React Testing Library & Jest - React UI testing tool
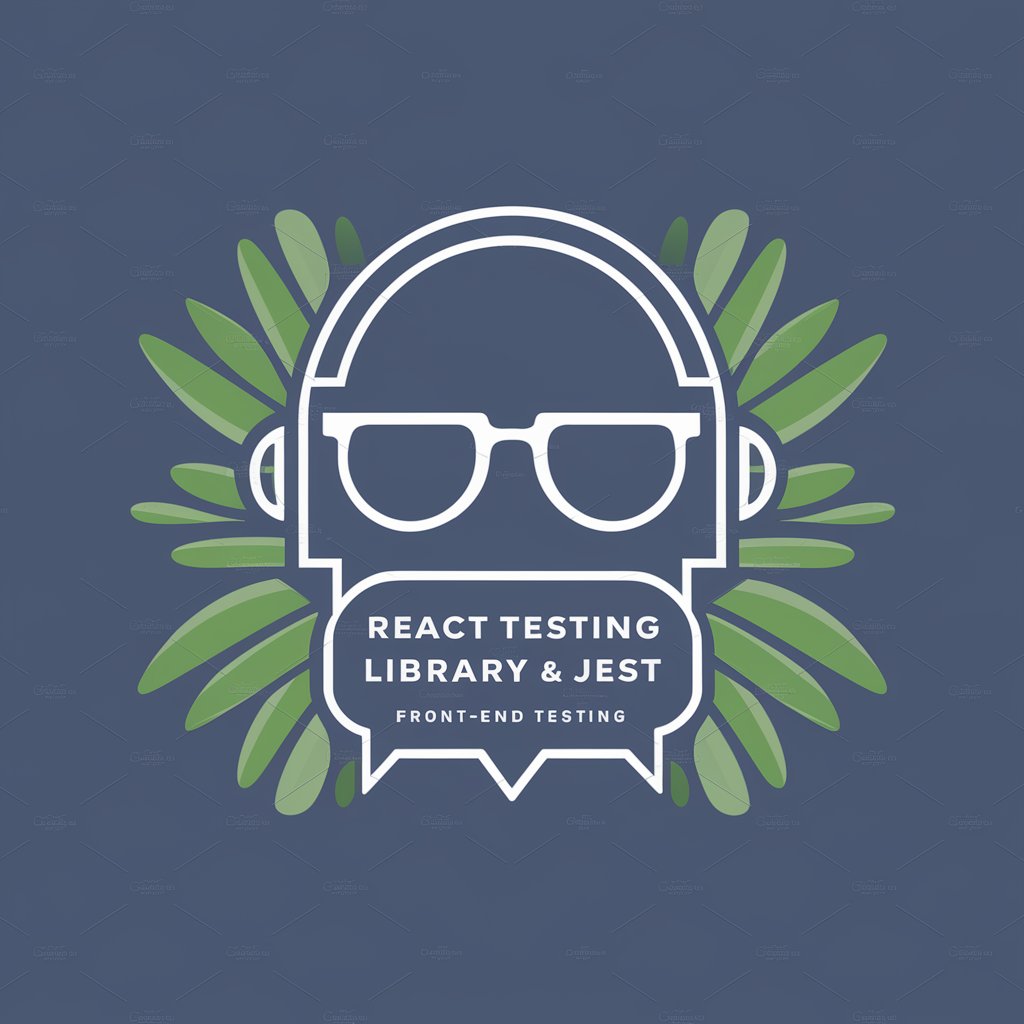
Welcome! Let's dive into expert front-end testing with React and Jest.
AI-powered React component testing
Design a modern logo for an AI assistant that specializes in...
Create a professional logo that represents an expert in React Testing Library...
Generate a logo that conveys reliability and precision for a front-end testing assistant...
Craft a logo for a tech-savvy AI assistant focused on testing with React and Jest...
Get Embed Code
Introduction to React Testing Library & Jest
React Testing Library (RTL) and Jest are popular tools used together to perform robust testing of React applications. RTL is designed to interact with your React components as a user would, by querying and manipulating the DOM in a way that mimics user behavior. It encourages testing in a way that focuses on the functionality of components rather than their implementation details. Jest, on the other hand, is a testing framework that provides a complete environment for JavaScript testing, including test runner, assertion functions, and mocks. It works seamlessly with RTL to simulate React components under test conditions. For example, a common scenario might involve using RTL to render a component and Jest to handle assertions and simulate user events like clicking or typing. Powered by ChatGPT-4o。
Main Functions of React Testing Library & Jest
Rendering components
Example
render(<Button />)
Scenario
Used to mount a React component in a virtual DOM for testing purposes. This allows developers to perform assertions or trigger events on the component as if it were running in a real browser environment.
Simulating user events
Example
fireEvent.click(screen.getByRole('button'))
Scenario
Enables simulating user interactions such as clicks, typing, or form submissions. This is essential for verifying the interactive aspects of components, ensuring they respond as expected to user inputs.
Assertions
Example
expect(screen.getByText(/save/i)).toBeInTheDocument()
Scenario
In conjunction with Jest, RTL allows developers to write assertions about the state of the DOM after various interactions or lifecycle events, verifying that the component behaves correctly under different conditions.
Mocking modules and API calls
Example
jest.mock('axios')
Scenario
Jest can intercept and simulate API calls or other module functionality, which is crucial for testing components that depend on external data or configurations without relying on live endpoints.
Asynchronous testing
Example
await waitFor(() => expect(queryByText('Loading')).not.toBeInTheDocument())
Scenario
Both RTL and Jest handle asynchronous events, such as fetching data after user interaction or timers. This allows testing of components that depend on asynchronous data or actions.
Ideal Users of React Testing Library & Jest
Front-end developers
Developers who focus on building user interfaces with React will find RTL and Jest invaluable for ensuring their components function correctly across different scenarios and maintain quality as the application scales.
Quality assurance engineers
QA professionals who specialize in automated testing can use RTL and Jest to write comprehensive test suites that validate both the functionality and stability of React applications.
Project managers and tech leads
Leaders who oversee development teams benefit from the integration of RTL and Jest into their development process, as it helps ensure deliverables meet business requirements and reduces the cost of fixing bugs post-deployment.
Using React Testing Library & Jest
Initial Setup
Visit yeschat.ai for a free trial without a login, and no ChatGPT Plus is required.
Install Dependencies
Set up your development environment by installing Node.js, then use npm or yarn to install Jest and React Testing Library.
Write Tests
Create test files using the *.test.js convention. Structure your tests using the Arrange-Act-Assert pattern to maintain readability and consistency.
Run Tests
Execute your tests using Jest's command line interface, typically through npm test or yarn test, which will run all files that end in .test.js.
Refine and Repeat
Analyze test results to refine your code and tests. Use the coverage reports generated by Jest to identify untested parts of your application.
Try other advanced and practical GPTs
Networking Call Prep
Smart Networking, Smarter Connections
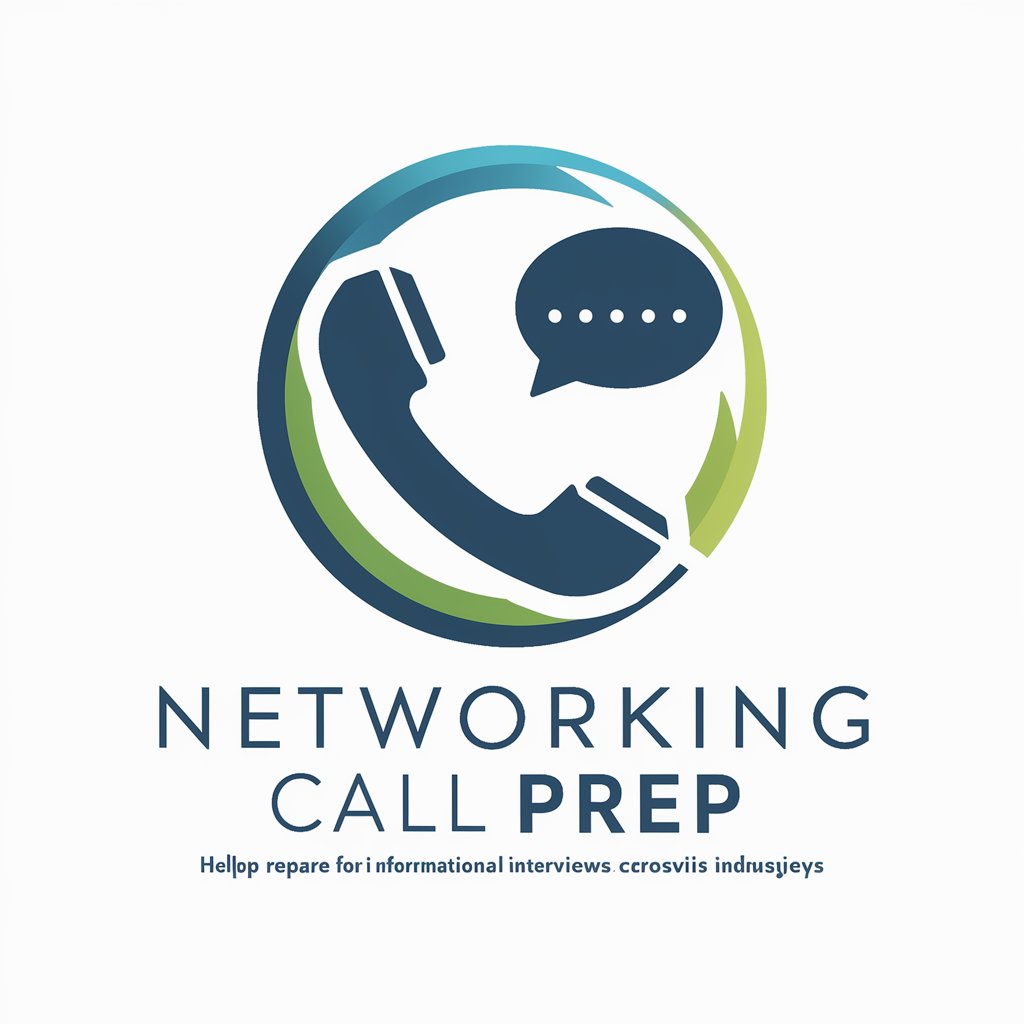
나만의 고민 상담 친구
Explore emotions with AI guidance
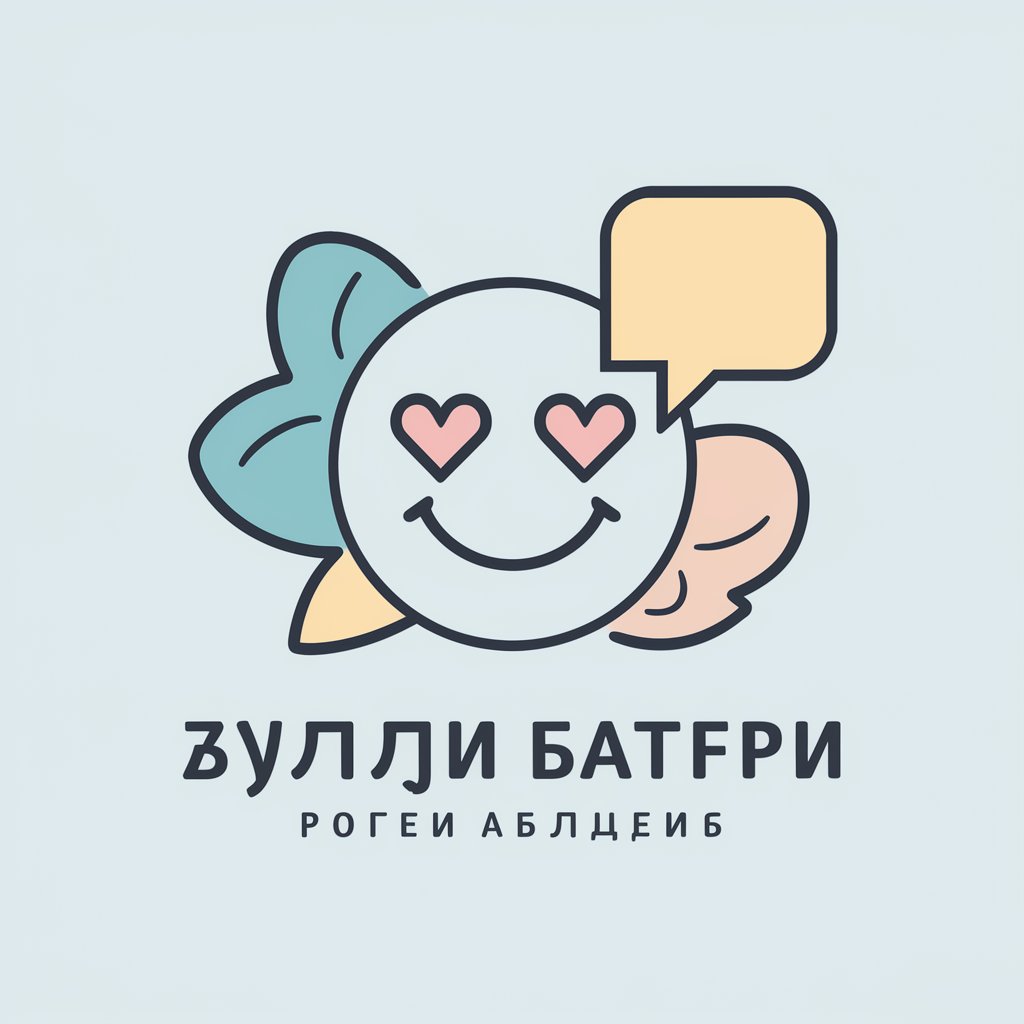
Ayuda con el texto
Empower your words with AI
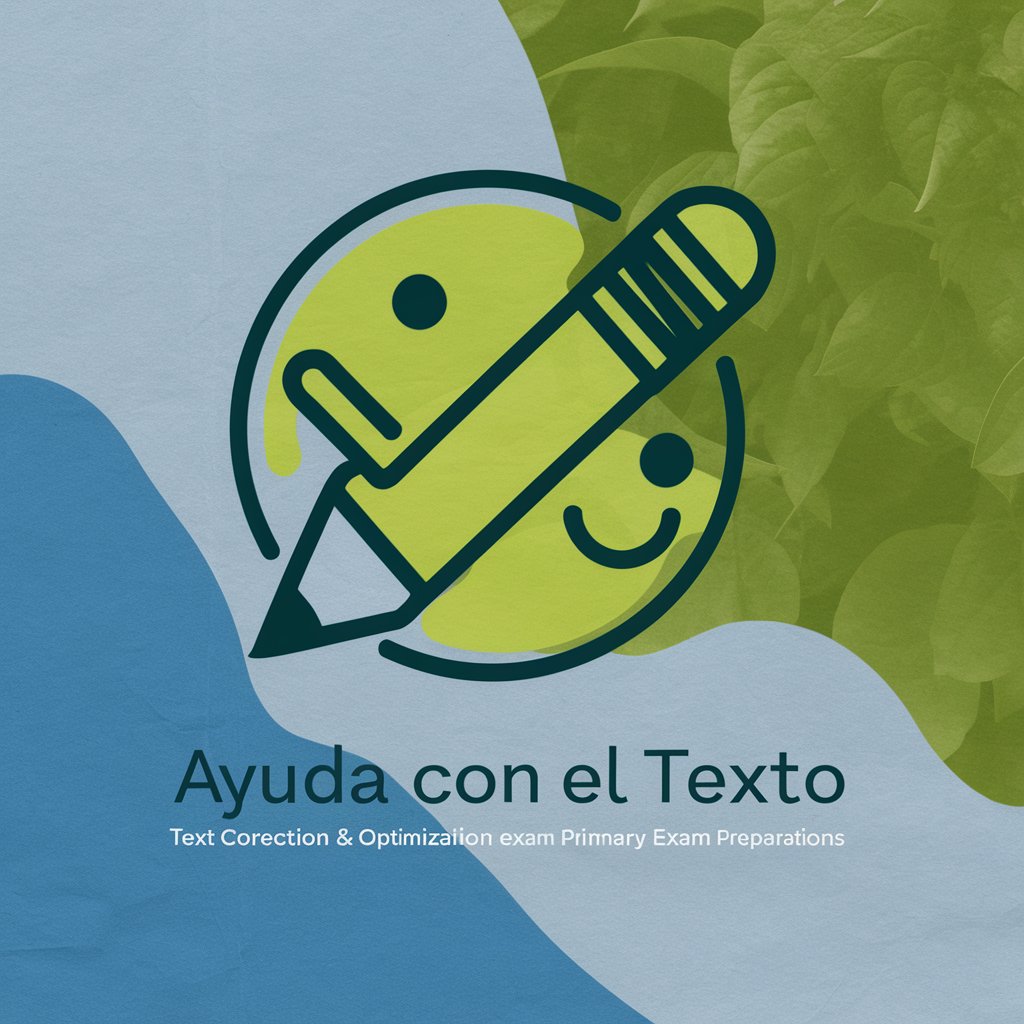
PPT 발표 대본 작성
Elevate Presentations with AI
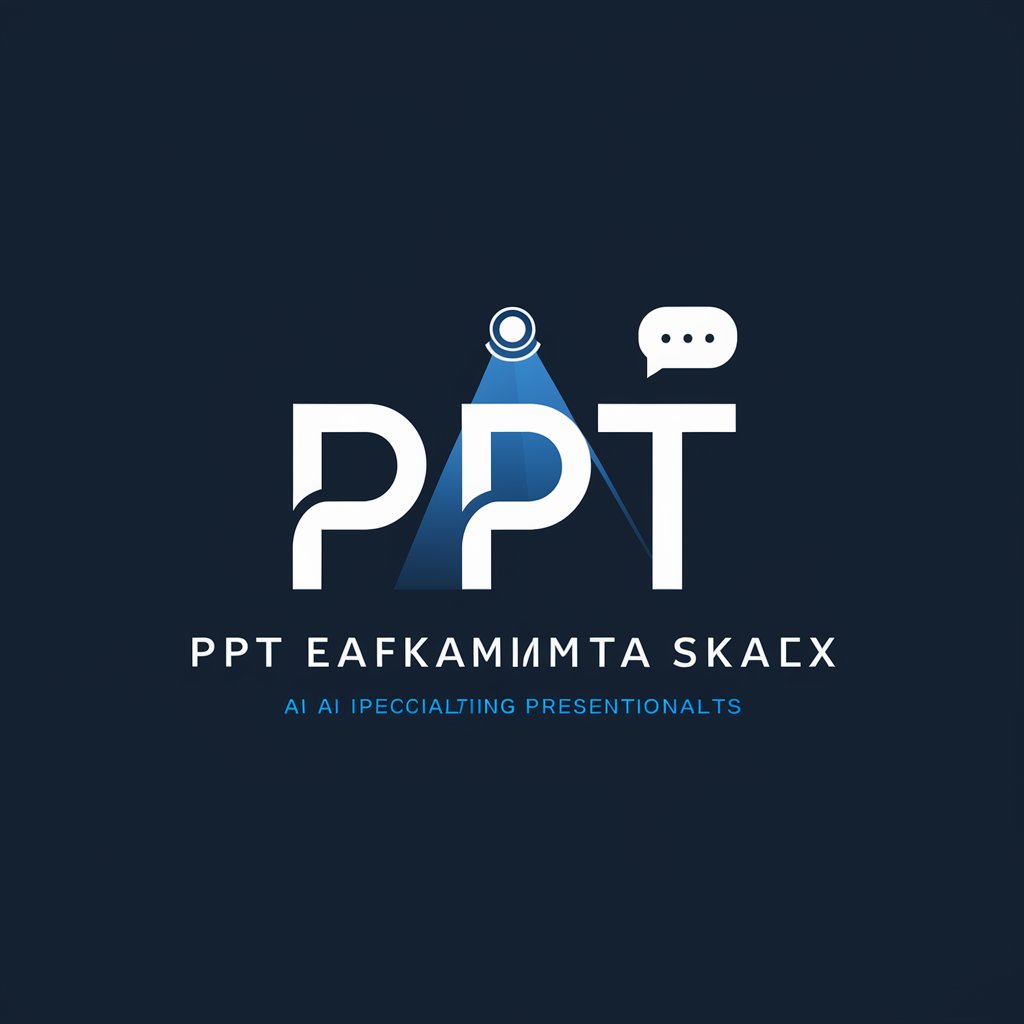
HOCHSCHUL aiMOOC
AI-powered academic learning modules
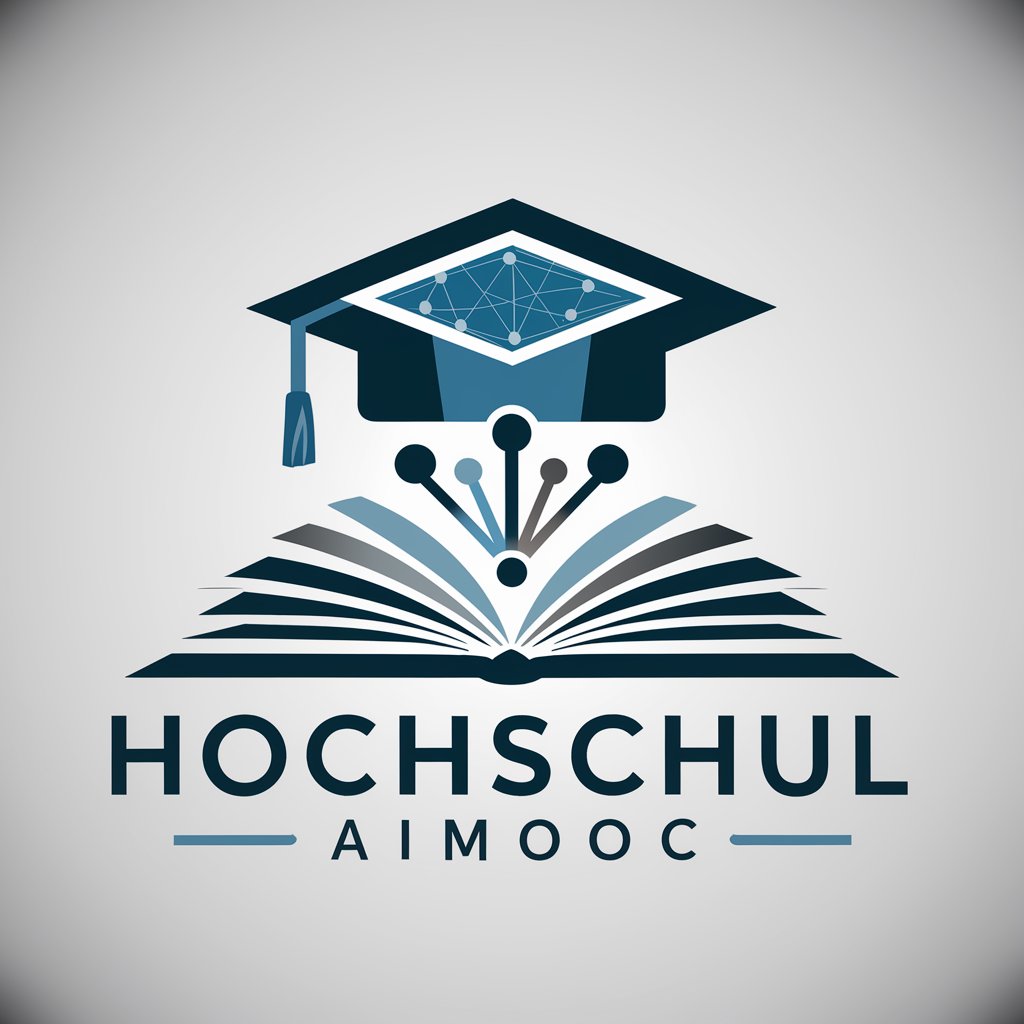
User Story per Interview erstellen
Empower Your Development with AI-Driven Stories
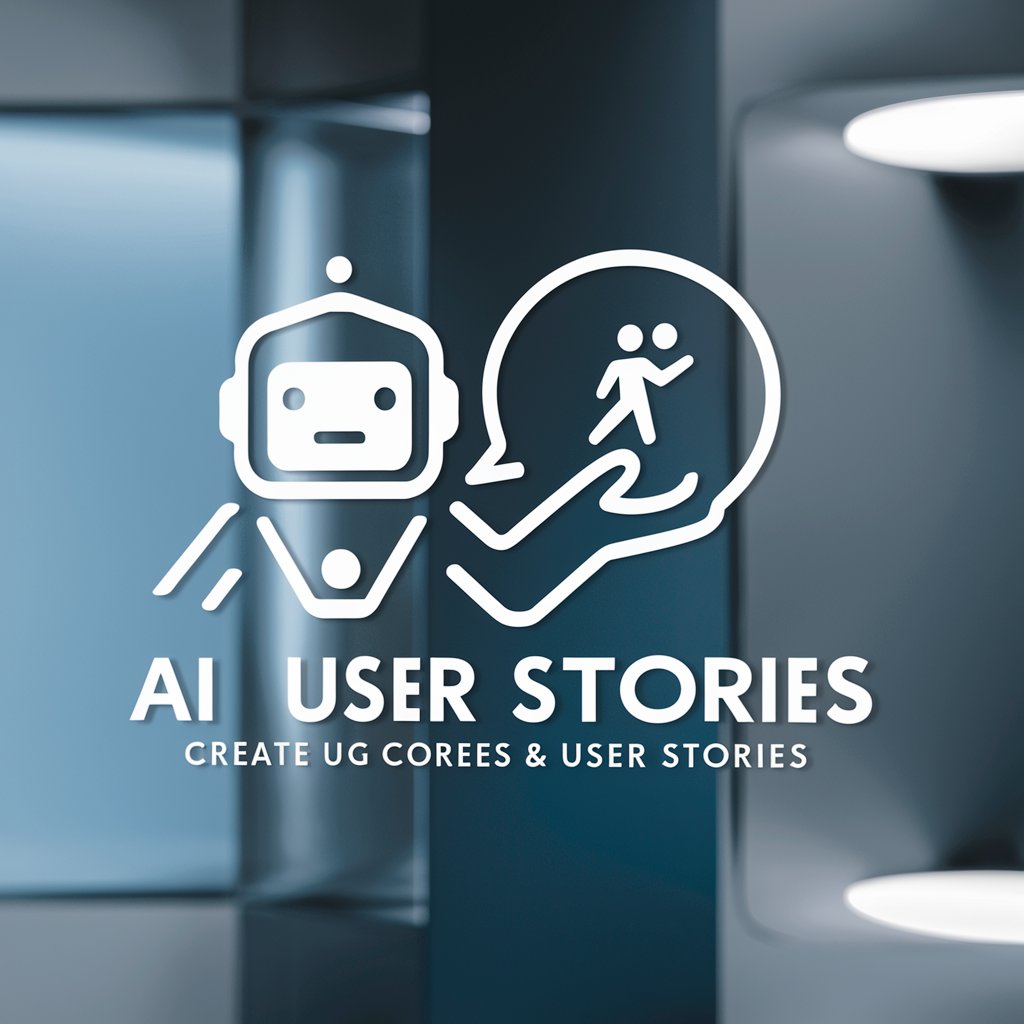
TCM
Comprehensive TCM insights, powered by AI.
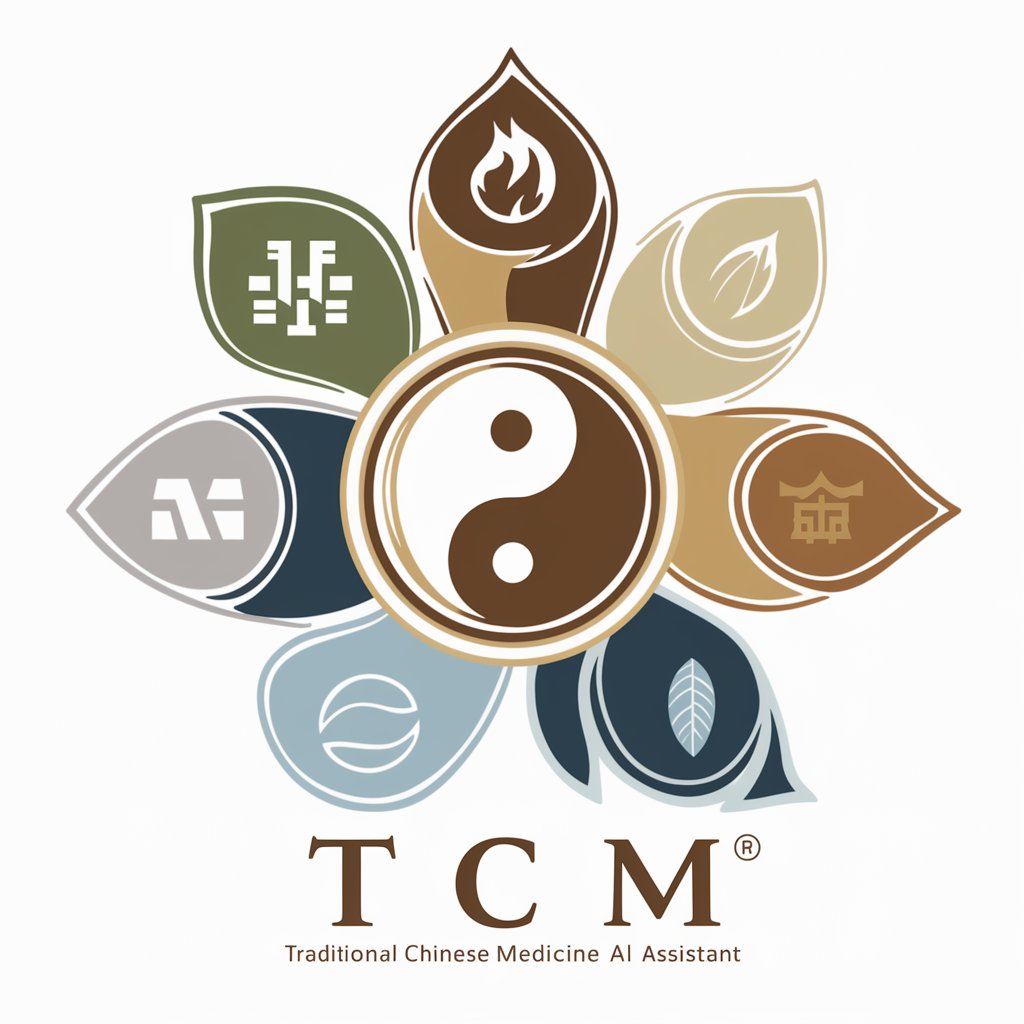
Affiliate Daily Deals
Empower Your Choices with AI
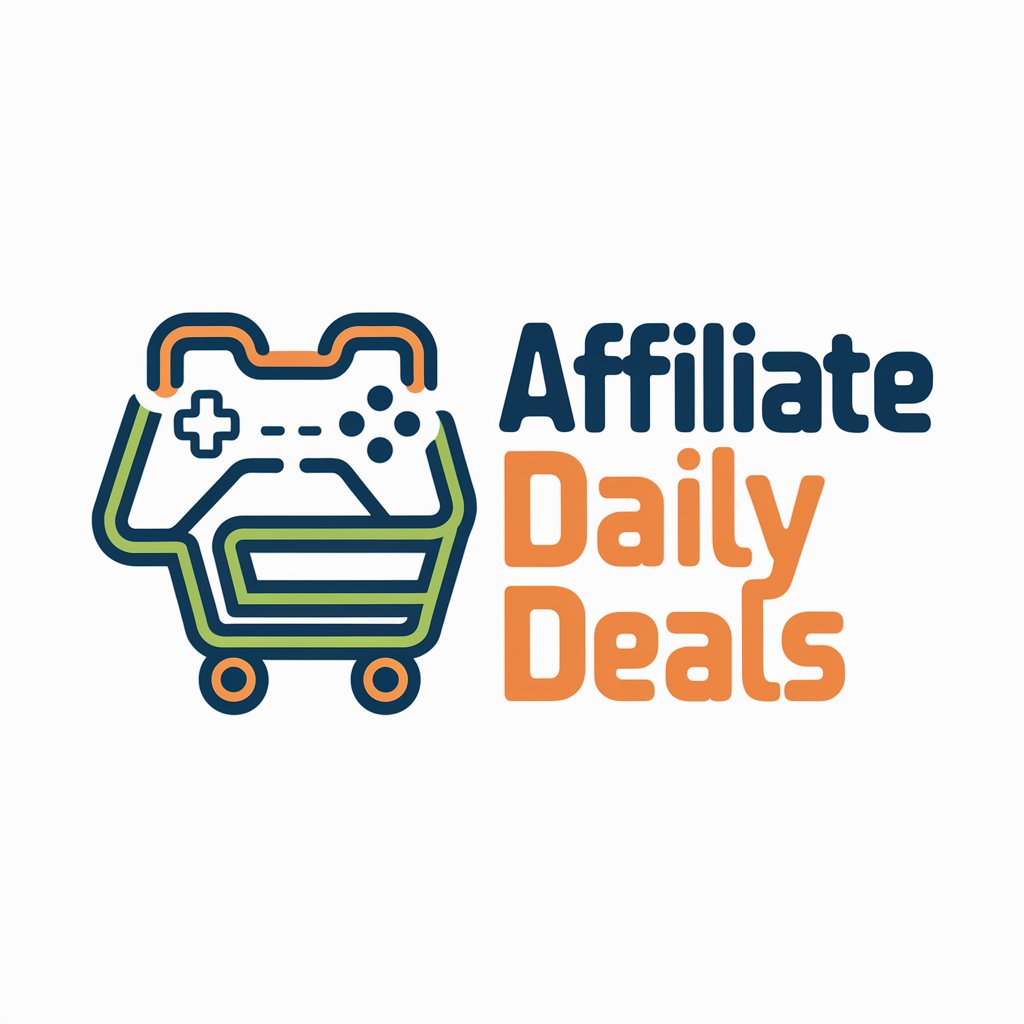
JW GPT
Enhance Your Spiritual Journey with AI
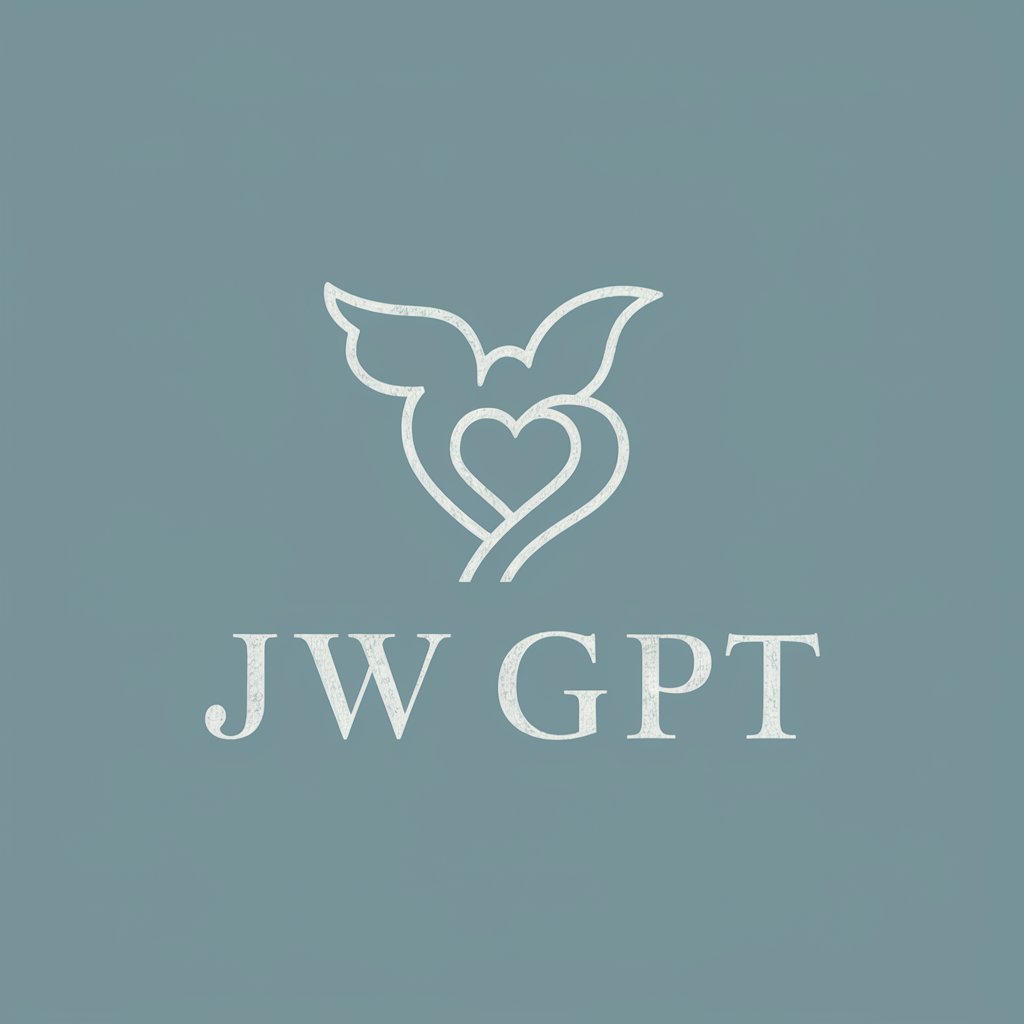
myText
Enhancing Text with AI Precision
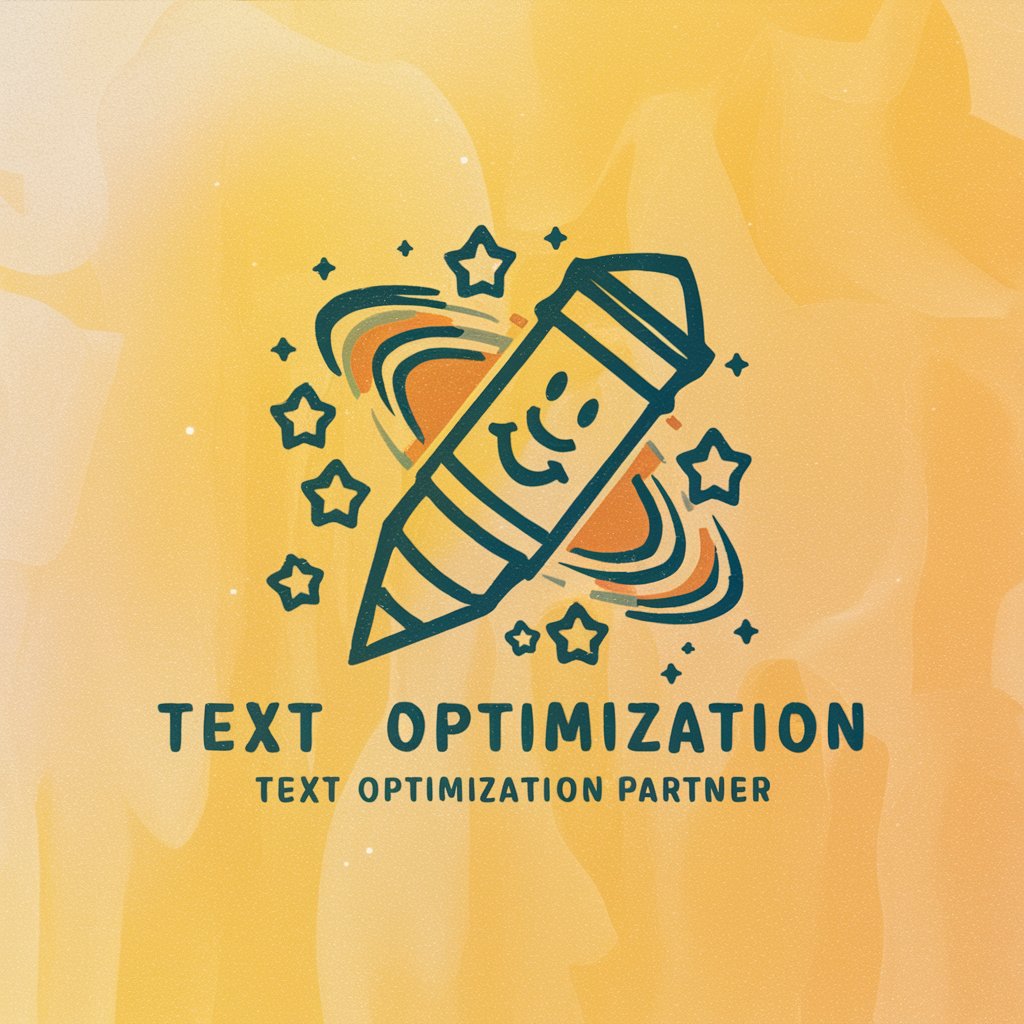
Serbian Translator
Instant Serbian translations, powered by AI
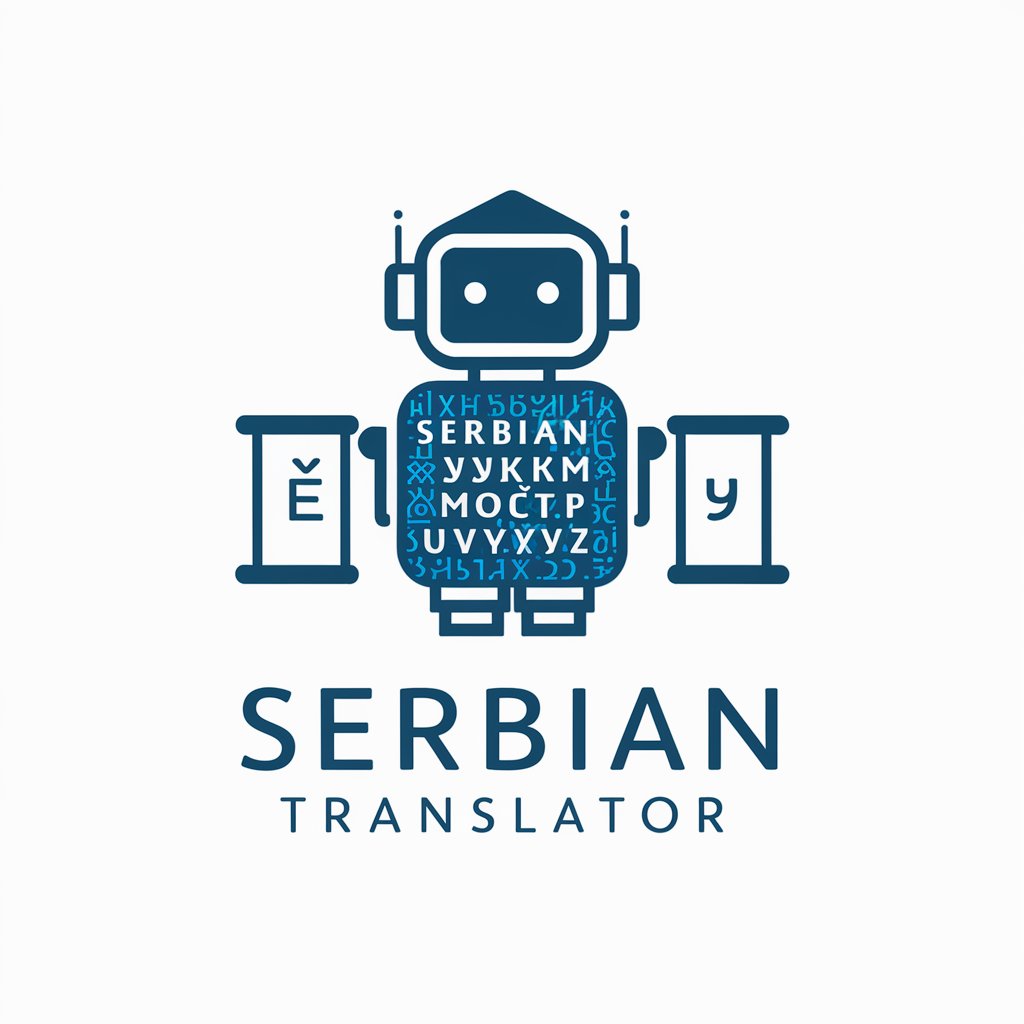
Economy News Analyzer
Stay Ahead with AI-Powered Economic Insights
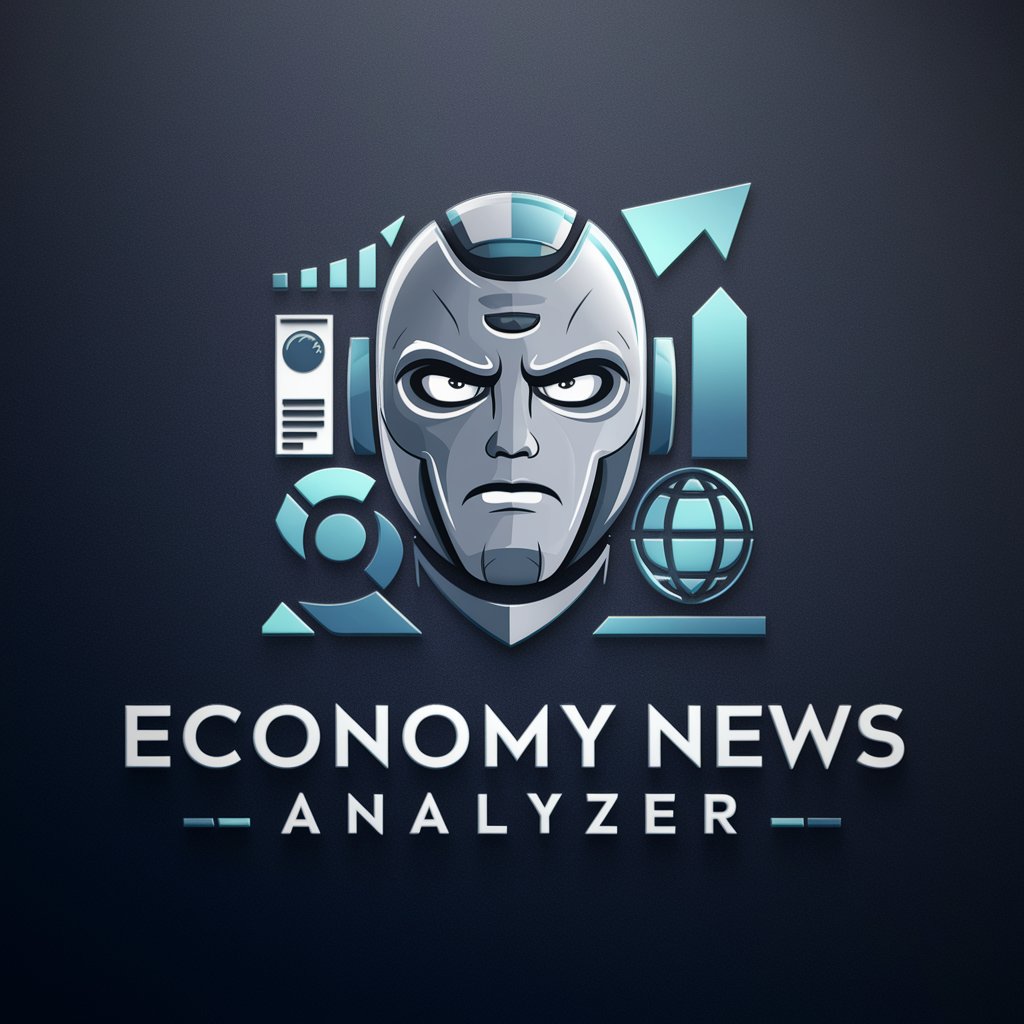
Common Questions on React Testing Library & Jest
What is the advantage of using React Testing Library?
React Testing Library promotes testing components in a way that resembles how users interact with the application, rather than testing implementation details.
How can I mock API calls in tests using Jest?
Jest allows you to mock functions, including API calls, using jest.mock() to intercept calls and provide custom responses, ensuring tests run quickly and deterministically.
What is the best practice for testing asynchronous components?
Use asynchronous utilities like waitFor or findBy queries in React Testing Library to handle components that depend on asynchronous operations like API calls or data fetching.
How do I test a component that uses Redux?
Wrap the component in a Provider with a mock store during testing. Tools like redux-mock-store can help simulate Redux state and actions in tests.
Can I use React Testing Library with TypeScript?
Yes, React Testing Library works well with TypeScript to provide type safety for your tests, enhancing reliability and developer experience.