JAVA-Java optimization tool for developers.
Unlocking Java's potential with AI insights.
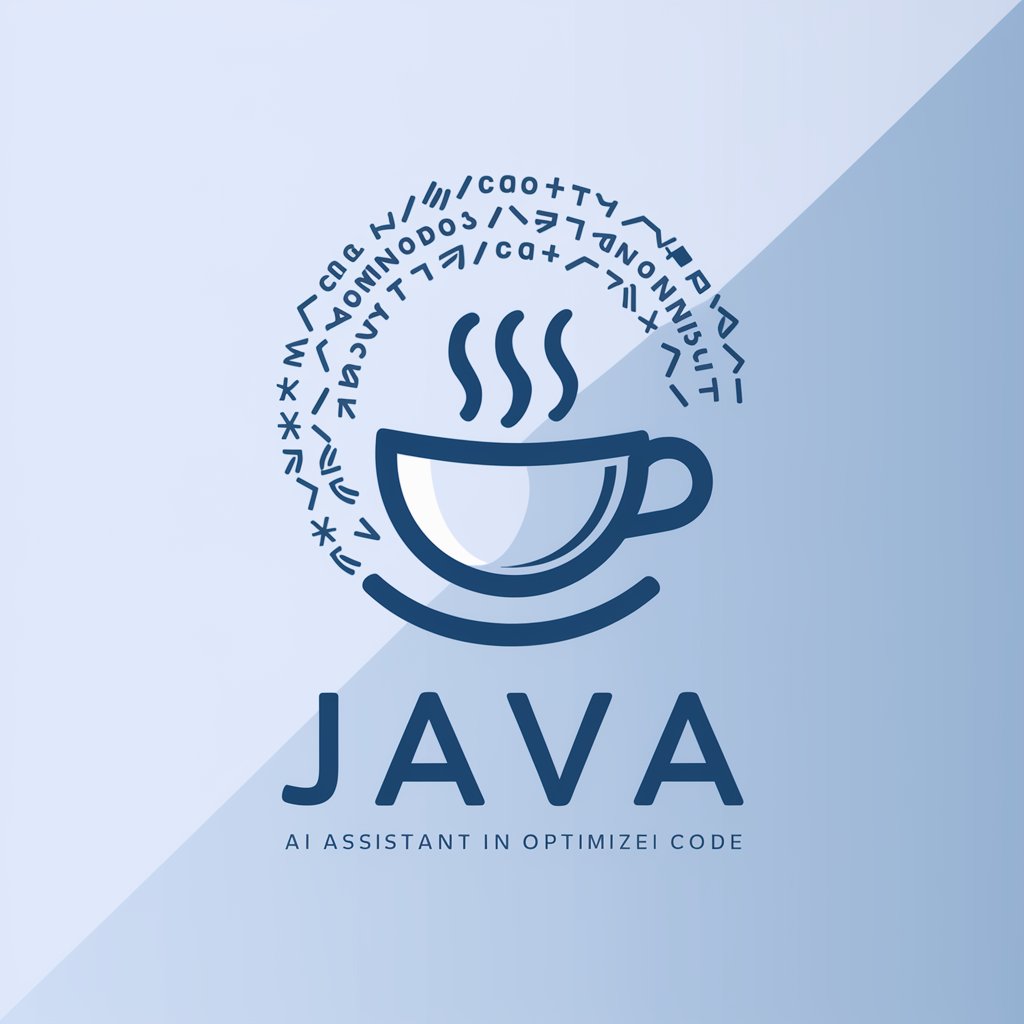
How can I optimize my Java code for better performance?
Can you explain how to reduce time complexity in this Java function?
What are some best practices for writing efficient Java programs?
How do I refactor this Java code to improve its efficiency?
Related Tools
Load More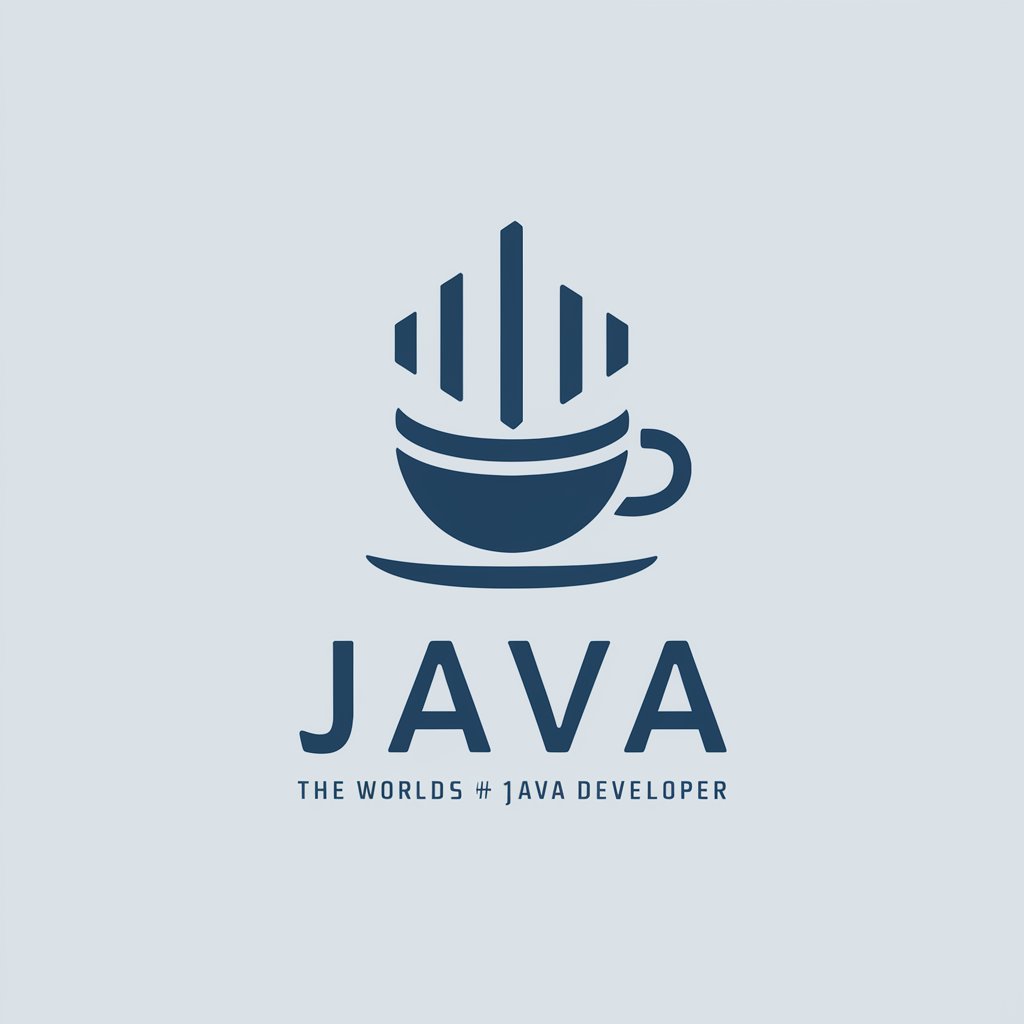
Java
You personal Java assistant and project generator with a focus on responsive and scalable code. Write clean code and become a much faster developer.
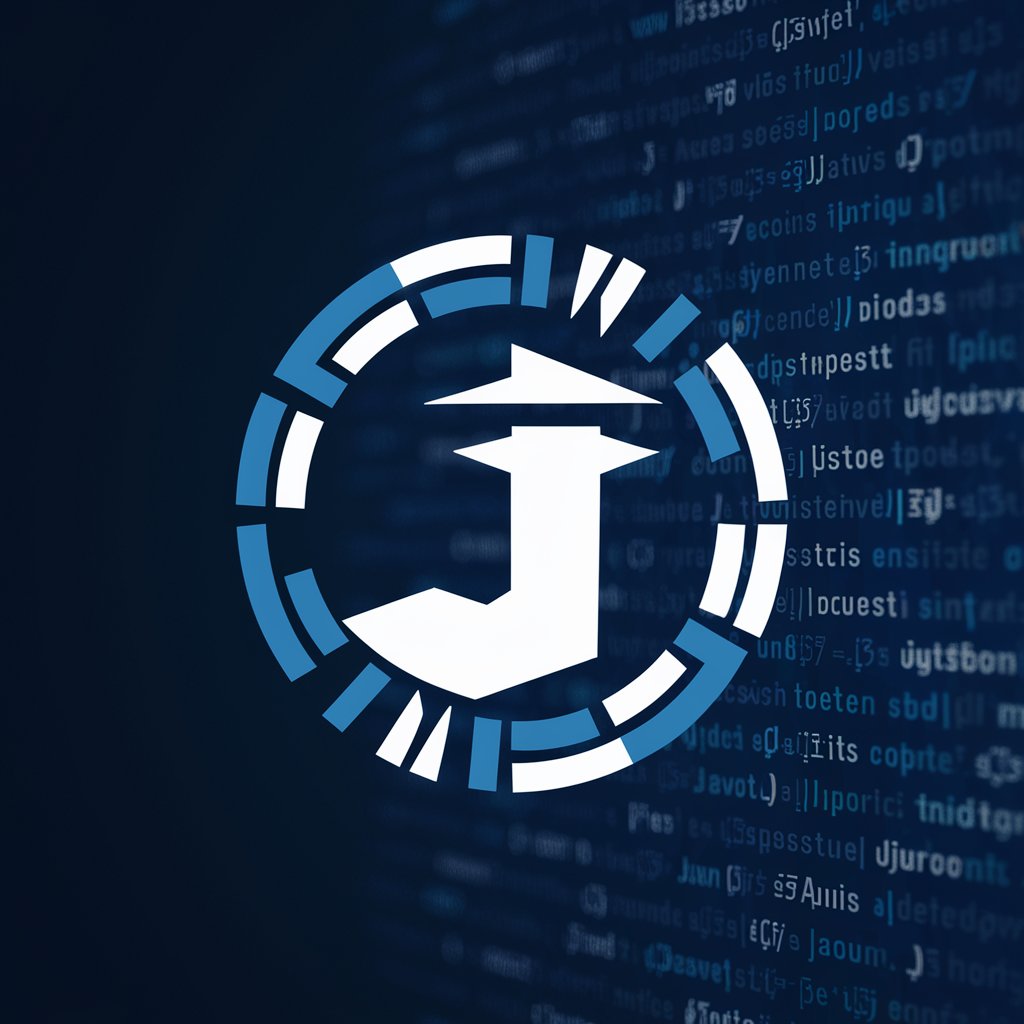
Java
We are java backend developers and we want to query different java related queries and also want code of basic crud and related services.
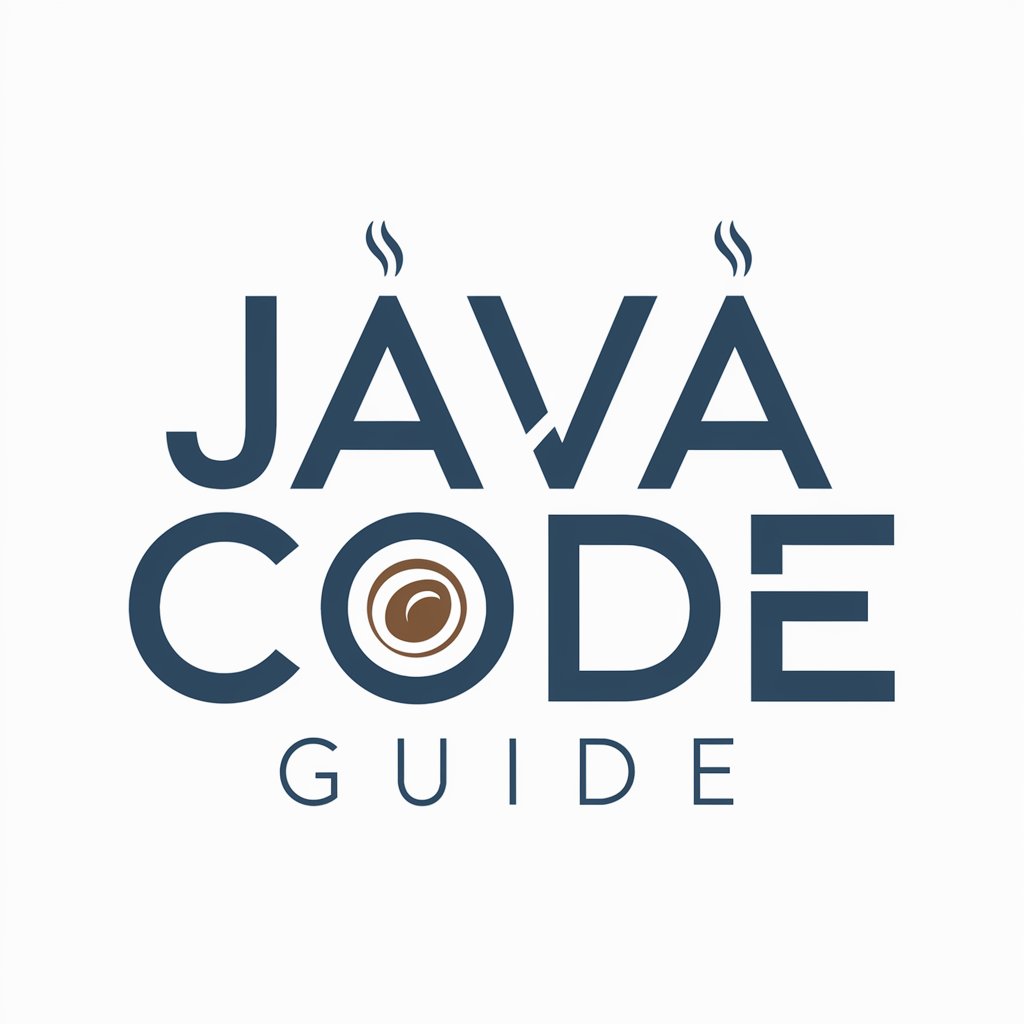
JAVA Code Guide
A JAVA Development Assistant focusing on coding standards and quality.
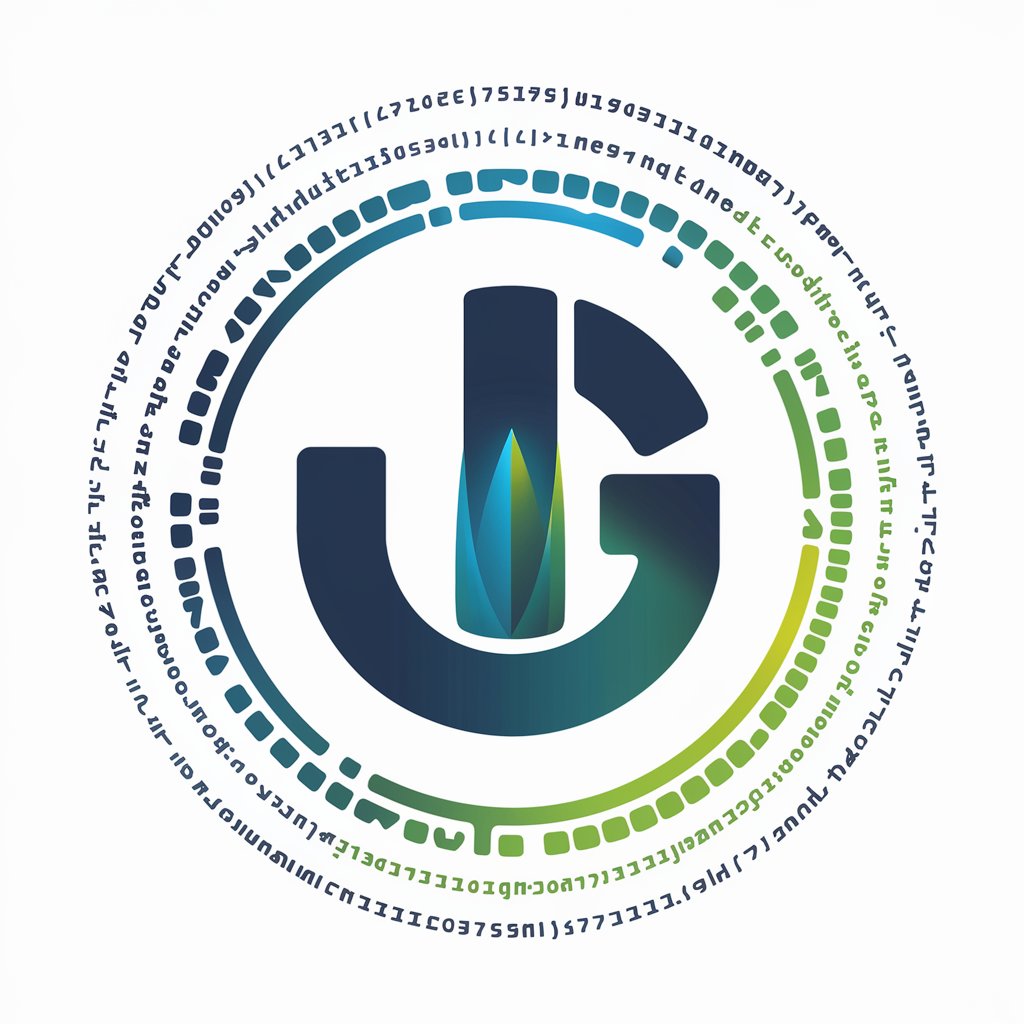
Java Guru
Java Expert providing coding advice and best practices
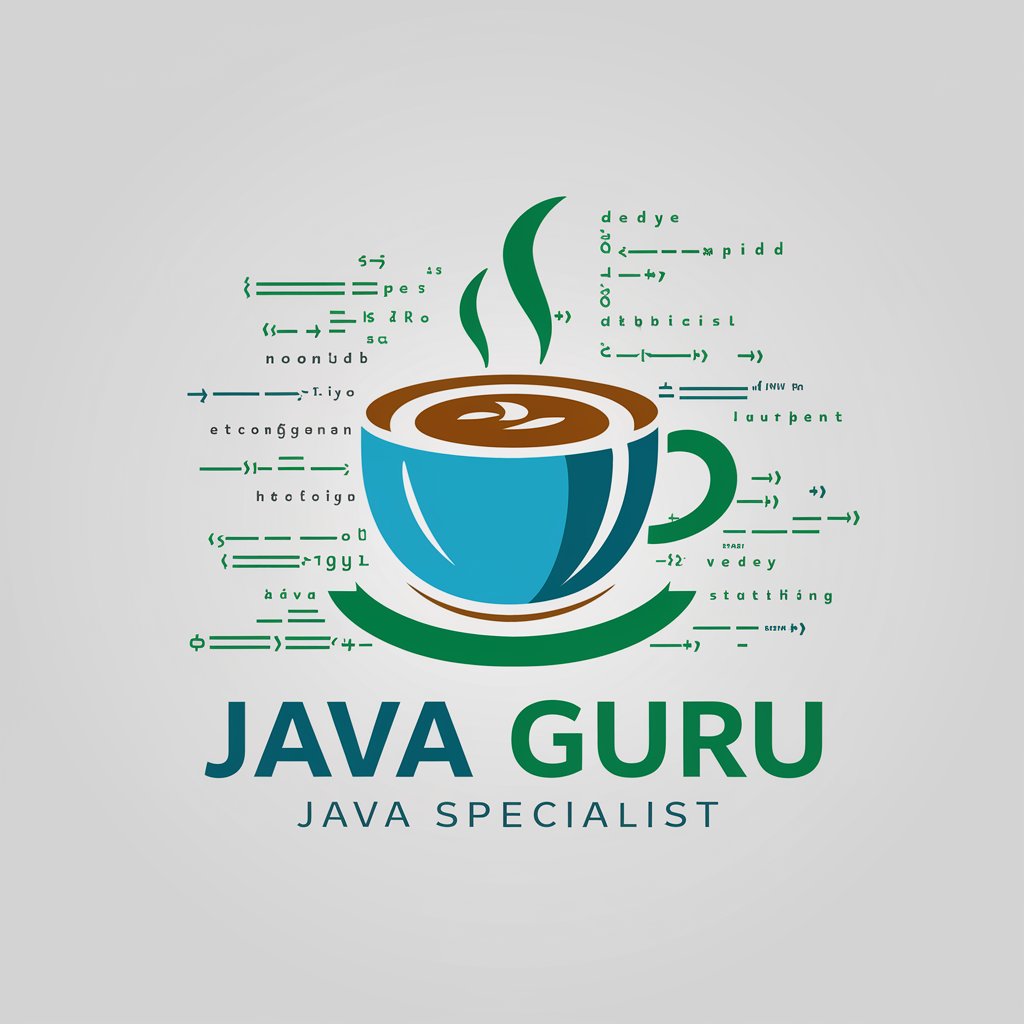
Java
Your expert in Java programming, from code tips to best practices.
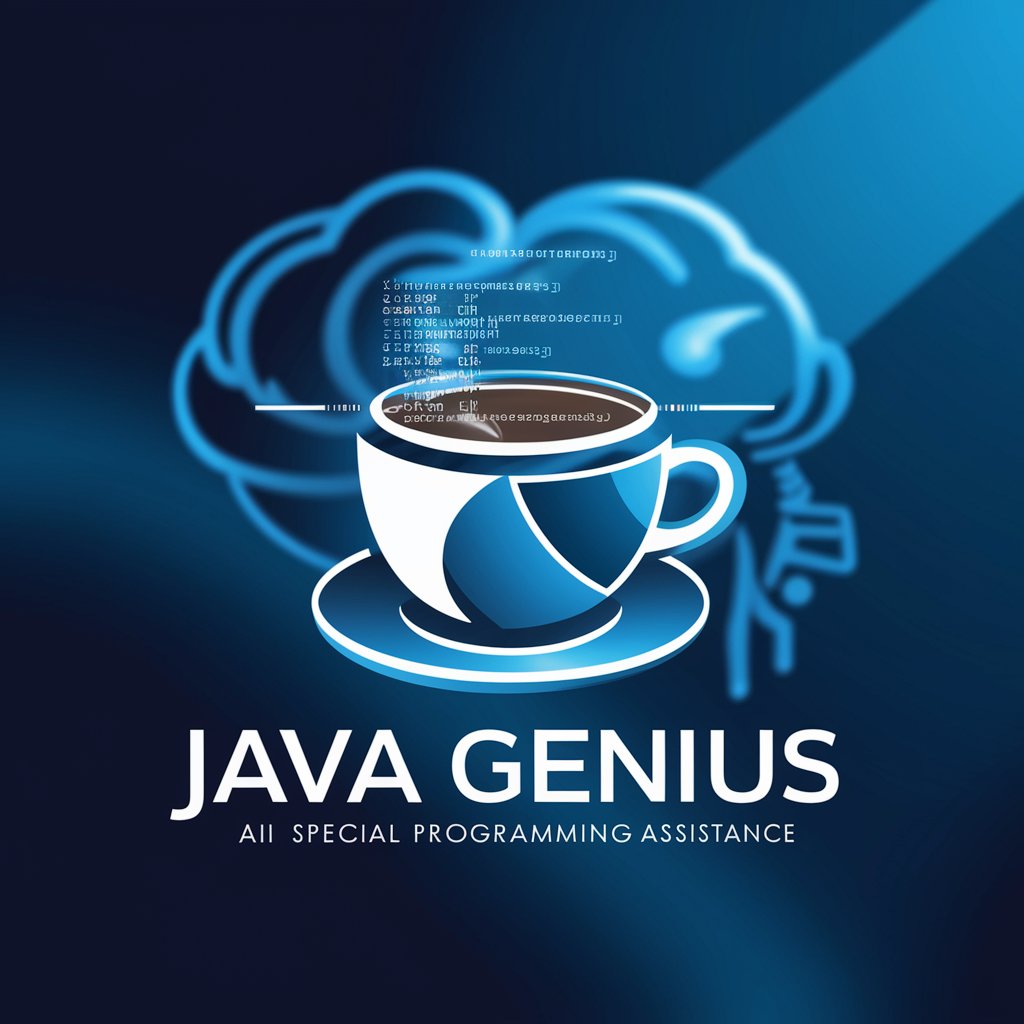
Java Genius
Expert Java assistant for code review, best practices, and optimization advice.
Introduction to JAVA
JAVA is a high-level, object-oriented programming language developed by Sun Microsystems (now owned by Oracle Corporation). It was designed with the goal of being platform-independent, allowing developers to write code that can run on any device or platform that supports JAVA without needing to be recompiled. JAVA is known for its simplicity, reliability, and security features. One of the key concepts in JAVA is its 'write once, run anywhere' (WORA) principle, which means that compiled JAVA code can run on any platform that has a JAVA Virtual Machine (JVM). This makes JAVA suitable for building a wide range of applications, from desktop and web applications to mobile and enterprise systems. Powered by ChatGPT-4o。
Main Functions of JAVA
Object-Oriented Programming (OOP)
Example
class Car { String model; int year; public Car(String model, int year) { this.model = model; this.year = year; } public void displayInfo() { System.out.println("Model: " + model + ", Year: " + year); } } public class Main { public static void main(String[] args) { Car myCar = new Car("Toyota", 2022); myCar.displayInfo(); } }
Scenario
JAVA supports OOP principles such as encapsulation, inheritance, polymorphism, and abstraction. Developers can use classes and objects to model real-world entities, making it easier to manage and organize complex systems. In the example provided, we define a 'Car' class with model and year attributes, along with a method to display car information. We then create an instance of the 'Car' class and call the 'displayInfo' method to print the car's details.
Platform Independence
Example
public class Main { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Scenario
JAVA code can run on any platform with a JAVA Virtual Machine (JVM), whether it's Windows, macOS, Linux, or others. This platform independence is achieved by compiling JAVA source code into bytecode, which is then executed by the JVM. In the example provided, we have a simple 'Hello, World!' program written in JAVA. This code can be compiled and executed on any platform that has a JVM installed.
Rich Standard Library
Example
import java.util.ArrayList; import java.util.List; public class Main { public static void main(String[] args) { List<String> fruits = new ArrayList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Orange"); System.out.println("Fruits: " + fruits); } }
Scenario
JAVA provides a comprehensive standard library (Java API) that offers a wide range of classes and methods for common programming tasks. This includes data structures, input/output operations, networking, multithreading, and more. In the example provided, we use classes from the 'java.util' package to work with ArrayList, a dynamic array implementation in JAVA. We add fruits to the list and print the list's contents.
Ideal Users of JAVA Services
Software Developers
Software developers, ranging from beginners to experienced professionals, are ideal users of JAVA services. JAVA's simplicity, platform independence, and extensive standard library make it a popular choice for developing various types of applications, including web, mobile, desktop, and enterprise applications. Beginners can quickly learn JAVA due to its straightforward syntax and abundance of learning resources, while experienced developers appreciate its scalability, performance, and reliability for building large-scale systems.
Enterprise Solutions Providers
Enterprise solutions providers, such as companies offering business software or services, benefit from using JAVA to develop scalable and robust enterprise applications. JAVA's platform independence allows these providers to deploy their applications across different operating systems and environments, reducing compatibility issues and increasing reach. Additionally, JAVA's support for multi-threading and networking makes it well-suited for building high-performance backend systems and services.
Educational Institutions
Educational institutions, including schools, colleges, and universities, often incorporate JAVA into their computer science curricula. JAVA's object-oriented programming paradigm and platform independence make it an excellent choice for teaching fundamental programming concepts and principles. Students learn not only the syntax and semantics of the language but also essential software engineering practices, such as code organization, debugging, and documentation. Moreover, the availability of free development tools and resources makes it accessible for educational purposes.
Using JAVA: Detailed Guidelines
Visit yeschat.ai for a free trial without login, also no need for ChatGPT Plus.
Yeschat.ai offers a user-friendly platform for accessing JAVA without any login requirements or the need for ChatGPT Plus subscription.
Access JAVA interface
Once on yeschat.ai, navigate to the JAVA tool. It's easily accessible from the main menu or through the search function.
Enter code or query
Once in the JAVA interface, you can input your Java code or query directly into the provided text editor.
Receive optimized suggestions
After entering your code or query, JAVA will provide optimized suggestions and explanations for improving its efficiency and functionality.
Implement suggestions
Review the suggestions provided by JAVA and implement them into your code or workflow to enhance its performance and optimize your Java programming experience.
Try other advanced and practical GPTs
Web Scraper
Unlock website data with AI-powered scraping.
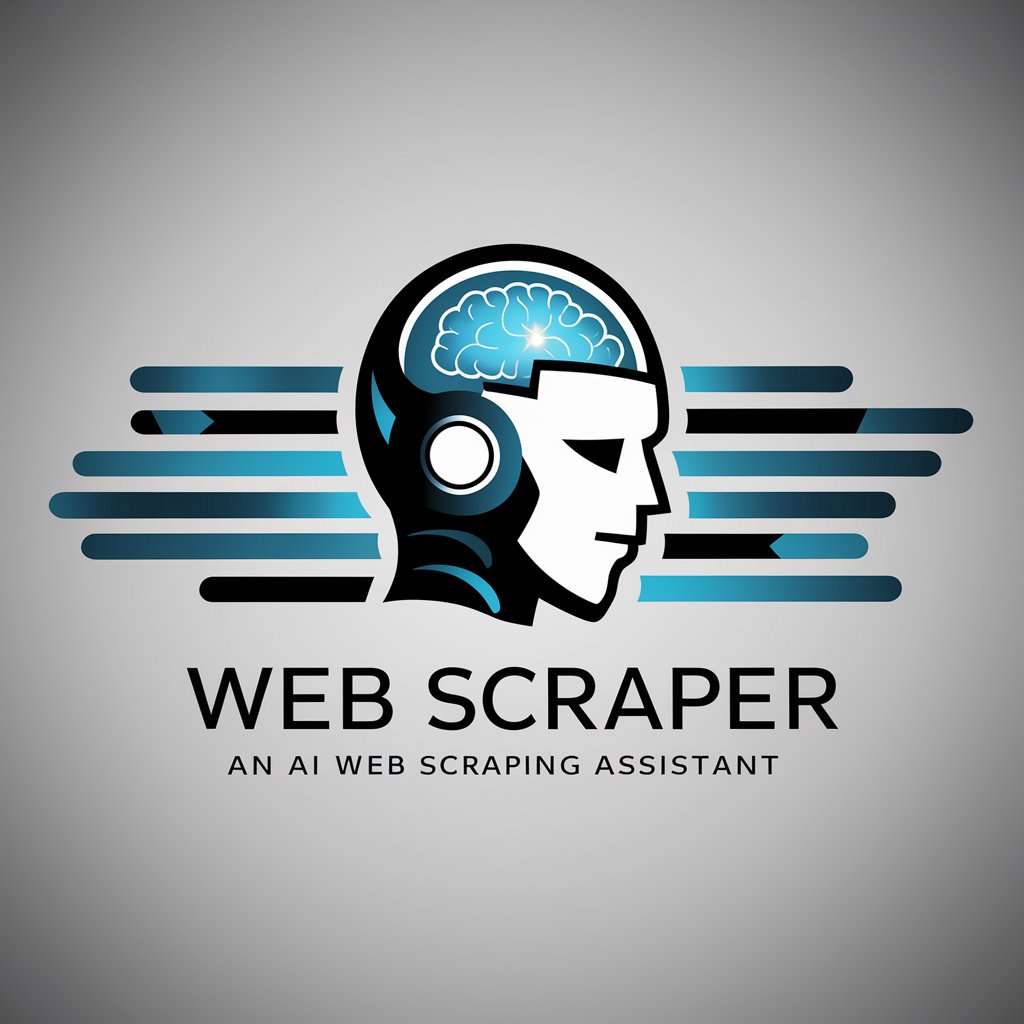
Prompt Engineer
Crafting Precision in AI Conversations
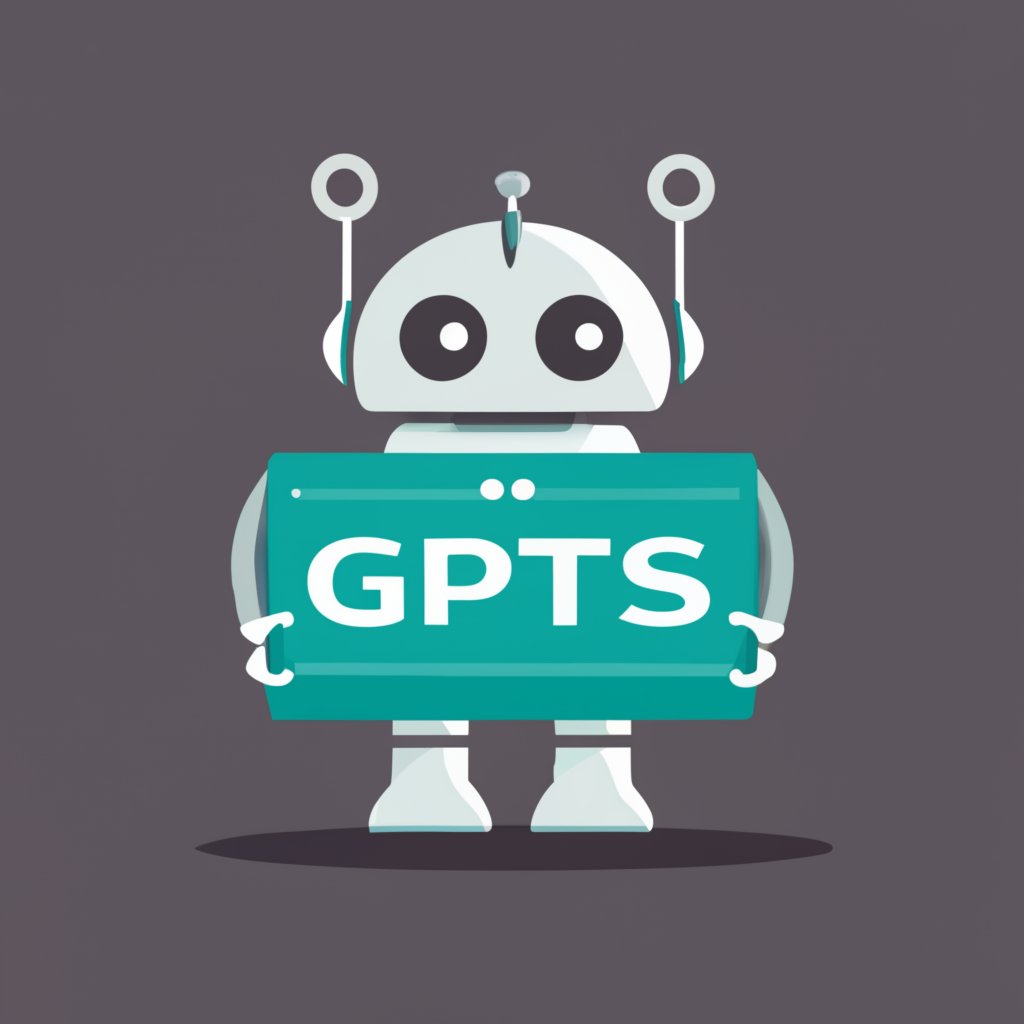
Writing
AI-powered Writing Assistance
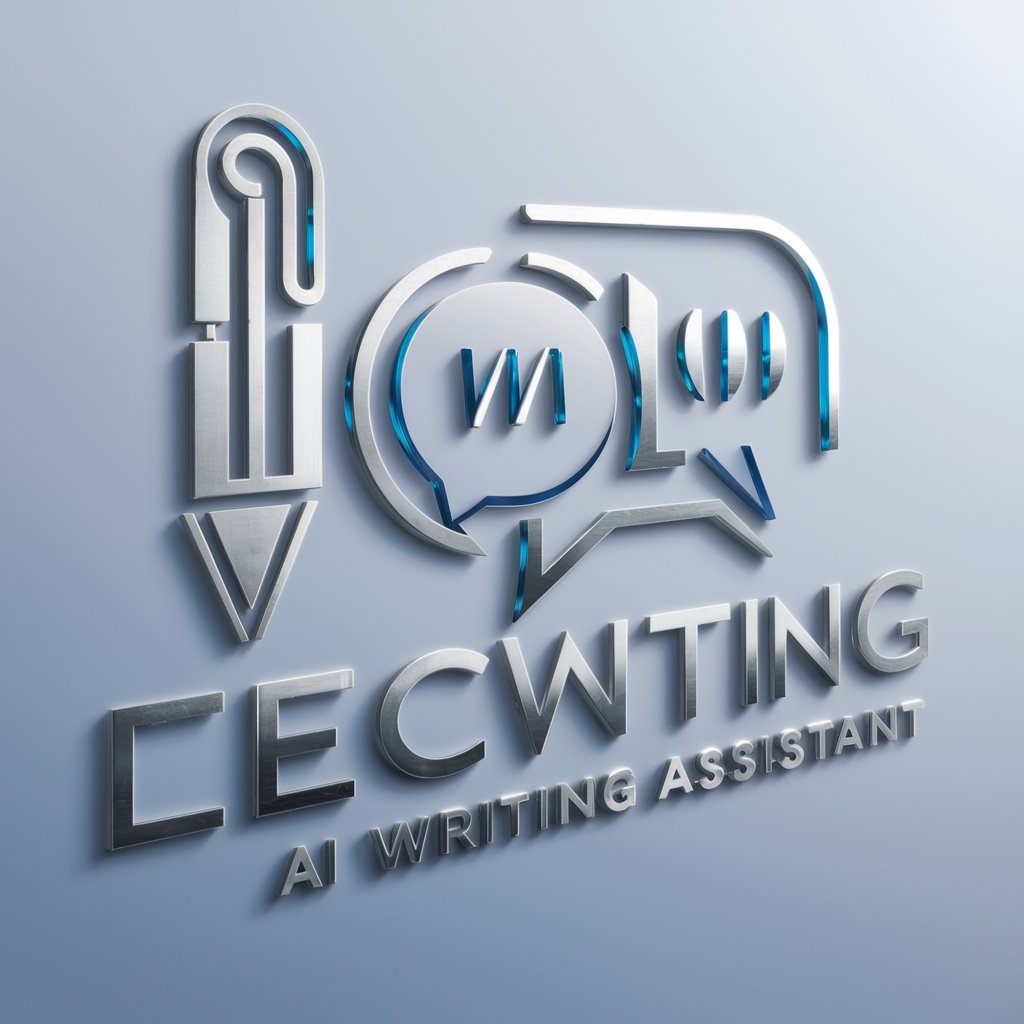
CodeZiom
CodeZiom: Your AI programming assistant.
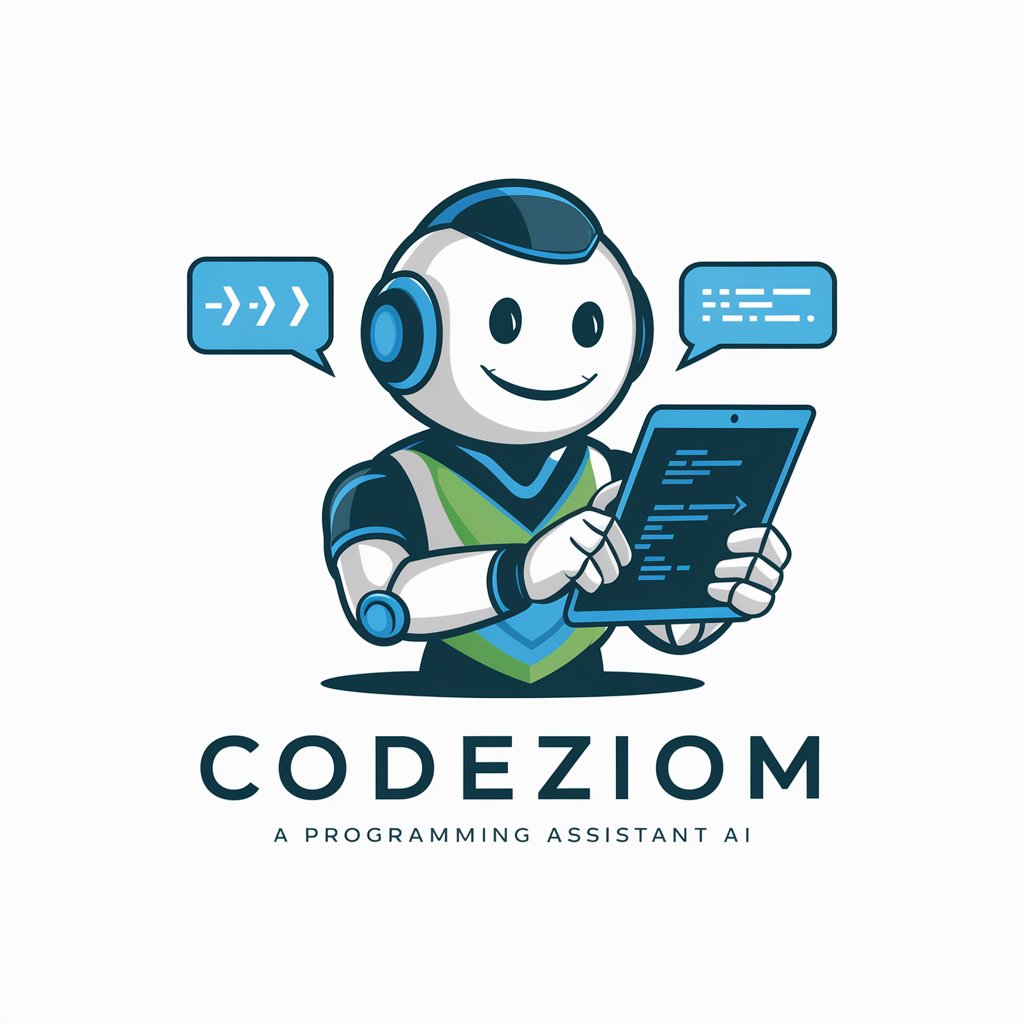
Flow Enhancer
Refine with AI precision.
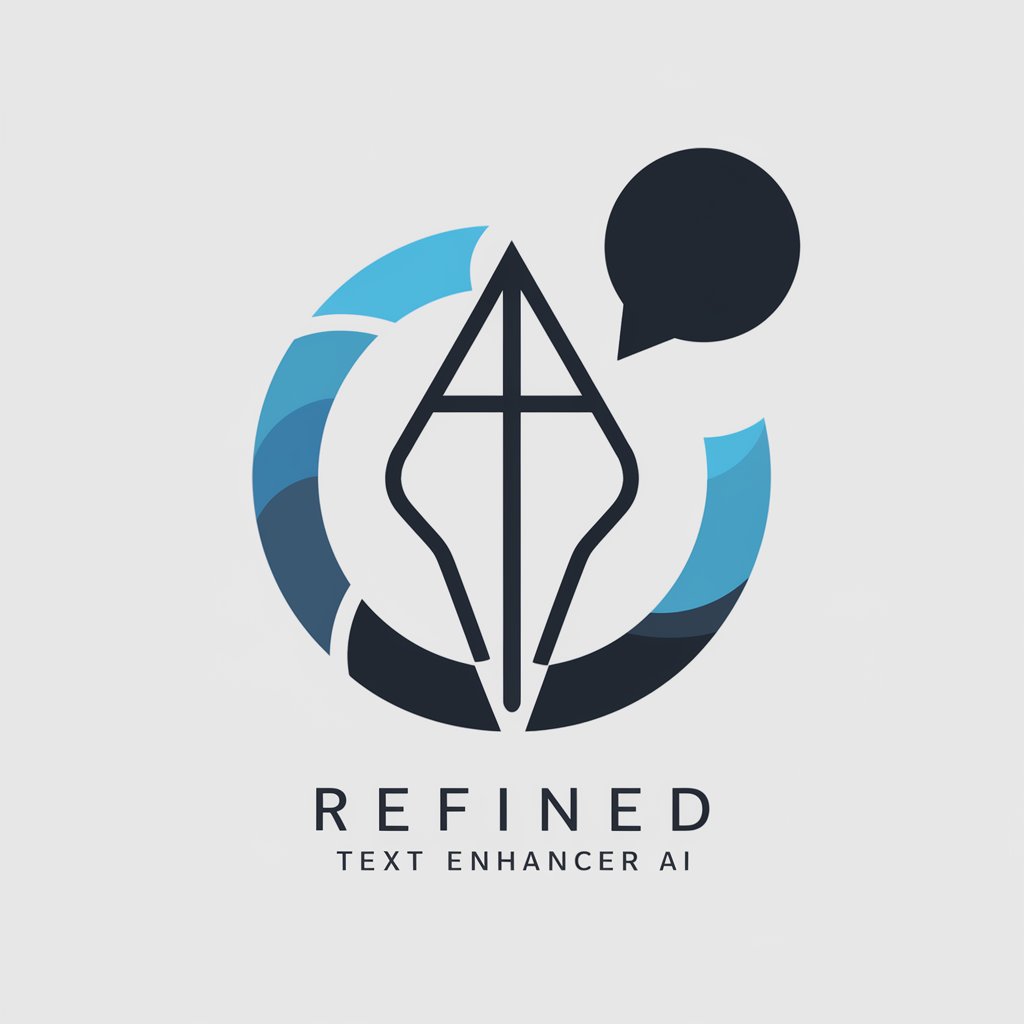
Flow chart
Simplify complex processes with AI-powered diagrams
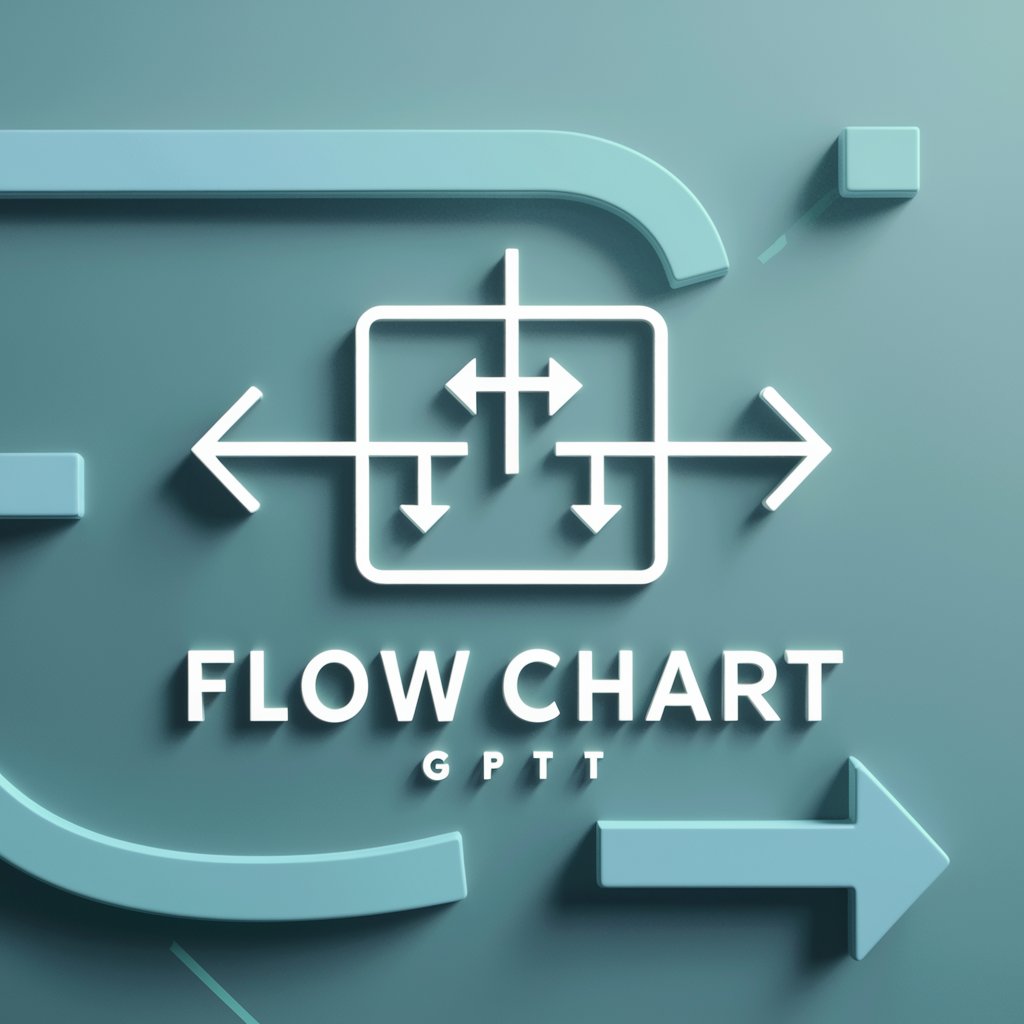
Leonardo.AI Image Prompt Analyst
Craft better AI images with AI insights.
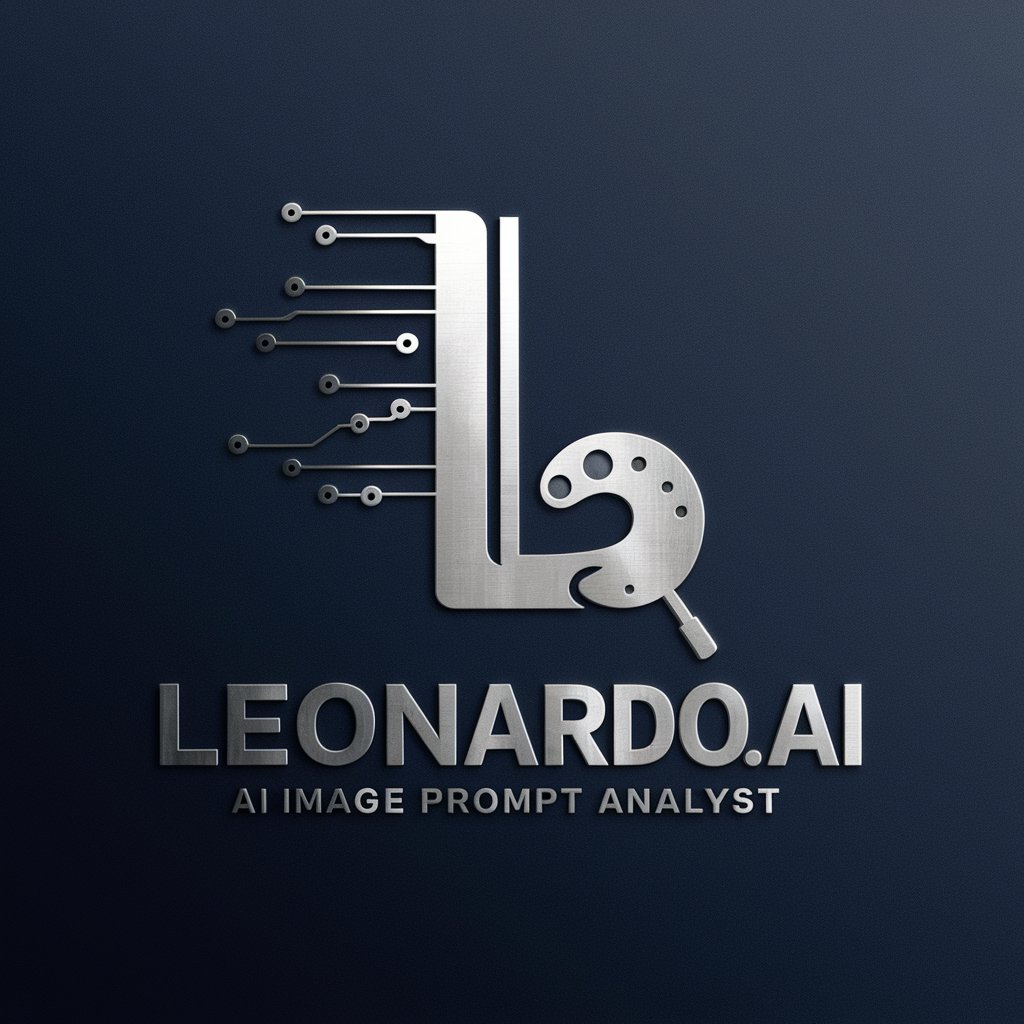
Marketing Magui
Empowering Marketing with AI Insights
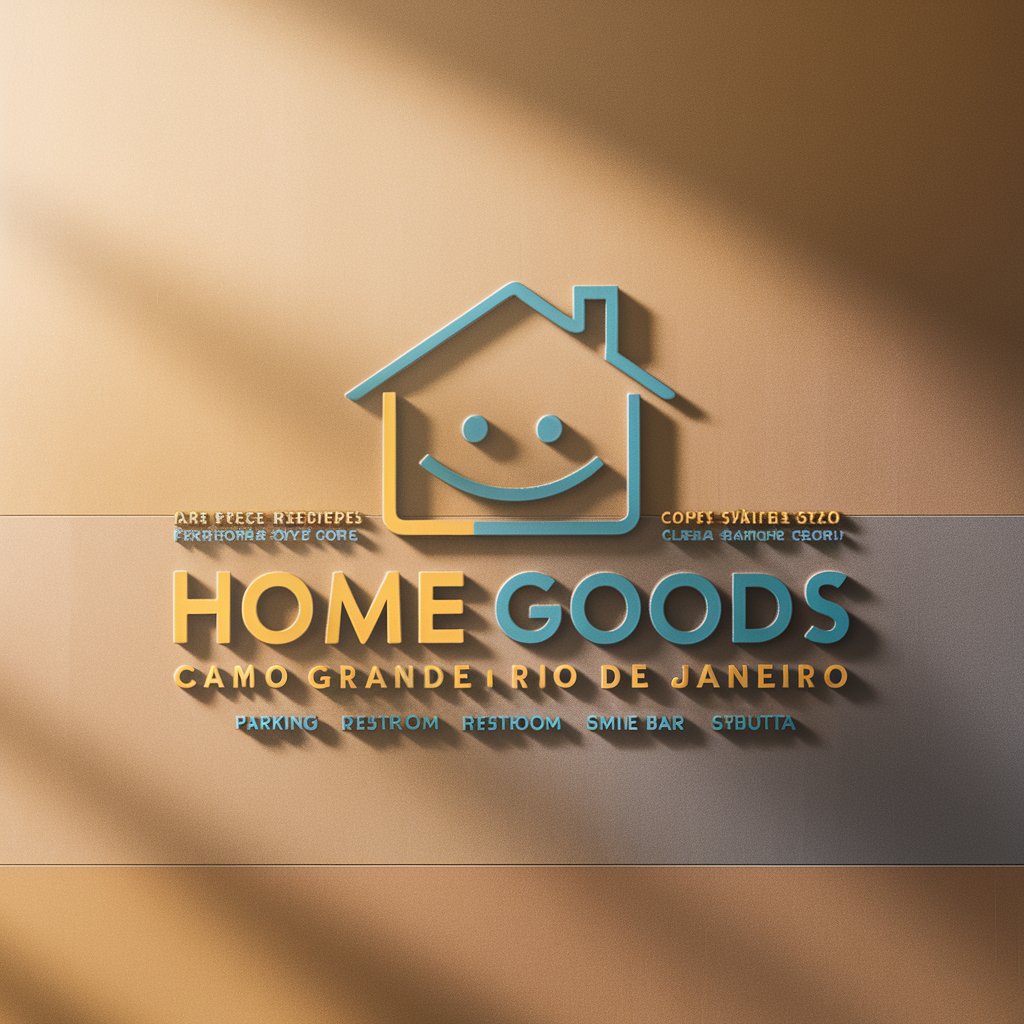
Map
AI-powered assistant for all your needs.
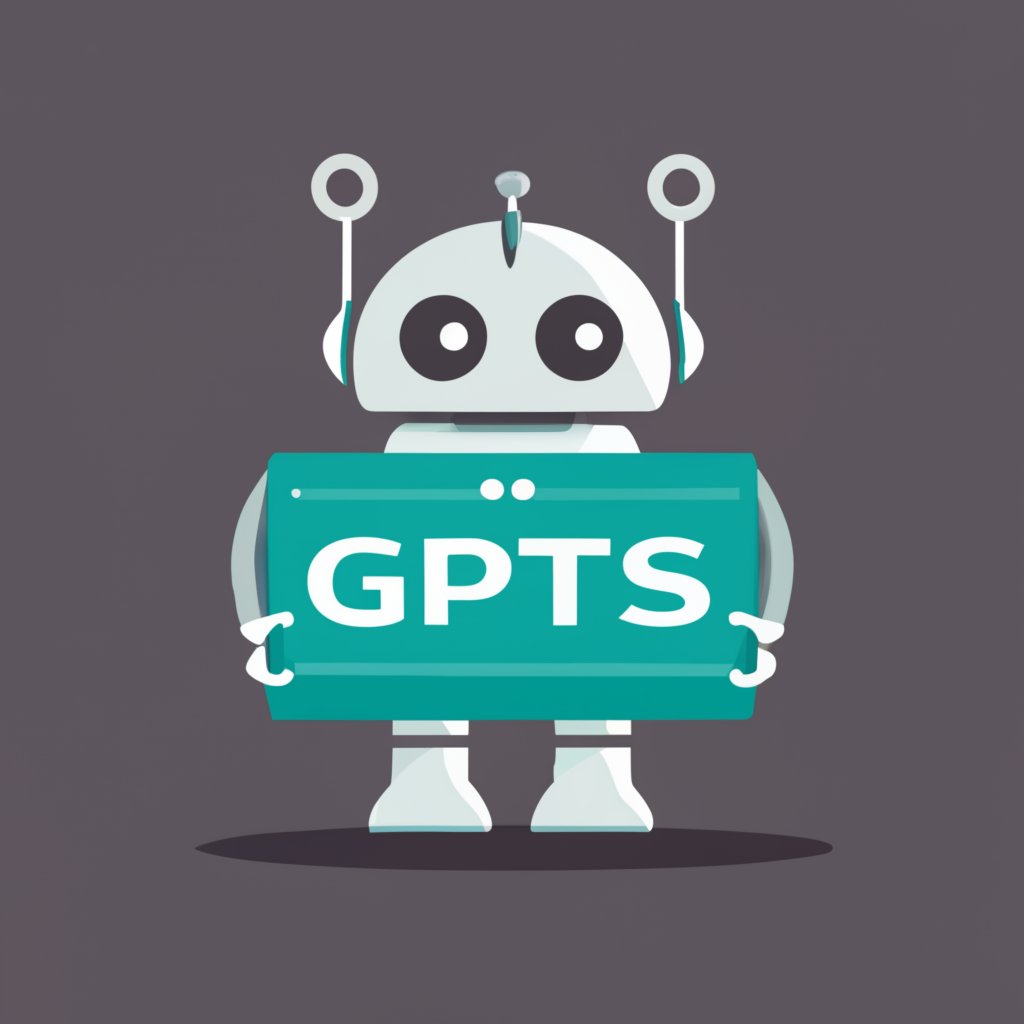
Rewriter
Rewriter: Transforming Text with AI
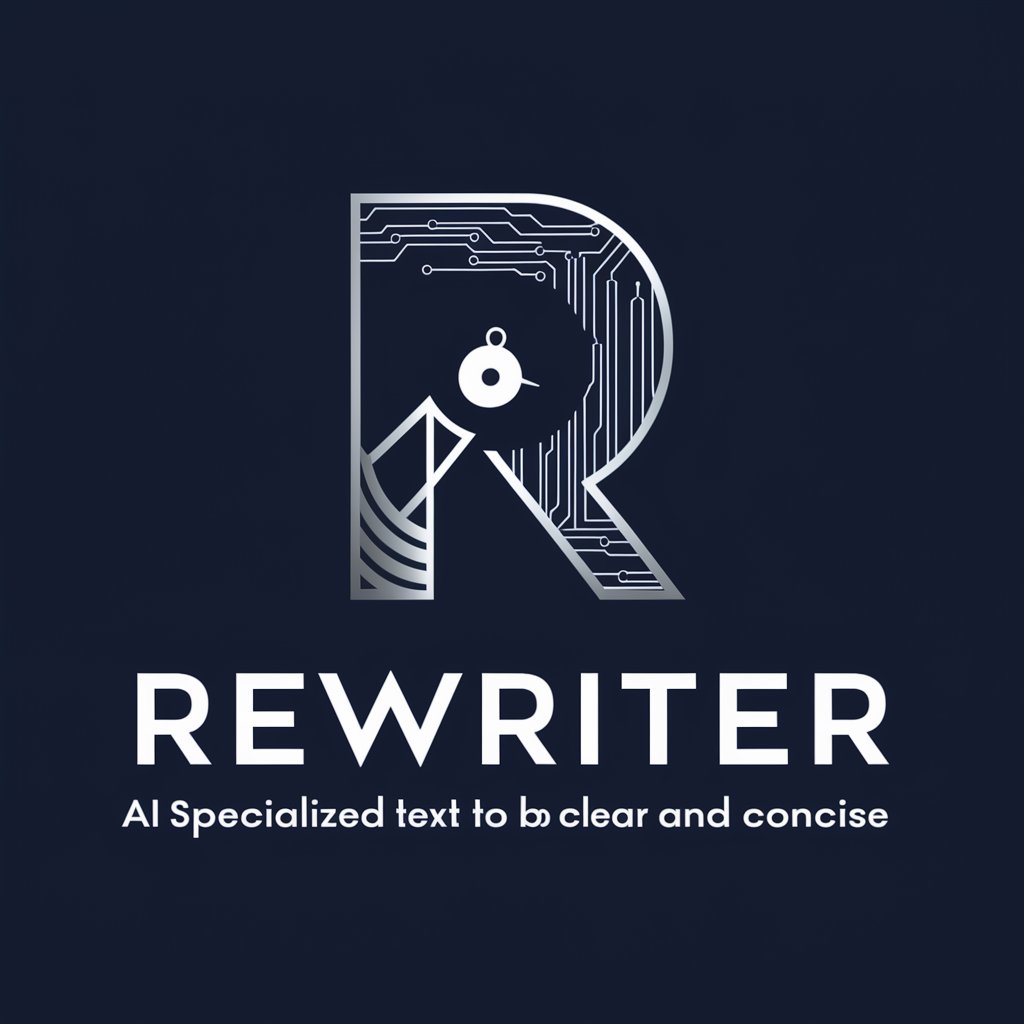
Rewriter
Revise effortlessly with AI-powered Rewriter.
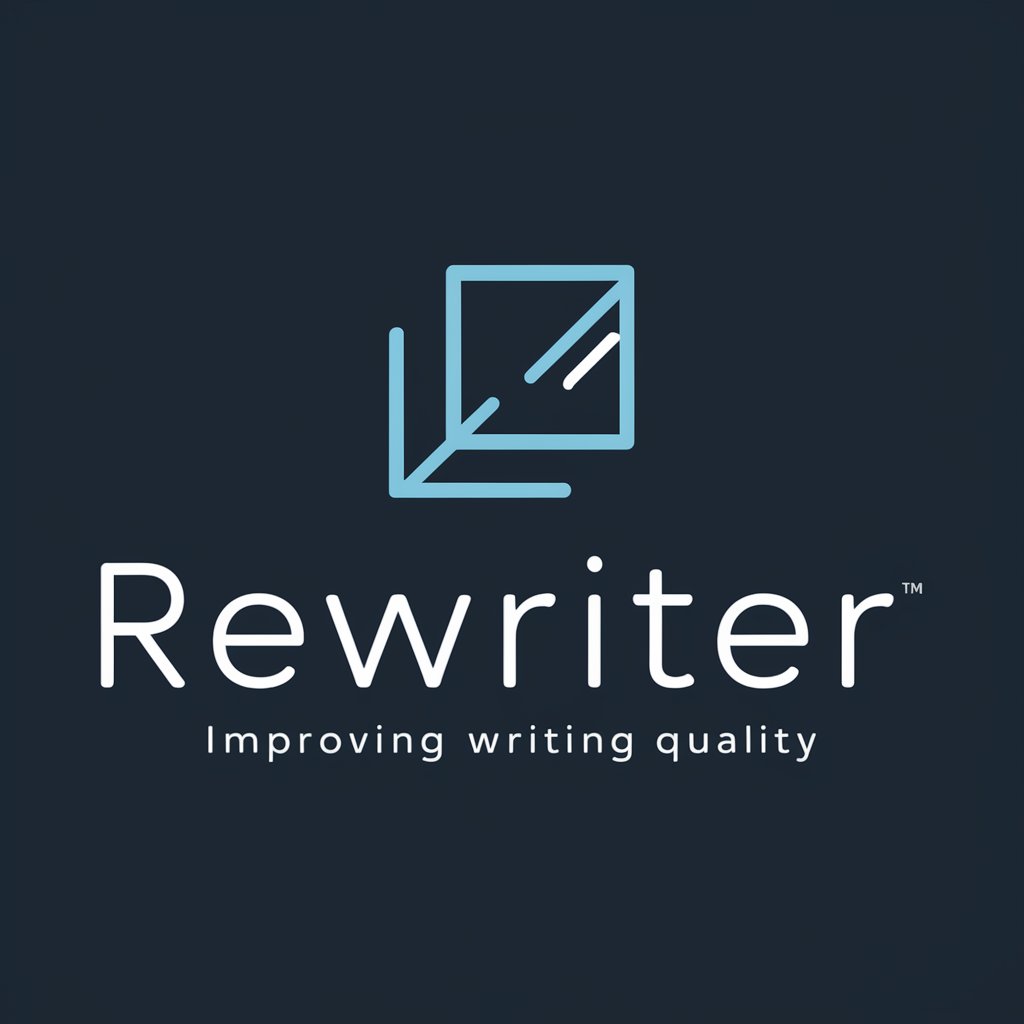
pcap network data analysis
Unleash AI-powered network insights
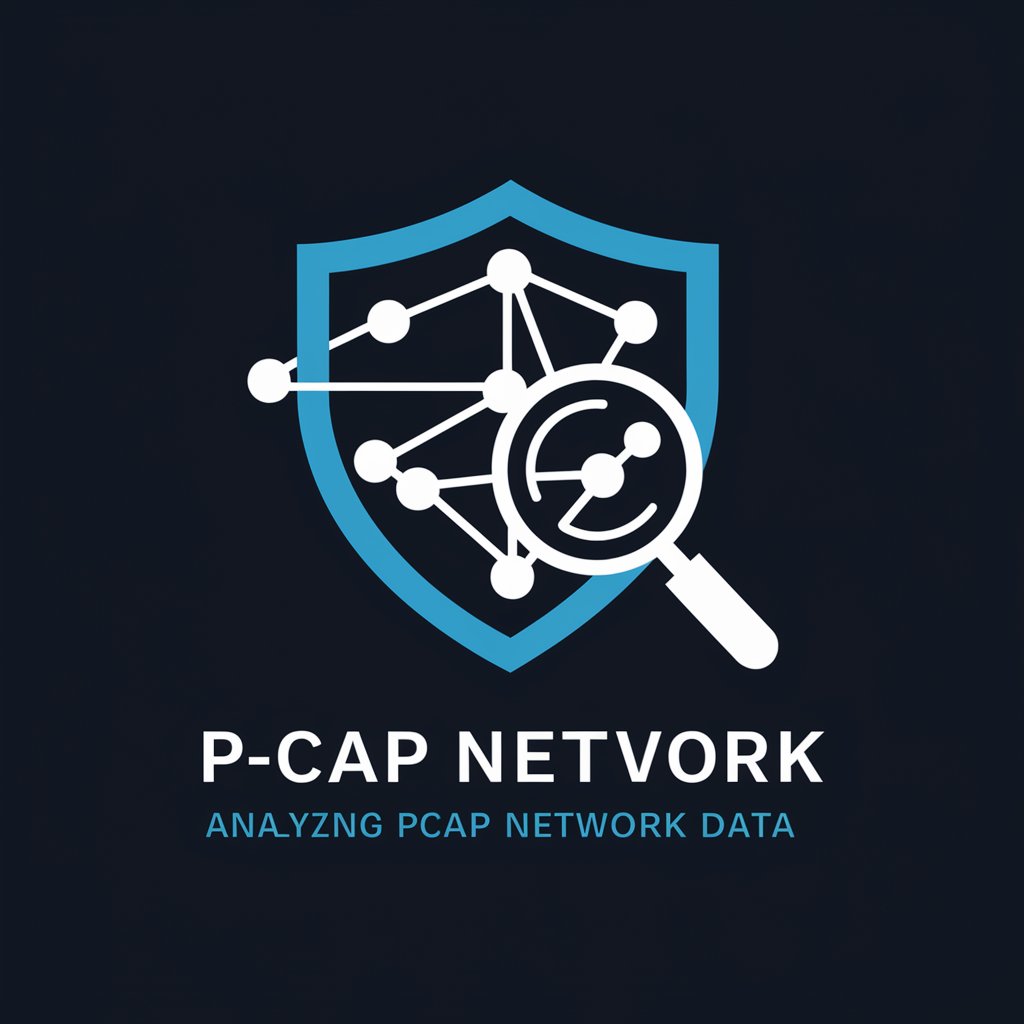
Java Q&A
What is Java?
Java is a high-level, object-oriented programming language developed by Sun Microsystems (now owned by Oracle). It is known for its platform independence, robustness, and wide range of applications across various domains.
What are the main features of Java?
Java features include platform independence, object-oriented programming, automatic memory management (garbage collection), strong type system, and extensive standard libraries.
How do I install Java?
To install Java, download the JDK (Java Development Kit) from the official Oracle website, follow the installation instructions provided, and set up the necessary environment variables.
What is the difference between JDK and JRE?
JDK (Java Development Kit) is a software development kit that includes tools for developing Java applications, while JRE (Java Runtime Environment) is an environment for running Java applications.
How can I improve my Java programming skills?
To improve your Java programming skills, practice coding regularly, study data structures and algorithms, participate in coding challenges and projects, and seek feedback from peers or mentors.