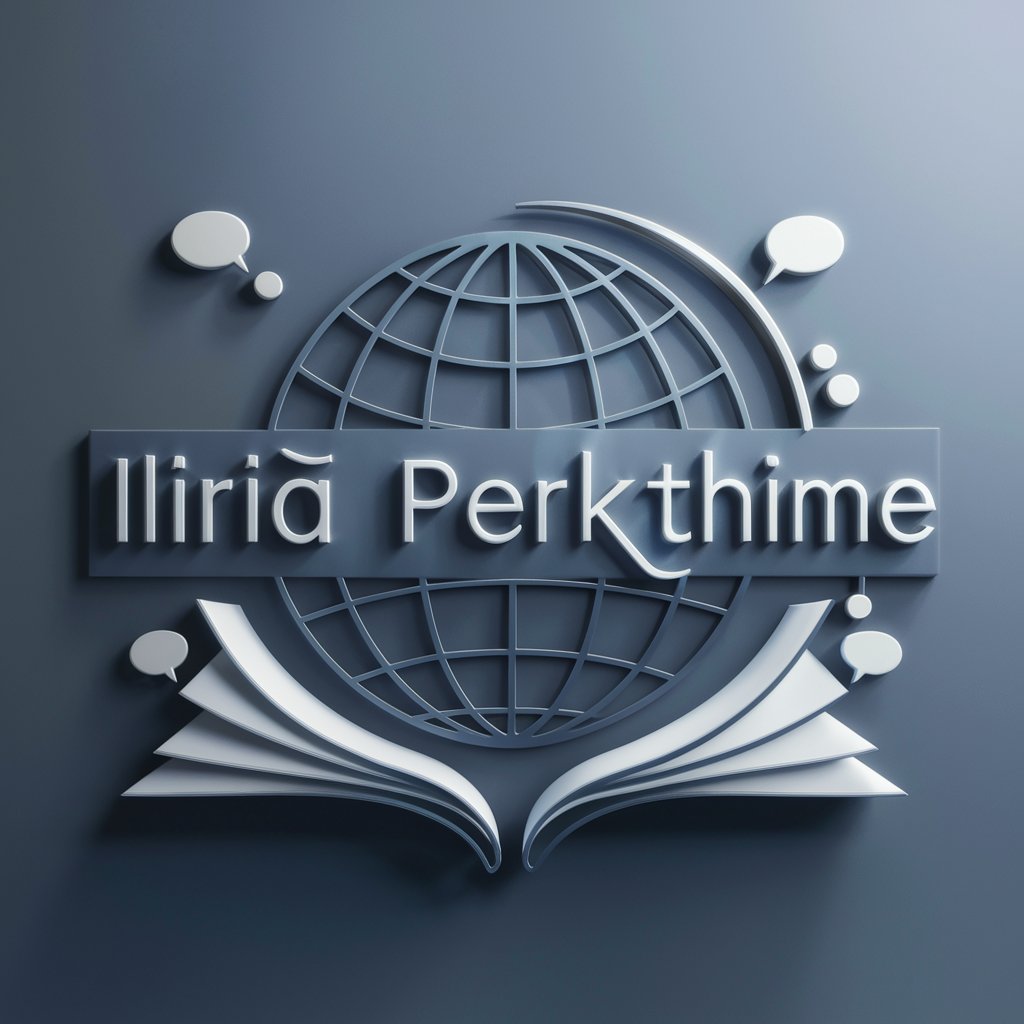
Django Rest API Q&A
What is Django Rest Framework?
Django Rest Framework is a powerful toolkit for building Web APIs in Django. It provides tools for serialization, authentication, permissions, viewsets, and more, making it easy to create robust APIs.
How do I authenticate users in Django Rest Framework?
Django Rest Framework supports various authentication methods such as session authentication, token authentication, and OAuth. You can choose the appropriate method based on your application's requirements.
Can Django Rest Framework handle file uploads?
Yes, Django Rest Framework can handle file uploads by using the FileUploadParser class. You can define a serializer with a FileField and configure your views to accept file uploads.
What are viewsets in Django Rest Framework?
Viewsets in Django Rest Framework are classes that provide CRUD (Create, Retrieve, Update, Delete) operations for a set of related resources. They combine the functionality of views and URL routing.
How can I customize the response format in Django Rest Framework?
You can customize the response format in Django Rest Framework by defining custom renderers. Renderers allow you to control the output format, such as JSON, XML, or HTML, for different types of requests.