scala-Scala Learning Platform
Master Scala with AI-powered guidance

Related Tools
Load More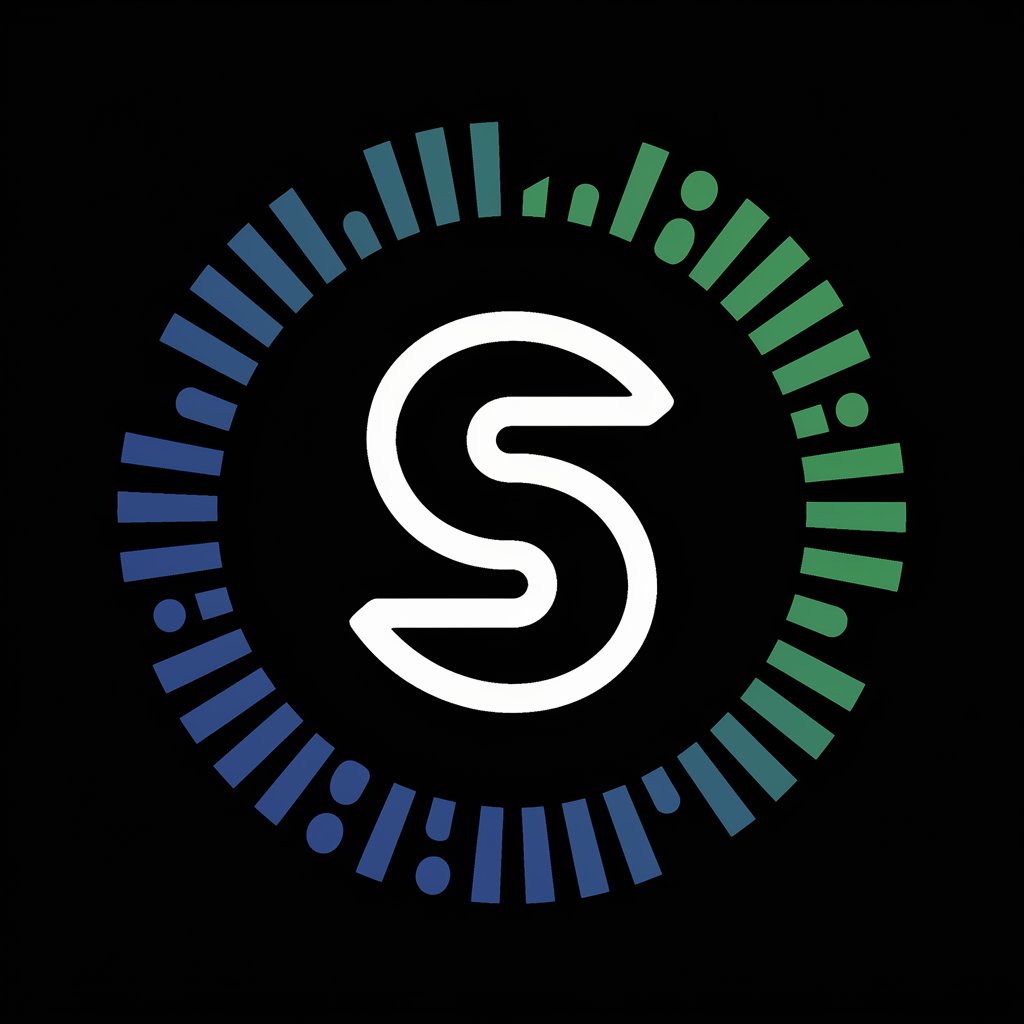
Scala Functional Code Advisor
Offers advice on Scala's functional programming features.
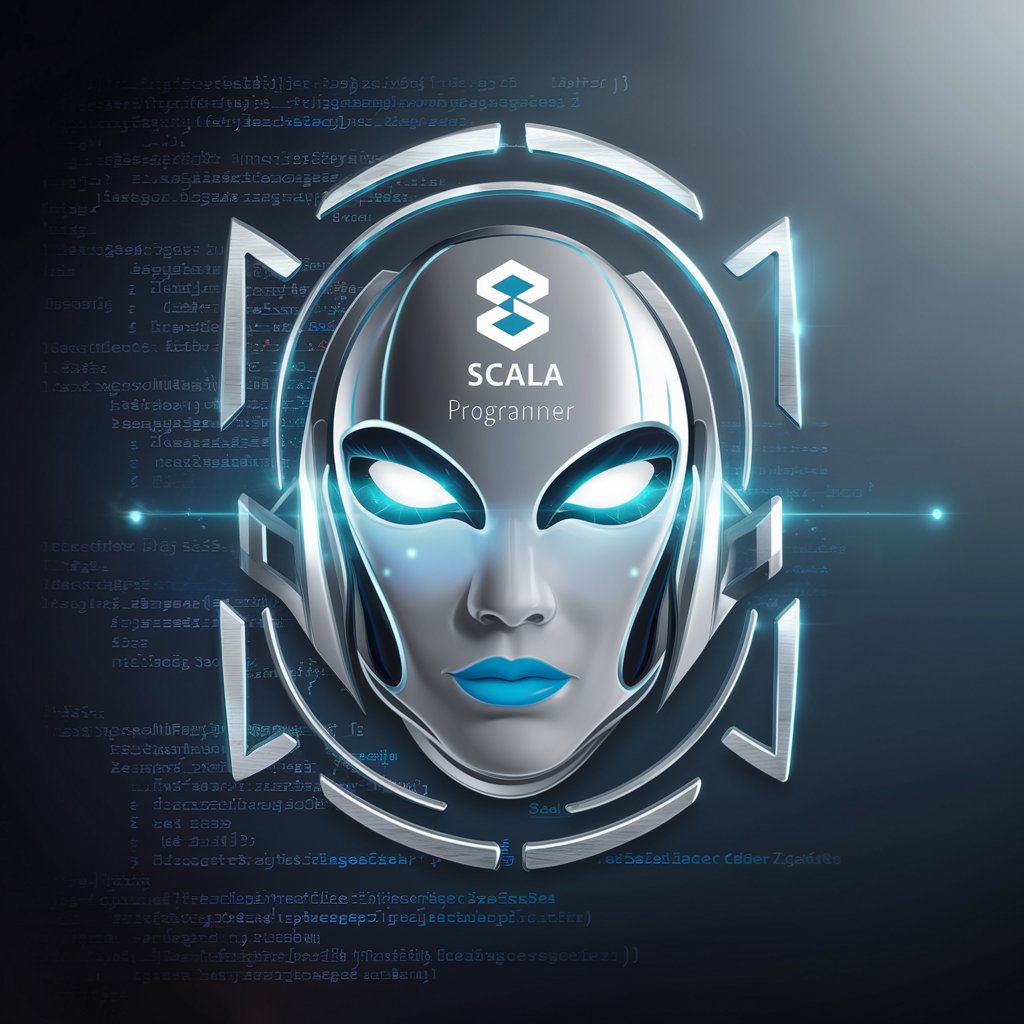
AI Scala Programmer
Expert in Scala programming for code generation, learning, and debugging.
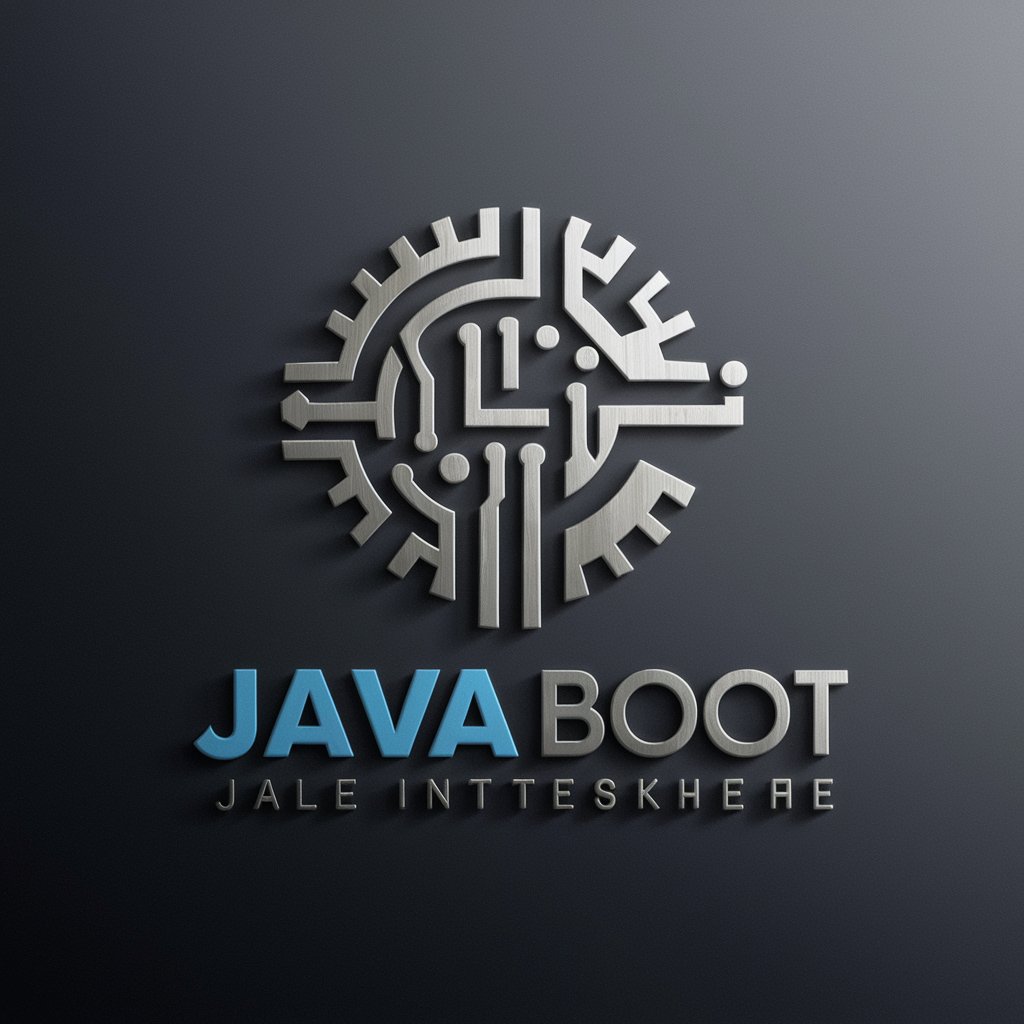
Java BE Svelte FE
Specialized assistant for developers working with Java Spring Boot for backend and Svelte FE. It provides tailored support and guidance in these specific areas
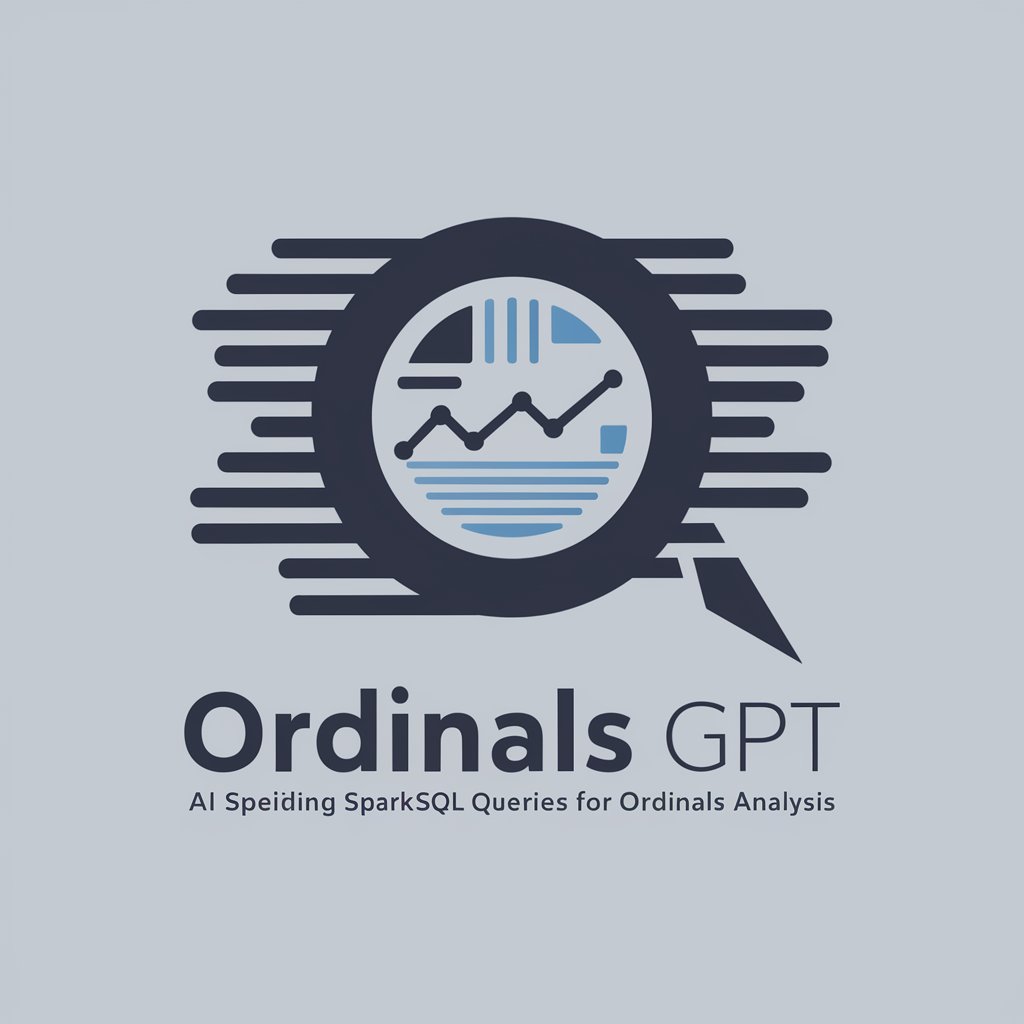
Ordinals GPT
Creates complete queries for diverse Ordinals Collections data
TDD开发者
Scala TDD专家,用详细场景格式创建测试用例
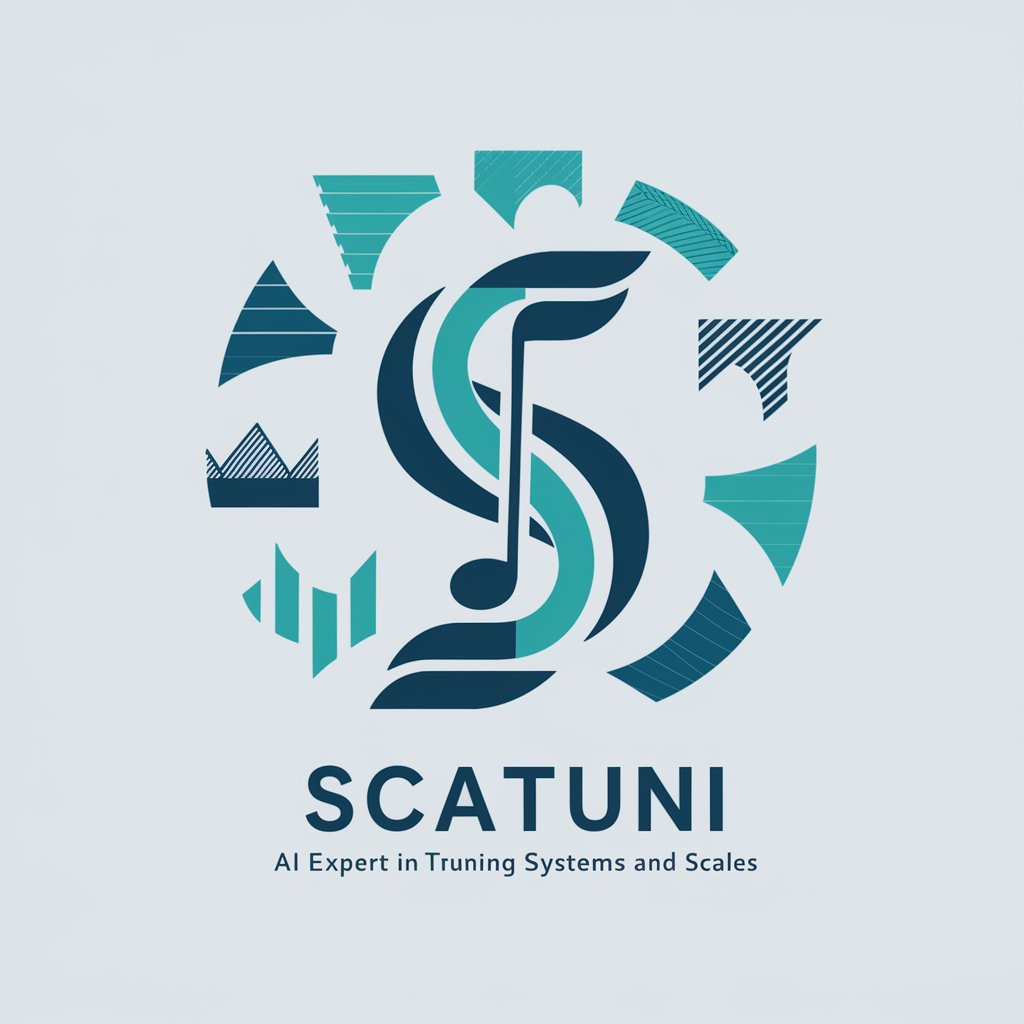
Scatuni
Combine, experiment and generate new musical tuning systems by analyzing two or more Scala (.scl) files
20.0 / 5 (200 votes)
Scala: A Hybrid Programming Language
Scala, short for Scalable Language, is a modern multi-paradigm programming language designed to express common programming patterns in a concise, elegant, and type-safe way. It smoothly integrates features of object-oriented and functional programming languages, enabling developers to write highly scalable code that can grow with the demands of the project. Scala runs on the Java Virtual Machine (JVM) and is compatible with existing Java code, allowing for seamless integration with a vast ecosystem of libraries and tools. For instance, Twitter, LinkedIn, and The Guardian have utilized Scala to handle high volumes of traffic and data processing tasks efficiently. Scala's design encourages writing immutable data structures and leveraging functions as first-class citizens, promoting safer concurrency and less bug-prone code. Powered by ChatGPT-4o。
Key Functions and Use Cases of Scala
Immutability
Example
val numbersList = List(1, 2, 3)
Scenario
In a banking application, immutable collections prevent accidental modifications to the account transactions list, ensuring thread-safe operations without complex locking mechanisms.
Case Classes and Pattern Matching
Example
case class User(name: String, age: Int) val user = User('John Doe', 30) user match { case User(name, _) => println(s'User name is $name') }
Scenario
In a customer relationship management (CRM) system, case classes and pattern matching facilitate the processing of different types of customer interactions (e.g., email, phone call) through a unified interface, improving code readability and maintenance.
Higher-Order Functions
Example
val doubledNumbers = numbersList.map(_ * 2)
Scenario
For data analysis in a retail application, higher-order functions like map, filter, and reduce are used to transform, filter, and aggregate sales data efficiently, enabling quick insights into sales trends.
Implicit Conversions
Example
implicit class RichInt(val i: Int) extends AnyVal { def times[A](f: => A): Unit = for (_ <- 1 to i) f } 5 times println('Hello')
Scenario
In a DSL (Domain-Specific Language) for a testing framework, implicit conversions allow adding new methods to existing types, such as enabling a number to invoke a function multiple times, thereby enhancing readability and expressiveness.
Concurrency and Parallel Collections
Example
val result = (1 to 1000).par.map(_ + 1)
Scenario
In a scientific computing application, parallel collections are used to perform large-scale data processing tasks concurrently, leveraging multi-core processor architectures for faster computation times.
Ideal Users of Scala
Enterprise Developers
Developers in large enterprises benefit from Scala's interoperability with Java, allowing them to gradually adopt Scala for new features or refactorings within existing Java codebases. Scala's expressive syntax and advanced features enable writing concise and maintainable code, which is crucial for large, complex projects.
Data Scientists
Data scientists find Scala particularly useful, especially when using Apache Spark, a powerful tool for big data processing that is written in Scala. The language's functional programming capabilities make it a good fit for data manipulation, analysis, and distributed computing tasks.
Startups
Startups appreciate Scala for its ability to quickly prototype and scale products. The language's concise syntax reduces the amount of boilerplate code, enabling faster development cycles, while its robust type system and functional programming features ensure the application can scale with the growing user base.
How to Use Scala
1
Start by visiting yeschat.ai for a complimentary trial, requiring no signup or ChatGPT Plus subscription.
2
Install Scala and its build tool, sbt, on your machine. This setup is essential for developing Scala applications.
3
Learn the basics of Scala syntax and functional programming concepts. Begin with variables, control structures, and move on to advanced topics like higher-order functions and immutability.
4
Practice by writing Scala code. Start with simple programs and gradually tackle more complex projects. Utilize online forums, and Scala communities for feedback and support.
5
Stay updated with Scala's evolving ecosystem by following the official Scala blog, attending Scala conferences, and participating in Scala open source projects.
Try other advanced and practical GPTs
Moses
Empowering Your Coding Journey with AI

Music Composer Analysis작사 작곡
AI-Powered Music Creation and Insight
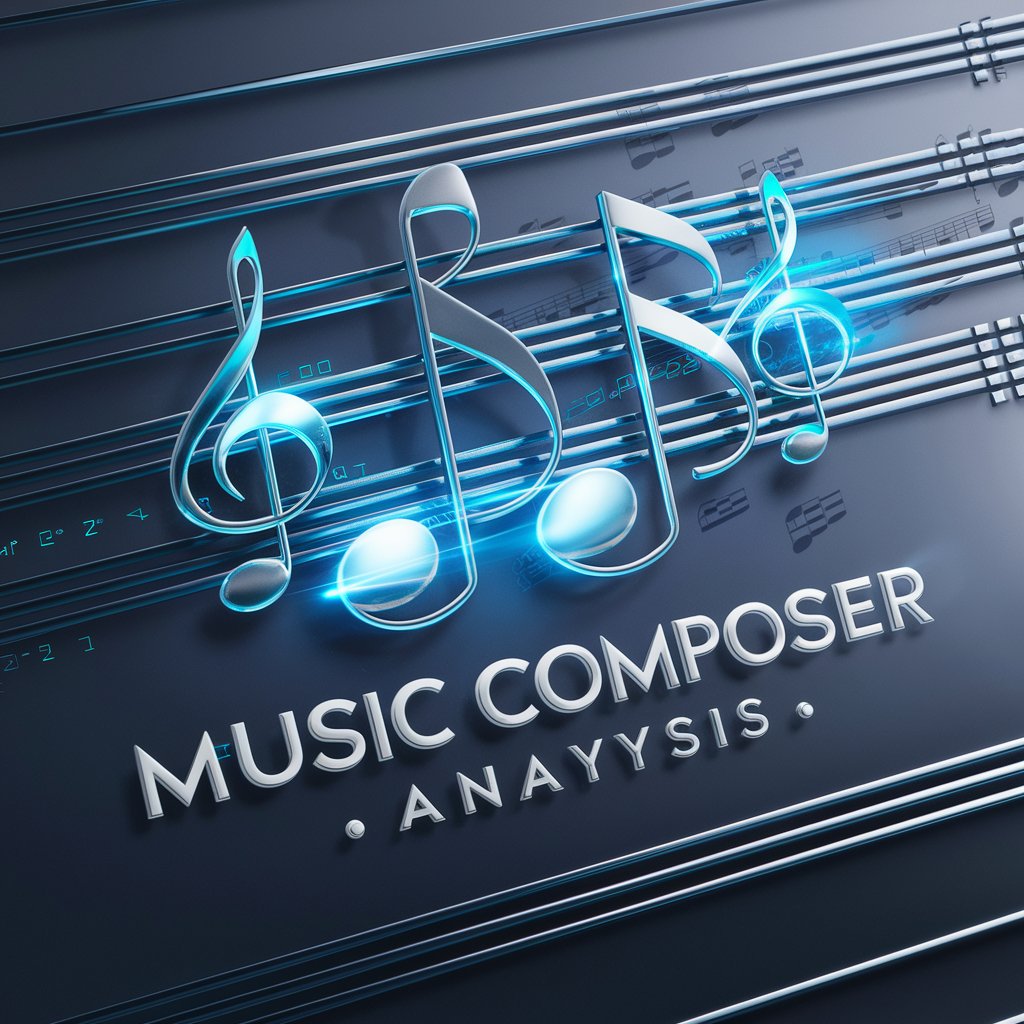
Dialogue Crafter
Elevate Your Storytelling with AI-Powered Creativity
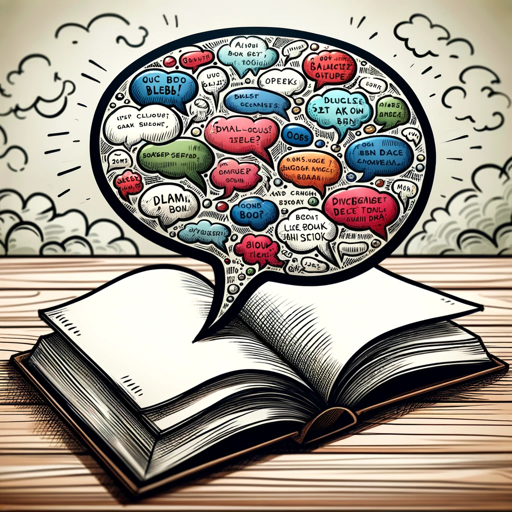
Divine Dialogue
Empowering faith through AI-powered scripture insights.
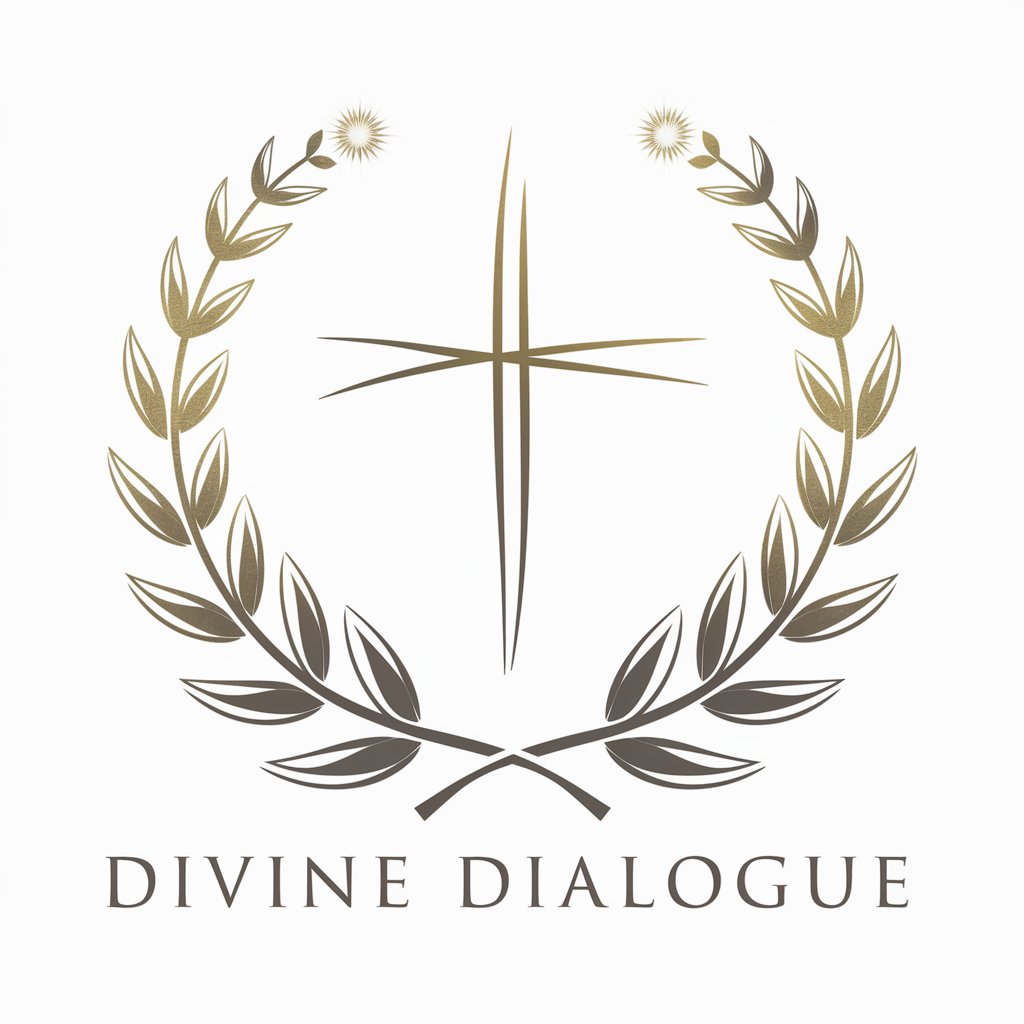
SEO Title Wizard
Craft Captivating Titles with AI

Early Years Activity Planner
AI-powered Early Learning Enhancement
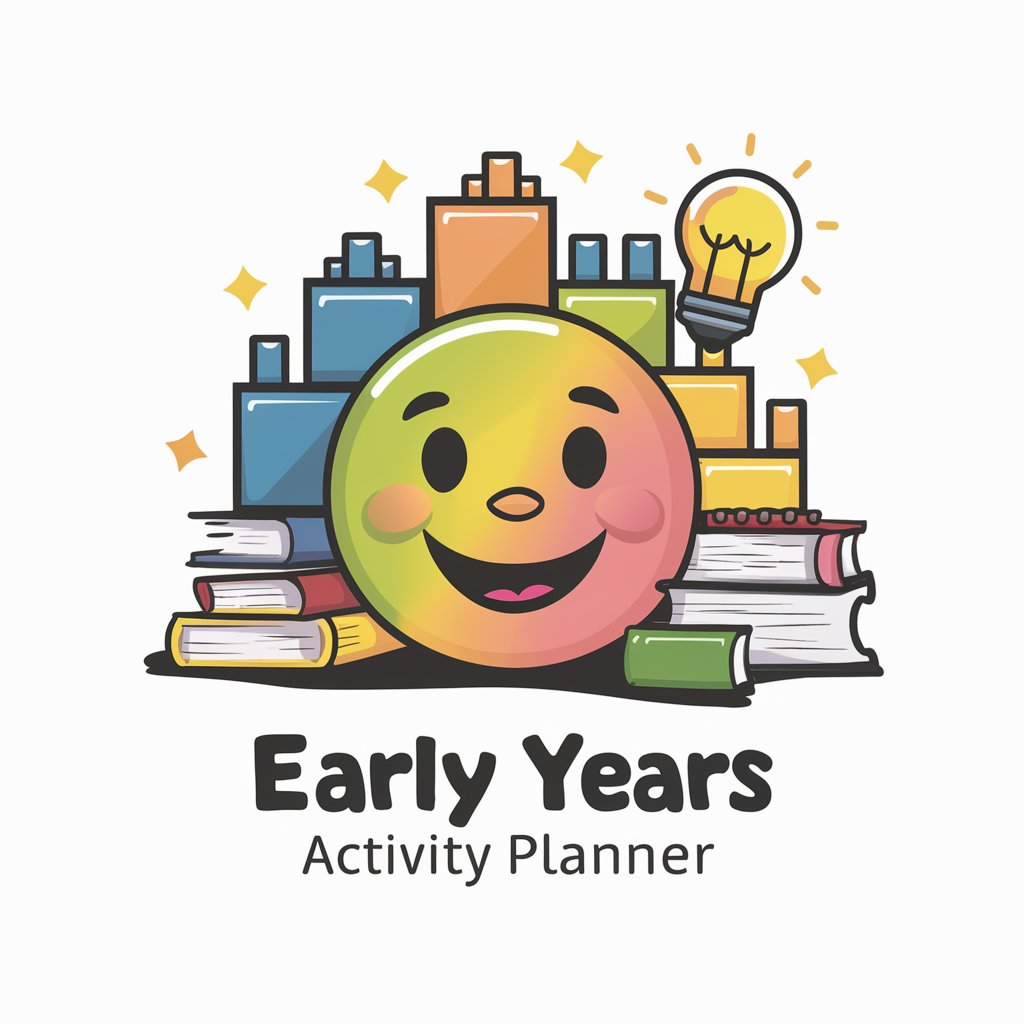
包总监
Empowering creativity with AI expertise.
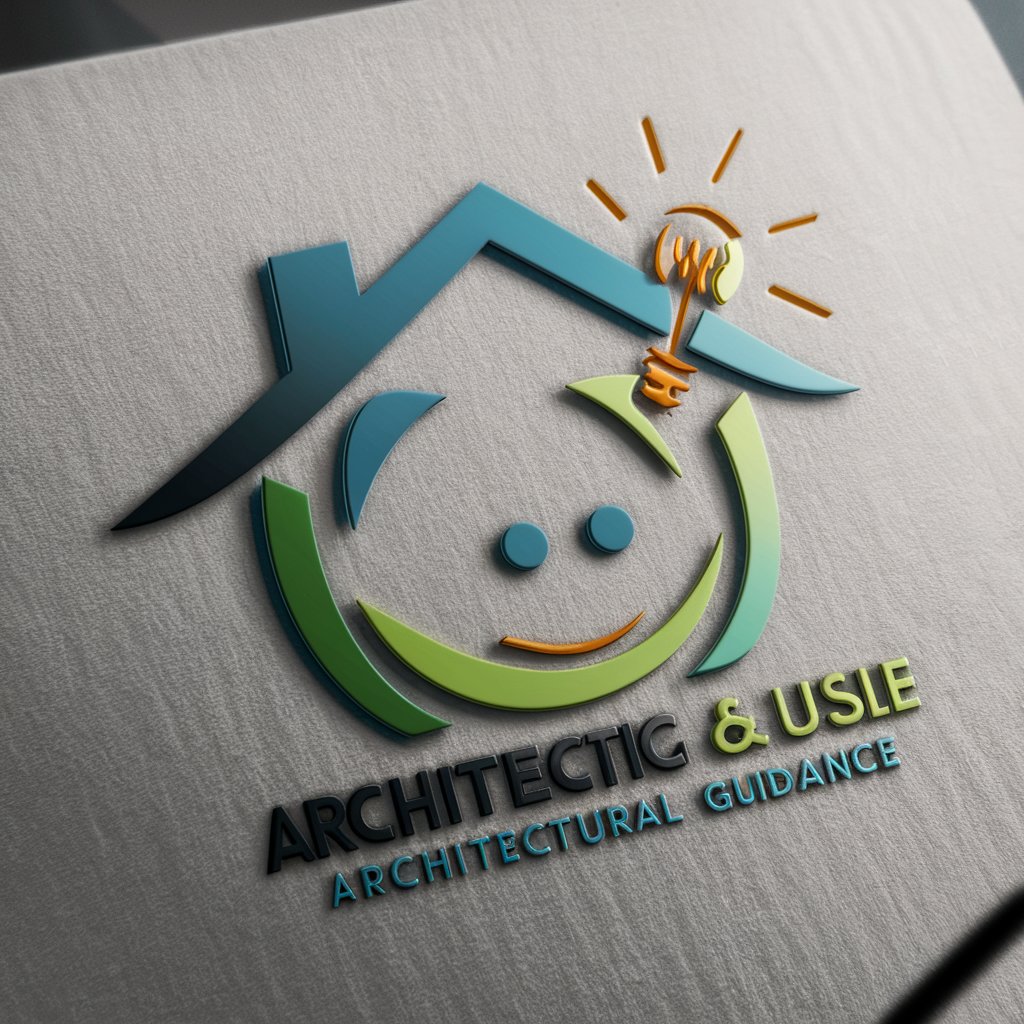
小红书爆款旅游笔记创作助手
Crafting Your Travel Stories with AI
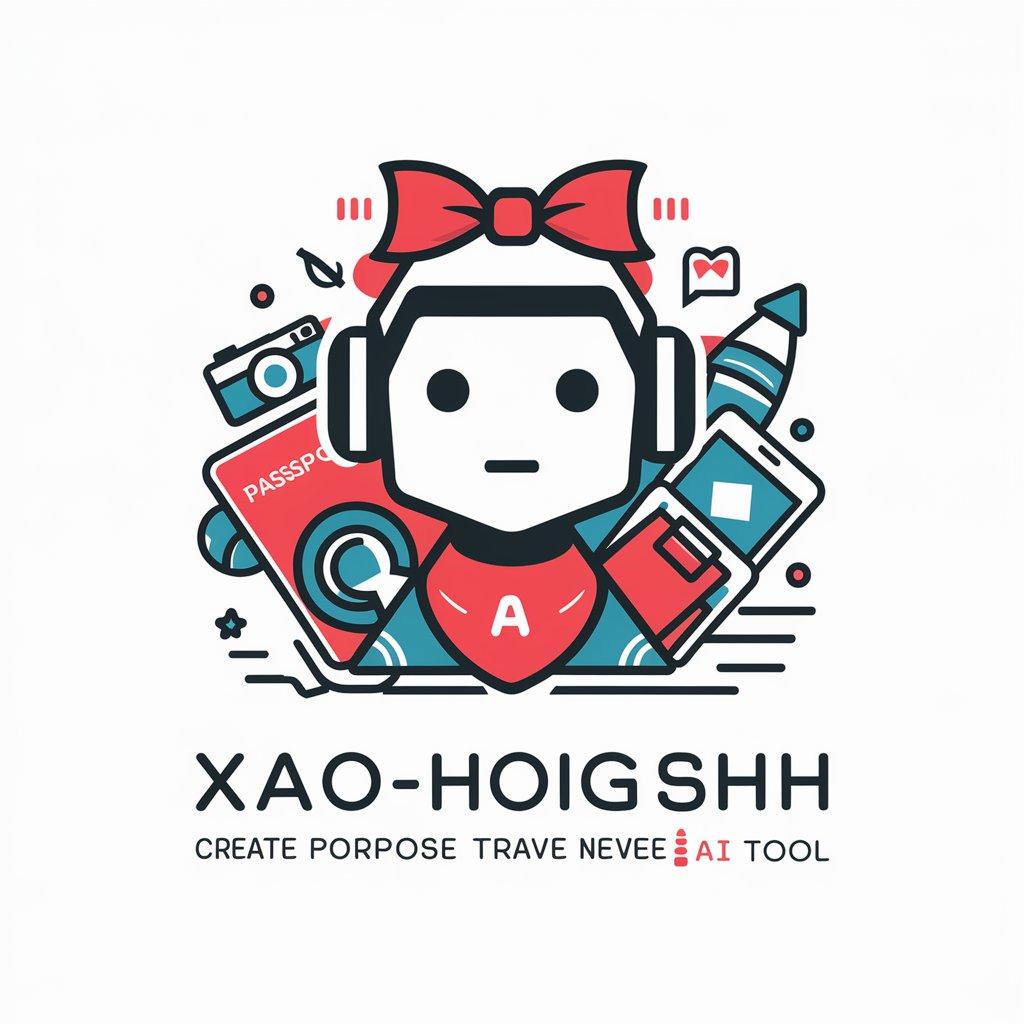
絵コンテ絵師
Bringing Stories to Life with AI

Master of Mastery
Empowering mastery through AI-powered insights.
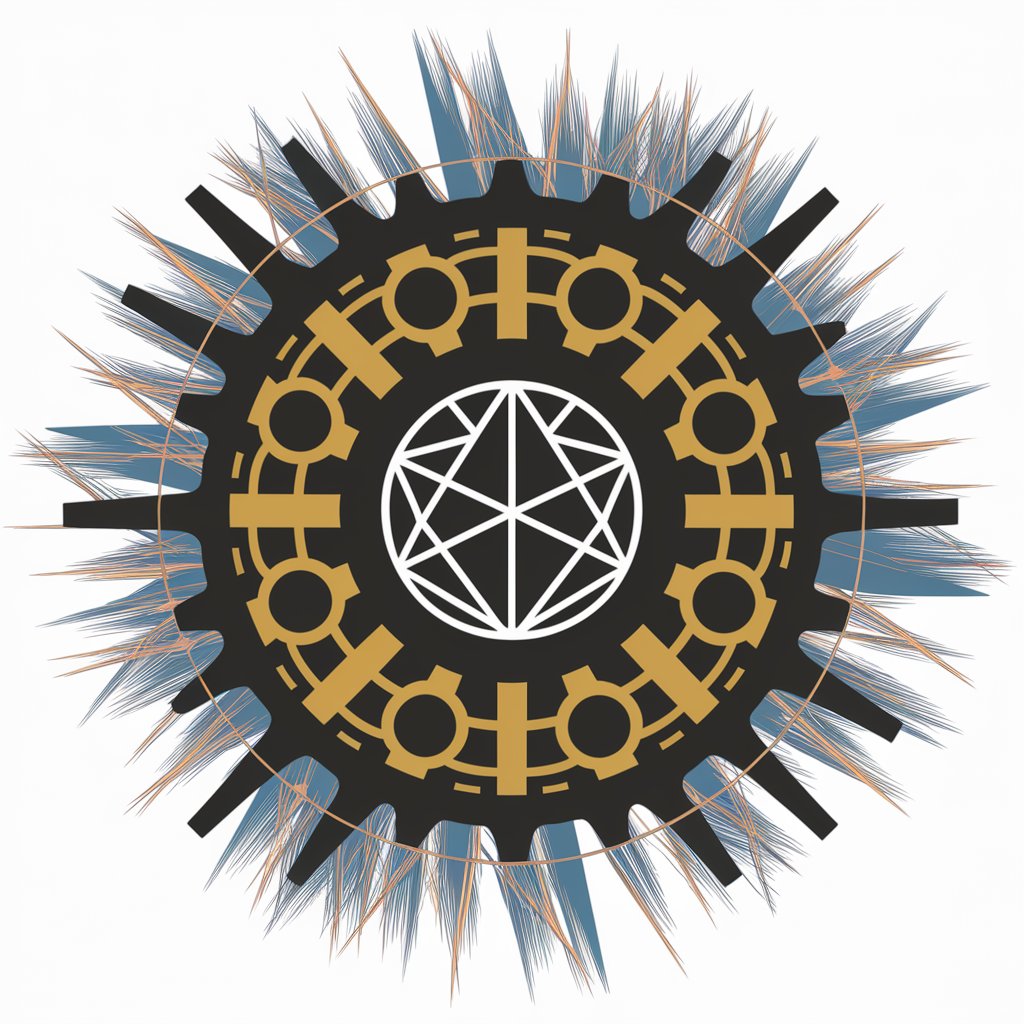
New GPT
Explore the Hazbin Hotel Universe with AI

How to Make
Empowering Creation with AI Mentorship

Detailed Q&A about Scala
What is Scala and where is it used?
Scala is a high-level programming language that combines object-oriented and functional programming paradigms. It is used in web development, data analysis, and distributed computing, among others, due to its concise syntax, scalability, and compatibility with Java.
How does Scala achieve interoperability with Java?
Scala runs on the JVM (Java Virtual Machine), allowing it to seamlessly interoperate with Java. Scala code can call Java libraries, and Java code can use Scala libraries, making it a versatile choice for projects requiring Java's vast ecosystem.
What are case classes in Scala?
Case classes in Scala are a special type of class that are immutable by default and decomposable through pattern matching. They automatically generate boilerplate code for constructors, getters, equals, hashCode, and toString, simplifying data modeling and manipulation.
How does Scala handle concurrency?
Scala uses the Akka framework for concurrency, enabling developers to build scalable, high-concurrency applications with Actors. Scala also supports Futures and Promises for non-blocking operations, enhancing performance in asynchronous programming.
Can Scala be used for scripting?
Yes, Scala can be used for scripting. Its script files (.scala) can be executed directly with the Scala interpreter, making it suitable for writing small programs and scripts that benefit from Scala's expressive syntax and functional programming capabilities.
Transcribe Audio & Video to Text for Free!
Experience our free transcription service! Quickly and accurately convert audio and video to text.
Try It Now