lit-HTML - Efficient Web Component Tool
Expert in TypeScript & Lit 3 web development
Craft dynamic web interfaces with AI efficiency.
How do I create a custom element in Lit?
Show me an example of a TypeScript interface.
Best practices for Lit 3 components?
How to manage state in a Lit project?
Get Embed Code
Introduction to lit-HTML
lit-HTML is a lightweight JavaScript library designed to efficiently render HTML templates. It is a core part of the Lit framework and serves as a core mechanism behind LitElement, providing a fast, declarative way to work with DOM elements. The primary goal of lit-HTML is to enable developers to create Web Components and UI elements with ease, while ensuring maximum performance by employing efficient DOM updates through the use of tagged template literals. Instead of updating the entire DOM subtree, lit-HTML intelligently updates only the parts that have changed, making it highly performant for dynamic UIs. Example usage: ```javascript import { html, render } from 'lit-html'; const myTemplate = (name) => html`
Main Functions of lit-HTML
html
Example
```javascript import { html, render } from 'lit-html'; const template = html`<div>Sample Template</div>`; render(template, document.body); ```
Scenario
Creates a lit-HTML template. Used in almost all scenarios involving the library. Enables developers to write HTML templates using JavaScript and tagged template literals.
render
Example
```javascript import { html, render } from 'lit-html'; const myTemplate = html`<p>Hello, lit-HTML!</p>`; render(myTemplate, document.getElementById('app-root')); ```
Scenario
Renders a lit-HTML template to a given container. The `render` function is ideal for updating the DOM quickly, ensuring only the modified parts are updated.
repeat
Example
```javascript import { html, render, repeat } from 'lit-html/directives/repeat.js'; const items = [1, 2, 3, 4, 5]; const myTemplate = html` <ul> ${repeat(items, (item) => item, (item) => html`<li>${item}</li>`)} </ul> `; render(myTemplate, document.body); ```
Scenario
Efficiently renders a list of items with unique identifiers. Ideal for displaying dynamic lists like search results, to-do items, or user-generated content.
ifDefined
Example
```javascript import { html, render } from 'lit-html'; import { ifDefined } from 'lit-html/directives/if-defined.js'; const myTemplate = (optionalClass) => html`<div class=${ifDefined(optionalClass)}></div>`; render(myTemplate('my-class'), document.body); render(myTemplate(undefined), document.body); ```
Scenario
Conditionally sets an attribute or value only if it's defined. Useful when dealing with optional attributes, such as conditional classes, styles, or properties.
unsafeHTML
Example
```javascript import { html, render } from 'lit-html'; import { unsafeHTML } from 'lit-html/directives/unsafe-html.js'; const userContent = '<p>This is user-generated content</p>'; const myTemplate = html` <div>${unsafeHTML(userContent)}</div> `; render(myTemplate, document.body); ```
Scenario
Renders potentially unsafe HTML strings directly into the DOM. Useful for embedding preformatted HTML content, such as data coming from a CMS or a rich text editor.
Ideal Users of lit-HTML
Web Developers Building Web Components
Developers who are focused on creating reusable, modular web components will find lit-HTML highly beneficial. The library's integration with LitElement makes it straightforward to develop performant, custom components.
Developers Focused on Performance Optimization
Performance-oriented developers who need to render dynamic, data-driven UIs will appreciate lit-HTML's efficient DOM updating mechanism, which minimizes unnecessary re-renders.
Front-End Developers Migrating from Frameworks like React or Angular
Developers familiar with JSX or Angular templates can easily transition to lit-HTML due to its similar declarative syntax. The lightweight nature of lit-HTML also helps simplify development compared to larger frameworks.
UI/UX Designers Prototyping Interactive UIs
UI/UX designers or developers working on rapid prototyping will benefit from the concise syntax and fast rendering capabilities of lit-HTML for creating interactive, high-fidelity prototypes.
How to Use Lit-HTML
Initiate trial
Start by visiting yeschat.ai to access a free trial instantly, with no need to log in or subscribe to ChatGPT Plus.
Install dependencies
Ensure you have Node.js installed on your system. Use npm or yarn to install Lit by running `npm install lit` or `yarn add lit` in your project directory.
Create a component
Use Lit to create a new web component. Define a class that extends `LitElement` and use the `@customElement` decorator to register it as a custom element.
Define templates
Leverage lit-HTML within your component by defining a `render` method that returns a template literal tagged with the `html` function for dynamic HTML rendering.
Integrate and test
Integrate the new component into your web application. Test it in various browsers to ensure compatibility and performance. Use Lit's reactive update cycle for efficient updates.
Try other advanced and practical GPTs
Специалист по неймингу
Crafting Names with AI Precision
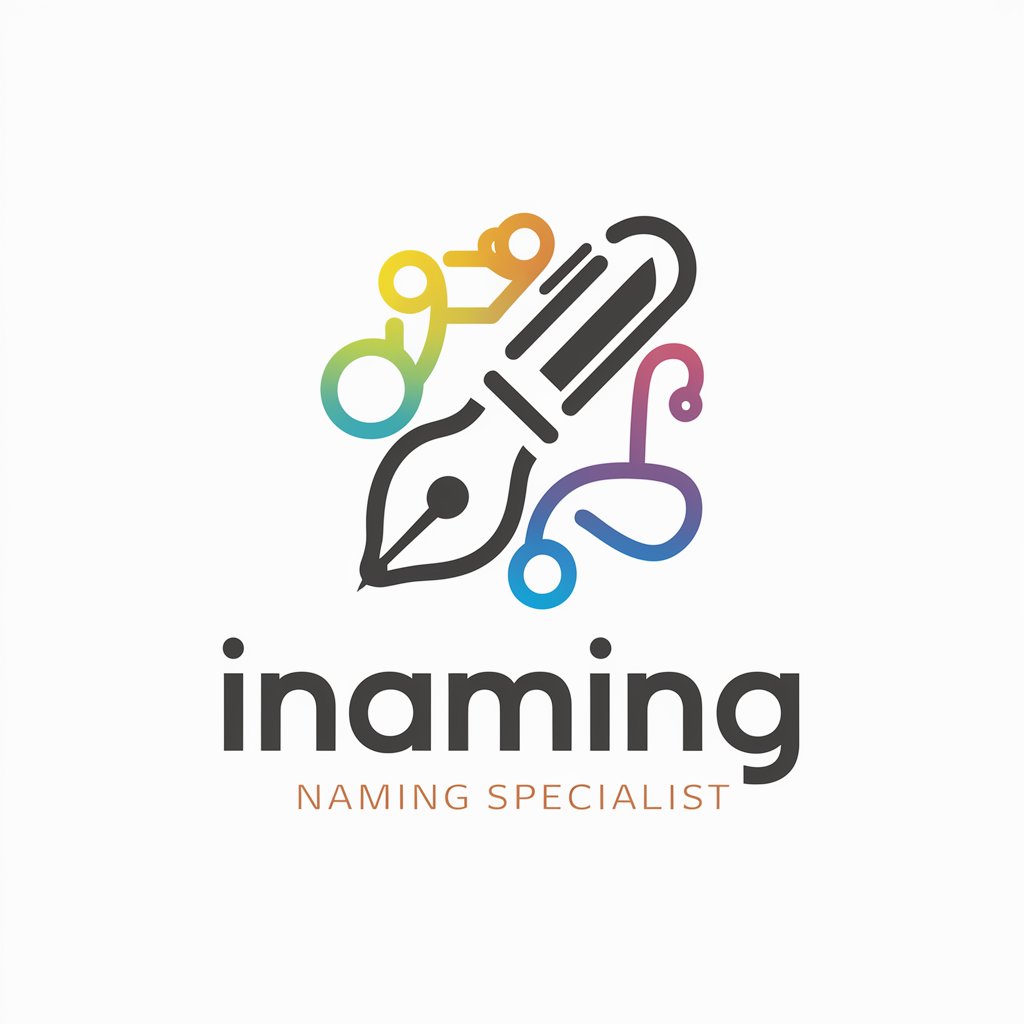
Correción de ortografía
Perfect Your Spanish with AI
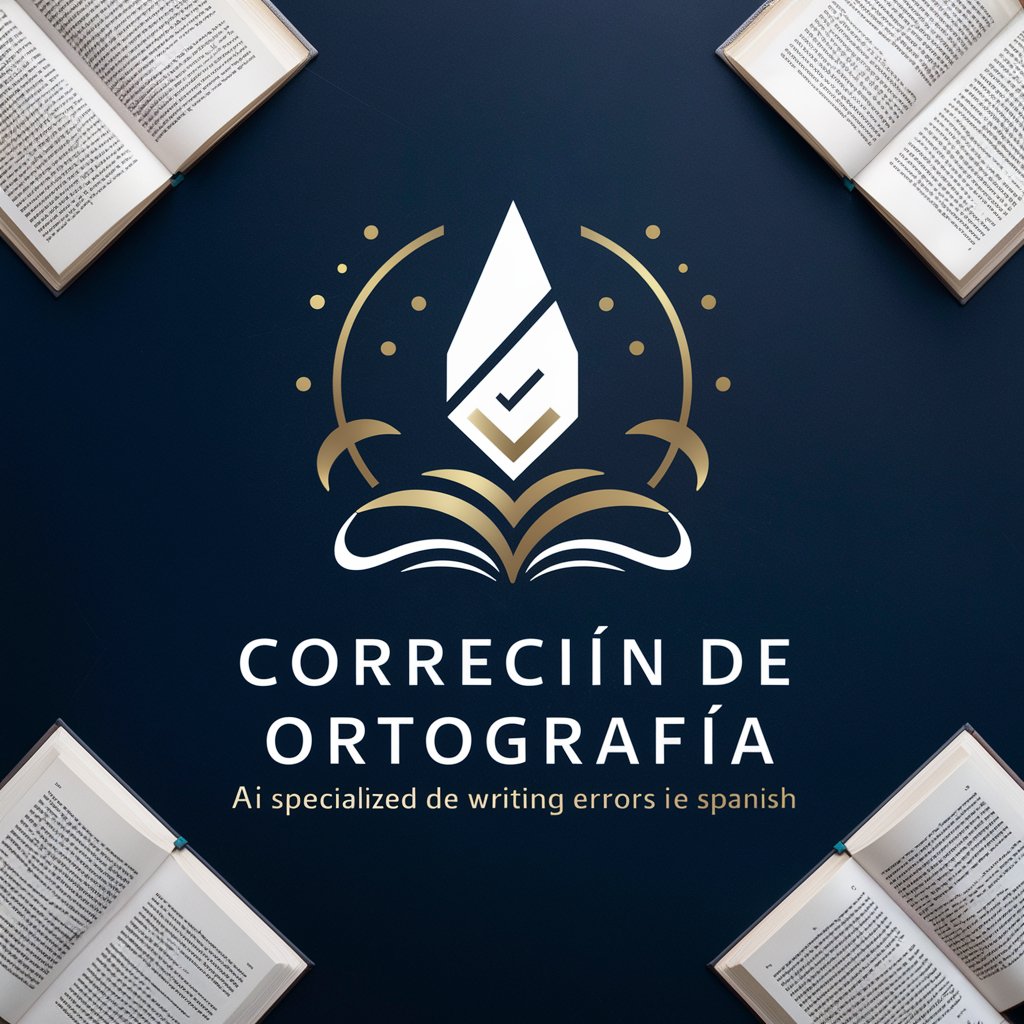
Mitochondria Extractor
Decoding Mitochondrial Science with AI
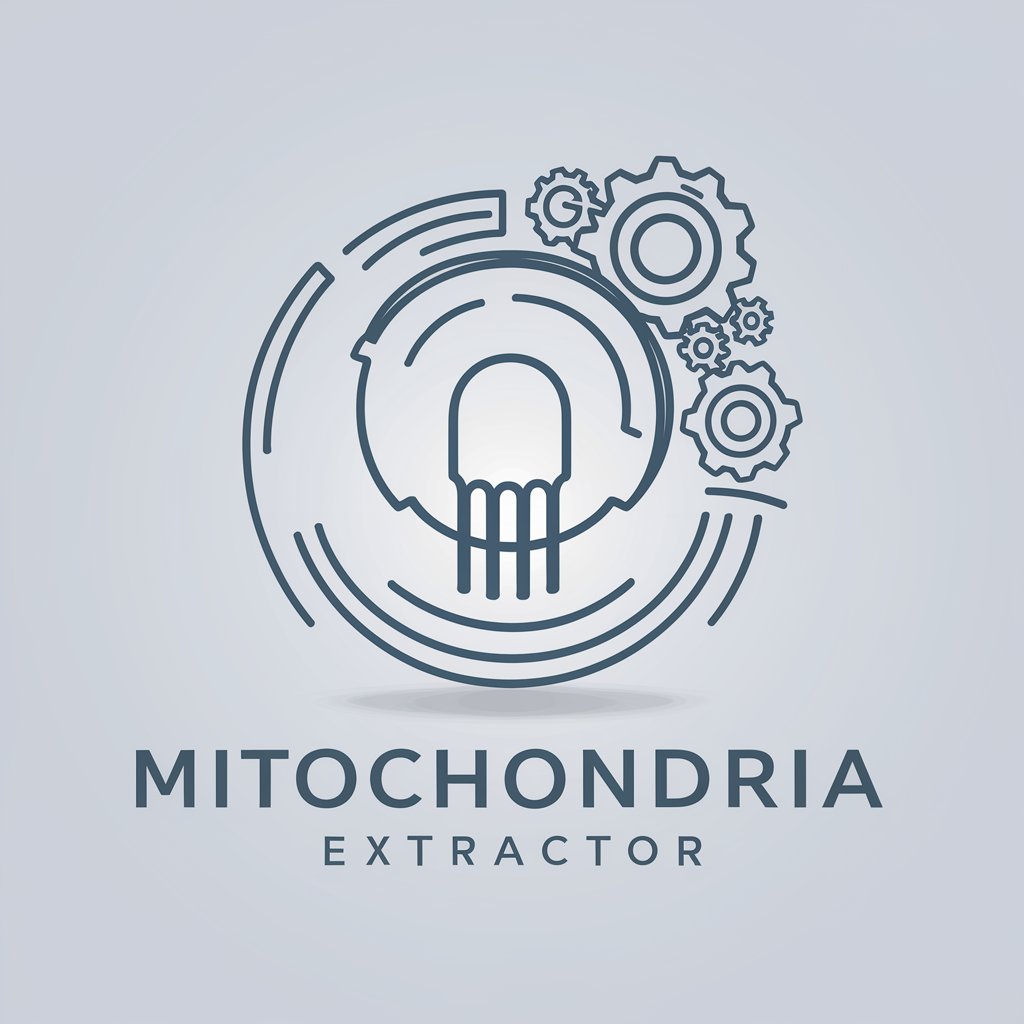
雑学動画台本 ver3
Empower Your Creativity with AI-Driven Scripts
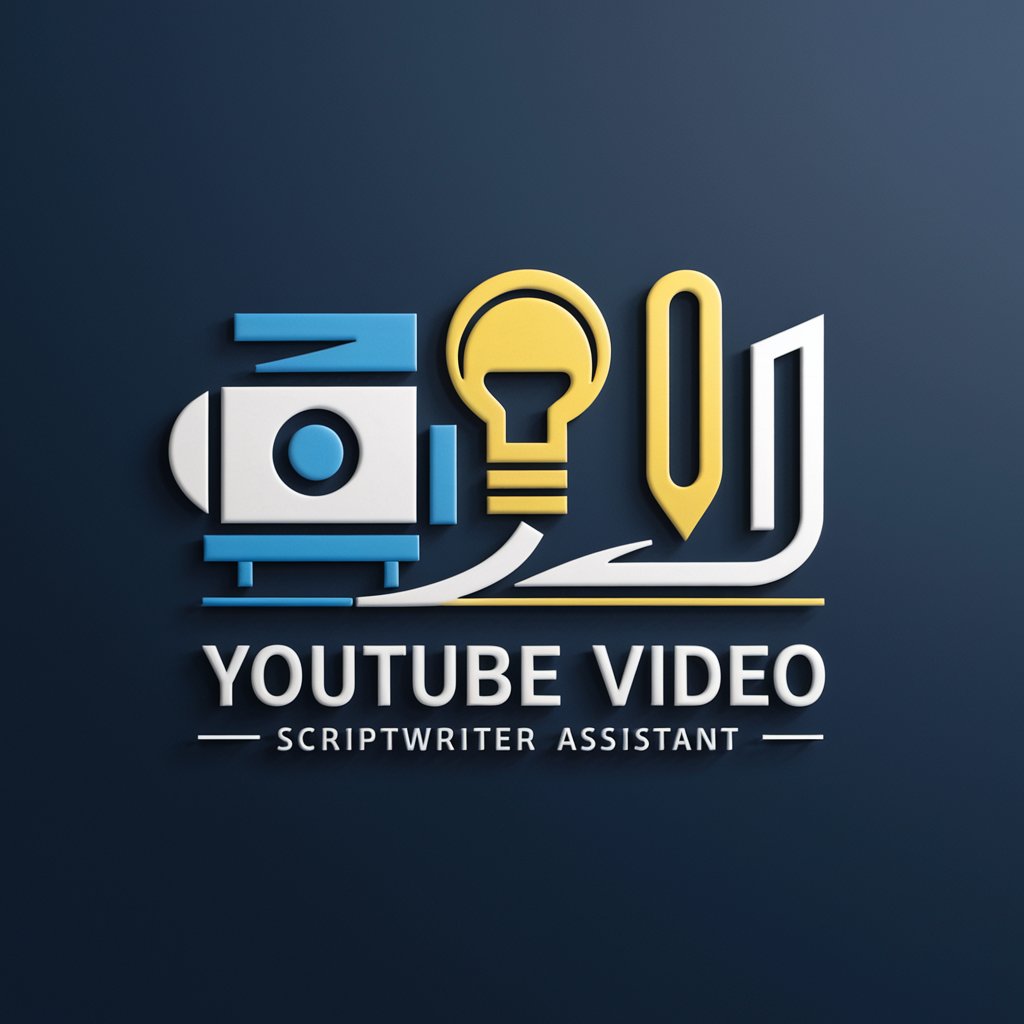
SNS インスパイア
AI-powered content generator for everyone
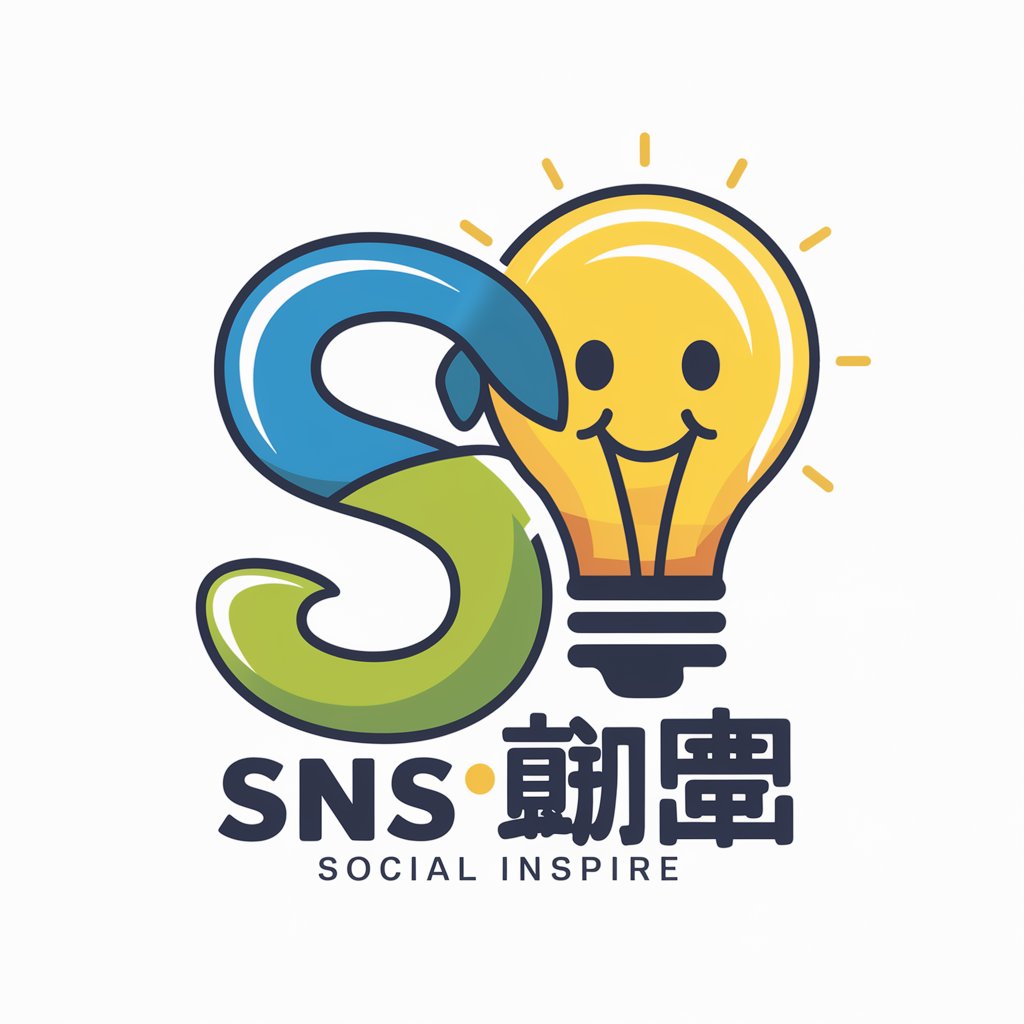
Twitter Analyzer
Uncover market trends powered by AI
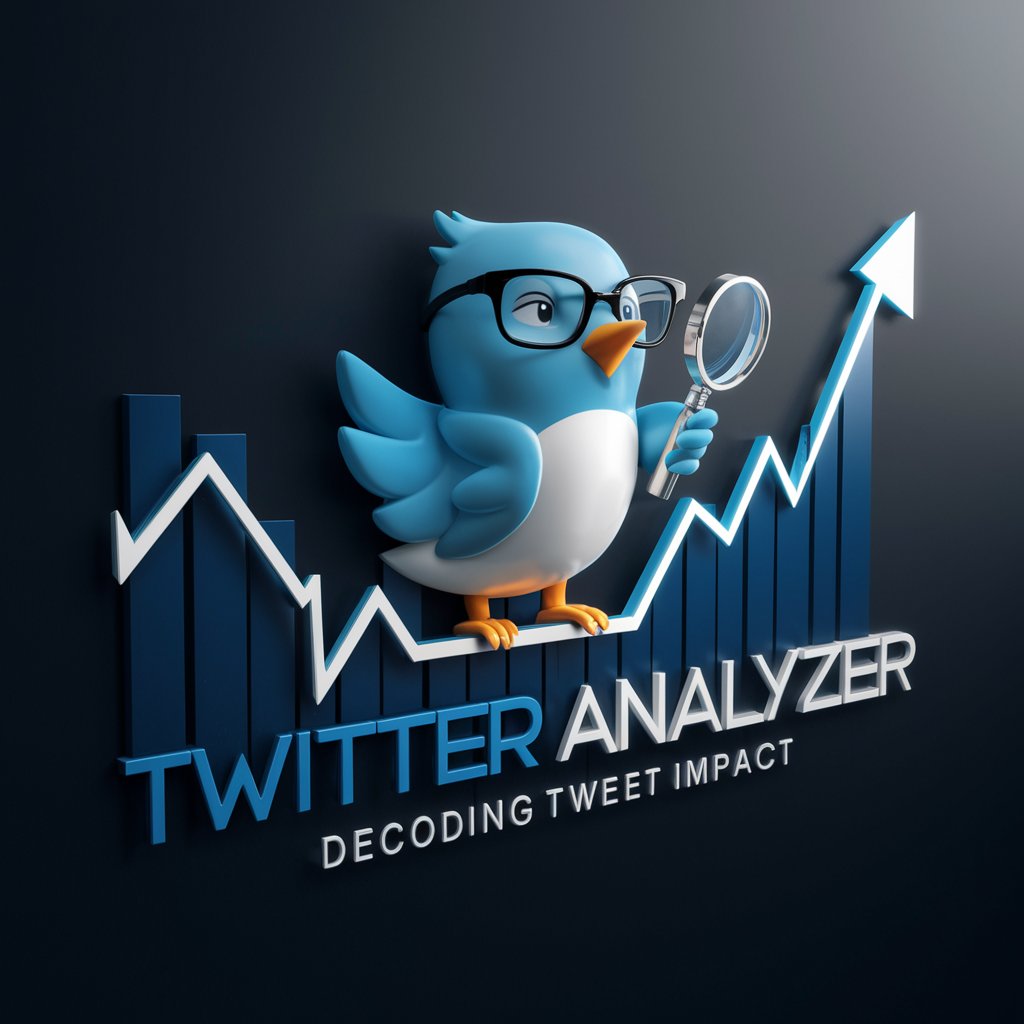
Český jazykový korektor
AI-powered Czech language correction tool
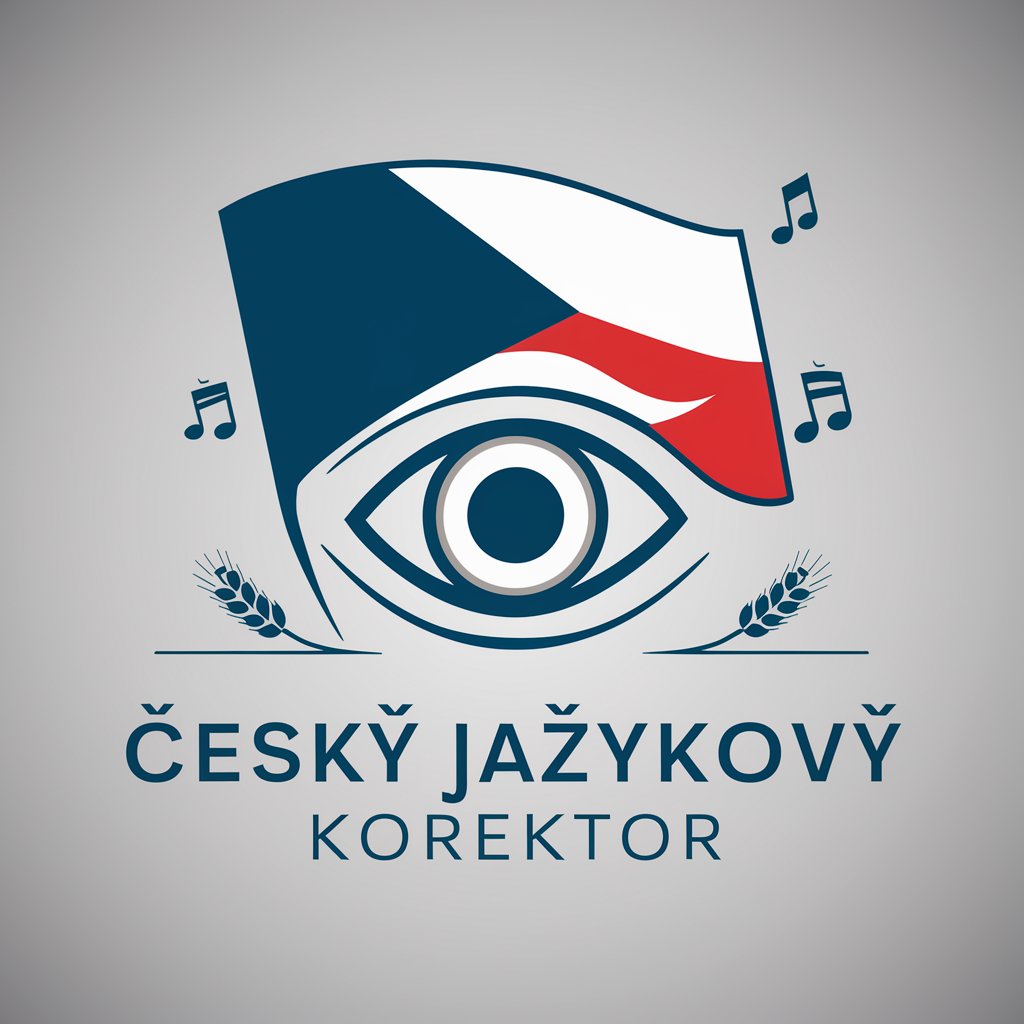
Criador de Vídeos
Empower Your Storytelling with AI
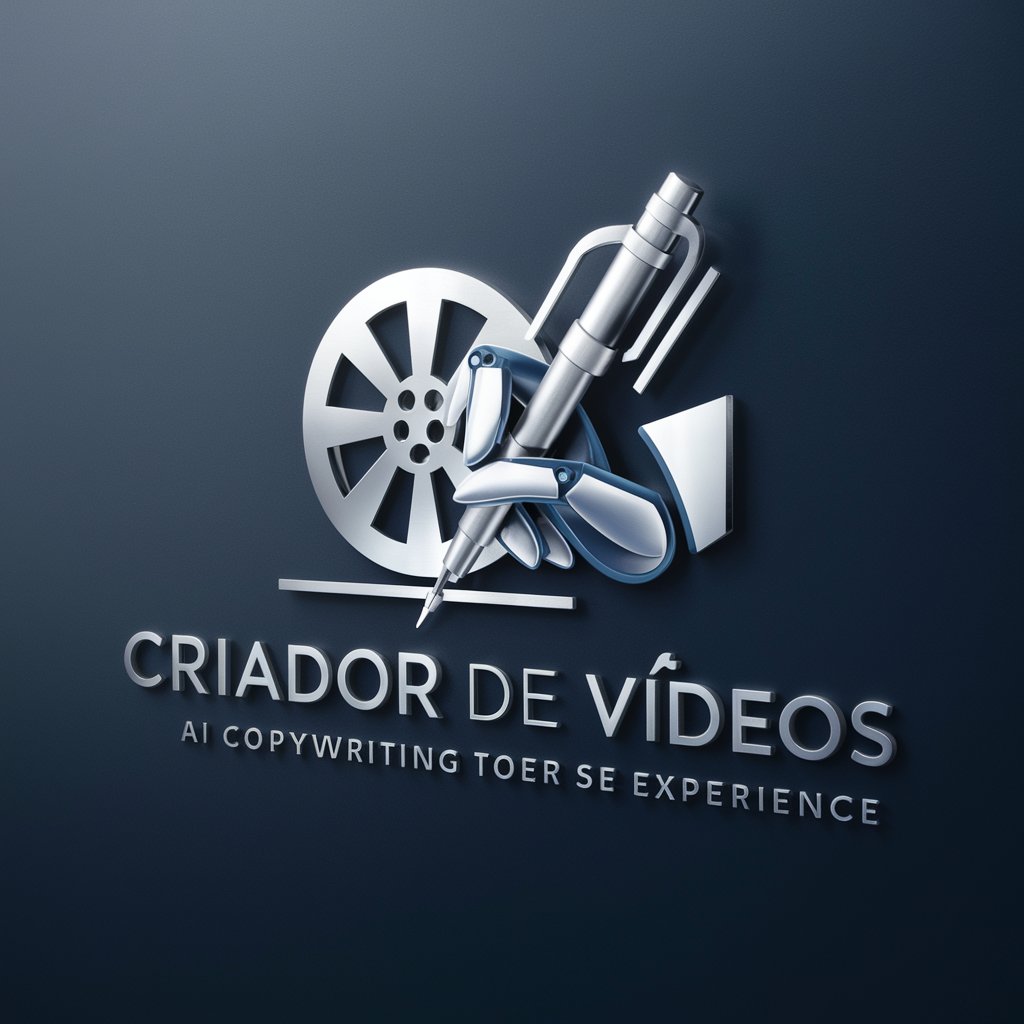
FinCHAT
Revolutionize communication with AI-powered insights.
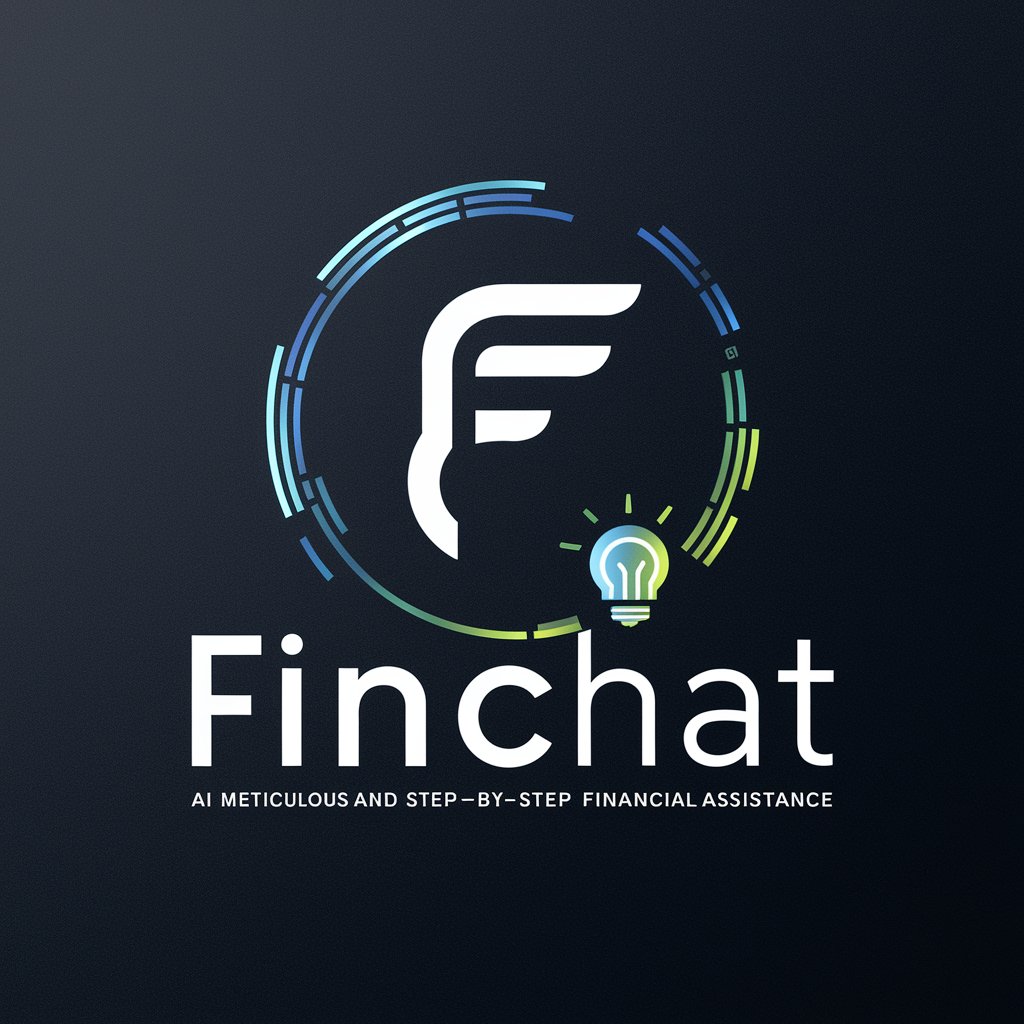
Generador de Examenes
Streamline Exam Creation with AI
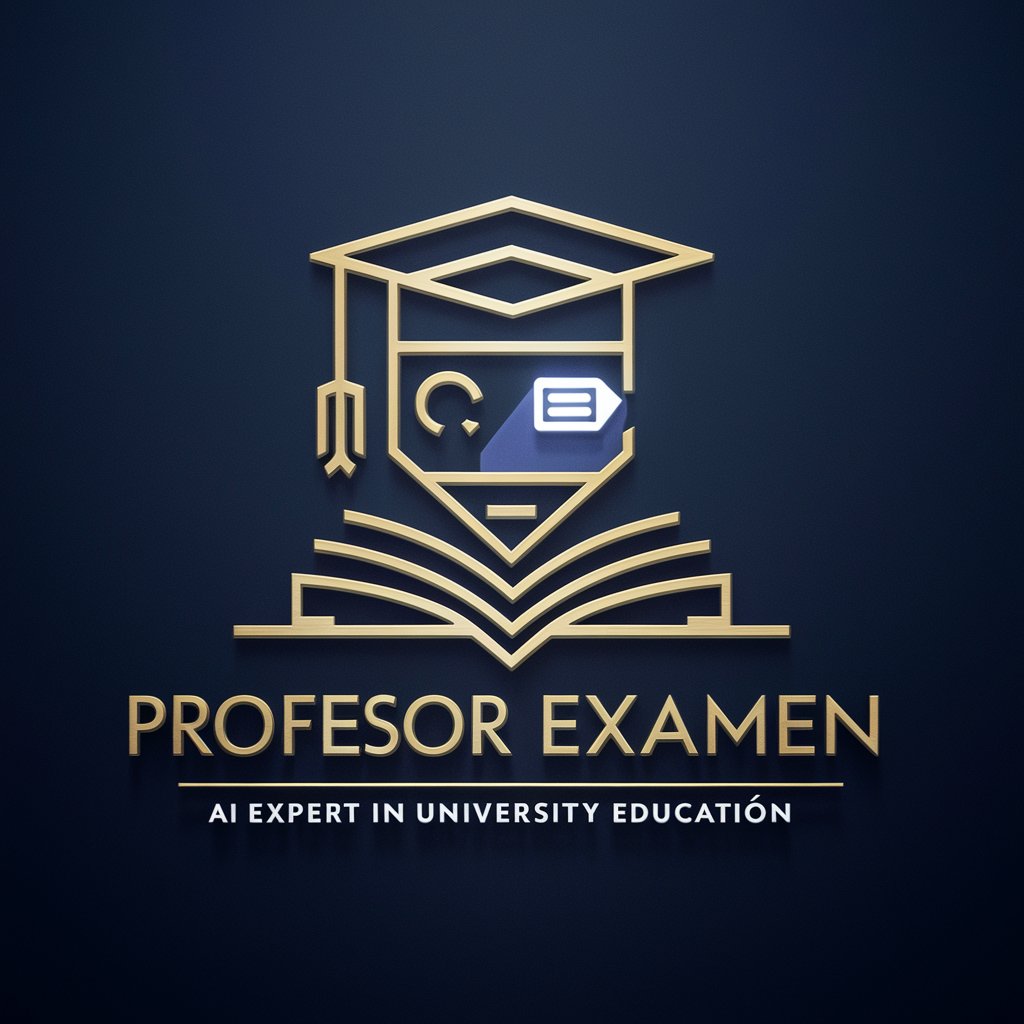
ChatCPT-5
Revolutionizing Information Discovery with AI
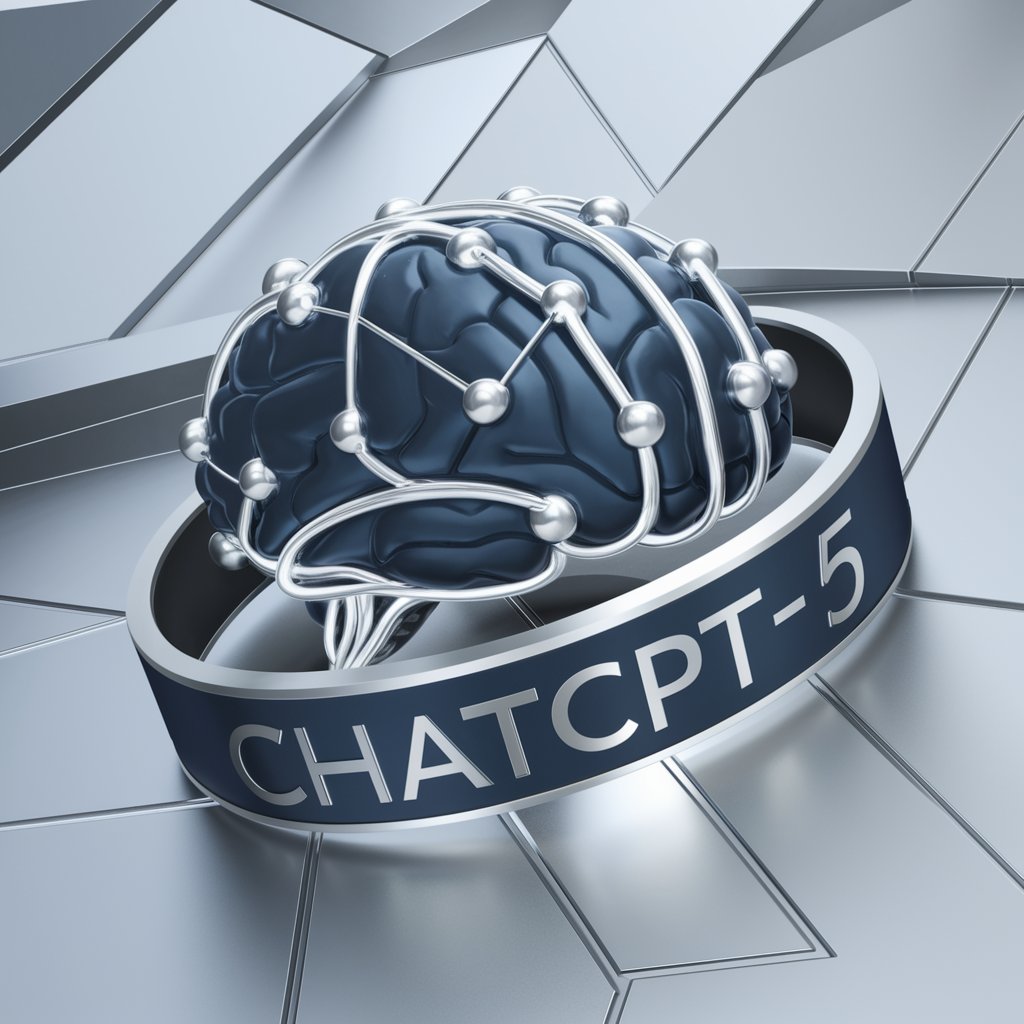
Germany
Powering Communication with AI
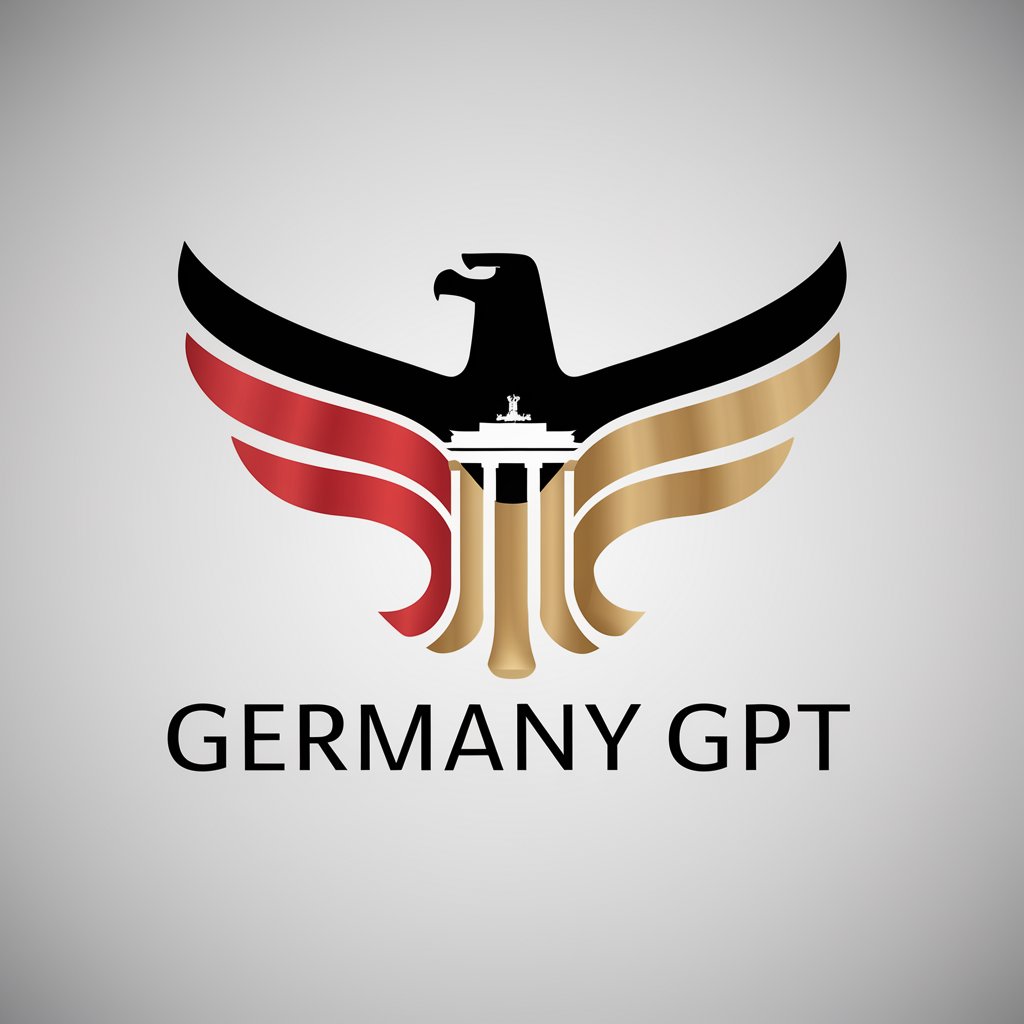
Frequently Asked Questions about Lit-HTML
What is lit-HTML?
Lit-HTML is a simple, modern library used to write HTML templates via JavaScript template literals. It's part of the Lit library which helps in building web components.
How does lit-HTML improve performance?
Lit-HTML efficiently updates HTML by changing only the parts of the DOM that have changed, rather than re-rendering entire templates. This partial update technique enhances performance, especially in dynamic applications.
Can lit-HTML be used with other libraries?
Yes, lit-HTML is highly interoperable and can be seamlessly integrated with other libraries and frameworks such as React, Vue, or Angular to enhance their component rendering capabilities.
What are custom directives in lit-HTML?
Custom directives in lit-HTML allow developers to create reusable, encapsulated logic to extend the functionality of lit-HTML templates, such as adding asynchronous handling or managing complex state changes.
Is lit-HTML suitable for large-scale applications?
Absolutely, lit-HTML's minimalistic approach combined with the powerful Web Components standard makes it an excellent choice for developing scalable and maintainable large-scale applications.